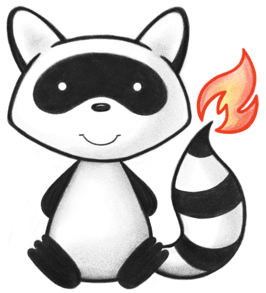
Package ca.uhn.fhir.jpa.search.cache
Interface ISearchCacheSvc
- All Known Implementing Classes:
DatabaseSearchCacheSvcImpl
public interface ISearchCacheSvc
-
Method Summary
Modifier and TypeMethodDescriptionfetchByUuid
(String theUuid, RequestPartitionId theRequestPartitionId) Fetch a search using its UUID.findCandidatesForReuse
(String theResourceType, String theQueryString, Instant theCreatedAfter, RequestPartitionId theRequestPartitionId) Look for any existing searches matching the given resource type and query string.void
pollForStaleSearchesAndDeleteThem
(RequestPartitionId theRequestPartitionId, Instant theDeadline) This method will be called periodically to delete stale searches.save
(Search theSearch, RequestPartitionId theRequestPartitionId) Places a new search of some sort in the cache, or updates an existing search.tryToMarkSearchAsInProgress
(Search theSearch, RequestPartitionId theRequestPartitionId) TODO: this is perhaps an inappropriate responsibility for this service
-
Method Details
-
save
Places a new search of some sort in the cache, or updates an existing search. The search passed in is guaranteed to have aUUID
so that is a good candidate for consistent identification.- Parameters:
theSearch
- The search to store- Returns:
- Returns a copy of the search as it was saved. Callers should use the returned Search object for any further processing.
-
fetchByUuid
Fetch a search using its UUID. The search should be fully loaded when it is returned (i.e. includes are fetched, so that access to its fields will not cause database errors if the current tranaction scope ends.- Parameters:
theUuid
- The search UUID- Returns:
- The search if it exists
-
tryToMarkSearchAsInProgress
Optional<Search> tryToMarkSearchAsInProgress(Search theSearch, RequestPartitionId theRequestPartitionId) TODO: this is perhaps an inappropriate responsibility for this serviceThis method marks a search as in progress, but should only allow exactly one call to do so across the cluster. This is done so that if two client threads request the next page at the exact same time (which is unlikely but not impossible) only one will actually proceed to load the next results and the other will just wait for them to arrive.
- Parameters:
theSearch
- The search to mark- Returns:
- This method should return an empty optional if the search was not marked (meaning that another thread
succeeded in marking it). If the search doesn't exist or some other error occurred, an exception will be thrown
instead of
Optional.empty()
-
findCandidatesForReuse
Optional<Search> findCandidatesForReuse(String theResourceType, String theQueryString, Instant theCreatedAfter, RequestPartitionId theRequestPartitionId) Look for any existing searches matching the given resource type and query string.This method is allowed to perform a "best effort" return, so it can return searches that don't match the query string exactly, or which have a created timestamp before
theCreatedAfter
date. The caller is responsible for removing any inappropriate Searches and picking the most relevant one.- Parameters:
theResourceType
- The resource type of the search. Results MUST match this typetheQueryString
- The query string. Results SHOULD match this typetheCreatedAfter
- Results SHOULD not include any searches created before this cutoff timestamptheRequestPartitionId
- Search should examine only the requested partitions. Cache MUST not return results matching the given partition IDs- Returns:
- A collection of candidate searches
-
pollForStaleSearchesAndDeleteThem
void pollForStaleSearchesAndDeleteThem(RequestPartitionId theRequestPartitionId, Instant theDeadline) This method will be called periodically to delete stale searches. Implementations are not required to do anything if they have some other mechanism for expiring stale results other than manually looking for them and deleting them.
-