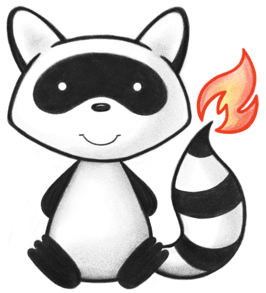
001package ca.uhn.fhir.jpa.config; 002 003import java.util.ArrayList; 004import java.util.List; 005import java.util.concurrent.Executor; 006import java.util.concurrent.Executors; 007 008import org.springframework.transaction.PlatformTransactionManager; 009import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 010 011import org.springframework.beans.factory.annotation.Autowired; 012import org.springframework.context.annotation.Bean; 013import org.springframework.context.annotation.Configuration; 014import org.springframework.context.annotation.Lazy; 015import org.springframework.data.jpa.repository.config.EnableJpaRepositories; 016import org.springframework.scheduling.annotation.EnableScheduling; 017import org.springframework.scheduling.annotation.SchedulingConfigurer; 018import org.springframework.scheduling.config.ScheduledTaskRegistrar; 019 020import ca.uhn.fhir.rest.api.IResourceSupportedSvc; 021import ca.uhn.fhir.context.FhirContext; 022import ca.uhn.fhir.model.api.IResource; 023import ca.uhn.fhir.rest.server.IResourceProvider; 024import ca.uhn.fhir.jpa.api.dao.*; 025import ca.uhn.fhir.jpa.dao.*; 026import ca.uhn.fhir.rest.server.provider.ResourceProviderFactory; 027 028@Configuration 029public class GeneratedDaoAndResourceProviderConfigDstu3 { 030@Autowired 031FhirContext myFhirContext; 032 033 @Bean(name="myResourceProvidersDstu3") 034 public ResourceProviderFactory resourceProvidersDstu3(IResourceSupportedSvc theResourceSupportedSvc) { 035 ResourceProviderFactory retVal = new ResourceProviderFactory(); 036 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Account") ? rpAccountDstu3() : null); 037 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ActivityDefinition") ? rpActivityDefinitionDstu3() : null); 038 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AdverseEvent") ? rpAdverseEventDstu3() : null); 039 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AllergyIntolerance") ? rpAllergyIntoleranceDstu3() : null); 040 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Appointment") ? rpAppointmentDstu3() : null); 041 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AppointmentResponse") ? rpAppointmentResponseDstu3() : null); 042 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AuditEvent") ? rpAuditEventDstu3() : null); 043 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Basic") ? rpBasicDstu3() : null); 044 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Binary") ? rpBinaryDstu3() : null); 045 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BodySite") ? rpBodySiteDstu3() : null); 046 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Bundle") ? rpBundleDstu3() : null); 047 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CapabilityStatement") ? rpCapabilityStatementDstu3() : null); 048 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CarePlan") ? rpCarePlanDstu3() : null); 049 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CareTeam") ? rpCareTeamDstu3() : null); 050 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ChargeItem") ? rpChargeItemDstu3() : null); 051 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Claim") ? rpClaimDstu3() : null); 052 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClaimResponse") ? rpClaimResponseDstu3() : null); 053 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClinicalImpression") ? rpClinicalImpressionDstu3() : null); 054 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CodeSystem") ? rpCodeSystemDstu3() : null); 055 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Communication") ? rpCommunicationDstu3() : null); 056 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CommunicationRequest") ? rpCommunicationRequestDstu3() : null); 057 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CompartmentDefinition") ? rpCompartmentDefinitionDstu3() : null); 058 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Composition") ? rpCompositionDstu3() : null); 059 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ConceptMap") ? rpConceptMapDstu3() : null); 060 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Condition") ? rpConditionDstu3() : null); 061 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Consent") ? rpConsentDstu3() : null); 062 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Contract") ? rpContractDstu3() : null); 063 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Coverage") ? rpCoverageDstu3() : null); 064 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DataElement") ? rpDataElementDstu3() : null); 065 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DetectedIssue") ? rpDetectedIssueDstu3() : null); 066 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Device") ? rpDeviceDstu3() : null); 067 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceComponent") ? rpDeviceComponentDstu3() : null); 068 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceMetric") ? rpDeviceMetricDstu3() : null); 069 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceRequest") ? rpDeviceRequestDstu3() : null); 070 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceUseStatement") ? rpDeviceUseStatementDstu3() : null); 071 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DiagnosticReport") ? rpDiagnosticReportDstu3() : null); 072 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentManifest") ? rpDocumentManifestDstu3() : null); 073 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentReference") ? rpDocumentReferenceDstu3() : null); 074 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EligibilityRequest") ? rpEligibilityRequestDstu3() : null); 075 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EligibilityResponse") ? rpEligibilityResponseDstu3() : null); 076 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Encounter") ? rpEncounterDstu3() : null); 077 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Endpoint") ? rpEndpointDstu3() : null); 078 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentRequest") ? rpEnrollmentRequestDstu3() : null); 079 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentResponse") ? rpEnrollmentResponseDstu3() : null); 080 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EpisodeOfCare") ? rpEpisodeOfCareDstu3() : null); 081 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExpansionProfile") ? rpExpansionProfileDstu3() : null); 082 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExplanationOfBenefit") ? rpExplanationOfBenefitDstu3() : null); 083 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("FamilyMemberHistory") ? rpFamilyMemberHistoryDstu3() : null); 084 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Flag") ? rpFlagDstu3() : null); 085 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Goal") ? rpGoalDstu3() : null); 086 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GraphDefinition") ? rpGraphDefinitionDstu3() : null); 087 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Group") ? rpGroupDstu3() : null); 088 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GuidanceResponse") ? rpGuidanceResponseDstu3() : null); 089 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("HealthcareService") ? rpHealthcareServiceDstu3() : null); 090 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImagingManifest") ? rpImagingManifestDstu3() : null); 091 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImagingStudy") ? rpImagingStudyDstu3() : null); 092 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Immunization") ? rpImmunizationDstu3() : null); 093 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImmunizationRecommendation") ? rpImmunizationRecommendationDstu3() : null); 094 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImplementationGuide") ? rpImplementationGuideDstu3() : null); 095 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Library") ? rpLibraryDstu3() : null); 096 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Linkage") ? rpLinkageDstu3() : null); 097 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("List") ? rpListResourceDstu3() : null); 098 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Location") ? rpLocationDstu3() : null); 099 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Measure") ? rpMeasureDstu3() : null); 100 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MeasureReport") ? rpMeasureReportDstu3() : null); 101 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Media") ? rpMediaDstu3() : null); 102 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Medication") ? rpMedicationDstu3() : null); 103 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationAdministration") ? rpMedicationAdministrationDstu3() : null); 104 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationDispense") ? rpMedicationDispenseDstu3() : null); 105 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationRequest") ? rpMedicationRequestDstu3() : null); 106 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationStatement") ? rpMedicationStatementDstu3() : null); 107 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageDefinition") ? rpMessageDefinitionDstu3() : null); 108 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageHeader") ? rpMessageHeaderDstu3() : null); 109 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NamingSystem") ? rpNamingSystemDstu3() : null); 110 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NutritionOrder") ? rpNutritionOrderDstu3() : null); 111 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Observation") ? rpObservationDstu3() : null); 112 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationDefinition") ? rpOperationDefinitionDstu3() : null); 113 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationOutcome") ? rpOperationOutcomeDstu3() : null); 114 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Organization") ? rpOrganizationDstu3() : null); 115 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Parameters") ? rpParametersDstu3() : null); 116 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Patient") ? rpPatientDstu3() : null); 117 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentNotice") ? rpPaymentNoticeDstu3() : null); 118 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentReconciliation") ? rpPaymentReconciliationDstu3() : null); 119 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Person") ? rpPersonDstu3() : null); 120 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PlanDefinition") ? rpPlanDefinitionDstu3() : null); 121 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Practitioner") ? rpPractitionerDstu3() : null); 122 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PractitionerRole") ? rpPractitionerRoleDstu3() : null); 123 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Procedure") ? rpProcedureDstu3() : null); 124 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ProcedureRequest") ? rpProcedureRequestDstu3() : null); 125 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ProcessRequest") ? rpProcessRequestDstu3() : null); 126 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ProcessResponse") ? rpProcessResponseDstu3() : null); 127 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Provenance") ? rpProvenanceDstu3() : null); 128 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Questionnaire") ? rpQuestionnaireDstu3() : null); 129 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("QuestionnaireResponse") ? rpQuestionnaireResponseDstu3() : null); 130 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ReferralRequest") ? rpReferralRequestDstu3() : null); 131 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RelatedPerson") ? rpRelatedPersonDstu3() : null); 132 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RequestGroup") ? rpRequestGroupDstu3() : null); 133 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchStudy") ? rpResearchStudyDstu3() : null); 134 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchSubject") ? rpResearchSubjectDstu3() : null); 135 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RiskAssessment") ? rpRiskAssessmentDstu3() : null); 136 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Schedule") ? rpScheduleDstu3() : null); 137 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SearchParameter") ? rpSearchParameterDstu3() : null); 138 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Sequence") ? rpSequenceDstu3() : null); 139 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ServiceDefinition") ? rpServiceDefinitionDstu3() : null); 140 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Slot") ? rpSlotDstu3() : null); 141 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Specimen") ? rpSpecimenDstu3() : null); 142 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureDefinition") ? rpStructureDefinitionDstu3() : null); 143 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureMap") ? rpStructureMapDstu3() : null); 144 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Subscription") ? rpSubscriptionDstu3() : null); 145 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Substance") ? rpSubstanceDstu3() : null); 146 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyDelivery") ? rpSupplyDeliveryDstu3() : null); 147 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyRequest") ? rpSupplyRequestDstu3() : null); 148 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Task") ? rpTaskDstu3() : null); 149 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestReport") ? rpTestReportDstu3() : null); 150 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestScript") ? rpTestScriptDstu3() : null); 151 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ValueSet") ? rpValueSetDstu3() : null); 152 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("VisionPrescription") ? rpVisionPrescriptionDstu3() : null); 153 return retVal; 154 } 155 156 157 @Bean(name="myResourceDaosDstu3") 158 public List<IFhirResourceDao<?>> resourceDaosDstu3(IResourceSupportedSvc theResourceSupportedSvc) { 159 List<IFhirResourceDao<?>> retVal = new ArrayList<IFhirResourceDao<?>>(); 160 if (theResourceSupportedSvc.isSupported("Account")) { 161 retVal.add(daoAccountDstu3()); 162 } 163 if (theResourceSupportedSvc.isSupported("ActivityDefinition")) { 164 retVal.add(daoActivityDefinitionDstu3()); 165 } 166 if (theResourceSupportedSvc.isSupported("AdverseEvent")) { 167 retVal.add(daoAdverseEventDstu3()); 168 } 169 if (theResourceSupportedSvc.isSupported("AllergyIntolerance")) { 170 retVal.add(daoAllergyIntoleranceDstu3()); 171 } 172 if (theResourceSupportedSvc.isSupported("Appointment")) { 173 retVal.add(daoAppointmentDstu3()); 174 } 175 if (theResourceSupportedSvc.isSupported("AppointmentResponse")) { 176 retVal.add(daoAppointmentResponseDstu3()); 177 } 178 if (theResourceSupportedSvc.isSupported("AuditEvent")) { 179 retVal.add(daoAuditEventDstu3()); 180 } 181 if (theResourceSupportedSvc.isSupported("Basic")) { 182 retVal.add(daoBasicDstu3()); 183 } 184 if (theResourceSupportedSvc.isSupported("Binary")) { 185 retVal.add(daoBinaryDstu3()); 186 } 187 if (theResourceSupportedSvc.isSupported("BodySite")) { 188 retVal.add(daoBodySiteDstu3()); 189 } 190 if (theResourceSupportedSvc.isSupported("Bundle")) { 191 retVal.add(daoBundleDstu3()); 192 } 193 if (theResourceSupportedSvc.isSupported("CapabilityStatement")) { 194 retVal.add(daoCapabilityStatementDstu3()); 195 } 196 if (theResourceSupportedSvc.isSupported("CarePlan")) { 197 retVal.add(daoCarePlanDstu3()); 198 } 199 if (theResourceSupportedSvc.isSupported("CareTeam")) { 200 retVal.add(daoCareTeamDstu3()); 201 } 202 if (theResourceSupportedSvc.isSupported("ChargeItem")) { 203 retVal.add(daoChargeItemDstu3()); 204 } 205 if (theResourceSupportedSvc.isSupported("Claim")) { 206 retVal.add(daoClaimDstu3()); 207 } 208 if (theResourceSupportedSvc.isSupported("ClaimResponse")) { 209 retVal.add(daoClaimResponseDstu3()); 210 } 211 if (theResourceSupportedSvc.isSupported("ClinicalImpression")) { 212 retVal.add(daoClinicalImpressionDstu3()); 213 } 214 if (theResourceSupportedSvc.isSupported("CodeSystem")) { 215 retVal.add(daoCodeSystemDstu3()); 216 } 217 if (theResourceSupportedSvc.isSupported("Communication")) { 218 retVal.add(daoCommunicationDstu3()); 219 } 220 if (theResourceSupportedSvc.isSupported("CommunicationRequest")) { 221 retVal.add(daoCommunicationRequestDstu3()); 222 } 223 if (theResourceSupportedSvc.isSupported("CompartmentDefinition")) { 224 retVal.add(daoCompartmentDefinitionDstu3()); 225 } 226 if (theResourceSupportedSvc.isSupported("Composition")) { 227 retVal.add(daoCompositionDstu3()); 228 } 229 if (theResourceSupportedSvc.isSupported("ConceptMap")) { 230 retVal.add(daoConceptMapDstu3()); 231 } 232 if (theResourceSupportedSvc.isSupported("Condition")) { 233 retVal.add(daoConditionDstu3()); 234 } 235 if (theResourceSupportedSvc.isSupported("Consent")) { 236 retVal.add(daoConsentDstu3()); 237 } 238 if (theResourceSupportedSvc.isSupported("Contract")) { 239 retVal.add(daoContractDstu3()); 240 } 241 if (theResourceSupportedSvc.isSupported("Coverage")) { 242 retVal.add(daoCoverageDstu3()); 243 } 244 if (theResourceSupportedSvc.isSupported("DataElement")) { 245 retVal.add(daoDataElementDstu3()); 246 } 247 if (theResourceSupportedSvc.isSupported("DetectedIssue")) { 248 retVal.add(daoDetectedIssueDstu3()); 249 } 250 if (theResourceSupportedSvc.isSupported("Device")) { 251 retVal.add(daoDeviceDstu3()); 252 } 253 if (theResourceSupportedSvc.isSupported("DeviceComponent")) { 254 retVal.add(daoDeviceComponentDstu3()); 255 } 256 if (theResourceSupportedSvc.isSupported("DeviceMetric")) { 257 retVal.add(daoDeviceMetricDstu3()); 258 } 259 if (theResourceSupportedSvc.isSupported("DeviceRequest")) { 260 retVal.add(daoDeviceRequestDstu3()); 261 } 262 if (theResourceSupportedSvc.isSupported("DeviceUseStatement")) { 263 retVal.add(daoDeviceUseStatementDstu3()); 264 } 265 if (theResourceSupportedSvc.isSupported("DiagnosticReport")) { 266 retVal.add(daoDiagnosticReportDstu3()); 267 } 268 if (theResourceSupportedSvc.isSupported("DocumentManifest")) { 269 retVal.add(daoDocumentManifestDstu3()); 270 } 271 if (theResourceSupportedSvc.isSupported("DocumentReference")) { 272 retVal.add(daoDocumentReferenceDstu3()); 273 } 274 if (theResourceSupportedSvc.isSupported("EligibilityRequest")) { 275 retVal.add(daoEligibilityRequestDstu3()); 276 } 277 if (theResourceSupportedSvc.isSupported("EligibilityResponse")) { 278 retVal.add(daoEligibilityResponseDstu3()); 279 } 280 if (theResourceSupportedSvc.isSupported("Encounter")) { 281 retVal.add(daoEncounterDstu3()); 282 } 283 if (theResourceSupportedSvc.isSupported("Endpoint")) { 284 retVal.add(daoEndpointDstu3()); 285 } 286 if (theResourceSupportedSvc.isSupported("EnrollmentRequest")) { 287 retVal.add(daoEnrollmentRequestDstu3()); 288 } 289 if (theResourceSupportedSvc.isSupported("EnrollmentResponse")) { 290 retVal.add(daoEnrollmentResponseDstu3()); 291 } 292 if (theResourceSupportedSvc.isSupported("EpisodeOfCare")) { 293 retVal.add(daoEpisodeOfCareDstu3()); 294 } 295 if (theResourceSupportedSvc.isSupported("ExpansionProfile")) { 296 retVal.add(daoExpansionProfileDstu3()); 297 } 298 if (theResourceSupportedSvc.isSupported("ExplanationOfBenefit")) { 299 retVal.add(daoExplanationOfBenefitDstu3()); 300 } 301 if (theResourceSupportedSvc.isSupported("FamilyMemberHistory")) { 302 retVal.add(daoFamilyMemberHistoryDstu3()); 303 } 304 if (theResourceSupportedSvc.isSupported("Flag")) { 305 retVal.add(daoFlagDstu3()); 306 } 307 if (theResourceSupportedSvc.isSupported("Goal")) { 308 retVal.add(daoGoalDstu3()); 309 } 310 if (theResourceSupportedSvc.isSupported("GraphDefinition")) { 311 retVal.add(daoGraphDefinitionDstu3()); 312 } 313 if (theResourceSupportedSvc.isSupported("Group")) { 314 retVal.add(daoGroupDstu3()); 315 } 316 if (theResourceSupportedSvc.isSupported("GuidanceResponse")) { 317 retVal.add(daoGuidanceResponseDstu3()); 318 } 319 if (theResourceSupportedSvc.isSupported("HealthcareService")) { 320 retVal.add(daoHealthcareServiceDstu3()); 321 } 322 if (theResourceSupportedSvc.isSupported("ImagingManifest")) { 323 retVal.add(daoImagingManifestDstu3()); 324 } 325 if (theResourceSupportedSvc.isSupported("ImagingStudy")) { 326 retVal.add(daoImagingStudyDstu3()); 327 } 328 if (theResourceSupportedSvc.isSupported("Immunization")) { 329 retVal.add(daoImmunizationDstu3()); 330 } 331 if (theResourceSupportedSvc.isSupported("ImmunizationRecommendation")) { 332 retVal.add(daoImmunizationRecommendationDstu3()); 333 } 334 if (theResourceSupportedSvc.isSupported("ImplementationGuide")) { 335 retVal.add(daoImplementationGuideDstu3()); 336 } 337 if (theResourceSupportedSvc.isSupported("Library")) { 338 retVal.add(daoLibraryDstu3()); 339 } 340 if (theResourceSupportedSvc.isSupported("Linkage")) { 341 retVal.add(daoLinkageDstu3()); 342 } 343 if (theResourceSupportedSvc.isSupported("List")) { 344 retVal.add(daoListResourceDstu3()); 345 } 346 if (theResourceSupportedSvc.isSupported("Location")) { 347 retVal.add(daoLocationDstu3()); 348 } 349 if (theResourceSupportedSvc.isSupported("Measure")) { 350 retVal.add(daoMeasureDstu3()); 351 } 352 if (theResourceSupportedSvc.isSupported("MeasureReport")) { 353 retVal.add(daoMeasureReportDstu3()); 354 } 355 if (theResourceSupportedSvc.isSupported("Media")) { 356 retVal.add(daoMediaDstu3()); 357 } 358 if (theResourceSupportedSvc.isSupported("Medication")) { 359 retVal.add(daoMedicationDstu3()); 360 } 361 if (theResourceSupportedSvc.isSupported("MedicationAdministration")) { 362 retVal.add(daoMedicationAdministrationDstu3()); 363 } 364 if (theResourceSupportedSvc.isSupported("MedicationDispense")) { 365 retVal.add(daoMedicationDispenseDstu3()); 366 } 367 if (theResourceSupportedSvc.isSupported("MedicationRequest")) { 368 retVal.add(daoMedicationRequestDstu3()); 369 } 370 if (theResourceSupportedSvc.isSupported("MedicationStatement")) { 371 retVal.add(daoMedicationStatementDstu3()); 372 } 373 if (theResourceSupportedSvc.isSupported("MessageDefinition")) { 374 retVal.add(daoMessageDefinitionDstu3()); 375 } 376 if (theResourceSupportedSvc.isSupported("MessageHeader")) { 377 retVal.add(daoMessageHeaderDstu3()); 378 } 379 if (theResourceSupportedSvc.isSupported("NamingSystem")) { 380 retVal.add(daoNamingSystemDstu3()); 381 } 382 if (theResourceSupportedSvc.isSupported("NutritionOrder")) { 383 retVal.add(daoNutritionOrderDstu3()); 384 } 385 if (theResourceSupportedSvc.isSupported("Observation")) { 386 retVal.add(daoObservationDstu3()); 387 } 388 if (theResourceSupportedSvc.isSupported("OperationDefinition")) { 389 retVal.add(daoOperationDefinitionDstu3()); 390 } 391 if (theResourceSupportedSvc.isSupported("OperationOutcome")) { 392 retVal.add(daoOperationOutcomeDstu3()); 393 } 394 if (theResourceSupportedSvc.isSupported("Organization")) { 395 retVal.add(daoOrganizationDstu3()); 396 } 397 if (theResourceSupportedSvc.isSupported("Parameters")) { 398 retVal.add(daoParametersDstu3()); 399 } 400 if (theResourceSupportedSvc.isSupported("Patient")) { 401 retVal.add(daoPatientDstu3()); 402 } 403 if (theResourceSupportedSvc.isSupported("PaymentNotice")) { 404 retVal.add(daoPaymentNoticeDstu3()); 405 } 406 if (theResourceSupportedSvc.isSupported("PaymentReconciliation")) { 407 retVal.add(daoPaymentReconciliationDstu3()); 408 } 409 if (theResourceSupportedSvc.isSupported("Person")) { 410 retVal.add(daoPersonDstu3()); 411 } 412 if (theResourceSupportedSvc.isSupported("PlanDefinition")) { 413 retVal.add(daoPlanDefinitionDstu3()); 414 } 415 if (theResourceSupportedSvc.isSupported("Practitioner")) { 416 retVal.add(daoPractitionerDstu3()); 417 } 418 if (theResourceSupportedSvc.isSupported("PractitionerRole")) { 419 retVal.add(daoPractitionerRoleDstu3()); 420 } 421 if (theResourceSupportedSvc.isSupported("Procedure")) { 422 retVal.add(daoProcedureDstu3()); 423 } 424 if (theResourceSupportedSvc.isSupported("ProcedureRequest")) { 425 retVal.add(daoProcedureRequestDstu3()); 426 } 427 if (theResourceSupportedSvc.isSupported("ProcessRequest")) { 428 retVal.add(daoProcessRequestDstu3()); 429 } 430 if (theResourceSupportedSvc.isSupported("ProcessResponse")) { 431 retVal.add(daoProcessResponseDstu3()); 432 } 433 if (theResourceSupportedSvc.isSupported("Provenance")) { 434 retVal.add(daoProvenanceDstu3()); 435 } 436 if (theResourceSupportedSvc.isSupported("Questionnaire")) { 437 retVal.add(daoQuestionnaireDstu3()); 438 } 439 if (theResourceSupportedSvc.isSupported("QuestionnaireResponse")) { 440 retVal.add(daoQuestionnaireResponseDstu3()); 441 } 442 if (theResourceSupportedSvc.isSupported("ReferralRequest")) { 443 retVal.add(daoReferralRequestDstu3()); 444 } 445 if (theResourceSupportedSvc.isSupported("RelatedPerson")) { 446 retVal.add(daoRelatedPersonDstu3()); 447 } 448 if (theResourceSupportedSvc.isSupported("RequestGroup")) { 449 retVal.add(daoRequestGroupDstu3()); 450 } 451 if (theResourceSupportedSvc.isSupported("ResearchStudy")) { 452 retVal.add(daoResearchStudyDstu3()); 453 } 454 if (theResourceSupportedSvc.isSupported("ResearchSubject")) { 455 retVal.add(daoResearchSubjectDstu3()); 456 } 457 if (theResourceSupportedSvc.isSupported("RiskAssessment")) { 458 retVal.add(daoRiskAssessmentDstu3()); 459 } 460 if (theResourceSupportedSvc.isSupported("Schedule")) { 461 retVal.add(daoScheduleDstu3()); 462 } 463 if (theResourceSupportedSvc.isSupported("SearchParameter")) { 464 retVal.add(daoSearchParameterDstu3()); 465 } 466 if (theResourceSupportedSvc.isSupported("Sequence")) { 467 retVal.add(daoSequenceDstu3()); 468 } 469 if (theResourceSupportedSvc.isSupported("ServiceDefinition")) { 470 retVal.add(daoServiceDefinitionDstu3()); 471 } 472 if (theResourceSupportedSvc.isSupported("Slot")) { 473 retVal.add(daoSlotDstu3()); 474 } 475 if (theResourceSupportedSvc.isSupported("Specimen")) { 476 retVal.add(daoSpecimenDstu3()); 477 } 478 if (theResourceSupportedSvc.isSupported("StructureDefinition")) { 479 retVal.add(daoStructureDefinitionDstu3()); 480 } 481 if (theResourceSupportedSvc.isSupported("StructureMap")) { 482 retVal.add(daoStructureMapDstu3()); 483 } 484 if (theResourceSupportedSvc.isSupported("Subscription")) { 485 retVal.add(daoSubscriptionDstu3()); 486 } 487 if (theResourceSupportedSvc.isSupported("Substance")) { 488 retVal.add(daoSubstanceDstu3()); 489 } 490 if (theResourceSupportedSvc.isSupported("SupplyDelivery")) { 491 retVal.add(daoSupplyDeliveryDstu3()); 492 } 493 if (theResourceSupportedSvc.isSupported("SupplyRequest")) { 494 retVal.add(daoSupplyRequestDstu3()); 495 } 496 if (theResourceSupportedSvc.isSupported("Task")) { 497 retVal.add(daoTaskDstu3()); 498 } 499 if (theResourceSupportedSvc.isSupported("TestReport")) { 500 retVal.add(daoTestReportDstu3()); 501 } 502 if (theResourceSupportedSvc.isSupported("TestScript")) { 503 retVal.add(daoTestScriptDstu3()); 504 } 505 if (theResourceSupportedSvc.isSupported("ValueSet")) { 506 retVal.add(daoValueSetDstu3()); 507 } 508 if (theResourceSupportedSvc.isSupported("VisionPrescription")) { 509 retVal.add(daoVisionPrescriptionDstu3()); 510 } 511 return retVal; 512 } 513 514 @Bean(name="myAccountDaoDstu3") 515 public 516 IFhirResourceDao<org.hl7.fhir.dstu3.model.Account> 517 daoAccountDstu3() { 518 519 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Account> retVal; 520 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Account>(); 521 retVal.setResourceType(org.hl7.fhir.dstu3.model.Account.class); 522 retVal.setContext(myFhirContext); 523 return retVal; 524 } 525 526 @Bean(name="myAccountRpDstu3") 527 @Lazy 528 public ca.uhn.fhir.jpa.rp.dstu3.AccountResourceProvider rpAccountDstu3() { 529 ca.uhn.fhir.jpa.rp.dstu3.AccountResourceProvider retVal; 530 retVal = new ca.uhn.fhir.jpa.rp.dstu3.AccountResourceProvider(); 531 retVal.setContext(myFhirContext); 532 retVal.setDao(daoAccountDstu3()); 533 return retVal; 534 } 535 536 @Bean(name="myActivityDefinitionDaoDstu3") 537 public 538 IFhirResourceDao<org.hl7.fhir.dstu3.model.ActivityDefinition> 539 daoActivityDefinitionDstu3() { 540 541 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ActivityDefinition> retVal; 542 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ActivityDefinition>(); 543 retVal.setResourceType(org.hl7.fhir.dstu3.model.ActivityDefinition.class); 544 retVal.setContext(myFhirContext); 545 return retVal; 546 } 547 548 @Bean(name="myActivityDefinitionRpDstu3") 549 @Lazy 550 public ca.uhn.fhir.jpa.rp.dstu3.ActivityDefinitionResourceProvider rpActivityDefinitionDstu3() { 551 ca.uhn.fhir.jpa.rp.dstu3.ActivityDefinitionResourceProvider retVal; 552 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ActivityDefinitionResourceProvider(); 553 retVal.setContext(myFhirContext); 554 retVal.setDao(daoActivityDefinitionDstu3()); 555 return retVal; 556 } 557 558 @Bean(name="myAdverseEventDaoDstu3") 559 public 560 IFhirResourceDao<org.hl7.fhir.dstu3.model.AdverseEvent> 561 daoAdverseEventDstu3() { 562 563 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.AdverseEvent> retVal; 564 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.AdverseEvent>(); 565 retVal.setResourceType(org.hl7.fhir.dstu3.model.AdverseEvent.class); 566 retVal.setContext(myFhirContext); 567 return retVal; 568 } 569 570 @Bean(name="myAdverseEventRpDstu3") 571 @Lazy 572 public ca.uhn.fhir.jpa.rp.dstu3.AdverseEventResourceProvider rpAdverseEventDstu3() { 573 ca.uhn.fhir.jpa.rp.dstu3.AdverseEventResourceProvider retVal; 574 retVal = new ca.uhn.fhir.jpa.rp.dstu3.AdverseEventResourceProvider(); 575 retVal.setContext(myFhirContext); 576 retVal.setDao(daoAdverseEventDstu3()); 577 return retVal; 578 } 579 580 @Bean(name="myAllergyIntoleranceDaoDstu3") 581 public 582 IFhirResourceDao<org.hl7.fhir.dstu3.model.AllergyIntolerance> 583 daoAllergyIntoleranceDstu3() { 584 585 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.AllergyIntolerance> retVal; 586 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.AllergyIntolerance>(); 587 retVal.setResourceType(org.hl7.fhir.dstu3.model.AllergyIntolerance.class); 588 retVal.setContext(myFhirContext); 589 return retVal; 590 } 591 592 @Bean(name="myAllergyIntoleranceRpDstu3") 593 @Lazy 594 public ca.uhn.fhir.jpa.rp.dstu3.AllergyIntoleranceResourceProvider rpAllergyIntoleranceDstu3() { 595 ca.uhn.fhir.jpa.rp.dstu3.AllergyIntoleranceResourceProvider retVal; 596 retVal = new ca.uhn.fhir.jpa.rp.dstu3.AllergyIntoleranceResourceProvider(); 597 retVal.setContext(myFhirContext); 598 retVal.setDao(daoAllergyIntoleranceDstu3()); 599 return retVal; 600 } 601 602 @Bean(name="myAppointmentDaoDstu3") 603 public 604 IFhirResourceDao<org.hl7.fhir.dstu3.model.Appointment> 605 daoAppointmentDstu3() { 606 607 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Appointment> retVal; 608 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Appointment>(); 609 retVal.setResourceType(org.hl7.fhir.dstu3.model.Appointment.class); 610 retVal.setContext(myFhirContext); 611 return retVal; 612 } 613 614 @Bean(name="myAppointmentRpDstu3") 615 @Lazy 616 public ca.uhn.fhir.jpa.rp.dstu3.AppointmentResourceProvider rpAppointmentDstu3() { 617 ca.uhn.fhir.jpa.rp.dstu3.AppointmentResourceProvider retVal; 618 retVal = new ca.uhn.fhir.jpa.rp.dstu3.AppointmentResourceProvider(); 619 retVal.setContext(myFhirContext); 620 retVal.setDao(daoAppointmentDstu3()); 621 return retVal; 622 } 623 624 @Bean(name="myAppointmentResponseDaoDstu3") 625 public 626 IFhirResourceDao<org.hl7.fhir.dstu3.model.AppointmentResponse> 627 daoAppointmentResponseDstu3() { 628 629 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.AppointmentResponse> retVal; 630 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.AppointmentResponse>(); 631 retVal.setResourceType(org.hl7.fhir.dstu3.model.AppointmentResponse.class); 632 retVal.setContext(myFhirContext); 633 return retVal; 634 } 635 636 @Bean(name="myAppointmentResponseRpDstu3") 637 @Lazy 638 public ca.uhn.fhir.jpa.rp.dstu3.AppointmentResponseResourceProvider rpAppointmentResponseDstu3() { 639 ca.uhn.fhir.jpa.rp.dstu3.AppointmentResponseResourceProvider retVal; 640 retVal = new ca.uhn.fhir.jpa.rp.dstu3.AppointmentResponseResourceProvider(); 641 retVal.setContext(myFhirContext); 642 retVal.setDao(daoAppointmentResponseDstu3()); 643 return retVal; 644 } 645 646 @Bean(name="myAuditEventDaoDstu3") 647 public 648 IFhirResourceDao<org.hl7.fhir.dstu3.model.AuditEvent> 649 daoAuditEventDstu3() { 650 651 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.AuditEvent> retVal; 652 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.AuditEvent>(); 653 retVal.setResourceType(org.hl7.fhir.dstu3.model.AuditEvent.class); 654 retVal.setContext(myFhirContext); 655 return retVal; 656 } 657 658 @Bean(name="myAuditEventRpDstu3") 659 @Lazy 660 public ca.uhn.fhir.jpa.rp.dstu3.AuditEventResourceProvider rpAuditEventDstu3() { 661 ca.uhn.fhir.jpa.rp.dstu3.AuditEventResourceProvider retVal; 662 retVal = new ca.uhn.fhir.jpa.rp.dstu3.AuditEventResourceProvider(); 663 retVal.setContext(myFhirContext); 664 retVal.setDao(daoAuditEventDstu3()); 665 return retVal; 666 } 667 668 @Bean(name="myBasicDaoDstu3") 669 public 670 IFhirResourceDao<org.hl7.fhir.dstu3.model.Basic> 671 daoBasicDstu3() { 672 673 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Basic> retVal; 674 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Basic>(); 675 retVal.setResourceType(org.hl7.fhir.dstu3.model.Basic.class); 676 retVal.setContext(myFhirContext); 677 return retVal; 678 } 679 680 @Bean(name="myBasicRpDstu3") 681 @Lazy 682 public ca.uhn.fhir.jpa.rp.dstu3.BasicResourceProvider rpBasicDstu3() { 683 ca.uhn.fhir.jpa.rp.dstu3.BasicResourceProvider retVal; 684 retVal = new ca.uhn.fhir.jpa.rp.dstu3.BasicResourceProvider(); 685 retVal.setContext(myFhirContext); 686 retVal.setDao(daoBasicDstu3()); 687 return retVal; 688 } 689 690 @Bean(name="myBinaryDaoDstu3") 691 public 692 IFhirResourceDao<org.hl7.fhir.dstu3.model.Binary> 693 daoBinaryDstu3() { 694 695 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Binary> retVal; 696 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Binary>(); 697 retVal.setResourceType(org.hl7.fhir.dstu3.model.Binary.class); 698 retVal.setContext(myFhirContext); 699 return retVal; 700 } 701 702 @Bean(name="myBinaryRpDstu3") 703 @Lazy 704 public ca.uhn.fhir.jpa.rp.dstu3.BinaryResourceProvider rpBinaryDstu3() { 705 ca.uhn.fhir.jpa.rp.dstu3.BinaryResourceProvider retVal; 706 retVal = new ca.uhn.fhir.jpa.rp.dstu3.BinaryResourceProvider(); 707 retVal.setContext(myFhirContext); 708 retVal.setDao(daoBinaryDstu3()); 709 return retVal; 710 } 711 712 @Bean(name="myBodySiteDaoDstu3") 713 public 714 IFhirResourceDao<org.hl7.fhir.dstu3.model.BodySite> 715 daoBodySiteDstu3() { 716 717 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.BodySite> retVal; 718 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.BodySite>(); 719 retVal.setResourceType(org.hl7.fhir.dstu3.model.BodySite.class); 720 retVal.setContext(myFhirContext); 721 return retVal; 722 } 723 724 @Bean(name="myBodySiteRpDstu3") 725 @Lazy 726 public ca.uhn.fhir.jpa.rp.dstu3.BodySiteResourceProvider rpBodySiteDstu3() { 727 ca.uhn.fhir.jpa.rp.dstu3.BodySiteResourceProvider retVal; 728 retVal = new ca.uhn.fhir.jpa.rp.dstu3.BodySiteResourceProvider(); 729 retVal.setContext(myFhirContext); 730 retVal.setDao(daoBodySiteDstu3()); 731 return retVal; 732 } 733 734 @Bean(name="myBundleDaoDstu3") 735 public 736 IFhirResourceDao<org.hl7.fhir.dstu3.model.Bundle> 737 daoBundleDstu3() { 738 739 ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<org.hl7.fhir.dstu3.model.Bundle> retVal; 740 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<>(); 741 retVal.setResourceType(org.hl7.fhir.dstu3.model.Bundle.class); 742 retVal.setContext(myFhirContext); 743 return retVal; 744 } 745 746 @Bean(name="myBundleRpDstu3") 747 @Lazy 748 public ca.uhn.fhir.jpa.rp.dstu3.BundleResourceProvider rpBundleDstu3() { 749 ca.uhn.fhir.jpa.rp.dstu3.BundleResourceProvider retVal; 750 retVal = new ca.uhn.fhir.jpa.rp.dstu3.BundleResourceProvider(); 751 retVal.setContext(myFhirContext); 752 retVal.setDao(daoBundleDstu3()); 753 return retVal; 754 } 755 756 @Bean(name="myCapabilityStatementDaoDstu3") 757 public 758 IFhirResourceDao<org.hl7.fhir.dstu3.model.CapabilityStatement> 759 daoCapabilityStatementDstu3() { 760 761 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.CapabilityStatement> retVal; 762 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.CapabilityStatement>(); 763 retVal.setResourceType(org.hl7.fhir.dstu3.model.CapabilityStatement.class); 764 retVal.setContext(myFhirContext); 765 return retVal; 766 } 767 768 @Bean(name="myCapabilityStatementRpDstu3") 769 @Lazy 770 public ca.uhn.fhir.jpa.rp.dstu3.CapabilityStatementResourceProvider rpCapabilityStatementDstu3() { 771 ca.uhn.fhir.jpa.rp.dstu3.CapabilityStatementResourceProvider retVal; 772 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CapabilityStatementResourceProvider(); 773 retVal.setContext(myFhirContext); 774 retVal.setDao(daoCapabilityStatementDstu3()); 775 return retVal; 776 } 777 778 @Bean(name="myCarePlanDaoDstu3") 779 public 780 IFhirResourceDao<org.hl7.fhir.dstu3.model.CarePlan> 781 daoCarePlanDstu3() { 782 783 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.CarePlan> retVal; 784 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.CarePlan>(); 785 retVal.setResourceType(org.hl7.fhir.dstu3.model.CarePlan.class); 786 retVal.setContext(myFhirContext); 787 return retVal; 788 } 789 790 @Bean(name="myCarePlanRpDstu3") 791 @Lazy 792 public ca.uhn.fhir.jpa.rp.dstu3.CarePlanResourceProvider rpCarePlanDstu3() { 793 ca.uhn.fhir.jpa.rp.dstu3.CarePlanResourceProvider retVal; 794 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CarePlanResourceProvider(); 795 retVal.setContext(myFhirContext); 796 retVal.setDao(daoCarePlanDstu3()); 797 return retVal; 798 } 799 800 @Bean(name="myCareTeamDaoDstu3") 801 public 802 IFhirResourceDao<org.hl7.fhir.dstu3.model.CareTeam> 803 daoCareTeamDstu3() { 804 805 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.CareTeam> retVal; 806 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.CareTeam>(); 807 retVal.setResourceType(org.hl7.fhir.dstu3.model.CareTeam.class); 808 retVal.setContext(myFhirContext); 809 return retVal; 810 } 811 812 @Bean(name="myCareTeamRpDstu3") 813 @Lazy 814 public ca.uhn.fhir.jpa.rp.dstu3.CareTeamResourceProvider rpCareTeamDstu3() { 815 ca.uhn.fhir.jpa.rp.dstu3.CareTeamResourceProvider retVal; 816 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CareTeamResourceProvider(); 817 retVal.setContext(myFhirContext); 818 retVal.setDao(daoCareTeamDstu3()); 819 return retVal; 820 } 821 822 @Bean(name="myChargeItemDaoDstu3") 823 public 824 IFhirResourceDao<org.hl7.fhir.dstu3.model.ChargeItem> 825 daoChargeItemDstu3() { 826 827 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ChargeItem> retVal; 828 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ChargeItem>(); 829 retVal.setResourceType(org.hl7.fhir.dstu3.model.ChargeItem.class); 830 retVal.setContext(myFhirContext); 831 return retVal; 832 } 833 834 @Bean(name="myChargeItemRpDstu3") 835 @Lazy 836 public ca.uhn.fhir.jpa.rp.dstu3.ChargeItemResourceProvider rpChargeItemDstu3() { 837 ca.uhn.fhir.jpa.rp.dstu3.ChargeItemResourceProvider retVal; 838 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ChargeItemResourceProvider(); 839 retVal.setContext(myFhirContext); 840 retVal.setDao(daoChargeItemDstu3()); 841 return retVal; 842 } 843 844 @Bean(name="myClaimDaoDstu3") 845 public 846 IFhirResourceDao<org.hl7.fhir.dstu3.model.Claim> 847 daoClaimDstu3() { 848 849 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Claim> retVal; 850 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Claim>(); 851 retVal.setResourceType(org.hl7.fhir.dstu3.model.Claim.class); 852 retVal.setContext(myFhirContext); 853 return retVal; 854 } 855 856 @Bean(name="myClaimRpDstu3") 857 @Lazy 858 public ca.uhn.fhir.jpa.rp.dstu3.ClaimResourceProvider rpClaimDstu3() { 859 ca.uhn.fhir.jpa.rp.dstu3.ClaimResourceProvider retVal; 860 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ClaimResourceProvider(); 861 retVal.setContext(myFhirContext); 862 retVal.setDao(daoClaimDstu3()); 863 return retVal; 864 } 865 866 @Bean(name="myClaimResponseDaoDstu3") 867 public 868 IFhirResourceDao<org.hl7.fhir.dstu3.model.ClaimResponse> 869 daoClaimResponseDstu3() { 870 871 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ClaimResponse> retVal; 872 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ClaimResponse>(); 873 retVal.setResourceType(org.hl7.fhir.dstu3.model.ClaimResponse.class); 874 retVal.setContext(myFhirContext); 875 return retVal; 876 } 877 878 @Bean(name="myClaimResponseRpDstu3") 879 @Lazy 880 public ca.uhn.fhir.jpa.rp.dstu3.ClaimResponseResourceProvider rpClaimResponseDstu3() { 881 ca.uhn.fhir.jpa.rp.dstu3.ClaimResponseResourceProvider retVal; 882 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ClaimResponseResourceProvider(); 883 retVal.setContext(myFhirContext); 884 retVal.setDao(daoClaimResponseDstu3()); 885 return retVal; 886 } 887 888 @Bean(name="myClinicalImpressionDaoDstu3") 889 public 890 IFhirResourceDao<org.hl7.fhir.dstu3.model.ClinicalImpression> 891 daoClinicalImpressionDstu3() { 892 893 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ClinicalImpression> retVal; 894 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ClinicalImpression>(); 895 retVal.setResourceType(org.hl7.fhir.dstu3.model.ClinicalImpression.class); 896 retVal.setContext(myFhirContext); 897 return retVal; 898 } 899 900 @Bean(name="myClinicalImpressionRpDstu3") 901 @Lazy 902 public ca.uhn.fhir.jpa.rp.dstu3.ClinicalImpressionResourceProvider rpClinicalImpressionDstu3() { 903 ca.uhn.fhir.jpa.rp.dstu3.ClinicalImpressionResourceProvider retVal; 904 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ClinicalImpressionResourceProvider(); 905 retVal.setContext(myFhirContext); 906 retVal.setDao(daoClinicalImpressionDstu3()); 907 return retVal; 908 } 909 910 @Bean(name="myCodeSystemDaoDstu3") 911 public 912 IFhirResourceDaoCodeSystem<org.hl7.fhir.dstu3.model.CodeSystem> 913 daoCodeSystemDstu3() { 914 915 ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem<org.hl7.fhir.dstu3.model.CodeSystem> retVal; 916 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem<>(); 917 retVal.setResourceType(org.hl7.fhir.dstu3.model.CodeSystem.class); 918 retVal.setContext(myFhirContext); 919 return retVal; 920 } 921 922 @Bean(name="myCodeSystemRpDstu3") 923 @Lazy 924 public ca.uhn.fhir.jpa.rp.dstu3.CodeSystemResourceProvider rpCodeSystemDstu3() { 925 ca.uhn.fhir.jpa.rp.dstu3.CodeSystemResourceProvider retVal; 926 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CodeSystemResourceProvider(); 927 retVal.setContext(myFhirContext); 928 retVal.setDao(daoCodeSystemDstu3()); 929 return retVal; 930 } 931 932 @Bean(name="myCommunicationDaoDstu3") 933 public 934 IFhirResourceDao<org.hl7.fhir.dstu3.model.Communication> 935 daoCommunicationDstu3() { 936 937 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Communication> retVal; 938 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Communication>(); 939 retVal.setResourceType(org.hl7.fhir.dstu3.model.Communication.class); 940 retVal.setContext(myFhirContext); 941 return retVal; 942 } 943 944 @Bean(name="myCommunicationRpDstu3") 945 @Lazy 946 public ca.uhn.fhir.jpa.rp.dstu3.CommunicationResourceProvider rpCommunicationDstu3() { 947 ca.uhn.fhir.jpa.rp.dstu3.CommunicationResourceProvider retVal; 948 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CommunicationResourceProvider(); 949 retVal.setContext(myFhirContext); 950 retVal.setDao(daoCommunicationDstu3()); 951 return retVal; 952 } 953 954 @Bean(name="myCommunicationRequestDaoDstu3") 955 public 956 IFhirResourceDao<org.hl7.fhir.dstu3.model.CommunicationRequest> 957 daoCommunicationRequestDstu3() { 958 959 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.CommunicationRequest> retVal; 960 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.CommunicationRequest>(); 961 retVal.setResourceType(org.hl7.fhir.dstu3.model.CommunicationRequest.class); 962 retVal.setContext(myFhirContext); 963 return retVal; 964 } 965 966 @Bean(name="myCommunicationRequestRpDstu3") 967 @Lazy 968 public ca.uhn.fhir.jpa.rp.dstu3.CommunicationRequestResourceProvider rpCommunicationRequestDstu3() { 969 ca.uhn.fhir.jpa.rp.dstu3.CommunicationRequestResourceProvider retVal; 970 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CommunicationRequestResourceProvider(); 971 retVal.setContext(myFhirContext); 972 retVal.setDao(daoCommunicationRequestDstu3()); 973 return retVal; 974 } 975 976 @Bean(name="myCompartmentDefinitionDaoDstu3") 977 public 978 IFhirResourceDao<org.hl7.fhir.dstu3.model.CompartmentDefinition> 979 daoCompartmentDefinitionDstu3() { 980 981 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.CompartmentDefinition> retVal; 982 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.CompartmentDefinition>(); 983 retVal.setResourceType(org.hl7.fhir.dstu3.model.CompartmentDefinition.class); 984 retVal.setContext(myFhirContext); 985 return retVal; 986 } 987 988 @Bean(name="myCompartmentDefinitionRpDstu3") 989 @Lazy 990 public ca.uhn.fhir.jpa.rp.dstu3.CompartmentDefinitionResourceProvider rpCompartmentDefinitionDstu3() { 991 ca.uhn.fhir.jpa.rp.dstu3.CompartmentDefinitionResourceProvider retVal; 992 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CompartmentDefinitionResourceProvider(); 993 retVal.setContext(myFhirContext); 994 retVal.setDao(daoCompartmentDefinitionDstu3()); 995 return retVal; 996 } 997 998 @Bean(name="myCompositionDaoDstu3") 999 public 1000 IFhirResourceDaoComposition<org.hl7.fhir.dstu3.model.Composition> 1001 daoCompositionDstu3() { 1002 1003 ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<org.hl7.fhir.dstu3.model.Composition> retVal; 1004 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<>(); 1005 retVal.setResourceType(org.hl7.fhir.dstu3.model.Composition.class); 1006 retVal.setContext(myFhirContext); 1007 return retVal; 1008 } 1009 1010 @Bean(name="myCompositionRpDstu3") 1011 @Lazy 1012 public ca.uhn.fhir.jpa.rp.dstu3.CompositionResourceProvider rpCompositionDstu3() { 1013 ca.uhn.fhir.jpa.rp.dstu3.CompositionResourceProvider retVal; 1014 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CompositionResourceProvider(); 1015 retVal.setContext(myFhirContext); 1016 retVal.setDao(daoCompositionDstu3()); 1017 return retVal; 1018 } 1019 1020 @Bean(name="myConceptMapDaoDstu3") 1021 public 1022 IFhirResourceDaoConceptMap<org.hl7.fhir.dstu3.model.ConceptMap> 1023 daoConceptMapDstu3() { 1024 1025 ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<org.hl7.fhir.dstu3.model.ConceptMap> retVal; 1026 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<>(); 1027 retVal.setResourceType(org.hl7.fhir.dstu3.model.ConceptMap.class); 1028 retVal.setContext(myFhirContext); 1029 return retVal; 1030 } 1031 1032 @Bean(name="myConceptMapRpDstu3") 1033 @Lazy 1034 public ca.uhn.fhir.jpa.rp.dstu3.ConceptMapResourceProvider rpConceptMapDstu3() { 1035 ca.uhn.fhir.jpa.rp.dstu3.ConceptMapResourceProvider retVal; 1036 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ConceptMapResourceProvider(); 1037 retVal.setContext(myFhirContext); 1038 retVal.setDao(daoConceptMapDstu3()); 1039 return retVal; 1040 } 1041 1042 @Bean(name="myConditionDaoDstu3") 1043 public 1044 IFhirResourceDao<org.hl7.fhir.dstu3.model.Condition> 1045 daoConditionDstu3() { 1046 1047 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Condition> retVal; 1048 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Condition>(); 1049 retVal.setResourceType(org.hl7.fhir.dstu3.model.Condition.class); 1050 retVal.setContext(myFhirContext); 1051 return retVal; 1052 } 1053 1054 @Bean(name="myConditionRpDstu3") 1055 @Lazy 1056 public ca.uhn.fhir.jpa.rp.dstu3.ConditionResourceProvider rpConditionDstu3() { 1057 ca.uhn.fhir.jpa.rp.dstu3.ConditionResourceProvider retVal; 1058 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ConditionResourceProvider(); 1059 retVal.setContext(myFhirContext); 1060 retVal.setDao(daoConditionDstu3()); 1061 return retVal; 1062 } 1063 1064 @Bean(name="myConsentDaoDstu3") 1065 public 1066 IFhirResourceDao<org.hl7.fhir.dstu3.model.Consent> 1067 daoConsentDstu3() { 1068 1069 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Consent> retVal; 1070 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Consent>(); 1071 retVal.setResourceType(org.hl7.fhir.dstu3.model.Consent.class); 1072 retVal.setContext(myFhirContext); 1073 return retVal; 1074 } 1075 1076 @Bean(name="myConsentRpDstu3") 1077 @Lazy 1078 public ca.uhn.fhir.jpa.rp.dstu3.ConsentResourceProvider rpConsentDstu3() { 1079 ca.uhn.fhir.jpa.rp.dstu3.ConsentResourceProvider retVal; 1080 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ConsentResourceProvider(); 1081 retVal.setContext(myFhirContext); 1082 retVal.setDao(daoConsentDstu3()); 1083 return retVal; 1084 } 1085 1086 @Bean(name="myContractDaoDstu3") 1087 public 1088 IFhirResourceDao<org.hl7.fhir.dstu3.model.Contract> 1089 daoContractDstu3() { 1090 1091 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Contract> retVal; 1092 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Contract>(); 1093 retVal.setResourceType(org.hl7.fhir.dstu3.model.Contract.class); 1094 retVal.setContext(myFhirContext); 1095 return retVal; 1096 } 1097 1098 @Bean(name="myContractRpDstu3") 1099 @Lazy 1100 public ca.uhn.fhir.jpa.rp.dstu3.ContractResourceProvider rpContractDstu3() { 1101 ca.uhn.fhir.jpa.rp.dstu3.ContractResourceProvider retVal; 1102 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ContractResourceProvider(); 1103 retVal.setContext(myFhirContext); 1104 retVal.setDao(daoContractDstu3()); 1105 return retVal; 1106 } 1107 1108 @Bean(name="myCoverageDaoDstu3") 1109 public 1110 IFhirResourceDao<org.hl7.fhir.dstu3.model.Coverage> 1111 daoCoverageDstu3() { 1112 1113 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Coverage> retVal; 1114 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Coverage>(); 1115 retVal.setResourceType(org.hl7.fhir.dstu3.model.Coverage.class); 1116 retVal.setContext(myFhirContext); 1117 return retVal; 1118 } 1119 1120 @Bean(name="myCoverageRpDstu3") 1121 @Lazy 1122 public ca.uhn.fhir.jpa.rp.dstu3.CoverageResourceProvider rpCoverageDstu3() { 1123 ca.uhn.fhir.jpa.rp.dstu3.CoverageResourceProvider retVal; 1124 retVal = new ca.uhn.fhir.jpa.rp.dstu3.CoverageResourceProvider(); 1125 retVal.setContext(myFhirContext); 1126 retVal.setDao(daoCoverageDstu3()); 1127 return retVal; 1128 } 1129 1130 @Bean(name="myDataElementDaoDstu3") 1131 public 1132 IFhirResourceDao<org.hl7.fhir.dstu3.model.DataElement> 1133 daoDataElementDstu3() { 1134 1135 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DataElement> retVal; 1136 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DataElement>(); 1137 retVal.setResourceType(org.hl7.fhir.dstu3.model.DataElement.class); 1138 retVal.setContext(myFhirContext); 1139 return retVal; 1140 } 1141 1142 @Bean(name="myDataElementRpDstu3") 1143 @Lazy 1144 public ca.uhn.fhir.jpa.rp.dstu3.DataElementResourceProvider rpDataElementDstu3() { 1145 ca.uhn.fhir.jpa.rp.dstu3.DataElementResourceProvider retVal; 1146 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DataElementResourceProvider(); 1147 retVal.setContext(myFhirContext); 1148 retVal.setDao(daoDataElementDstu3()); 1149 return retVal; 1150 } 1151 1152 @Bean(name="myDetectedIssueDaoDstu3") 1153 public 1154 IFhirResourceDao<org.hl7.fhir.dstu3.model.DetectedIssue> 1155 daoDetectedIssueDstu3() { 1156 1157 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DetectedIssue> retVal; 1158 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DetectedIssue>(); 1159 retVal.setResourceType(org.hl7.fhir.dstu3.model.DetectedIssue.class); 1160 retVal.setContext(myFhirContext); 1161 return retVal; 1162 } 1163 1164 @Bean(name="myDetectedIssueRpDstu3") 1165 @Lazy 1166 public ca.uhn.fhir.jpa.rp.dstu3.DetectedIssueResourceProvider rpDetectedIssueDstu3() { 1167 ca.uhn.fhir.jpa.rp.dstu3.DetectedIssueResourceProvider retVal; 1168 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DetectedIssueResourceProvider(); 1169 retVal.setContext(myFhirContext); 1170 retVal.setDao(daoDetectedIssueDstu3()); 1171 return retVal; 1172 } 1173 1174 @Bean(name="myDeviceDaoDstu3") 1175 public 1176 IFhirResourceDao<org.hl7.fhir.dstu3.model.Device> 1177 daoDeviceDstu3() { 1178 1179 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Device> retVal; 1180 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Device>(); 1181 retVal.setResourceType(org.hl7.fhir.dstu3.model.Device.class); 1182 retVal.setContext(myFhirContext); 1183 return retVal; 1184 } 1185 1186 @Bean(name="myDeviceRpDstu3") 1187 @Lazy 1188 public ca.uhn.fhir.jpa.rp.dstu3.DeviceResourceProvider rpDeviceDstu3() { 1189 ca.uhn.fhir.jpa.rp.dstu3.DeviceResourceProvider retVal; 1190 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DeviceResourceProvider(); 1191 retVal.setContext(myFhirContext); 1192 retVal.setDao(daoDeviceDstu3()); 1193 return retVal; 1194 } 1195 1196 @Bean(name="myDeviceComponentDaoDstu3") 1197 public 1198 IFhirResourceDao<org.hl7.fhir.dstu3.model.DeviceComponent> 1199 daoDeviceComponentDstu3() { 1200 1201 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DeviceComponent> retVal; 1202 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DeviceComponent>(); 1203 retVal.setResourceType(org.hl7.fhir.dstu3.model.DeviceComponent.class); 1204 retVal.setContext(myFhirContext); 1205 return retVal; 1206 } 1207 1208 @Bean(name="myDeviceComponentRpDstu3") 1209 @Lazy 1210 public ca.uhn.fhir.jpa.rp.dstu3.DeviceComponentResourceProvider rpDeviceComponentDstu3() { 1211 ca.uhn.fhir.jpa.rp.dstu3.DeviceComponentResourceProvider retVal; 1212 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DeviceComponentResourceProvider(); 1213 retVal.setContext(myFhirContext); 1214 retVal.setDao(daoDeviceComponentDstu3()); 1215 return retVal; 1216 } 1217 1218 @Bean(name="myDeviceMetricDaoDstu3") 1219 public 1220 IFhirResourceDao<org.hl7.fhir.dstu3.model.DeviceMetric> 1221 daoDeviceMetricDstu3() { 1222 1223 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DeviceMetric> retVal; 1224 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DeviceMetric>(); 1225 retVal.setResourceType(org.hl7.fhir.dstu3.model.DeviceMetric.class); 1226 retVal.setContext(myFhirContext); 1227 return retVal; 1228 } 1229 1230 @Bean(name="myDeviceMetricRpDstu3") 1231 @Lazy 1232 public ca.uhn.fhir.jpa.rp.dstu3.DeviceMetricResourceProvider rpDeviceMetricDstu3() { 1233 ca.uhn.fhir.jpa.rp.dstu3.DeviceMetricResourceProvider retVal; 1234 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DeviceMetricResourceProvider(); 1235 retVal.setContext(myFhirContext); 1236 retVal.setDao(daoDeviceMetricDstu3()); 1237 return retVal; 1238 } 1239 1240 @Bean(name="myDeviceRequestDaoDstu3") 1241 public 1242 IFhirResourceDao<org.hl7.fhir.dstu3.model.DeviceRequest> 1243 daoDeviceRequestDstu3() { 1244 1245 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DeviceRequest> retVal; 1246 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DeviceRequest>(); 1247 retVal.setResourceType(org.hl7.fhir.dstu3.model.DeviceRequest.class); 1248 retVal.setContext(myFhirContext); 1249 return retVal; 1250 } 1251 1252 @Bean(name="myDeviceRequestRpDstu3") 1253 @Lazy 1254 public ca.uhn.fhir.jpa.rp.dstu3.DeviceRequestResourceProvider rpDeviceRequestDstu3() { 1255 ca.uhn.fhir.jpa.rp.dstu3.DeviceRequestResourceProvider retVal; 1256 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DeviceRequestResourceProvider(); 1257 retVal.setContext(myFhirContext); 1258 retVal.setDao(daoDeviceRequestDstu3()); 1259 return retVal; 1260 } 1261 1262 @Bean(name="myDeviceUseStatementDaoDstu3") 1263 public 1264 IFhirResourceDao<org.hl7.fhir.dstu3.model.DeviceUseStatement> 1265 daoDeviceUseStatementDstu3() { 1266 1267 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DeviceUseStatement> retVal; 1268 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DeviceUseStatement>(); 1269 retVal.setResourceType(org.hl7.fhir.dstu3.model.DeviceUseStatement.class); 1270 retVal.setContext(myFhirContext); 1271 return retVal; 1272 } 1273 1274 @Bean(name="myDeviceUseStatementRpDstu3") 1275 @Lazy 1276 public ca.uhn.fhir.jpa.rp.dstu3.DeviceUseStatementResourceProvider rpDeviceUseStatementDstu3() { 1277 ca.uhn.fhir.jpa.rp.dstu3.DeviceUseStatementResourceProvider retVal; 1278 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DeviceUseStatementResourceProvider(); 1279 retVal.setContext(myFhirContext); 1280 retVal.setDao(daoDeviceUseStatementDstu3()); 1281 return retVal; 1282 } 1283 1284 @Bean(name="myDiagnosticReportDaoDstu3") 1285 public 1286 IFhirResourceDao<org.hl7.fhir.dstu3.model.DiagnosticReport> 1287 daoDiagnosticReportDstu3() { 1288 1289 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DiagnosticReport> retVal; 1290 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DiagnosticReport>(); 1291 retVal.setResourceType(org.hl7.fhir.dstu3.model.DiagnosticReport.class); 1292 retVal.setContext(myFhirContext); 1293 return retVal; 1294 } 1295 1296 @Bean(name="myDiagnosticReportRpDstu3") 1297 @Lazy 1298 public ca.uhn.fhir.jpa.rp.dstu3.DiagnosticReportResourceProvider rpDiagnosticReportDstu3() { 1299 ca.uhn.fhir.jpa.rp.dstu3.DiagnosticReportResourceProvider retVal; 1300 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DiagnosticReportResourceProvider(); 1301 retVal.setContext(myFhirContext); 1302 retVal.setDao(daoDiagnosticReportDstu3()); 1303 return retVal; 1304 } 1305 1306 @Bean(name="myDocumentManifestDaoDstu3") 1307 public 1308 IFhirResourceDao<org.hl7.fhir.dstu3.model.DocumentManifest> 1309 daoDocumentManifestDstu3() { 1310 1311 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DocumentManifest> retVal; 1312 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DocumentManifest>(); 1313 retVal.setResourceType(org.hl7.fhir.dstu3.model.DocumentManifest.class); 1314 retVal.setContext(myFhirContext); 1315 return retVal; 1316 } 1317 1318 @Bean(name="myDocumentManifestRpDstu3") 1319 @Lazy 1320 public ca.uhn.fhir.jpa.rp.dstu3.DocumentManifestResourceProvider rpDocumentManifestDstu3() { 1321 ca.uhn.fhir.jpa.rp.dstu3.DocumentManifestResourceProvider retVal; 1322 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DocumentManifestResourceProvider(); 1323 retVal.setContext(myFhirContext); 1324 retVal.setDao(daoDocumentManifestDstu3()); 1325 return retVal; 1326 } 1327 1328 @Bean(name="myDocumentReferenceDaoDstu3") 1329 public 1330 IFhirResourceDao<org.hl7.fhir.dstu3.model.DocumentReference> 1331 daoDocumentReferenceDstu3() { 1332 1333 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.DocumentReference> retVal; 1334 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.DocumentReference>(); 1335 retVal.setResourceType(org.hl7.fhir.dstu3.model.DocumentReference.class); 1336 retVal.setContext(myFhirContext); 1337 return retVal; 1338 } 1339 1340 @Bean(name="myDocumentReferenceRpDstu3") 1341 @Lazy 1342 public ca.uhn.fhir.jpa.rp.dstu3.DocumentReferenceResourceProvider rpDocumentReferenceDstu3() { 1343 ca.uhn.fhir.jpa.rp.dstu3.DocumentReferenceResourceProvider retVal; 1344 retVal = new ca.uhn.fhir.jpa.rp.dstu3.DocumentReferenceResourceProvider(); 1345 retVal.setContext(myFhirContext); 1346 retVal.setDao(daoDocumentReferenceDstu3()); 1347 return retVal; 1348 } 1349 1350 @Bean(name="myEligibilityRequestDaoDstu3") 1351 public 1352 IFhirResourceDao<org.hl7.fhir.dstu3.model.EligibilityRequest> 1353 daoEligibilityRequestDstu3() { 1354 1355 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.EligibilityRequest> retVal; 1356 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.EligibilityRequest>(); 1357 retVal.setResourceType(org.hl7.fhir.dstu3.model.EligibilityRequest.class); 1358 retVal.setContext(myFhirContext); 1359 return retVal; 1360 } 1361 1362 @Bean(name="myEligibilityRequestRpDstu3") 1363 @Lazy 1364 public ca.uhn.fhir.jpa.rp.dstu3.EligibilityRequestResourceProvider rpEligibilityRequestDstu3() { 1365 ca.uhn.fhir.jpa.rp.dstu3.EligibilityRequestResourceProvider retVal; 1366 retVal = new ca.uhn.fhir.jpa.rp.dstu3.EligibilityRequestResourceProvider(); 1367 retVal.setContext(myFhirContext); 1368 retVal.setDao(daoEligibilityRequestDstu3()); 1369 return retVal; 1370 } 1371 1372 @Bean(name="myEligibilityResponseDaoDstu3") 1373 public 1374 IFhirResourceDao<org.hl7.fhir.dstu3.model.EligibilityResponse> 1375 daoEligibilityResponseDstu3() { 1376 1377 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.EligibilityResponse> retVal; 1378 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.EligibilityResponse>(); 1379 retVal.setResourceType(org.hl7.fhir.dstu3.model.EligibilityResponse.class); 1380 retVal.setContext(myFhirContext); 1381 return retVal; 1382 } 1383 1384 @Bean(name="myEligibilityResponseRpDstu3") 1385 @Lazy 1386 public ca.uhn.fhir.jpa.rp.dstu3.EligibilityResponseResourceProvider rpEligibilityResponseDstu3() { 1387 ca.uhn.fhir.jpa.rp.dstu3.EligibilityResponseResourceProvider retVal; 1388 retVal = new ca.uhn.fhir.jpa.rp.dstu3.EligibilityResponseResourceProvider(); 1389 retVal.setContext(myFhirContext); 1390 retVal.setDao(daoEligibilityResponseDstu3()); 1391 return retVal; 1392 } 1393 1394 @Bean(name="myEncounterDaoDstu3") 1395 public 1396 IFhirResourceDaoEncounter<org.hl7.fhir.dstu3.model.Encounter> 1397 daoEncounterDstu3() { 1398 1399 ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<org.hl7.fhir.dstu3.model.Encounter> retVal; 1400 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<>(); 1401 retVal.setResourceType(org.hl7.fhir.dstu3.model.Encounter.class); 1402 retVal.setContext(myFhirContext); 1403 return retVal; 1404 } 1405 1406 @Bean(name="myEncounterRpDstu3") 1407 @Lazy 1408 public ca.uhn.fhir.jpa.rp.dstu3.EncounterResourceProvider rpEncounterDstu3() { 1409 ca.uhn.fhir.jpa.rp.dstu3.EncounterResourceProvider retVal; 1410 retVal = new ca.uhn.fhir.jpa.rp.dstu3.EncounterResourceProvider(); 1411 retVal.setContext(myFhirContext); 1412 retVal.setDao(daoEncounterDstu3()); 1413 return retVal; 1414 } 1415 1416 @Bean(name="myEndpointDaoDstu3") 1417 public 1418 IFhirResourceDao<org.hl7.fhir.dstu3.model.Endpoint> 1419 daoEndpointDstu3() { 1420 1421 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Endpoint> retVal; 1422 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Endpoint>(); 1423 retVal.setResourceType(org.hl7.fhir.dstu3.model.Endpoint.class); 1424 retVal.setContext(myFhirContext); 1425 return retVal; 1426 } 1427 1428 @Bean(name="myEndpointRpDstu3") 1429 @Lazy 1430 public ca.uhn.fhir.jpa.rp.dstu3.EndpointResourceProvider rpEndpointDstu3() { 1431 ca.uhn.fhir.jpa.rp.dstu3.EndpointResourceProvider retVal; 1432 retVal = new ca.uhn.fhir.jpa.rp.dstu3.EndpointResourceProvider(); 1433 retVal.setContext(myFhirContext); 1434 retVal.setDao(daoEndpointDstu3()); 1435 return retVal; 1436 } 1437 1438 @Bean(name="myEnrollmentRequestDaoDstu3") 1439 public 1440 IFhirResourceDao<org.hl7.fhir.dstu3.model.EnrollmentRequest> 1441 daoEnrollmentRequestDstu3() { 1442 1443 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.EnrollmentRequest> retVal; 1444 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.EnrollmentRequest>(); 1445 retVal.setResourceType(org.hl7.fhir.dstu3.model.EnrollmentRequest.class); 1446 retVal.setContext(myFhirContext); 1447 return retVal; 1448 } 1449 1450 @Bean(name="myEnrollmentRequestRpDstu3") 1451 @Lazy 1452 public ca.uhn.fhir.jpa.rp.dstu3.EnrollmentRequestResourceProvider rpEnrollmentRequestDstu3() { 1453 ca.uhn.fhir.jpa.rp.dstu3.EnrollmentRequestResourceProvider retVal; 1454 retVal = new ca.uhn.fhir.jpa.rp.dstu3.EnrollmentRequestResourceProvider(); 1455 retVal.setContext(myFhirContext); 1456 retVal.setDao(daoEnrollmentRequestDstu3()); 1457 return retVal; 1458 } 1459 1460 @Bean(name="myEnrollmentResponseDaoDstu3") 1461 public 1462 IFhirResourceDao<org.hl7.fhir.dstu3.model.EnrollmentResponse> 1463 daoEnrollmentResponseDstu3() { 1464 1465 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.EnrollmentResponse> retVal; 1466 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.EnrollmentResponse>(); 1467 retVal.setResourceType(org.hl7.fhir.dstu3.model.EnrollmentResponse.class); 1468 retVal.setContext(myFhirContext); 1469 return retVal; 1470 } 1471 1472 @Bean(name="myEnrollmentResponseRpDstu3") 1473 @Lazy 1474 public ca.uhn.fhir.jpa.rp.dstu3.EnrollmentResponseResourceProvider rpEnrollmentResponseDstu3() { 1475 ca.uhn.fhir.jpa.rp.dstu3.EnrollmentResponseResourceProvider retVal; 1476 retVal = new ca.uhn.fhir.jpa.rp.dstu3.EnrollmentResponseResourceProvider(); 1477 retVal.setContext(myFhirContext); 1478 retVal.setDao(daoEnrollmentResponseDstu3()); 1479 return retVal; 1480 } 1481 1482 @Bean(name="myEpisodeOfCareDaoDstu3") 1483 public 1484 IFhirResourceDao<org.hl7.fhir.dstu3.model.EpisodeOfCare> 1485 daoEpisodeOfCareDstu3() { 1486 1487 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.EpisodeOfCare> retVal; 1488 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.EpisodeOfCare>(); 1489 retVal.setResourceType(org.hl7.fhir.dstu3.model.EpisodeOfCare.class); 1490 retVal.setContext(myFhirContext); 1491 return retVal; 1492 } 1493 1494 @Bean(name="myEpisodeOfCareRpDstu3") 1495 @Lazy 1496 public ca.uhn.fhir.jpa.rp.dstu3.EpisodeOfCareResourceProvider rpEpisodeOfCareDstu3() { 1497 ca.uhn.fhir.jpa.rp.dstu3.EpisodeOfCareResourceProvider retVal; 1498 retVal = new ca.uhn.fhir.jpa.rp.dstu3.EpisodeOfCareResourceProvider(); 1499 retVal.setContext(myFhirContext); 1500 retVal.setDao(daoEpisodeOfCareDstu3()); 1501 return retVal; 1502 } 1503 1504 @Bean(name="myExpansionProfileDaoDstu3") 1505 public 1506 IFhirResourceDao<org.hl7.fhir.dstu3.model.ExpansionProfile> 1507 daoExpansionProfileDstu3() { 1508 1509 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ExpansionProfile> retVal; 1510 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ExpansionProfile>(); 1511 retVal.setResourceType(org.hl7.fhir.dstu3.model.ExpansionProfile.class); 1512 retVal.setContext(myFhirContext); 1513 return retVal; 1514 } 1515 1516 @Bean(name="myExpansionProfileRpDstu3") 1517 @Lazy 1518 public ca.uhn.fhir.jpa.rp.dstu3.ExpansionProfileResourceProvider rpExpansionProfileDstu3() { 1519 ca.uhn.fhir.jpa.rp.dstu3.ExpansionProfileResourceProvider retVal; 1520 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ExpansionProfileResourceProvider(); 1521 retVal.setContext(myFhirContext); 1522 retVal.setDao(daoExpansionProfileDstu3()); 1523 return retVal; 1524 } 1525 1526 @Bean(name="myExplanationOfBenefitDaoDstu3") 1527 public 1528 IFhirResourceDao<org.hl7.fhir.dstu3.model.ExplanationOfBenefit> 1529 daoExplanationOfBenefitDstu3() { 1530 1531 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ExplanationOfBenefit> retVal; 1532 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ExplanationOfBenefit>(); 1533 retVal.setResourceType(org.hl7.fhir.dstu3.model.ExplanationOfBenefit.class); 1534 retVal.setContext(myFhirContext); 1535 return retVal; 1536 } 1537 1538 @Bean(name="myExplanationOfBenefitRpDstu3") 1539 @Lazy 1540 public ca.uhn.fhir.jpa.rp.dstu3.ExplanationOfBenefitResourceProvider rpExplanationOfBenefitDstu3() { 1541 ca.uhn.fhir.jpa.rp.dstu3.ExplanationOfBenefitResourceProvider retVal; 1542 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ExplanationOfBenefitResourceProvider(); 1543 retVal.setContext(myFhirContext); 1544 retVal.setDao(daoExplanationOfBenefitDstu3()); 1545 return retVal; 1546 } 1547 1548 @Bean(name="myFamilyMemberHistoryDaoDstu3") 1549 public 1550 IFhirResourceDao<org.hl7.fhir.dstu3.model.FamilyMemberHistory> 1551 daoFamilyMemberHistoryDstu3() { 1552 1553 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.FamilyMemberHistory> retVal; 1554 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.FamilyMemberHistory>(); 1555 retVal.setResourceType(org.hl7.fhir.dstu3.model.FamilyMemberHistory.class); 1556 retVal.setContext(myFhirContext); 1557 return retVal; 1558 } 1559 1560 @Bean(name="myFamilyMemberHistoryRpDstu3") 1561 @Lazy 1562 public ca.uhn.fhir.jpa.rp.dstu3.FamilyMemberHistoryResourceProvider rpFamilyMemberHistoryDstu3() { 1563 ca.uhn.fhir.jpa.rp.dstu3.FamilyMemberHistoryResourceProvider retVal; 1564 retVal = new ca.uhn.fhir.jpa.rp.dstu3.FamilyMemberHistoryResourceProvider(); 1565 retVal.setContext(myFhirContext); 1566 retVal.setDao(daoFamilyMemberHistoryDstu3()); 1567 return retVal; 1568 } 1569 1570 @Bean(name="myFlagDaoDstu3") 1571 public 1572 IFhirResourceDao<org.hl7.fhir.dstu3.model.Flag> 1573 daoFlagDstu3() { 1574 1575 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Flag> retVal; 1576 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Flag>(); 1577 retVal.setResourceType(org.hl7.fhir.dstu3.model.Flag.class); 1578 retVal.setContext(myFhirContext); 1579 return retVal; 1580 } 1581 1582 @Bean(name="myFlagRpDstu3") 1583 @Lazy 1584 public ca.uhn.fhir.jpa.rp.dstu3.FlagResourceProvider rpFlagDstu3() { 1585 ca.uhn.fhir.jpa.rp.dstu3.FlagResourceProvider retVal; 1586 retVal = new ca.uhn.fhir.jpa.rp.dstu3.FlagResourceProvider(); 1587 retVal.setContext(myFhirContext); 1588 retVal.setDao(daoFlagDstu3()); 1589 return retVal; 1590 } 1591 1592 @Bean(name="myGoalDaoDstu3") 1593 public 1594 IFhirResourceDao<org.hl7.fhir.dstu3.model.Goal> 1595 daoGoalDstu3() { 1596 1597 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Goal> retVal; 1598 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Goal>(); 1599 retVal.setResourceType(org.hl7.fhir.dstu3.model.Goal.class); 1600 retVal.setContext(myFhirContext); 1601 return retVal; 1602 } 1603 1604 @Bean(name="myGoalRpDstu3") 1605 @Lazy 1606 public ca.uhn.fhir.jpa.rp.dstu3.GoalResourceProvider rpGoalDstu3() { 1607 ca.uhn.fhir.jpa.rp.dstu3.GoalResourceProvider retVal; 1608 retVal = new ca.uhn.fhir.jpa.rp.dstu3.GoalResourceProvider(); 1609 retVal.setContext(myFhirContext); 1610 retVal.setDao(daoGoalDstu3()); 1611 return retVal; 1612 } 1613 1614 @Bean(name="myGraphDefinitionDaoDstu3") 1615 public 1616 IFhirResourceDao<org.hl7.fhir.dstu3.model.GraphDefinition> 1617 daoGraphDefinitionDstu3() { 1618 1619 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.GraphDefinition> retVal; 1620 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.GraphDefinition>(); 1621 retVal.setResourceType(org.hl7.fhir.dstu3.model.GraphDefinition.class); 1622 retVal.setContext(myFhirContext); 1623 return retVal; 1624 } 1625 1626 @Bean(name="myGraphDefinitionRpDstu3") 1627 @Lazy 1628 public ca.uhn.fhir.jpa.rp.dstu3.GraphDefinitionResourceProvider rpGraphDefinitionDstu3() { 1629 ca.uhn.fhir.jpa.rp.dstu3.GraphDefinitionResourceProvider retVal; 1630 retVal = new ca.uhn.fhir.jpa.rp.dstu3.GraphDefinitionResourceProvider(); 1631 retVal.setContext(myFhirContext); 1632 retVal.setDao(daoGraphDefinitionDstu3()); 1633 return retVal; 1634 } 1635 1636 @Bean(name="myGroupDaoDstu3") 1637 public 1638 IFhirResourceDao<org.hl7.fhir.dstu3.model.Group> 1639 daoGroupDstu3() { 1640 1641 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Group> retVal; 1642 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Group>(); 1643 retVal.setResourceType(org.hl7.fhir.dstu3.model.Group.class); 1644 retVal.setContext(myFhirContext); 1645 return retVal; 1646 } 1647 1648 @Bean(name="myGroupRpDstu3") 1649 @Lazy 1650 public ca.uhn.fhir.jpa.rp.dstu3.GroupResourceProvider rpGroupDstu3() { 1651 ca.uhn.fhir.jpa.rp.dstu3.GroupResourceProvider retVal; 1652 retVal = new ca.uhn.fhir.jpa.rp.dstu3.GroupResourceProvider(); 1653 retVal.setContext(myFhirContext); 1654 retVal.setDao(daoGroupDstu3()); 1655 return retVal; 1656 } 1657 1658 @Bean(name="myGuidanceResponseDaoDstu3") 1659 public 1660 IFhirResourceDao<org.hl7.fhir.dstu3.model.GuidanceResponse> 1661 daoGuidanceResponseDstu3() { 1662 1663 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.GuidanceResponse> retVal; 1664 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.GuidanceResponse>(); 1665 retVal.setResourceType(org.hl7.fhir.dstu3.model.GuidanceResponse.class); 1666 retVal.setContext(myFhirContext); 1667 return retVal; 1668 } 1669 1670 @Bean(name="myGuidanceResponseRpDstu3") 1671 @Lazy 1672 public ca.uhn.fhir.jpa.rp.dstu3.GuidanceResponseResourceProvider rpGuidanceResponseDstu3() { 1673 ca.uhn.fhir.jpa.rp.dstu3.GuidanceResponseResourceProvider retVal; 1674 retVal = new ca.uhn.fhir.jpa.rp.dstu3.GuidanceResponseResourceProvider(); 1675 retVal.setContext(myFhirContext); 1676 retVal.setDao(daoGuidanceResponseDstu3()); 1677 return retVal; 1678 } 1679 1680 @Bean(name="myHealthcareServiceDaoDstu3") 1681 public 1682 IFhirResourceDao<org.hl7.fhir.dstu3.model.HealthcareService> 1683 daoHealthcareServiceDstu3() { 1684 1685 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.HealthcareService> retVal; 1686 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.HealthcareService>(); 1687 retVal.setResourceType(org.hl7.fhir.dstu3.model.HealthcareService.class); 1688 retVal.setContext(myFhirContext); 1689 return retVal; 1690 } 1691 1692 @Bean(name="myHealthcareServiceRpDstu3") 1693 @Lazy 1694 public ca.uhn.fhir.jpa.rp.dstu3.HealthcareServiceResourceProvider rpHealthcareServiceDstu3() { 1695 ca.uhn.fhir.jpa.rp.dstu3.HealthcareServiceResourceProvider retVal; 1696 retVal = new ca.uhn.fhir.jpa.rp.dstu3.HealthcareServiceResourceProvider(); 1697 retVal.setContext(myFhirContext); 1698 retVal.setDao(daoHealthcareServiceDstu3()); 1699 return retVal; 1700 } 1701 1702 @Bean(name="myImagingManifestDaoDstu3") 1703 public 1704 IFhirResourceDao<org.hl7.fhir.dstu3.model.ImagingManifest> 1705 daoImagingManifestDstu3() { 1706 1707 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ImagingManifest> retVal; 1708 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ImagingManifest>(); 1709 retVal.setResourceType(org.hl7.fhir.dstu3.model.ImagingManifest.class); 1710 retVal.setContext(myFhirContext); 1711 return retVal; 1712 } 1713 1714 @Bean(name="myImagingManifestRpDstu3") 1715 @Lazy 1716 public ca.uhn.fhir.jpa.rp.dstu3.ImagingManifestResourceProvider rpImagingManifestDstu3() { 1717 ca.uhn.fhir.jpa.rp.dstu3.ImagingManifestResourceProvider retVal; 1718 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ImagingManifestResourceProvider(); 1719 retVal.setContext(myFhirContext); 1720 retVal.setDao(daoImagingManifestDstu3()); 1721 return retVal; 1722 } 1723 1724 @Bean(name="myImagingStudyDaoDstu3") 1725 public 1726 IFhirResourceDao<org.hl7.fhir.dstu3.model.ImagingStudy> 1727 daoImagingStudyDstu3() { 1728 1729 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ImagingStudy> retVal; 1730 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ImagingStudy>(); 1731 retVal.setResourceType(org.hl7.fhir.dstu3.model.ImagingStudy.class); 1732 retVal.setContext(myFhirContext); 1733 return retVal; 1734 } 1735 1736 @Bean(name="myImagingStudyRpDstu3") 1737 @Lazy 1738 public ca.uhn.fhir.jpa.rp.dstu3.ImagingStudyResourceProvider rpImagingStudyDstu3() { 1739 ca.uhn.fhir.jpa.rp.dstu3.ImagingStudyResourceProvider retVal; 1740 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ImagingStudyResourceProvider(); 1741 retVal.setContext(myFhirContext); 1742 retVal.setDao(daoImagingStudyDstu3()); 1743 return retVal; 1744 } 1745 1746 @Bean(name="myImmunizationDaoDstu3") 1747 public 1748 IFhirResourceDao<org.hl7.fhir.dstu3.model.Immunization> 1749 daoImmunizationDstu3() { 1750 1751 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Immunization> retVal; 1752 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Immunization>(); 1753 retVal.setResourceType(org.hl7.fhir.dstu3.model.Immunization.class); 1754 retVal.setContext(myFhirContext); 1755 return retVal; 1756 } 1757 1758 @Bean(name="myImmunizationRpDstu3") 1759 @Lazy 1760 public ca.uhn.fhir.jpa.rp.dstu3.ImmunizationResourceProvider rpImmunizationDstu3() { 1761 ca.uhn.fhir.jpa.rp.dstu3.ImmunizationResourceProvider retVal; 1762 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ImmunizationResourceProvider(); 1763 retVal.setContext(myFhirContext); 1764 retVal.setDao(daoImmunizationDstu3()); 1765 return retVal; 1766 } 1767 1768 @Bean(name="myImmunizationRecommendationDaoDstu3") 1769 public 1770 IFhirResourceDao<org.hl7.fhir.dstu3.model.ImmunizationRecommendation> 1771 daoImmunizationRecommendationDstu3() { 1772 1773 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ImmunizationRecommendation> retVal; 1774 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ImmunizationRecommendation>(); 1775 retVal.setResourceType(org.hl7.fhir.dstu3.model.ImmunizationRecommendation.class); 1776 retVal.setContext(myFhirContext); 1777 return retVal; 1778 } 1779 1780 @Bean(name="myImmunizationRecommendationRpDstu3") 1781 @Lazy 1782 public ca.uhn.fhir.jpa.rp.dstu3.ImmunizationRecommendationResourceProvider rpImmunizationRecommendationDstu3() { 1783 ca.uhn.fhir.jpa.rp.dstu3.ImmunizationRecommendationResourceProvider retVal; 1784 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ImmunizationRecommendationResourceProvider(); 1785 retVal.setContext(myFhirContext); 1786 retVal.setDao(daoImmunizationRecommendationDstu3()); 1787 return retVal; 1788 } 1789 1790 @Bean(name="myImplementationGuideDaoDstu3") 1791 public 1792 IFhirResourceDao<org.hl7.fhir.dstu3.model.ImplementationGuide> 1793 daoImplementationGuideDstu3() { 1794 1795 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ImplementationGuide> retVal; 1796 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ImplementationGuide>(); 1797 retVal.setResourceType(org.hl7.fhir.dstu3.model.ImplementationGuide.class); 1798 retVal.setContext(myFhirContext); 1799 return retVal; 1800 } 1801 1802 @Bean(name="myImplementationGuideRpDstu3") 1803 @Lazy 1804 public ca.uhn.fhir.jpa.rp.dstu3.ImplementationGuideResourceProvider rpImplementationGuideDstu3() { 1805 ca.uhn.fhir.jpa.rp.dstu3.ImplementationGuideResourceProvider retVal; 1806 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ImplementationGuideResourceProvider(); 1807 retVal.setContext(myFhirContext); 1808 retVal.setDao(daoImplementationGuideDstu3()); 1809 return retVal; 1810 } 1811 1812 @Bean(name="myLibraryDaoDstu3") 1813 public 1814 IFhirResourceDao<org.hl7.fhir.dstu3.model.Library> 1815 daoLibraryDstu3() { 1816 1817 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Library> retVal; 1818 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Library>(); 1819 retVal.setResourceType(org.hl7.fhir.dstu3.model.Library.class); 1820 retVal.setContext(myFhirContext); 1821 return retVal; 1822 } 1823 1824 @Bean(name="myLibraryRpDstu3") 1825 @Lazy 1826 public ca.uhn.fhir.jpa.rp.dstu3.LibraryResourceProvider rpLibraryDstu3() { 1827 ca.uhn.fhir.jpa.rp.dstu3.LibraryResourceProvider retVal; 1828 retVal = new ca.uhn.fhir.jpa.rp.dstu3.LibraryResourceProvider(); 1829 retVal.setContext(myFhirContext); 1830 retVal.setDao(daoLibraryDstu3()); 1831 return retVal; 1832 } 1833 1834 @Bean(name="myLinkageDaoDstu3") 1835 public 1836 IFhirResourceDao<org.hl7.fhir.dstu3.model.Linkage> 1837 daoLinkageDstu3() { 1838 1839 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Linkage> retVal; 1840 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Linkage>(); 1841 retVal.setResourceType(org.hl7.fhir.dstu3.model.Linkage.class); 1842 retVal.setContext(myFhirContext); 1843 return retVal; 1844 } 1845 1846 @Bean(name="myLinkageRpDstu3") 1847 @Lazy 1848 public ca.uhn.fhir.jpa.rp.dstu3.LinkageResourceProvider rpLinkageDstu3() { 1849 ca.uhn.fhir.jpa.rp.dstu3.LinkageResourceProvider retVal; 1850 retVal = new ca.uhn.fhir.jpa.rp.dstu3.LinkageResourceProvider(); 1851 retVal.setContext(myFhirContext); 1852 retVal.setDao(daoLinkageDstu3()); 1853 return retVal; 1854 } 1855 1856 @Bean(name="myListDaoDstu3") 1857 public 1858 IFhirResourceDao<org.hl7.fhir.dstu3.model.ListResource> 1859 daoListResourceDstu3() { 1860 1861 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ListResource> retVal; 1862 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ListResource>(); 1863 retVal.setResourceType(org.hl7.fhir.dstu3.model.ListResource.class); 1864 retVal.setContext(myFhirContext); 1865 return retVal; 1866 } 1867 1868 @Bean(name="myListResourceRpDstu3") 1869 @Lazy 1870 public ca.uhn.fhir.jpa.rp.dstu3.ListResourceResourceProvider rpListResourceDstu3() { 1871 ca.uhn.fhir.jpa.rp.dstu3.ListResourceResourceProvider retVal; 1872 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ListResourceResourceProvider(); 1873 retVal.setContext(myFhirContext); 1874 retVal.setDao(daoListResourceDstu3()); 1875 return retVal; 1876 } 1877 1878 @Bean(name="myLocationDaoDstu3") 1879 public 1880 IFhirResourceDao<org.hl7.fhir.dstu3.model.Location> 1881 daoLocationDstu3() { 1882 1883 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Location> retVal; 1884 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Location>(); 1885 retVal.setResourceType(org.hl7.fhir.dstu3.model.Location.class); 1886 retVal.setContext(myFhirContext); 1887 return retVal; 1888 } 1889 1890 @Bean(name="myLocationRpDstu3") 1891 @Lazy 1892 public ca.uhn.fhir.jpa.rp.dstu3.LocationResourceProvider rpLocationDstu3() { 1893 ca.uhn.fhir.jpa.rp.dstu3.LocationResourceProvider retVal; 1894 retVal = new ca.uhn.fhir.jpa.rp.dstu3.LocationResourceProvider(); 1895 retVal.setContext(myFhirContext); 1896 retVal.setDao(daoLocationDstu3()); 1897 return retVal; 1898 } 1899 1900 @Bean(name="myMeasureDaoDstu3") 1901 public 1902 IFhirResourceDao<org.hl7.fhir.dstu3.model.Measure> 1903 daoMeasureDstu3() { 1904 1905 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Measure> retVal; 1906 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Measure>(); 1907 retVal.setResourceType(org.hl7.fhir.dstu3.model.Measure.class); 1908 retVal.setContext(myFhirContext); 1909 return retVal; 1910 } 1911 1912 @Bean(name="myMeasureRpDstu3") 1913 @Lazy 1914 public ca.uhn.fhir.jpa.rp.dstu3.MeasureResourceProvider rpMeasureDstu3() { 1915 ca.uhn.fhir.jpa.rp.dstu3.MeasureResourceProvider retVal; 1916 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MeasureResourceProvider(); 1917 retVal.setContext(myFhirContext); 1918 retVal.setDao(daoMeasureDstu3()); 1919 return retVal; 1920 } 1921 1922 @Bean(name="myMeasureReportDaoDstu3") 1923 public 1924 IFhirResourceDao<org.hl7.fhir.dstu3.model.MeasureReport> 1925 daoMeasureReportDstu3() { 1926 1927 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.MeasureReport> retVal; 1928 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.MeasureReport>(); 1929 retVal.setResourceType(org.hl7.fhir.dstu3.model.MeasureReport.class); 1930 retVal.setContext(myFhirContext); 1931 return retVal; 1932 } 1933 1934 @Bean(name="myMeasureReportRpDstu3") 1935 @Lazy 1936 public ca.uhn.fhir.jpa.rp.dstu3.MeasureReportResourceProvider rpMeasureReportDstu3() { 1937 ca.uhn.fhir.jpa.rp.dstu3.MeasureReportResourceProvider retVal; 1938 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MeasureReportResourceProvider(); 1939 retVal.setContext(myFhirContext); 1940 retVal.setDao(daoMeasureReportDstu3()); 1941 return retVal; 1942 } 1943 1944 @Bean(name="myMediaDaoDstu3") 1945 public 1946 IFhirResourceDao<org.hl7.fhir.dstu3.model.Media> 1947 daoMediaDstu3() { 1948 1949 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Media> retVal; 1950 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Media>(); 1951 retVal.setResourceType(org.hl7.fhir.dstu3.model.Media.class); 1952 retVal.setContext(myFhirContext); 1953 return retVal; 1954 } 1955 1956 @Bean(name="myMediaRpDstu3") 1957 @Lazy 1958 public ca.uhn.fhir.jpa.rp.dstu3.MediaResourceProvider rpMediaDstu3() { 1959 ca.uhn.fhir.jpa.rp.dstu3.MediaResourceProvider retVal; 1960 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MediaResourceProvider(); 1961 retVal.setContext(myFhirContext); 1962 retVal.setDao(daoMediaDstu3()); 1963 return retVal; 1964 } 1965 1966 @Bean(name="myMedicationDaoDstu3") 1967 public 1968 IFhirResourceDao<org.hl7.fhir.dstu3.model.Medication> 1969 daoMedicationDstu3() { 1970 1971 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Medication> retVal; 1972 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Medication>(); 1973 retVal.setResourceType(org.hl7.fhir.dstu3.model.Medication.class); 1974 retVal.setContext(myFhirContext); 1975 return retVal; 1976 } 1977 1978 @Bean(name="myMedicationRpDstu3") 1979 @Lazy 1980 public ca.uhn.fhir.jpa.rp.dstu3.MedicationResourceProvider rpMedicationDstu3() { 1981 ca.uhn.fhir.jpa.rp.dstu3.MedicationResourceProvider retVal; 1982 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MedicationResourceProvider(); 1983 retVal.setContext(myFhirContext); 1984 retVal.setDao(daoMedicationDstu3()); 1985 return retVal; 1986 } 1987 1988 @Bean(name="myMedicationAdministrationDaoDstu3") 1989 public 1990 IFhirResourceDao<org.hl7.fhir.dstu3.model.MedicationAdministration> 1991 daoMedicationAdministrationDstu3() { 1992 1993 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.MedicationAdministration> retVal; 1994 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.MedicationAdministration>(); 1995 retVal.setResourceType(org.hl7.fhir.dstu3.model.MedicationAdministration.class); 1996 retVal.setContext(myFhirContext); 1997 return retVal; 1998 } 1999 2000 @Bean(name="myMedicationAdministrationRpDstu3") 2001 @Lazy 2002 public ca.uhn.fhir.jpa.rp.dstu3.MedicationAdministrationResourceProvider rpMedicationAdministrationDstu3() { 2003 ca.uhn.fhir.jpa.rp.dstu3.MedicationAdministrationResourceProvider retVal; 2004 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MedicationAdministrationResourceProvider(); 2005 retVal.setContext(myFhirContext); 2006 retVal.setDao(daoMedicationAdministrationDstu3()); 2007 return retVal; 2008 } 2009 2010 @Bean(name="myMedicationDispenseDaoDstu3") 2011 public 2012 IFhirResourceDao<org.hl7.fhir.dstu3.model.MedicationDispense> 2013 daoMedicationDispenseDstu3() { 2014 2015 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.MedicationDispense> retVal; 2016 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.MedicationDispense>(); 2017 retVal.setResourceType(org.hl7.fhir.dstu3.model.MedicationDispense.class); 2018 retVal.setContext(myFhirContext); 2019 return retVal; 2020 } 2021 2022 @Bean(name="myMedicationDispenseRpDstu3") 2023 @Lazy 2024 public ca.uhn.fhir.jpa.rp.dstu3.MedicationDispenseResourceProvider rpMedicationDispenseDstu3() { 2025 ca.uhn.fhir.jpa.rp.dstu3.MedicationDispenseResourceProvider retVal; 2026 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MedicationDispenseResourceProvider(); 2027 retVal.setContext(myFhirContext); 2028 retVal.setDao(daoMedicationDispenseDstu3()); 2029 return retVal; 2030 } 2031 2032 @Bean(name="myMedicationRequestDaoDstu3") 2033 public 2034 IFhirResourceDao<org.hl7.fhir.dstu3.model.MedicationRequest> 2035 daoMedicationRequestDstu3() { 2036 2037 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.MedicationRequest> retVal; 2038 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.MedicationRequest>(); 2039 retVal.setResourceType(org.hl7.fhir.dstu3.model.MedicationRequest.class); 2040 retVal.setContext(myFhirContext); 2041 return retVal; 2042 } 2043 2044 @Bean(name="myMedicationRequestRpDstu3") 2045 @Lazy 2046 public ca.uhn.fhir.jpa.rp.dstu3.MedicationRequestResourceProvider rpMedicationRequestDstu3() { 2047 ca.uhn.fhir.jpa.rp.dstu3.MedicationRequestResourceProvider retVal; 2048 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MedicationRequestResourceProvider(); 2049 retVal.setContext(myFhirContext); 2050 retVal.setDao(daoMedicationRequestDstu3()); 2051 return retVal; 2052 } 2053 2054 @Bean(name="myMedicationStatementDaoDstu3") 2055 public 2056 IFhirResourceDao<org.hl7.fhir.dstu3.model.MedicationStatement> 2057 daoMedicationStatementDstu3() { 2058 2059 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.MedicationStatement> retVal; 2060 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.MedicationStatement>(); 2061 retVal.setResourceType(org.hl7.fhir.dstu3.model.MedicationStatement.class); 2062 retVal.setContext(myFhirContext); 2063 return retVal; 2064 } 2065 2066 @Bean(name="myMedicationStatementRpDstu3") 2067 @Lazy 2068 public ca.uhn.fhir.jpa.rp.dstu3.MedicationStatementResourceProvider rpMedicationStatementDstu3() { 2069 ca.uhn.fhir.jpa.rp.dstu3.MedicationStatementResourceProvider retVal; 2070 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MedicationStatementResourceProvider(); 2071 retVal.setContext(myFhirContext); 2072 retVal.setDao(daoMedicationStatementDstu3()); 2073 return retVal; 2074 } 2075 2076 @Bean(name="myMessageDefinitionDaoDstu3") 2077 public 2078 IFhirResourceDao<org.hl7.fhir.dstu3.model.MessageDefinition> 2079 daoMessageDefinitionDstu3() { 2080 2081 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.MessageDefinition> retVal; 2082 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.MessageDefinition>(); 2083 retVal.setResourceType(org.hl7.fhir.dstu3.model.MessageDefinition.class); 2084 retVal.setContext(myFhirContext); 2085 return retVal; 2086 } 2087 2088 @Bean(name="myMessageDefinitionRpDstu3") 2089 @Lazy 2090 public ca.uhn.fhir.jpa.rp.dstu3.MessageDefinitionResourceProvider rpMessageDefinitionDstu3() { 2091 ca.uhn.fhir.jpa.rp.dstu3.MessageDefinitionResourceProvider retVal; 2092 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MessageDefinitionResourceProvider(); 2093 retVal.setContext(myFhirContext); 2094 retVal.setDao(daoMessageDefinitionDstu3()); 2095 return retVal; 2096 } 2097 2098 @Bean(name="myMessageHeaderDaoDstu3") 2099 public 2100 IFhirResourceDao<org.hl7.fhir.dstu3.model.MessageHeader> 2101 daoMessageHeaderDstu3() { 2102 2103 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.MessageHeader> retVal; 2104 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.MessageHeader>(); 2105 retVal.setResourceType(org.hl7.fhir.dstu3.model.MessageHeader.class); 2106 retVal.setContext(myFhirContext); 2107 return retVal; 2108 } 2109 2110 @Bean(name="myMessageHeaderRpDstu3") 2111 @Lazy 2112 public ca.uhn.fhir.jpa.rp.dstu3.MessageHeaderResourceProvider rpMessageHeaderDstu3() { 2113 ca.uhn.fhir.jpa.rp.dstu3.MessageHeaderResourceProvider retVal; 2114 retVal = new ca.uhn.fhir.jpa.rp.dstu3.MessageHeaderResourceProvider(); 2115 retVal.setContext(myFhirContext); 2116 retVal.setDao(daoMessageHeaderDstu3()); 2117 return retVal; 2118 } 2119 2120 @Bean(name="myNamingSystemDaoDstu3") 2121 public 2122 IFhirResourceDao<org.hl7.fhir.dstu3.model.NamingSystem> 2123 daoNamingSystemDstu3() { 2124 2125 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.NamingSystem> retVal; 2126 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.NamingSystem>(); 2127 retVal.setResourceType(org.hl7.fhir.dstu3.model.NamingSystem.class); 2128 retVal.setContext(myFhirContext); 2129 return retVal; 2130 } 2131 2132 @Bean(name="myNamingSystemRpDstu3") 2133 @Lazy 2134 public ca.uhn.fhir.jpa.rp.dstu3.NamingSystemResourceProvider rpNamingSystemDstu3() { 2135 ca.uhn.fhir.jpa.rp.dstu3.NamingSystemResourceProvider retVal; 2136 retVal = new ca.uhn.fhir.jpa.rp.dstu3.NamingSystemResourceProvider(); 2137 retVal.setContext(myFhirContext); 2138 retVal.setDao(daoNamingSystemDstu3()); 2139 return retVal; 2140 } 2141 2142 @Bean(name="myNutritionOrderDaoDstu3") 2143 public 2144 IFhirResourceDao<org.hl7.fhir.dstu3.model.NutritionOrder> 2145 daoNutritionOrderDstu3() { 2146 2147 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.NutritionOrder> retVal; 2148 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.NutritionOrder>(); 2149 retVal.setResourceType(org.hl7.fhir.dstu3.model.NutritionOrder.class); 2150 retVal.setContext(myFhirContext); 2151 return retVal; 2152 } 2153 2154 @Bean(name="myNutritionOrderRpDstu3") 2155 @Lazy 2156 public ca.uhn.fhir.jpa.rp.dstu3.NutritionOrderResourceProvider rpNutritionOrderDstu3() { 2157 ca.uhn.fhir.jpa.rp.dstu3.NutritionOrderResourceProvider retVal; 2158 retVal = new ca.uhn.fhir.jpa.rp.dstu3.NutritionOrderResourceProvider(); 2159 retVal.setContext(myFhirContext); 2160 retVal.setDao(daoNutritionOrderDstu3()); 2161 return retVal; 2162 } 2163 2164 @Bean(name="myObservationDaoDstu3") 2165 public 2166 IFhirResourceDaoObservation<org.hl7.fhir.dstu3.model.Observation> 2167 daoObservationDstu3() { 2168 2169 ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<org.hl7.fhir.dstu3.model.Observation> retVal; 2170 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<>(); 2171 retVal.setResourceType(org.hl7.fhir.dstu3.model.Observation.class); 2172 retVal.setContext(myFhirContext); 2173 return retVal; 2174 } 2175 2176 @Bean(name="myObservationRpDstu3") 2177 @Lazy 2178 public ca.uhn.fhir.jpa.rp.dstu3.ObservationResourceProvider rpObservationDstu3() { 2179 ca.uhn.fhir.jpa.rp.dstu3.ObservationResourceProvider retVal; 2180 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ObservationResourceProvider(); 2181 retVal.setContext(myFhirContext); 2182 retVal.setDao(daoObservationDstu3()); 2183 return retVal; 2184 } 2185 2186 @Bean(name="myOperationDefinitionDaoDstu3") 2187 public 2188 IFhirResourceDao<org.hl7.fhir.dstu3.model.OperationDefinition> 2189 daoOperationDefinitionDstu3() { 2190 2191 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.OperationDefinition> retVal; 2192 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.OperationDefinition>(); 2193 retVal.setResourceType(org.hl7.fhir.dstu3.model.OperationDefinition.class); 2194 retVal.setContext(myFhirContext); 2195 return retVal; 2196 } 2197 2198 @Bean(name="myOperationDefinitionRpDstu3") 2199 @Lazy 2200 public ca.uhn.fhir.jpa.rp.dstu3.OperationDefinitionResourceProvider rpOperationDefinitionDstu3() { 2201 ca.uhn.fhir.jpa.rp.dstu3.OperationDefinitionResourceProvider retVal; 2202 retVal = new ca.uhn.fhir.jpa.rp.dstu3.OperationDefinitionResourceProvider(); 2203 retVal.setContext(myFhirContext); 2204 retVal.setDao(daoOperationDefinitionDstu3()); 2205 return retVal; 2206 } 2207 2208 @Bean(name="myOperationOutcomeDaoDstu3") 2209 public 2210 IFhirResourceDao<org.hl7.fhir.dstu3.model.OperationOutcome> 2211 daoOperationOutcomeDstu3() { 2212 2213 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.OperationOutcome> retVal; 2214 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.OperationOutcome>(); 2215 retVal.setResourceType(org.hl7.fhir.dstu3.model.OperationOutcome.class); 2216 retVal.setContext(myFhirContext); 2217 return retVal; 2218 } 2219 2220 @Bean(name="myOperationOutcomeRpDstu3") 2221 @Lazy 2222 public ca.uhn.fhir.jpa.rp.dstu3.OperationOutcomeResourceProvider rpOperationOutcomeDstu3() { 2223 ca.uhn.fhir.jpa.rp.dstu3.OperationOutcomeResourceProvider retVal; 2224 retVal = new ca.uhn.fhir.jpa.rp.dstu3.OperationOutcomeResourceProvider(); 2225 retVal.setContext(myFhirContext); 2226 retVal.setDao(daoOperationOutcomeDstu3()); 2227 return retVal; 2228 } 2229 2230 @Bean(name="myOrganizationDaoDstu3") 2231 public 2232 IFhirResourceDao<org.hl7.fhir.dstu3.model.Organization> 2233 daoOrganizationDstu3() { 2234 2235 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Organization> retVal; 2236 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Organization>(); 2237 retVal.setResourceType(org.hl7.fhir.dstu3.model.Organization.class); 2238 retVal.setContext(myFhirContext); 2239 return retVal; 2240 } 2241 2242 @Bean(name="myOrganizationRpDstu3") 2243 @Lazy 2244 public ca.uhn.fhir.jpa.rp.dstu3.OrganizationResourceProvider rpOrganizationDstu3() { 2245 ca.uhn.fhir.jpa.rp.dstu3.OrganizationResourceProvider retVal; 2246 retVal = new ca.uhn.fhir.jpa.rp.dstu3.OrganizationResourceProvider(); 2247 retVal.setContext(myFhirContext); 2248 retVal.setDao(daoOrganizationDstu3()); 2249 return retVal; 2250 } 2251 2252 @Bean(name="myParametersDaoDstu3") 2253 public 2254 IFhirResourceDao<org.hl7.fhir.dstu3.model.Parameters> 2255 daoParametersDstu3() { 2256 2257 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Parameters> retVal; 2258 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Parameters>(); 2259 retVal.setResourceType(org.hl7.fhir.dstu3.model.Parameters.class); 2260 retVal.setContext(myFhirContext); 2261 return retVal; 2262 } 2263 2264 @Bean(name="myParametersRpDstu3") 2265 @Lazy 2266 public ca.uhn.fhir.jpa.rp.dstu3.ParametersResourceProvider rpParametersDstu3() { 2267 ca.uhn.fhir.jpa.rp.dstu3.ParametersResourceProvider retVal; 2268 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ParametersResourceProvider(); 2269 retVal.setContext(myFhirContext); 2270 retVal.setDao(daoParametersDstu3()); 2271 return retVal; 2272 } 2273 2274 @Bean(name="myPatientDaoDstu3") 2275 public 2276 IFhirResourceDaoPatient<org.hl7.fhir.dstu3.model.Patient> 2277 daoPatientDstu3() { 2278 2279 ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<org.hl7.fhir.dstu3.model.Patient> retVal; 2280 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<>(); 2281 retVal.setResourceType(org.hl7.fhir.dstu3.model.Patient.class); 2282 retVal.setContext(myFhirContext); 2283 return retVal; 2284 } 2285 2286 @Bean(name="myPatientRpDstu3") 2287 @Lazy 2288 public ca.uhn.fhir.jpa.rp.dstu3.PatientResourceProvider rpPatientDstu3() { 2289 ca.uhn.fhir.jpa.rp.dstu3.PatientResourceProvider retVal; 2290 retVal = new ca.uhn.fhir.jpa.rp.dstu3.PatientResourceProvider(); 2291 retVal.setContext(myFhirContext); 2292 retVal.setDao(daoPatientDstu3()); 2293 return retVal; 2294 } 2295 2296 @Bean(name="myPaymentNoticeDaoDstu3") 2297 public 2298 IFhirResourceDao<org.hl7.fhir.dstu3.model.PaymentNotice> 2299 daoPaymentNoticeDstu3() { 2300 2301 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.PaymentNotice> retVal; 2302 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.PaymentNotice>(); 2303 retVal.setResourceType(org.hl7.fhir.dstu3.model.PaymentNotice.class); 2304 retVal.setContext(myFhirContext); 2305 return retVal; 2306 } 2307 2308 @Bean(name="myPaymentNoticeRpDstu3") 2309 @Lazy 2310 public ca.uhn.fhir.jpa.rp.dstu3.PaymentNoticeResourceProvider rpPaymentNoticeDstu3() { 2311 ca.uhn.fhir.jpa.rp.dstu3.PaymentNoticeResourceProvider retVal; 2312 retVal = new ca.uhn.fhir.jpa.rp.dstu3.PaymentNoticeResourceProvider(); 2313 retVal.setContext(myFhirContext); 2314 retVal.setDao(daoPaymentNoticeDstu3()); 2315 return retVal; 2316 } 2317 2318 @Bean(name="myPaymentReconciliationDaoDstu3") 2319 public 2320 IFhirResourceDao<org.hl7.fhir.dstu3.model.PaymentReconciliation> 2321 daoPaymentReconciliationDstu3() { 2322 2323 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.PaymentReconciliation> retVal; 2324 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.PaymentReconciliation>(); 2325 retVal.setResourceType(org.hl7.fhir.dstu3.model.PaymentReconciliation.class); 2326 retVal.setContext(myFhirContext); 2327 return retVal; 2328 } 2329 2330 @Bean(name="myPaymentReconciliationRpDstu3") 2331 @Lazy 2332 public ca.uhn.fhir.jpa.rp.dstu3.PaymentReconciliationResourceProvider rpPaymentReconciliationDstu3() { 2333 ca.uhn.fhir.jpa.rp.dstu3.PaymentReconciliationResourceProvider retVal; 2334 retVal = new ca.uhn.fhir.jpa.rp.dstu3.PaymentReconciliationResourceProvider(); 2335 retVal.setContext(myFhirContext); 2336 retVal.setDao(daoPaymentReconciliationDstu3()); 2337 return retVal; 2338 } 2339 2340 @Bean(name="myPersonDaoDstu3") 2341 public 2342 IFhirResourceDao<org.hl7.fhir.dstu3.model.Person> 2343 daoPersonDstu3() { 2344 2345 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Person> retVal; 2346 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Person>(); 2347 retVal.setResourceType(org.hl7.fhir.dstu3.model.Person.class); 2348 retVal.setContext(myFhirContext); 2349 return retVal; 2350 } 2351 2352 @Bean(name="myPersonRpDstu3") 2353 @Lazy 2354 public ca.uhn.fhir.jpa.rp.dstu3.PersonResourceProvider rpPersonDstu3() { 2355 ca.uhn.fhir.jpa.rp.dstu3.PersonResourceProvider retVal; 2356 retVal = new ca.uhn.fhir.jpa.rp.dstu3.PersonResourceProvider(); 2357 retVal.setContext(myFhirContext); 2358 retVal.setDao(daoPersonDstu3()); 2359 return retVal; 2360 } 2361 2362 @Bean(name="myPlanDefinitionDaoDstu3") 2363 public 2364 IFhirResourceDao<org.hl7.fhir.dstu3.model.PlanDefinition> 2365 daoPlanDefinitionDstu3() { 2366 2367 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.PlanDefinition> retVal; 2368 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.PlanDefinition>(); 2369 retVal.setResourceType(org.hl7.fhir.dstu3.model.PlanDefinition.class); 2370 retVal.setContext(myFhirContext); 2371 return retVal; 2372 } 2373 2374 @Bean(name="myPlanDefinitionRpDstu3") 2375 @Lazy 2376 public ca.uhn.fhir.jpa.rp.dstu3.PlanDefinitionResourceProvider rpPlanDefinitionDstu3() { 2377 ca.uhn.fhir.jpa.rp.dstu3.PlanDefinitionResourceProvider retVal; 2378 retVal = new ca.uhn.fhir.jpa.rp.dstu3.PlanDefinitionResourceProvider(); 2379 retVal.setContext(myFhirContext); 2380 retVal.setDao(daoPlanDefinitionDstu3()); 2381 return retVal; 2382 } 2383 2384 @Bean(name="myPractitionerDaoDstu3") 2385 public 2386 IFhirResourceDao<org.hl7.fhir.dstu3.model.Practitioner> 2387 daoPractitionerDstu3() { 2388 2389 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Practitioner> retVal; 2390 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Practitioner>(); 2391 retVal.setResourceType(org.hl7.fhir.dstu3.model.Practitioner.class); 2392 retVal.setContext(myFhirContext); 2393 return retVal; 2394 } 2395 2396 @Bean(name="myPractitionerRpDstu3") 2397 @Lazy 2398 public ca.uhn.fhir.jpa.rp.dstu3.PractitionerResourceProvider rpPractitionerDstu3() { 2399 ca.uhn.fhir.jpa.rp.dstu3.PractitionerResourceProvider retVal; 2400 retVal = new ca.uhn.fhir.jpa.rp.dstu3.PractitionerResourceProvider(); 2401 retVal.setContext(myFhirContext); 2402 retVal.setDao(daoPractitionerDstu3()); 2403 return retVal; 2404 } 2405 2406 @Bean(name="myPractitionerRoleDaoDstu3") 2407 public 2408 IFhirResourceDao<org.hl7.fhir.dstu3.model.PractitionerRole> 2409 daoPractitionerRoleDstu3() { 2410 2411 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.PractitionerRole> retVal; 2412 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.PractitionerRole>(); 2413 retVal.setResourceType(org.hl7.fhir.dstu3.model.PractitionerRole.class); 2414 retVal.setContext(myFhirContext); 2415 return retVal; 2416 } 2417 2418 @Bean(name="myPractitionerRoleRpDstu3") 2419 @Lazy 2420 public ca.uhn.fhir.jpa.rp.dstu3.PractitionerRoleResourceProvider rpPractitionerRoleDstu3() { 2421 ca.uhn.fhir.jpa.rp.dstu3.PractitionerRoleResourceProvider retVal; 2422 retVal = new ca.uhn.fhir.jpa.rp.dstu3.PractitionerRoleResourceProvider(); 2423 retVal.setContext(myFhirContext); 2424 retVal.setDao(daoPractitionerRoleDstu3()); 2425 return retVal; 2426 } 2427 2428 @Bean(name="myProcedureDaoDstu3") 2429 public 2430 IFhirResourceDao<org.hl7.fhir.dstu3.model.Procedure> 2431 daoProcedureDstu3() { 2432 2433 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Procedure> retVal; 2434 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Procedure>(); 2435 retVal.setResourceType(org.hl7.fhir.dstu3.model.Procedure.class); 2436 retVal.setContext(myFhirContext); 2437 return retVal; 2438 } 2439 2440 @Bean(name="myProcedureRpDstu3") 2441 @Lazy 2442 public ca.uhn.fhir.jpa.rp.dstu3.ProcedureResourceProvider rpProcedureDstu3() { 2443 ca.uhn.fhir.jpa.rp.dstu3.ProcedureResourceProvider retVal; 2444 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ProcedureResourceProvider(); 2445 retVal.setContext(myFhirContext); 2446 retVal.setDao(daoProcedureDstu3()); 2447 return retVal; 2448 } 2449 2450 @Bean(name="myProcedureRequestDaoDstu3") 2451 public 2452 IFhirResourceDao<org.hl7.fhir.dstu3.model.ProcedureRequest> 2453 daoProcedureRequestDstu3() { 2454 2455 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ProcedureRequest> retVal; 2456 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ProcedureRequest>(); 2457 retVal.setResourceType(org.hl7.fhir.dstu3.model.ProcedureRequest.class); 2458 retVal.setContext(myFhirContext); 2459 return retVal; 2460 } 2461 2462 @Bean(name="myProcedureRequestRpDstu3") 2463 @Lazy 2464 public ca.uhn.fhir.jpa.rp.dstu3.ProcedureRequestResourceProvider rpProcedureRequestDstu3() { 2465 ca.uhn.fhir.jpa.rp.dstu3.ProcedureRequestResourceProvider retVal; 2466 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ProcedureRequestResourceProvider(); 2467 retVal.setContext(myFhirContext); 2468 retVal.setDao(daoProcedureRequestDstu3()); 2469 return retVal; 2470 } 2471 2472 @Bean(name="myProcessRequestDaoDstu3") 2473 public 2474 IFhirResourceDao<org.hl7.fhir.dstu3.model.ProcessRequest> 2475 daoProcessRequestDstu3() { 2476 2477 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ProcessRequest> retVal; 2478 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ProcessRequest>(); 2479 retVal.setResourceType(org.hl7.fhir.dstu3.model.ProcessRequest.class); 2480 retVal.setContext(myFhirContext); 2481 return retVal; 2482 } 2483 2484 @Bean(name="myProcessRequestRpDstu3") 2485 @Lazy 2486 public ca.uhn.fhir.jpa.rp.dstu3.ProcessRequestResourceProvider rpProcessRequestDstu3() { 2487 ca.uhn.fhir.jpa.rp.dstu3.ProcessRequestResourceProvider retVal; 2488 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ProcessRequestResourceProvider(); 2489 retVal.setContext(myFhirContext); 2490 retVal.setDao(daoProcessRequestDstu3()); 2491 return retVal; 2492 } 2493 2494 @Bean(name="myProcessResponseDaoDstu3") 2495 public 2496 IFhirResourceDao<org.hl7.fhir.dstu3.model.ProcessResponse> 2497 daoProcessResponseDstu3() { 2498 2499 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ProcessResponse> retVal; 2500 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ProcessResponse>(); 2501 retVal.setResourceType(org.hl7.fhir.dstu3.model.ProcessResponse.class); 2502 retVal.setContext(myFhirContext); 2503 return retVal; 2504 } 2505 2506 @Bean(name="myProcessResponseRpDstu3") 2507 @Lazy 2508 public ca.uhn.fhir.jpa.rp.dstu3.ProcessResponseResourceProvider rpProcessResponseDstu3() { 2509 ca.uhn.fhir.jpa.rp.dstu3.ProcessResponseResourceProvider retVal; 2510 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ProcessResponseResourceProvider(); 2511 retVal.setContext(myFhirContext); 2512 retVal.setDao(daoProcessResponseDstu3()); 2513 return retVal; 2514 } 2515 2516 @Bean(name="myProvenanceDaoDstu3") 2517 public 2518 IFhirResourceDao<org.hl7.fhir.dstu3.model.Provenance> 2519 daoProvenanceDstu3() { 2520 2521 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Provenance> retVal; 2522 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Provenance>(); 2523 retVal.setResourceType(org.hl7.fhir.dstu3.model.Provenance.class); 2524 retVal.setContext(myFhirContext); 2525 return retVal; 2526 } 2527 2528 @Bean(name="myProvenanceRpDstu3") 2529 @Lazy 2530 public ca.uhn.fhir.jpa.rp.dstu3.ProvenanceResourceProvider rpProvenanceDstu3() { 2531 ca.uhn.fhir.jpa.rp.dstu3.ProvenanceResourceProvider retVal; 2532 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ProvenanceResourceProvider(); 2533 retVal.setContext(myFhirContext); 2534 retVal.setDao(daoProvenanceDstu3()); 2535 return retVal; 2536 } 2537 2538 @Bean(name="myQuestionnaireDaoDstu3") 2539 public 2540 IFhirResourceDao<org.hl7.fhir.dstu3.model.Questionnaire> 2541 daoQuestionnaireDstu3() { 2542 2543 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Questionnaire> retVal; 2544 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Questionnaire>(); 2545 retVal.setResourceType(org.hl7.fhir.dstu3.model.Questionnaire.class); 2546 retVal.setContext(myFhirContext); 2547 return retVal; 2548 } 2549 2550 @Bean(name="myQuestionnaireRpDstu3") 2551 @Lazy 2552 public ca.uhn.fhir.jpa.rp.dstu3.QuestionnaireResourceProvider rpQuestionnaireDstu3() { 2553 ca.uhn.fhir.jpa.rp.dstu3.QuestionnaireResourceProvider retVal; 2554 retVal = new ca.uhn.fhir.jpa.rp.dstu3.QuestionnaireResourceProvider(); 2555 retVal.setContext(myFhirContext); 2556 retVal.setDao(daoQuestionnaireDstu3()); 2557 return retVal; 2558 } 2559 2560 @Bean(name="myQuestionnaireResponseDaoDstu3") 2561 public 2562 IFhirResourceDao<org.hl7.fhir.dstu3.model.QuestionnaireResponse> 2563 daoQuestionnaireResponseDstu3() { 2564 2565 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.QuestionnaireResponse> retVal; 2566 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.QuestionnaireResponse>(); 2567 retVal.setResourceType(org.hl7.fhir.dstu3.model.QuestionnaireResponse.class); 2568 retVal.setContext(myFhirContext); 2569 return retVal; 2570 } 2571 2572 @Bean(name="myQuestionnaireResponseRpDstu3") 2573 @Lazy 2574 public ca.uhn.fhir.jpa.rp.dstu3.QuestionnaireResponseResourceProvider rpQuestionnaireResponseDstu3() { 2575 ca.uhn.fhir.jpa.rp.dstu3.QuestionnaireResponseResourceProvider retVal; 2576 retVal = new ca.uhn.fhir.jpa.rp.dstu3.QuestionnaireResponseResourceProvider(); 2577 retVal.setContext(myFhirContext); 2578 retVal.setDao(daoQuestionnaireResponseDstu3()); 2579 return retVal; 2580 } 2581 2582 @Bean(name="myReferralRequestDaoDstu3") 2583 public 2584 IFhirResourceDao<org.hl7.fhir.dstu3.model.ReferralRequest> 2585 daoReferralRequestDstu3() { 2586 2587 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ReferralRequest> retVal; 2588 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ReferralRequest>(); 2589 retVal.setResourceType(org.hl7.fhir.dstu3.model.ReferralRequest.class); 2590 retVal.setContext(myFhirContext); 2591 return retVal; 2592 } 2593 2594 @Bean(name="myReferralRequestRpDstu3") 2595 @Lazy 2596 public ca.uhn.fhir.jpa.rp.dstu3.ReferralRequestResourceProvider rpReferralRequestDstu3() { 2597 ca.uhn.fhir.jpa.rp.dstu3.ReferralRequestResourceProvider retVal; 2598 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ReferralRequestResourceProvider(); 2599 retVal.setContext(myFhirContext); 2600 retVal.setDao(daoReferralRequestDstu3()); 2601 return retVal; 2602 } 2603 2604 @Bean(name="myRelatedPersonDaoDstu3") 2605 public 2606 IFhirResourceDao<org.hl7.fhir.dstu3.model.RelatedPerson> 2607 daoRelatedPersonDstu3() { 2608 2609 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.RelatedPerson> retVal; 2610 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.RelatedPerson>(); 2611 retVal.setResourceType(org.hl7.fhir.dstu3.model.RelatedPerson.class); 2612 retVal.setContext(myFhirContext); 2613 return retVal; 2614 } 2615 2616 @Bean(name="myRelatedPersonRpDstu3") 2617 @Lazy 2618 public ca.uhn.fhir.jpa.rp.dstu3.RelatedPersonResourceProvider rpRelatedPersonDstu3() { 2619 ca.uhn.fhir.jpa.rp.dstu3.RelatedPersonResourceProvider retVal; 2620 retVal = new ca.uhn.fhir.jpa.rp.dstu3.RelatedPersonResourceProvider(); 2621 retVal.setContext(myFhirContext); 2622 retVal.setDao(daoRelatedPersonDstu3()); 2623 return retVal; 2624 } 2625 2626 @Bean(name="myRequestGroupDaoDstu3") 2627 public 2628 IFhirResourceDao<org.hl7.fhir.dstu3.model.RequestGroup> 2629 daoRequestGroupDstu3() { 2630 2631 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.RequestGroup> retVal; 2632 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.RequestGroup>(); 2633 retVal.setResourceType(org.hl7.fhir.dstu3.model.RequestGroup.class); 2634 retVal.setContext(myFhirContext); 2635 return retVal; 2636 } 2637 2638 @Bean(name="myRequestGroupRpDstu3") 2639 @Lazy 2640 public ca.uhn.fhir.jpa.rp.dstu3.RequestGroupResourceProvider rpRequestGroupDstu3() { 2641 ca.uhn.fhir.jpa.rp.dstu3.RequestGroupResourceProvider retVal; 2642 retVal = new ca.uhn.fhir.jpa.rp.dstu3.RequestGroupResourceProvider(); 2643 retVal.setContext(myFhirContext); 2644 retVal.setDao(daoRequestGroupDstu3()); 2645 return retVal; 2646 } 2647 2648 @Bean(name="myResearchStudyDaoDstu3") 2649 public 2650 IFhirResourceDao<org.hl7.fhir.dstu3.model.ResearchStudy> 2651 daoResearchStudyDstu3() { 2652 2653 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ResearchStudy> retVal; 2654 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ResearchStudy>(); 2655 retVal.setResourceType(org.hl7.fhir.dstu3.model.ResearchStudy.class); 2656 retVal.setContext(myFhirContext); 2657 return retVal; 2658 } 2659 2660 @Bean(name="myResearchStudyRpDstu3") 2661 @Lazy 2662 public ca.uhn.fhir.jpa.rp.dstu3.ResearchStudyResourceProvider rpResearchStudyDstu3() { 2663 ca.uhn.fhir.jpa.rp.dstu3.ResearchStudyResourceProvider retVal; 2664 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ResearchStudyResourceProvider(); 2665 retVal.setContext(myFhirContext); 2666 retVal.setDao(daoResearchStudyDstu3()); 2667 return retVal; 2668 } 2669 2670 @Bean(name="myResearchSubjectDaoDstu3") 2671 public 2672 IFhirResourceDao<org.hl7.fhir.dstu3.model.ResearchSubject> 2673 daoResearchSubjectDstu3() { 2674 2675 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ResearchSubject> retVal; 2676 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ResearchSubject>(); 2677 retVal.setResourceType(org.hl7.fhir.dstu3.model.ResearchSubject.class); 2678 retVal.setContext(myFhirContext); 2679 return retVal; 2680 } 2681 2682 @Bean(name="myResearchSubjectRpDstu3") 2683 @Lazy 2684 public ca.uhn.fhir.jpa.rp.dstu3.ResearchSubjectResourceProvider rpResearchSubjectDstu3() { 2685 ca.uhn.fhir.jpa.rp.dstu3.ResearchSubjectResourceProvider retVal; 2686 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ResearchSubjectResourceProvider(); 2687 retVal.setContext(myFhirContext); 2688 retVal.setDao(daoResearchSubjectDstu3()); 2689 return retVal; 2690 } 2691 2692 @Bean(name="myRiskAssessmentDaoDstu3") 2693 public 2694 IFhirResourceDao<org.hl7.fhir.dstu3.model.RiskAssessment> 2695 daoRiskAssessmentDstu3() { 2696 2697 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.RiskAssessment> retVal; 2698 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.RiskAssessment>(); 2699 retVal.setResourceType(org.hl7.fhir.dstu3.model.RiskAssessment.class); 2700 retVal.setContext(myFhirContext); 2701 return retVal; 2702 } 2703 2704 @Bean(name="myRiskAssessmentRpDstu3") 2705 @Lazy 2706 public ca.uhn.fhir.jpa.rp.dstu3.RiskAssessmentResourceProvider rpRiskAssessmentDstu3() { 2707 ca.uhn.fhir.jpa.rp.dstu3.RiskAssessmentResourceProvider retVal; 2708 retVal = new ca.uhn.fhir.jpa.rp.dstu3.RiskAssessmentResourceProvider(); 2709 retVal.setContext(myFhirContext); 2710 retVal.setDao(daoRiskAssessmentDstu3()); 2711 return retVal; 2712 } 2713 2714 @Bean(name="myScheduleDaoDstu3") 2715 public 2716 IFhirResourceDao<org.hl7.fhir.dstu3.model.Schedule> 2717 daoScheduleDstu3() { 2718 2719 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Schedule> retVal; 2720 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Schedule>(); 2721 retVal.setResourceType(org.hl7.fhir.dstu3.model.Schedule.class); 2722 retVal.setContext(myFhirContext); 2723 return retVal; 2724 } 2725 2726 @Bean(name="myScheduleRpDstu3") 2727 @Lazy 2728 public ca.uhn.fhir.jpa.rp.dstu3.ScheduleResourceProvider rpScheduleDstu3() { 2729 ca.uhn.fhir.jpa.rp.dstu3.ScheduleResourceProvider retVal; 2730 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ScheduleResourceProvider(); 2731 retVal.setContext(myFhirContext); 2732 retVal.setDao(daoScheduleDstu3()); 2733 return retVal; 2734 } 2735 2736 @Bean(name="mySearchParameterDaoDstu3") 2737 public 2738 IFhirResourceDaoSearchParameter<org.hl7.fhir.dstu3.model.SearchParameter> 2739 daoSearchParameterDstu3() { 2740 2741 ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<org.hl7.fhir.dstu3.model.SearchParameter> retVal; 2742 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<>(); 2743 retVal.setResourceType(org.hl7.fhir.dstu3.model.SearchParameter.class); 2744 retVal.setContext(myFhirContext); 2745 return retVal; 2746 } 2747 2748 @Bean(name="mySearchParameterRpDstu3") 2749 @Lazy 2750 public ca.uhn.fhir.jpa.rp.dstu3.SearchParameterResourceProvider rpSearchParameterDstu3() { 2751 ca.uhn.fhir.jpa.rp.dstu3.SearchParameterResourceProvider retVal; 2752 retVal = new ca.uhn.fhir.jpa.rp.dstu3.SearchParameterResourceProvider(); 2753 retVal.setContext(myFhirContext); 2754 retVal.setDao(daoSearchParameterDstu3()); 2755 return retVal; 2756 } 2757 2758 @Bean(name="mySequenceDaoDstu3") 2759 public 2760 IFhirResourceDao<org.hl7.fhir.dstu3.model.Sequence> 2761 daoSequenceDstu3() { 2762 2763 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Sequence> retVal; 2764 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Sequence>(); 2765 retVal.setResourceType(org.hl7.fhir.dstu3.model.Sequence.class); 2766 retVal.setContext(myFhirContext); 2767 return retVal; 2768 } 2769 2770 @Bean(name="mySequenceRpDstu3") 2771 @Lazy 2772 public ca.uhn.fhir.jpa.rp.dstu3.SequenceResourceProvider rpSequenceDstu3() { 2773 ca.uhn.fhir.jpa.rp.dstu3.SequenceResourceProvider retVal; 2774 retVal = new ca.uhn.fhir.jpa.rp.dstu3.SequenceResourceProvider(); 2775 retVal.setContext(myFhirContext); 2776 retVal.setDao(daoSequenceDstu3()); 2777 return retVal; 2778 } 2779 2780 @Bean(name="myServiceDefinitionDaoDstu3") 2781 public 2782 IFhirResourceDao<org.hl7.fhir.dstu3.model.ServiceDefinition> 2783 daoServiceDefinitionDstu3() { 2784 2785 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.ServiceDefinition> retVal; 2786 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.ServiceDefinition>(); 2787 retVal.setResourceType(org.hl7.fhir.dstu3.model.ServiceDefinition.class); 2788 retVal.setContext(myFhirContext); 2789 return retVal; 2790 } 2791 2792 @Bean(name="myServiceDefinitionRpDstu3") 2793 @Lazy 2794 public ca.uhn.fhir.jpa.rp.dstu3.ServiceDefinitionResourceProvider rpServiceDefinitionDstu3() { 2795 ca.uhn.fhir.jpa.rp.dstu3.ServiceDefinitionResourceProvider retVal; 2796 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ServiceDefinitionResourceProvider(); 2797 retVal.setContext(myFhirContext); 2798 retVal.setDao(daoServiceDefinitionDstu3()); 2799 return retVal; 2800 } 2801 2802 @Bean(name="mySlotDaoDstu3") 2803 public 2804 IFhirResourceDao<org.hl7.fhir.dstu3.model.Slot> 2805 daoSlotDstu3() { 2806 2807 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Slot> retVal; 2808 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Slot>(); 2809 retVal.setResourceType(org.hl7.fhir.dstu3.model.Slot.class); 2810 retVal.setContext(myFhirContext); 2811 return retVal; 2812 } 2813 2814 @Bean(name="mySlotRpDstu3") 2815 @Lazy 2816 public ca.uhn.fhir.jpa.rp.dstu3.SlotResourceProvider rpSlotDstu3() { 2817 ca.uhn.fhir.jpa.rp.dstu3.SlotResourceProvider retVal; 2818 retVal = new ca.uhn.fhir.jpa.rp.dstu3.SlotResourceProvider(); 2819 retVal.setContext(myFhirContext); 2820 retVal.setDao(daoSlotDstu3()); 2821 return retVal; 2822 } 2823 2824 @Bean(name="mySpecimenDaoDstu3") 2825 public 2826 IFhirResourceDao<org.hl7.fhir.dstu3.model.Specimen> 2827 daoSpecimenDstu3() { 2828 2829 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Specimen> retVal; 2830 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Specimen>(); 2831 retVal.setResourceType(org.hl7.fhir.dstu3.model.Specimen.class); 2832 retVal.setContext(myFhirContext); 2833 return retVal; 2834 } 2835 2836 @Bean(name="mySpecimenRpDstu3") 2837 @Lazy 2838 public ca.uhn.fhir.jpa.rp.dstu3.SpecimenResourceProvider rpSpecimenDstu3() { 2839 ca.uhn.fhir.jpa.rp.dstu3.SpecimenResourceProvider retVal; 2840 retVal = new ca.uhn.fhir.jpa.rp.dstu3.SpecimenResourceProvider(); 2841 retVal.setContext(myFhirContext); 2842 retVal.setDao(daoSpecimenDstu3()); 2843 return retVal; 2844 } 2845 2846 @Bean(name="myStructureDefinitionDaoDstu3") 2847 public 2848 IFhirResourceDaoStructureDefinition<org.hl7.fhir.dstu3.model.StructureDefinition> 2849 daoStructureDefinitionDstu3() { 2850 2851 ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<org.hl7.fhir.dstu3.model.StructureDefinition> retVal; 2852 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<>(); 2853 retVal.setResourceType(org.hl7.fhir.dstu3.model.StructureDefinition.class); 2854 retVal.setContext(myFhirContext); 2855 return retVal; 2856 } 2857 2858 @Bean(name="myStructureDefinitionRpDstu3") 2859 @Lazy 2860 public ca.uhn.fhir.jpa.rp.dstu3.StructureDefinitionResourceProvider rpStructureDefinitionDstu3() { 2861 ca.uhn.fhir.jpa.rp.dstu3.StructureDefinitionResourceProvider retVal; 2862 retVal = new ca.uhn.fhir.jpa.rp.dstu3.StructureDefinitionResourceProvider(); 2863 retVal.setContext(myFhirContext); 2864 retVal.setDao(daoStructureDefinitionDstu3()); 2865 return retVal; 2866 } 2867 2868 @Bean(name="myStructureMapDaoDstu3") 2869 public 2870 IFhirResourceDao<org.hl7.fhir.dstu3.model.StructureMap> 2871 daoStructureMapDstu3() { 2872 2873 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.StructureMap> retVal; 2874 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.StructureMap>(); 2875 retVal.setResourceType(org.hl7.fhir.dstu3.model.StructureMap.class); 2876 retVal.setContext(myFhirContext); 2877 return retVal; 2878 } 2879 2880 @Bean(name="myStructureMapRpDstu3") 2881 @Lazy 2882 public ca.uhn.fhir.jpa.rp.dstu3.StructureMapResourceProvider rpStructureMapDstu3() { 2883 ca.uhn.fhir.jpa.rp.dstu3.StructureMapResourceProvider retVal; 2884 retVal = new ca.uhn.fhir.jpa.rp.dstu3.StructureMapResourceProvider(); 2885 retVal.setContext(myFhirContext); 2886 retVal.setDao(daoStructureMapDstu3()); 2887 return retVal; 2888 } 2889 2890 @Bean(name="mySubscriptionDaoDstu3") 2891 public 2892 IFhirResourceDaoSubscription<org.hl7.fhir.dstu3.model.Subscription> 2893 daoSubscriptionDstu3() { 2894 2895 ca.uhn.fhir.jpa.dao.dstu3.FhirResourceDaoSubscriptionDstu3 retVal; 2896 retVal = new ca.uhn.fhir.jpa.dao.dstu3.FhirResourceDaoSubscriptionDstu3(); 2897 retVal.setResourceType(org.hl7.fhir.dstu3.model.Subscription.class); 2898 retVal.setContext(myFhirContext); 2899 return retVal; 2900 } 2901 2902 @Bean(name="mySubscriptionRpDstu3") 2903 @Lazy 2904 public ca.uhn.fhir.jpa.rp.dstu3.SubscriptionResourceProvider rpSubscriptionDstu3() { 2905 ca.uhn.fhir.jpa.rp.dstu3.SubscriptionResourceProvider retVal; 2906 retVal = new ca.uhn.fhir.jpa.rp.dstu3.SubscriptionResourceProvider(); 2907 retVal.setContext(myFhirContext); 2908 retVal.setDao(daoSubscriptionDstu3()); 2909 return retVal; 2910 } 2911 2912 @Bean(name="mySubstanceDaoDstu3") 2913 public 2914 IFhirResourceDao<org.hl7.fhir.dstu3.model.Substance> 2915 daoSubstanceDstu3() { 2916 2917 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Substance> retVal; 2918 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Substance>(); 2919 retVal.setResourceType(org.hl7.fhir.dstu3.model.Substance.class); 2920 retVal.setContext(myFhirContext); 2921 return retVal; 2922 } 2923 2924 @Bean(name="mySubstanceRpDstu3") 2925 @Lazy 2926 public ca.uhn.fhir.jpa.rp.dstu3.SubstanceResourceProvider rpSubstanceDstu3() { 2927 ca.uhn.fhir.jpa.rp.dstu3.SubstanceResourceProvider retVal; 2928 retVal = new ca.uhn.fhir.jpa.rp.dstu3.SubstanceResourceProvider(); 2929 retVal.setContext(myFhirContext); 2930 retVal.setDao(daoSubstanceDstu3()); 2931 return retVal; 2932 } 2933 2934 @Bean(name="mySupplyDeliveryDaoDstu3") 2935 public 2936 IFhirResourceDao<org.hl7.fhir.dstu3.model.SupplyDelivery> 2937 daoSupplyDeliveryDstu3() { 2938 2939 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.SupplyDelivery> retVal; 2940 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.SupplyDelivery>(); 2941 retVal.setResourceType(org.hl7.fhir.dstu3.model.SupplyDelivery.class); 2942 retVal.setContext(myFhirContext); 2943 return retVal; 2944 } 2945 2946 @Bean(name="mySupplyDeliveryRpDstu3") 2947 @Lazy 2948 public ca.uhn.fhir.jpa.rp.dstu3.SupplyDeliveryResourceProvider rpSupplyDeliveryDstu3() { 2949 ca.uhn.fhir.jpa.rp.dstu3.SupplyDeliveryResourceProvider retVal; 2950 retVal = new ca.uhn.fhir.jpa.rp.dstu3.SupplyDeliveryResourceProvider(); 2951 retVal.setContext(myFhirContext); 2952 retVal.setDao(daoSupplyDeliveryDstu3()); 2953 return retVal; 2954 } 2955 2956 @Bean(name="mySupplyRequestDaoDstu3") 2957 public 2958 IFhirResourceDao<org.hl7.fhir.dstu3.model.SupplyRequest> 2959 daoSupplyRequestDstu3() { 2960 2961 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.SupplyRequest> retVal; 2962 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.SupplyRequest>(); 2963 retVal.setResourceType(org.hl7.fhir.dstu3.model.SupplyRequest.class); 2964 retVal.setContext(myFhirContext); 2965 return retVal; 2966 } 2967 2968 @Bean(name="mySupplyRequestRpDstu3") 2969 @Lazy 2970 public ca.uhn.fhir.jpa.rp.dstu3.SupplyRequestResourceProvider rpSupplyRequestDstu3() { 2971 ca.uhn.fhir.jpa.rp.dstu3.SupplyRequestResourceProvider retVal; 2972 retVal = new ca.uhn.fhir.jpa.rp.dstu3.SupplyRequestResourceProvider(); 2973 retVal.setContext(myFhirContext); 2974 retVal.setDao(daoSupplyRequestDstu3()); 2975 return retVal; 2976 } 2977 2978 @Bean(name="myTaskDaoDstu3") 2979 public 2980 IFhirResourceDao<org.hl7.fhir.dstu3.model.Task> 2981 daoTaskDstu3() { 2982 2983 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.Task> retVal; 2984 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.Task>(); 2985 retVal.setResourceType(org.hl7.fhir.dstu3.model.Task.class); 2986 retVal.setContext(myFhirContext); 2987 return retVal; 2988 } 2989 2990 @Bean(name="myTaskRpDstu3") 2991 @Lazy 2992 public ca.uhn.fhir.jpa.rp.dstu3.TaskResourceProvider rpTaskDstu3() { 2993 ca.uhn.fhir.jpa.rp.dstu3.TaskResourceProvider retVal; 2994 retVal = new ca.uhn.fhir.jpa.rp.dstu3.TaskResourceProvider(); 2995 retVal.setContext(myFhirContext); 2996 retVal.setDao(daoTaskDstu3()); 2997 return retVal; 2998 } 2999 3000 @Bean(name="myTestReportDaoDstu3") 3001 public 3002 IFhirResourceDao<org.hl7.fhir.dstu3.model.TestReport> 3003 daoTestReportDstu3() { 3004 3005 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.TestReport> retVal; 3006 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.TestReport>(); 3007 retVal.setResourceType(org.hl7.fhir.dstu3.model.TestReport.class); 3008 retVal.setContext(myFhirContext); 3009 return retVal; 3010 } 3011 3012 @Bean(name="myTestReportRpDstu3") 3013 @Lazy 3014 public ca.uhn.fhir.jpa.rp.dstu3.TestReportResourceProvider rpTestReportDstu3() { 3015 ca.uhn.fhir.jpa.rp.dstu3.TestReportResourceProvider retVal; 3016 retVal = new ca.uhn.fhir.jpa.rp.dstu3.TestReportResourceProvider(); 3017 retVal.setContext(myFhirContext); 3018 retVal.setDao(daoTestReportDstu3()); 3019 return retVal; 3020 } 3021 3022 @Bean(name="myTestScriptDaoDstu3") 3023 public 3024 IFhirResourceDao<org.hl7.fhir.dstu3.model.TestScript> 3025 daoTestScriptDstu3() { 3026 3027 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.TestScript> retVal; 3028 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.TestScript>(); 3029 retVal.setResourceType(org.hl7.fhir.dstu3.model.TestScript.class); 3030 retVal.setContext(myFhirContext); 3031 return retVal; 3032 } 3033 3034 @Bean(name="myTestScriptRpDstu3") 3035 @Lazy 3036 public ca.uhn.fhir.jpa.rp.dstu3.TestScriptResourceProvider rpTestScriptDstu3() { 3037 ca.uhn.fhir.jpa.rp.dstu3.TestScriptResourceProvider retVal; 3038 retVal = new ca.uhn.fhir.jpa.rp.dstu3.TestScriptResourceProvider(); 3039 retVal.setContext(myFhirContext); 3040 retVal.setDao(daoTestScriptDstu3()); 3041 return retVal; 3042 } 3043 3044 @Bean(name="myValueSetDaoDstu3") 3045 public 3046 IFhirResourceDaoValueSet<org.hl7.fhir.dstu3.model.ValueSet> 3047 daoValueSetDstu3() { 3048 3049 ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<org.hl7.fhir.dstu3.model.ValueSet> retVal; 3050 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<>(); 3051 retVal.setResourceType(org.hl7.fhir.dstu3.model.ValueSet.class); 3052 retVal.setContext(myFhirContext); 3053 return retVal; 3054 } 3055 3056 @Bean(name="myValueSetRpDstu3") 3057 @Lazy 3058 public ca.uhn.fhir.jpa.rp.dstu3.ValueSetResourceProvider rpValueSetDstu3() { 3059 ca.uhn.fhir.jpa.rp.dstu3.ValueSetResourceProvider retVal; 3060 retVal = new ca.uhn.fhir.jpa.rp.dstu3.ValueSetResourceProvider(); 3061 retVal.setContext(myFhirContext); 3062 retVal.setDao(daoValueSetDstu3()); 3063 return retVal; 3064 } 3065 3066 @Bean(name="myVisionPrescriptionDaoDstu3") 3067 public 3068 IFhirResourceDao<org.hl7.fhir.dstu3.model.VisionPrescription> 3069 daoVisionPrescriptionDstu3() { 3070 3071 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.dstu3.model.VisionPrescription> retVal; 3072 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.dstu3.model.VisionPrescription>(); 3073 retVal.setResourceType(org.hl7.fhir.dstu3.model.VisionPrescription.class); 3074 retVal.setContext(myFhirContext); 3075 return retVal; 3076 } 3077 3078 @Bean(name="myVisionPrescriptionRpDstu3") 3079 @Lazy 3080 public ca.uhn.fhir.jpa.rp.dstu3.VisionPrescriptionResourceProvider rpVisionPrescriptionDstu3() { 3081 ca.uhn.fhir.jpa.rp.dstu3.VisionPrescriptionResourceProvider retVal; 3082 retVal = new ca.uhn.fhir.jpa.rp.dstu3.VisionPrescriptionResourceProvider(); 3083 retVal.setContext(myFhirContext); 3084 retVal.setDao(daoVisionPrescriptionDstu3()); 3085 return retVal; 3086 } 3087 3088 3089 3090 3091 3092 3093}