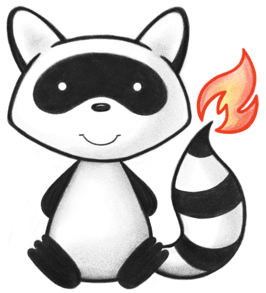
001package ca.uhn.fhir.jpa.config; 002 003import java.util.ArrayList; 004import java.util.List; 005import java.util.concurrent.Executor; 006import java.util.concurrent.Executors; 007 008import org.springframework.transaction.PlatformTransactionManager; 009import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 010 011import org.springframework.beans.factory.annotation.Autowired; 012import org.springframework.context.annotation.Bean; 013import org.springframework.context.annotation.Configuration; 014import org.springframework.context.annotation.Lazy; 015import org.springframework.data.jpa.repository.config.EnableJpaRepositories; 016import org.springframework.scheduling.annotation.EnableScheduling; 017import org.springframework.scheduling.annotation.SchedulingConfigurer; 018import org.springframework.scheduling.config.ScheduledTaskRegistrar; 019 020import ca.uhn.fhir.rest.api.IResourceSupportedSvc; 021import ca.uhn.fhir.context.FhirContext; 022import ca.uhn.fhir.model.api.IResource; 023import ca.uhn.fhir.rest.server.IResourceProvider; 024import ca.uhn.fhir.jpa.api.dao.*; 025import ca.uhn.fhir.jpa.dao.*; 026import ca.uhn.fhir.rest.server.provider.ResourceProviderFactory; 027 028@Configuration 029public class GeneratedDaoAndResourceProviderConfigDstu2 { 030@Autowired 031FhirContext myFhirContext; 032 033 @Bean(name="myResourceProvidersDstu2") 034 public ResourceProviderFactory resourceProvidersDstu2(IResourceSupportedSvc theResourceSupportedSvc) { 035 ResourceProviderFactory retVal = new ResourceProviderFactory(); 036 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Account") ? rpAccountDstu2() : null); 037 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AllergyIntolerance") ? rpAllergyIntoleranceDstu2() : null); 038 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Appointment") ? rpAppointmentDstu2() : null); 039 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AppointmentResponse") ? rpAppointmentResponseDstu2() : null); 040 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AuditEvent") ? rpAuditEventDstu2() : null); 041 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Basic") ? rpBasicDstu2() : null); 042 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Binary") ? rpBinaryDstu2() : null); 043 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BodySite") ? rpBodySiteDstu2() : null); 044 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Bundle") ? rpBundleDstu2() : null); 045 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CarePlan") ? rpCarePlanDstu2() : null); 046 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Claim") ? rpClaimDstu2() : null); 047 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClaimResponse") ? rpClaimResponseDstu2() : null); 048 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClinicalImpression") ? rpClinicalImpressionDstu2() : null); 049 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Communication") ? rpCommunicationDstu2() : null); 050 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CommunicationRequest") ? rpCommunicationRequestDstu2() : null); 051 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Composition") ? rpCompositionDstu2() : null); 052 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ConceptMap") ? rpConceptMapDstu2() : null); 053 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Condition") ? rpConditionDstu2() : null); 054 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Conformance") ? rpConformanceDstu2() : null); 055 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Contract") ? rpContractDstu2() : null); 056 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Coverage") ? rpCoverageDstu2() : null); 057 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DataElement") ? rpDataElementDstu2() : null); 058 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DetectedIssue") ? rpDetectedIssueDstu2() : null); 059 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Device") ? rpDeviceDstu2() : null); 060 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceComponent") ? rpDeviceComponentDstu2() : null); 061 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceMetric") ? rpDeviceMetricDstu2() : null); 062 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceUseRequest") ? rpDeviceUseRequestDstu2() : null); 063 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceUseStatement") ? rpDeviceUseStatementDstu2() : null); 064 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DiagnosticOrder") ? rpDiagnosticOrderDstu2() : null); 065 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DiagnosticReport") ? rpDiagnosticReportDstu2() : null); 066 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentManifest") ? rpDocumentManifestDstu2() : null); 067 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentReference") ? rpDocumentReferenceDstu2() : null); 068 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EligibilityRequest") ? rpEligibilityRequestDstu2() : null); 069 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EligibilityResponse") ? rpEligibilityResponseDstu2() : null); 070 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Encounter") ? rpEncounterDstu2() : null); 071 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentRequest") ? rpEnrollmentRequestDstu2() : null); 072 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentResponse") ? rpEnrollmentResponseDstu2() : null); 073 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EpisodeOfCare") ? rpEpisodeOfCareDstu2() : null); 074 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExplanationOfBenefit") ? rpExplanationOfBenefitDstu2() : null); 075 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("FamilyMemberHistory") ? rpFamilyMemberHistoryDstu2() : null); 076 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Flag") ? rpFlagDstu2() : null); 077 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Goal") ? rpGoalDstu2() : null); 078 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Group") ? rpGroupDstu2() : null); 079 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("HealthcareService") ? rpHealthcareServiceDstu2() : null); 080 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImagingObjectSelection") ? rpImagingObjectSelectionDstu2() : null); 081 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImagingStudy") ? rpImagingStudyDstu2() : null); 082 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Immunization") ? rpImmunizationDstu2() : null); 083 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImmunizationRecommendation") ? rpImmunizationRecommendationDstu2() : null); 084 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImplementationGuide") ? rpImplementationGuideDstu2() : null); 085 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("List") ? rpListResourceDstu2() : null); 086 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Location") ? rpLocationDstu2() : null); 087 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Media") ? rpMediaDstu2() : null); 088 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Medication") ? rpMedicationDstu2() : null); 089 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationAdministration") ? rpMedicationAdministrationDstu2() : null); 090 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationDispense") ? rpMedicationDispenseDstu2() : null); 091 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationOrder") ? rpMedicationOrderDstu2() : null); 092 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationStatement") ? rpMedicationStatementDstu2() : null); 093 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageHeader") ? rpMessageHeaderDstu2() : null); 094 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NamingSystem") ? rpNamingSystemDstu2() : null); 095 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NutritionOrder") ? rpNutritionOrderDstu2() : null); 096 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Observation") ? rpObservationDstu2() : null); 097 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationDefinition") ? rpOperationDefinitionDstu2() : null); 098 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationOutcome") ? rpOperationOutcomeDstu2() : null); 099 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Order") ? rpOrderDstu2() : null); 100 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OrderResponse") ? rpOrderResponseDstu2() : null); 101 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Organization") ? rpOrganizationDstu2() : null); 102 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Parameters") ? rpParametersDstu2() : null); 103 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Patient") ? rpPatientDstu2() : null); 104 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentNotice") ? rpPaymentNoticeDstu2() : null); 105 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentReconciliation") ? rpPaymentReconciliationDstu2() : null); 106 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Person") ? rpPersonDstu2() : null); 107 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Practitioner") ? rpPractitionerDstu2() : null); 108 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Procedure") ? rpProcedureDstu2() : null); 109 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ProcedureRequest") ? rpProcedureRequestDstu2() : null); 110 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ProcessRequest") ? rpProcessRequestDstu2() : null); 111 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ProcessResponse") ? rpProcessResponseDstu2() : null); 112 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Provenance") ? rpProvenanceDstu2() : null); 113 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Questionnaire") ? rpQuestionnaireDstu2() : null); 114 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("QuestionnaireResponse") ? rpQuestionnaireResponseDstu2() : null); 115 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ReferralRequest") ? rpReferralRequestDstu2() : null); 116 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RelatedPerson") ? rpRelatedPersonDstu2() : null); 117 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RiskAssessment") ? rpRiskAssessmentDstu2() : null); 118 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Schedule") ? rpScheduleDstu2() : null); 119 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SearchParameter") ? rpSearchParameterDstu2() : null); 120 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Slot") ? rpSlotDstu2() : null); 121 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Specimen") ? rpSpecimenDstu2() : null); 122 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureDefinition") ? rpStructureDefinitionDstu2() : null); 123 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Subscription") ? rpSubscriptionDstu2() : null); 124 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Substance") ? rpSubstanceDstu2() : null); 125 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyDelivery") ? rpSupplyDeliveryDstu2() : null); 126 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyRequest") ? rpSupplyRequestDstu2() : null); 127 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestScript") ? rpTestScriptDstu2() : null); 128 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ValueSet") ? rpValueSetDstu2() : null); 129 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("VisionPrescription") ? rpVisionPrescriptionDstu2() : null); 130 return retVal; 131 } 132 133 134 @Bean(name="myResourceDaosDstu2") 135 public List<IFhirResourceDao<?>> resourceDaosDstu2(IResourceSupportedSvc theResourceSupportedSvc) { 136 List<IFhirResourceDao<?>> retVal = new ArrayList<IFhirResourceDao<?>>(); 137 if (theResourceSupportedSvc.isSupported("Account")) { 138 retVal.add(daoAccountDstu2()); 139 } 140 if (theResourceSupportedSvc.isSupported("AllergyIntolerance")) { 141 retVal.add(daoAllergyIntoleranceDstu2()); 142 } 143 if (theResourceSupportedSvc.isSupported("Appointment")) { 144 retVal.add(daoAppointmentDstu2()); 145 } 146 if (theResourceSupportedSvc.isSupported("AppointmentResponse")) { 147 retVal.add(daoAppointmentResponseDstu2()); 148 } 149 if (theResourceSupportedSvc.isSupported("AuditEvent")) { 150 retVal.add(daoAuditEventDstu2()); 151 } 152 if (theResourceSupportedSvc.isSupported("Basic")) { 153 retVal.add(daoBasicDstu2()); 154 } 155 if (theResourceSupportedSvc.isSupported("Binary")) { 156 retVal.add(daoBinaryDstu2()); 157 } 158 if (theResourceSupportedSvc.isSupported("BodySite")) { 159 retVal.add(daoBodySiteDstu2()); 160 } 161 if (theResourceSupportedSvc.isSupported("Bundle")) { 162 retVal.add(daoBundleDstu2()); 163 } 164 if (theResourceSupportedSvc.isSupported("CarePlan")) { 165 retVal.add(daoCarePlanDstu2()); 166 } 167 if (theResourceSupportedSvc.isSupported("Claim")) { 168 retVal.add(daoClaimDstu2()); 169 } 170 if (theResourceSupportedSvc.isSupported("ClaimResponse")) { 171 retVal.add(daoClaimResponseDstu2()); 172 } 173 if (theResourceSupportedSvc.isSupported("ClinicalImpression")) { 174 retVal.add(daoClinicalImpressionDstu2()); 175 } 176 if (theResourceSupportedSvc.isSupported("Communication")) { 177 retVal.add(daoCommunicationDstu2()); 178 } 179 if (theResourceSupportedSvc.isSupported("CommunicationRequest")) { 180 retVal.add(daoCommunicationRequestDstu2()); 181 } 182 if (theResourceSupportedSvc.isSupported("Composition")) { 183 retVal.add(daoCompositionDstu2()); 184 } 185 if (theResourceSupportedSvc.isSupported("ConceptMap")) { 186 retVal.add(daoConceptMapDstu2()); 187 } 188 if (theResourceSupportedSvc.isSupported("Condition")) { 189 retVal.add(daoConditionDstu2()); 190 } 191 if (theResourceSupportedSvc.isSupported("Conformance")) { 192 retVal.add(daoConformanceDstu2()); 193 } 194 if (theResourceSupportedSvc.isSupported("Contract")) { 195 retVal.add(daoContractDstu2()); 196 } 197 if (theResourceSupportedSvc.isSupported("Coverage")) { 198 retVal.add(daoCoverageDstu2()); 199 } 200 if (theResourceSupportedSvc.isSupported("DataElement")) { 201 retVal.add(daoDataElementDstu2()); 202 } 203 if (theResourceSupportedSvc.isSupported("DetectedIssue")) { 204 retVal.add(daoDetectedIssueDstu2()); 205 } 206 if (theResourceSupportedSvc.isSupported("Device")) { 207 retVal.add(daoDeviceDstu2()); 208 } 209 if (theResourceSupportedSvc.isSupported("DeviceComponent")) { 210 retVal.add(daoDeviceComponentDstu2()); 211 } 212 if (theResourceSupportedSvc.isSupported("DeviceMetric")) { 213 retVal.add(daoDeviceMetricDstu2()); 214 } 215 if (theResourceSupportedSvc.isSupported("DeviceUseRequest")) { 216 retVal.add(daoDeviceUseRequestDstu2()); 217 } 218 if (theResourceSupportedSvc.isSupported("DeviceUseStatement")) { 219 retVal.add(daoDeviceUseStatementDstu2()); 220 } 221 if (theResourceSupportedSvc.isSupported("DiagnosticOrder")) { 222 retVal.add(daoDiagnosticOrderDstu2()); 223 } 224 if (theResourceSupportedSvc.isSupported("DiagnosticReport")) { 225 retVal.add(daoDiagnosticReportDstu2()); 226 } 227 if (theResourceSupportedSvc.isSupported("DocumentManifest")) { 228 retVal.add(daoDocumentManifestDstu2()); 229 } 230 if (theResourceSupportedSvc.isSupported("DocumentReference")) { 231 retVal.add(daoDocumentReferenceDstu2()); 232 } 233 if (theResourceSupportedSvc.isSupported("EligibilityRequest")) { 234 retVal.add(daoEligibilityRequestDstu2()); 235 } 236 if (theResourceSupportedSvc.isSupported("EligibilityResponse")) { 237 retVal.add(daoEligibilityResponseDstu2()); 238 } 239 if (theResourceSupportedSvc.isSupported("Encounter")) { 240 retVal.add(daoEncounterDstu2()); 241 } 242 if (theResourceSupportedSvc.isSupported("EnrollmentRequest")) { 243 retVal.add(daoEnrollmentRequestDstu2()); 244 } 245 if (theResourceSupportedSvc.isSupported("EnrollmentResponse")) { 246 retVal.add(daoEnrollmentResponseDstu2()); 247 } 248 if (theResourceSupportedSvc.isSupported("EpisodeOfCare")) { 249 retVal.add(daoEpisodeOfCareDstu2()); 250 } 251 if (theResourceSupportedSvc.isSupported("ExplanationOfBenefit")) { 252 retVal.add(daoExplanationOfBenefitDstu2()); 253 } 254 if (theResourceSupportedSvc.isSupported("FamilyMemberHistory")) { 255 retVal.add(daoFamilyMemberHistoryDstu2()); 256 } 257 if (theResourceSupportedSvc.isSupported("Flag")) { 258 retVal.add(daoFlagDstu2()); 259 } 260 if (theResourceSupportedSvc.isSupported("Goal")) { 261 retVal.add(daoGoalDstu2()); 262 } 263 if (theResourceSupportedSvc.isSupported("Group")) { 264 retVal.add(daoGroupDstu2()); 265 } 266 if (theResourceSupportedSvc.isSupported("HealthcareService")) { 267 retVal.add(daoHealthcareServiceDstu2()); 268 } 269 if (theResourceSupportedSvc.isSupported("ImagingObjectSelection")) { 270 retVal.add(daoImagingObjectSelectionDstu2()); 271 } 272 if (theResourceSupportedSvc.isSupported("ImagingStudy")) { 273 retVal.add(daoImagingStudyDstu2()); 274 } 275 if (theResourceSupportedSvc.isSupported("Immunization")) { 276 retVal.add(daoImmunizationDstu2()); 277 } 278 if (theResourceSupportedSvc.isSupported("ImmunizationRecommendation")) { 279 retVal.add(daoImmunizationRecommendationDstu2()); 280 } 281 if (theResourceSupportedSvc.isSupported("ImplementationGuide")) { 282 retVal.add(daoImplementationGuideDstu2()); 283 } 284 if (theResourceSupportedSvc.isSupported("List")) { 285 retVal.add(daoListResourceDstu2()); 286 } 287 if (theResourceSupportedSvc.isSupported("Location")) { 288 retVal.add(daoLocationDstu2()); 289 } 290 if (theResourceSupportedSvc.isSupported("Media")) { 291 retVal.add(daoMediaDstu2()); 292 } 293 if (theResourceSupportedSvc.isSupported("Medication")) { 294 retVal.add(daoMedicationDstu2()); 295 } 296 if (theResourceSupportedSvc.isSupported("MedicationAdministration")) { 297 retVal.add(daoMedicationAdministrationDstu2()); 298 } 299 if (theResourceSupportedSvc.isSupported("MedicationDispense")) { 300 retVal.add(daoMedicationDispenseDstu2()); 301 } 302 if (theResourceSupportedSvc.isSupported("MedicationOrder")) { 303 retVal.add(daoMedicationOrderDstu2()); 304 } 305 if (theResourceSupportedSvc.isSupported("MedicationStatement")) { 306 retVal.add(daoMedicationStatementDstu2()); 307 } 308 if (theResourceSupportedSvc.isSupported("MessageHeader")) { 309 retVal.add(daoMessageHeaderDstu2()); 310 } 311 if (theResourceSupportedSvc.isSupported("NamingSystem")) { 312 retVal.add(daoNamingSystemDstu2()); 313 } 314 if (theResourceSupportedSvc.isSupported("NutritionOrder")) { 315 retVal.add(daoNutritionOrderDstu2()); 316 } 317 if (theResourceSupportedSvc.isSupported("Observation")) { 318 retVal.add(daoObservationDstu2()); 319 } 320 if (theResourceSupportedSvc.isSupported("OperationDefinition")) { 321 retVal.add(daoOperationDefinitionDstu2()); 322 } 323 if (theResourceSupportedSvc.isSupported("OperationOutcome")) { 324 retVal.add(daoOperationOutcomeDstu2()); 325 } 326 if (theResourceSupportedSvc.isSupported("Order")) { 327 retVal.add(daoOrderDstu2()); 328 } 329 if (theResourceSupportedSvc.isSupported("OrderResponse")) { 330 retVal.add(daoOrderResponseDstu2()); 331 } 332 if (theResourceSupportedSvc.isSupported("Organization")) { 333 retVal.add(daoOrganizationDstu2()); 334 } 335 if (theResourceSupportedSvc.isSupported("Parameters")) { 336 retVal.add(daoParametersDstu2()); 337 } 338 if (theResourceSupportedSvc.isSupported("Patient")) { 339 retVal.add(daoPatientDstu2()); 340 } 341 if (theResourceSupportedSvc.isSupported("PaymentNotice")) { 342 retVal.add(daoPaymentNoticeDstu2()); 343 } 344 if (theResourceSupportedSvc.isSupported("PaymentReconciliation")) { 345 retVal.add(daoPaymentReconciliationDstu2()); 346 } 347 if (theResourceSupportedSvc.isSupported("Person")) { 348 retVal.add(daoPersonDstu2()); 349 } 350 if (theResourceSupportedSvc.isSupported("Practitioner")) { 351 retVal.add(daoPractitionerDstu2()); 352 } 353 if (theResourceSupportedSvc.isSupported("Procedure")) { 354 retVal.add(daoProcedureDstu2()); 355 } 356 if (theResourceSupportedSvc.isSupported("ProcedureRequest")) { 357 retVal.add(daoProcedureRequestDstu2()); 358 } 359 if (theResourceSupportedSvc.isSupported("ProcessRequest")) { 360 retVal.add(daoProcessRequestDstu2()); 361 } 362 if (theResourceSupportedSvc.isSupported("ProcessResponse")) { 363 retVal.add(daoProcessResponseDstu2()); 364 } 365 if (theResourceSupportedSvc.isSupported("Provenance")) { 366 retVal.add(daoProvenanceDstu2()); 367 } 368 if (theResourceSupportedSvc.isSupported("Questionnaire")) { 369 retVal.add(daoQuestionnaireDstu2()); 370 } 371 if (theResourceSupportedSvc.isSupported("QuestionnaireResponse")) { 372 retVal.add(daoQuestionnaireResponseDstu2()); 373 } 374 if (theResourceSupportedSvc.isSupported("ReferralRequest")) { 375 retVal.add(daoReferralRequestDstu2()); 376 } 377 if (theResourceSupportedSvc.isSupported("RelatedPerson")) { 378 retVal.add(daoRelatedPersonDstu2()); 379 } 380 if (theResourceSupportedSvc.isSupported("RiskAssessment")) { 381 retVal.add(daoRiskAssessmentDstu2()); 382 } 383 if (theResourceSupportedSvc.isSupported("Schedule")) { 384 retVal.add(daoScheduleDstu2()); 385 } 386 if (theResourceSupportedSvc.isSupported("SearchParameter")) { 387 retVal.add(daoSearchParameterDstu2()); 388 } 389 if (theResourceSupportedSvc.isSupported("Slot")) { 390 retVal.add(daoSlotDstu2()); 391 } 392 if (theResourceSupportedSvc.isSupported("Specimen")) { 393 retVal.add(daoSpecimenDstu2()); 394 } 395 if (theResourceSupportedSvc.isSupported("StructureDefinition")) { 396 retVal.add(daoStructureDefinitionDstu2()); 397 } 398 if (theResourceSupportedSvc.isSupported("Subscription")) { 399 retVal.add(daoSubscriptionDstu2()); 400 } 401 if (theResourceSupportedSvc.isSupported("Substance")) { 402 retVal.add(daoSubstanceDstu2()); 403 } 404 if (theResourceSupportedSvc.isSupported("SupplyDelivery")) { 405 retVal.add(daoSupplyDeliveryDstu2()); 406 } 407 if (theResourceSupportedSvc.isSupported("SupplyRequest")) { 408 retVal.add(daoSupplyRequestDstu2()); 409 } 410 if (theResourceSupportedSvc.isSupported("TestScript")) { 411 retVal.add(daoTestScriptDstu2()); 412 } 413 if (theResourceSupportedSvc.isSupported("ValueSet")) { 414 retVal.add(daoValueSetDstu2()); 415 } 416 if (theResourceSupportedSvc.isSupported("VisionPrescription")) { 417 retVal.add(daoVisionPrescriptionDstu2()); 418 } 419 return retVal; 420 } 421 422 @Bean(name="myAccountDaoDstu2") 423 public 424 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Account> 425 daoAccountDstu2() { 426 427 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Account> retVal; 428 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Account>(); 429 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Account.class); 430 retVal.setContext(myFhirContext); 431 return retVal; 432 } 433 434 @Bean(name="myAccountRpDstu2") 435 @Lazy 436 public ca.uhn.fhir.jpa.rp.dstu2.AccountResourceProvider rpAccountDstu2() { 437 ca.uhn.fhir.jpa.rp.dstu2.AccountResourceProvider retVal; 438 retVal = new ca.uhn.fhir.jpa.rp.dstu2.AccountResourceProvider(); 439 retVal.setContext(myFhirContext); 440 retVal.setDao(daoAccountDstu2()); 441 return retVal; 442 } 443 444 @Bean(name="myAllergyIntoleranceDaoDstu2") 445 public 446 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.AllergyIntolerance> 447 daoAllergyIntoleranceDstu2() { 448 449 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.AllergyIntolerance> retVal; 450 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.AllergyIntolerance>(); 451 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.AllergyIntolerance.class); 452 retVal.setContext(myFhirContext); 453 return retVal; 454 } 455 456 @Bean(name="myAllergyIntoleranceRpDstu2") 457 @Lazy 458 public ca.uhn.fhir.jpa.rp.dstu2.AllergyIntoleranceResourceProvider rpAllergyIntoleranceDstu2() { 459 ca.uhn.fhir.jpa.rp.dstu2.AllergyIntoleranceResourceProvider retVal; 460 retVal = new ca.uhn.fhir.jpa.rp.dstu2.AllergyIntoleranceResourceProvider(); 461 retVal.setContext(myFhirContext); 462 retVal.setDao(daoAllergyIntoleranceDstu2()); 463 return retVal; 464 } 465 466 @Bean(name="myAppointmentDaoDstu2") 467 public 468 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Appointment> 469 daoAppointmentDstu2() { 470 471 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Appointment> retVal; 472 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Appointment>(); 473 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Appointment.class); 474 retVal.setContext(myFhirContext); 475 return retVal; 476 } 477 478 @Bean(name="myAppointmentRpDstu2") 479 @Lazy 480 public ca.uhn.fhir.jpa.rp.dstu2.AppointmentResourceProvider rpAppointmentDstu2() { 481 ca.uhn.fhir.jpa.rp.dstu2.AppointmentResourceProvider retVal; 482 retVal = new ca.uhn.fhir.jpa.rp.dstu2.AppointmentResourceProvider(); 483 retVal.setContext(myFhirContext); 484 retVal.setDao(daoAppointmentDstu2()); 485 return retVal; 486 } 487 488 @Bean(name="myAppointmentResponseDaoDstu2") 489 public 490 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.AppointmentResponse> 491 daoAppointmentResponseDstu2() { 492 493 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.AppointmentResponse> retVal; 494 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.AppointmentResponse>(); 495 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.AppointmentResponse.class); 496 retVal.setContext(myFhirContext); 497 return retVal; 498 } 499 500 @Bean(name="myAppointmentResponseRpDstu2") 501 @Lazy 502 public ca.uhn.fhir.jpa.rp.dstu2.AppointmentResponseResourceProvider rpAppointmentResponseDstu2() { 503 ca.uhn.fhir.jpa.rp.dstu2.AppointmentResponseResourceProvider retVal; 504 retVal = new ca.uhn.fhir.jpa.rp.dstu2.AppointmentResponseResourceProvider(); 505 retVal.setContext(myFhirContext); 506 retVal.setDao(daoAppointmentResponseDstu2()); 507 return retVal; 508 } 509 510 @Bean(name="myAuditEventDaoDstu2") 511 public 512 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.AuditEvent> 513 daoAuditEventDstu2() { 514 515 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.AuditEvent> retVal; 516 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.AuditEvent>(); 517 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.AuditEvent.class); 518 retVal.setContext(myFhirContext); 519 return retVal; 520 } 521 522 @Bean(name="myAuditEventRpDstu2") 523 @Lazy 524 public ca.uhn.fhir.jpa.rp.dstu2.AuditEventResourceProvider rpAuditEventDstu2() { 525 ca.uhn.fhir.jpa.rp.dstu2.AuditEventResourceProvider retVal; 526 retVal = new ca.uhn.fhir.jpa.rp.dstu2.AuditEventResourceProvider(); 527 retVal.setContext(myFhirContext); 528 retVal.setDao(daoAuditEventDstu2()); 529 return retVal; 530 } 531 532 @Bean(name="myBasicDaoDstu2") 533 public 534 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Basic> 535 daoBasicDstu2() { 536 537 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Basic> retVal; 538 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Basic>(); 539 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Basic.class); 540 retVal.setContext(myFhirContext); 541 return retVal; 542 } 543 544 @Bean(name="myBasicRpDstu2") 545 @Lazy 546 public ca.uhn.fhir.jpa.rp.dstu2.BasicResourceProvider rpBasicDstu2() { 547 ca.uhn.fhir.jpa.rp.dstu2.BasicResourceProvider retVal; 548 retVal = new ca.uhn.fhir.jpa.rp.dstu2.BasicResourceProvider(); 549 retVal.setContext(myFhirContext); 550 retVal.setDao(daoBasicDstu2()); 551 return retVal; 552 } 553 554 @Bean(name="myBinaryDaoDstu2") 555 public 556 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Binary> 557 daoBinaryDstu2() { 558 559 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Binary> retVal; 560 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Binary>(); 561 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Binary.class); 562 retVal.setContext(myFhirContext); 563 return retVal; 564 } 565 566 @Bean(name="myBinaryRpDstu2") 567 @Lazy 568 public ca.uhn.fhir.jpa.rp.dstu2.BinaryResourceProvider rpBinaryDstu2() { 569 ca.uhn.fhir.jpa.rp.dstu2.BinaryResourceProvider retVal; 570 retVal = new ca.uhn.fhir.jpa.rp.dstu2.BinaryResourceProvider(); 571 retVal.setContext(myFhirContext); 572 retVal.setDao(daoBinaryDstu2()); 573 return retVal; 574 } 575 576 @Bean(name="myBodySiteDaoDstu2") 577 public 578 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.BodySite> 579 daoBodySiteDstu2() { 580 581 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.BodySite> retVal; 582 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.BodySite>(); 583 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.BodySite.class); 584 retVal.setContext(myFhirContext); 585 return retVal; 586 } 587 588 @Bean(name="myBodySiteRpDstu2") 589 @Lazy 590 public ca.uhn.fhir.jpa.rp.dstu2.BodySiteResourceProvider rpBodySiteDstu2() { 591 ca.uhn.fhir.jpa.rp.dstu2.BodySiteResourceProvider retVal; 592 retVal = new ca.uhn.fhir.jpa.rp.dstu2.BodySiteResourceProvider(); 593 retVal.setContext(myFhirContext); 594 retVal.setDao(daoBodySiteDstu2()); 595 return retVal; 596 } 597 598 @Bean(name="myBundleDaoDstu2") 599 public 600 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Bundle> 601 daoBundleDstu2() { 602 603 ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<ca.uhn.fhir.model.dstu2.resource.Bundle> retVal; 604 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<>(); 605 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Bundle.class); 606 retVal.setContext(myFhirContext); 607 return retVal; 608 } 609 610 @Bean(name="myBundleRpDstu2") 611 @Lazy 612 public ca.uhn.fhir.jpa.rp.dstu2.BundleResourceProvider rpBundleDstu2() { 613 ca.uhn.fhir.jpa.rp.dstu2.BundleResourceProvider retVal; 614 retVal = new ca.uhn.fhir.jpa.rp.dstu2.BundleResourceProvider(); 615 retVal.setContext(myFhirContext); 616 retVal.setDao(daoBundleDstu2()); 617 return retVal; 618 } 619 620 @Bean(name="myCarePlanDaoDstu2") 621 public 622 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.CarePlan> 623 daoCarePlanDstu2() { 624 625 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.CarePlan> retVal; 626 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.CarePlan>(); 627 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.CarePlan.class); 628 retVal.setContext(myFhirContext); 629 return retVal; 630 } 631 632 @Bean(name="myCarePlanRpDstu2") 633 @Lazy 634 public ca.uhn.fhir.jpa.rp.dstu2.CarePlanResourceProvider rpCarePlanDstu2() { 635 ca.uhn.fhir.jpa.rp.dstu2.CarePlanResourceProvider retVal; 636 retVal = new ca.uhn.fhir.jpa.rp.dstu2.CarePlanResourceProvider(); 637 retVal.setContext(myFhirContext); 638 retVal.setDao(daoCarePlanDstu2()); 639 return retVal; 640 } 641 642 @Bean(name="myClaimDaoDstu2") 643 public 644 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Claim> 645 daoClaimDstu2() { 646 647 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Claim> retVal; 648 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Claim>(); 649 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Claim.class); 650 retVal.setContext(myFhirContext); 651 return retVal; 652 } 653 654 @Bean(name="myClaimRpDstu2") 655 @Lazy 656 public ca.uhn.fhir.jpa.rp.dstu2.ClaimResourceProvider rpClaimDstu2() { 657 ca.uhn.fhir.jpa.rp.dstu2.ClaimResourceProvider retVal; 658 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ClaimResourceProvider(); 659 retVal.setContext(myFhirContext); 660 retVal.setDao(daoClaimDstu2()); 661 return retVal; 662 } 663 664 @Bean(name="myClaimResponseDaoDstu2") 665 public 666 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ClaimResponse> 667 daoClaimResponseDstu2() { 668 669 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ClaimResponse> retVal; 670 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ClaimResponse>(); 671 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ClaimResponse.class); 672 retVal.setContext(myFhirContext); 673 return retVal; 674 } 675 676 @Bean(name="myClaimResponseRpDstu2") 677 @Lazy 678 public ca.uhn.fhir.jpa.rp.dstu2.ClaimResponseResourceProvider rpClaimResponseDstu2() { 679 ca.uhn.fhir.jpa.rp.dstu2.ClaimResponseResourceProvider retVal; 680 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ClaimResponseResourceProvider(); 681 retVal.setContext(myFhirContext); 682 retVal.setDao(daoClaimResponseDstu2()); 683 return retVal; 684 } 685 686 @Bean(name="myClinicalImpressionDaoDstu2") 687 public 688 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ClinicalImpression> 689 daoClinicalImpressionDstu2() { 690 691 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ClinicalImpression> retVal; 692 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ClinicalImpression>(); 693 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ClinicalImpression.class); 694 retVal.setContext(myFhirContext); 695 return retVal; 696 } 697 698 @Bean(name="myClinicalImpressionRpDstu2") 699 @Lazy 700 public ca.uhn.fhir.jpa.rp.dstu2.ClinicalImpressionResourceProvider rpClinicalImpressionDstu2() { 701 ca.uhn.fhir.jpa.rp.dstu2.ClinicalImpressionResourceProvider retVal; 702 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ClinicalImpressionResourceProvider(); 703 retVal.setContext(myFhirContext); 704 retVal.setDao(daoClinicalImpressionDstu2()); 705 return retVal; 706 } 707 708 @Bean(name="myCommunicationDaoDstu2") 709 public 710 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Communication> 711 daoCommunicationDstu2() { 712 713 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Communication> retVal; 714 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Communication>(); 715 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Communication.class); 716 retVal.setContext(myFhirContext); 717 return retVal; 718 } 719 720 @Bean(name="myCommunicationRpDstu2") 721 @Lazy 722 public ca.uhn.fhir.jpa.rp.dstu2.CommunicationResourceProvider rpCommunicationDstu2() { 723 ca.uhn.fhir.jpa.rp.dstu2.CommunicationResourceProvider retVal; 724 retVal = new ca.uhn.fhir.jpa.rp.dstu2.CommunicationResourceProvider(); 725 retVal.setContext(myFhirContext); 726 retVal.setDao(daoCommunicationDstu2()); 727 return retVal; 728 } 729 730 @Bean(name="myCommunicationRequestDaoDstu2") 731 public 732 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.CommunicationRequest> 733 daoCommunicationRequestDstu2() { 734 735 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.CommunicationRequest> retVal; 736 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.CommunicationRequest>(); 737 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.CommunicationRequest.class); 738 retVal.setContext(myFhirContext); 739 return retVal; 740 } 741 742 @Bean(name="myCommunicationRequestRpDstu2") 743 @Lazy 744 public ca.uhn.fhir.jpa.rp.dstu2.CommunicationRequestResourceProvider rpCommunicationRequestDstu2() { 745 ca.uhn.fhir.jpa.rp.dstu2.CommunicationRequestResourceProvider retVal; 746 retVal = new ca.uhn.fhir.jpa.rp.dstu2.CommunicationRequestResourceProvider(); 747 retVal.setContext(myFhirContext); 748 retVal.setDao(daoCommunicationRequestDstu2()); 749 return retVal; 750 } 751 752 @Bean(name="myCompositionDaoDstu2") 753 public 754 IFhirResourceDaoComposition<ca.uhn.fhir.model.dstu2.resource.Composition> 755 daoCompositionDstu2() { 756 757 ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<ca.uhn.fhir.model.dstu2.resource.Composition> retVal; 758 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<>(); 759 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Composition.class); 760 retVal.setContext(myFhirContext); 761 return retVal; 762 } 763 764 @Bean(name="myCompositionRpDstu2") 765 @Lazy 766 public ca.uhn.fhir.jpa.rp.dstu2.CompositionResourceProvider rpCompositionDstu2() { 767 ca.uhn.fhir.jpa.rp.dstu2.CompositionResourceProvider retVal; 768 retVal = new ca.uhn.fhir.jpa.rp.dstu2.CompositionResourceProvider(); 769 retVal.setContext(myFhirContext); 770 retVal.setDao(daoCompositionDstu2()); 771 return retVal; 772 } 773 774 @Bean(name="myConceptMapDaoDstu2") 775 public 776 IFhirResourceDaoConceptMap<ca.uhn.fhir.model.dstu2.resource.ConceptMap> 777 daoConceptMapDstu2() { 778 779 ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<ca.uhn.fhir.model.dstu2.resource.ConceptMap> retVal; 780 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<>(); 781 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ConceptMap.class); 782 retVal.setContext(myFhirContext); 783 return retVal; 784 } 785 786 @Bean(name="myConceptMapRpDstu2") 787 @Lazy 788 public ca.uhn.fhir.jpa.rp.dstu2.ConceptMapResourceProvider rpConceptMapDstu2() { 789 ca.uhn.fhir.jpa.rp.dstu2.ConceptMapResourceProvider retVal; 790 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ConceptMapResourceProvider(); 791 retVal.setContext(myFhirContext); 792 retVal.setDao(daoConceptMapDstu2()); 793 return retVal; 794 } 795 796 @Bean(name="myConditionDaoDstu2") 797 public 798 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Condition> 799 daoConditionDstu2() { 800 801 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Condition> retVal; 802 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Condition>(); 803 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Condition.class); 804 retVal.setContext(myFhirContext); 805 return retVal; 806 } 807 808 @Bean(name="myConditionRpDstu2") 809 @Lazy 810 public ca.uhn.fhir.jpa.rp.dstu2.ConditionResourceProvider rpConditionDstu2() { 811 ca.uhn.fhir.jpa.rp.dstu2.ConditionResourceProvider retVal; 812 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ConditionResourceProvider(); 813 retVal.setContext(myFhirContext); 814 retVal.setDao(daoConditionDstu2()); 815 return retVal; 816 } 817 818 @Bean(name="myConformanceDaoDstu2") 819 public 820 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Conformance> 821 daoConformanceDstu2() { 822 823 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Conformance> retVal; 824 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Conformance>(); 825 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Conformance.class); 826 retVal.setContext(myFhirContext); 827 return retVal; 828 } 829 830 @Bean(name="myConformanceRpDstu2") 831 @Lazy 832 public ca.uhn.fhir.jpa.rp.dstu2.ConformanceResourceProvider rpConformanceDstu2() { 833 ca.uhn.fhir.jpa.rp.dstu2.ConformanceResourceProvider retVal; 834 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ConformanceResourceProvider(); 835 retVal.setContext(myFhirContext); 836 retVal.setDao(daoConformanceDstu2()); 837 return retVal; 838 } 839 840 @Bean(name="myContractDaoDstu2") 841 public 842 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Contract> 843 daoContractDstu2() { 844 845 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Contract> retVal; 846 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Contract>(); 847 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Contract.class); 848 retVal.setContext(myFhirContext); 849 return retVal; 850 } 851 852 @Bean(name="myContractRpDstu2") 853 @Lazy 854 public ca.uhn.fhir.jpa.rp.dstu2.ContractResourceProvider rpContractDstu2() { 855 ca.uhn.fhir.jpa.rp.dstu2.ContractResourceProvider retVal; 856 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ContractResourceProvider(); 857 retVal.setContext(myFhirContext); 858 retVal.setDao(daoContractDstu2()); 859 return retVal; 860 } 861 862 @Bean(name="myCoverageDaoDstu2") 863 public 864 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Coverage> 865 daoCoverageDstu2() { 866 867 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Coverage> retVal; 868 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Coverage>(); 869 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Coverage.class); 870 retVal.setContext(myFhirContext); 871 return retVal; 872 } 873 874 @Bean(name="myCoverageRpDstu2") 875 @Lazy 876 public ca.uhn.fhir.jpa.rp.dstu2.CoverageResourceProvider rpCoverageDstu2() { 877 ca.uhn.fhir.jpa.rp.dstu2.CoverageResourceProvider retVal; 878 retVal = new ca.uhn.fhir.jpa.rp.dstu2.CoverageResourceProvider(); 879 retVal.setContext(myFhirContext); 880 retVal.setDao(daoCoverageDstu2()); 881 return retVal; 882 } 883 884 @Bean(name="myDataElementDaoDstu2") 885 public 886 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DataElement> 887 daoDataElementDstu2() { 888 889 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DataElement> retVal; 890 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DataElement>(); 891 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DataElement.class); 892 retVal.setContext(myFhirContext); 893 return retVal; 894 } 895 896 @Bean(name="myDataElementRpDstu2") 897 @Lazy 898 public ca.uhn.fhir.jpa.rp.dstu2.DataElementResourceProvider rpDataElementDstu2() { 899 ca.uhn.fhir.jpa.rp.dstu2.DataElementResourceProvider retVal; 900 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DataElementResourceProvider(); 901 retVal.setContext(myFhirContext); 902 retVal.setDao(daoDataElementDstu2()); 903 return retVal; 904 } 905 906 @Bean(name="myDetectedIssueDaoDstu2") 907 public 908 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DetectedIssue> 909 daoDetectedIssueDstu2() { 910 911 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DetectedIssue> retVal; 912 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DetectedIssue>(); 913 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DetectedIssue.class); 914 retVal.setContext(myFhirContext); 915 return retVal; 916 } 917 918 @Bean(name="myDetectedIssueRpDstu2") 919 @Lazy 920 public ca.uhn.fhir.jpa.rp.dstu2.DetectedIssueResourceProvider rpDetectedIssueDstu2() { 921 ca.uhn.fhir.jpa.rp.dstu2.DetectedIssueResourceProvider retVal; 922 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DetectedIssueResourceProvider(); 923 retVal.setContext(myFhirContext); 924 retVal.setDao(daoDetectedIssueDstu2()); 925 return retVal; 926 } 927 928 @Bean(name="myDeviceDaoDstu2") 929 public 930 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Device> 931 daoDeviceDstu2() { 932 933 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Device> retVal; 934 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Device>(); 935 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Device.class); 936 retVal.setContext(myFhirContext); 937 return retVal; 938 } 939 940 @Bean(name="myDeviceRpDstu2") 941 @Lazy 942 public ca.uhn.fhir.jpa.rp.dstu2.DeviceResourceProvider rpDeviceDstu2() { 943 ca.uhn.fhir.jpa.rp.dstu2.DeviceResourceProvider retVal; 944 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DeviceResourceProvider(); 945 retVal.setContext(myFhirContext); 946 retVal.setDao(daoDeviceDstu2()); 947 return retVal; 948 } 949 950 @Bean(name="myDeviceComponentDaoDstu2") 951 public 952 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceComponent> 953 daoDeviceComponentDstu2() { 954 955 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceComponent> retVal; 956 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceComponent>(); 957 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DeviceComponent.class); 958 retVal.setContext(myFhirContext); 959 return retVal; 960 } 961 962 @Bean(name="myDeviceComponentRpDstu2") 963 @Lazy 964 public ca.uhn.fhir.jpa.rp.dstu2.DeviceComponentResourceProvider rpDeviceComponentDstu2() { 965 ca.uhn.fhir.jpa.rp.dstu2.DeviceComponentResourceProvider retVal; 966 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DeviceComponentResourceProvider(); 967 retVal.setContext(myFhirContext); 968 retVal.setDao(daoDeviceComponentDstu2()); 969 return retVal; 970 } 971 972 @Bean(name="myDeviceMetricDaoDstu2") 973 public 974 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceMetric> 975 daoDeviceMetricDstu2() { 976 977 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceMetric> retVal; 978 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceMetric>(); 979 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DeviceMetric.class); 980 retVal.setContext(myFhirContext); 981 return retVal; 982 } 983 984 @Bean(name="myDeviceMetricRpDstu2") 985 @Lazy 986 public ca.uhn.fhir.jpa.rp.dstu2.DeviceMetricResourceProvider rpDeviceMetricDstu2() { 987 ca.uhn.fhir.jpa.rp.dstu2.DeviceMetricResourceProvider retVal; 988 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DeviceMetricResourceProvider(); 989 retVal.setContext(myFhirContext); 990 retVal.setDao(daoDeviceMetricDstu2()); 991 return retVal; 992 } 993 994 @Bean(name="myDeviceUseRequestDaoDstu2") 995 public 996 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceUseRequest> 997 daoDeviceUseRequestDstu2() { 998 999 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceUseRequest> retVal; 1000 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceUseRequest>(); 1001 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DeviceUseRequest.class); 1002 retVal.setContext(myFhirContext); 1003 return retVal; 1004 } 1005 1006 @Bean(name="myDeviceUseRequestRpDstu2") 1007 @Lazy 1008 public ca.uhn.fhir.jpa.rp.dstu2.DeviceUseRequestResourceProvider rpDeviceUseRequestDstu2() { 1009 ca.uhn.fhir.jpa.rp.dstu2.DeviceUseRequestResourceProvider retVal; 1010 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DeviceUseRequestResourceProvider(); 1011 retVal.setContext(myFhirContext); 1012 retVal.setDao(daoDeviceUseRequestDstu2()); 1013 return retVal; 1014 } 1015 1016 @Bean(name="myDeviceUseStatementDaoDstu2") 1017 public 1018 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceUseStatement> 1019 daoDeviceUseStatementDstu2() { 1020 1021 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceUseStatement> retVal; 1022 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DeviceUseStatement>(); 1023 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DeviceUseStatement.class); 1024 retVal.setContext(myFhirContext); 1025 return retVal; 1026 } 1027 1028 @Bean(name="myDeviceUseStatementRpDstu2") 1029 @Lazy 1030 public ca.uhn.fhir.jpa.rp.dstu2.DeviceUseStatementResourceProvider rpDeviceUseStatementDstu2() { 1031 ca.uhn.fhir.jpa.rp.dstu2.DeviceUseStatementResourceProvider retVal; 1032 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DeviceUseStatementResourceProvider(); 1033 retVal.setContext(myFhirContext); 1034 retVal.setDao(daoDeviceUseStatementDstu2()); 1035 return retVal; 1036 } 1037 1038 @Bean(name="myDiagnosticOrderDaoDstu2") 1039 public 1040 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DiagnosticOrder> 1041 daoDiagnosticOrderDstu2() { 1042 1043 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DiagnosticOrder> retVal; 1044 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DiagnosticOrder>(); 1045 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DiagnosticOrder.class); 1046 retVal.setContext(myFhirContext); 1047 return retVal; 1048 } 1049 1050 @Bean(name="myDiagnosticOrderRpDstu2") 1051 @Lazy 1052 public ca.uhn.fhir.jpa.rp.dstu2.DiagnosticOrderResourceProvider rpDiagnosticOrderDstu2() { 1053 ca.uhn.fhir.jpa.rp.dstu2.DiagnosticOrderResourceProvider retVal; 1054 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DiagnosticOrderResourceProvider(); 1055 retVal.setContext(myFhirContext); 1056 retVal.setDao(daoDiagnosticOrderDstu2()); 1057 return retVal; 1058 } 1059 1060 @Bean(name="myDiagnosticReportDaoDstu2") 1061 public 1062 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DiagnosticReport> 1063 daoDiagnosticReportDstu2() { 1064 1065 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DiagnosticReport> retVal; 1066 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DiagnosticReport>(); 1067 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DiagnosticReport.class); 1068 retVal.setContext(myFhirContext); 1069 return retVal; 1070 } 1071 1072 @Bean(name="myDiagnosticReportRpDstu2") 1073 @Lazy 1074 public ca.uhn.fhir.jpa.rp.dstu2.DiagnosticReportResourceProvider rpDiagnosticReportDstu2() { 1075 ca.uhn.fhir.jpa.rp.dstu2.DiagnosticReportResourceProvider retVal; 1076 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DiagnosticReportResourceProvider(); 1077 retVal.setContext(myFhirContext); 1078 retVal.setDao(daoDiagnosticReportDstu2()); 1079 return retVal; 1080 } 1081 1082 @Bean(name="myDocumentManifestDaoDstu2") 1083 public 1084 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DocumentManifest> 1085 daoDocumentManifestDstu2() { 1086 1087 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DocumentManifest> retVal; 1088 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DocumentManifest>(); 1089 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DocumentManifest.class); 1090 retVal.setContext(myFhirContext); 1091 return retVal; 1092 } 1093 1094 @Bean(name="myDocumentManifestRpDstu2") 1095 @Lazy 1096 public ca.uhn.fhir.jpa.rp.dstu2.DocumentManifestResourceProvider rpDocumentManifestDstu2() { 1097 ca.uhn.fhir.jpa.rp.dstu2.DocumentManifestResourceProvider retVal; 1098 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DocumentManifestResourceProvider(); 1099 retVal.setContext(myFhirContext); 1100 retVal.setDao(daoDocumentManifestDstu2()); 1101 return retVal; 1102 } 1103 1104 @Bean(name="myDocumentReferenceDaoDstu2") 1105 public 1106 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DocumentReference> 1107 daoDocumentReferenceDstu2() { 1108 1109 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.DocumentReference> retVal; 1110 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.DocumentReference>(); 1111 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.DocumentReference.class); 1112 retVal.setContext(myFhirContext); 1113 return retVal; 1114 } 1115 1116 @Bean(name="myDocumentReferenceRpDstu2") 1117 @Lazy 1118 public ca.uhn.fhir.jpa.rp.dstu2.DocumentReferenceResourceProvider rpDocumentReferenceDstu2() { 1119 ca.uhn.fhir.jpa.rp.dstu2.DocumentReferenceResourceProvider retVal; 1120 retVal = new ca.uhn.fhir.jpa.rp.dstu2.DocumentReferenceResourceProvider(); 1121 retVal.setContext(myFhirContext); 1122 retVal.setDao(daoDocumentReferenceDstu2()); 1123 return retVal; 1124 } 1125 1126 @Bean(name="myEligibilityRequestDaoDstu2") 1127 public 1128 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EligibilityRequest> 1129 daoEligibilityRequestDstu2() { 1130 1131 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EligibilityRequest> retVal; 1132 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.EligibilityRequest>(); 1133 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.EligibilityRequest.class); 1134 retVal.setContext(myFhirContext); 1135 return retVal; 1136 } 1137 1138 @Bean(name="myEligibilityRequestRpDstu2") 1139 @Lazy 1140 public ca.uhn.fhir.jpa.rp.dstu2.EligibilityRequestResourceProvider rpEligibilityRequestDstu2() { 1141 ca.uhn.fhir.jpa.rp.dstu2.EligibilityRequestResourceProvider retVal; 1142 retVal = new ca.uhn.fhir.jpa.rp.dstu2.EligibilityRequestResourceProvider(); 1143 retVal.setContext(myFhirContext); 1144 retVal.setDao(daoEligibilityRequestDstu2()); 1145 return retVal; 1146 } 1147 1148 @Bean(name="myEligibilityResponseDaoDstu2") 1149 public 1150 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EligibilityResponse> 1151 daoEligibilityResponseDstu2() { 1152 1153 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EligibilityResponse> retVal; 1154 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.EligibilityResponse>(); 1155 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.EligibilityResponse.class); 1156 retVal.setContext(myFhirContext); 1157 return retVal; 1158 } 1159 1160 @Bean(name="myEligibilityResponseRpDstu2") 1161 @Lazy 1162 public ca.uhn.fhir.jpa.rp.dstu2.EligibilityResponseResourceProvider rpEligibilityResponseDstu2() { 1163 ca.uhn.fhir.jpa.rp.dstu2.EligibilityResponseResourceProvider retVal; 1164 retVal = new ca.uhn.fhir.jpa.rp.dstu2.EligibilityResponseResourceProvider(); 1165 retVal.setContext(myFhirContext); 1166 retVal.setDao(daoEligibilityResponseDstu2()); 1167 return retVal; 1168 } 1169 1170 @Bean(name="myEncounterDaoDstu2") 1171 public 1172 IFhirResourceDaoEncounter<ca.uhn.fhir.model.dstu2.resource.Encounter> 1173 daoEncounterDstu2() { 1174 1175 ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<ca.uhn.fhir.model.dstu2.resource.Encounter> retVal; 1176 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<>(); 1177 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Encounter.class); 1178 retVal.setContext(myFhirContext); 1179 return retVal; 1180 } 1181 1182 @Bean(name="myEncounterRpDstu2") 1183 @Lazy 1184 public ca.uhn.fhir.jpa.rp.dstu2.EncounterResourceProvider rpEncounterDstu2() { 1185 ca.uhn.fhir.jpa.rp.dstu2.EncounterResourceProvider retVal; 1186 retVal = new ca.uhn.fhir.jpa.rp.dstu2.EncounterResourceProvider(); 1187 retVal.setContext(myFhirContext); 1188 retVal.setDao(daoEncounterDstu2()); 1189 return retVal; 1190 } 1191 1192 @Bean(name="myEnrollmentRequestDaoDstu2") 1193 public 1194 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EnrollmentRequest> 1195 daoEnrollmentRequestDstu2() { 1196 1197 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EnrollmentRequest> retVal; 1198 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.EnrollmentRequest>(); 1199 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.EnrollmentRequest.class); 1200 retVal.setContext(myFhirContext); 1201 return retVal; 1202 } 1203 1204 @Bean(name="myEnrollmentRequestRpDstu2") 1205 @Lazy 1206 public ca.uhn.fhir.jpa.rp.dstu2.EnrollmentRequestResourceProvider rpEnrollmentRequestDstu2() { 1207 ca.uhn.fhir.jpa.rp.dstu2.EnrollmentRequestResourceProvider retVal; 1208 retVal = new ca.uhn.fhir.jpa.rp.dstu2.EnrollmentRequestResourceProvider(); 1209 retVal.setContext(myFhirContext); 1210 retVal.setDao(daoEnrollmentRequestDstu2()); 1211 return retVal; 1212 } 1213 1214 @Bean(name="myEnrollmentResponseDaoDstu2") 1215 public 1216 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EnrollmentResponse> 1217 daoEnrollmentResponseDstu2() { 1218 1219 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EnrollmentResponse> retVal; 1220 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.EnrollmentResponse>(); 1221 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.EnrollmentResponse.class); 1222 retVal.setContext(myFhirContext); 1223 return retVal; 1224 } 1225 1226 @Bean(name="myEnrollmentResponseRpDstu2") 1227 @Lazy 1228 public ca.uhn.fhir.jpa.rp.dstu2.EnrollmentResponseResourceProvider rpEnrollmentResponseDstu2() { 1229 ca.uhn.fhir.jpa.rp.dstu2.EnrollmentResponseResourceProvider retVal; 1230 retVal = new ca.uhn.fhir.jpa.rp.dstu2.EnrollmentResponseResourceProvider(); 1231 retVal.setContext(myFhirContext); 1232 retVal.setDao(daoEnrollmentResponseDstu2()); 1233 return retVal; 1234 } 1235 1236 @Bean(name="myEpisodeOfCareDaoDstu2") 1237 public 1238 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EpisodeOfCare> 1239 daoEpisodeOfCareDstu2() { 1240 1241 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.EpisodeOfCare> retVal; 1242 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.EpisodeOfCare>(); 1243 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.EpisodeOfCare.class); 1244 retVal.setContext(myFhirContext); 1245 return retVal; 1246 } 1247 1248 @Bean(name="myEpisodeOfCareRpDstu2") 1249 @Lazy 1250 public ca.uhn.fhir.jpa.rp.dstu2.EpisodeOfCareResourceProvider rpEpisodeOfCareDstu2() { 1251 ca.uhn.fhir.jpa.rp.dstu2.EpisodeOfCareResourceProvider retVal; 1252 retVal = new ca.uhn.fhir.jpa.rp.dstu2.EpisodeOfCareResourceProvider(); 1253 retVal.setContext(myFhirContext); 1254 retVal.setDao(daoEpisodeOfCareDstu2()); 1255 return retVal; 1256 } 1257 1258 @Bean(name="myExplanationOfBenefitDaoDstu2") 1259 public 1260 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ExplanationOfBenefit> 1261 daoExplanationOfBenefitDstu2() { 1262 1263 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ExplanationOfBenefit> retVal; 1264 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ExplanationOfBenefit>(); 1265 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ExplanationOfBenefit.class); 1266 retVal.setContext(myFhirContext); 1267 return retVal; 1268 } 1269 1270 @Bean(name="myExplanationOfBenefitRpDstu2") 1271 @Lazy 1272 public ca.uhn.fhir.jpa.rp.dstu2.ExplanationOfBenefitResourceProvider rpExplanationOfBenefitDstu2() { 1273 ca.uhn.fhir.jpa.rp.dstu2.ExplanationOfBenefitResourceProvider retVal; 1274 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ExplanationOfBenefitResourceProvider(); 1275 retVal.setContext(myFhirContext); 1276 retVal.setDao(daoExplanationOfBenefitDstu2()); 1277 return retVal; 1278 } 1279 1280 @Bean(name="myFamilyMemberHistoryDaoDstu2") 1281 public 1282 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.FamilyMemberHistory> 1283 daoFamilyMemberHistoryDstu2() { 1284 1285 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.FamilyMemberHistory> retVal; 1286 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.FamilyMemberHistory>(); 1287 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.FamilyMemberHistory.class); 1288 retVal.setContext(myFhirContext); 1289 return retVal; 1290 } 1291 1292 @Bean(name="myFamilyMemberHistoryRpDstu2") 1293 @Lazy 1294 public ca.uhn.fhir.jpa.rp.dstu2.FamilyMemberHistoryResourceProvider rpFamilyMemberHistoryDstu2() { 1295 ca.uhn.fhir.jpa.rp.dstu2.FamilyMemberHistoryResourceProvider retVal; 1296 retVal = new ca.uhn.fhir.jpa.rp.dstu2.FamilyMemberHistoryResourceProvider(); 1297 retVal.setContext(myFhirContext); 1298 retVal.setDao(daoFamilyMemberHistoryDstu2()); 1299 return retVal; 1300 } 1301 1302 @Bean(name="myFlagDaoDstu2") 1303 public 1304 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Flag> 1305 daoFlagDstu2() { 1306 1307 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Flag> retVal; 1308 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Flag>(); 1309 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Flag.class); 1310 retVal.setContext(myFhirContext); 1311 return retVal; 1312 } 1313 1314 @Bean(name="myFlagRpDstu2") 1315 @Lazy 1316 public ca.uhn.fhir.jpa.rp.dstu2.FlagResourceProvider rpFlagDstu2() { 1317 ca.uhn.fhir.jpa.rp.dstu2.FlagResourceProvider retVal; 1318 retVal = new ca.uhn.fhir.jpa.rp.dstu2.FlagResourceProvider(); 1319 retVal.setContext(myFhirContext); 1320 retVal.setDao(daoFlagDstu2()); 1321 return retVal; 1322 } 1323 1324 @Bean(name="myGoalDaoDstu2") 1325 public 1326 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Goal> 1327 daoGoalDstu2() { 1328 1329 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Goal> retVal; 1330 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Goal>(); 1331 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Goal.class); 1332 retVal.setContext(myFhirContext); 1333 return retVal; 1334 } 1335 1336 @Bean(name="myGoalRpDstu2") 1337 @Lazy 1338 public ca.uhn.fhir.jpa.rp.dstu2.GoalResourceProvider rpGoalDstu2() { 1339 ca.uhn.fhir.jpa.rp.dstu2.GoalResourceProvider retVal; 1340 retVal = new ca.uhn.fhir.jpa.rp.dstu2.GoalResourceProvider(); 1341 retVal.setContext(myFhirContext); 1342 retVal.setDao(daoGoalDstu2()); 1343 return retVal; 1344 } 1345 1346 @Bean(name="myGroupDaoDstu2") 1347 public 1348 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Group> 1349 daoGroupDstu2() { 1350 1351 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Group> retVal; 1352 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Group>(); 1353 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Group.class); 1354 retVal.setContext(myFhirContext); 1355 return retVal; 1356 } 1357 1358 @Bean(name="myGroupRpDstu2") 1359 @Lazy 1360 public ca.uhn.fhir.jpa.rp.dstu2.GroupResourceProvider rpGroupDstu2() { 1361 ca.uhn.fhir.jpa.rp.dstu2.GroupResourceProvider retVal; 1362 retVal = new ca.uhn.fhir.jpa.rp.dstu2.GroupResourceProvider(); 1363 retVal.setContext(myFhirContext); 1364 retVal.setDao(daoGroupDstu2()); 1365 return retVal; 1366 } 1367 1368 @Bean(name="myHealthcareServiceDaoDstu2") 1369 public 1370 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.HealthcareService> 1371 daoHealthcareServiceDstu2() { 1372 1373 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.HealthcareService> retVal; 1374 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.HealthcareService>(); 1375 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.HealthcareService.class); 1376 retVal.setContext(myFhirContext); 1377 return retVal; 1378 } 1379 1380 @Bean(name="myHealthcareServiceRpDstu2") 1381 @Lazy 1382 public ca.uhn.fhir.jpa.rp.dstu2.HealthcareServiceResourceProvider rpHealthcareServiceDstu2() { 1383 ca.uhn.fhir.jpa.rp.dstu2.HealthcareServiceResourceProvider retVal; 1384 retVal = new ca.uhn.fhir.jpa.rp.dstu2.HealthcareServiceResourceProvider(); 1385 retVal.setContext(myFhirContext); 1386 retVal.setDao(daoHealthcareServiceDstu2()); 1387 return retVal; 1388 } 1389 1390 @Bean(name="myImagingObjectSelectionDaoDstu2") 1391 public 1392 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ImagingObjectSelection> 1393 daoImagingObjectSelectionDstu2() { 1394 1395 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ImagingObjectSelection> retVal; 1396 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ImagingObjectSelection>(); 1397 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ImagingObjectSelection.class); 1398 retVal.setContext(myFhirContext); 1399 return retVal; 1400 } 1401 1402 @Bean(name="myImagingObjectSelectionRpDstu2") 1403 @Lazy 1404 public ca.uhn.fhir.jpa.rp.dstu2.ImagingObjectSelectionResourceProvider rpImagingObjectSelectionDstu2() { 1405 ca.uhn.fhir.jpa.rp.dstu2.ImagingObjectSelectionResourceProvider retVal; 1406 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ImagingObjectSelectionResourceProvider(); 1407 retVal.setContext(myFhirContext); 1408 retVal.setDao(daoImagingObjectSelectionDstu2()); 1409 return retVal; 1410 } 1411 1412 @Bean(name="myImagingStudyDaoDstu2") 1413 public 1414 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ImagingStudy> 1415 daoImagingStudyDstu2() { 1416 1417 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ImagingStudy> retVal; 1418 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ImagingStudy>(); 1419 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ImagingStudy.class); 1420 retVal.setContext(myFhirContext); 1421 return retVal; 1422 } 1423 1424 @Bean(name="myImagingStudyRpDstu2") 1425 @Lazy 1426 public ca.uhn.fhir.jpa.rp.dstu2.ImagingStudyResourceProvider rpImagingStudyDstu2() { 1427 ca.uhn.fhir.jpa.rp.dstu2.ImagingStudyResourceProvider retVal; 1428 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ImagingStudyResourceProvider(); 1429 retVal.setContext(myFhirContext); 1430 retVal.setDao(daoImagingStudyDstu2()); 1431 return retVal; 1432 } 1433 1434 @Bean(name="myImmunizationDaoDstu2") 1435 public 1436 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Immunization> 1437 daoImmunizationDstu2() { 1438 1439 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Immunization> retVal; 1440 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Immunization>(); 1441 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Immunization.class); 1442 retVal.setContext(myFhirContext); 1443 return retVal; 1444 } 1445 1446 @Bean(name="myImmunizationRpDstu2") 1447 @Lazy 1448 public ca.uhn.fhir.jpa.rp.dstu2.ImmunizationResourceProvider rpImmunizationDstu2() { 1449 ca.uhn.fhir.jpa.rp.dstu2.ImmunizationResourceProvider retVal; 1450 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ImmunizationResourceProvider(); 1451 retVal.setContext(myFhirContext); 1452 retVal.setDao(daoImmunizationDstu2()); 1453 return retVal; 1454 } 1455 1456 @Bean(name="myImmunizationRecommendationDaoDstu2") 1457 public 1458 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ImmunizationRecommendation> 1459 daoImmunizationRecommendationDstu2() { 1460 1461 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ImmunizationRecommendation> retVal; 1462 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ImmunizationRecommendation>(); 1463 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ImmunizationRecommendation.class); 1464 retVal.setContext(myFhirContext); 1465 return retVal; 1466 } 1467 1468 @Bean(name="myImmunizationRecommendationRpDstu2") 1469 @Lazy 1470 public ca.uhn.fhir.jpa.rp.dstu2.ImmunizationRecommendationResourceProvider rpImmunizationRecommendationDstu2() { 1471 ca.uhn.fhir.jpa.rp.dstu2.ImmunizationRecommendationResourceProvider retVal; 1472 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ImmunizationRecommendationResourceProvider(); 1473 retVal.setContext(myFhirContext); 1474 retVal.setDao(daoImmunizationRecommendationDstu2()); 1475 return retVal; 1476 } 1477 1478 @Bean(name="myImplementationGuideDaoDstu2") 1479 public 1480 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ImplementationGuide> 1481 daoImplementationGuideDstu2() { 1482 1483 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ImplementationGuide> retVal; 1484 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ImplementationGuide>(); 1485 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ImplementationGuide.class); 1486 retVal.setContext(myFhirContext); 1487 return retVal; 1488 } 1489 1490 @Bean(name="myImplementationGuideRpDstu2") 1491 @Lazy 1492 public ca.uhn.fhir.jpa.rp.dstu2.ImplementationGuideResourceProvider rpImplementationGuideDstu2() { 1493 ca.uhn.fhir.jpa.rp.dstu2.ImplementationGuideResourceProvider retVal; 1494 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ImplementationGuideResourceProvider(); 1495 retVal.setContext(myFhirContext); 1496 retVal.setDao(daoImplementationGuideDstu2()); 1497 return retVal; 1498 } 1499 1500 @Bean(name="myListDaoDstu2") 1501 public 1502 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ListResource> 1503 daoListResourceDstu2() { 1504 1505 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ListResource> retVal; 1506 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ListResource>(); 1507 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ListResource.class); 1508 retVal.setContext(myFhirContext); 1509 return retVal; 1510 } 1511 1512 @Bean(name="myListResourceRpDstu2") 1513 @Lazy 1514 public ca.uhn.fhir.jpa.rp.dstu2.ListResourceResourceProvider rpListResourceDstu2() { 1515 ca.uhn.fhir.jpa.rp.dstu2.ListResourceResourceProvider retVal; 1516 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ListResourceResourceProvider(); 1517 retVal.setContext(myFhirContext); 1518 retVal.setDao(daoListResourceDstu2()); 1519 return retVal; 1520 } 1521 1522 @Bean(name="myLocationDaoDstu2") 1523 public 1524 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Location> 1525 daoLocationDstu2() { 1526 1527 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Location> retVal; 1528 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Location>(); 1529 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Location.class); 1530 retVal.setContext(myFhirContext); 1531 return retVal; 1532 } 1533 1534 @Bean(name="myLocationRpDstu2") 1535 @Lazy 1536 public ca.uhn.fhir.jpa.rp.dstu2.LocationResourceProvider rpLocationDstu2() { 1537 ca.uhn.fhir.jpa.rp.dstu2.LocationResourceProvider retVal; 1538 retVal = new ca.uhn.fhir.jpa.rp.dstu2.LocationResourceProvider(); 1539 retVal.setContext(myFhirContext); 1540 retVal.setDao(daoLocationDstu2()); 1541 return retVal; 1542 } 1543 1544 @Bean(name="myMediaDaoDstu2") 1545 public 1546 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Media> 1547 daoMediaDstu2() { 1548 1549 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Media> retVal; 1550 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Media>(); 1551 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Media.class); 1552 retVal.setContext(myFhirContext); 1553 return retVal; 1554 } 1555 1556 @Bean(name="myMediaRpDstu2") 1557 @Lazy 1558 public ca.uhn.fhir.jpa.rp.dstu2.MediaResourceProvider rpMediaDstu2() { 1559 ca.uhn.fhir.jpa.rp.dstu2.MediaResourceProvider retVal; 1560 retVal = new ca.uhn.fhir.jpa.rp.dstu2.MediaResourceProvider(); 1561 retVal.setContext(myFhirContext); 1562 retVal.setDao(daoMediaDstu2()); 1563 return retVal; 1564 } 1565 1566 @Bean(name="myMedicationDaoDstu2") 1567 public 1568 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Medication> 1569 daoMedicationDstu2() { 1570 1571 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Medication> retVal; 1572 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Medication>(); 1573 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Medication.class); 1574 retVal.setContext(myFhirContext); 1575 return retVal; 1576 } 1577 1578 @Bean(name="myMedicationRpDstu2") 1579 @Lazy 1580 public ca.uhn.fhir.jpa.rp.dstu2.MedicationResourceProvider rpMedicationDstu2() { 1581 ca.uhn.fhir.jpa.rp.dstu2.MedicationResourceProvider retVal; 1582 retVal = new ca.uhn.fhir.jpa.rp.dstu2.MedicationResourceProvider(); 1583 retVal.setContext(myFhirContext); 1584 retVal.setDao(daoMedicationDstu2()); 1585 return retVal; 1586 } 1587 1588 @Bean(name="myMedicationAdministrationDaoDstu2") 1589 public 1590 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationAdministration> 1591 daoMedicationAdministrationDstu2() { 1592 1593 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationAdministration> retVal; 1594 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationAdministration>(); 1595 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.MedicationAdministration.class); 1596 retVal.setContext(myFhirContext); 1597 return retVal; 1598 } 1599 1600 @Bean(name="myMedicationAdministrationRpDstu2") 1601 @Lazy 1602 public ca.uhn.fhir.jpa.rp.dstu2.MedicationAdministrationResourceProvider rpMedicationAdministrationDstu2() { 1603 ca.uhn.fhir.jpa.rp.dstu2.MedicationAdministrationResourceProvider retVal; 1604 retVal = new ca.uhn.fhir.jpa.rp.dstu2.MedicationAdministrationResourceProvider(); 1605 retVal.setContext(myFhirContext); 1606 retVal.setDao(daoMedicationAdministrationDstu2()); 1607 return retVal; 1608 } 1609 1610 @Bean(name="myMedicationDispenseDaoDstu2") 1611 public 1612 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationDispense> 1613 daoMedicationDispenseDstu2() { 1614 1615 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationDispense> retVal; 1616 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationDispense>(); 1617 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.MedicationDispense.class); 1618 retVal.setContext(myFhirContext); 1619 return retVal; 1620 } 1621 1622 @Bean(name="myMedicationDispenseRpDstu2") 1623 @Lazy 1624 public ca.uhn.fhir.jpa.rp.dstu2.MedicationDispenseResourceProvider rpMedicationDispenseDstu2() { 1625 ca.uhn.fhir.jpa.rp.dstu2.MedicationDispenseResourceProvider retVal; 1626 retVal = new ca.uhn.fhir.jpa.rp.dstu2.MedicationDispenseResourceProvider(); 1627 retVal.setContext(myFhirContext); 1628 retVal.setDao(daoMedicationDispenseDstu2()); 1629 return retVal; 1630 } 1631 1632 @Bean(name="myMedicationOrderDaoDstu2") 1633 public 1634 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationOrder> 1635 daoMedicationOrderDstu2() { 1636 1637 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationOrder> retVal; 1638 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationOrder>(); 1639 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.MedicationOrder.class); 1640 retVal.setContext(myFhirContext); 1641 return retVal; 1642 } 1643 1644 @Bean(name="myMedicationOrderRpDstu2") 1645 @Lazy 1646 public ca.uhn.fhir.jpa.rp.dstu2.MedicationOrderResourceProvider rpMedicationOrderDstu2() { 1647 ca.uhn.fhir.jpa.rp.dstu2.MedicationOrderResourceProvider retVal; 1648 retVal = new ca.uhn.fhir.jpa.rp.dstu2.MedicationOrderResourceProvider(); 1649 retVal.setContext(myFhirContext); 1650 retVal.setDao(daoMedicationOrderDstu2()); 1651 return retVal; 1652 } 1653 1654 @Bean(name="myMedicationStatementDaoDstu2") 1655 public 1656 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationStatement> 1657 daoMedicationStatementDstu2() { 1658 1659 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationStatement> retVal; 1660 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.MedicationStatement>(); 1661 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.MedicationStatement.class); 1662 retVal.setContext(myFhirContext); 1663 return retVal; 1664 } 1665 1666 @Bean(name="myMedicationStatementRpDstu2") 1667 @Lazy 1668 public ca.uhn.fhir.jpa.rp.dstu2.MedicationStatementResourceProvider rpMedicationStatementDstu2() { 1669 ca.uhn.fhir.jpa.rp.dstu2.MedicationStatementResourceProvider retVal; 1670 retVal = new ca.uhn.fhir.jpa.rp.dstu2.MedicationStatementResourceProvider(); 1671 retVal.setContext(myFhirContext); 1672 retVal.setDao(daoMedicationStatementDstu2()); 1673 return retVal; 1674 } 1675 1676 @Bean(name="myMessageHeaderDaoDstu2") 1677 public 1678 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MessageHeader> 1679 daoMessageHeaderDstu2() { 1680 1681 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.MessageHeader> retVal; 1682 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.MessageHeader>(); 1683 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.MessageHeader.class); 1684 retVal.setContext(myFhirContext); 1685 return retVal; 1686 } 1687 1688 @Bean(name="myMessageHeaderRpDstu2") 1689 @Lazy 1690 public ca.uhn.fhir.jpa.rp.dstu2.MessageHeaderResourceProvider rpMessageHeaderDstu2() { 1691 ca.uhn.fhir.jpa.rp.dstu2.MessageHeaderResourceProvider retVal; 1692 retVal = new ca.uhn.fhir.jpa.rp.dstu2.MessageHeaderResourceProvider(); 1693 retVal.setContext(myFhirContext); 1694 retVal.setDao(daoMessageHeaderDstu2()); 1695 return retVal; 1696 } 1697 1698 @Bean(name="myNamingSystemDaoDstu2") 1699 public 1700 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.NamingSystem> 1701 daoNamingSystemDstu2() { 1702 1703 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.NamingSystem> retVal; 1704 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.NamingSystem>(); 1705 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.NamingSystem.class); 1706 retVal.setContext(myFhirContext); 1707 return retVal; 1708 } 1709 1710 @Bean(name="myNamingSystemRpDstu2") 1711 @Lazy 1712 public ca.uhn.fhir.jpa.rp.dstu2.NamingSystemResourceProvider rpNamingSystemDstu2() { 1713 ca.uhn.fhir.jpa.rp.dstu2.NamingSystemResourceProvider retVal; 1714 retVal = new ca.uhn.fhir.jpa.rp.dstu2.NamingSystemResourceProvider(); 1715 retVal.setContext(myFhirContext); 1716 retVal.setDao(daoNamingSystemDstu2()); 1717 return retVal; 1718 } 1719 1720 @Bean(name="myNutritionOrderDaoDstu2") 1721 public 1722 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.NutritionOrder> 1723 daoNutritionOrderDstu2() { 1724 1725 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.NutritionOrder> retVal; 1726 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.NutritionOrder>(); 1727 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.NutritionOrder.class); 1728 retVal.setContext(myFhirContext); 1729 return retVal; 1730 } 1731 1732 @Bean(name="myNutritionOrderRpDstu2") 1733 @Lazy 1734 public ca.uhn.fhir.jpa.rp.dstu2.NutritionOrderResourceProvider rpNutritionOrderDstu2() { 1735 ca.uhn.fhir.jpa.rp.dstu2.NutritionOrderResourceProvider retVal; 1736 retVal = new ca.uhn.fhir.jpa.rp.dstu2.NutritionOrderResourceProvider(); 1737 retVal.setContext(myFhirContext); 1738 retVal.setDao(daoNutritionOrderDstu2()); 1739 return retVal; 1740 } 1741 1742 @Bean(name="myObservationDaoDstu2") 1743 public 1744 IFhirResourceDaoObservation<ca.uhn.fhir.model.dstu2.resource.Observation> 1745 daoObservationDstu2() { 1746 1747 ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<ca.uhn.fhir.model.dstu2.resource.Observation> retVal; 1748 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<>(); 1749 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Observation.class); 1750 retVal.setContext(myFhirContext); 1751 return retVal; 1752 } 1753 1754 @Bean(name="myObservationRpDstu2") 1755 @Lazy 1756 public ca.uhn.fhir.jpa.rp.dstu2.ObservationResourceProvider rpObservationDstu2() { 1757 ca.uhn.fhir.jpa.rp.dstu2.ObservationResourceProvider retVal; 1758 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ObservationResourceProvider(); 1759 retVal.setContext(myFhirContext); 1760 retVal.setDao(daoObservationDstu2()); 1761 return retVal; 1762 } 1763 1764 @Bean(name="myOperationDefinitionDaoDstu2") 1765 public 1766 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.OperationDefinition> 1767 daoOperationDefinitionDstu2() { 1768 1769 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.OperationDefinition> retVal; 1770 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.OperationDefinition>(); 1771 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.OperationDefinition.class); 1772 retVal.setContext(myFhirContext); 1773 return retVal; 1774 } 1775 1776 @Bean(name="myOperationDefinitionRpDstu2") 1777 @Lazy 1778 public ca.uhn.fhir.jpa.rp.dstu2.OperationDefinitionResourceProvider rpOperationDefinitionDstu2() { 1779 ca.uhn.fhir.jpa.rp.dstu2.OperationDefinitionResourceProvider retVal; 1780 retVal = new ca.uhn.fhir.jpa.rp.dstu2.OperationDefinitionResourceProvider(); 1781 retVal.setContext(myFhirContext); 1782 retVal.setDao(daoOperationDefinitionDstu2()); 1783 return retVal; 1784 } 1785 1786 @Bean(name="myOperationOutcomeDaoDstu2") 1787 public 1788 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.OperationOutcome> 1789 daoOperationOutcomeDstu2() { 1790 1791 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.OperationOutcome> retVal; 1792 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.OperationOutcome>(); 1793 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.OperationOutcome.class); 1794 retVal.setContext(myFhirContext); 1795 return retVal; 1796 } 1797 1798 @Bean(name="myOperationOutcomeRpDstu2") 1799 @Lazy 1800 public ca.uhn.fhir.jpa.rp.dstu2.OperationOutcomeResourceProvider rpOperationOutcomeDstu2() { 1801 ca.uhn.fhir.jpa.rp.dstu2.OperationOutcomeResourceProvider retVal; 1802 retVal = new ca.uhn.fhir.jpa.rp.dstu2.OperationOutcomeResourceProvider(); 1803 retVal.setContext(myFhirContext); 1804 retVal.setDao(daoOperationOutcomeDstu2()); 1805 return retVal; 1806 } 1807 1808 @Bean(name="myOrderDaoDstu2") 1809 public 1810 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Order> 1811 daoOrderDstu2() { 1812 1813 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Order> retVal; 1814 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Order>(); 1815 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Order.class); 1816 retVal.setContext(myFhirContext); 1817 return retVal; 1818 } 1819 1820 @Bean(name="myOrderRpDstu2") 1821 @Lazy 1822 public ca.uhn.fhir.jpa.rp.dstu2.OrderResourceProvider rpOrderDstu2() { 1823 ca.uhn.fhir.jpa.rp.dstu2.OrderResourceProvider retVal; 1824 retVal = new ca.uhn.fhir.jpa.rp.dstu2.OrderResourceProvider(); 1825 retVal.setContext(myFhirContext); 1826 retVal.setDao(daoOrderDstu2()); 1827 return retVal; 1828 } 1829 1830 @Bean(name="myOrderResponseDaoDstu2") 1831 public 1832 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.OrderResponse> 1833 daoOrderResponseDstu2() { 1834 1835 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.OrderResponse> retVal; 1836 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.OrderResponse>(); 1837 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.OrderResponse.class); 1838 retVal.setContext(myFhirContext); 1839 return retVal; 1840 } 1841 1842 @Bean(name="myOrderResponseRpDstu2") 1843 @Lazy 1844 public ca.uhn.fhir.jpa.rp.dstu2.OrderResponseResourceProvider rpOrderResponseDstu2() { 1845 ca.uhn.fhir.jpa.rp.dstu2.OrderResponseResourceProvider retVal; 1846 retVal = new ca.uhn.fhir.jpa.rp.dstu2.OrderResponseResourceProvider(); 1847 retVal.setContext(myFhirContext); 1848 retVal.setDao(daoOrderResponseDstu2()); 1849 return retVal; 1850 } 1851 1852 @Bean(name="myOrganizationDaoDstu2") 1853 public 1854 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Organization> 1855 daoOrganizationDstu2() { 1856 1857 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Organization> retVal; 1858 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Organization>(); 1859 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Organization.class); 1860 retVal.setContext(myFhirContext); 1861 return retVal; 1862 } 1863 1864 @Bean(name="myOrganizationRpDstu2") 1865 @Lazy 1866 public ca.uhn.fhir.jpa.rp.dstu2.OrganizationResourceProvider rpOrganizationDstu2() { 1867 ca.uhn.fhir.jpa.rp.dstu2.OrganizationResourceProvider retVal; 1868 retVal = new ca.uhn.fhir.jpa.rp.dstu2.OrganizationResourceProvider(); 1869 retVal.setContext(myFhirContext); 1870 retVal.setDao(daoOrganizationDstu2()); 1871 return retVal; 1872 } 1873 1874 @Bean(name="myParametersDaoDstu2") 1875 public 1876 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Parameters> 1877 daoParametersDstu2() { 1878 1879 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Parameters> retVal; 1880 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Parameters>(); 1881 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Parameters.class); 1882 retVal.setContext(myFhirContext); 1883 return retVal; 1884 } 1885 1886 @Bean(name="myParametersRpDstu2") 1887 @Lazy 1888 public ca.uhn.fhir.jpa.rp.dstu2.ParametersResourceProvider rpParametersDstu2() { 1889 ca.uhn.fhir.jpa.rp.dstu2.ParametersResourceProvider retVal; 1890 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ParametersResourceProvider(); 1891 retVal.setContext(myFhirContext); 1892 retVal.setDao(daoParametersDstu2()); 1893 return retVal; 1894 } 1895 1896 @Bean(name="myPatientDaoDstu2") 1897 public 1898 IFhirResourceDaoPatient<ca.uhn.fhir.model.dstu2.resource.Patient> 1899 daoPatientDstu2() { 1900 1901 ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<ca.uhn.fhir.model.dstu2.resource.Patient> retVal; 1902 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<>(); 1903 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Patient.class); 1904 retVal.setContext(myFhirContext); 1905 return retVal; 1906 } 1907 1908 @Bean(name="myPatientRpDstu2") 1909 @Lazy 1910 public ca.uhn.fhir.jpa.rp.dstu2.PatientResourceProvider rpPatientDstu2() { 1911 ca.uhn.fhir.jpa.rp.dstu2.PatientResourceProvider retVal; 1912 retVal = new ca.uhn.fhir.jpa.rp.dstu2.PatientResourceProvider(); 1913 retVal.setContext(myFhirContext); 1914 retVal.setDao(daoPatientDstu2()); 1915 return retVal; 1916 } 1917 1918 @Bean(name="myPaymentNoticeDaoDstu2") 1919 public 1920 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.PaymentNotice> 1921 daoPaymentNoticeDstu2() { 1922 1923 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.PaymentNotice> retVal; 1924 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.PaymentNotice>(); 1925 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.PaymentNotice.class); 1926 retVal.setContext(myFhirContext); 1927 return retVal; 1928 } 1929 1930 @Bean(name="myPaymentNoticeRpDstu2") 1931 @Lazy 1932 public ca.uhn.fhir.jpa.rp.dstu2.PaymentNoticeResourceProvider rpPaymentNoticeDstu2() { 1933 ca.uhn.fhir.jpa.rp.dstu2.PaymentNoticeResourceProvider retVal; 1934 retVal = new ca.uhn.fhir.jpa.rp.dstu2.PaymentNoticeResourceProvider(); 1935 retVal.setContext(myFhirContext); 1936 retVal.setDao(daoPaymentNoticeDstu2()); 1937 return retVal; 1938 } 1939 1940 @Bean(name="myPaymentReconciliationDaoDstu2") 1941 public 1942 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.PaymentReconciliation> 1943 daoPaymentReconciliationDstu2() { 1944 1945 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.PaymentReconciliation> retVal; 1946 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.PaymentReconciliation>(); 1947 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.PaymentReconciliation.class); 1948 retVal.setContext(myFhirContext); 1949 return retVal; 1950 } 1951 1952 @Bean(name="myPaymentReconciliationRpDstu2") 1953 @Lazy 1954 public ca.uhn.fhir.jpa.rp.dstu2.PaymentReconciliationResourceProvider rpPaymentReconciliationDstu2() { 1955 ca.uhn.fhir.jpa.rp.dstu2.PaymentReconciliationResourceProvider retVal; 1956 retVal = new ca.uhn.fhir.jpa.rp.dstu2.PaymentReconciliationResourceProvider(); 1957 retVal.setContext(myFhirContext); 1958 retVal.setDao(daoPaymentReconciliationDstu2()); 1959 return retVal; 1960 } 1961 1962 @Bean(name="myPersonDaoDstu2") 1963 public 1964 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Person> 1965 daoPersonDstu2() { 1966 1967 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Person> retVal; 1968 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Person>(); 1969 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Person.class); 1970 retVal.setContext(myFhirContext); 1971 return retVal; 1972 } 1973 1974 @Bean(name="myPersonRpDstu2") 1975 @Lazy 1976 public ca.uhn.fhir.jpa.rp.dstu2.PersonResourceProvider rpPersonDstu2() { 1977 ca.uhn.fhir.jpa.rp.dstu2.PersonResourceProvider retVal; 1978 retVal = new ca.uhn.fhir.jpa.rp.dstu2.PersonResourceProvider(); 1979 retVal.setContext(myFhirContext); 1980 retVal.setDao(daoPersonDstu2()); 1981 return retVal; 1982 } 1983 1984 @Bean(name="myPractitionerDaoDstu2") 1985 public 1986 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Practitioner> 1987 daoPractitionerDstu2() { 1988 1989 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Practitioner> retVal; 1990 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Practitioner>(); 1991 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Practitioner.class); 1992 retVal.setContext(myFhirContext); 1993 return retVal; 1994 } 1995 1996 @Bean(name="myPractitionerRpDstu2") 1997 @Lazy 1998 public ca.uhn.fhir.jpa.rp.dstu2.PractitionerResourceProvider rpPractitionerDstu2() { 1999 ca.uhn.fhir.jpa.rp.dstu2.PractitionerResourceProvider retVal; 2000 retVal = new ca.uhn.fhir.jpa.rp.dstu2.PractitionerResourceProvider(); 2001 retVal.setContext(myFhirContext); 2002 retVal.setDao(daoPractitionerDstu2()); 2003 return retVal; 2004 } 2005 2006 @Bean(name="myProcedureDaoDstu2") 2007 public 2008 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Procedure> 2009 daoProcedureDstu2() { 2010 2011 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Procedure> retVal; 2012 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Procedure>(); 2013 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Procedure.class); 2014 retVal.setContext(myFhirContext); 2015 return retVal; 2016 } 2017 2018 @Bean(name="myProcedureRpDstu2") 2019 @Lazy 2020 public ca.uhn.fhir.jpa.rp.dstu2.ProcedureResourceProvider rpProcedureDstu2() { 2021 ca.uhn.fhir.jpa.rp.dstu2.ProcedureResourceProvider retVal; 2022 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ProcedureResourceProvider(); 2023 retVal.setContext(myFhirContext); 2024 retVal.setDao(daoProcedureDstu2()); 2025 return retVal; 2026 } 2027 2028 @Bean(name="myProcedureRequestDaoDstu2") 2029 public 2030 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcedureRequest> 2031 daoProcedureRequestDstu2() { 2032 2033 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcedureRequest> retVal; 2034 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcedureRequest>(); 2035 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ProcedureRequest.class); 2036 retVal.setContext(myFhirContext); 2037 return retVal; 2038 } 2039 2040 @Bean(name="myProcedureRequestRpDstu2") 2041 @Lazy 2042 public ca.uhn.fhir.jpa.rp.dstu2.ProcedureRequestResourceProvider rpProcedureRequestDstu2() { 2043 ca.uhn.fhir.jpa.rp.dstu2.ProcedureRequestResourceProvider retVal; 2044 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ProcedureRequestResourceProvider(); 2045 retVal.setContext(myFhirContext); 2046 retVal.setDao(daoProcedureRequestDstu2()); 2047 return retVal; 2048 } 2049 2050 @Bean(name="myProcessRequestDaoDstu2") 2051 public 2052 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcessRequest> 2053 daoProcessRequestDstu2() { 2054 2055 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcessRequest> retVal; 2056 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcessRequest>(); 2057 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ProcessRequest.class); 2058 retVal.setContext(myFhirContext); 2059 return retVal; 2060 } 2061 2062 @Bean(name="myProcessRequestRpDstu2") 2063 @Lazy 2064 public ca.uhn.fhir.jpa.rp.dstu2.ProcessRequestResourceProvider rpProcessRequestDstu2() { 2065 ca.uhn.fhir.jpa.rp.dstu2.ProcessRequestResourceProvider retVal; 2066 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ProcessRequestResourceProvider(); 2067 retVal.setContext(myFhirContext); 2068 retVal.setDao(daoProcessRequestDstu2()); 2069 return retVal; 2070 } 2071 2072 @Bean(name="myProcessResponseDaoDstu2") 2073 public 2074 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcessResponse> 2075 daoProcessResponseDstu2() { 2076 2077 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcessResponse> retVal; 2078 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ProcessResponse>(); 2079 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ProcessResponse.class); 2080 retVal.setContext(myFhirContext); 2081 return retVal; 2082 } 2083 2084 @Bean(name="myProcessResponseRpDstu2") 2085 @Lazy 2086 public ca.uhn.fhir.jpa.rp.dstu2.ProcessResponseResourceProvider rpProcessResponseDstu2() { 2087 ca.uhn.fhir.jpa.rp.dstu2.ProcessResponseResourceProvider retVal; 2088 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ProcessResponseResourceProvider(); 2089 retVal.setContext(myFhirContext); 2090 retVal.setDao(daoProcessResponseDstu2()); 2091 return retVal; 2092 } 2093 2094 @Bean(name="myProvenanceDaoDstu2") 2095 public 2096 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Provenance> 2097 daoProvenanceDstu2() { 2098 2099 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Provenance> retVal; 2100 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Provenance>(); 2101 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Provenance.class); 2102 retVal.setContext(myFhirContext); 2103 return retVal; 2104 } 2105 2106 @Bean(name="myProvenanceRpDstu2") 2107 @Lazy 2108 public ca.uhn.fhir.jpa.rp.dstu2.ProvenanceResourceProvider rpProvenanceDstu2() { 2109 ca.uhn.fhir.jpa.rp.dstu2.ProvenanceResourceProvider retVal; 2110 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ProvenanceResourceProvider(); 2111 retVal.setContext(myFhirContext); 2112 retVal.setDao(daoProvenanceDstu2()); 2113 return retVal; 2114 } 2115 2116 @Bean(name="myQuestionnaireDaoDstu2") 2117 public 2118 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Questionnaire> 2119 daoQuestionnaireDstu2() { 2120 2121 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Questionnaire> retVal; 2122 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Questionnaire>(); 2123 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Questionnaire.class); 2124 retVal.setContext(myFhirContext); 2125 return retVal; 2126 } 2127 2128 @Bean(name="myQuestionnaireRpDstu2") 2129 @Lazy 2130 public ca.uhn.fhir.jpa.rp.dstu2.QuestionnaireResourceProvider rpQuestionnaireDstu2() { 2131 ca.uhn.fhir.jpa.rp.dstu2.QuestionnaireResourceProvider retVal; 2132 retVal = new ca.uhn.fhir.jpa.rp.dstu2.QuestionnaireResourceProvider(); 2133 retVal.setContext(myFhirContext); 2134 retVal.setDao(daoQuestionnaireDstu2()); 2135 return retVal; 2136 } 2137 2138 @Bean(name="myQuestionnaireResponseDaoDstu2") 2139 public 2140 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.QuestionnaireResponse> 2141 daoQuestionnaireResponseDstu2() { 2142 2143 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.QuestionnaireResponse> retVal; 2144 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.QuestionnaireResponse>(); 2145 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.QuestionnaireResponse.class); 2146 retVal.setContext(myFhirContext); 2147 return retVal; 2148 } 2149 2150 @Bean(name="myQuestionnaireResponseRpDstu2") 2151 @Lazy 2152 public ca.uhn.fhir.jpa.rp.dstu2.QuestionnaireResponseResourceProvider rpQuestionnaireResponseDstu2() { 2153 ca.uhn.fhir.jpa.rp.dstu2.QuestionnaireResponseResourceProvider retVal; 2154 retVal = new ca.uhn.fhir.jpa.rp.dstu2.QuestionnaireResponseResourceProvider(); 2155 retVal.setContext(myFhirContext); 2156 retVal.setDao(daoQuestionnaireResponseDstu2()); 2157 return retVal; 2158 } 2159 2160 @Bean(name="myReferralRequestDaoDstu2") 2161 public 2162 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ReferralRequest> 2163 daoReferralRequestDstu2() { 2164 2165 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.ReferralRequest> retVal; 2166 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.ReferralRequest>(); 2167 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ReferralRequest.class); 2168 retVal.setContext(myFhirContext); 2169 return retVal; 2170 } 2171 2172 @Bean(name="myReferralRequestRpDstu2") 2173 @Lazy 2174 public ca.uhn.fhir.jpa.rp.dstu2.ReferralRequestResourceProvider rpReferralRequestDstu2() { 2175 ca.uhn.fhir.jpa.rp.dstu2.ReferralRequestResourceProvider retVal; 2176 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ReferralRequestResourceProvider(); 2177 retVal.setContext(myFhirContext); 2178 retVal.setDao(daoReferralRequestDstu2()); 2179 return retVal; 2180 } 2181 2182 @Bean(name="myRelatedPersonDaoDstu2") 2183 public 2184 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.RelatedPerson> 2185 daoRelatedPersonDstu2() { 2186 2187 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.RelatedPerson> retVal; 2188 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.RelatedPerson>(); 2189 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.RelatedPerson.class); 2190 retVal.setContext(myFhirContext); 2191 return retVal; 2192 } 2193 2194 @Bean(name="myRelatedPersonRpDstu2") 2195 @Lazy 2196 public ca.uhn.fhir.jpa.rp.dstu2.RelatedPersonResourceProvider rpRelatedPersonDstu2() { 2197 ca.uhn.fhir.jpa.rp.dstu2.RelatedPersonResourceProvider retVal; 2198 retVal = new ca.uhn.fhir.jpa.rp.dstu2.RelatedPersonResourceProvider(); 2199 retVal.setContext(myFhirContext); 2200 retVal.setDao(daoRelatedPersonDstu2()); 2201 return retVal; 2202 } 2203 2204 @Bean(name="myRiskAssessmentDaoDstu2") 2205 public 2206 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.RiskAssessment> 2207 daoRiskAssessmentDstu2() { 2208 2209 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.RiskAssessment> retVal; 2210 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.RiskAssessment>(); 2211 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.RiskAssessment.class); 2212 retVal.setContext(myFhirContext); 2213 return retVal; 2214 } 2215 2216 @Bean(name="myRiskAssessmentRpDstu2") 2217 @Lazy 2218 public ca.uhn.fhir.jpa.rp.dstu2.RiskAssessmentResourceProvider rpRiskAssessmentDstu2() { 2219 ca.uhn.fhir.jpa.rp.dstu2.RiskAssessmentResourceProvider retVal; 2220 retVal = new ca.uhn.fhir.jpa.rp.dstu2.RiskAssessmentResourceProvider(); 2221 retVal.setContext(myFhirContext); 2222 retVal.setDao(daoRiskAssessmentDstu2()); 2223 return retVal; 2224 } 2225 2226 @Bean(name="myScheduleDaoDstu2") 2227 public 2228 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Schedule> 2229 daoScheduleDstu2() { 2230 2231 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Schedule> retVal; 2232 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Schedule>(); 2233 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Schedule.class); 2234 retVal.setContext(myFhirContext); 2235 return retVal; 2236 } 2237 2238 @Bean(name="myScheduleRpDstu2") 2239 @Lazy 2240 public ca.uhn.fhir.jpa.rp.dstu2.ScheduleResourceProvider rpScheduleDstu2() { 2241 ca.uhn.fhir.jpa.rp.dstu2.ScheduleResourceProvider retVal; 2242 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ScheduleResourceProvider(); 2243 retVal.setContext(myFhirContext); 2244 retVal.setDao(daoScheduleDstu2()); 2245 return retVal; 2246 } 2247 2248 @Bean(name="mySearchParameterDaoDstu2") 2249 public 2250 IFhirResourceDaoSearchParameter<ca.uhn.fhir.model.dstu2.resource.SearchParameter> 2251 daoSearchParameterDstu2() { 2252 2253 ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<ca.uhn.fhir.model.dstu2.resource.SearchParameter> retVal; 2254 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<>(); 2255 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.SearchParameter.class); 2256 retVal.setContext(myFhirContext); 2257 return retVal; 2258 } 2259 2260 @Bean(name="mySearchParameterRpDstu2") 2261 @Lazy 2262 public ca.uhn.fhir.jpa.rp.dstu2.SearchParameterResourceProvider rpSearchParameterDstu2() { 2263 ca.uhn.fhir.jpa.rp.dstu2.SearchParameterResourceProvider retVal; 2264 retVal = new ca.uhn.fhir.jpa.rp.dstu2.SearchParameterResourceProvider(); 2265 retVal.setContext(myFhirContext); 2266 retVal.setDao(daoSearchParameterDstu2()); 2267 return retVal; 2268 } 2269 2270 @Bean(name="mySlotDaoDstu2") 2271 public 2272 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Slot> 2273 daoSlotDstu2() { 2274 2275 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Slot> retVal; 2276 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Slot>(); 2277 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Slot.class); 2278 retVal.setContext(myFhirContext); 2279 return retVal; 2280 } 2281 2282 @Bean(name="mySlotRpDstu2") 2283 @Lazy 2284 public ca.uhn.fhir.jpa.rp.dstu2.SlotResourceProvider rpSlotDstu2() { 2285 ca.uhn.fhir.jpa.rp.dstu2.SlotResourceProvider retVal; 2286 retVal = new ca.uhn.fhir.jpa.rp.dstu2.SlotResourceProvider(); 2287 retVal.setContext(myFhirContext); 2288 retVal.setDao(daoSlotDstu2()); 2289 return retVal; 2290 } 2291 2292 @Bean(name="mySpecimenDaoDstu2") 2293 public 2294 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Specimen> 2295 daoSpecimenDstu2() { 2296 2297 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Specimen> retVal; 2298 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Specimen>(); 2299 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Specimen.class); 2300 retVal.setContext(myFhirContext); 2301 return retVal; 2302 } 2303 2304 @Bean(name="mySpecimenRpDstu2") 2305 @Lazy 2306 public ca.uhn.fhir.jpa.rp.dstu2.SpecimenResourceProvider rpSpecimenDstu2() { 2307 ca.uhn.fhir.jpa.rp.dstu2.SpecimenResourceProvider retVal; 2308 retVal = new ca.uhn.fhir.jpa.rp.dstu2.SpecimenResourceProvider(); 2309 retVal.setContext(myFhirContext); 2310 retVal.setDao(daoSpecimenDstu2()); 2311 return retVal; 2312 } 2313 2314 @Bean(name="myStructureDefinitionDaoDstu2") 2315 public 2316 IFhirResourceDaoStructureDefinition<ca.uhn.fhir.model.dstu2.resource.StructureDefinition> 2317 daoStructureDefinitionDstu2() { 2318 2319 ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<ca.uhn.fhir.model.dstu2.resource.StructureDefinition> retVal; 2320 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<>(); 2321 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.StructureDefinition.class); 2322 retVal.setContext(myFhirContext); 2323 return retVal; 2324 } 2325 2326 @Bean(name="myStructureDefinitionRpDstu2") 2327 @Lazy 2328 public ca.uhn.fhir.jpa.rp.dstu2.StructureDefinitionResourceProvider rpStructureDefinitionDstu2() { 2329 ca.uhn.fhir.jpa.rp.dstu2.StructureDefinitionResourceProvider retVal; 2330 retVal = new ca.uhn.fhir.jpa.rp.dstu2.StructureDefinitionResourceProvider(); 2331 retVal.setContext(myFhirContext); 2332 retVal.setDao(daoStructureDefinitionDstu2()); 2333 return retVal; 2334 } 2335 2336 @Bean(name="mySubscriptionDaoDstu2") 2337 public 2338 IFhirResourceDaoSubscription<ca.uhn.fhir.model.dstu2.resource.Subscription> 2339 daoSubscriptionDstu2() { 2340 2341 ca.uhn.fhir.jpa.dao.FhirResourceDaoSubscriptionDstu2 retVal; 2342 retVal = new ca.uhn.fhir.jpa.dao.FhirResourceDaoSubscriptionDstu2(); 2343 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Subscription.class); 2344 retVal.setContext(myFhirContext); 2345 return retVal; 2346 } 2347 2348 @Bean(name="mySubscriptionRpDstu2") 2349 @Lazy 2350 public ca.uhn.fhir.jpa.rp.dstu2.SubscriptionResourceProvider rpSubscriptionDstu2() { 2351 ca.uhn.fhir.jpa.rp.dstu2.SubscriptionResourceProvider retVal; 2352 retVal = new ca.uhn.fhir.jpa.rp.dstu2.SubscriptionResourceProvider(); 2353 retVal.setContext(myFhirContext); 2354 retVal.setDao(daoSubscriptionDstu2()); 2355 return retVal; 2356 } 2357 2358 @Bean(name="mySubstanceDaoDstu2") 2359 public 2360 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Substance> 2361 daoSubstanceDstu2() { 2362 2363 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.Substance> retVal; 2364 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.Substance>(); 2365 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.Substance.class); 2366 retVal.setContext(myFhirContext); 2367 return retVal; 2368 } 2369 2370 @Bean(name="mySubstanceRpDstu2") 2371 @Lazy 2372 public ca.uhn.fhir.jpa.rp.dstu2.SubstanceResourceProvider rpSubstanceDstu2() { 2373 ca.uhn.fhir.jpa.rp.dstu2.SubstanceResourceProvider retVal; 2374 retVal = new ca.uhn.fhir.jpa.rp.dstu2.SubstanceResourceProvider(); 2375 retVal.setContext(myFhirContext); 2376 retVal.setDao(daoSubstanceDstu2()); 2377 return retVal; 2378 } 2379 2380 @Bean(name="mySupplyDeliveryDaoDstu2") 2381 public 2382 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.SupplyDelivery> 2383 daoSupplyDeliveryDstu2() { 2384 2385 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.SupplyDelivery> retVal; 2386 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.SupplyDelivery>(); 2387 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.SupplyDelivery.class); 2388 retVal.setContext(myFhirContext); 2389 return retVal; 2390 } 2391 2392 @Bean(name="mySupplyDeliveryRpDstu2") 2393 @Lazy 2394 public ca.uhn.fhir.jpa.rp.dstu2.SupplyDeliveryResourceProvider rpSupplyDeliveryDstu2() { 2395 ca.uhn.fhir.jpa.rp.dstu2.SupplyDeliveryResourceProvider retVal; 2396 retVal = new ca.uhn.fhir.jpa.rp.dstu2.SupplyDeliveryResourceProvider(); 2397 retVal.setContext(myFhirContext); 2398 retVal.setDao(daoSupplyDeliveryDstu2()); 2399 return retVal; 2400 } 2401 2402 @Bean(name="mySupplyRequestDaoDstu2") 2403 public 2404 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.SupplyRequest> 2405 daoSupplyRequestDstu2() { 2406 2407 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.SupplyRequest> retVal; 2408 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.SupplyRequest>(); 2409 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.SupplyRequest.class); 2410 retVal.setContext(myFhirContext); 2411 return retVal; 2412 } 2413 2414 @Bean(name="mySupplyRequestRpDstu2") 2415 @Lazy 2416 public ca.uhn.fhir.jpa.rp.dstu2.SupplyRequestResourceProvider rpSupplyRequestDstu2() { 2417 ca.uhn.fhir.jpa.rp.dstu2.SupplyRequestResourceProvider retVal; 2418 retVal = new ca.uhn.fhir.jpa.rp.dstu2.SupplyRequestResourceProvider(); 2419 retVal.setContext(myFhirContext); 2420 retVal.setDao(daoSupplyRequestDstu2()); 2421 return retVal; 2422 } 2423 2424 @Bean(name="myTestScriptDaoDstu2") 2425 public 2426 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.TestScript> 2427 daoTestScriptDstu2() { 2428 2429 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.TestScript> retVal; 2430 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.TestScript>(); 2431 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.TestScript.class); 2432 retVal.setContext(myFhirContext); 2433 return retVal; 2434 } 2435 2436 @Bean(name="myTestScriptRpDstu2") 2437 @Lazy 2438 public ca.uhn.fhir.jpa.rp.dstu2.TestScriptResourceProvider rpTestScriptDstu2() { 2439 ca.uhn.fhir.jpa.rp.dstu2.TestScriptResourceProvider retVal; 2440 retVal = new ca.uhn.fhir.jpa.rp.dstu2.TestScriptResourceProvider(); 2441 retVal.setContext(myFhirContext); 2442 retVal.setDao(daoTestScriptDstu2()); 2443 return retVal; 2444 } 2445 2446 @Bean(name="myValueSetDaoDstu2") 2447 public 2448 IFhirResourceDaoValueSet<ca.uhn.fhir.model.dstu2.resource.ValueSet> 2449 daoValueSetDstu2() { 2450 2451 ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<ca.uhn.fhir.model.dstu2.resource.ValueSet> retVal; 2452 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<>(); 2453 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.ValueSet.class); 2454 retVal.setContext(myFhirContext); 2455 return retVal; 2456 } 2457 2458 @Bean(name="myValueSetRpDstu2") 2459 @Lazy 2460 public ca.uhn.fhir.jpa.rp.dstu2.ValueSetResourceProvider rpValueSetDstu2() { 2461 ca.uhn.fhir.jpa.rp.dstu2.ValueSetResourceProvider retVal; 2462 retVal = new ca.uhn.fhir.jpa.rp.dstu2.ValueSetResourceProvider(); 2463 retVal.setContext(myFhirContext); 2464 retVal.setDao(daoValueSetDstu2()); 2465 return retVal; 2466 } 2467 2468 @Bean(name="myVisionPrescriptionDaoDstu2") 2469 public 2470 IFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.VisionPrescription> 2471 daoVisionPrescriptionDstu2() { 2472 2473 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<ca.uhn.fhir.model.dstu2.resource.VisionPrescription> retVal; 2474 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<ca.uhn.fhir.model.dstu2.resource.VisionPrescription>(); 2475 retVal.setResourceType(ca.uhn.fhir.model.dstu2.resource.VisionPrescription.class); 2476 retVal.setContext(myFhirContext); 2477 return retVal; 2478 } 2479 2480 @Bean(name="myVisionPrescriptionRpDstu2") 2481 @Lazy 2482 public ca.uhn.fhir.jpa.rp.dstu2.VisionPrescriptionResourceProvider rpVisionPrescriptionDstu2() { 2483 ca.uhn.fhir.jpa.rp.dstu2.VisionPrescriptionResourceProvider retVal; 2484 retVal = new ca.uhn.fhir.jpa.rp.dstu2.VisionPrescriptionResourceProvider(); 2485 retVal.setContext(myFhirContext); 2486 retVal.setDao(daoVisionPrescriptionDstu2()); 2487 return retVal; 2488 } 2489 2490 2491 2492 2493 2494 2495}