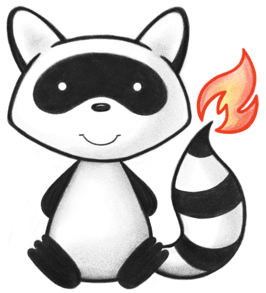
001package ca.uhn.fhir.jpa.config; 002 003import java.util.ArrayList; 004import java.util.List; 005import java.util.concurrent.Executor; 006import java.util.concurrent.Executors; 007 008import org.springframework.transaction.PlatformTransactionManager; 009import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 010 011import org.springframework.beans.factory.annotation.Autowired; 012import org.springframework.context.annotation.Bean; 013import org.springframework.context.annotation.Configuration; 014import org.springframework.context.annotation.Lazy; 015import org.springframework.data.jpa.repository.config.EnableJpaRepositories; 016import org.springframework.scheduling.annotation.EnableScheduling; 017import org.springframework.scheduling.annotation.SchedulingConfigurer; 018import org.springframework.scheduling.config.ScheduledTaskRegistrar; 019 020import ca.uhn.fhir.rest.api.IResourceSupportedSvc; 021import ca.uhn.fhir.context.FhirContext; 022import ca.uhn.fhir.model.api.IResource; 023import ca.uhn.fhir.rest.server.IResourceProvider; 024import ca.uhn.fhir.jpa.api.dao.*; 025import ca.uhn.fhir.jpa.dao.*; 026import ca.uhn.fhir.rest.server.provider.ResourceProviderFactory; 027 028@Configuration 029public class GeneratedDaoAndResourceProviderConfigR4 { 030@Autowired 031FhirContext myFhirContext; 032 033 @Bean(name="myResourceProvidersR4") 034 public ResourceProviderFactory resourceProvidersR4(IResourceSupportedSvc theResourceSupportedSvc) { 035 ResourceProviderFactory retVal = new ResourceProviderFactory(); 036 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Account") ? rpAccountR4() : null); 037 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ActivityDefinition") ? rpActivityDefinitionR4() : null); 038 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AdverseEvent") ? rpAdverseEventR4() : null); 039 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AllergyIntolerance") ? rpAllergyIntoleranceR4() : null); 040 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Appointment") ? rpAppointmentR4() : null); 041 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AppointmentResponse") ? rpAppointmentResponseR4() : null); 042 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AuditEvent") ? rpAuditEventR4() : null); 043 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Basic") ? rpBasicR4() : null); 044 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Binary") ? rpBinaryR4() : null); 045 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BiologicallyDerivedProduct") ? rpBiologicallyDerivedProductR4() : null); 046 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BodyStructure") ? rpBodyStructureR4() : null); 047 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Bundle") ? rpBundleR4() : null); 048 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CapabilityStatement") ? rpCapabilityStatementR4() : null); 049 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CarePlan") ? rpCarePlanR4() : null); 050 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CareTeam") ? rpCareTeamR4() : null); 051 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CatalogEntry") ? rpCatalogEntryR4() : null); 052 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ChargeItem") ? rpChargeItemR4() : null); 053 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ChargeItemDefinition") ? rpChargeItemDefinitionR4() : null); 054 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Claim") ? rpClaimR4() : null); 055 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClaimResponse") ? rpClaimResponseR4() : null); 056 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClinicalImpression") ? rpClinicalImpressionR4() : null); 057 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CodeSystem") ? rpCodeSystemR4() : null); 058 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Communication") ? rpCommunicationR4() : null); 059 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CommunicationRequest") ? rpCommunicationRequestR4() : null); 060 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CompartmentDefinition") ? rpCompartmentDefinitionR4() : null); 061 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Composition") ? rpCompositionR4() : null); 062 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ConceptMap") ? rpConceptMapR4() : null); 063 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Condition") ? rpConditionR4() : null); 064 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Consent") ? rpConsentR4() : null); 065 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Contract") ? rpContractR4() : null); 066 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Coverage") ? rpCoverageR4() : null); 067 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CoverageEligibilityRequest") ? rpCoverageEligibilityRequestR4() : null); 068 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CoverageEligibilityResponse") ? rpCoverageEligibilityResponseR4() : null); 069 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DetectedIssue") ? rpDetectedIssueR4() : null); 070 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Device") ? rpDeviceR4() : null); 071 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceDefinition") ? rpDeviceDefinitionR4() : null); 072 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceMetric") ? rpDeviceMetricR4() : null); 073 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceRequest") ? rpDeviceRequestR4() : null); 074 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceUseStatement") ? rpDeviceUseStatementR4() : null); 075 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DiagnosticReport") ? rpDiagnosticReportR4() : null); 076 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentManifest") ? rpDocumentManifestR4() : null); 077 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentReference") ? rpDocumentReferenceR4() : null); 078 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EffectEvidenceSynthesis") ? rpEffectEvidenceSynthesisR4() : null); 079 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Encounter") ? rpEncounterR4() : null); 080 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Endpoint") ? rpEndpointR4() : null); 081 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentRequest") ? rpEnrollmentRequestR4() : null); 082 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentResponse") ? rpEnrollmentResponseR4() : null); 083 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EpisodeOfCare") ? rpEpisodeOfCareR4() : null); 084 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EventDefinition") ? rpEventDefinitionR4() : null); 085 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Evidence") ? rpEvidenceR4() : null); 086 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EvidenceVariable") ? rpEvidenceVariableR4() : null); 087 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExampleScenario") ? rpExampleScenarioR4() : null); 088 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExplanationOfBenefit") ? rpExplanationOfBenefitR4() : null); 089 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("FamilyMemberHistory") ? rpFamilyMemberHistoryR4() : null); 090 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Flag") ? rpFlagR4() : null); 091 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Goal") ? rpGoalR4() : null); 092 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GraphDefinition") ? rpGraphDefinitionR4() : null); 093 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Group") ? rpGroupR4() : null); 094 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GuidanceResponse") ? rpGuidanceResponseR4() : null); 095 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("HealthcareService") ? rpHealthcareServiceR4() : null); 096 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImagingStudy") ? rpImagingStudyR4() : null); 097 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Immunization") ? rpImmunizationR4() : null); 098 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImmunizationEvaluation") ? rpImmunizationEvaluationR4() : null); 099 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImmunizationRecommendation") ? rpImmunizationRecommendationR4() : null); 100 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImplementationGuide") ? rpImplementationGuideR4() : null); 101 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("InsurancePlan") ? rpInsurancePlanR4() : null); 102 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Invoice") ? rpInvoiceR4() : null); 103 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Library") ? rpLibraryR4() : null); 104 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Linkage") ? rpLinkageR4() : null); 105 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("List") ? rpListResourceR4() : null); 106 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Location") ? rpLocationR4() : null); 107 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Measure") ? rpMeasureR4() : null); 108 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MeasureReport") ? rpMeasureReportR4() : null); 109 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Media") ? rpMediaR4() : null); 110 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Medication") ? rpMedicationR4() : null); 111 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationAdministration") ? rpMedicationAdministrationR4() : null); 112 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationDispense") ? rpMedicationDispenseR4() : null); 113 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationKnowledge") ? rpMedicationKnowledgeR4() : null); 114 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationRequest") ? rpMedicationRequestR4() : null); 115 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationStatement") ? rpMedicationStatementR4() : null); 116 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProduct") ? rpMedicinalProductR4() : null); 117 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductAuthorization") ? rpMedicinalProductAuthorizationR4() : null); 118 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductContraindication") ? rpMedicinalProductContraindicationR4() : null); 119 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductIndication") ? rpMedicinalProductIndicationR4() : null); 120 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductIngredient") ? rpMedicinalProductIngredientR4() : null); 121 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductInteraction") ? rpMedicinalProductInteractionR4() : null); 122 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductManufactured") ? rpMedicinalProductManufacturedR4() : null); 123 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductPackaged") ? rpMedicinalProductPackagedR4() : null); 124 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductPharmaceutical") ? rpMedicinalProductPharmaceuticalR4() : null); 125 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductUndesirableEffect") ? rpMedicinalProductUndesirableEffectR4() : null); 126 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageDefinition") ? rpMessageDefinitionR4() : null); 127 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageHeader") ? rpMessageHeaderR4() : null); 128 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MolecularSequence") ? rpMolecularSequenceR4() : null); 129 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NamingSystem") ? rpNamingSystemR4() : null); 130 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NutritionOrder") ? rpNutritionOrderR4() : null); 131 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Observation") ? rpObservationR4() : null); 132 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ObservationDefinition") ? rpObservationDefinitionR4() : null); 133 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationDefinition") ? rpOperationDefinitionR4() : null); 134 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationOutcome") ? rpOperationOutcomeR4() : null); 135 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Organization") ? rpOrganizationR4() : null); 136 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OrganizationAffiliation") ? rpOrganizationAffiliationR4() : null); 137 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Parameters") ? rpParametersR4() : null); 138 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Patient") ? rpPatientR4() : null); 139 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentNotice") ? rpPaymentNoticeR4() : null); 140 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentReconciliation") ? rpPaymentReconciliationR4() : null); 141 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Person") ? rpPersonR4() : null); 142 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PlanDefinition") ? rpPlanDefinitionR4() : null); 143 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Practitioner") ? rpPractitionerR4() : null); 144 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PractitionerRole") ? rpPractitionerRoleR4() : null); 145 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Procedure") ? rpProcedureR4() : null); 146 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Provenance") ? rpProvenanceR4() : null); 147 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Questionnaire") ? rpQuestionnaireR4() : null); 148 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("QuestionnaireResponse") ? rpQuestionnaireResponseR4() : null); 149 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RelatedPerson") ? rpRelatedPersonR4() : null); 150 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RequestGroup") ? rpRequestGroupR4() : null); 151 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchDefinition") ? rpResearchDefinitionR4() : null); 152 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchElementDefinition") ? rpResearchElementDefinitionR4() : null); 153 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchStudy") ? rpResearchStudyR4() : null); 154 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchSubject") ? rpResearchSubjectR4() : null); 155 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RiskAssessment") ? rpRiskAssessmentR4() : null); 156 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RiskEvidenceSynthesis") ? rpRiskEvidenceSynthesisR4() : null); 157 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Schedule") ? rpScheduleR4() : null); 158 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SearchParameter") ? rpSearchParameterR4() : null); 159 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ServiceRequest") ? rpServiceRequestR4() : null); 160 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Slot") ? rpSlotR4() : null); 161 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Specimen") ? rpSpecimenR4() : null); 162 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SpecimenDefinition") ? rpSpecimenDefinitionR4() : null); 163 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureDefinition") ? rpStructureDefinitionR4() : null); 164 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureMap") ? rpStructureMapR4() : null); 165 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Subscription") ? rpSubscriptionR4() : null); 166 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Substance") ? rpSubstanceR4() : null); 167 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceNucleicAcid") ? rpSubstanceNucleicAcidR4() : null); 168 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstancePolymer") ? rpSubstancePolymerR4() : null); 169 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceProtein") ? rpSubstanceProteinR4() : null); 170 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceReferenceInformation") ? rpSubstanceReferenceInformationR4() : null); 171 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceSourceMaterial") ? rpSubstanceSourceMaterialR4() : null); 172 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceSpecification") ? rpSubstanceSpecificationR4() : null); 173 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyDelivery") ? rpSupplyDeliveryR4() : null); 174 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyRequest") ? rpSupplyRequestR4() : null); 175 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Task") ? rpTaskR4() : null); 176 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TerminologyCapabilities") ? rpTerminologyCapabilitiesR4() : null); 177 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestReport") ? rpTestReportR4() : null); 178 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestScript") ? rpTestScriptR4() : null); 179 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ValueSet") ? rpValueSetR4() : null); 180 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("VerificationResult") ? rpVerificationResultR4() : null); 181 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("VisionPrescription") ? rpVisionPrescriptionR4() : null); 182 return retVal; 183 } 184 185 186 @Bean(name="myResourceDaosR4") 187 public List<IFhirResourceDao<?>> resourceDaosR4(IResourceSupportedSvc theResourceSupportedSvc) { 188 List<IFhirResourceDao<?>> retVal = new ArrayList<IFhirResourceDao<?>>(); 189 if (theResourceSupportedSvc.isSupported("Account")) { 190 retVal.add(daoAccountR4()); 191 } 192 if (theResourceSupportedSvc.isSupported("ActivityDefinition")) { 193 retVal.add(daoActivityDefinitionR4()); 194 } 195 if (theResourceSupportedSvc.isSupported("AdverseEvent")) { 196 retVal.add(daoAdverseEventR4()); 197 } 198 if (theResourceSupportedSvc.isSupported("AllergyIntolerance")) { 199 retVal.add(daoAllergyIntoleranceR4()); 200 } 201 if (theResourceSupportedSvc.isSupported("Appointment")) { 202 retVal.add(daoAppointmentR4()); 203 } 204 if (theResourceSupportedSvc.isSupported("AppointmentResponse")) { 205 retVal.add(daoAppointmentResponseR4()); 206 } 207 if (theResourceSupportedSvc.isSupported("AuditEvent")) { 208 retVal.add(daoAuditEventR4()); 209 } 210 if (theResourceSupportedSvc.isSupported("Basic")) { 211 retVal.add(daoBasicR4()); 212 } 213 if (theResourceSupportedSvc.isSupported("Binary")) { 214 retVal.add(daoBinaryR4()); 215 } 216 if (theResourceSupportedSvc.isSupported("BiologicallyDerivedProduct")) { 217 retVal.add(daoBiologicallyDerivedProductR4()); 218 } 219 if (theResourceSupportedSvc.isSupported("BodyStructure")) { 220 retVal.add(daoBodyStructureR4()); 221 } 222 if (theResourceSupportedSvc.isSupported("Bundle")) { 223 retVal.add(daoBundleR4()); 224 } 225 if (theResourceSupportedSvc.isSupported("CapabilityStatement")) { 226 retVal.add(daoCapabilityStatementR4()); 227 } 228 if (theResourceSupportedSvc.isSupported("CarePlan")) { 229 retVal.add(daoCarePlanR4()); 230 } 231 if (theResourceSupportedSvc.isSupported("CareTeam")) { 232 retVal.add(daoCareTeamR4()); 233 } 234 if (theResourceSupportedSvc.isSupported("CatalogEntry")) { 235 retVal.add(daoCatalogEntryR4()); 236 } 237 if (theResourceSupportedSvc.isSupported("ChargeItem")) { 238 retVal.add(daoChargeItemR4()); 239 } 240 if (theResourceSupportedSvc.isSupported("ChargeItemDefinition")) { 241 retVal.add(daoChargeItemDefinitionR4()); 242 } 243 if (theResourceSupportedSvc.isSupported("Claim")) { 244 retVal.add(daoClaimR4()); 245 } 246 if (theResourceSupportedSvc.isSupported("ClaimResponse")) { 247 retVal.add(daoClaimResponseR4()); 248 } 249 if (theResourceSupportedSvc.isSupported("ClinicalImpression")) { 250 retVal.add(daoClinicalImpressionR4()); 251 } 252 if (theResourceSupportedSvc.isSupported("CodeSystem")) { 253 retVal.add(daoCodeSystemR4()); 254 } 255 if (theResourceSupportedSvc.isSupported("Communication")) { 256 retVal.add(daoCommunicationR4()); 257 } 258 if (theResourceSupportedSvc.isSupported("CommunicationRequest")) { 259 retVal.add(daoCommunicationRequestR4()); 260 } 261 if (theResourceSupportedSvc.isSupported("CompartmentDefinition")) { 262 retVal.add(daoCompartmentDefinitionR4()); 263 } 264 if (theResourceSupportedSvc.isSupported("Composition")) { 265 retVal.add(daoCompositionR4()); 266 } 267 if (theResourceSupportedSvc.isSupported("ConceptMap")) { 268 retVal.add(daoConceptMapR4()); 269 } 270 if (theResourceSupportedSvc.isSupported("Condition")) { 271 retVal.add(daoConditionR4()); 272 } 273 if (theResourceSupportedSvc.isSupported("Consent")) { 274 retVal.add(daoConsentR4()); 275 } 276 if (theResourceSupportedSvc.isSupported("Contract")) { 277 retVal.add(daoContractR4()); 278 } 279 if (theResourceSupportedSvc.isSupported("Coverage")) { 280 retVal.add(daoCoverageR4()); 281 } 282 if (theResourceSupportedSvc.isSupported("CoverageEligibilityRequest")) { 283 retVal.add(daoCoverageEligibilityRequestR4()); 284 } 285 if (theResourceSupportedSvc.isSupported("CoverageEligibilityResponse")) { 286 retVal.add(daoCoverageEligibilityResponseR4()); 287 } 288 if (theResourceSupportedSvc.isSupported("DetectedIssue")) { 289 retVal.add(daoDetectedIssueR4()); 290 } 291 if (theResourceSupportedSvc.isSupported("Device")) { 292 retVal.add(daoDeviceR4()); 293 } 294 if (theResourceSupportedSvc.isSupported("DeviceDefinition")) { 295 retVal.add(daoDeviceDefinitionR4()); 296 } 297 if (theResourceSupportedSvc.isSupported("DeviceMetric")) { 298 retVal.add(daoDeviceMetricR4()); 299 } 300 if (theResourceSupportedSvc.isSupported("DeviceRequest")) { 301 retVal.add(daoDeviceRequestR4()); 302 } 303 if (theResourceSupportedSvc.isSupported("DeviceUseStatement")) { 304 retVal.add(daoDeviceUseStatementR4()); 305 } 306 if (theResourceSupportedSvc.isSupported("DiagnosticReport")) { 307 retVal.add(daoDiagnosticReportR4()); 308 } 309 if (theResourceSupportedSvc.isSupported("DocumentManifest")) { 310 retVal.add(daoDocumentManifestR4()); 311 } 312 if (theResourceSupportedSvc.isSupported("DocumentReference")) { 313 retVal.add(daoDocumentReferenceR4()); 314 } 315 if (theResourceSupportedSvc.isSupported("EffectEvidenceSynthesis")) { 316 retVal.add(daoEffectEvidenceSynthesisR4()); 317 } 318 if (theResourceSupportedSvc.isSupported("Encounter")) { 319 retVal.add(daoEncounterR4()); 320 } 321 if (theResourceSupportedSvc.isSupported("Endpoint")) { 322 retVal.add(daoEndpointR4()); 323 } 324 if (theResourceSupportedSvc.isSupported("EnrollmentRequest")) { 325 retVal.add(daoEnrollmentRequestR4()); 326 } 327 if (theResourceSupportedSvc.isSupported("EnrollmentResponse")) { 328 retVal.add(daoEnrollmentResponseR4()); 329 } 330 if (theResourceSupportedSvc.isSupported("EpisodeOfCare")) { 331 retVal.add(daoEpisodeOfCareR4()); 332 } 333 if (theResourceSupportedSvc.isSupported("EventDefinition")) { 334 retVal.add(daoEventDefinitionR4()); 335 } 336 if (theResourceSupportedSvc.isSupported("Evidence")) { 337 retVal.add(daoEvidenceR4()); 338 } 339 if (theResourceSupportedSvc.isSupported("EvidenceVariable")) { 340 retVal.add(daoEvidenceVariableR4()); 341 } 342 if (theResourceSupportedSvc.isSupported("ExampleScenario")) { 343 retVal.add(daoExampleScenarioR4()); 344 } 345 if (theResourceSupportedSvc.isSupported("ExplanationOfBenefit")) { 346 retVal.add(daoExplanationOfBenefitR4()); 347 } 348 if (theResourceSupportedSvc.isSupported("FamilyMemberHistory")) { 349 retVal.add(daoFamilyMemberHistoryR4()); 350 } 351 if (theResourceSupportedSvc.isSupported("Flag")) { 352 retVal.add(daoFlagR4()); 353 } 354 if (theResourceSupportedSvc.isSupported("Goal")) { 355 retVal.add(daoGoalR4()); 356 } 357 if (theResourceSupportedSvc.isSupported("GraphDefinition")) { 358 retVal.add(daoGraphDefinitionR4()); 359 } 360 if (theResourceSupportedSvc.isSupported("Group")) { 361 retVal.add(daoGroupR4()); 362 } 363 if (theResourceSupportedSvc.isSupported("GuidanceResponse")) { 364 retVal.add(daoGuidanceResponseR4()); 365 } 366 if (theResourceSupportedSvc.isSupported("HealthcareService")) { 367 retVal.add(daoHealthcareServiceR4()); 368 } 369 if (theResourceSupportedSvc.isSupported("ImagingStudy")) { 370 retVal.add(daoImagingStudyR4()); 371 } 372 if (theResourceSupportedSvc.isSupported("Immunization")) { 373 retVal.add(daoImmunizationR4()); 374 } 375 if (theResourceSupportedSvc.isSupported("ImmunizationEvaluation")) { 376 retVal.add(daoImmunizationEvaluationR4()); 377 } 378 if (theResourceSupportedSvc.isSupported("ImmunizationRecommendation")) { 379 retVal.add(daoImmunizationRecommendationR4()); 380 } 381 if (theResourceSupportedSvc.isSupported("ImplementationGuide")) { 382 retVal.add(daoImplementationGuideR4()); 383 } 384 if (theResourceSupportedSvc.isSupported("InsurancePlan")) { 385 retVal.add(daoInsurancePlanR4()); 386 } 387 if (theResourceSupportedSvc.isSupported("Invoice")) { 388 retVal.add(daoInvoiceR4()); 389 } 390 if (theResourceSupportedSvc.isSupported("Library")) { 391 retVal.add(daoLibraryR4()); 392 } 393 if (theResourceSupportedSvc.isSupported("Linkage")) { 394 retVal.add(daoLinkageR4()); 395 } 396 if (theResourceSupportedSvc.isSupported("List")) { 397 retVal.add(daoListResourceR4()); 398 } 399 if (theResourceSupportedSvc.isSupported("Location")) { 400 retVal.add(daoLocationR4()); 401 } 402 if (theResourceSupportedSvc.isSupported("Measure")) { 403 retVal.add(daoMeasureR4()); 404 } 405 if (theResourceSupportedSvc.isSupported("MeasureReport")) { 406 retVal.add(daoMeasureReportR4()); 407 } 408 if (theResourceSupportedSvc.isSupported("Media")) { 409 retVal.add(daoMediaR4()); 410 } 411 if (theResourceSupportedSvc.isSupported("Medication")) { 412 retVal.add(daoMedicationR4()); 413 } 414 if (theResourceSupportedSvc.isSupported("MedicationAdministration")) { 415 retVal.add(daoMedicationAdministrationR4()); 416 } 417 if (theResourceSupportedSvc.isSupported("MedicationDispense")) { 418 retVal.add(daoMedicationDispenseR4()); 419 } 420 if (theResourceSupportedSvc.isSupported("MedicationKnowledge")) { 421 retVal.add(daoMedicationKnowledgeR4()); 422 } 423 if (theResourceSupportedSvc.isSupported("MedicationRequest")) { 424 retVal.add(daoMedicationRequestR4()); 425 } 426 if (theResourceSupportedSvc.isSupported("MedicationStatement")) { 427 retVal.add(daoMedicationStatementR4()); 428 } 429 if (theResourceSupportedSvc.isSupported("MedicinalProduct")) { 430 retVal.add(daoMedicinalProductR4()); 431 } 432 if (theResourceSupportedSvc.isSupported("MedicinalProductAuthorization")) { 433 retVal.add(daoMedicinalProductAuthorizationR4()); 434 } 435 if (theResourceSupportedSvc.isSupported("MedicinalProductContraindication")) { 436 retVal.add(daoMedicinalProductContraindicationR4()); 437 } 438 if (theResourceSupportedSvc.isSupported("MedicinalProductIndication")) { 439 retVal.add(daoMedicinalProductIndicationR4()); 440 } 441 if (theResourceSupportedSvc.isSupported("MedicinalProductIngredient")) { 442 retVal.add(daoMedicinalProductIngredientR4()); 443 } 444 if (theResourceSupportedSvc.isSupported("MedicinalProductInteraction")) { 445 retVal.add(daoMedicinalProductInteractionR4()); 446 } 447 if (theResourceSupportedSvc.isSupported("MedicinalProductManufactured")) { 448 retVal.add(daoMedicinalProductManufacturedR4()); 449 } 450 if (theResourceSupportedSvc.isSupported("MedicinalProductPackaged")) { 451 retVal.add(daoMedicinalProductPackagedR4()); 452 } 453 if (theResourceSupportedSvc.isSupported("MedicinalProductPharmaceutical")) { 454 retVal.add(daoMedicinalProductPharmaceuticalR4()); 455 } 456 if (theResourceSupportedSvc.isSupported("MedicinalProductUndesirableEffect")) { 457 retVal.add(daoMedicinalProductUndesirableEffectR4()); 458 } 459 if (theResourceSupportedSvc.isSupported("MessageDefinition")) { 460 retVal.add(daoMessageDefinitionR4()); 461 } 462 if (theResourceSupportedSvc.isSupported("MessageHeader")) { 463 retVal.add(daoMessageHeaderR4()); 464 } 465 if (theResourceSupportedSvc.isSupported("MolecularSequence")) { 466 retVal.add(daoMolecularSequenceR4()); 467 } 468 if (theResourceSupportedSvc.isSupported("NamingSystem")) { 469 retVal.add(daoNamingSystemR4()); 470 } 471 if (theResourceSupportedSvc.isSupported("NutritionOrder")) { 472 retVal.add(daoNutritionOrderR4()); 473 } 474 if (theResourceSupportedSvc.isSupported("Observation")) { 475 retVal.add(daoObservationR4()); 476 } 477 if (theResourceSupportedSvc.isSupported("ObservationDefinition")) { 478 retVal.add(daoObservationDefinitionR4()); 479 } 480 if (theResourceSupportedSvc.isSupported("OperationDefinition")) { 481 retVal.add(daoOperationDefinitionR4()); 482 } 483 if (theResourceSupportedSvc.isSupported("OperationOutcome")) { 484 retVal.add(daoOperationOutcomeR4()); 485 } 486 if (theResourceSupportedSvc.isSupported("Organization")) { 487 retVal.add(daoOrganizationR4()); 488 } 489 if (theResourceSupportedSvc.isSupported("OrganizationAffiliation")) { 490 retVal.add(daoOrganizationAffiliationR4()); 491 } 492 if (theResourceSupportedSvc.isSupported("Parameters")) { 493 retVal.add(daoParametersR4()); 494 } 495 if (theResourceSupportedSvc.isSupported("Patient")) { 496 retVal.add(daoPatientR4()); 497 } 498 if (theResourceSupportedSvc.isSupported("PaymentNotice")) { 499 retVal.add(daoPaymentNoticeR4()); 500 } 501 if (theResourceSupportedSvc.isSupported("PaymentReconciliation")) { 502 retVal.add(daoPaymentReconciliationR4()); 503 } 504 if (theResourceSupportedSvc.isSupported("Person")) { 505 retVal.add(daoPersonR4()); 506 } 507 if (theResourceSupportedSvc.isSupported("PlanDefinition")) { 508 retVal.add(daoPlanDefinitionR4()); 509 } 510 if (theResourceSupportedSvc.isSupported("Practitioner")) { 511 retVal.add(daoPractitionerR4()); 512 } 513 if (theResourceSupportedSvc.isSupported("PractitionerRole")) { 514 retVal.add(daoPractitionerRoleR4()); 515 } 516 if (theResourceSupportedSvc.isSupported("Procedure")) { 517 retVal.add(daoProcedureR4()); 518 } 519 if (theResourceSupportedSvc.isSupported("Provenance")) { 520 retVal.add(daoProvenanceR4()); 521 } 522 if (theResourceSupportedSvc.isSupported("Questionnaire")) { 523 retVal.add(daoQuestionnaireR4()); 524 } 525 if (theResourceSupportedSvc.isSupported("QuestionnaireResponse")) { 526 retVal.add(daoQuestionnaireResponseR4()); 527 } 528 if (theResourceSupportedSvc.isSupported("RelatedPerson")) { 529 retVal.add(daoRelatedPersonR4()); 530 } 531 if (theResourceSupportedSvc.isSupported("RequestGroup")) { 532 retVal.add(daoRequestGroupR4()); 533 } 534 if (theResourceSupportedSvc.isSupported("ResearchDefinition")) { 535 retVal.add(daoResearchDefinitionR4()); 536 } 537 if (theResourceSupportedSvc.isSupported("ResearchElementDefinition")) { 538 retVal.add(daoResearchElementDefinitionR4()); 539 } 540 if (theResourceSupportedSvc.isSupported("ResearchStudy")) { 541 retVal.add(daoResearchStudyR4()); 542 } 543 if (theResourceSupportedSvc.isSupported("ResearchSubject")) { 544 retVal.add(daoResearchSubjectR4()); 545 } 546 if (theResourceSupportedSvc.isSupported("RiskAssessment")) { 547 retVal.add(daoRiskAssessmentR4()); 548 } 549 if (theResourceSupportedSvc.isSupported("RiskEvidenceSynthesis")) { 550 retVal.add(daoRiskEvidenceSynthesisR4()); 551 } 552 if (theResourceSupportedSvc.isSupported("Schedule")) { 553 retVal.add(daoScheduleR4()); 554 } 555 if (theResourceSupportedSvc.isSupported("SearchParameter")) { 556 retVal.add(daoSearchParameterR4()); 557 } 558 if (theResourceSupportedSvc.isSupported("ServiceRequest")) { 559 retVal.add(daoServiceRequestR4()); 560 } 561 if (theResourceSupportedSvc.isSupported("Slot")) { 562 retVal.add(daoSlotR4()); 563 } 564 if (theResourceSupportedSvc.isSupported("Specimen")) { 565 retVal.add(daoSpecimenR4()); 566 } 567 if (theResourceSupportedSvc.isSupported("SpecimenDefinition")) { 568 retVal.add(daoSpecimenDefinitionR4()); 569 } 570 if (theResourceSupportedSvc.isSupported("StructureDefinition")) { 571 retVal.add(daoStructureDefinitionR4()); 572 } 573 if (theResourceSupportedSvc.isSupported("StructureMap")) { 574 retVal.add(daoStructureMapR4()); 575 } 576 if (theResourceSupportedSvc.isSupported("Subscription")) { 577 retVal.add(daoSubscriptionR4()); 578 } 579 if (theResourceSupportedSvc.isSupported("Substance")) { 580 retVal.add(daoSubstanceR4()); 581 } 582 if (theResourceSupportedSvc.isSupported("SubstanceNucleicAcid")) { 583 retVal.add(daoSubstanceNucleicAcidR4()); 584 } 585 if (theResourceSupportedSvc.isSupported("SubstancePolymer")) { 586 retVal.add(daoSubstancePolymerR4()); 587 } 588 if (theResourceSupportedSvc.isSupported("SubstanceProtein")) { 589 retVal.add(daoSubstanceProteinR4()); 590 } 591 if (theResourceSupportedSvc.isSupported("SubstanceReferenceInformation")) { 592 retVal.add(daoSubstanceReferenceInformationR4()); 593 } 594 if (theResourceSupportedSvc.isSupported("SubstanceSourceMaterial")) { 595 retVal.add(daoSubstanceSourceMaterialR4()); 596 } 597 if (theResourceSupportedSvc.isSupported("SubstanceSpecification")) { 598 retVal.add(daoSubstanceSpecificationR4()); 599 } 600 if (theResourceSupportedSvc.isSupported("SupplyDelivery")) { 601 retVal.add(daoSupplyDeliveryR4()); 602 } 603 if (theResourceSupportedSvc.isSupported("SupplyRequest")) { 604 retVal.add(daoSupplyRequestR4()); 605 } 606 if (theResourceSupportedSvc.isSupported("Task")) { 607 retVal.add(daoTaskR4()); 608 } 609 if (theResourceSupportedSvc.isSupported("TerminologyCapabilities")) { 610 retVal.add(daoTerminologyCapabilitiesR4()); 611 } 612 if (theResourceSupportedSvc.isSupported("TestReport")) { 613 retVal.add(daoTestReportR4()); 614 } 615 if (theResourceSupportedSvc.isSupported("TestScript")) { 616 retVal.add(daoTestScriptR4()); 617 } 618 if (theResourceSupportedSvc.isSupported("ValueSet")) { 619 retVal.add(daoValueSetR4()); 620 } 621 if (theResourceSupportedSvc.isSupported("VerificationResult")) { 622 retVal.add(daoVerificationResultR4()); 623 } 624 if (theResourceSupportedSvc.isSupported("VisionPrescription")) { 625 retVal.add(daoVisionPrescriptionR4()); 626 } 627 return retVal; 628 } 629 630 @Bean(name="myAccountDaoR4") 631 public 632 IFhirResourceDao<org.hl7.fhir.r4.model.Account> 633 daoAccountR4() { 634 635 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Account> retVal; 636 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Account>(); 637 retVal.setResourceType(org.hl7.fhir.r4.model.Account.class); 638 retVal.setContext(myFhirContext); 639 return retVal; 640 } 641 642 @Bean(name="myAccountRpR4") 643 @Lazy 644 public ca.uhn.fhir.jpa.rp.r4.AccountResourceProvider rpAccountR4() { 645 ca.uhn.fhir.jpa.rp.r4.AccountResourceProvider retVal; 646 retVal = new ca.uhn.fhir.jpa.rp.r4.AccountResourceProvider(); 647 retVal.setContext(myFhirContext); 648 retVal.setDao(daoAccountR4()); 649 return retVal; 650 } 651 652 @Bean(name="myActivityDefinitionDaoR4") 653 public 654 IFhirResourceDao<org.hl7.fhir.r4.model.ActivityDefinition> 655 daoActivityDefinitionR4() { 656 657 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ActivityDefinition> retVal; 658 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ActivityDefinition>(); 659 retVal.setResourceType(org.hl7.fhir.r4.model.ActivityDefinition.class); 660 retVal.setContext(myFhirContext); 661 return retVal; 662 } 663 664 @Bean(name="myActivityDefinitionRpR4") 665 @Lazy 666 public ca.uhn.fhir.jpa.rp.r4.ActivityDefinitionResourceProvider rpActivityDefinitionR4() { 667 ca.uhn.fhir.jpa.rp.r4.ActivityDefinitionResourceProvider retVal; 668 retVal = new ca.uhn.fhir.jpa.rp.r4.ActivityDefinitionResourceProvider(); 669 retVal.setContext(myFhirContext); 670 retVal.setDao(daoActivityDefinitionR4()); 671 return retVal; 672 } 673 674 @Bean(name="myAdverseEventDaoR4") 675 public 676 IFhirResourceDao<org.hl7.fhir.r4.model.AdverseEvent> 677 daoAdverseEventR4() { 678 679 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.AdverseEvent> retVal; 680 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.AdverseEvent>(); 681 retVal.setResourceType(org.hl7.fhir.r4.model.AdverseEvent.class); 682 retVal.setContext(myFhirContext); 683 return retVal; 684 } 685 686 @Bean(name="myAdverseEventRpR4") 687 @Lazy 688 public ca.uhn.fhir.jpa.rp.r4.AdverseEventResourceProvider rpAdverseEventR4() { 689 ca.uhn.fhir.jpa.rp.r4.AdverseEventResourceProvider retVal; 690 retVal = new ca.uhn.fhir.jpa.rp.r4.AdverseEventResourceProvider(); 691 retVal.setContext(myFhirContext); 692 retVal.setDao(daoAdverseEventR4()); 693 return retVal; 694 } 695 696 @Bean(name="myAllergyIntoleranceDaoR4") 697 public 698 IFhirResourceDao<org.hl7.fhir.r4.model.AllergyIntolerance> 699 daoAllergyIntoleranceR4() { 700 701 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.AllergyIntolerance> retVal; 702 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.AllergyIntolerance>(); 703 retVal.setResourceType(org.hl7.fhir.r4.model.AllergyIntolerance.class); 704 retVal.setContext(myFhirContext); 705 return retVal; 706 } 707 708 @Bean(name="myAllergyIntoleranceRpR4") 709 @Lazy 710 public ca.uhn.fhir.jpa.rp.r4.AllergyIntoleranceResourceProvider rpAllergyIntoleranceR4() { 711 ca.uhn.fhir.jpa.rp.r4.AllergyIntoleranceResourceProvider retVal; 712 retVal = new ca.uhn.fhir.jpa.rp.r4.AllergyIntoleranceResourceProvider(); 713 retVal.setContext(myFhirContext); 714 retVal.setDao(daoAllergyIntoleranceR4()); 715 return retVal; 716 } 717 718 @Bean(name="myAppointmentDaoR4") 719 public 720 IFhirResourceDao<org.hl7.fhir.r4.model.Appointment> 721 daoAppointmentR4() { 722 723 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Appointment> retVal; 724 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Appointment>(); 725 retVal.setResourceType(org.hl7.fhir.r4.model.Appointment.class); 726 retVal.setContext(myFhirContext); 727 return retVal; 728 } 729 730 @Bean(name="myAppointmentRpR4") 731 @Lazy 732 public ca.uhn.fhir.jpa.rp.r4.AppointmentResourceProvider rpAppointmentR4() { 733 ca.uhn.fhir.jpa.rp.r4.AppointmentResourceProvider retVal; 734 retVal = new ca.uhn.fhir.jpa.rp.r4.AppointmentResourceProvider(); 735 retVal.setContext(myFhirContext); 736 retVal.setDao(daoAppointmentR4()); 737 return retVal; 738 } 739 740 @Bean(name="myAppointmentResponseDaoR4") 741 public 742 IFhirResourceDao<org.hl7.fhir.r4.model.AppointmentResponse> 743 daoAppointmentResponseR4() { 744 745 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.AppointmentResponse> retVal; 746 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.AppointmentResponse>(); 747 retVal.setResourceType(org.hl7.fhir.r4.model.AppointmentResponse.class); 748 retVal.setContext(myFhirContext); 749 return retVal; 750 } 751 752 @Bean(name="myAppointmentResponseRpR4") 753 @Lazy 754 public ca.uhn.fhir.jpa.rp.r4.AppointmentResponseResourceProvider rpAppointmentResponseR4() { 755 ca.uhn.fhir.jpa.rp.r4.AppointmentResponseResourceProvider retVal; 756 retVal = new ca.uhn.fhir.jpa.rp.r4.AppointmentResponseResourceProvider(); 757 retVal.setContext(myFhirContext); 758 retVal.setDao(daoAppointmentResponseR4()); 759 return retVal; 760 } 761 762 @Bean(name="myAuditEventDaoR4") 763 public 764 IFhirResourceDao<org.hl7.fhir.r4.model.AuditEvent> 765 daoAuditEventR4() { 766 767 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.AuditEvent> retVal; 768 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.AuditEvent>(); 769 retVal.setResourceType(org.hl7.fhir.r4.model.AuditEvent.class); 770 retVal.setContext(myFhirContext); 771 return retVal; 772 } 773 774 @Bean(name="myAuditEventRpR4") 775 @Lazy 776 public ca.uhn.fhir.jpa.rp.r4.AuditEventResourceProvider rpAuditEventR4() { 777 ca.uhn.fhir.jpa.rp.r4.AuditEventResourceProvider retVal; 778 retVal = new ca.uhn.fhir.jpa.rp.r4.AuditEventResourceProvider(); 779 retVal.setContext(myFhirContext); 780 retVal.setDao(daoAuditEventR4()); 781 return retVal; 782 } 783 784 @Bean(name="myBasicDaoR4") 785 public 786 IFhirResourceDao<org.hl7.fhir.r4.model.Basic> 787 daoBasicR4() { 788 789 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Basic> retVal; 790 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Basic>(); 791 retVal.setResourceType(org.hl7.fhir.r4.model.Basic.class); 792 retVal.setContext(myFhirContext); 793 return retVal; 794 } 795 796 @Bean(name="myBasicRpR4") 797 @Lazy 798 public ca.uhn.fhir.jpa.rp.r4.BasicResourceProvider rpBasicR4() { 799 ca.uhn.fhir.jpa.rp.r4.BasicResourceProvider retVal; 800 retVal = new ca.uhn.fhir.jpa.rp.r4.BasicResourceProvider(); 801 retVal.setContext(myFhirContext); 802 retVal.setDao(daoBasicR4()); 803 return retVal; 804 } 805 806 @Bean(name="myBinaryDaoR4") 807 public 808 IFhirResourceDao<org.hl7.fhir.r4.model.Binary> 809 daoBinaryR4() { 810 811 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Binary> retVal; 812 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Binary>(); 813 retVal.setResourceType(org.hl7.fhir.r4.model.Binary.class); 814 retVal.setContext(myFhirContext); 815 return retVal; 816 } 817 818 @Bean(name="myBinaryRpR4") 819 @Lazy 820 public ca.uhn.fhir.jpa.rp.r4.BinaryResourceProvider rpBinaryR4() { 821 ca.uhn.fhir.jpa.rp.r4.BinaryResourceProvider retVal; 822 retVal = new ca.uhn.fhir.jpa.rp.r4.BinaryResourceProvider(); 823 retVal.setContext(myFhirContext); 824 retVal.setDao(daoBinaryR4()); 825 return retVal; 826 } 827 828 @Bean(name="myBiologicallyDerivedProductDaoR4") 829 public 830 IFhirResourceDao<org.hl7.fhir.r4.model.BiologicallyDerivedProduct> 831 daoBiologicallyDerivedProductR4() { 832 833 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.BiologicallyDerivedProduct> retVal; 834 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.BiologicallyDerivedProduct>(); 835 retVal.setResourceType(org.hl7.fhir.r4.model.BiologicallyDerivedProduct.class); 836 retVal.setContext(myFhirContext); 837 return retVal; 838 } 839 840 @Bean(name="myBiologicallyDerivedProductRpR4") 841 @Lazy 842 public ca.uhn.fhir.jpa.rp.r4.BiologicallyDerivedProductResourceProvider rpBiologicallyDerivedProductR4() { 843 ca.uhn.fhir.jpa.rp.r4.BiologicallyDerivedProductResourceProvider retVal; 844 retVal = new ca.uhn.fhir.jpa.rp.r4.BiologicallyDerivedProductResourceProvider(); 845 retVal.setContext(myFhirContext); 846 retVal.setDao(daoBiologicallyDerivedProductR4()); 847 return retVal; 848 } 849 850 @Bean(name="myBodyStructureDaoR4") 851 public 852 IFhirResourceDao<org.hl7.fhir.r4.model.BodyStructure> 853 daoBodyStructureR4() { 854 855 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.BodyStructure> retVal; 856 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.BodyStructure>(); 857 retVal.setResourceType(org.hl7.fhir.r4.model.BodyStructure.class); 858 retVal.setContext(myFhirContext); 859 return retVal; 860 } 861 862 @Bean(name="myBodyStructureRpR4") 863 @Lazy 864 public ca.uhn.fhir.jpa.rp.r4.BodyStructureResourceProvider rpBodyStructureR4() { 865 ca.uhn.fhir.jpa.rp.r4.BodyStructureResourceProvider retVal; 866 retVal = new ca.uhn.fhir.jpa.rp.r4.BodyStructureResourceProvider(); 867 retVal.setContext(myFhirContext); 868 retVal.setDao(daoBodyStructureR4()); 869 return retVal; 870 } 871 872 @Bean(name="myBundleDaoR4") 873 public 874 IFhirResourceDao<org.hl7.fhir.r4.model.Bundle> 875 daoBundleR4() { 876 877 ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<org.hl7.fhir.r4.model.Bundle> retVal; 878 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<>(); 879 retVal.setResourceType(org.hl7.fhir.r4.model.Bundle.class); 880 retVal.setContext(myFhirContext); 881 return retVal; 882 } 883 884 @Bean(name="myBundleRpR4") 885 @Lazy 886 public ca.uhn.fhir.jpa.rp.r4.BundleResourceProvider rpBundleR4() { 887 ca.uhn.fhir.jpa.rp.r4.BundleResourceProvider retVal; 888 retVal = new ca.uhn.fhir.jpa.rp.r4.BundleResourceProvider(); 889 retVal.setContext(myFhirContext); 890 retVal.setDao(daoBundleR4()); 891 return retVal; 892 } 893 894 @Bean(name="myCapabilityStatementDaoR4") 895 public 896 IFhirResourceDao<org.hl7.fhir.r4.model.CapabilityStatement> 897 daoCapabilityStatementR4() { 898 899 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.CapabilityStatement> retVal; 900 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.CapabilityStatement>(); 901 retVal.setResourceType(org.hl7.fhir.r4.model.CapabilityStatement.class); 902 retVal.setContext(myFhirContext); 903 return retVal; 904 } 905 906 @Bean(name="myCapabilityStatementRpR4") 907 @Lazy 908 public ca.uhn.fhir.jpa.rp.r4.CapabilityStatementResourceProvider rpCapabilityStatementR4() { 909 ca.uhn.fhir.jpa.rp.r4.CapabilityStatementResourceProvider retVal; 910 retVal = new ca.uhn.fhir.jpa.rp.r4.CapabilityStatementResourceProvider(); 911 retVal.setContext(myFhirContext); 912 retVal.setDao(daoCapabilityStatementR4()); 913 return retVal; 914 } 915 916 @Bean(name="myCarePlanDaoR4") 917 public 918 IFhirResourceDao<org.hl7.fhir.r4.model.CarePlan> 919 daoCarePlanR4() { 920 921 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.CarePlan> retVal; 922 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.CarePlan>(); 923 retVal.setResourceType(org.hl7.fhir.r4.model.CarePlan.class); 924 retVal.setContext(myFhirContext); 925 return retVal; 926 } 927 928 @Bean(name="myCarePlanRpR4") 929 @Lazy 930 public ca.uhn.fhir.jpa.rp.r4.CarePlanResourceProvider rpCarePlanR4() { 931 ca.uhn.fhir.jpa.rp.r4.CarePlanResourceProvider retVal; 932 retVal = new ca.uhn.fhir.jpa.rp.r4.CarePlanResourceProvider(); 933 retVal.setContext(myFhirContext); 934 retVal.setDao(daoCarePlanR4()); 935 return retVal; 936 } 937 938 @Bean(name="myCareTeamDaoR4") 939 public 940 IFhirResourceDao<org.hl7.fhir.r4.model.CareTeam> 941 daoCareTeamR4() { 942 943 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.CareTeam> retVal; 944 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.CareTeam>(); 945 retVal.setResourceType(org.hl7.fhir.r4.model.CareTeam.class); 946 retVal.setContext(myFhirContext); 947 return retVal; 948 } 949 950 @Bean(name="myCareTeamRpR4") 951 @Lazy 952 public ca.uhn.fhir.jpa.rp.r4.CareTeamResourceProvider rpCareTeamR4() { 953 ca.uhn.fhir.jpa.rp.r4.CareTeamResourceProvider retVal; 954 retVal = new ca.uhn.fhir.jpa.rp.r4.CareTeamResourceProvider(); 955 retVal.setContext(myFhirContext); 956 retVal.setDao(daoCareTeamR4()); 957 return retVal; 958 } 959 960 @Bean(name="myCatalogEntryDaoR4") 961 public 962 IFhirResourceDao<org.hl7.fhir.r4.model.CatalogEntry> 963 daoCatalogEntryR4() { 964 965 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.CatalogEntry> retVal; 966 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.CatalogEntry>(); 967 retVal.setResourceType(org.hl7.fhir.r4.model.CatalogEntry.class); 968 retVal.setContext(myFhirContext); 969 return retVal; 970 } 971 972 @Bean(name="myCatalogEntryRpR4") 973 @Lazy 974 public ca.uhn.fhir.jpa.rp.r4.CatalogEntryResourceProvider rpCatalogEntryR4() { 975 ca.uhn.fhir.jpa.rp.r4.CatalogEntryResourceProvider retVal; 976 retVal = new ca.uhn.fhir.jpa.rp.r4.CatalogEntryResourceProvider(); 977 retVal.setContext(myFhirContext); 978 retVal.setDao(daoCatalogEntryR4()); 979 return retVal; 980 } 981 982 @Bean(name="myChargeItemDaoR4") 983 public 984 IFhirResourceDao<org.hl7.fhir.r4.model.ChargeItem> 985 daoChargeItemR4() { 986 987 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ChargeItem> retVal; 988 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ChargeItem>(); 989 retVal.setResourceType(org.hl7.fhir.r4.model.ChargeItem.class); 990 retVal.setContext(myFhirContext); 991 return retVal; 992 } 993 994 @Bean(name="myChargeItemRpR4") 995 @Lazy 996 public ca.uhn.fhir.jpa.rp.r4.ChargeItemResourceProvider rpChargeItemR4() { 997 ca.uhn.fhir.jpa.rp.r4.ChargeItemResourceProvider retVal; 998 retVal = new ca.uhn.fhir.jpa.rp.r4.ChargeItemResourceProvider(); 999 retVal.setContext(myFhirContext); 1000 retVal.setDao(daoChargeItemR4()); 1001 return retVal; 1002 } 1003 1004 @Bean(name="myChargeItemDefinitionDaoR4") 1005 public 1006 IFhirResourceDao<org.hl7.fhir.r4.model.ChargeItemDefinition> 1007 daoChargeItemDefinitionR4() { 1008 1009 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ChargeItemDefinition> retVal; 1010 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ChargeItemDefinition>(); 1011 retVal.setResourceType(org.hl7.fhir.r4.model.ChargeItemDefinition.class); 1012 retVal.setContext(myFhirContext); 1013 return retVal; 1014 } 1015 1016 @Bean(name="myChargeItemDefinitionRpR4") 1017 @Lazy 1018 public ca.uhn.fhir.jpa.rp.r4.ChargeItemDefinitionResourceProvider rpChargeItemDefinitionR4() { 1019 ca.uhn.fhir.jpa.rp.r4.ChargeItemDefinitionResourceProvider retVal; 1020 retVal = new ca.uhn.fhir.jpa.rp.r4.ChargeItemDefinitionResourceProvider(); 1021 retVal.setContext(myFhirContext); 1022 retVal.setDao(daoChargeItemDefinitionR4()); 1023 return retVal; 1024 } 1025 1026 @Bean(name="myClaimDaoR4") 1027 public 1028 IFhirResourceDao<org.hl7.fhir.r4.model.Claim> 1029 daoClaimR4() { 1030 1031 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Claim> retVal; 1032 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Claim>(); 1033 retVal.setResourceType(org.hl7.fhir.r4.model.Claim.class); 1034 retVal.setContext(myFhirContext); 1035 return retVal; 1036 } 1037 1038 @Bean(name="myClaimRpR4") 1039 @Lazy 1040 public ca.uhn.fhir.jpa.rp.r4.ClaimResourceProvider rpClaimR4() { 1041 ca.uhn.fhir.jpa.rp.r4.ClaimResourceProvider retVal; 1042 retVal = new ca.uhn.fhir.jpa.rp.r4.ClaimResourceProvider(); 1043 retVal.setContext(myFhirContext); 1044 retVal.setDao(daoClaimR4()); 1045 return retVal; 1046 } 1047 1048 @Bean(name="myClaimResponseDaoR4") 1049 public 1050 IFhirResourceDao<org.hl7.fhir.r4.model.ClaimResponse> 1051 daoClaimResponseR4() { 1052 1053 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ClaimResponse> retVal; 1054 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ClaimResponse>(); 1055 retVal.setResourceType(org.hl7.fhir.r4.model.ClaimResponse.class); 1056 retVal.setContext(myFhirContext); 1057 return retVal; 1058 } 1059 1060 @Bean(name="myClaimResponseRpR4") 1061 @Lazy 1062 public ca.uhn.fhir.jpa.rp.r4.ClaimResponseResourceProvider rpClaimResponseR4() { 1063 ca.uhn.fhir.jpa.rp.r4.ClaimResponseResourceProvider retVal; 1064 retVal = new ca.uhn.fhir.jpa.rp.r4.ClaimResponseResourceProvider(); 1065 retVal.setContext(myFhirContext); 1066 retVal.setDao(daoClaimResponseR4()); 1067 return retVal; 1068 } 1069 1070 @Bean(name="myClinicalImpressionDaoR4") 1071 public 1072 IFhirResourceDao<org.hl7.fhir.r4.model.ClinicalImpression> 1073 daoClinicalImpressionR4() { 1074 1075 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ClinicalImpression> retVal; 1076 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ClinicalImpression>(); 1077 retVal.setResourceType(org.hl7.fhir.r4.model.ClinicalImpression.class); 1078 retVal.setContext(myFhirContext); 1079 return retVal; 1080 } 1081 1082 @Bean(name="myClinicalImpressionRpR4") 1083 @Lazy 1084 public ca.uhn.fhir.jpa.rp.r4.ClinicalImpressionResourceProvider rpClinicalImpressionR4() { 1085 ca.uhn.fhir.jpa.rp.r4.ClinicalImpressionResourceProvider retVal; 1086 retVal = new ca.uhn.fhir.jpa.rp.r4.ClinicalImpressionResourceProvider(); 1087 retVal.setContext(myFhirContext); 1088 retVal.setDao(daoClinicalImpressionR4()); 1089 return retVal; 1090 } 1091 1092 @Bean(name="myCodeSystemDaoR4") 1093 public 1094 IFhirResourceDaoCodeSystem<org.hl7.fhir.r4.model.CodeSystem> 1095 daoCodeSystemR4() { 1096 1097 ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem<org.hl7.fhir.r4.model.CodeSystem> retVal; 1098 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem<>(); 1099 retVal.setResourceType(org.hl7.fhir.r4.model.CodeSystem.class); 1100 retVal.setContext(myFhirContext); 1101 return retVal; 1102 } 1103 1104 @Bean(name="myCodeSystemRpR4") 1105 @Lazy 1106 public ca.uhn.fhir.jpa.rp.r4.CodeSystemResourceProvider rpCodeSystemR4() { 1107 ca.uhn.fhir.jpa.rp.r4.CodeSystemResourceProvider retVal; 1108 retVal = new ca.uhn.fhir.jpa.rp.r4.CodeSystemResourceProvider(); 1109 retVal.setContext(myFhirContext); 1110 retVal.setDao(daoCodeSystemR4()); 1111 return retVal; 1112 } 1113 1114 @Bean(name="myCommunicationDaoR4") 1115 public 1116 IFhirResourceDao<org.hl7.fhir.r4.model.Communication> 1117 daoCommunicationR4() { 1118 1119 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Communication> retVal; 1120 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Communication>(); 1121 retVal.setResourceType(org.hl7.fhir.r4.model.Communication.class); 1122 retVal.setContext(myFhirContext); 1123 return retVal; 1124 } 1125 1126 @Bean(name="myCommunicationRpR4") 1127 @Lazy 1128 public ca.uhn.fhir.jpa.rp.r4.CommunicationResourceProvider rpCommunicationR4() { 1129 ca.uhn.fhir.jpa.rp.r4.CommunicationResourceProvider retVal; 1130 retVal = new ca.uhn.fhir.jpa.rp.r4.CommunicationResourceProvider(); 1131 retVal.setContext(myFhirContext); 1132 retVal.setDao(daoCommunicationR4()); 1133 return retVal; 1134 } 1135 1136 @Bean(name="myCommunicationRequestDaoR4") 1137 public 1138 IFhirResourceDao<org.hl7.fhir.r4.model.CommunicationRequest> 1139 daoCommunicationRequestR4() { 1140 1141 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.CommunicationRequest> retVal; 1142 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.CommunicationRequest>(); 1143 retVal.setResourceType(org.hl7.fhir.r4.model.CommunicationRequest.class); 1144 retVal.setContext(myFhirContext); 1145 return retVal; 1146 } 1147 1148 @Bean(name="myCommunicationRequestRpR4") 1149 @Lazy 1150 public ca.uhn.fhir.jpa.rp.r4.CommunicationRequestResourceProvider rpCommunicationRequestR4() { 1151 ca.uhn.fhir.jpa.rp.r4.CommunicationRequestResourceProvider retVal; 1152 retVal = new ca.uhn.fhir.jpa.rp.r4.CommunicationRequestResourceProvider(); 1153 retVal.setContext(myFhirContext); 1154 retVal.setDao(daoCommunicationRequestR4()); 1155 return retVal; 1156 } 1157 1158 @Bean(name="myCompartmentDefinitionDaoR4") 1159 public 1160 IFhirResourceDao<org.hl7.fhir.r4.model.CompartmentDefinition> 1161 daoCompartmentDefinitionR4() { 1162 1163 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.CompartmentDefinition> retVal; 1164 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.CompartmentDefinition>(); 1165 retVal.setResourceType(org.hl7.fhir.r4.model.CompartmentDefinition.class); 1166 retVal.setContext(myFhirContext); 1167 return retVal; 1168 } 1169 1170 @Bean(name="myCompartmentDefinitionRpR4") 1171 @Lazy 1172 public ca.uhn.fhir.jpa.rp.r4.CompartmentDefinitionResourceProvider rpCompartmentDefinitionR4() { 1173 ca.uhn.fhir.jpa.rp.r4.CompartmentDefinitionResourceProvider retVal; 1174 retVal = new ca.uhn.fhir.jpa.rp.r4.CompartmentDefinitionResourceProvider(); 1175 retVal.setContext(myFhirContext); 1176 retVal.setDao(daoCompartmentDefinitionR4()); 1177 return retVal; 1178 } 1179 1180 @Bean(name="myCompositionDaoR4") 1181 public 1182 IFhirResourceDaoComposition<org.hl7.fhir.r4.model.Composition> 1183 daoCompositionR4() { 1184 1185 ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<org.hl7.fhir.r4.model.Composition> retVal; 1186 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<>(); 1187 retVal.setResourceType(org.hl7.fhir.r4.model.Composition.class); 1188 retVal.setContext(myFhirContext); 1189 return retVal; 1190 } 1191 1192 @Bean(name="myCompositionRpR4") 1193 @Lazy 1194 public ca.uhn.fhir.jpa.rp.r4.CompositionResourceProvider rpCompositionR4() { 1195 ca.uhn.fhir.jpa.rp.r4.CompositionResourceProvider retVal; 1196 retVal = new ca.uhn.fhir.jpa.rp.r4.CompositionResourceProvider(); 1197 retVal.setContext(myFhirContext); 1198 retVal.setDao(daoCompositionR4()); 1199 return retVal; 1200 } 1201 1202 @Bean(name="myConceptMapDaoR4") 1203 public 1204 IFhirResourceDaoConceptMap<org.hl7.fhir.r4.model.ConceptMap> 1205 daoConceptMapR4() { 1206 1207 ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<org.hl7.fhir.r4.model.ConceptMap> retVal; 1208 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<>(); 1209 retVal.setResourceType(org.hl7.fhir.r4.model.ConceptMap.class); 1210 retVal.setContext(myFhirContext); 1211 return retVal; 1212 } 1213 1214 @Bean(name="myConceptMapRpR4") 1215 @Lazy 1216 public ca.uhn.fhir.jpa.rp.r4.ConceptMapResourceProvider rpConceptMapR4() { 1217 ca.uhn.fhir.jpa.rp.r4.ConceptMapResourceProvider retVal; 1218 retVal = new ca.uhn.fhir.jpa.rp.r4.ConceptMapResourceProvider(); 1219 retVal.setContext(myFhirContext); 1220 retVal.setDao(daoConceptMapR4()); 1221 return retVal; 1222 } 1223 1224 @Bean(name="myConditionDaoR4") 1225 public 1226 IFhirResourceDao<org.hl7.fhir.r4.model.Condition> 1227 daoConditionR4() { 1228 1229 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Condition> retVal; 1230 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Condition>(); 1231 retVal.setResourceType(org.hl7.fhir.r4.model.Condition.class); 1232 retVal.setContext(myFhirContext); 1233 return retVal; 1234 } 1235 1236 @Bean(name="myConditionRpR4") 1237 @Lazy 1238 public ca.uhn.fhir.jpa.rp.r4.ConditionResourceProvider rpConditionR4() { 1239 ca.uhn.fhir.jpa.rp.r4.ConditionResourceProvider retVal; 1240 retVal = new ca.uhn.fhir.jpa.rp.r4.ConditionResourceProvider(); 1241 retVal.setContext(myFhirContext); 1242 retVal.setDao(daoConditionR4()); 1243 return retVal; 1244 } 1245 1246 @Bean(name="myConsentDaoR4") 1247 public 1248 IFhirResourceDao<org.hl7.fhir.r4.model.Consent> 1249 daoConsentR4() { 1250 1251 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Consent> retVal; 1252 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Consent>(); 1253 retVal.setResourceType(org.hl7.fhir.r4.model.Consent.class); 1254 retVal.setContext(myFhirContext); 1255 return retVal; 1256 } 1257 1258 @Bean(name="myConsentRpR4") 1259 @Lazy 1260 public ca.uhn.fhir.jpa.rp.r4.ConsentResourceProvider rpConsentR4() { 1261 ca.uhn.fhir.jpa.rp.r4.ConsentResourceProvider retVal; 1262 retVal = new ca.uhn.fhir.jpa.rp.r4.ConsentResourceProvider(); 1263 retVal.setContext(myFhirContext); 1264 retVal.setDao(daoConsentR4()); 1265 return retVal; 1266 } 1267 1268 @Bean(name="myContractDaoR4") 1269 public 1270 IFhirResourceDao<org.hl7.fhir.r4.model.Contract> 1271 daoContractR4() { 1272 1273 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Contract> retVal; 1274 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Contract>(); 1275 retVal.setResourceType(org.hl7.fhir.r4.model.Contract.class); 1276 retVal.setContext(myFhirContext); 1277 return retVal; 1278 } 1279 1280 @Bean(name="myContractRpR4") 1281 @Lazy 1282 public ca.uhn.fhir.jpa.rp.r4.ContractResourceProvider rpContractR4() { 1283 ca.uhn.fhir.jpa.rp.r4.ContractResourceProvider retVal; 1284 retVal = new ca.uhn.fhir.jpa.rp.r4.ContractResourceProvider(); 1285 retVal.setContext(myFhirContext); 1286 retVal.setDao(daoContractR4()); 1287 return retVal; 1288 } 1289 1290 @Bean(name="myCoverageDaoR4") 1291 public 1292 IFhirResourceDao<org.hl7.fhir.r4.model.Coverage> 1293 daoCoverageR4() { 1294 1295 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Coverage> retVal; 1296 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Coverage>(); 1297 retVal.setResourceType(org.hl7.fhir.r4.model.Coverage.class); 1298 retVal.setContext(myFhirContext); 1299 return retVal; 1300 } 1301 1302 @Bean(name="myCoverageRpR4") 1303 @Lazy 1304 public ca.uhn.fhir.jpa.rp.r4.CoverageResourceProvider rpCoverageR4() { 1305 ca.uhn.fhir.jpa.rp.r4.CoverageResourceProvider retVal; 1306 retVal = new ca.uhn.fhir.jpa.rp.r4.CoverageResourceProvider(); 1307 retVal.setContext(myFhirContext); 1308 retVal.setDao(daoCoverageR4()); 1309 return retVal; 1310 } 1311 1312 @Bean(name="myCoverageEligibilityRequestDaoR4") 1313 public 1314 IFhirResourceDao<org.hl7.fhir.r4.model.CoverageEligibilityRequest> 1315 daoCoverageEligibilityRequestR4() { 1316 1317 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.CoverageEligibilityRequest> retVal; 1318 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.CoverageEligibilityRequest>(); 1319 retVal.setResourceType(org.hl7.fhir.r4.model.CoverageEligibilityRequest.class); 1320 retVal.setContext(myFhirContext); 1321 return retVal; 1322 } 1323 1324 @Bean(name="myCoverageEligibilityRequestRpR4") 1325 @Lazy 1326 public ca.uhn.fhir.jpa.rp.r4.CoverageEligibilityRequestResourceProvider rpCoverageEligibilityRequestR4() { 1327 ca.uhn.fhir.jpa.rp.r4.CoverageEligibilityRequestResourceProvider retVal; 1328 retVal = new ca.uhn.fhir.jpa.rp.r4.CoverageEligibilityRequestResourceProvider(); 1329 retVal.setContext(myFhirContext); 1330 retVal.setDao(daoCoverageEligibilityRequestR4()); 1331 return retVal; 1332 } 1333 1334 @Bean(name="myCoverageEligibilityResponseDaoR4") 1335 public 1336 IFhirResourceDao<org.hl7.fhir.r4.model.CoverageEligibilityResponse> 1337 daoCoverageEligibilityResponseR4() { 1338 1339 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.CoverageEligibilityResponse> retVal; 1340 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.CoverageEligibilityResponse>(); 1341 retVal.setResourceType(org.hl7.fhir.r4.model.CoverageEligibilityResponse.class); 1342 retVal.setContext(myFhirContext); 1343 return retVal; 1344 } 1345 1346 @Bean(name="myCoverageEligibilityResponseRpR4") 1347 @Lazy 1348 public ca.uhn.fhir.jpa.rp.r4.CoverageEligibilityResponseResourceProvider rpCoverageEligibilityResponseR4() { 1349 ca.uhn.fhir.jpa.rp.r4.CoverageEligibilityResponseResourceProvider retVal; 1350 retVal = new ca.uhn.fhir.jpa.rp.r4.CoverageEligibilityResponseResourceProvider(); 1351 retVal.setContext(myFhirContext); 1352 retVal.setDao(daoCoverageEligibilityResponseR4()); 1353 return retVal; 1354 } 1355 1356 @Bean(name="myDetectedIssueDaoR4") 1357 public 1358 IFhirResourceDao<org.hl7.fhir.r4.model.DetectedIssue> 1359 daoDetectedIssueR4() { 1360 1361 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.DetectedIssue> retVal; 1362 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.DetectedIssue>(); 1363 retVal.setResourceType(org.hl7.fhir.r4.model.DetectedIssue.class); 1364 retVal.setContext(myFhirContext); 1365 return retVal; 1366 } 1367 1368 @Bean(name="myDetectedIssueRpR4") 1369 @Lazy 1370 public ca.uhn.fhir.jpa.rp.r4.DetectedIssueResourceProvider rpDetectedIssueR4() { 1371 ca.uhn.fhir.jpa.rp.r4.DetectedIssueResourceProvider retVal; 1372 retVal = new ca.uhn.fhir.jpa.rp.r4.DetectedIssueResourceProvider(); 1373 retVal.setContext(myFhirContext); 1374 retVal.setDao(daoDetectedIssueR4()); 1375 return retVal; 1376 } 1377 1378 @Bean(name="myDeviceDaoR4") 1379 public 1380 IFhirResourceDao<org.hl7.fhir.r4.model.Device> 1381 daoDeviceR4() { 1382 1383 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Device> retVal; 1384 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Device>(); 1385 retVal.setResourceType(org.hl7.fhir.r4.model.Device.class); 1386 retVal.setContext(myFhirContext); 1387 return retVal; 1388 } 1389 1390 @Bean(name="myDeviceRpR4") 1391 @Lazy 1392 public ca.uhn.fhir.jpa.rp.r4.DeviceResourceProvider rpDeviceR4() { 1393 ca.uhn.fhir.jpa.rp.r4.DeviceResourceProvider retVal; 1394 retVal = new ca.uhn.fhir.jpa.rp.r4.DeviceResourceProvider(); 1395 retVal.setContext(myFhirContext); 1396 retVal.setDao(daoDeviceR4()); 1397 return retVal; 1398 } 1399 1400 @Bean(name="myDeviceDefinitionDaoR4") 1401 public 1402 IFhirResourceDao<org.hl7.fhir.r4.model.DeviceDefinition> 1403 daoDeviceDefinitionR4() { 1404 1405 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.DeviceDefinition> retVal; 1406 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.DeviceDefinition>(); 1407 retVal.setResourceType(org.hl7.fhir.r4.model.DeviceDefinition.class); 1408 retVal.setContext(myFhirContext); 1409 return retVal; 1410 } 1411 1412 @Bean(name="myDeviceDefinitionRpR4") 1413 @Lazy 1414 public ca.uhn.fhir.jpa.rp.r4.DeviceDefinitionResourceProvider rpDeviceDefinitionR4() { 1415 ca.uhn.fhir.jpa.rp.r4.DeviceDefinitionResourceProvider retVal; 1416 retVal = new ca.uhn.fhir.jpa.rp.r4.DeviceDefinitionResourceProvider(); 1417 retVal.setContext(myFhirContext); 1418 retVal.setDao(daoDeviceDefinitionR4()); 1419 return retVal; 1420 } 1421 1422 @Bean(name="myDeviceMetricDaoR4") 1423 public 1424 IFhirResourceDao<org.hl7.fhir.r4.model.DeviceMetric> 1425 daoDeviceMetricR4() { 1426 1427 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.DeviceMetric> retVal; 1428 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.DeviceMetric>(); 1429 retVal.setResourceType(org.hl7.fhir.r4.model.DeviceMetric.class); 1430 retVal.setContext(myFhirContext); 1431 return retVal; 1432 } 1433 1434 @Bean(name="myDeviceMetricRpR4") 1435 @Lazy 1436 public ca.uhn.fhir.jpa.rp.r4.DeviceMetricResourceProvider rpDeviceMetricR4() { 1437 ca.uhn.fhir.jpa.rp.r4.DeviceMetricResourceProvider retVal; 1438 retVal = new ca.uhn.fhir.jpa.rp.r4.DeviceMetricResourceProvider(); 1439 retVal.setContext(myFhirContext); 1440 retVal.setDao(daoDeviceMetricR4()); 1441 return retVal; 1442 } 1443 1444 @Bean(name="myDeviceRequestDaoR4") 1445 public 1446 IFhirResourceDao<org.hl7.fhir.r4.model.DeviceRequest> 1447 daoDeviceRequestR4() { 1448 1449 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.DeviceRequest> retVal; 1450 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.DeviceRequest>(); 1451 retVal.setResourceType(org.hl7.fhir.r4.model.DeviceRequest.class); 1452 retVal.setContext(myFhirContext); 1453 return retVal; 1454 } 1455 1456 @Bean(name="myDeviceRequestRpR4") 1457 @Lazy 1458 public ca.uhn.fhir.jpa.rp.r4.DeviceRequestResourceProvider rpDeviceRequestR4() { 1459 ca.uhn.fhir.jpa.rp.r4.DeviceRequestResourceProvider retVal; 1460 retVal = new ca.uhn.fhir.jpa.rp.r4.DeviceRequestResourceProvider(); 1461 retVal.setContext(myFhirContext); 1462 retVal.setDao(daoDeviceRequestR4()); 1463 return retVal; 1464 } 1465 1466 @Bean(name="myDeviceUseStatementDaoR4") 1467 public 1468 IFhirResourceDao<org.hl7.fhir.r4.model.DeviceUseStatement> 1469 daoDeviceUseStatementR4() { 1470 1471 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.DeviceUseStatement> retVal; 1472 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.DeviceUseStatement>(); 1473 retVal.setResourceType(org.hl7.fhir.r4.model.DeviceUseStatement.class); 1474 retVal.setContext(myFhirContext); 1475 return retVal; 1476 } 1477 1478 @Bean(name="myDeviceUseStatementRpR4") 1479 @Lazy 1480 public ca.uhn.fhir.jpa.rp.r4.DeviceUseStatementResourceProvider rpDeviceUseStatementR4() { 1481 ca.uhn.fhir.jpa.rp.r4.DeviceUseStatementResourceProvider retVal; 1482 retVal = new ca.uhn.fhir.jpa.rp.r4.DeviceUseStatementResourceProvider(); 1483 retVal.setContext(myFhirContext); 1484 retVal.setDao(daoDeviceUseStatementR4()); 1485 return retVal; 1486 } 1487 1488 @Bean(name="myDiagnosticReportDaoR4") 1489 public 1490 IFhirResourceDao<org.hl7.fhir.r4.model.DiagnosticReport> 1491 daoDiagnosticReportR4() { 1492 1493 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.DiagnosticReport> retVal; 1494 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.DiagnosticReport>(); 1495 retVal.setResourceType(org.hl7.fhir.r4.model.DiagnosticReport.class); 1496 retVal.setContext(myFhirContext); 1497 return retVal; 1498 } 1499 1500 @Bean(name="myDiagnosticReportRpR4") 1501 @Lazy 1502 public ca.uhn.fhir.jpa.rp.r4.DiagnosticReportResourceProvider rpDiagnosticReportR4() { 1503 ca.uhn.fhir.jpa.rp.r4.DiagnosticReportResourceProvider retVal; 1504 retVal = new ca.uhn.fhir.jpa.rp.r4.DiagnosticReportResourceProvider(); 1505 retVal.setContext(myFhirContext); 1506 retVal.setDao(daoDiagnosticReportR4()); 1507 return retVal; 1508 } 1509 1510 @Bean(name="myDocumentManifestDaoR4") 1511 public 1512 IFhirResourceDao<org.hl7.fhir.r4.model.DocumentManifest> 1513 daoDocumentManifestR4() { 1514 1515 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.DocumentManifest> retVal; 1516 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.DocumentManifest>(); 1517 retVal.setResourceType(org.hl7.fhir.r4.model.DocumentManifest.class); 1518 retVal.setContext(myFhirContext); 1519 return retVal; 1520 } 1521 1522 @Bean(name="myDocumentManifestRpR4") 1523 @Lazy 1524 public ca.uhn.fhir.jpa.rp.r4.DocumentManifestResourceProvider rpDocumentManifestR4() { 1525 ca.uhn.fhir.jpa.rp.r4.DocumentManifestResourceProvider retVal; 1526 retVal = new ca.uhn.fhir.jpa.rp.r4.DocumentManifestResourceProvider(); 1527 retVal.setContext(myFhirContext); 1528 retVal.setDao(daoDocumentManifestR4()); 1529 return retVal; 1530 } 1531 1532 @Bean(name="myDocumentReferenceDaoR4") 1533 public 1534 IFhirResourceDao<org.hl7.fhir.r4.model.DocumentReference> 1535 daoDocumentReferenceR4() { 1536 1537 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.DocumentReference> retVal; 1538 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.DocumentReference>(); 1539 retVal.setResourceType(org.hl7.fhir.r4.model.DocumentReference.class); 1540 retVal.setContext(myFhirContext); 1541 return retVal; 1542 } 1543 1544 @Bean(name="myDocumentReferenceRpR4") 1545 @Lazy 1546 public ca.uhn.fhir.jpa.rp.r4.DocumentReferenceResourceProvider rpDocumentReferenceR4() { 1547 ca.uhn.fhir.jpa.rp.r4.DocumentReferenceResourceProvider retVal; 1548 retVal = new ca.uhn.fhir.jpa.rp.r4.DocumentReferenceResourceProvider(); 1549 retVal.setContext(myFhirContext); 1550 retVal.setDao(daoDocumentReferenceR4()); 1551 return retVal; 1552 } 1553 1554 @Bean(name="myEffectEvidenceSynthesisDaoR4") 1555 public 1556 IFhirResourceDao<org.hl7.fhir.r4.model.EffectEvidenceSynthesis> 1557 daoEffectEvidenceSynthesisR4() { 1558 1559 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.EffectEvidenceSynthesis> retVal; 1560 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.EffectEvidenceSynthesis>(); 1561 retVal.setResourceType(org.hl7.fhir.r4.model.EffectEvidenceSynthesis.class); 1562 retVal.setContext(myFhirContext); 1563 return retVal; 1564 } 1565 1566 @Bean(name="myEffectEvidenceSynthesisRpR4") 1567 @Lazy 1568 public ca.uhn.fhir.jpa.rp.r4.EffectEvidenceSynthesisResourceProvider rpEffectEvidenceSynthesisR4() { 1569 ca.uhn.fhir.jpa.rp.r4.EffectEvidenceSynthesisResourceProvider retVal; 1570 retVal = new ca.uhn.fhir.jpa.rp.r4.EffectEvidenceSynthesisResourceProvider(); 1571 retVal.setContext(myFhirContext); 1572 retVal.setDao(daoEffectEvidenceSynthesisR4()); 1573 return retVal; 1574 } 1575 1576 @Bean(name="myEncounterDaoR4") 1577 public 1578 IFhirResourceDaoEncounter<org.hl7.fhir.r4.model.Encounter> 1579 daoEncounterR4() { 1580 1581 ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<org.hl7.fhir.r4.model.Encounter> retVal; 1582 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<>(); 1583 retVal.setResourceType(org.hl7.fhir.r4.model.Encounter.class); 1584 retVal.setContext(myFhirContext); 1585 return retVal; 1586 } 1587 1588 @Bean(name="myEncounterRpR4") 1589 @Lazy 1590 public ca.uhn.fhir.jpa.rp.r4.EncounterResourceProvider rpEncounterR4() { 1591 ca.uhn.fhir.jpa.rp.r4.EncounterResourceProvider retVal; 1592 retVal = new ca.uhn.fhir.jpa.rp.r4.EncounterResourceProvider(); 1593 retVal.setContext(myFhirContext); 1594 retVal.setDao(daoEncounterR4()); 1595 return retVal; 1596 } 1597 1598 @Bean(name="myEndpointDaoR4") 1599 public 1600 IFhirResourceDao<org.hl7.fhir.r4.model.Endpoint> 1601 daoEndpointR4() { 1602 1603 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Endpoint> retVal; 1604 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Endpoint>(); 1605 retVal.setResourceType(org.hl7.fhir.r4.model.Endpoint.class); 1606 retVal.setContext(myFhirContext); 1607 return retVal; 1608 } 1609 1610 @Bean(name="myEndpointRpR4") 1611 @Lazy 1612 public ca.uhn.fhir.jpa.rp.r4.EndpointResourceProvider rpEndpointR4() { 1613 ca.uhn.fhir.jpa.rp.r4.EndpointResourceProvider retVal; 1614 retVal = new ca.uhn.fhir.jpa.rp.r4.EndpointResourceProvider(); 1615 retVal.setContext(myFhirContext); 1616 retVal.setDao(daoEndpointR4()); 1617 return retVal; 1618 } 1619 1620 @Bean(name="myEnrollmentRequestDaoR4") 1621 public 1622 IFhirResourceDao<org.hl7.fhir.r4.model.EnrollmentRequest> 1623 daoEnrollmentRequestR4() { 1624 1625 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.EnrollmentRequest> retVal; 1626 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.EnrollmentRequest>(); 1627 retVal.setResourceType(org.hl7.fhir.r4.model.EnrollmentRequest.class); 1628 retVal.setContext(myFhirContext); 1629 return retVal; 1630 } 1631 1632 @Bean(name="myEnrollmentRequestRpR4") 1633 @Lazy 1634 public ca.uhn.fhir.jpa.rp.r4.EnrollmentRequestResourceProvider rpEnrollmentRequestR4() { 1635 ca.uhn.fhir.jpa.rp.r4.EnrollmentRequestResourceProvider retVal; 1636 retVal = new ca.uhn.fhir.jpa.rp.r4.EnrollmentRequestResourceProvider(); 1637 retVal.setContext(myFhirContext); 1638 retVal.setDao(daoEnrollmentRequestR4()); 1639 return retVal; 1640 } 1641 1642 @Bean(name="myEnrollmentResponseDaoR4") 1643 public 1644 IFhirResourceDao<org.hl7.fhir.r4.model.EnrollmentResponse> 1645 daoEnrollmentResponseR4() { 1646 1647 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.EnrollmentResponse> retVal; 1648 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.EnrollmentResponse>(); 1649 retVal.setResourceType(org.hl7.fhir.r4.model.EnrollmentResponse.class); 1650 retVal.setContext(myFhirContext); 1651 return retVal; 1652 } 1653 1654 @Bean(name="myEnrollmentResponseRpR4") 1655 @Lazy 1656 public ca.uhn.fhir.jpa.rp.r4.EnrollmentResponseResourceProvider rpEnrollmentResponseR4() { 1657 ca.uhn.fhir.jpa.rp.r4.EnrollmentResponseResourceProvider retVal; 1658 retVal = new ca.uhn.fhir.jpa.rp.r4.EnrollmentResponseResourceProvider(); 1659 retVal.setContext(myFhirContext); 1660 retVal.setDao(daoEnrollmentResponseR4()); 1661 return retVal; 1662 } 1663 1664 @Bean(name="myEpisodeOfCareDaoR4") 1665 public 1666 IFhirResourceDao<org.hl7.fhir.r4.model.EpisodeOfCare> 1667 daoEpisodeOfCareR4() { 1668 1669 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.EpisodeOfCare> retVal; 1670 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.EpisodeOfCare>(); 1671 retVal.setResourceType(org.hl7.fhir.r4.model.EpisodeOfCare.class); 1672 retVal.setContext(myFhirContext); 1673 return retVal; 1674 } 1675 1676 @Bean(name="myEpisodeOfCareRpR4") 1677 @Lazy 1678 public ca.uhn.fhir.jpa.rp.r4.EpisodeOfCareResourceProvider rpEpisodeOfCareR4() { 1679 ca.uhn.fhir.jpa.rp.r4.EpisodeOfCareResourceProvider retVal; 1680 retVal = new ca.uhn.fhir.jpa.rp.r4.EpisodeOfCareResourceProvider(); 1681 retVal.setContext(myFhirContext); 1682 retVal.setDao(daoEpisodeOfCareR4()); 1683 return retVal; 1684 } 1685 1686 @Bean(name="myEventDefinitionDaoR4") 1687 public 1688 IFhirResourceDao<org.hl7.fhir.r4.model.EventDefinition> 1689 daoEventDefinitionR4() { 1690 1691 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.EventDefinition> retVal; 1692 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.EventDefinition>(); 1693 retVal.setResourceType(org.hl7.fhir.r4.model.EventDefinition.class); 1694 retVal.setContext(myFhirContext); 1695 return retVal; 1696 } 1697 1698 @Bean(name="myEventDefinitionRpR4") 1699 @Lazy 1700 public ca.uhn.fhir.jpa.rp.r4.EventDefinitionResourceProvider rpEventDefinitionR4() { 1701 ca.uhn.fhir.jpa.rp.r4.EventDefinitionResourceProvider retVal; 1702 retVal = new ca.uhn.fhir.jpa.rp.r4.EventDefinitionResourceProvider(); 1703 retVal.setContext(myFhirContext); 1704 retVal.setDao(daoEventDefinitionR4()); 1705 return retVal; 1706 } 1707 1708 @Bean(name="myEvidenceDaoR4") 1709 public 1710 IFhirResourceDao<org.hl7.fhir.r4.model.Evidence> 1711 daoEvidenceR4() { 1712 1713 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Evidence> retVal; 1714 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Evidence>(); 1715 retVal.setResourceType(org.hl7.fhir.r4.model.Evidence.class); 1716 retVal.setContext(myFhirContext); 1717 return retVal; 1718 } 1719 1720 @Bean(name="myEvidenceRpR4") 1721 @Lazy 1722 public ca.uhn.fhir.jpa.rp.r4.EvidenceResourceProvider rpEvidenceR4() { 1723 ca.uhn.fhir.jpa.rp.r4.EvidenceResourceProvider retVal; 1724 retVal = new ca.uhn.fhir.jpa.rp.r4.EvidenceResourceProvider(); 1725 retVal.setContext(myFhirContext); 1726 retVal.setDao(daoEvidenceR4()); 1727 return retVal; 1728 } 1729 1730 @Bean(name="myEvidenceVariableDaoR4") 1731 public 1732 IFhirResourceDao<org.hl7.fhir.r4.model.EvidenceVariable> 1733 daoEvidenceVariableR4() { 1734 1735 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.EvidenceVariable> retVal; 1736 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.EvidenceVariable>(); 1737 retVal.setResourceType(org.hl7.fhir.r4.model.EvidenceVariable.class); 1738 retVal.setContext(myFhirContext); 1739 return retVal; 1740 } 1741 1742 @Bean(name="myEvidenceVariableRpR4") 1743 @Lazy 1744 public ca.uhn.fhir.jpa.rp.r4.EvidenceVariableResourceProvider rpEvidenceVariableR4() { 1745 ca.uhn.fhir.jpa.rp.r4.EvidenceVariableResourceProvider retVal; 1746 retVal = new ca.uhn.fhir.jpa.rp.r4.EvidenceVariableResourceProvider(); 1747 retVal.setContext(myFhirContext); 1748 retVal.setDao(daoEvidenceVariableR4()); 1749 return retVal; 1750 } 1751 1752 @Bean(name="myExampleScenarioDaoR4") 1753 public 1754 IFhirResourceDao<org.hl7.fhir.r4.model.ExampleScenario> 1755 daoExampleScenarioR4() { 1756 1757 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ExampleScenario> retVal; 1758 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ExampleScenario>(); 1759 retVal.setResourceType(org.hl7.fhir.r4.model.ExampleScenario.class); 1760 retVal.setContext(myFhirContext); 1761 return retVal; 1762 } 1763 1764 @Bean(name="myExampleScenarioRpR4") 1765 @Lazy 1766 public ca.uhn.fhir.jpa.rp.r4.ExampleScenarioResourceProvider rpExampleScenarioR4() { 1767 ca.uhn.fhir.jpa.rp.r4.ExampleScenarioResourceProvider retVal; 1768 retVal = new ca.uhn.fhir.jpa.rp.r4.ExampleScenarioResourceProvider(); 1769 retVal.setContext(myFhirContext); 1770 retVal.setDao(daoExampleScenarioR4()); 1771 return retVal; 1772 } 1773 1774 @Bean(name="myExplanationOfBenefitDaoR4") 1775 public 1776 IFhirResourceDao<org.hl7.fhir.r4.model.ExplanationOfBenefit> 1777 daoExplanationOfBenefitR4() { 1778 1779 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ExplanationOfBenefit> retVal; 1780 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ExplanationOfBenefit>(); 1781 retVal.setResourceType(org.hl7.fhir.r4.model.ExplanationOfBenefit.class); 1782 retVal.setContext(myFhirContext); 1783 return retVal; 1784 } 1785 1786 @Bean(name="myExplanationOfBenefitRpR4") 1787 @Lazy 1788 public ca.uhn.fhir.jpa.rp.r4.ExplanationOfBenefitResourceProvider rpExplanationOfBenefitR4() { 1789 ca.uhn.fhir.jpa.rp.r4.ExplanationOfBenefitResourceProvider retVal; 1790 retVal = new ca.uhn.fhir.jpa.rp.r4.ExplanationOfBenefitResourceProvider(); 1791 retVal.setContext(myFhirContext); 1792 retVal.setDao(daoExplanationOfBenefitR4()); 1793 return retVal; 1794 } 1795 1796 @Bean(name="myFamilyMemberHistoryDaoR4") 1797 public 1798 IFhirResourceDao<org.hl7.fhir.r4.model.FamilyMemberHistory> 1799 daoFamilyMemberHistoryR4() { 1800 1801 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.FamilyMemberHistory> retVal; 1802 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.FamilyMemberHistory>(); 1803 retVal.setResourceType(org.hl7.fhir.r4.model.FamilyMemberHistory.class); 1804 retVal.setContext(myFhirContext); 1805 return retVal; 1806 } 1807 1808 @Bean(name="myFamilyMemberHistoryRpR4") 1809 @Lazy 1810 public ca.uhn.fhir.jpa.rp.r4.FamilyMemberHistoryResourceProvider rpFamilyMemberHistoryR4() { 1811 ca.uhn.fhir.jpa.rp.r4.FamilyMemberHistoryResourceProvider retVal; 1812 retVal = new ca.uhn.fhir.jpa.rp.r4.FamilyMemberHistoryResourceProvider(); 1813 retVal.setContext(myFhirContext); 1814 retVal.setDao(daoFamilyMemberHistoryR4()); 1815 return retVal; 1816 } 1817 1818 @Bean(name="myFlagDaoR4") 1819 public 1820 IFhirResourceDao<org.hl7.fhir.r4.model.Flag> 1821 daoFlagR4() { 1822 1823 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Flag> retVal; 1824 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Flag>(); 1825 retVal.setResourceType(org.hl7.fhir.r4.model.Flag.class); 1826 retVal.setContext(myFhirContext); 1827 return retVal; 1828 } 1829 1830 @Bean(name="myFlagRpR4") 1831 @Lazy 1832 public ca.uhn.fhir.jpa.rp.r4.FlagResourceProvider rpFlagR4() { 1833 ca.uhn.fhir.jpa.rp.r4.FlagResourceProvider retVal; 1834 retVal = new ca.uhn.fhir.jpa.rp.r4.FlagResourceProvider(); 1835 retVal.setContext(myFhirContext); 1836 retVal.setDao(daoFlagR4()); 1837 return retVal; 1838 } 1839 1840 @Bean(name="myGoalDaoR4") 1841 public 1842 IFhirResourceDao<org.hl7.fhir.r4.model.Goal> 1843 daoGoalR4() { 1844 1845 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Goal> retVal; 1846 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Goal>(); 1847 retVal.setResourceType(org.hl7.fhir.r4.model.Goal.class); 1848 retVal.setContext(myFhirContext); 1849 return retVal; 1850 } 1851 1852 @Bean(name="myGoalRpR4") 1853 @Lazy 1854 public ca.uhn.fhir.jpa.rp.r4.GoalResourceProvider rpGoalR4() { 1855 ca.uhn.fhir.jpa.rp.r4.GoalResourceProvider retVal; 1856 retVal = new ca.uhn.fhir.jpa.rp.r4.GoalResourceProvider(); 1857 retVal.setContext(myFhirContext); 1858 retVal.setDao(daoGoalR4()); 1859 return retVal; 1860 } 1861 1862 @Bean(name="myGraphDefinitionDaoR4") 1863 public 1864 IFhirResourceDao<org.hl7.fhir.r4.model.GraphDefinition> 1865 daoGraphDefinitionR4() { 1866 1867 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.GraphDefinition> retVal; 1868 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.GraphDefinition>(); 1869 retVal.setResourceType(org.hl7.fhir.r4.model.GraphDefinition.class); 1870 retVal.setContext(myFhirContext); 1871 return retVal; 1872 } 1873 1874 @Bean(name="myGraphDefinitionRpR4") 1875 @Lazy 1876 public ca.uhn.fhir.jpa.rp.r4.GraphDefinitionResourceProvider rpGraphDefinitionR4() { 1877 ca.uhn.fhir.jpa.rp.r4.GraphDefinitionResourceProvider retVal; 1878 retVal = new ca.uhn.fhir.jpa.rp.r4.GraphDefinitionResourceProvider(); 1879 retVal.setContext(myFhirContext); 1880 retVal.setDao(daoGraphDefinitionR4()); 1881 return retVal; 1882 } 1883 1884 @Bean(name="myGroupDaoR4") 1885 public 1886 IFhirResourceDao<org.hl7.fhir.r4.model.Group> 1887 daoGroupR4() { 1888 1889 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Group> retVal; 1890 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Group>(); 1891 retVal.setResourceType(org.hl7.fhir.r4.model.Group.class); 1892 retVal.setContext(myFhirContext); 1893 return retVal; 1894 } 1895 1896 @Bean(name="myGroupRpR4") 1897 @Lazy 1898 public ca.uhn.fhir.jpa.rp.r4.GroupResourceProvider rpGroupR4() { 1899 ca.uhn.fhir.jpa.rp.r4.GroupResourceProvider retVal; 1900 retVal = new ca.uhn.fhir.jpa.rp.r4.GroupResourceProvider(); 1901 retVal.setContext(myFhirContext); 1902 retVal.setDao(daoGroupR4()); 1903 return retVal; 1904 } 1905 1906 @Bean(name="myGuidanceResponseDaoR4") 1907 public 1908 IFhirResourceDao<org.hl7.fhir.r4.model.GuidanceResponse> 1909 daoGuidanceResponseR4() { 1910 1911 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.GuidanceResponse> retVal; 1912 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.GuidanceResponse>(); 1913 retVal.setResourceType(org.hl7.fhir.r4.model.GuidanceResponse.class); 1914 retVal.setContext(myFhirContext); 1915 return retVal; 1916 } 1917 1918 @Bean(name="myGuidanceResponseRpR4") 1919 @Lazy 1920 public ca.uhn.fhir.jpa.rp.r4.GuidanceResponseResourceProvider rpGuidanceResponseR4() { 1921 ca.uhn.fhir.jpa.rp.r4.GuidanceResponseResourceProvider retVal; 1922 retVal = new ca.uhn.fhir.jpa.rp.r4.GuidanceResponseResourceProvider(); 1923 retVal.setContext(myFhirContext); 1924 retVal.setDao(daoGuidanceResponseR4()); 1925 return retVal; 1926 } 1927 1928 @Bean(name="myHealthcareServiceDaoR4") 1929 public 1930 IFhirResourceDao<org.hl7.fhir.r4.model.HealthcareService> 1931 daoHealthcareServiceR4() { 1932 1933 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.HealthcareService> retVal; 1934 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.HealthcareService>(); 1935 retVal.setResourceType(org.hl7.fhir.r4.model.HealthcareService.class); 1936 retVal.setContext(myFhirContext); 1937 return retVal; 1938 } 1939 1940 @Bean(name="myHealthcareServiceRpR4") 1941 @Lazy 1942 public ca.uhn.fhir.jpa.rp.r4.HealthcareServiceResourceProvider rpHealthcareServiceR4() { 1943 ca.uhn.fhir.jpa.rp.r4.HealthcareServiceResourceProvider retVal; 1944 retVal = new ca.uhn.fhir.jpa.rp.r4.HealthcareServiceResourceProvider(); 1945 retVal.setContext(myFhirContext); 1946 retVal.setDao(daoHealthcareServiceR4()); 1947 return retVal; 1948 } 1949 1950 @Bean(name="myImagingStudyDaoR4") 1951 public 1952 IFhirResourceDao<org.hl7.fhir.r4.model.ImagingStudy> 1953 daoImagingStudyR4() { 1954 1955 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ImagingStudy> retVal; 1956 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ImagingStudy>(); 1957 retVal.setResourceType(org.hl7.fhir.r4.model.ImagingStudy.class); 1958 retVal.setContext(myFhirContext); 1959 return retVal; 1960 } 1961 1962 @Bean(name="myImagingStudyRpR4") 1963 @Lazy 1964 public ca.uhn.fhir.jpa.rp.r4.ImagingStudyResourceProvider rpImagingStudyR4() { 1965 ca.uhn.fhir.jpa.rp.r4.ImagingStudyResourceProvider retVal; 1966 retVal = new ca.uhn.fhir.jpa.rp.r4.ImagingStudyResourceProvider(); 1967 retVal.setContext(myFhirContext); 1968 retVal.setDao(daoImagingStudyR4()); 1969 return retVal; 1970 } 1971 1972 @Bean(name="myImmunizationDaoR4") 1973 public 1974 IFhirResourceDao<org.hl7.fhir.r4.model.Immunization> 1975 daoImmunizationR4() { 1976 1977 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Immunization> retVal; 1978 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Immunization>(); 1979 retVal.setResourceType(org.hl7.fhir.r4.model.Immunization.class); 1980 retVal.setContext(myFhirContext); 1981 return retVal; 1982 } 1983 1984 @Bean(name="myImmunizationRpR4") 1985 @Lazy 1986 public ca.uhn.fhir.jpa.rp.r4.ImmunizationResourceProvider rpImmunizationR4() { 1987 ca.uhn.fhir.jpa.rp.r4.ImmunizationResourceProvider retVal; 1988 retVal = new ca.uhn.fhir.jpa.rp.r4.ImmunizationResourceProvider(); 1989 retVal.setContext(myFhirContext); 1990 retVal.setDao(daoImmunizationR4()); 1991 return retVal; 1992 } 1993 1994 @Bean(name="myImmunizationEvaluationDaoR4") 1995 public 1996 IFhirResourceDao<org.hl7.fhir.r4.model.ImmunizationEvaluation> 1997 daoImmunizationEvaluationR4() { 1998 1999 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ImmunizationEvaluation> retVal; 2000 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ImmunizationEvaluation>(); 2001 retVal.setResourceType(org.hl7.fhir.r4.model.ImmunizationEvaluation.class); 2002 retVal.setContext(myFhirContext); 2003 return retVal; 2004 } 2005 2006 @Bean(name="myImmunizationEvaluationRpR4") 2007 @Lazy 2008 public ca.uhn.fhir.jpa.rp.r4.ImmunizationEvaluationResourceProvider rpImmunizationEvaluationR4() { 2009 ca.uhn.fhir.jpa.rp.r4.ImmunizationEvaluationResourceProvider retVal; 2010 retVal = new ca.uhn.fhir.jpa.rp.r4.ImmunizationEvaluationResourceProvider(); 2011 retVal.setContext(myFhirContext); 2012 retVal.setDao(daoImmunizationEvaluationR4()); 2013 return retVal; 2014 } 2015 2016 @Bean(name="myImmunizationRecommendationDaoR4") 2017 public 2018 IFhirResourceDao<org.hl7.fhir.r4.model.ImmunizationRecommendation> 2019 daoImmunizationRecommendationR4() { 2020 2021 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ImmunizationRecommendation> retVal; 2022 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ImmunizationRecommendation>(); 2023 retVal.setResourceType(org.hl7.fhir.r4.model.ImmunizationRecommendation.class); 2024 retVal.setContext(myFhirContext); 2025 return retVal; 2026 } 2027 2028 @Bean(name="myImmunizationRecommendationRpR4") 2029 @Lazy 2030 public ca.uhn.fhir.jpa.rp.r4.ImmunizationRecommendationResourceProvider rpImmunizationRecommendationR4() { 2031 ca.uhn.fhir.jpa.rp.r4.ImmunizationRecommendationResourceProvider retVal; 2032 retVal = new ca.uhn.fhir.jpa.rp.r4.ImmunizationRecommendationResourceProvider(); 2033 retVal.setContext(myFhirContext); 2034 retVal.setDao(daoImmunizationRecommendationR4()); 2035 return retVal; 2036 } 2037 2038 @Bean(name="myImplementationGuideDaoR4") 2039 public 2040 IFhirResourceDao<org.hl7.fhir.r4.model.ImplementationGuide> 2041 daoImplementationGuideR4() { 2042 2043 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ImplementationGuide> retVal; 2044 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ImplementationGuide>(); 2045 retVal.setResourceType(org.hl7.fhir.r4.model.ImplementationGuide.class); 2046 retVal.setContext(myFhirContext); 2047 return retVal; 2048 } 2049 2050 @Bean(name="myImplementationGuideRpR4") 2051 @Lazy 2052 public ca.uhn.fhir.jpa.rp.r4.ImplementationGuideResourceProvider rpImplementationGuideR4() { 2053 ca.uhn.fhir.jpa.rp.r4.ImplementationGuideResourceProvider retVal; 2054 retVal = new ca.uhn.fhir.jpa.rp.r4.ImplementationGuideResourceProvider(); 2055 retVal.setContext(myFhirContext); 2056 retVal.setDao(daoImplementationGuideR4()); 2057 return retVal; 2058 } 2059 2060 @Bean(name="myInsurancePlanDaoR4") 2061 public 2062 IFhirResourceDao<org.hl7.fhir.r4.model.InsurancePlan> 2063 daoInsurancePlanR4() { 2064 2065 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.InsurancePlan> retVal; 2066 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.InsurancePlan>(); 2067 retVal.setResourceType(org.hl7.fhir.r4.model.InsurancePlan.class); 2068 retVal.setContext(myFhirContext); 2069 return retVal; 2070 } 2071 2072 @Bean(name="myInsurancePlanRpR4") 2073 @Lazy 2074 public ca.uhn.fhir.jpa.rp.r4.InsurancePlanResourceProvider rpInsurancePlanR4() { 2075 ca.uhn.fhir.jpa.rp.r4.InsurancePlanResourceProvider retVal; 2076 retVal = new ca.uhn.fhir.jpa.rp.r4.InsurancePlanResourceProvider(); 2077 retVal.setContext(myFhirContext); 2078 retVal.setDao(daoInsurancePlanR4()); 2079 return retVal; 2080 } 2081 2082 @Bean(name="myInvoiceDaoR4") 2083 public 2084 IFhirResourceDao<org.hl7.fhir.r4.model.Invoice> 2085 daoInvoiceR4() { 2086 2087 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Invoice> retVal; 2088 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Invoice>(); 2089 retVal.setResourceType(org.hl7.fhir.r4.model.Invoice.class); 2090 retVal.setContext(myFhirContext); 2091 return retVal; 2092 } 2093 2094 @Bean(name="myInvoiceRpR4") 2095 @Lazy 2096 public ca.uhn.fhir.jpa.rp.r4.InvoiceResourceProvider rpInvoiceR4() { 2097 ca.uhn.fhir.jpa.rp.r4.InvoiceResourceProvider retVal; 2098 retVal = new ca.uhn.fhir.jpa.rp.r4.InvoiceResourceProvider(); 2099 retVal.setContext(myFhirContext); 2100 retVal.setDao(daoInvoiceR4()); 2101 return retVal; 2102 } 2103 2104 @Bean(name="myLibraryDaoR4") 2105 public 2106 IFhirResourceDao<org.hl7.fhir.r4.model.Library> 2107 daoLibraryR4() { 2108 2109 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Library> retVal; 2110 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Library>(); 2111 retVal.setResourceType(org.hl7.fhir.r4.model.Library.class); 2112 retVal.setContext(myFhirContext); 2113 return retVal; 2114 } 2115 2116 @Bean(name="myLibraryRpR4") 2117 @Lazy 2118 public ca.uhn.fhir.jpa.rp.r4.LibraryResourceProvider rpLibraryR4() { 2119 ca.uhn.fhir.jpa.rp.r4.LibraryResourceProvider retVal; 2120 retVal = new ca.uhn.fhir.jpa.rp.r4.LibraryResourceProvider(); 2121 retVal.setContext(myFhirContext); 2122 retVal.setDao(daoLibraryR4()); 2123 return retVal; 2124 } 2125 2126 @Bean(name="myLinkageDaoR4") 2127 public 2128 IFhirResourceDao<org.hl7.fhir.r4.model.Linkage> 2129 daoLinkageR4() { 2130 2131 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Linkage> retVal; 2132 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Linkage>(); 2133 retVal.setResourceType(org.hl7.fhir.r4.model.Linkage.class); 2134 retVal.setContext(myFhirContext); 2135 return retVal; 2136 } 2137 2138 @Bean(name="myLinkageRpR4") 2139 @Lazy 2140 public ca.uhn.fhir.jpa.rp.r4.LinkageResourceProvider rpLinkageR4() { 2141 ca.uhn.fhir.jpa.rp.r4.LinkageResourceProvider retVal; 2142 retVal = new ca.uhn.fhir.jpa.rp.r4.LinkageResourceProvider(); 2143 retVal.setContext(myFhirContext); 2144 retVal.setDao(daoLinkageR4()); 2145 return retVal; 2146 } 2147 2148 @Bean(name="myListDaoR4") 2149 public 2150 IFhirResourceDao<org.hl7.fhir.r4.model.ListResource> 2151 daoListResourceR4() { 2152 2153 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ListResource> retVal; 2154 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ListResource>(); 2155 retVal.setResourceType(org.hl7.fhir.r4.model.ListResource.class); 2156 retVal.setContext(myFhirContext); 2157 return retVal; 2158 } 2159 2160 @Bean(name="myListResourceRpR4") 2161 @Lazy 2162 public ca.uhn.fhir.jpa.rp.r4.ListResourceResourceProvider rpListResourceR4() { 2163 ca.uhn.fhir.jpa.rp.r4.ListResourceResourceProvider retVal; 2164 retVal = new ca.uhn.fhir.jpa.rp.r4.ListResourceResourceProvider(); 2165 retVal.setContext(myFhirContext); 2166 retVal.setDao(daoListResourceR4()); 2167 return retVal; 2168 } 2169 2170 @Bean(name="myLocationDaoR4") 2171 public 2172 IFhirResourceDao<org.hl7.fhir.r4.model.Location> 2173 daoLocationR4() { 2174 2175 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Location> retVal; 2176 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Location>(); 2177 retVal.setResourceType(org.hl7.fhir.r4.model.Location.class); 2178 retVal.setContext(myFhirContext); 2179 return retVal; 2180 } 2181 2182 @Bean(name="myLocationRpR4") 2183 @Lazy 2184 public ca.uhn.fhir.jpa.rp.r4.LocationResourceProvider rpLocationR4() { 2185 ca.uhn.fhir.jpa.rp.r4.LocationResourceProvider retVal; 2186 retVal = new ca.uhn.fhir.jpa.rp.r4.LocationResourceProvider(); 2187 retVal.setContext(myFhirContext); 2188 retVal.setDao(daoLocationR4()); 2189 return retVal; 2190 } 2191 2192 @Bean(name="myMeasureDaoR4") 2193 public 2194 IFhirResourceDao<org.hl7.fhir.r4.model.Measure> 2195 daoMeasureR4() { 2196 2197 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Measure> retVal; 2198 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Measure>(); 2199 retVal.setResourceType(org.hl7.fhir.r4.model.Measure.class); 2200 retVal.setContext(myFhirContext); 2201 return retVal; 2202 } 2203 2204 @Bean(name="myMeasureRpR4") 2205 @Lazy 2206 public ca.uhn.fhir.jpa.rp.r4.MeasureResourceProvider rpMeasureR4() { 2207 ca.uhn.fhir.jpa.rp.r4.MeasureResourceProvider retVal; 2208 retVal = new ca.uhn.fhir.jpa.rp.r4.MeasureResourceProvider(); 2209 retVal.setContext(myFhirContext); 2210 retVal.setDao(daoMeasureR4()); 2211 return retVal; 2212 } 2213 2214 @Bean(name="myMeasureReportDaoR4") 2215 public 2216 IFhirResourceDao<org.hl7.fhir.r4.model.MeasureReport> 2217 daoMeasureReportR4() { 2218 2219 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MeasureReport> retVal; 2220 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MeasureReport>(); 2221 retVal.setResourceType(org.hl7.fhir.r4.model.MeasureReport.class); 2222 retVal.setContext(myFhirContext); 2223 return retVal; 2224 } 2225 2226 @Bean(name="myMeasureReportRpR4") 2227 @Lazy 2228 public ca.uhn.fhir.jpa.rp.r4.MeasureReportResourceProvider rpMeasureReportR4() { 2229 ca.uhn.fhir.jpa.rp.r4.MeasureReportResourceProvider retVal; 2230 retVal = new ca.uhn.fhir.jpa.rp.r4.MeasureReportResourceProvider(); 2231 retVal.setContext(myFhirContext); 2232 retVal.setDao(daoMeasureReportR4()); 2233 return retVal; 2234 } 2235 2236 @Bean(name="myMediaDaoR4") 2237 public 2238 IFhirResourceDao<org.hl7.fhir.r4.model.Media> 2239 daoMediaR4() { 2240 2241 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Media> retVal; 2242 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Media>(); 2243 retVal.setResourceType(org.hl7.fhir.r4.model.Media.class); 2244 retVal.setContext(myFhirContext); 2245 return retVal; 2246 } 2247 2248 @Bean(name="myMediaRpR4") 2249 @Lazy 2250 public ca.uhn.fhir.jpa.rp.r4.MediaResourceProvider rpMediaR4() { 2251 ca.uhn.fhir.jpa.rp.r4.MediaResourceProvider retVal; 2252 retVal = new ca.uhn.fhir.jpa.rp.r4.MediaResourceProvider(); 2253 retVal.setContext(myFhirContext); 2254 retVal.setDao(daoMediaR4()); 2255 return retVal; 2256 } 2257 2258 @Bean(name="myMedicationDaoR4") 2259 public 2260 IFhirResourceDao<org.hl7.fhir.r4.model.Medication> 2261 daoMedicationR4() { 2262 2263 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Medication> retVal; 2264 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Medication>(); 2265 retVal.setResourceType(org.hl7.fhir.r4.model.Medication.class); 2266 retVal.setContext(myFhirContext); 2267 return retVal; 2268 } 2269 2270 @Bean(name="myMedicationRpR4") 2271 @Lazy 2272 public ca.uhn.fhir.jpa.rp.r4.MedicationResourceProvider rpMedicationR4() { 2273 ca.uhn.fhir.jpa.rp.r4.MedicationResourceProvider retVal; 2274 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicationResourceProvider(); 2275 retVal.setContext(myFhirContext); 2276 retVal.setDao(daoMedicationR4()); 2277 return retVal; 2278 } 2279 2280 @Bean(name="myMedicationAdministrationDaoR4") 2281 public 2282 IFhirResourceDao<org.hl7.fhir.r4.model.MedicationAdministration> 2283 daoMedicationAdministrationR4() { 2284 2285 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicationAdministration> retVal; 2286 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicationAdministration>(); 2287 retVal.setResourceType(org.hl7.fhir.r4.model.MedicationAdministration.class); 2288 retVal.setContext(myFhirContext); 2289 return retVal; 2290 } 2291 2292 @Bean(name="myMedicationAdministrationRpR4") 2293 @Lazy 2294 public ca.uhn.fhir.jpa.rp.r4.MedicationAdministrationResourceProvider rpMedicationAdministrationR4() { 2295 ca.uhn.fhir.jpa.rp.r4.MedicationAdministrationResourceProvider retVal; 2296 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicationAdministrationResourceProvider(); 2297 retVal.setContext(myFhirContext); 2298 retVal.setDao(daoMedicationAdministrationR4()); 2299 return retVal; 2300 } 2301 2302 @Bean(name="myMedicationDispenseDaoR4") 2303 public 2304 IFhirResourceDao<org.hl7.fhir.r4.model.MedicationDispense> 2305 daoMedicationDispenseR4() { 2306 2307 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicationDispense> retVal; 2308 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicationDispense>(); 2309 retVal.setResourceType(org.hl7.fhir.r4.model.MedicationDispense.class); 2310 retVal.setContext(myFhirContext); 2311 return retVal; 2312 } 2313 2314 @Bean(name="myMedicationDispenseRpR4") 2315 @Lazy 2316 public ca.uhn.fhir.jpa.rp.r4.MedicationDispenseResourceProvider rpMedicationDispenseR4() { 2317 ca.uhn.fhir.jpa.rp.r4.MedicationDispenseResourceProvider retVal; 2318 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicationDispenseResourceProvider(); 2319 retVal.setContext(myFhirContext); 2320 retVal.setDao(daoMedicationDispenseR4()); 2321 return retVal; 2322 } 2323 2324 @Bean(name="myMedicationKnowledgeDaoR4") 2325 public 2326 IFhirResourceDao<org.hl7.fhir.r4.model.MedicationKnowledge> 2327 daoMedicationKnowledgeR4() { 2328 2329 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicationKnowledge> retVal; 2330 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicationKnowledge>(); 2331 retVal.setResourceType(org.hl7.fhir.r4.model.MedicationKnowledge.class); 2332 retVal.setContext(myFhirContext); 2333 return retVal; 2334 } 2335 2336 @Bean(name="myMedicationKnowledgeRpR4") 2337 @Lazy 2338 public ca.uhn.fhir.jpa.rp.r4.MedicationKnowledgeResourceProvider rpMedicationKnowledgeR4() { 2339 ca.uhn.fhir.jpa.rp.r4.MedicationKnowledgeResourceProvider retVal; 2340 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicationKnowledgeResourceProvider(); 2341 retVal.setContext(myFhirContext); 2342 retVal.setDao(daoMedicationKnowledgeR4()); 2343 return retVal; 2344 } 2345 2346 @Bean(name="myMedicationRequestDaoR4") 2347 public 2348 IFhirResourceDao<org.hl7.fhir.r4.model.MedicationRequest> 2349 daoMedicationRequestR4() { 2350 2351 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicationRequest> retVal; 2352 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicationRequest>(); 2353 retVal.setResourceType(org.hl7.fhir.r4.model.MedicationRequest.class); 2354 retVal.setContext(myFhirContext); 2355 return retVal; 2356 } 2357 2358 @Bean(name="myMedicationRequestRpR4") 2359 @Lazy 2360 public ca.uhn.fhir.jpa.rp.r4.MedicationRequestResourceProvider rpMedicationRequestR4() { 2361 ca.uhn.fhir.jpa.rp.r4.MedicationRequestResourceProvider retVal; 2362 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicationRequestResourceProvider(); 2363 retVal.setContext(myFhirContext); 2364 retVal.setDao(daoMedicationRequestR4()); 2365 return retVal; 2366 } 2367 2368 @Bean(name="myMedicationStatementDaoR4") 2369 public 2370 IFhirResourceDao<org.hl7.fhir.r4.model.MedicationStatement> 2371 daoMedicationStatementR4() { 2372 2373 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicationStatement> retVal; 2374 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicationStatement>(); 2375 retVal.setResourceType(org.hl7.fhir.r4.model.MedicationStatement.class); 2376 retVal.setContext(myFhirContext); 2377 return retVal; 2378 } 2379 2380 @Bean(name="myMedicationStatementRpR4") 2381 @Lazy 2382 public ca.uhn.fhir.jpa.rp.r4.MedicationStatementResourceProvider rpMedicationStatementR4() { 2383 ca.uhn.fhir.jpa.rp.r4.MedicationStatementResourceProvider retVal; 2384 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicationStatementResourceProvider(); 2385 retVal.setContext(myFhirContext); 2386 retVal.setDao(daoMedicationStatementR4()); 2387 return retVal; 2388 } 2389 2390 @Bean(name="myMedicinalProductDaoR4") 2391 public 2392 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProduct> 2393 daoMedicinalProductR4() { 2394 2395 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProduct> retVal; 2396 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProduct>(); 2397 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProduct.class); 2398 retVal.setContext(myFhirContext); 2399 return retVal; 2400 } 2401 2402 @Bean(name="myMedicinalProductRpR4") 2403 @Lazy 2404 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductResourceProvider rpMedicinalProductR4() { 2405 ca.uhn.fhir.jpa.rp.r4.MedicinalProductResourceProvider retVal; 2406 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductResourceProvider(); 2407 retVal.setContext(myFhirContext); 2408 retVal.setDao(daoMedicinalProductR4()); 2409 return retVal; 2410 } 2411 2412 @Bean(name="myMedicinalProductAuthorizationDaoR4") 2413 public 2414 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductAuthorization> 2415 daoMedicinalProductAuthorizationR4() { 2416 2417 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductAuthorization> retVal; 2418 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductAuthorization>(); 2419 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductAuthorization.class); 2420 retVal.setContext(myFhirContext); 2421 return retVal; 2422 } 2423 2424 @Bean(name="myMedicinalProductAuthorizationRpR4") 2425 @Lazy 2426 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductAuthorizationResourceProvider rpMedicinalProductAuthorizationR4() { 2427 ca.uhn.fhir.jpa.rp.r4.MedicinalProductAuthorizationResourceProvider retVal; 2428 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductAuthorizationResourceProvider(); 2429 retVal.setContext(myFhirContext); 2430 retVal.setDao(daoMedicinalProductAuthorizationR4()); 2431 return retVal; 2432 } 2433 2434 @Bean(name="myMedicinalProductContraindicationDaoR4") 2435 public 2436 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductContraindication> 2437 daoMedicinalProductContraindicationR4() { 2438 2439 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductContraindication> retVal; 2440 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductContraindication>(); 2441 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductContraindication.class); 2442 retVal.setContext(myFhirContext); 2443 return retVal; 2444 } 2445 2446 @Bean(name="myMedicinalProductContraindicationRpR4") 2447 @Lazy 2448 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductContraindicationResourceProvider rpMedicinalProductContraindicationR4() { 2449 ca.uhn.fhir.jpa.rp.r4.MedicinalProductContraindicationResourceProvider retVal; 2450 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductContraindicationResourceProvider(); 2451 retVal.setContext(myFhirContext); 2452 retVal.setDao(daoMedicinalProductContraindicationR4()); 2453 return retVal; 2454 } 2455 2456 @Bean(name="myMedicinalProductIndicationDaoR4") 2457 public 2458 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductIndication> 2459 daoMedicinalProductIndicationR4() { 2460 2461 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductIndication> retVal; 2462 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductIndication>(); 2463 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductIndication.class); 2464 retVal.setContext(myFhirContext); 2465 return retVal; 2466 } 2467 2468 @Bean(name="myMedicinalProductIndicationRpR4") 2469 @Lazy 2470 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductIndicationResourceProvider rpMedicinalProductIndicationR4() { 2471 ca.uhn.fhir.jpa.rp.r4.MedicinalProductIndicationResourceProvider retVal; 2472 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductIndicationResourceProvider(); 2473 retVal.setContext(myFhirContext); 2474 retVal.setDao(daoMedicinalProductIndicationR4()); 2475 return retVal; 2476 } 2477 2478 @Bean(name="myMedicinalProductIngredientDaoR4") 2479 public 2480 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductIngredient> 2481 daoMedicinalProductIngredientR4() { 2482 2483 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductIngredient> retVal; 2484 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductIngredient>(); 2485 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductIngredient.class); 2486 retVal.setContext(myFhirContext); 2487 return retVal; 2488 } 2489 2490 @Bean(name="myMedicinalProductIngredientRpR4") 2491 @Lazy 2492 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductIngredientResourceProvider rpMedicinalProductIngredientR4() { 2493 ca.uhn.fhir.jpa.rp.r4.MedicinalProductIngredientResourceProvider retVal; 2494 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductIngredientResourceProvider(); 2495 retVal.setContext(myFhirContext); 2496 retVal.setDao(daoMedicinalProductIngredientR4()); 2497 return retVal; 2498 } 2499 2500 @Bean(name="myMedicinalProductInteractionDaoR4") 2501 public 2502 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductInteraction> 2503 daoMedicinalProductInteractionR4() { 2504 2505 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductInteraction> retVal; 2506 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductInteraction>(); 2507 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductInteraction.class); 2508 retVal.setContext(myFhirContext); 2509 return retVal; 2510 } 2511 2512 @Bean(name="myMedicinalProductInteractionRpR4") 2513 @Lazy 2514 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductInteractionResourceProvider rpMedicinalProductInteractionR4() { 2515 ca.uhn.fhir.jpa.rp.r4.MedicinalProductInteractionResourceProvider retVal; 2516 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductInteractionResourceProvider(); 2517 retVal.setContext(myFhirContext); 2518 retVal.setDao(daoMedicinalProductInteractionR4()); 2519 return retVal; 2520 } 2521 2522 @Bean(name="myMedicinalProductManufacturedDaoR4") 2523 public 2524 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductManufactured> 2525 daoMedicinalProductManufacturedR4() { 2526 2527 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductManufactured> retVal; 2528 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductManufactured>(); 2529 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductManufactured.class); 2530 retVal.setContext(myFhirContext); 2531 return retVal; 2532 } 2533 2534 @Bean(name="myMedicinalProductManufacturedRpR4") 2535 @Lazy 2536 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductManufacturedResourceProvider rpMedicinalProductManufacturedR4() { 2537 ca.uhn.fhir.jpa.rp.r4.MedicinalProductManufacturedResourceProvider retVal; 2538 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductManufacturedResourceProvider(); 2539 retVal.setContext(myFhirContext); 2540 retVal.setDao(daoMedicinalProductManufacturedR4()); 2541 return retVal; 2542 } 2543 2544 @Bean(name="myMedicinalProductPackagedDaoR4") 2545 public 2546 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductPackaged> 2547 daoMedicinalProductPackagedR4() { 2548 2549 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductPackaged> retVal; 2550 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductPackaged>(); 2551 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductPackaged.class); 2552 retVal.setContext(myFhirContext); 2553 return retVal; 2554 } 2555 2556 @Bean(name="myMedicinalProductPackagedRpR4") 2557 @Lazy 2558 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductPackagedResourceProvider rpMedicinalProductPackagedR4() { 2559 ca.uhn.fhir.jpa.rp.r4.MedicinalProductPackagedResourceProvider retVal; 2560 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductPackagedResourceProvider(); 2561 retVal.setContext(myFhirContext); 2562 retVal.setDao(daoMedicinalProductPackagedR4()); 2563 return retVal; 2564 } 2565 2566 @Bean(name="myMedicinalProductPharmaceuticalDaoR4") 2567 public 2568 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductPharmaceutical> 2569 daoMedicinalProductPharmaceuticalR4() { 2570 2571 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductPharmaceutical> retVal; 2572 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductPharmaceutical>(); 2573 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductPharmaceutical.class); 2574 retVal.setContext(myFhirContext); 2575 return retVal; 2576 } 2577 2578 @Bean(name="myMedicinalProductPharmaceuticalRpR4") 2579 @Lazy 2580 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductPharmaceuticalResourceProvider rpMedicinalProductPharmaceuticalR4() { 2581 ca.uhn.fhir.jpa.rp.r4.MedicinalProductPharmaceuticalResourceProvider retVal; 2582 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductPharmaceuticalResourceProvider(); 2583 retVal.setContext(myFhirContext); 2584 retVal.setDao(daoMedicinalProductPharmaceuticalR4()); 2585 return retVal; 2586 } 2587 2588 @Bean(name="myMedicinalProductUndesirableEffectDaoR4") 2589 public 2590 IFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductUndesirableEffect> 2591 daoMedicinalProductUndesirableEffectR4() { 2592 2593 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MedicinalProductUndesirableEffect> retVal; 2594 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MedicinalProductUndesirableEffect>(); 2595 retVal.setResourceType(org.hl7.fhir.r4.model.MedicinalProductUndesirableEffect.class); 2596 retVal.setContext(myFhirContext); 2597 return retVal; 2598 } 2599 2600 @Bean(name="myMedicinalProductUndesirableEffectRpR4") 2601 @Lazy 2602 public ca.uhn.fhir.jpa.rp.r4.MedicinalProductUndesirableEffectResourceProvider rpMedicinalProductUndesirableEffectR4() { 2603 ca.uhn.fhir.jpa.rp.r4.MedicinalProductUndesirableEffectResourceProvider retVal; 2604 retVal = new ca.uhn.fhir.jpa.rp.r4.MedicinalProductUndesirableEffectResourceProvider(); 2605 retVal.setContext(myFhirContext); 2606 retVal.setDao(daoMedicinalProductUndesirableEffectR4()); 2607 return retVal; 2608 } 2609 2610 @Bean(name="myMessageDefinitionDaoR4") 2611 public 2612 IFhirResourceDao<org.hl7.fhir.r4.model.MessageDefinition> 2613 daoMessageDefinitionR4() { 2614 2615 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MessageDefinition> retVal; 2616 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MessageDefinition>(); 2617 retVal.setResourceType(org.hl7.fhir.r4.model.MessageDefinition.class); 2618 retVal.setContext(myFhirContext); 2619 return retVal; 2620 } 2621 2622 @Bean(name="myMessageDefinitionRpR4") 2623 @Lazy 2624 public ca.uhn.fhir.jpa.rp.r4.MessageDefinitionResourceProvider rpMessageDefinitionR4() { 2625 ca.uhn.fhir.jpa.rp.r4.MessageDefinitionResourceProvider retVal; 2626 retVal = new ca.uhn.fhir.jpa.rp.r4.MessageDefinitionResourceProvider(); 2627 retVal.setContext(myFhirContext); 2628 retVal.setDao(daoMessageDefinitionR4()); 2629 return retVal; 2630 } 2631 2632 @Bean(name="myMessageHeaderDaoR4") 2633 public 2634 IFhirResourceDao<org.hl7.fhir.r4.model.MessageHeader> 2635 daoMessageHeaderR4() { 2636 2637 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MessageHeader> retVal; 2638 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MessageHeader>(); 2639 retVal.setResourceType(org.hl7.fhir.r4.model.MessageHeader.class); 2640 retVal.setContext(myFhirContext); 2641 return retVal; 2642 } 2643 2644 @Bean(name="myMessageHeaderRpR4") 2645 @Lazy 2646 public ca.uhn.fhir.jpa.rp.r4.MessageHeaderResourceProvider rpMessageHeaderR4() { 2647 ca.uhn.fhir.jpa.rp.r4.MessageHeaderResourceProvider retVal; 2648 retVal = new ca.uhn.fhir.jpa.rp.r4.MessageHeaderResourceProvider(); 2649 retVal.setContext(myFhirContext); 2650 retVal.setDao(daoMessageHeaderR4()); 2651 return retVal; 2652 } 2653 2654 @Bean(name="myMolecularSequenceDaoR4") 2655 public 2656 IFhirResourceDao<org.hl7.fhir.r4.model.MolecularSequence> 2657 daoMolecularSequenceR4() { 2658 2659 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.MolecularSequence> retVal; 2660 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.MolecularSequence>(); 2661 retVal.setResourceType(org.hl7.fhir.r4.model.MolecularSequence.class); 2662 retVal.setContext(myFhirContext); 2663 return retVal; 2664 } 2665 2666 @Bean(name="myMolecularSequenceRpR4") 2667 @Lazy 2668 public ca.uhn.fhir.jpa.rp.r4.MolecularSequenceResourceProvider rpMolecularSequenceR4() { 2669 ca.uhn.fhir.jpa.rp.r4.MolecularSequenceResourceProvider retVal; 2670 retVal = new ca.uhn.fhir.jpa.rp.r4.MolecularSequenceResourceProvider(); 2671 retVal.setContext(myFhirContext); 2672 retVal.setDao(daoMolecularSequenceR4()); 2673 return retVal; 2674 } 2675 2676 @Bean(name="myNamingSystemDaoR4") 2677 public 2678 IFhirResourceDao<org.hl7.fhir.r4.model.NamingSystem> 2679 daoNamingSystemR4() { 2680 2681 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.NamingSystem> retVal; 2682 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.NamingSystem>(); 2683 retVal.setResourceType(org.hl7.fhir.r4.model.NamingSystem.class); 2684 retVal.setContext(myFhirContext); 2685 return retVal; 2686 } 2687 2688 @Bean(name="myNamingSystemRpR4") 2689 @Lazy 2690 public ca.uhn.fhir.jpa.rp.r4.NamingSystemResourceProvider rpNamingSystemR4() { 2691 ca.uhn.fhir.jpa.rp.r4.NamingSystemResourceProvider retVal; 2692 retVal = new ca.uhn.fhir.jpa.rp.r4.NamingSystemResourceProvider(); 2693 retVal.setContext(myFhirContext); 2694 retVal.setDao(daoNamingSystemR4()); 2695 return retVal; 2696 } 2697 2698 @Bean(name="myNutritionOrderDaoR4") 2699 public 2700 IFhirResourceDao<org.hl7.fhir.r4.model.NutritionOrder> 2701 daoNutritionOrderR4() { 2702 2703 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.NutritionOrder> retVal; 2704 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.NutritionOrder>(); 2705 retVal.setResourceType(org.hl7.fhir.r4.model.NutritionOrder.class); 2706 retVal.setContext(myFhirContext); 2707 return retVal; 2708 } 2709 2710 @Bean(name="myNutritionOrderRpR4") 2711 @Lazy 2712 public ca.uhn.fhir.jpa.rp.r4.NutritionOrderResourceProvider rpNutritionOrderR4() { 2713 ca.uhn.fhir.jpa.rp.r4.NutritionOrderResourceProvider retVal; 2714 retVal = new ca.uhn.fhir.jpa.rp.r4.NutritionOrderResourceProvider(); 2715 retVal.setContext(myFhirContext); 2716 retVal.setDao(daoNutritionOrderR4()); 2717 return retVal; 2718 } 2719 2720 @Bean(name="myObservationDaoR4") 2721 public 2722 IFhirResourceDaoObservation<org.hl7.fhir.r4.model.Observation> 2723 daoObservationR4() { 2724 2725 ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<org.hl7.fhir.r4.model.Observation> retVal; 2726 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<>(); 2727 retVal.setResourceType(org.hl7.fhir.r4.model.Observation.class); 2728 retVal.setContext(myFhirContext); 2729 return retVal; 2730 } 2731 2732 @Bean(name="myObservationRpR4") 2733 @Lazy 2734 public ca.uhn.fhir.jpa.rp.r4.ObservationResourceProvider rpObservationR4() { 2735 ca.uhn.fhir.jpa.rp.r4.ObservationResourceProvider retVal; 2736 retVal = new ca.uhn.fhir.jpa.rp.r4.ObservationResourceProvider(); 2737 retVal.setContext(myFhirContext); 2738 retVal.setDao(daoObservationR4()); 2739 return retVal; 2740 } 2741 2742 @Bean(name="myObservationDefinitionDaoR4") 2743 public 2744 IFhirResourceDao<org.hl7.fhir.r4.model.ObservationDefinition> 2745 daoObservationDefinitionR4() { 2746 2747 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ObservationDefinition> retVal; 2748 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ObservationDefinition>(); 2749 retVal.setResourceType(org.hl7.fhir.r4.model.ObservationDefinition.class); 2750 retVal.setContext(myFhirContext); 2751 return retVal; 2752 } 2753 2754 @Bean(name="myObservationDefinitionRpR4") 2755 @Lazy 2756 public ca.uhn.fhir.jpa.rp.r4.ObservationDefinitionResourceProvider rpObservationDefinitionR4() { 2757 ca.uhn.fhir.jpa.rp.r4.ObservationDefinitionResourceProvider retVal; 2758 retVal = new ca.uhn.fhir.jpa.rp.r4.ObservationDefinitionResourceProvider(); 2759 retVal.setContext(myFhirContext); 2760 retVal.setDao(daoObservationDefinitionR4()); 2761 return retVal; 2762 } 2763 2764 @Bean(name="myOperationDefinitionDaoR4") 2765 public 2766 IFhirResourceDao<org.hl7.fhir.r4.model.OperationDefinition> 2767 daoOperationDefinitionR4() { 2768 2769 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.OperationDefinition> retVal; 2770 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.OperationDefinition>(); 2771 retVal.setResourceType(org.hl7.fhir.r4.model.OperationDefinition.class); 2772 retVal.setContext(myFhirContext); 2773 return retVal; 2774 } 2775 2776 @Bean(name="myOperationDefinitionRpR4") 2777 @Lazy 2778 public ca.uhn.fhir.jpa.rp.r4.OperationDefinitionResourceProvider rpOperationDefinitionR4() { 2779 ca.uhn.fhir.jpa.rp.r4.OperationDefinitionResourceProvider retVal; 2780 retVal = new ca.uhn.fhir.jpa.rp.r4.OperationDefinitionResourceProvider(); 2781 retVal.setContext(myFhirContext); 2782 retVal.setDao(daoOperationDefinitionR4()); 2783 return retVal; 2784 } 2785 2786 @Bean(name="myOperationOutcomeDaoR4") 2787 public 2788 IFhirResourceDao<org.hl7.fhir.r4.model.OperationOutcome> 2789 daoOperationOutcomeR4() { 2790 2791 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.OperationOutcome> retVal; 2792 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.OperationOutcome>(); 2793 retVal.setResourceType(org.hl7.fhir.r4.model.OperationOutcome.class); 2794 retVal.setContext(myFhirContext); 2795 return retVal; 2796 } 2797 2798 @Bean(name="myOperationOutcomeRpR4") 2799 @Lazy 2800 public ca.uhn.fhir.jpa.rp.r4.OperationOutcomeResourceProvider rpOperationOutcomeR4() { 2801 ca.uhn.fhir.jpa.rp.r4.OperationOutcomeResourceProvider retVal; 2802 retVal = new ca.uhn.fhir.jpa.rp.r4.OperationOutcomeResourceProvider(); 2803 retVal.setContext(myFhirContext); 2804 retVal.setDao(daoOperationOutcomeR4()); 2805 return retVal; 2806 } 2807 2808 @Bean(name="myOrganizationDaoR4") 2809 public 2810 IFhirResourceDao<org.hl7.fhir.r4.model.Organization> 2811 daoOrganizationR4() { 2812 2813 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Organization> retVal; 2814 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Organization>(); 2815 retVal.setResourceType(org.hl7.fhir.r4.model.Organization.class); 2816 retVal.setContext(myFhirContext); 2817 return retVal; 2818 } 2819 2820 @Bean(name="myOrganizationRpR4") 2821 @Lazy 2822 public ca.uhn.fhir.jpa.rp.r4.OrganizationResourceProvider rpOrganizationR4() { 2823 ca.uhn.fhir.jpa.rp.r4.OrganizationResourceProvider retVal; 2824 retVal = new ca.uhn.fhir.jpa.rp.r4.OrganizationResourceProvider(); 2825 retVal.setContext(myFhirContext); 2826 retVal.setDao(daoOrganizationR4()); 2827 return retVal; 2828 } 2829 2830 @Bean(name="myOrganizationAffiliationDaoR4") 2831 public 2832 IFhirResourceDao<org.hl7.fhir.r4.model.OrganizationAffiliation> 2833 daoOrganizationAffiliationR4() { 2834 2835 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.OrganizationAffiliation> retVal; 2836 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.OrganizationAffiliation>(); 2837 retVal.setResourceType(org.hl7.fhir.r4.model.OrganizationAffiliation.class); 2838 retVal.setContext(myFhirContext); 2839 return retVal; 2840 } 2841 2842 @Bean(name="myOrganizationAffiliationRpR4") 2843 @Lazy 2844 public ca.uhn.fhir.jpa.rp.r4.OrganizationAffiliationResourceProvider rpOrganizationAffiliationR4() { 2845 ca.uhn.fhir.jpa.rp.r4.OrganizationAffiliationResourceProvider retVal; 2846 retVal = new ca.uhn.fhir.jpa.rp.r4.OrganizationAffiliationResourceProvider(); 2847 retVal.setContext(myFhirContext); 2848 retVal.setDao(daoOrganizationAffiliationR4()); 2849 return retVal; 2850 } 2851 2852 @Bean(name="myParametersDaoR4") 2853 public 2854 IFhirResourceDao<org.hl7.fhir.r4.model.Parameters> 2855 daoParametersR4() { 2856 2857 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Parameters> retVal; 2858 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Parameters>(); 2859 retVal.setResourceType(org.hl7.fhir.r4.model.Parameters.class); 2860 retVal.setContext(myFhirContext); 2861 return retVal; 2862 } 2863 2864 @Bean(name="myParametersRpR4") 2865 @Lazy 2866 public ca.uhn.fhir.jpa.rp.r4.ParametersResourceProvider rpParametersR4() { 2867 ca.uhn.fhir.jpa.rp.r4.ParametersResourceProvider retVal; 2868 retVal = new ca.uhn.fhir.jpa.rp.r4.ParametersResourceProvider(); 2869 retVal.setContext(myFhirContext); 2870 retVal.setDao(daoParametersR4()); 2871 return retVal; 2872 } 2873 2874 @Bean(name="myPatientDaoR4") 2875 public 2876 IFhirResourceDaoPatient<org.hl7.fhir.r4.model.Patient> 2877 daoPatientR4() { 2878 2879 ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<org.hl7.fhir.r4.model.Patient> retVal; 2880 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<>(); 2881 retVal.setResourceType(org.hl7.fhir.r4.model.Patient.class); 2882 retVal.setContext(myFhirContext); 2883 return retVal; 2884 } 2885 2886 @Bean(name="myPatientRpR4") 2887 @Lazy 2888 public ca.uhn.fhir.jpa.rp.r4.PatientResourceProvider rpPatientR4() { 2889 ca.uhn.fhir.jpa.rp.r4.PatientResourceProvider retVal; 2890 retVal = new ca.uhn.fhir.jpa.rp.r4.PatientResourceProvider(); 2891 retVal.setContext(myFhirContext); 2892 retVal.setDao(daoPatientR4()); 2893 return retVal; 2894 } 2895 2896 @Bean(name="myPaymentNoticeDaoR4") 2897 public 2898 IFhirResourceDao<org.hl7.fhir.r4.model.PaymentNotice> 2899 daoPaymentNoticeR4() { 2900 2901 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.PaymentNotice> retVal; 2902 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.PaymentNotice>(); 2903 retVal.setResourceType(org.hl7.fhir.r4.model.PaymentNotice.class); 2904 retVal.setContext(myFhirContext); 2905 return retVal; 2906 } 2907 2908 @Bean(name="myPaymentNoticeRpR4") 2909 @Lazy 2910 public ca.uhn.fhir.jpa.rp.r4.PaymentNoticeResourceProvider rpPaymentNoticeR4() { 2911 ca.uhn.fhir.jpa.rp.r4.PaymentNoticeResourceProvider retVal; 2912 retVal = new ca.uhn.fhir.jpa.rp.r4.PaymentNoticeResourceProvider(); 2913 retVal.setContext(myFhirContext); 2914 retVal.setDao(daoPaymentNoticeR4()); 2915 return retVal; 2916 } 2917 2918 @Bean(name="myPaymentReconciliationDaoR4") 2919 public 2920 IFhirResourceDao<org.hl7.fhir.r4.model.PaymentReconciliation> 2921 daoPaymentReconciliationR4() { 2922 2923 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.PaymentReconciliation> retVal; 2924 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.PaymentReconciliation>(); 2925 retVal.setResourceType(org.hl7.fhir.r4.model.PaymentReconciliation.class); 2926 retVal.setContext(myFhirContext); 2927 return retVal; 2928 } 2929 2930 @Bean(name="myPaymentReconciliationRpR4") 2931 @Lazy 2932 public ca.uhn.fhir.jpa.rp.r4.PaymentReconciliationResourceProvider rpPaymentReconciliationR4() { 2933 ca.uhn.fhir.jpa.rp.r4.PaymentReconciliationResourceProvider retVal; 2934 retVal = new ca.uhn.fhir.jpa.rp.r4.PaymentReconciliationResourceProvider(); 2935 retVal.setContext(myFhirContext); 2936 retVal.setDao(daoPaymentReconciliationR4()); 2937 return retVal; 2938 } 2939 2940 @Bean(name="myPersonDaoR4") 2941 public 2942 IFhirResourceDao<org.hl7.fhir.r4.model.Person> 2943 daoPersonR4() { 2944 2945 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Person> retVal; 2946 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Person>(); 2947 retVal.setResourceType(org.hl7.fhir.r4.model.Person.class); 2948 retVal.setContext(myFhirContext); 2949 return retVal; 2950 } 2951 2952 @Bean(name="myPersonRpR4") 2953 @Lazy 2954 public ca.uhn.fhir.jpa.rp.r4.PersonResourceProvider rpPersonR4() { 2955 ca.uhn.fhir.jpa.rp.r4.PersonResourceProvider retVal; 2956 retVal = new ca.uhn.fhir.jpa.rp.r4.PersonResourceProvider(); 2957 retVal.setContext(myFhirContext); 2958 retVal.setDao(daoPersonR4()); 2959 return retVal; 2960 } 2961 2962 @Bean(name="myPlanDefinitionDaoR4") 2963 public 2964 IFhirResourceDao<org.hl7.fhir.r4.model.PlanDefinition> 2965 daoPlanDefinitionR4() { 2966 2967 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.PlanDefinition> retVal; 2968 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.PlanDefinition>(); 2969 retVal.setResourceType(org.hl7.fhir.r4.model.PlanDefinition.class); 2970 retVal.setContext(myFhirContext); 2971 return retVal; 2972 } 2973 2974 @Bean(name="myPlanDefinitionRpR4") 2975 @Lazy 2976 public ca.uhn.fhir.jpa.rp.r4.PlanDefinitionResourceProvider rpPlanDefinitionR4() { 2977 ca.uhn.fhir.jpa.rp.r4.PlanDefinitionResourceProvider retVal; 2978 retVal = new ca.uhn.fhir.jpa.rp.r4.PlanDefinitionResourceProvider(); 2979 retVal.setContext(myFhirContext); 2980 retVal.setDao(daoPlanDefinitionR4()); 2981 return retVal; 2982 } 2983 2984 @Bean(name="myPractitionerDaoR4") 2985 public 2986 IFhirResourceDao<org.hl7.fhir.r4.model.Practitioner> 2987 daoPractitionerR4() { 2988 2989 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Practitioner> retVal; 2990 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Practitioner>(); 2991 retVal.setResourceType(org.hl7.fhir.r4.model.Practitioner.class); 2992 retVal.setContext(myFhirContext); 2993 return retVal; 2994 } 2995 2996 @Bean(name="myPractitionerRpR4") 2997 @Lazy 2998 public ca.uhn.fhir.jpa.rp.r4.PractitionerResourceProvider rpPractitionerR4() { 2999 ca.uhn.fhir.jpa.rp.r4.PractitionerResourceProvider retVal; 3000 retVal = new ca.uhn.fhir.jpa.rp.r4.PractitionerResourceProvider(); 3001 retVal.setContext(myFhirContext); 3002 retVal.setDao(daoPractitionerR4()); 3003 return retVal; 3004 } 3005 3006 @Bean(name="myPractitionerRoleDaoR4") 3007 public 3008 IFhirResourceDao<org.hl7.fhir.r4.model.PractitionerRole> 3009 daoPractitionerRoleR4() { 3010 3011 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.PractitionerRole> retVal; 3012 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.PractitionerRole>(); 3013 retVal.setResourceType(org.hl7.fhir.r4.model.PractitionerRole.class); 3014 retVal.setContext(myFhirContext); 3015 return retVal; 3016 } 3017 3018 @Bean(name="myPractitionerRoleRpR4") 3019 @Lazy 3020 public ca.uhn.fhir.jpa.rp.r4.PractitionerRoleResourceProvider rpPractitionerRoleR4() { 3021 ca.uhn.fhir.jpa.rp.r4.PractitionerRoleResourceProvider retVal; 3022 retVal = new ca.uhn.fhir.jpa.rp.r4.PractitionerRoleResourceProvider(); 3023 retVal.setContext(myFhirContext); 3024 retVal.setDao(daoPractitionerRoleR4()); 3025 return retVal; 3026 } 3027 3028 @Bean(name="myProcedureDaoR4") 3029 public 3030 IFhirResourceDao<org.hl7.fhir.r4.model.Procedure> 3031 daoProcedureR4() { 3032 3033 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Procedure> retVal; 3034 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Procedure>(); 3035 retVal.setResourceType(org.hl7.fhir.r4.model.Procedure.class); 3036 retVal.setContext(myFhirContext); 3037 return retVal; 3038 } 3039 3040 @Bean(name="myProcedureRpR4") 3041 @Lazy 3042 public ca.uhn.fhir.jpa.rp.r4.ProcedureResourceProvider rpProcedureR4() { 3043 ca.uhn.fhir.jpa.rp.r4.ProcedureResourceProvider retVal; 3044 retVal = new ca.uhn.fhir.jpa.rp.r4.ProcedureResourceProvider(); 3045 retVal.setContext(myFhirContext); 3046 retVal.setDao(daoProcedureR4()); 3047 return retVal; 3048 } 3049 3050 @Bean(name="myProvenanceDaoR4") 3051 public 3052 IFhirResourceDao<org.hl7.fhir.r4.model.Provenance> 3053 daoProvenanceR4() { 3054 3055 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Provenance> retVal; 3056 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Provenance>(); 3057 retVal.setResourceType(org.hl7.fhir.r4.model.Provenance.class); 3058 retVal.setContext(myFhirContext); 3059 return retVal; 3060 } 3061 3062 @Bean(name="myProvenanceRpR4") 3063 @Lazy 3064 public ca.uhn.fhir.jpa.rp.r4.ProvenanceResourceProvider rpProvenanceR4() { 3065 ca.uhn.fhir.jpa.rp.r4.ProvenanceResourceProvider retVal; 3066 retVal = new ca.uhn.fhir.jpa.rp.r4.ProvenanceResourceProvider(); 3067 retVal.setContext(myFhirContext); 3068 retVal.setDao(daoProvenanceR4()); 3069 return retVal; 3070 } 3071 3072 @Bean(name="myQuestionnaireDaoR4") 3073 public 3074 IFhirResourceDao<org.hl7.fhir.r4.model.Questionnaire> 3075 daoQuestionnaireR4() { 3076 3077 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Questionnaire> retVal; 3078 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Questionnaire>(); 3079 retVal.setResourceType(org.hl7.fhir.r4.model.Questionnaire.class); 3080 retVal.setContext(myFhirContext); 3081 return retVal; 3082 } 3083 3084 @Bean(name="myQuestionnaireRpR4") 3085 @Lazy 3086 public ca.uhn.fhir.jpa.rp.r4.QuestionnaireResourceProvider rpQuestionnaireR4() { 3087 ca.uhn.fhir.jpa.rp.r4.QuestionnaireResourceProvider retVal; 3088 retVal = new ca.uhn.fhir.jpa.rp.r4.QuestionnaireResourceProvider(); 3089 retVal.setContext(myFhirContext); 3090 retVal.setDao(daoQuestionnaireR4()); 3091 return retVal; 3092 } 3093 3094 @Bean(name="myQuestionnaireResponseDaoR4") 3095 public 3096 IFhirResourceDao<org.hl7.fhir.r4.model.QuestionnaireResponse> 3097 daoQuestionnaireResponseR4() { 3098 3099 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.QuestionnaireResponse> retVal; 3100 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.QuestionnaireResponse>(); 3101 retVal.setResourceType(org.hl7.fhir.r4.model.QuestionnaireResponse.class); 3102 retVal.setContext(myFhirContext); 3103 return retVal; 3104 } 3105 3106 @Bean(name="myQuestionnaireResponseRpR4") 3107 @Lazy 3108 public ca.uhn.fhir.jpa.rp.r4.QuestionnaireResponseResourceProvider rpQuestionnaireResponseR4() { 3109 ca.uhn.fhir.jpa.rp.r4.QuestionnaireResponseResourceProvider retVal; 3110 retVal = new ca.uhn.fhir.jpa.rp.r4.QuestionnaireResponseResourceProvider(); 3111 retVal.setContext(myFhirContext); 3112 retVal.setDao(daoQuestionnaireResponseR4()); 3113 return retVal; 3114 } 3115 3116 @Bean(name="myRelatedPersonDaoR4") 3117 public 3118 IFhirResourceDao<org.hl7.fhir.r4.model.RelatedPerson> 3119 daoRelatedPersonR4() { 3120 3121 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.RelatedPerson> retVal; 3122 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.RelatedPerson>(); 3123 retVal.setResourceType(org.hl7.fhir.r4.model.RelatedPerson.class); 3124 retVal.setContext(myFhirContext); 3125 return retVal; 3126 } 3127 3128 @Bean(name="myRelatedPersonRpR4") 3129 @Lazy 3130 public ca.uhn.fhir.jpa.rp.r4.RelatedPersonResourceProvider rpRelatedPersonR4() { 3131 ca.uhn.fhir.jpa.rp.r4.RelatedPersonResourceProvider retVal; 3132 retVal = new ca.uhn.fhir.jpa.rp.r4.RelatedPersonResourceProvider(); 3133 retVal.setContext(myFhirContext); 3134 retVal.setDao(daoRelatedPersonR4()); 3135 return retVal; 3136 } 3137 3138 @Bean(name="myRequestGroupDaoR4") 3139 public 3140 IFhirResourceDao<org.hl7.fhir.r4.model.RequestGroup> 3141 daoRequestGroupR4() { 3142 3143 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.RequestGroup> retVal; 3144 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.RequestGroup>(); 3145 retVal.setResourceType(org.hl7.fhir.r4.model.RequestGroup.class); 3146 retVal.setContext(myFhirContext); 3147 return retVal; 3148 } 3149 3150 @Bean(name="myRequestGroupRpR4") 3151 @Lazy 3152 public ca.uhn.fhir.jpa.rp.r4.RequestGroupResourceProvider rpRequestGroupR4() { 3153 ca.uhn.fhir.jpa.rp.r4.RequestGroupResourceProvider retVal; 3154 retVal = new ca.uhn.fhir.jpa.rp.r4.RequestGroupResourceProvider(); 3155 retVal.setContext(myFhirContext); 3156 retVal.setDao(daoRequestGroupR4()); 3157 return retVal; 3158 } 3159 3160 @Bean(name="myResearchDefinitionDaoR4") 3161 public 3162 IFhirResourceDao<org.hl7.fhir.r4.model.ResearchDefinition> 3163 daoResearchDefinitionR4() { 3164 3165 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ResearchDefinition> retVal; 3166 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ResearchDefinition>(); 3167 retVal.setResourceType(org.hl7.fhir.r4.model.ResearchDefinition.class); 3168 retVal.setContext(myFhirContext); 3169 return retVal; 3170 } 3171 3172 @Bean(name="myResearchDefinitionRpR4") 3173 @Lazy 3174 public ca.uhn.fhir.jpa.rp.r4.ResearchDefinitionResourceProvider rpResearchDefinitionR4() { 3175 ca.uhn.fhir.jpa.rp.r4.ResearchDefinitionResourceProvider retVal; 3176 retVal = new ca.uhn.fhir.jpa.rp.r4.ResearchDefinitionResourceProvider(); 3177 retVal.setContext(myFhirContext); 3178 retVal.setDao(daoResearchDefinitionR4()); 3179 return retVal; 3180 } 3181 3182 @Bean(name="myResearchElementDefinitionDaoR4") 3183 public 3184 IFhirResourceDao<org.hl7.fhir.r4.model.ResearchElementDefinition> 3185 daoResearchElementDefinitionR4() { 3186 3187 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ResearchElementDefinition> retVal; 3188 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ResearchElementDefinition>(); 3189 retVal.setResourceType(org.hl7.fhir.r4.model.ResearchElementDefinition.class); 3190 retVal.setContext(myFhirContext); 3191 return retVal; 3192 } 3193 3194 @Bean(name="myResearchElementDefinitionRpR4") 3195 @Lazy 3196 public ca.uhn.fhir.jpa.rp.r4.ResearchElementDefinitionResourceProvider rpResearchElementDefinitionR4() { 3197 ca.uhn.fhir.jpa.rp.r4.ResearchElementDefinitionResourceProvider retVal; 3198 retVal = new ca.uhn.fhir.jpa.rp.r4.ResearchElementDefinitionResourceProvider(); 3199 retVal.setContext(myFhirContext); 3200 retVal.setDao(daoResearchElementDefinitionR4()); 3201 return retVal; 3202 } 3203 3204 @Bean(name="myResearchStudyDaoR4") 3205 public 3206 IFhirResourceDao<org.hl7.fhir.r4.model.ResearchStudy> 3207 daoResearchStudyR4() { 3208 3209 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ResearchStudy> retVal; 3210 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ResearchStudy>(); 3211 retVal.setResourceType(org.hl7.fhir.r4.model.ResearchStudy.class); 3212 retVal.setContext(myFhirContext); 3213 return retVal; 3214 } 3215 3216 @Bean(name="myResearchStudyRpR4") 3217 @Lazy 3218 public ca.uhn.fhir.jpa.rp.r4.ResearchStudyResourceProvider rpResearchStudyR4() { 3219 ca.uhn.fhir.jpa.rp.r4.ResearchStudyResourceProvider retVal; 3220 retVal = new ca.uhn.fhir.jpa.rp.r4.ResearchStudyResourceProvider(); 3221 retVal.setContext(myFhirContext); 3222 retVal.setDao(daoResearchStudyR4()); 3223 return retVal; 3224 } 3225 3226 @Bean(name="myResearchSubjectDaoR4") 3227 public 3228 IFhirResourceDao<org.hl7.fhir.r4.model.ResearchSubject> 3229 daoResearchSubjectR4() { 3230 3231 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ResearchSubject> retVal; 3232 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ResearchSubject>(); 3233 retVal.setResourceType(org.hl7.fhir.r4.model.ResearchSubject.class); 3234 retVal.setContext(myFhirContext); 3235 return retVal; 3236 } 3237 3238 @Bean(name="myResearchSubjectRpR4") 3239 @Lazy 3240 public ca.uhn.fhir.jpa.rp.r4.ResearchSubjectResourceProvider rpResearchSubjectR4() { 3241 ca.uhn.fhir.jpa.rp.r4.ResearchSubjectResourceProvider retVal; 3242 retVal = new ca.uhn.fhir.jpa.rp.r4.ResearchSubjectResourceProvider(); 3243 retVal.setContext(myFhirContext); 3244 retVal.setDao(daoResearchSubjectR4()); 3245 return retVal; 3246 } 3247 3248 @Bean(name="myRiskAssessmentDaoR4") 3249 public 3250 IFhirResourceDao<org.hl7.fhir.r4.model.RiskAssessment> 3251 daoRiskAssessmentR4() { 3252 3253 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.RiskAssessment> retVal; 3254 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.RiskAssessment>(); 3255 retVal.setResourceType(org.hl7.fhir.r4.model.RiskAssessment.class); 3256 retVal.setContext(myFhirContext); 3257 return retVal; 3258 } 3259 3260 @Bean(name="myRiskAssessmentRpR4") 3261 @Lazy 3262 public ca.uhn.fhir.jpa.rp.r4.RiskAssessmentResourceProvider rpRiskAssessmentR4() { 3263 ca.uhn.fhir.jpa.rp.r4.RiskAssessmentResourceProvider retVal; 3264 retVal = new ca.uhn.fhir.jpa.rp.r4.RiskAssessmentResourceProvider(); 3265 retVal.setContext(myFhirContext); 3266 retVal.setDao(daoRiskAssessmentR4()); 3267 return retVal; 3268 } 3269 3270 @Bean(name="myRiskEvidenceSynthesisDaoR4") 3271 public 3272 IFhirResourceDao<org.hl7.fhir.r4.model.RiskEvidenceSynthesis> 3273 daoRiskEvidenceSynthesisR4() { 3274 3275 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.RiskEvidenceSynthesis> retVal; 3276 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.RiskEvidenceSynthesis>(); 3277 retVal.setResourceType(org.hl7.fhir.r4.model.RiskEvidenceSynthesis.class); 3278 retVal.setContext(myFhirContext); 3279 return retVal; 3280 } 3281 3282 @Bean(name="myRiskEvidenceSynthesisRpR4") 3283 @Lazy 3284 public ca.uhn.fhir.jpa.rp.r4.RiskEvidenceSynthesisResourceProvider rpRiskEvidenceSynthesisR4() { 3285 ca.uhn.fhir.jpa.rp.r4.RiskEvidenceSynthesisResourceProvider retVal; 3286 retVal = new ca.uhn.fhir.jpa.rp.r4.RiskEvidenceSynthesisResourceProvider(); 3287 retVal.setContext(myFhirContext); 3288 retVal.setDao(daoRiskEvidenceSynthesisR4()); 3289 return retVal; 3290 } 3291 3292 @Bean(name="myScheduleDaoR4") 3293 public 3294 IFhirResourceDao<org.hl7.fhir.r4.model.Schedule> 3295 daoScheduleR4() { 3296 3297 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Schedule> retVal; 3298 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Schedule>(); 3299 retVal.setResourceType(org.hl7.fhir.r4.model.Schedule.class); 3300 retVal.setContext(myFhirContext); 3301 return retVal; 3302 } 3303 3304 @Bean(name="myScheduleRpR4") 3305 @Lazy 3306 public ca.uhn.fhir.jpa.rp.r4.ScheduleResourceProvider rpScheduleR4() { 3307 ca.uhn.fhir.jpa.rp.r4.ScheduleResourceProvider retVal; 3308 retVal = new ca.uhn.fhir.jpa.rp.r4.ScheduleResourceProvider(); 3309 retVal.setContext(myFhirContext); 3310 retVal.setDao(daoScheduleR4()); 3311 return retVal; 3312 } 3313 3314 @Bean(name="mySearchParameterDaoR4") 3315 public 3316 IFhirResourceDaoSearchParameter<org.hl7.fhir.r4.model.SearchParameter> 3317 daoSearchParameterR4() { 3318 3319 ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<org.hl7.fhir.r4.model.SearchParameter> retVal; 3320 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<>(); 3321 retVal.setResourceType(org.hl7.fhir.r4.model.SearchParameter.class); 3322 retVal.setContext(myFhirContext); 3323 return retVal; 3324 } 3325 3326 @Bean(name="mySearchParameterRpR4") 3327 @Lazy 3328 public ca.uhn.fhir.jpa.rp.r4.SearchParameterResourceProvider rpSearchParameterR4() { 3329 ca.uhn.fhir.jpa.rp.r4.SearchParameterResourceProvider retVal; 3330 retVal = new ca.uhn.fhir.jpa.rp.r4.SearchParameterResourceProvider(); 3331 retVal.setContext(myFhirContext); 3332 retVal.setDao(daoSearchParameterR4()); 3333 return retVal; 3334 } 3335 3336 @Bean(name="myServiceRequestDaoR4") 3337 public 3338 IFhirResourceDao<org.hl7.fhir.r4.model.ServiceRequest> 3339 daoServiceRequestR4() { 3340 3341 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.ServiceRequest> retVal; 3342 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.ServiceRequest>(); 3343 retVal.setResourceType(org.hl7.fhir.r4.model.ServiceRequest.class); 3344 retVal.setContext(myFhirContext); 3345 return retVal; 3346 } 3347 3348 @Bean(name="myServiceRequestRpR4") 3349 @Lazy 3350 public ca.uhn.fhir.jpa.rp.r4.ServiceRequestResourceProvider rpServiceRequestR4() { 3351 ca.uhn.fhir.jpa.rp.r4.ServiceRequestResourceProvider retVal; 3352 retVal = new ca.uhn.fhir.jpa.rp.r4.ServiceRequestResourceProvider(); 3353 retVal.setContext(myFhirContext); 3354 retVal.setDao(daoServiceRequestR4()); 3355 return retVal; 3356 } 3357 3358 @Bean(name="mySlotDaoR4") 3359 public 3360 IFhirResourceDao<org.hl7.fhir.r4.model.Slot> 3361 daoSlotR4() { 3362 3363 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Slot> retVal; 3364 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Slot>(); 3365 retVal.setResourceType(org.hl7.fhir.r4.model.Slot.class); 3366 retVal.setContext(myFhirContext); 3367 return retVal; 3368 } 3369 3370 @Bean(name="mySlotRpR4") 3371 @Lazy 3372 public ca.uhn.fhir.jpa.rp.r4.SlotResourceProvider rpSlotR4() { 3373 ca.uhn.fhir.jpa.rp.r4.SlotResourceProvider retVal; 3374 retVal = new ca.uhn.fhir.jpa.rp.r4.SlotResourceProvider(); 3375 retVal.setContext(myFhirContext); 3376 retVal.setDao(daoSlotR4()); 3377 return retVal; 3378 } 3379 3380 @Bean(name="mySpecimenDaoR4") 3381 public 3382 IFhirResourceDao<org.hl7.fhir.r4.model.Specimen> 3383 daoSpecimenR4() { 3384 3385 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Specimen> retVal; 3386 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Specimen>(); 3387 retVal.setResourceType(org.hl7.fhir.r4.model.Specimen.class); 3388 retVal.setContext(myFhirContext); 3389 return retVal; 3390 } 3391 3392 @Bean(name="mySpecimenRpR4") 3393 @Lazy 3394 public ca.uhn.fhir.jpa.rp.r4.SpecimenResourceProvider rpSpecimenR4() { 3395 ca.uhn.fhir.jpa.rp.r4.SpecimenResourceProvider retVal; 3396 retVal = new ca.uhn.fhir.jpa.rp.r4.SpecimenResourceProvider(); 3397 retVal.setContext(myFhirContext); 3398 retVal.setDao(daoSpecimenR4()); 3399 return retVal; 3400 } 3401 3402 @Bean(name="mySpecimenDefinitionDaoR4") 3403 public 3404 IFhirResourceDao<org.hl7.fhir.r4.model.SpecimenDefinition> 3405 daoSpecimenDefinitionR4() { 3406 3407 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SpecimenDefinition> retVal; 3408 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SpecimenDefinition>(); 3409 retVal.setResourceType(org.hl7.fhir.r4.model.SpecimenDefinition.class); 3410 retVal.setContext(myFhirContext); 3411 return retVal; 3412 } 3413 3414 @Bean(name="mySpecimenDefinitionRpR4") 3415 @Lazy 3416 public ca.uhn.fhir.jpa.rp.r4.SpecimenDefinitionResourceProvider rpSpecimenDefinitionR4() { 3417 ca.uhn.fhir.jpa.rp.r4.SpecimenDefinitionResourceProvider retVal; 3418 retVal = new ca.uhn.fhir.jpa.rp.r4.SpecimenDefinitionResourceProvider(); 3419 retVal.setContext(myFhirContext); 3420 retVal.setDao(daoSpecimenDefinitionR4()); 3421 return retVal; 3422 } 3423 3424 @Bean(name="myStructureDefinitionDaoR4") 3425 public 3426 IFhirResourceDaoStructureDefinition<org.hl7.fhir.r4.model.StructureDefinition> 3427 daoStructureDefinitionR4() { 3428 3429 ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<org.hl7.fhir.r4.model.StructureDefinition> retVal; 3430 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<>(); 3431 retVal.setResourceType(org.hl7.fhir.r4.model.StructureDefinition.class); 3432 retVal.setContext(myFhirContext); 3433 return retVal; 3434 } 3435 3436 @Bean(name="myStructureDefinitionRpR4") 3437 @Lazy 3438 public ca.uhn.fhir.jpa.rp.r4.StructureDefinitionResourceProvider rpStructureDefinitionR4() { 3439 ca.uhn.fhir.jpa.rp.r4.StructureDefinitionResourceProvider retVal; 3440 retVal = new ca.uhn.fhir.jpa.rp.r4.StructureDefinitionResourceProvider(); 3441 retVal.setContext(myFhirContext); 3442 retVal.setDao(daoStructureDefinitionR4()); 3443 return retVal; 3444 } 3445 3446 @Bean(name="myStructureMapDaoR4") 3447 public 3448 IFhirResourceDao<org.hl7.fhir.r4.model.StructureMap> 3449 daoStructureMapR4() { 3450 3451 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.StructureMap> retVal; 3452 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.StructureMap>(); 3453 retVal.setResourceType(org.hl7.fhir.r4.model.StructureMap.class); 3454 retVal.setContext(myFhirContext); 3455 return retVal; 3456 } 3457 3458 @Bean(name="myStructureMapRpR4") 3459 @Lazy 3460 public ca.uhn.fhir.jpa.rp.r4.StructureMapResourceProvider rpStructureMapR4() { 3461 ca.uhn.fhir.jpa.rp.r4.StructureMapResourceProvider retVal; 3462 retVal = new ca.uhn.fhir.jpa.rp.r4.StructureMapResourceProvider(); 3463 retVal.setContext(myFhirContext); 3464 retVal.setDao(daoStructureMapR4()); 3465 return retVal; 3466 } 3467 3468 @Bean(name="mySubscriptionDaoR4") 3469 public 3470 IFhirResourceDaoSubscription<org.hl7.fhir.r4.model.Subscription> 3471 daoSubscriptionR4() { 3472 3473 ca.uhn.fhir.jpa.dao.r4.FhirResourceDaoSubscriptionR4 retVal; 3474 retVal = new ca.uhn.fhir.jpa.dao.r4.FhirResourceDaoSubscriptionR4(); 3475 retVal.setResourceType(org.hl7.fhir.r4.model.Subscription.class); 3476 retVal.setContext(myFhirContext); 3477 return retVal; 3478 } 3479 3480 @Bean(name="mySubscriptionRpR4") 3481 @Lazy 3482 public ca.uhn.fhir.jpa.rp.r4.SubscriptionResourceProvider rpSubscriptionR4() { 3483 ca.uhn.fhir.jpa.rp.r4.SubscriptionResourceProvider retVal; 3484 retVal = new ca.uhn.fhir.jpa.rp.r4.SubscriptionResourceProvider(); 3485 retVal.setContext(myFhirContext); 3486 retVal.setDao(daoSubscriptionR4()); 3487 return retVal; 3488 } 3489 3490 @Bean(name="mySubstanceDaoR4") 3491 public 3492 IFhirResourceDao<org.hl7.fhir.r4.model.Substance> 3493 daoSubstanceR4() { 3494 3495 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Substance> retVal; 3496 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Substance>(); 3497 retVal.setResourceType(org.hl7.fhir.r4.model.Substance.class); 3498 retVal.setContext(myFhirContext); 3499 return retVal; 3500 } 3501 3502 @Bean(name="mySubstanceRpR4") 3503 @Lazy 3504 public ca.uhn.fhir.jpa.rp.r4.SubstanceResourceProvider rpSubstanceR4() { 3505 ca.uhn.fhir.jpa.rp.r4.SubstanceResourceProvider retVal; 3506 retVal = new ca.uhn.fhir.jpa.rp.r4.SubstanceResourceProvider(); 3507 retVal.setContext(myFhirContext); 3508 retVal.setDao(daoSubstanceR4()); 3509 return retVal; 3510 } 3511 3512 @Bean(name="mySubstanceNucleicAcidDaoR4") 3513 public 3514 IFhirResourceDao<org.hl7.fhir.r4.model.SubstanceNucleicAcid> 3515 daoSubstanceNucleicAcidR4() { 3516 3517 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SubstanceNucleicAcid> retVal; 3518 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SubstanceNucleicAcid>(); 3519 retVal.setResourceType(org.hl7.fhir.r4.model.SubstanceNucleicAcid.class); 3520 retVal.setContext(myFhirContext); 3521 return retVal; 3522 } 3523 3524 @Bean(name="mySubstanceNucleicAcidRpR4") 3525 @Lazy 3526 public ca.uhn.fhir.jpa.rp.r4.SubstanceNucleicAcidResourceProvider rpSubstanceNucleicAcidR4() { 3527 ca.uhn.fhir.jpa.rp.r4.SubstanceNucleicAcidResourceProvider retVal; 3528 retVal = new ca.uhn.fhir.jpa.rp.r4.SubstanceNucleicAcidResourceProvider(); 3529 retVal.setContext(myFhirContext); 3530 retVal.setDao(daoSubstanceNucleicAcidR4()); 3531 return retVal; 3532 } 3533 3534 @Bean(name="mySubstancePolymerDaoR4") 3535 public 3536 IFhirResourceDao<org.hl7.fhir.r4.model.SubstancePolymer> 3537 daoSubstancePolymerR4() { 3538 3539 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SubstancePolymer> retVal; 3540 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SubstancePolymer>(); 3541 retVal.setResourceType(org.hl7.fhir.r4.model.SubstancePolymer.class); 3542 retVal.setContext(myFhirContext); 3543 return retVal; 3544 } 3545 3546 @Bean(name="mySubstancePolymerRpR4") 3547 @Lazy 3548 public ca.uhn.fhir.jpa.rp.r4.SubstancePolymerResourceProvider rpSubstancePolymerR4() { 3549 ca.uhn.fhir.jpa.rp.r4.SubstancePolymerResourceProvider retVal; 3550 retVal = new ca.uhn.fhir.jpa.rp.r4.SubstancePolymerResourceProvider(); 3551 retVal.setContext(myFhirContext); 3552 retVal.setDao(daoSubstancePolymerR4()); 3553 return retVal; 3554 } 3555 3556 @Bean(name="mySubstanceProteinDaoR4") 3557 public 3558 IFhirResourceDao<org.hl7.fhir.r4.model.SubstanceProtein> 3559 daoSubstanceProteinR4() { 3560 3561 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SubstanceProtein> retVal; 3562 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SubstanceProtein>(); 3563 retVal.setResourceType(org.hl7.fhir.r4.model.SubstanceProtein.class); 3564 retVal.setContext(myFhirContext); 3565 return retVal; 3566 } 3567 3568 @Bean(name="mySubstanceProteinRpR4") 3569 @Lazy 3570 public ca.uhn.fhir.jpa.rp.r4.SubstanceProteinResourceProvider rpSubstanceProteinR4() { 3571 ca.uhn.fhir.jpa.rp.r4.SubstanceProteinResourceProvider retVal; 3572 retVal = new ca.uhn.fhir.jpa.rp.r4.SubstanceProteinResourceProvider(); 3573 retVal.setContext(myFhirContext); 3574 retVal.setDao(daoSubstanceProteinR4()); 3575 return retVal; 3576 } 3577 3578 @Bean(name="mySubstanceReferenceInformationDaoR4") 3579 public 3580 IFhirResourceDao<org.hl7.fhir.r4.model.SubstanceReferenceInformation> 3581 daoSubstanceReferenceInformationR4() { 3582 3583 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SubstanceReferenceInformation> retVal; 3584 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SubstanceReferenceInformation>(); 3585 retVal.setResourceType(org.hl7.fhir.r4.model.SubstanceReferenceInformation.class); 3586 retVal.setContext(myFhirContext); 3587 return retVal; 3588 } 3589 3590 @Bean(name="mySubstanceReferenceInformationRpR4") 3591 @Lazy 3592 public ca.uhn.fhir.jpa.rp.r4.SubstanceReferenceInformationResourceProvider rpSubstanceReferenceInformationR4() { 3593 ca.uhn.fhir.jpa.rp.r4.SubstanceReferenceInformationResourceProvider retVal; 3594 retVal = new ca.uhn.fhir.jpa.rp.r4.SubstanceReferenceInformationResourceProvider(); 3595 retVal.setContext(myFhirContext); 3596 retVal.setDao(daoSubstanceReferenceInformationR4()); 3597 return retVal; 3598 } 3599 3600 @Bean(name="mySubstanceSourceMaterialDaoR4") 3601 public 3602 IFhirResourceDao<org.hl7.fhir.r4.model.SubstanceSourceMaterial> 3603 daoSubstanceSourceMaterialR4() { 3604 3605 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SubstanceSourceMaterial> retVal; 3606 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SubstanceSourceMaterial>(); 3607 retVal.setResourceType(org.hl7.fhir.r4.model.SubstanceSourceMaterial.class); 3608 retVal.setContext(myFhirContext); 3609 return retVal; 3610 } 3611 3612 @Bean(name="mySubstanceSourceMaterialRpR4") 3613 @Lazy 3614 public ca.uhn.fhir.jpa.rp.r4.SubstanceSourceMaterialResourceProvider rpSubstanceSourceMaterialR4() { 3615 ca.uhn.fhir.jpa.rp.r4.SubstanceSourceMaterialResourceProvider retVal; 3616 retVal = new ca.uhn.fhir.jpa.rp.r4.SubstanceSourceMaterialResourceProvider(); 3617 retVal.setContext(myFhirContext); 3618 retVal.setDao(daoSubstanceSourceMaterialR4()); 3619 return retVal; 3620 } 3621 3622 @Bean(name="mySubstanceSpecificationDaoR4") 3623 public 3624 IFhirResourceDao<org.hl7.fhir.r4.model.SubstanceSpecification> 3625 daoSubstanceSpecificationR4() { 3626 3627 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SubstanceSpecification> retVal; 3628 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SubstanceSpecification>(); 3629 retVal.setResourceType(org.hl7.fhir.r4.model.SubstanceSpecification.class); 3630 retVal.setContext(myFhirContext); 3631 return retVal; 3632 } 3633 3634 @Bean(name="mySubstanceSpecificationRpR4") 3635 @Lazy 3636 public ca.uhn.fhir.jpa.rp.r4.SubstanceSpecificationResourceProvider rpSubstanceSpecificationR4() { 3637 ca.uhn.fhir.jpa.rp.r4.SubstanceSpecificationResourceProvider retVal; 3638 retVal = new ca.uhn.fhir.jpa.rp.r4.SubstanceSpecificationResourceProvider(); 3639 retVal.setContext(myFhirContext); 3640 retVal.setDao(daoSubstanceSpecificationR4()); 3641 return retVal; 3642 } 3643 3644 @Bean(name="mySupplyDeliveryDaoR4") 3645 public 3646 IFhirResourceDao<org.hl7.fhir.r4.model.SupplyDelivery> 3647 daoSupplyDeliveryR4() { 3648 3649 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SupplyDelivery> retVal; 3650 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SupplyDelivery>(); 3651 retVal.setResourceType(org.hl7.fhir.r4.model.SupplyDelivery.class); 3652 retVal.setContext(myFhirContext); 3653 return retVal; 3654 } 3655 3656 @Bean(name="mySupplyDeliveryRpR4") 3657 @Lazy 3658 public ca.uhn.fhir.jpa.rp.r4.SupplyDeliveryResourceProvider rpSupplyDeliveryR4() { 3659 ca.uhn.fhir.jpa.rp.r4.SupplyDeliveryResourceProvider retVal; 3660 retVal = new ca.uhn.fhir.jpa.rp.r4.SupplyDeliveryResourceProvider(); 3661 retVal.setContext(myFhirContext); 3662 retVal.setDao(daoSupplyDeliveryR4()); 3663 return retVal; 3664 } 3665 3666 @Bean(name="mySupplyRequestDaoR4") 3667 public 3668 IFhirResourceDao<org.hl7.fhir.r4.model.SupplyRequest> 3669 daoSupplyRequestR4() { 3670 3671 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.SupplyRequest> retVal; 3672 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.SupplyRequest>(); 3673 retVal.setResourceType(org.hl7.fhir.r4.model.SupplyRequest.class); 3674 retVal.setContext(myFhirContext); 3675 return retVal; 3676 } 3677 3678 @Bean(name="mySupplyRequestRpR4") 3679 @Lazy 3680 public ca.uhn.fhir.jpa.rp.r4.SupplyRequestResourceProvider rpSupplyRequestR4() { 3681 ca.uhn.fhir.jpa.rp.r4.SupplyRequestResourceProvider retVal; 3682 retVal = new ca.uhn.fhir.jpa.rp.r4.SupplyRequestResourceProvider(); 3683 retVal.setContext(myFhirContext); 3684 retVal.setDao(daoSupplyRequestR4()); 3685 return retVal; 3686 } 3687 3688 @Bean(name="myTaskDaoR4") 3689 public 3690 IFhirResourceDao<org.hl7.fhir.r4.model.Task> 3691 daoTaskR4() { 3692 3693 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.Task> retVal; 3694 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.Task>(); 3695 retVal.setResourceType(org.hl7.fhir.r4.model.Task.class); 3696 retVal.setContext(myFhirContext); 3697 return retVal; 3698 } 3699 3700 @Bean(name="myTaskRpR4") 3701 @Lazy 3702 public ca.uhn.fhir.jpa.rp.r4.TaskResourceProvider rpTaskR4() { 3703 ca.uhn.fhir.jpa.rp.r4.TaskResourceProvider retVal; 3704 retVal = new ca.uhn.fhir.jpa.rp.r4.TaskResourceProvider(); 3705 retVal.setContext(myFhirContext); 3706 retVal.setDao(daoTaskR4()); 3707 return retVal; 3708 } 3709 3710 @Bean(name="myTerminologyCapabilitiesDaoR4") 3711 public 3712 IFhirResourceDao<org.hl7.fhir.r4.model.TerminologyCapabilities> 3713 daoTerminologyCapabilitiesR4() { 3714 3715 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.TerminologyCapabilities> retVal; 3716 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.TerminologyCapabilities>(); 3717 retVal.setResourceType(org.hl7.fhir.r4.model.TerminologyCapabilities.class); 3718 retVal.setContext(myFhirContext); 3719 return retVal; 3720 } 3721 3722 @Bean(name="myTerminologyCapabilitiesRpR4") 3723 @Lazy 3724 public ca.uhn.fhir.jpa.rp.r4.TerminologyCapabilitiesResourceProvider rpTerminologyCapabilitiesR4() { 3725 ca.uhn.fhir.jpa.rp.r4.TerminologyCapabilitiesResourceProvider retVal; 3726 retVal = new ca.uhn.fhir.jpa.rp.r4.TerminologyCapabilitiesResourceProvider(); 3727 retVal.setContext(myFhirContext); 3728 retVal.setDao(daoTerminologyCapabilitiesR4()); 3729 return retVal; 3730 } 3731 3732 @Bean(name="myTestReportDaoR4") 3733 public 3734 IFhirResourceDao<org.hl7.fhir.r4.model.TestReport> 3735 daoTestReportR4() { 3736 3737 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.TestReport> retVal; 3738 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.TestReport>(); 3739 retVal.setResourceType(org.hl7.fhir.r4.model.TestReport.class); 3740 retVal.setContext(myFhirContext); 3741 return retVal; 3742 } 3743 3744 @Bean(name="myTestReportRpR4") 3745 @Lazy 3746 public ca.uhn.fhir.jpa.rp.r4.TestReportResourceProvider rpTestReportR4() { 3747 ca.uhn.fhir.jpa.rp.r4.TestReportResourceProvider retVal; 3748 retVal = new ca.uhn.fhir.jpa.rp.r4.TestReportResourceProvider(); 3749 retVal.setContext(myFhirContext); 3750 retVal.setDao(daoTestReportR4()); 3751 return retVal; 3752 } 3753 3754 @Bean(name="myTestScriptDaoR4") 3755 public 3756 IFhirResourceDao<org.hl7.fhir.r4.model.TestScript> 3757 daoTestScriptR4() { 3758 3759 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.TestScript> retVal; 3760 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.TestScript>(); 3761 retVal.setResourceType(org.hl7.fhir.r4.model.TestScript.class); 3762 retVal.setContext(myFhirContext); 3763 return retVal; 3764 } 3765 3766 @Bean(name="myTestScriptRpR4") 3767 @Lazy 3768 public ca.uhn.fhir.jpa.rp.r4.TestScriptResourceProvider rpTestScriptR4() { 3769 ca.uhn.fhir.jpa.rp.r4.TestScriptResourceProvider retVal; 3770 retVal = new ca.uhn.fhir.jpa.rp.r4.TestScriptResourceProvider(); 3771 retVal.setContext(myFhirContext); 3772 retVal.setDao(daoTestScriptR4()); 3773 return retVal; 3774 } 3775 3776 @Bean(name="myValueSetDaoR4") 3777 public 3778 IFhirResourceDaoValueSet<org.hl7.fhir.r4.model.ValueSet> 3779 daoValueSetR4() { 3780 3781 ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<org.hl7.fhir.r4.model.ValueSet> retVal; 3782 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<>(); 3783 retVal.setResourceType(org.hl7.fhir.r4.model.ValueSet.class); 3784 retVal.setContext(myFhirContext); 3785 return retVal; 3786 } 3787 3788 @Bean(name="myValueSetRpR4") 3789 @Lazy 3790 public ca.uhn.fhir.jpa.rp.r4.ValueSetResourceProvider rpValueSetR4() { 3791 ca.uhn.fhir.jpa.rp.r4.ValueSetResourceProvider retVal; 3792 retVal = new ca.uhn.fhir.jpa.rp.r4.ValueSetResourceProvider(); 3793 retVal.setContext(myFhirContext); 3794 retVal.setDao(daoValueSetR4()); 3795 return retVal; 3796 } 3797 3798 @Bean(name="myVerificationResultDaoR4") 3799 public 3800 IFhirResourceDao<org.hl7.fhir.r4.model.VerificationResult> 3801 daoVerificationResultR4() { 3802 3803 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.VerificationResult> retVal; 3804 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.VerificationResult>(); 3805 retVal.setResourceType(org.hl7.fhir.r4.model.VerificationResult.class); 3806 retVal.setContext(myFhirContext); 3807 return retVal; 3808 } 3809 3810 @Bean(name="myVerificationResultRpR4") 3811 @Lazy 3812 public ca.uhn.fhir.jpa.rp.r4.VerificationResultResourceProvider rpVerificationResultR4() { 3813 ca.uhn.fhir.jpa.rp.r4.VerificationResultResourceProvider retVal; 3814 retVal = new ca.uhn.fhir.jpa.rp.r4.VerificationResultResourceProvider(); 3815 retVal.setContext(myFhirContext); 3816 retVal.setDao(daoVerificationResultR4()); 3817 return retVal; 3818 } 3819 3820 @Bean(name="myVisionPrescriptionDaoR4") 3821 public 3822 IFhirResourceDao<org.hl7.fhir.r4.model.VisionPrescription> 3823 daoVisionPrescriptionR4() { 3824 3825 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4.model.VisionPrescription> retVal; 3826 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4.model.VisionPrescription>(); 3827 retVal.setResourceType(org.hl7.fhir.r4.model.VisionPrescription.class); 3828 retVal.setContext(myFhirContext); 3829 return retVal; 3830 } 3831 3832 @Bean(name="myVisionPrescriptionRpR4") 3833 @Lazy 3834 public ca.uhn.fhir.jpa.rp.r4.VisionPrescriptionResourceProvider rpVisionPrescriptionR4() { 3835 ca.uhn.fhir.jpa.rp.r4.VisionPrescriptionResourceProvider retVal; 3836 retVal = new ca.uhn.fhir.jpa.rp.r4.VisionPrescriptionResourceProvider(); 3837 retVal.setContext(myFhirContext); 3838 retVal.setDao(daoVisionPrescriptionR4()); 3839 return retVal; 3840 } 3841 3842 3843 3844 3845 3846 3847}