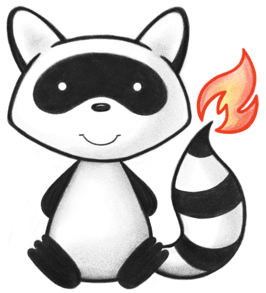
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.config; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 024import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 025import ca.uhn.fhir.jpa.api.svc.IBatch2DaoSvc; 026import ca.uhn.fhir.jpa.api.svc.IDeleteExpungeSvc; 027import ca.uhn.fhir.jpa.api.svc.IIdHelperService; 028import ca.uhn.fhir.jpa.batch2.Batch2DaoSvcImpl; 029import ca.uhn.fhir.jpa.dao.IFulltextSearchSvc; 030import ca.uhn.fhir.jpa.dao.data.IResourceLinkDao; 031import ca.uhn.fhir.jpa.dao.data.IResourceTableDao; 032import ca.uhn.fhir.jpa.dao.expunge.ResourceTableFKProvider; 033import ca.uhn.fhir.jpa.dao.tx.IHapiTransactionService; 034import ca.uhn.fhir.jpa.delete.batch2.DeleteExpungeSqlBuilder; 035import ca.uhn.fhir.jpa.delete.batch2.DeleteExpungeSvcImpl; 036import ca.uhn.fhir.jpa.model.config.PartitionSettings; 037import ca.uhn.fhir.jpa.searchparam.MatchUrlService; 038import jakarta.persistence.EntityManager; 039import org.springframework.beans.factory.annotation.Autowired; 040import org.springframework.context.annotation.Bean; 041 042public class Batch2SupportConfig { 043 044 @Bean 045 public IBatch2DaoSvc batch2DaoSvc( 046 IResourceTableDao theResourceTableDao, 047 IResourceLinkDao theResourceLinkDao, 048 MatchUrlService theMatchUrlService, 049 DaoRegistry theDaoRegistry, 050 FhirContext theFhirContext, 051 IHapiTransactionService theTransactionService) { 052 return new Batch2DaoSvcImpl( 053 theResourceTableDao, 054 theResourceLinkDao, 055 theMatchUrlService, 056 theDaoRegistry, 057 theFhirContext, 058 theTransactionService); 059 } 060 061 @Bean 062 public IDeleteExpungeSvc deleteExpungeSvc( 063 EntityManager theEntityManager, 064 DeleteExpungeSqlBuilder theDeleteExpungeSqlBuilder, 065 @Autowired(required = false) IFulltextSearchSvc theFullTextSearchSvc) { 066 return new DeleteExpungeSvcImpl(theEntityManager, theDeleteExpungeSqlBuilder, theFullTextSearchSvc); 067 } 068 069 @Bean 070 DeleteExpungeSqlBuilder deleteExpungeSqlBuilder( 071 ResourceTableFKProvider theResourceTableFKProvider, 072 JpaStorageSettings theStorageSettings, 073 IIdHelperService theIdHelper, 074 IResourceLinkDao theResourceLinkDao, 075 PartitionSettings thePartitionSettings) { 076 return new DeleteExpungeSqlBuilder( 077 theResourceTableFKProvider, theStorageSettings, theIdHelper, theResourceLinkDao, thePartitionSettings); 078 } 079}