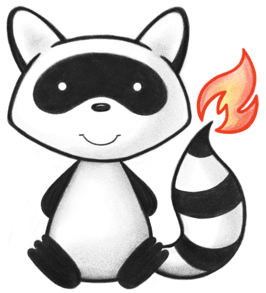
001package ca.uhn.fhir.jpa.config; 002 003import java.util.ArrayList; 004import java.util.List; 005import java.util.concurrent.Executor; 006import java.util.concurrent.Executors; 007 008import org.springframework.transaction.PlatformTransactionManager; 009import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 010 011import org.springframework.beans.factory.annotation.Autowired; 012import org.springframework.context.annotation.Bean; 013import org.springframework.context.annotation.Configuration; 014import org.springframework.context.annotation.Lazy; 015import org.springframework.data.jpa.repository.config.EnableJpaRepositories; 016import org.springframework.scheduling.annotation.EnableScheduling; 017import org.springframework.scheduling.annotation.SchedulingConfigurer; 018import org.springframework.scheduling.config.ScheduledTaskRegistrar; 019 020import ca.uhn.fhir.rest.api.IResourceSupportedSvc; 021import ca.uhn.fhir.context.FhirContext; 022import ca.uhn.fhir.model.api.IResource; 023import ca.uhn.fhir.rest.server.IResourceProvider; 024import ca.uhn.fhir.jpa.api.dao.*; 025import ca.uhn.fhir.jpa.dao.*; 026import ca.uhn.fhir.rest.server.provider.ResourceProviderFactory; 027 028@Configuration 029public class GeneratedDaoAndResourceProviderConfigR4B { 030@Autowired 031FhirContext myFhirContext; 032 033 @Bean(name="myResourceProvidersR4B") 034 public ResourceProviderFactory resourceProvidersR4B(IResourceSupportedSvc theResourceSupportedSvc) { 035 ResourceProviderFactory retVal = new ResourceProviderFactory(); 036 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Account") ? rpAccountR4B() : null); 037 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ActivityDefinition") ? rpActivityDefinitionR4B() : null); 038 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AdministrableProductDefinition") ? rpAdministrableProductDefinitionR4B() : null); 039 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AdverseEvent") ? rpAdverseEventR4B() : null); 040 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AllergyIntolerance") ? rpAllergyIntoleranceR4B() : null); 041 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Appointment") ? rpAppointmentR4B() : null); 042 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AppointmentResponse") ? rpAppointmentResponseR4B() : null); 043 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AuditEvent") ? rpAuditEventR4B() : null); 044 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Basic") ? rpBasicR4B() : null); 045 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Binary") ? rpBinaryR4B() : null); 046 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BiologicallyDerivedProduct") ? rpBiologicallyDerivedProductR4B() : null); 047 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BodyStructure") ? rpBodyStructureR4B() : null); 048 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Bundle") ? rpBundleR4B() : null); 049 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CapabilityStatement") ? rpCapabilityStatementR4B() : null); 050 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CarePlan") ? rpCarePlanR4B() : null); 051 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CareTeam") ? rpCareTeamR4B() : null); 052 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CatalogEntry") ? rpCatalogEntryR4B() : null); 053 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ChargeItem") ? rpChargeItemR4B() : null); 054 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ChargeItemDefinition") ? rpChargeItemDefinitionR4B() : null); 055 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Citation") ? rpCitationR4B() : null); 056 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Claim") ? rpClaimR4B() : null); 057 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClaimResponse") ? rpClaimResponseR4B() : null); 058 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClinicalImpression") ? rpClinicalImpressionR4B() : null); 059 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClinicalUseDefinition") ? rpClinicalUseDefinitionR4B() : null); 060 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CodeSystem") ? rpCodeSystemR4B() : null); 061 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Communication") ? rpCommunicationR4B() : null); 062 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CommunicationRequest") ? rpCommunicationRequestR4B() : null); 063 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CompartmentDefinition") ? rpCompartmentDefinitionR4B() : null); 064 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Composition") ? rpCompositionR4B() : null); 065 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ConceptMap") ? rpConceptMapR4B() : null); 066 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Condition") ? rpConditionR4B() : null); 067 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Consent") ? rpConsentR4B() : null); 068 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Contract") ? rpContractR4B() : null); 069 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Coverage") ? rpCoverageR4B() : null); 070 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CoverageEligibilityRequest") ? rpCoverageEligibilityRequestR4B() : null); 071 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CoverageEligibilityResponse") ? rpCoverageEligibilityResponseR4B() : null); 072 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DetectedIssue") ? rpDetectedIssueR4B() : null); 073 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Device") ? rpDeviceR4B() : null); 074 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceDefinition") ? rpDeviceDefinitionR4B() : null); 075 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceMetric") ? rpDeviceMetricR4B() : null); 076 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceRequest") ? rpDeviceRequestR4B() : null); 077 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceUseStatement") ? rpDeviceUseStatementR4B() : null); 078 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DiagnosticReport") ? rpDiagnosticReportR4B() : null); 079 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentManifest") ? rpDocumentManifestR4B() : null); 080 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentReference") ? rpDocumentReferenceR4B() : null); 081 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Encounter") ? rpEncounterR4B() : null); 082 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Endpoint") ? rpEndpointR4B() : null); 083 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentRequest") ? rpEnrollmentRequestR4B() : null); 084 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentResponse") ? rpEnrollmentResponseR4B() : null); 085 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EpisodeOfCare") ? rpEpisodeOfCareR4B() : null); 086 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EventDefinition") ? rpEventDefinitionR4B() : null); 087 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Evidence") ? rpEvidenceR4B() : null); 088 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EvidenceReport") ? rpEvidenceReportR4B() : null); 089 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EvidenceVariable") ? rpEvidenceVariableR4B() : null); 090 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExampleScenario") ? rpExampleScenarioR4B() : null); 091 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExplanationOfBenefit") ? rpExplanationOfBenefitR4B() : null); 092 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("FamilyMemberHistory") ? rpFamilyMemberHistoryR4B() : null); 093 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Flag") ? rpFlagR4B() : null); 094 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Goal") ? rpGoalR4B() : null); 095 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GraphDefinition") ? rpGraphDefinitionR4B() : null); 096 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Group") ? rpGroupR4B() : null); 097 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GuidanceResponse") ? rpGuidanceResponseR4B() : null); 098 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("HealthcareService") ? rpHealthcareServiceR4B() : null); 099 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImagingStudy") ? rpImagingStudyR4B() : null); 100 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Immunization") ? rpImmunizationR4B() : null); 101 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImmunizationEvaluation") ? rpImmunizationEvaluationR4B() : null); 102 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImmunizationRecommendation") ? rpImmunizationRecommendationR4B() : null); 103 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImplementationGuide") ? rpImplementationGuideR4B() : null); 104 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Ingredient") ? rpIngredientR4B() : null); 105 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("InsurancePlan") ? rpInsurancePlanR4B() : null); 106 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Invoice") ? rpInvoiceR4B() : null); 107 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Library") ? rpLibraryR4B() : null); 108 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Linkage") ? rpLinkageR4B() : null); 109 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("List") ? rpListResourceR4B() : null); 110 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Location") ? rpLocationR4B() : null); 111 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ManufacturedItemDefinition") ? rpManufacturedItemDefinitionR4B() : null); 112 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Measure") ? rpMeasureR4B() : null); 113 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MeasureReport") ? rpMeasureReportR4B() : null); 114 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Media") ? rpMediaR4B() : null); 115 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Medication") ? rpMedicationR4B() : null); 116 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationAdministration") ? rpMedicationAdministrationR4B() : null); 117 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationDispense") ? rpMedicationDispenseR4B() : null); 118 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationKnowledge") ? rpMedicationKnowledgeR4B() : null); 119 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationRequest") ? rpMedicationRequestR4B() : null); 120 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationStatement") ? rpMedicationStatementR4B() : null); 121 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductDefinition") ? rpMedicinalProductDefinitionR4B() : null); 122 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageDefinition") ? rpMessageDefinitionR4B() : null); 123 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageHeader") ? rpMessageHeaderR4B() : null); 124 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MolecularSequence") ? rpMolecularSequenceR4B() : null); 125 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NamingSystem") ? rpNamingSystemR4B() : null); 126 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NutritionOrder") ? rpNutritionOrderR4B() : null); 127 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NutritionProduct") ? rpNutritionProductR4B() : null); 128 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Observation") ? rpObservationR4B() : null); 129 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ObservationDefinition") ? rpObservationDefinitionR4B() : null); 130 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationDefinition") ? rpOperationDefinitionR4B() : null); 131 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationOutcome") ? rpOperationOutcomeR4B() : null); 132 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Organization") ? rpOrganizationR4B() : null); 133 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OrganizationAffiliation") ? rpOrganizationAffiliationR4B() : null); 134 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PackagedProductDefinition") ? rpPackagedProductDefinitionR4B() : null); 135 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Parameters") ? rpParametersR4B() : null); 136 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Patient") ? rpPatientR4B() : null); 137 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentNotice") ? rpPaymentNoticeR4B() : null); 138 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentReconciliation") ? rpPaymentReconciliationR4B() : null); 139 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Person") ? rpPersonR4B() : null); 140 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PlanDefinition") ? rpPlanDefinitionR4B() : null); 141 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Practitioner") ? rpPractitionerR4B() : null); 142 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PractitionerRole") ? rpPractitionerRoleR4B() : null); 143 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Procedure") ? rpProcedureR4B() : null); 144 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Provenance") ? rpProvenanceR4B() : null); 145 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Questionnaire") ? rpQuestionnaireR4B() : null); 146 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("QuestionnaireResponse") ? rpQuestionnaireResponseR4B() : null); 147 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RegulatedAuthorization") ? rpRegulatedAuthorizationR4B() : null); 148 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RelatedPerson") ? rpRelatedPersonR4B() : null); 149 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RequestGroup") ? rpRequestGroupR4B() : null); 150 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchDefinition") ? rpResearchDefinitionR4B() : null); 151 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchElementDefinition") ? rpResearchElementDefinitionR4B() : null); 152 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchStudy") ? rpResearchStudyR4B() : null); 153 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchSubject") ? rpResearchSubjectR4B() : null); 154 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RiskAssessment") ? rpRiskAssessmentR4B() : null); 155 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Schedule") ? rpScheduleR4B() : null); 156 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SearchParameter") ? rpSearchParameterR4B() : null); 157 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ServiceRequest") ? rpServiceRequestR4B() : null); 158 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Slot") ? rpSlotR4B() : null); 159 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Specimen") ? rpSpecimenR4B() : null); 160 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SpecimenDefinition") ? rpSpecimenDefinitionR4B() : null); 161 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureDefinition") ? rpStructureDefinitionR4B() : null); 162 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureMap") ? rpStructureMapR4B() : null); 163 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Subscription") ? rpSubscriptionR4B() : null); 164 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubscriptionStatus") ? rpSubscriptionStatusR4B() : null); 165 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubscriptionTopic") ? rpSubscriptionTopicR4B() : null); 166 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Substance") ? rpSubstanceR4B() : null); 167 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceDefinition") ? rpSubstanceDefinitionR4B() : null); 168 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyDelivery") ? rpSupplyDeliveryR4B() : null); 169 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyRequest") ? rpSupplyRequestR4B() : null); 170 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Task") ? rpTaskR4B() : null); 171 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TerminologyCapabilities") ? rpTerminologyCapabilitiesR4B() : null); 172 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestReport") ? rpTestReportR4B() : null); 173 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestScript") ? rpTestScriptR4B() : null); 174 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ValueSet") ? rpValueSetR4B() : null); 175 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("VerificationResult") ? rpVerificationResultR4B() : null); 176 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("VisionPrescription") ? rpVisionPrescriptionR4B() : null); 177 return retVal; 178 } 179 180 181 @Bean(name="myResourceDaosR4B") 182 public List<IFhirResourceDao<?>> resourceDaosR4B(IResourceSupportedSvc theResourceSupportedSvc) { 183 List<IFhirResourceDao<?>> retVal = new ArrayList<IFhirResourceDao<?>>(); 184 if (theResourceSupportedSvc.isSupported("Account")) { 185 retVal.add(daoAccountR4B()); 186 } 187 if (theResourceSupportedSvc.isSupported("ActivityDefinition")) { 188 retVal.add(daoActivityDefinitionR4B()); 189 } 190 if (theResourceSupportedSvc.isSupported("AdministrableProductDefinition")) { 191 retVal.add(daoAdministrableProductDefinitionR4B()); 192 } 193 if (theResourceSupportedSvc.isSupported("AdverseEvent")) { 194 retVal.add(daoAdverseEventR4B()); 195 } 196 if (theResourceSupportedSvc.isSupported("AllergyIntolerance")) { 197 retVal.add(daoAllergyIntoleranceR4B()); 198 } 199 if (theResourceSupportedSvc.isSupported("Appointment")) { 200 retVal.add(daoAppointmentR4B()); 201 } 202 if (theResourceSupportedSvc.isSupported("AppointmentResponse")) { 203 retVal.add(daoAppointmentResponseR4B()); 204 } 205 if (theResourceSupportedSvc.isSupported("AuditEvent")) { 206 retVal.add(daoAuditEventR4B()); 207 } 208 if (theResourceSupportedSvc.isSupported("Basic")) { 209 retVal.add(daoBasicR4B()); 210 } 211 if (theResourceSupportedSvc.isSupported("Binary")) { 212 retVal.add(daoBinaryR4B()); 213 } 214 if (theResourceSupportedSvc.isSupported("BiologicallyDerivedProduct")) { 215 retVal.add(daoBiologicallyDerivedProductR4B()); 216 } 217 if (theResourceSupportedSvc.isSupported("BodyStructure")) { 218 retVal.add(daoBodyStructureR4B()); 219 } 220 if (theResourceSupportedSvc.isSupported("Bundle")) { 221 retVal.add(daoBundleR4B()); 222 } 223 if (theResourceSupportedSvc.isSupported("CapabilityStatement")) { 224 retVal.add(daoCapabilityStatementR4B()); 225 } 226 if (theResourceSupportedSvc.isSupported("CarePlan")) { 227 retVal.add(daoCarePlanR4B()); 228 } 229 if (theResourceSupportedSvc.isSupported("CareTeam")) { 230 retVal.add(daoCareTeamR4B()); 231 } 232 if (theResourceSupportedSvc.isSupported("CatalogEntry")) { 233 retVal.add(daoCatalogEntryR4B()); 234 } 235 if (theResourceSupportedSvc.isSupported("ChargeItem")) { 236 retVal.add(daoChargeItemR4B()); 237 } 238 if (theResourceSupportedSvc.isSupported("ChargeItemDefinition")) { 239 retVal.add(daoChargeItemDefinitionR4B()); 240 } 241 if (theResourceSupportedSvc.isSupported("Citation")) { 242 retVal.add(daoCitationR4B()); 243 } 244 if (theResourceSupportedSvc.isSupported("Claim")) { 245 retVal.add(daoClaimR4B()); 246 } 247 if (theResourceSupportedSvc.isSupported("ClaimResponse")) { 248 retVal.add(daoClaimResponseR4B()); 249 } 250 if (theResourceSupportedSvc.isSupported("ClinicalImpression")) { 251 retVal.add(daoClinicalImpressionR4B()); 252 } 253 if (theResourceSupportedSvc.isSupported("ClinicalUseDefinition")) { 254 retVal.add(daoClinicalUseDefinitionR4B()); 255 } 256 if (theResourceSupportedSvc.isSupported("CodeSystem")) { 257 retVal.add(daoCodeSystemR4B()); 258 } 259 if (theResourceSupportedSvc.isSupported("Communication")) { 260 retVal.add(daoCommunicationR4B()); 261 } 262 if (theResourceSupportedSvc.isSupported("CommunicationRequest")) { 263 retVal.add(daoCommunicationRequestR4B()); 264 } 265 if (theResourceSupportedSvc.isSupported("CompartmentDefinition")) { 266 retVal.add(daoCompartmentDefinitionR4B()); 267 } 268 if (theResourceSupportedSvc.isSupported("Composition")) { 269 retVal.add(daoCompositionR4B()); 270 } 271 if (theResourceSupportedSvc.isSupported("ConceptMap")) { 272 retVal.add(daoConceptMapR4B()); 273 } 274 if (theResourceSupportedSvc.isSupported("Condition")) { 275 retVal.add(daoConditionR4B()); 276 } 277 if (theResourceSupportedSvc.isSupported("Consent")) { 278 retVal.add(daoConsentR4B()); 279 } 280 if (theResourceSupportedSvc.isSupported("Contract")) { 281 retVal.add(daoContractR4B()); 282 } 283 if (theResourceSupportedSvc.isSupported("Coverage")) { 284 retVal.add(daoCoverageR4B()); 285 } 286 if (theResourceSupportedSvc.isSupported("CoverageEligibilityRequest")) { 287 retVal.add(daoCoverageEligibilityRequestR4B()); 288 } 289 if (theResourceSupportedSvc.isSupported("CoverageEligibilityResponse")) { 290 retVal.add(daoCoverageEligibilityResponseR4B()); 291 } 292 if (theResourceSupportedSvc.isSupported("DetectedIssue")) { 293 retVal.add(daoDetectedIssueR4B()); 294 } 295 if (theResourceSupportedSvc.isSupported("Device")) { 296 retVal.add(daoDeviceR4B()); 297 } 298 if (theResourceSupportedSvc.isSupported("DeviceDefinition")) { 299 retVal.add(daoDeviceDefinitionR4B()); 300 } 301 if (theResourceSupportedSvc.isSupported("DeviceMetric")) { 302 retVal.add(daoDeviceMetricR4B()); 303 } 304 if (theResourceSupportedSvc.isSupported("DeviceRequest")) { 305 retVal.add(daoDeviceRequestR4B()); 306 } 307 if (theResourceSupportedSvc.isSupported("DeviceUseStatement")) { 308 retVal.add(daoDeviceUseStatementR4B()); 309 } 310 if (theResourceSupportedSvc.isSupported("DiagnosticReport")) { 311 retVal.add(daoDiagnosticReportR4B()); 312 } 313 if (theResourceSupportedSvc.isSupported("DocumentManifest")) { 314 retVal.add(daoDocumentManifestR4B()); 315 } 316 if (theResourceSupportedSvc.isSupported("DocumentReference")) { 317 retVal.add(daoDocumentReferenceR4B()); 318 } 319 if (theResourceSupportedSvc.isSupported("Encounter")) { 320 retVal.add(daoEncounterR4B()); 321 } 322 if (theResourceSupportedSvc.isSupported("Endpoint")) { 323 retVal.add(daoEndpointR4B()); 324 } 325 if (theResourceSupportedSvc.isSupported("EnrollmentRequest")) { 326 retVal.add(daoEnrollmentRequestR4B()); 327 } 328 if (theResourceSupportedSvc.isSupported("EnrollmentResponse")) { 329 retVal.add(daoEnrollmentResponseR4B()); 330 } 331 if (theResourceSupportedSvc.isSupported("EpisodeOfCare")) { 332 retVal.add(daoEpisodeOfCareR4B()); 333 } 334 if (theResourceSupportedSvc.isSupported("EventDefinition")) { 335 retVal.add(daoEventDefinitionR4B()); 336 } 337 if (theResourceSupportedSvc.isSupported("Evidence")) { 338 retVal.add(daoEvidenceR4B()); 339 } 340 if (theResourceSupportedSvc.isSupported("EvidenceReport")) { 341 retVal.add(daoEvidenceReportR4B()); 342 } 343 if (theResourceSupportedSvc.isSupported("EvidenceVariable")) { 344 retVal.add(daoEvidenceVariableR4B()); 345 } 346 if (theResourceSupportedSvc.isSupported("ExampleScenario")) { 347 retVal.add(daoExampleScenarioR4B()); 348 } 349 if (theResourceSupportedSvc.isSupported("ExplanationOfBenefit")) { 350 retVal.add(daoExplanationOfBenefitR4B()); 351 } 352 if (theResourceSupportedSvc.isSupported("FamilyMemberHistory")) { 353 retVal.add(daoFamilyMemberHistoryR4B()); 354 } 355 if (theResourceSupportedSvc.isSupported("Flag")) { 356 retVal.add(daoFlagR4B()); 357 } 358 if (theResourceSupportedSvc.isSupported("Goal")) { 359 retVal.add(daoGoalR4B()); 360 } 361 if (theResourceSupportedSvc.isSupported("GraphDefinition")) { 362 retVal.add(daoGraphDefinitionR4B()); 363 } 364 if (theResourceSupportedSvc.isSupported("Group")) { 365 retVal.add(daoGroupR4B()); 366 } 367 if (theResourceSupportedSvc.isSupported("GuidanceResponse")) { 368 retVal.add(daoGuidanceResponseR4B()); 369 } 370 if (theResourceSupportedSvc.isSupported("HealthcareService")) { 371 retVal.add(daoHealthcareServiceR4B()); 372 } 373 if (theResourceSupportedSvc.isSupported("ImagingStudy")) { 374 retVal.add(daoImagingStudyR4B()); 375 } 376 if (theResourceSupportedSvc.isSupported("Immunization")) { 377 retVal.add(daoImmunizationR4B()); 378 } 379 if (theResourceSupportedSvc.isSupported("ImmunizationEvaluation")) { 380 retVal.add(daoImmunizationEvaluationR4B()); 381 } 382 if (theResourceSupportedSvc.isSupported("ImmunizationRecommendation")) { 383 retVal.add(daoImmunizationRecommendationR4B()); 384 } 385 if (theResourceSupportedSvc.isSupported("ImplementationGuide")) { 386 retVal.add(daoImplementationGuideR4B()); 387 } 388 if (theResourceSupportedSvc.isSupported("Ingredient")) { 389 retVal.add(daoIngredientR4B()); 390 } 391 if (theResourceSupportedSvc.isSupported("InsurancePlan")) { 392 retVal.add(daoInsurancePlanR4B()); 393 } 394 if (theResourceSupportedSvc.isSupported("Invoice")) { 395 retVal.add(daoInvoiceR4B()); 396 } 397 if (theResourceSupportedSvc.isSupported("Library")) { 398 retVal.add(daoLibraryR4B()); 399 } 400 if (theResourceSupportedSvc.isSupported("Linkage")) { 401 retVal.add(daoLinkageR4B()); 402 } 403 if (theResourceSupportedSvc.isSupported("List")) { 404 retVal.add(daoListResourceR4B()); 405 } 406 if (theResourceSupportedSvc.isSupported("Location")) { 407 retVal.add(daoLocationR4B()); 408 } 409 if (theResourceSupportedSvc.isSupported("ManufacturedItemDefinition")) { 410 retVal.add(daoManufacturedItemDefinitionR4B()); 411 } 412 if (theResourceSupportedSvc.isSupported("Measure")) { 413 retVal.add(daoMeasureR4B()); 414 } 415 if (theResourceSupportedSvc.isSupported("MeasureReport")) { 416 retVal.add(daoMeasureReportR4B()); 417 } 418 if (theResourceSupportedSvc.isSupported("Media")) { 419 retVal.add(daoMediaR4B()); 420 } 421 if (theResourceSupportedSvc.isSupported("Medication")) { 422 retVal.add(daoMedicationR4B()); 423 } 424 if (theResourceSupportedSvc.isSupported("MedicationAdministration")) { 425 retVal.add(daoMedicationAdministrationR4B()); 426 } 427 if (theResourceSupportedSvc.isSupported("MedicationDispense")) { 428 retVal.add(daoMedicationDispenseR4B()); 429 } 430 if (theResourceSupportedSvc.isSupported("MedicationKnowledge")) { 431 retVal.add(daoMedicationKnowledgeR4B()); 432 } 433 if (theResourceSupportedSvc.isSupported("MedicationRequest")) { 434 retVal.add(daoMedicationRequestR4B()); 435 } 436 if (theResourceSupportedSvc.isSupported("MedicationStatement")) { 437 retVal.add(daoMedicationStatementR4B()); 438 } 439 if (theResourceSupportedSvc.isSupported("MedicinalProductDefinition")) { 440 retVal.add(daoMedicinalProductDefinitionR4B()); 441 } 442 if (theResourceSupportedSvc.isSupported("MessageDefinition")) { 443 retVal.add(daoMessageDefinitionR4B()); 444 } 445 if (theResourceSupportedSvc.isSupported("MessageHeader")) { 446 retVal.add(daoMessageHeaderR4B()); 447 } 448 if (theResourceSupportedSvc.isSupported("MolecularSequence")) { 449 retVal.add(daoMolecularSequenceR4B()); 450 } 451 if (theResourceSupportedSvc.isSupported("NamingSystem")) { 452 retVal.add(daoNamingSystemR4B()); 453 } 454 if (theResourceSupportedSvc.isSupported("NutritionOrder")) { 455 retVal.add(daoNutritionOrderR4B()); 456 } 457 if (theResourceSupportedSvc.isSupported("NutritionProduct")) { 458 retVal.add(daoNutritionProductR4B()); 459 } 460 if (theResourceSupportedSvc.isSupported("Observation")) { 461 retVal.add(daoObservationR4B()); 462 } 463 if (theResourceSupportedSvc.isSupported("ObservationDefinition")) { 464 retVal.add(daoObservationDefinitionR4B()); 465 } 466 if (theResourceSupportedSvc.isSupported("OperationDefinition")) { 467 retVal.add(daoOperationDefinitionR4B()); 468 } 469 if (theResourceSupportedSvc.isSupported("OperationOutcome")) { 470 retVal.add(daoOperationOutcomeR4B()); 471 } 472 if (theResourceSupportedSvc.isSupported("Organization")) { 473 retVal.add(daoOrganizationR4B()); 474 } 475 if (theResourceSupportedSvc.isSupported("OrganizationAffiliation")) { 476 retVal.add(daoOrganizationAffiliationR4B()); 477 } 478 if (theResourceSupportedSvc.isSupported("PackagedProductDefinition")) { 479 retVal.add(daoPackagedProductDefinitionR4B()); 480 } 481 if (theResourceSupportedSvc.isSupported("Parameters")) { 482 retVal.add(daoParametersR4B()); 483 } 484 if (theResourceSupportedSvc.isSupported("Patient")) { 485 retVal.add(daoPatientR4B()); 486 } 487 if (theResourceSupportedSvc.isSupported("PaymentNotice")) { 488 retVal.add(daoPaymentNoticeR4B()); 489 } 490 if (theResourceSupportedSvc.isSupported("PaymentReconciliation")) { 491 retVal.add(daoPaymentReconciliationR4B()); 492 } 493 if (theResourceSupportedSvc.isSupported("Person")) { 494 retVal.add(daoPersonR4B()); 495 } 496 if (theResourceSupportedSvc.isSupported("PlanDefinition")) { 497 retVal.add(daoPlanDefinitionR4B()); 498 } 499 if (theResourceSupportedSvc.isSupported("Practitioner")) { 500 retVal.add(daoPractitionerR4B()); 501 } 502 if (theResourceSupportedSvc.isSupported("PractitionerRole")) { 503 retVal.add(daoPractitionerRoleR4B()); 504 } 505 if (theResourceSupportedSvc.isSupported("Procedure")) { 506 retVal.add(daoProcedureR4B()); 507 } 508 if (theResourceSupportedSvc.isSupported("Provenance")) { 509 retVal.add(daoProvenanceR4B()); 510 } 511 if (theResourceSupportedSvc.isSupported("Questionnaire")) { 512 retVal.add(daoQuestionnaireR4B()); 513 } 514 if (theResourceSupportedSvc.isSupported("QuestionnaireResponse")) { 515 retVal.add(daoQuestionnaireResponseR4B()); 516 } 517 if (theResourceSupportedSvc.isSupported("RegulatedAuthorization")) { 518 retVal.add(daoRegulatedAuthorizationR4B()); 519 } 520 if (theResourceSupportedSvc.isSupported("RelatedPerson")) { 521 retVal.add(daoRelatedPersonR4B()); 522 } 523 if (theResourceSupportedSvc.isSupported("RequestGroup")) { 524 retVal.add(daoRequestGroupR4B()); 525 } 526 if (theResourceSupportedSvc.isSupported("ResearchDefinition")) { 527 retVal.add(daoResearchDefinitionR4B()); 528 } 529 if (theResourceSupportedSvc.isSupported("ResearchElementDefinition")) { 530 retVal.add(daoResearchElementDefinitionR4B()); 531 } 532 if (theResourceSupportedSvc.isSupported("ResearchStudy")) { 533 retVal.add(daoResearchStudyR4B()); 534 } 535 if (theResourceSupportedSvc.isSupported("ResearchSubject")) { 536 retVal.add(daoResearchSubjectR4B()); 537 } 538 if (theResourceSupportedSvc.isSupported("RiskAssessment")) { 539 retVal.add(daoRiskAssessmentR4B()); 540 } 541 if (theResourceSupportedSvc.isSupported("Schedule")) { 542 retVal.add(daoScheduleR4B()); 543 } 544 if (theResourceSupportedSvc.isSupported("SearchParameter")) { 545 retVal.add(daoSearchParameterR4B()); 546 } 547 if (theResourceSupportedSvc.isSupported("ServiceRequest")) { 548 retVal.add(daoServiceRequestR4B()); 549 } 550 if (theResourceSupportedSvc.isSupported("Slot")) { 551 retVal.add(daoSlotR4B()); 552 } 553 if (theResourceSupportedSvc.isSupported("Specimen")) { 554 retVal.add(daoSpecimenR4B()); 555 } 556 if (theResourceSupportedSvc.isSupported("SpecimenDefinition")) { 557 retVal.add(daoSpecimenDefinitionR4B()); 558 } 559 if (theResourceSupportedSvc.isSupported("StructureDefinition")) { 560 retVal.add(daoStructureDefinitionR4B()); 561 } 562 if (theResourceSupportedSvc.isSupported("StructureMap")) { 563 retVal.add(daoStructureMapR4B()); 564 } 565 if (theResourceSupportedSvc.isSupported("Subscription")) { 566 retVal.add(daoSubscriptionR4B()); 567 } 568 if (theResourceSupportedSvc.isSupported("SubscriptionStatus")) { 569 retVal.add(daoSubscriptionStatusR4B()); 570 } 571 if (theResourceSupportedSvc.isSupported("SubscriptionTopic")) { 572 retVal.add(daoSubscriptionTopicR4B()); 573 } 574 if (theResourceSupportedSvc.isSupported("Substance")) { 575 retVal.add(daoSubstanceR4B()); 576 } 577 if (theResourceSupportedSvc.isSupported("SubstanceDefinition")) { 578 retVal.add(daoSubstanceDefinitionR4B()); 579 } 580 if (theResourceSupportedSvc.isSupported("SupplyDelivery")) { 581 retVal.add(daoSupplyDeliveryR4B()); 582 } 583 if (theResourceSupportedSvc.isSupported("SupplyRequest")) { 584 retVal.add(daoSupplyRequestR4B()); 585 } 586 if (theResourceSupportedSvc.isSupported("Task")) { 587 retVal.add(daoTaskR4B()); 588 } 589 if (theResourceSupportedSvc.isSupported("TerminologyCapabilities")) { 590 retVal.add(daoTerminologyCapabilitiesR4B()); 591 } 592 if (theResourceSupportedSvc.isSupported("TestReport")) { 593 retVal.add(daoTestReportR4B()); 594 } 595 if (theResourceSupportedSvc.isSupported("TestScript")) { 596 retVal.add(daoTestScriptR4B()); 597 } 598 if (theResourceSupportedSvc.isSupported("ValueSet")) { 599 retVal.add(daoValueSetR4B()); 600 } 601 if (theResourceSupportedSvc.isSupported("VerificationResult")) { 602 retVal.add(daoVerificationResultR4B()); 603 } 604 if (theResourceSupportedSvc.isSupported("VisionPrescription")) { 605 retVal.add(daoVisionPrescriptionR4B()); 606 } 607 return retVal; 608 } 609 610 @Bean(name="myAccountDaoR4B") 611 public 612 IFhirResourceDao<org.hl7.fhir.r4b.model.Account> 613 daoAccountR4B() { 614 615 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Account> retVal; 616 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Account>(); 617 retVal.setResourceType(org.hl7.fhir.r4b.model.Account.class); 618 retVal.setContext(myFhirContext); 619 return retVal; 620 } 621 622 @Bean(name="myAccountRpR4B") 623 @Lazy 624 public ca.uhn.fhir.jpa.rp.r4b.AccountResourceProvider rpAccountR4B() { 625 ca.uhn.fhir.jpa.rp.r4b.AccountResourceProvider retVal; 626 retVal = new ca.uhn.fhir.jpa.rp.r4b.AccountResourceProvider(); 627 retVal.setContext(myFhirContext); 628 retVal.setDao(daoAccountR4B()); 629 return retVal; 630 } 631 632 @Bean(name="myActivityDefinitionDaoR4B") 633 public 634 IFhirResourceDao<org.hl7.fhir.r4b.model.ActivityDefinition> 635 daoActivityDefinitionR4B() { 636 637 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ActivityDefinition> retVal; 638 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ActivityDefinition>(); 639 retVal.setResourceType(org.hl7.fhir.r4b.model.ActivityDefinition.class); 640 retVal.setContext(myFhirContext); 641 return retVal; 642 } 643 644 @Bean(name="myActivityDefinitionRpR4B") 645 @Lazy 646 public ca.uhn.fhir.jpa.rp.r4b.ActivityDefinitionResourceProvider rpActivityDefinitionR4B() { 647 ca.uhn.fhir.jpa.rp.r4b.ActivityDefinitionResourceProvider retVal; 648 retVal = new ca.uhn.fhir.jpa.rp.r4b.ActivityDefinitionResourceProvider(); 649 retVal.setContext(myFhirContext); 650 retVal.setDao(daoActivityDefinitionR4B()); 651 return retVal; 652 } 653 654 @Bean(name="myAdministrableProductDefinitionDaoR4B") 655 public 656 IFhirResourceDao<org.hl7.fhir.r4b.model.AdministrableProductDefinition> 657 daoAdministrableProductDefinitionR4B() { 658 659 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.AdministrableProductDefinition> retVal; 660 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.AdministrableProductDefinition>(); 661 retVal.setResourceType(org.hl7.fhir.r4b.model.AdministrableProductDefinition.class); 662 retVal.setContext(myFhirContext); 663 return retVal; 664 } 665 666 @Bean(name="myAdministrableProductDefinitionRpR4B") 667 @Lazy 668 public ca.uhn.fhir.jpa.rp.r4b.AdministrableProductDefinitionResourceProvider rpAdministrableProductDefinitionR4B() { 669 ca.uhn.fhir.jpa.rp.r4b.AdministrableProductDefinitionResourceProvider retVal; 670 retVal = new ca.uhn.fhir.jpa.rp.r4b.AdministrableProductDefinitionResourceProvider(); 671 retVal.setContext(myFhirContext); 672 retVal.setDao(daoAdministrableProductDefinitionR4B()); 673 return retVal; 674 } 675 676 @Bean(name="myAdverseEventDaoR4B") 677 public 678 IFhirResourceDao<org.hl7.fhir.r4b.model.AdverseEvent> 679 daoAdverseEventR4B() { 680 681 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.AdverseEvent> retVal; 682 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.AdverseEvent>(); 683 retVal.setResourceType(org.hl7.fhir.r4b.model.AdverseEvent.class); 684 retVal.setContext(myFhirContext); 685 return retVal; 686 } 687 688 @Bean(name="myAdverseEventRpR4B") 689 @Lazy 690 public ca.uhn.fhir.jpa.rp.r4b.AdverseEventResourceProvider rpAdverseEventR4B() { 691 ca.uhn.fhir.jpa.rp.r4b.AdverseEventResourceProvider retVal; 692 retVal = new ca.uhn.fhir.jpa.rp.r4b.AdverseEventResourceProvider(); 693 retVal.setContext(myFhirContext); 694 retVal.setDao(daoAdverseEventR4B()); 695 return retVal; 696 } 697 698 @Bean(name="myAllergyIntoleranceDaoR4B") 699 public 700 IFhirResourceDao<org.hl7.fhir.r4b.model.AllergyIntolerance> 701 daoAllergyIntoleranceR4B() { 702 703 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.AllergyIntolerance> retVal; 704 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.AllergyIntolerance>(); 705 retVal.setResourceType(org.hl7.fhir.r4b.model.AllergyIntolerance.class); 706 retVal.setContext(myFhirContext); 707 return retVal; 708 } 709 710 @Bean(name="myAllergyIntoleranceRpR4B") 711 @Lazy 712 public ca.uhn.fhir.jpa.rp.r4b.AllergyIntoleranceResourceProvider rpAllergyIntoleranceR4B() { 713 ca.uhn.fhir.jpa.rp.r4b.AllergyIntoleranceResourceProvider retVal; 714 retVal = new ca.uhn.fhir.jpa.rp.r4b.AllergyIntoleranceResourceProvider(); 715 retVal.setContext(myFhirContext); 716 retVal.setDao(daoAllergyIntoleranceR4B()); 717 return retVal; 718 } 719 720 @Bean(name="myAppointmentDaoR4B") 721 public 722 IFhirResourceDao<org.hl7.fhir.r4b.model.Appointment> 723 daoAppointmentR4B() { 724 725 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Appointment> retVal; 726 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Appointment>(); 727 retVal.setResourceType(org.hl7.fhir.r4b.model.Appointment.class); 728 retVal.setContext(myFhirContext); 729 return retVal; 730 } 731 732 @Bean(name="myAppointmentRpR4B") 733 @Lazy 734 public ca.uhn.fhir.jpa.rp.r4b.AppointmentResourceProvider rpAppointmentR4B() { 735 ca.uhn.fhir.jpa.rp.r4b.AppointmentResourceProvider retVal; 736 retVal = new ca.uhn.fhir.jpa.rp.r4b.AppointmentResourceProvider(); 737 retVal.setContext(myFhirContext); 738 retVal.setDao(daoAppointmentR4B()); 739 return retVal; 740 } 741 742 @Bean(name="myAppointmentResponseDaoR4B") 743 public 744 IFhirResourceDao<org.hl7.fhir.r4b.model.AppointmentResponse> 745 daoAppointmentResponseR4B() { 746 747 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.AppointmentResponse> retVal; 748 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.AppointmentResponse>(); 749 retVal.setResourceType(org.hl7.fhir.r4b.model.AppointmentResponse.class); 750 retVal.setContext(myFhirContext); 751 return retVal; 752 } 753 754 @Bean(name="myAppointmentResponseRpR4B") 755 @Lazy 756 public ca.uhn.fhir.jpa.rp.r4b.AppointmentResponseResourceProvider rpAppointmentResponseR4B() { 757 ca.uhn.fhir.jpa.rp.r4b.AppointmentResponseResourceProvider retVal; 758 retVal = new ca.uhn.fhir.jpa.rp.r4b.AppointmentResponseResourceProvider(); 759 retVal.setContext(myFhirContext); 760 retVal.setDao(daoAppointmentResponseR4B()); 761 return retVal; 762 } 763 764 @Bean(name="myAuditEventDaoR4B") 765 public 766 IFhirResourceDao<org.hl7.fhir.r4b.model.AuditEvent> 767 daoAuditEventR4B() { 768 769 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.AuditEvent> retVal; 770 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.AuditEvent>(); 771 retVal.setResourceType(org.hl7.fhir.r4b.model.AuditEvent.class); 772 retVal.setContext(myFhirContext); 773 return retVal; 774 } 775 776 @Bean(name="myAuditEventRpR4B") 777 @Lazy 778 public ca.uhn.fhir.jpa.rp.r4b.AuditEventResourceProvider rpAuditEventR4B() { 779 ca.uhn.fhir.jpa.rp.r4b.AuditEventResourceProvider retVal; 780 retVal = new ca.uhn.fhir.jpa.rp.r4b.AuditEventResourceProvider(); 781 retVal.setContext(myFhirContext); 782 retVal.setDao(daoAuditEventR4B()); 783 return retVal; 784 } 785 786 @Bean(name="myBasicDaoR4B") 787 public 788 IFhirResourceDao<org.hl7.fhir.r4b.model.Basic> 789 daoBasicR4B() { 790 791 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Basic> retVal; 792 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Basic>(); 793 retVal.setResourceType(org.hl7.fhir.r4b.model.Basic.class); 794 retVal.setContext(myFhirContext); 795 return retVal; 796 } 797 798 @Bean(name="myBasicRpR4B") 799 @Lazy 800 public ca.uhn.fhir.jpa.rp.r4b.BasicResourceProvider rpBasicR4B() { 801 ca.uhn.fhir.jpa.rp.r4b.BasicResourceProvider retVal; 802 retVal = new ca.uhn.fhir.jpa.rp.r4b.BasicResourceProvider(); 803 retVal.setContext(myFhirContext); 804 retVal.setDao(daoBasicR4B()); 805 return retVal; 806 } 807 808 @Bean(name="myBinaryDaoR4B") 809 public 810 IFhirResourceDao<org.hl7.fhir.r4b.model.Binary> 811 daoBinaryR4B() { 812 813 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Binary> retVal; 814 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Binary>(); 815 retVal.setResourceType(org.hl7.fhir.r4b.model.Binary.class); 816 retVal.setContext(myFhirContext); 817 return retVal; 818 } 819 820 @Bean(name="myBinaryRpR4B") 821 @Lazy 822 public ca.uhn.fhir.jpa.rp.r4b.BinaryResourceProvider rpBinaryR4B() { 823 ca.uhn.fhir.jpa.rp.r4b.BinaryResourceProvider retVal; 824 retVal = new ca.uhn.fhir.jpa.rp.r4b.BinaryResourceProvider(); 825 retVal.setContext(myFhirContext); 826 retVal.setDao(daoBinaryR4B()); 827 return retVal; 828 } 829 830 @Bean(name="myBiologicallyDerivedProductDaoR4B") 831 public 832 IFhirResourceDao<org.hl7.fhir.r4b.model.BiologicallyDerivedProduct> 833 daoBiologicallyDerivedProductR4B() { 834 835 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.BiologicallyDerivedProduct> retVal; 836 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.BiologicallyDerivedProduct>(); 837 retVal.setResourceType(org.hl7.fhir.r4b.model.BiologicallyDerivedProduct.class); 838 retVal.setContext(myFhirContext); 839 return retVal; 840 } 841 842 @Bean(name="myBiologicallyDerivedProductRpR4B") 843 @Lazy 844 public ca.uhn.fhir.jpa.rp.r4b.BiologicallyDerivedProductResourceProvider rpBiologicallyDerivedProductR4B() { 845 ca.uhn.fhir.jpa.rp.r4b.BiologicallyDerivedProductResourceProvider retVal; 846 retVal = new ca.uhn.fhir.jpa.rp.r4b.BiologicallyDerivedProductResourceProvider(); 847 retVal.setContext(myFhirContext); 848 retVal.setDao(daoBiologicallyDerivedProductR4B()); 849 return retVal; 850 } 851 852 @Bean(name="myBodyStructureDaoR4B") 853 public 854 IFhirResourceDao<org.hl7.fhir.r4b.model.BodyStructure> 855 daoBodyStructureR4B() { 856 857 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.BodyStructure> retVal; 858 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.BodyStructure>(); 859 retVal.setResourceType(org.hl7.fhir.r4b.model.BodyStructure.class); 860 retVal.setContext(myFhirContext); 861 return retVal; 862 } 863 864 @Bean(name="myBodyStructureRpR4B") 865 @Lazy 866 public ca.uhn.fhir.jpa.rp.r4b.BodyStructureResourceProvider rpBodyStructureR4B() { 867 ca.uhn.fhir.jpa.rp.r4b.BodyStructureResourceProvider retVal; 868 retVal = new ca.uhn.fhir.jpa.rp.r4b.BodyStructureResourceProvider(); 869 retVal.setContext(myFhirContext); 870 retVal.setDao(daoBodyStructureR4B()); 871 return retVal; 872 } 873 874 @Bean(name="myBundleDaoR4B") 875 public 876 IFhirResourceDao<org.hl7.fhir.r4b.model.Bundle> 877 daoBundleR4B() { 878 879 ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<org.hl7.fhir.r4b.model.Bundle> retVal; 880 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<>(); 881 retVal.setResourceType(org.hl7.fhir.r4b.model.Bundle.class); 882 retVal.setContext(myFhirContext); 883 return retVal; 884 } 885 886 @Bean(name="myBundleRpR4B") 887 @Lazy 888 public ca.uhn.fhir.jpa.rp.r4b.BundleResourceProvider rpBundleR4B() { 889 ca.uhn.fhir.jpa.rp.r4b.BundleResourceProvider retVal; 890 retVal = new ca.uhn.fhir.jpa.rp.r4b.BundleResourceProvider(); 891 retVal.setContext(myFhirContext); 892 retVal.setDao(daoBundleR4B()); 893 return retVal; 894 } 895 896 @Bean(name="myCapabilityStatementDaoR4B") 897 public 898 IFhirResourceDao<org.hl7.fhir.r4b.model.CapabilityStatement> 899 daoCapabilityStatementR4B() { 900 901 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.CapabilityStatement> retVal; 902 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.CapabilityStatement>(); 903 retVal.setResourceType(org.hl7.fhir.r4b.model.CapabilityStatement.class); 904 retVal.setContext(myFhirContext); 905 return retVal; 906 } 907 908 @Bean(name="myCapabilityStatementRpR4B") 909 @Lazy 910 public ca.uhn.fhir.jpa.rp.r4b.CapabilityStatementResourceProvider rpCapabilityStatementR4B() { 911 ca.uhn.fhir.jpa.rp.r4b.CapabilityStatementResourceProvider retVal; 912 retVal = new ca.uhn.fhir.jpa.rp.r4b.CapabilityStatementResourceProvider(); 913 retVal.setContext(myFhirContext); 914 retVal.setDao(daoCapabilityStatementR4B()); 915 return retVal; 916 } 917 918 @Bean(name="myCarePlanDaoR4B") 919 public 920 IFhirResourceDao<org.hl7.fhir.r4b.model.CarePlan> 921 daoCarePlanR4B() { 922 923 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.CarePlan> retVal; 924 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.CarePlan>(); 925 retVal.setResourceType(org.hl7.fhir.r4b.model.CarePlan.class); 926 retVal.setContext(myFhirContext); 927 return retVal; 928 } 929 930 @Bean(name="myCarePlanRpR4B") 931 @Lazy 932 public ca.uhn.fhir.jpa.rp.r4b.CarePlanResourceProvider rpCarePlanR4B() { 933 ca.uhn.fhir.jpa.rp.r4b.CarePlanResourceProvider retVal; 934 retVal = new ca.uhn.fhir.jpa.rp.r4b.CarePlanResourceProvider(); 935 retVal.setContext(myFhirContext); 936 retVal.setDao(daoCarePlanR4B()); 937 return retVal; 938 } 939 940 @Bean(name="myCareTeamDaoR4B") 941 public 942 IFhirResourceDao<org.hl7.fhir.r4b.model.CareTeam> 943 daoCareTeamR4B() { 944 945 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.CareTeam> retVal; 946 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.CareTeam>(); 947 retVal.setResourceType(org.hl7.fhir.r4b.model.CareTeam.class); 948 retVal.setContext(myFhirContext); 949 return retVal; 950 } 951 952 @Bean(name="myCareTeamRpR4B") 953 @Lazy 954 public ca.uhn.fhir.jpa.rp.r4b.CareTeamResourceProvider rpCareTeamR4B() { 955 ca.uhn.fhir.jpa.rp.r4b.CareTeamResourceProvider retVal; 956 retVal = new ca.uhn.fhir.jpa.rp.r4b.CareTeamResourceProvider(); 957 retVal.setContext(myFhirContext); 958 retVal.setDao(daoCareTeamR4B()); 959 return retVal; 960 } 961 962 @Bean(name="myCatalogEntryDaoR4B") 963 public 964 IFhirResourceDao<org.hl7.fhir.r4b.model.CatalogEntry> 965 daoCatalogEntryR4B() { 966 967 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.CatalogEntry> retVal; 968 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.CatalogEntry>(); 969 retVal.setResourceType(org.hl7.fhir.r4b.model.CatalogEntry.class); 970 retVal.setContext(myFhirContext); 971 return retVal; 972 } 973 974 @Bean(name="myCatalogEntryRpR4B") 975 @Lazy 976 public ca.uhn.fhir.jpa.rp.r4b.CatalogEntryResourceProvider rpCatalogEntryR4B() { 977 ca.uhn.fhir.jpa.rp.r4b.CatalogEntryResourceProvider retVal; 978 retVal = new ca.uhn.fhir.jpa.rp.r4b.CatalogEntryResourceProvider(); 979 retVal.setContext(myFhirContext); 980 retVal.setDao(daoCatalogEntryR4B()); 981 return retVal; 982 } 983 984 @Bean(name="myChargeItemDaoR4B") 985 public 986 IFhirResourceDao<org.hl7.fhir.r4b.model.ChargeItem> 987 daoChargeItemR4B() { 988 989 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ChargeItem> retVal; 990 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ChargeItem>(); 991 retVal.setResourceType(org.hl7.fhir.r4b.model.ChargeItem.class); 992 retVal.setContext(myFhirContext); 993 return retVal; 994 } 995 996 @Bean(name="myChargeItemRpR4B") 997 @Lazy 998 public ca.uhn.fhir.jpa.rp.r4b.ChargeItemResourceProvider rpChargeItemR4B() { 999 ca.uhn.fhir.jpa.rp.r4b.ChargeItemResourceProvider retVal; 1000 retVal = new ca.uhn.fhir.jpa.rp.r4b.ChargeItemResourceProvider(); 1001 retVal.setContext(myFhirContext); 1002 retVal.setDao(daoChargeItemR4B()); 1003 return retVal; 1004 } 1005 1006 @Bean(name="myChargeItemDefinitionDaoR4B") 1007 public 1008 IFhirResourceDao<org.hl7.fhir.r4b.model.ChargeItemDefinition> 1009 daoChargeItemDefinitionR4B() { 1010 1011 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ChargeItemDefinition> retVal; 1012 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ChargeItemDefinition>(); 1013 retVal.setResourceType(org.hl7.fhir.r4b.model.ChargeItemDefinition.class); 1014 retVal.setContext(myFhirContext); 1015 return retVal; 1016 } 1017 1018 @Bean(name="myChargeItemDefinitionRpR4B") 1019 @Lazy 1020 public ca.uhn.fhir.jpa.rp.r4b.ChargeItemDefinitionResourceProvider rpChargeItemDefinitionR4B() { 1021 ca.uhn.fhir.jpa.rp.r4b.ChargeItemDefinitionResourceProvider retVal; 1022 retVal = new ca.uhn.fhir.jpa.rp.r4b.ChargeItemDefinitionResourceProvider(); 1023 retVal.setContext(myFhirContext); 1024 retVal.setDao(daoChargeItemDefinitionR4B()); 1025 return retVal; 1026 } 1027 1028 @Bean(name="myCitationDaoR4B") 1029 public 1030 IFhirResourceDao<org.hl7.fhir.r4b.model.Citation> 1031 daoCitationR4B() { 1032 1033 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Citation> retVal; 1034 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Citation>(); 1035 retVal.setResourceType(org.hl7.fhir.r4b.model.Citation.class); 1036 retVal.setContext(myFhirContext); 1037 return retVal; 1038 } 1039 1040 @Bean(name="myCitationRpR4B") 1041 @Lazy 1042 public ca.uhn.fhir.jpa.rp.r4b.CitationResourceProvider rpCitationR4B() { 1043 ca.uhn.fhir.jpa.rp.r4b.CitationResourceProvider retVal; 1044 retVal = new ca.uhn.fhir.jpa.rp.r4b.CitationResourceProvider(); 1045 retVal.setContext(myFhirContext); 1046 retVal.setDao(daoCitationR4B()); 1047 return retVal; 1048 } 1049 1050 @Bean(name="myClaimDaoR4B") 1051 public 1052 IFhirResourceDao<org.hl7.fhir.r4b.model.Claim> 1053 daoClaimR4B() { 1054 1055 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Claim> retVal; 1056 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Claim>(); 1057 retVal.setResourceType(org.hl7.fhir.r4b.model.Claim.class); 1058 retVal.setContext(myFhirContext); 1059 return retVal; 1060 } 1061 1062 @Bean(name="myClaimRpR4B") 1063 @Lazy 1064 public ca.uhn.fhir.jpa.rp.r4b.ClaimResourceProvider rpClaimR4B() { 1065 ca.uhn.fhir.jpa.rp.r4b.ClaimResourceProvider retVal; 1066 retVal = new ca.uhn.fhir.jpa.rp.r4b.ClaimResourceProvider(); 1067 retVal.setContext(myFhirContext); 1068 retVal.setDao(daoClaimR4B()); 1069 return retVal; 1070 } 1071 1072 @Bean(name="myClaimResponseDaoR4B") 1073 public 1074 IFhirResourceDao<org.hl7.fhir.r4b.model.ClaimResponse> 1075 daoClaimResponseR4B() { 1076 1077 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ClaimResponse> retVal; 1078 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ClaimResponse>(); 1079 retVal.setResourceType(org.hl7.fhir.r4b.model.ClaimResponse.class); 1080 retVal.setContext(myFhirContext); 1081 return retVal; 1082 } 1083 1084 @Bean(name="myClaimResponseRpR4B") 1085 @Lazy 1086 public ca.uhn.fhir.jpa.rp.r4b.ClaimResponseResourceProvider rpClaimResponseR4B() { 1087 ca.uhn.fhir.jpa.rp.r4b.ClaimResponseResourceProvider retVal; 1088 retVal = new ca.uhn.fhir.jpa.rp.r4b.ClaimResponseResourceProvider(); 1089 retVal.setContext(myFhirContext); 1090 retVal.setDao(daoClaimResponseR4B()); 1091 return retVal; 1092 } 1093 1094 @Bean(name="myClinicalImpressionDaoR4B") 1095 public 1096 IFhirResourceDao<org.hl7.fhir.r4b.model.ClinicalImpression> 1097 daoClinicalImpressionR4B() { 1098 1099 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ClinicalImpression> retVal; 1100 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ClinicalImpression>(); 1101 retVal.setResourceType(org.hl7.fhir.r4b.model.ClinicalImpression.class); 1102 retVal.setContext(myFhirContext); 1103 return retVal; 1104 } 1105 1106 @Bean(name="myClinicalImpressionRpR4B") 1107 @Lazy 1108 public ca.uhn.fhir.jpa.rp.r4b.ClinicalImpressionResourceProvider rpClinicalImpressionR4B() { 1109 ca.uhn.fhir.jpa.rp.r4b.ClinicalImpressionResourceProvider retVal; 1110 retVal = new ca.uhn.fhir.jpa.rp.r4b.ClinicalImpressionResourceProvider(); 1111 retVal.setContext(myFhirContext); 1112 retVal.setDao(daoClinicalImpressionR4B()); 1113 return retVal; 1114 } 1115 1116 @Bean(name="myClinicalUseDefinitionDaoR4B") 1117 public 1118 IFhirResourceDao<org.hl7.fhir.r4b.model.ClinicalUseDefinition> 1119 daoClinicalUseDefinitionR4B() { 1120 1121 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ClinicalUseDefinition> retVal; 1122 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ClinicalUseDefinition>(); 1123 retVal.setResourceType(org.hl7.fhir.r4b.model.ClinicalUseDefinition.class); 1124 retVal.setContext(myFhirContext); 1125 return retVal; 1126 } 1127 1128 @Bean(name="myClinicalUseDefinitionRpR4B") 1129 @Lazy 1130 public ca.uhn.fhir.jpa.rp.r4b.ClinicalUseDefinitionResourceProvider rpClinicalUseDefinitionR4B() { 1131 ca.uhn.fhir.jpa.rp.r4b.ClinicalUseDefinitionResourceProvider retVal; 1132 retVal = new ca.uhn.fhir.jpa.rp.r4b.ClinicalUseDefinitionResourceProvider(); 1133 retVal.setContext(myFhirContext); 1134 retVal.setDao(daoClinicalUseDefinitionR4B()); 1135 return retVal; 1136 } 1137 1138 @Bean(name="myCodeSystemDaoR4B") 1139 public 1140 IFhirResourceDaoCodeSystem<org.hl7.fhir.r4b.model.CodeSystem> 1141 daoCodeSystemR4B() { 1142 1143 ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem<org.hl7.fhir.r4b.model.CodeSystem> retVal; 1144 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem<>(); 1145 retVal.setResourceType(org.hl7.fhir.r4b.model.CodeSystem.class); 1146 retVal.setContext(myFhirContext); 1147 return retVal; 1148 } 1149 1150 @Bean(name="myCodeSystemRpR4B") 1151 @Lazy 1152 public ca.uhn.fhir.jpa.rp.r4b.CodeSystemResourceProvider rpCodeSystemR4B() { 1153 ca.uhn.fhir.jpa.rp.r4b.CodeSystemResourceProvider retVal; 1154 retVal = new ca.uhn.fhir.jpa.rp.r4b.CodeSystemResourceProvider(); 1155 retVal.setContext(myFhirContext); 1156 retVal.setDao(daoCodeSystemR4B()); 1157 return retVal; 1158 } 1159 1160 @Bean(name="myCommunicationDaoR4B") 1161 public 1162 IFhirResourceDao<org.hl7.fhir.r4b.model.Communication> 1163 daoCommunicationR4B() { 1164 1165 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Communication> retVal; 1166 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Communication>(); 1167 retVal.setResourceType(org.hl7.fhir.r4b.model.Communication.class); 1168 retVal.setContext(myFhirContext); 1169 return retVal; 1170 } 1171 1172 @Bean(name="myCommunicationRpR4B") 1173 @Lazy 1174 public ca.uhn.fhir.jpa.rp.r4b.CommunicationResourceProvider rpCommunicationR4B() { 1175 ca.uhn.fhir.jpa.rp.r4b.CommunicationResourceProvider retVal; 1176 retVal = new ca.uhn.fhir.jpa.rp.r4b.CommunicationResourceProvider(); 1177 retVal.setContext(myFhirContext); 1178 retVal.setDao(daoCommunicationR4B()); 1179 return retVal; 1180 } 1181 1182 @Bean(name="myCommunicationRequestDaoR4B") 1183 public 1184 IFhirResourceDao<org.hl7.fhir.r4b.model.CommunicationRequest> 1185 daoCommunicationRequestR4B() { 1186 1187 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.CommunicationRequest> retVal; 1188 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.CommunicationRequest>(); 1189 retVal.setResourceType(org.hl7.fhir.r4b.model.CommunicationRequest.class); 1190 retVal.setContext(myFhirContext); 1191 return retVal; 1192 } 1193 1194 @Bean(name="myCommunicationRequestRpR4B") 1195 @Lazy 1196 public ca.uhn.fhir.jpa.rp.r4b.CommunicationRequestResourceProvider rpCommunicationRequestR4B() { 1197 ca.uhn.fhir.jpa.rp.r4b.CommunicationRequestResourceProvider retVal; 1198 retVal = new ca.uhn.fhir.jpa.rp.r4b.CommunicationRequestResourceProvider(); 1199 retVal.setContext(myFhirContext); 1200 retVal.setDao(daoCommunicationRequestR4B()); 1201 return retVal; 1202 } 1203 1204 @Bean(name="myCompartmentDefinitionDaoR4B") 1205 public 1206 IFhirResourceDao<org.hl7.fhir.r4b.model.CompartmentDefinition> 1207 daoCompartmentDefinitionR4B() { 1208 1209 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.CompartmentDefinition> retVal; 1210 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.CompartmentDefinition>(); 1211 retVal.setResourceType(org.hl7.fhir.r4b.model.CompartmentDefinition.class); 1212 retVal.setContext(myFhirContext); 1213 return retVal; 1214 } 1215 1216 @Bean(name="myCompartmentDefinitionRpR4B") 1217 @Lazy 1218 public ca.uhn.fhir.jpa.rp.r4b.CompartmentDefinitionResourceProvider rpCompartmentDefinitionR4B() { 1219 ca.uhn.fhir.jpa.rp.r4b.CompartmentDefinitionResourceProvider retVal; 1220 retVal = new ca.uhn.fhir.jpa.rp.r4b.CompartmentDefinitionResourceProvider(); 1221 retVal.setContext(myFhirContext); 1222 retVal.setDao(daoCompartmentDefinitionR4B()); 1223 return retVal; 1224 } 1225 1226 @Bean(name="myCompositionDaoR4B") 1227 public 1228 IFhirResourceDaoComposition<org.hl7.fhir.r4b.model.Composition> 1229 daoCompositionR4B() { 1230 1231 ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<org.hl7.fhir.r4b.model.Composition> retVal; 1232 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<>(); 1233 retVal.setResourceType(org.hl7.fhir.r4b.model.Composition.class); 1234 retVal.setContext(myFhirContext); 1235 return retVal; 1236 } 1237 1238 @Bean(name="myCompositionRpR4B") 1239 @Lazy 1240 public ca.uhn.fhir.jpa.rp.r4b.CompositionResourceProvider rpCompositionR4B() { 1241 ca.uhn.fhir.jpa.rp.r4b.CompositionResourceProvider retVal; 1242 retVal = new ca.uhn.fhir.jpa.rp.r4b.CompositionResourceProvider(); 1243 retVal.setContext(myFhirContext); 1244 retVal.setDao(daoCompositionR4B()); 1245 return retVal; 1246 } 1247 1248 @Bean(name="myConceptMapDaoR4B") 1249 public 1250 IFhirResourceDaoConceptMap<org.hl7.fhir.r4b.model.ConceptMap> 1251 daoConceptMapR4B() { 1252 1253 ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<org.hl7.fhir.r4b.model.ConceptMap> retVal; 1254 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<>(); 1255 retVal.setResourceType(org.hl7.fhir.r4b.model.ConceptMap.class); 1256 retVal.setContext(myFhirContext); 1257 return retVal; 1258 } 1259 1260 @Bean(name="myConceptMapRpR4B") 1261 @Lazy 1262 public ca.uhn.fhir.jpa.rp.r4b.ConceptMapResourceProvider rpConceptMapR4B() { 1263 ca.uhn.fhir.jpa.rp.r4b.ConceptMapResourceProvider retVal; 1264 retVal = new ca.uhn.fhir.jpa.rp.r4b.ConceptMapResourceProvider(); 1265 retVal.setContext(myFhirContext); 1266 retVal.setDao(daoConceptMapR4B()); 1267 return retVal; 1268 } 1269 1270 @Bean(name="myConditionDaoR4B") 1271 public 1272 IFhirResourceDao<org.hl7.fhir.r4b.model.Condition> 1273 daoConditionR4B() { 1274 1275 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Condition> retVal; 1276 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Condition>(); 1277 retVal.setResourceType(org.hl7.fhir.r4b.model.Condition.class); 1278 retVal.setContext(myFhirContext); 1279 return retVal; 1280 } 1281 1282 @Bean(name="myConditionRpR4B") 1283 @Lazy 1284 public ca.uhn.fhir.jpa.rp.r4b.ConditionResourceProvider rpConditionR4B() { 1285 ca.uhn.fhir.jpa.rp.r4b.ConditionResourceProvider retVal; 1286 retVal = new ca.uhn.fhir.jpa.rp.r4b.ConditionResourceProvider(); 1287 retVal.setContext(myFhirContext); 1288 retVal.setDao(daoConditionR4B()); 1289 return retVal; 1290 } 1291 1292 @Bean(name="myConsentDaoR4B") 1293 public 1294 IFhirResourceDao<org.hl7.fhir.r4b.model.Consent> 1295 daoConsentR4B() { 1296 1297 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Consent> retVal; 1298 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Consent>(); 1299 retVal.setResourceType(org.hl7.fhir.r4b.model.Consent.class); 1300 retVal.setContext(myFhirContext); 1301 return retVal; 1302 } 1303 1304 @Bean(name="myConsentRpR4B") 1305 @Lazy 1306 public ca.uhn.fhir.jpa.rp.r4b.ConsentResourceProvider rpConsentR4B() { 1307 ca.uhn.fhir.jpa.rp.r4b.ConsentResourceProvider retVal; 1308 retVal = new ca.uhn.fhir.jpa.rp.r4b.ConsentResourceProvider(); 1309 retVal.setContext(myFhirContext); 1310 retVal.setDao(daoConsentR4B()); 1311 return retVal; 1312 } 1313 1314 @Bean(name="myContractDaoR4B") 1315 public 1316 IFhirResourceDao<org.hl7.fhir.r4b.model.Contract> 1317 daoContractR4B() { 1318 1319 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Contract> retVal; 1320 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Contract>(); 1321 retVal.setResourceType(org.hl7.fhir.r4b.model.Contract.class); 1322 retVal.setContext(myFhirContext); 1323 return retVal; 1324 } 1325 1326 @Bean(name="myContractRpR4B") 1327 @Lazy 1328 public ca.uhn.fhir.jpa.rp.r4b.ContractResourceProvider rpContractR4B() { 1329 ca.uhn.fhir.jpa.rp.r4b.ContractResourceProvider retVal; 1330 retVal = new ca.uhn.fhir.jpa.rp.r4b.ContractResourceProvider(); 1331 retVal.setContext(myFhirContext); 1332 retVal.setDao(daoContractR4B()); 1333 return retVal; 1334 } 1335 1336 @Bean(name="myCoverageDaoR4B") 1337 public 1338 IFhirResourceDao<org.hl7.fhir.r4b.model.Coverage> 1339 daoCoverageR4B() { 1340 1341 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Coverage> retVal; 1342 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Coverage>(); 1343 retVal.setResourceType(org.hl7.fhir.r4b.model.Coverage.class); 1344 retVal.setContext(myFhirContext); 1345 return retVal; 1346 } 1347 1348 @Bean(name="myCoverageRpR4B") 1349 @Lazy 1350 public ca.uhn.fhir.jpa.rp.r4b.CoverageResourceProvider rpCoverageR4B() { 1351 ca.uhn.fhir.jpa.rp.r4b.CoverageResourceProvider retVal; 1352 retVal = new ca.uhn.fhir.jpa.rp.r4b.CoverageResourceProvider(); 1353 retVal.setContext(myFhirContext); 1354 retVal.setDao(daoCoverageR4B()); 1355 return retVal; 1356 } 1357 1358 @Bean(name="myCoverageEligibilityRequestDaoR4B") 1359 public 1360 IFhirResourceDao<org.hl7.fhir.r4b.model.CoverageEligibilityRequest> 1361 daoCoverageEligibilityRequestR4B() { 1362 1363 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.CoverageEligibilityRequest> retVal; 1364 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.CoverageEligibilityRequest>(); 1365 retVal.setResourceType(org.hl7.fhir.r4b.model.CoverageEligibilityRequest.class); 1366 retVal.setContext(myFhirContext); 1367 return retVal; 1368 } 1369 1370 @Bean(name="myCoverageEligibilityRequestRpR4B") 1371 @Lazy 1372 public ca.uhn.fhir.jpa.rp.r4b.CoverageEligibilityRequestResourceProvider rpCoverageEligibilityRequestR4B() { 1373 ca.uhn.fhir.jpa.rp.r4b.CoverageEligibilityRequestResourceProvider retVal; 1374 retVal = new ca.uhn.fhir.jpa.rp.r4b.CoverageEligibilityRequestResourceProvider(); 1375 retVal.setContext(myFhirContext); 1376 retVal.setDao(daoCoverageEligibilityRequestR4B()); 1377 return retVal; 1378 } 1379 1380 @Bean(name="myCoverageEligibilityResponseDaoR4B") 1381 public 1382 IFhirResourceDao<org.hl7.fhir.r4b.model.CoverageEligibilityResponse> 1383 daoCoverageEligibilityResponseR4B() { 1384 1385 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.CoverageEligibilityResponse> retVal; 1386 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.CoverageEligibilityResponse>(); 1387 retVal.setResourceType(org.hl7.fhir.r4b.model.CoverageEligibilityResponse.class); 1388 retVal.setContext(myFhirContext); 1389 return retVal; 1390 } 1391 1392 @Bean(name="myCoverageEligibilityResponseRpR4B") 1393 @Lazy 1394 public ca.uhn.fhir.jpa.rp.r4b.CoverageEligibilityResponseResourceProvider rpCoverageEligibilityResponseR4B() { 1395 ca.uhn.fhir.jpa.rp.r4b.CoverageEligibilityResponseResourceProvider retVal; 1396 retVal = new ca.uhn.fhir.jpa.rp.r4b.CoverageEligibilityResponseResourceProvider(); 1397 retVal.setContext(myFhirContext); 1398 retVal.setDao(daoCoverageEligibilityResponseR4B()); 1399 return retVal; 1400 } 1401 1402 @Bean(name="myDetectedIssueDaoR4B") 1403 public 1404 IFhirResourceDao<org.hl7.fhir.r4b.model.DetectedIssue> 1405 daoDetectedIssueR4B() { 1406 1407 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.DetectedIssue> retVal; 1408 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.DetectedIssue>(); 1409 retVal.setResourceType(org.hl7.fhir.r4b.model.DetectedIssue.class); 1410 retVal.setContext(myFhirContext); 1411 return retVal; 1412 } 1413 1414 @Bean(name="myDetectedIssueRpR4B") 1415 @Lazy 1416 public ca.uhn.fhir.jpa.rp.r4b.DetectedIssueResourceProvider rpDetectedIssueR4B() { 1417 ca.uhn.fhir.jpa.rp.r4b.DetectedIssueResourceProvider retVal; 1418 retVal = new ca.uhn.fhir.jpa.rp.r4b.DetectedIssueResourceProvider(); 1419 retVal.setContext(myFhirContext); 1420 retVal.setDao(daoDetectedIssueR4B()); 1421 return retVal; 1422 } 1423 1424 @Bean(name="myDeviceDaoR4B") 1425 public 1426 IFhirResourceDao<org.hl7.fhir.r4b.model.Device> 1427 daoDeviceR4B() { 1428 1429 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Device> retVal; 1430 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Device>(); 1431 retVal.setResourceType(org.hl7.fhir.r4b.model.Device.class); 1432 retVal.setContext(myFhirContext); 1433 return retVal; 1434 } 1435 1436 @Bean(name="myDeviceRpR4B") 1437 @Lazy 1438 public ca.uhn.fhir.jpa.rp.r4b.DeviceResourceProvider rpDeviceR4B() { 1439 ca.uhn.fhir.jpa.rp.r4b.DeviceResourceProvider retVal; 1440 retVal = new ca.uhn.fhir.jpa.rp.r4b.DeviceResourceProvider(); 1441 retVal.setContext(myFhirContext); 1442 retVal.setDao(daoDeviceR4B()); 1443 return retVal; 1444 } 1445 1446 @Bean(name="myDeviceDefinitionDaoR4B") 1447 public 1448 IFhirResourceDao<org.hl7.fhir.r4b.model.DeviceDefinition> 1449 daoDeviceDefinitionR4B() { 1450 1451 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.DeviceDefinition> retVal; 1452 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.DeviceDefinition>(); 1453 retVal.setResourceType(org.hl7.fhir.r4b.model.DeviceDefinition.class); 1454 retVal.setContext(myFhirContext); 1455 return retVal; 1456 } 1457 1458 @Bean(name="myDeviceDefinitionRpR4B") 1459 @Lazy 1460 public ca.uhn.fhir.jpa.rp.r4b.DeviceDefinitionResourceProvider rpDeviceDefinitionR4B() { 1461 ca.uhn.fhir.jpa.rp.r4b.DeviceDefinitionResourceProvider retVal; 1462 retVal = new ca.uhn.fhir.jpa.rp.r4b.DeviceDefinitionResourceProvider(); 1463 retVal.setContext(myFhirContext); 1464 retVal.setDao(daoDeviceDefinitionR4B()); 1465 return retVal; 1466 } 1467 1468 @Bean(name="myDeviceMetricDaoR4B") 1469 public 1470 IFhirResourceDao<org.hl7.fhir.r4b.model.DeviceMetric> 1471 daoDeviceMetricR4B() { 1472 1473 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.DeviceMetric> retVal; 1474 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.DeviceMetric>(); 1475 retVal.setResourceType(org.hl7.fhir.r4b.model.DeviceMetric.class); 1476 retVal.setContext(myFhirContext); 1477 return retVal; 1478 } 1479 1480 @Bean(name="myDeviceMetricRpR4B") 1481 @Lazy 1482 public ca.uhn.fhir.jpa.rp.r4b.DeviceMetricResourceProvider rpDeviceMetricR4B() { 1483 ca.uhn.fhir.jpa.rp.r4b.DeviceMetricResourceProvider retVal; 1484 retVal = new ca.uhn.fhir.jpa.rp.r4b.DeviceMetricResourceProvider(); 1485 retVal.setContext(myFhirContext); 1486 retVal.setDao(daoDeviceMetricR4B()); 1487 return retVal; 1488 } 1489 1490 @Bean(name="myDeviceRequestDaoR4B") 1491 public 1492 IFhirResourceDao<org.hl7.fhir.r4b.model.DeviceRequest> 1493 daoDeviceRequestR4B() { 1494 1495 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.DeviceRequest> retVal; 1496 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.DeviceRequest>(); 1497 retVal.setResourceType(org.hl7.fhir.r4b.model.DeviceRequest.class); 1498 retVal.setContext(myFhirContext); 1499 return retVal; 1500 } 1501 1502 @Bean(name="myDeviceRequestRpR4B") 1503 @Lazy 1504 public ca.uhn.fhir.jpa.rp.r4b.DeviceRequestResourceProvider rpDeviceRequestR4B() { 1505 ca.uhn.fhir.jpa.rp.r4b.DeviceRequestResourceProvider retVal; 1506 retVal = new ca.uhn.fhir.jpa.rp.r4b.DeviceRequestResourceProvider(); 1507 retVal.setContext(myFhirContext); 1508 retVal.setDao(daoDeviceRequestR4B()); 1509 return retVal; 1510 } 1511 1512 @Bean(name="myDeviceUseStatementDaoR4B") 1513 public 1514 IFhirResourceDao<org.hl7.fhir.r4b.model.DeviceUseStatement> 1515 daoDeviceUseStatementR4B() { 1516 1517 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.DeviceUseStatement> retVal; 1518 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.DeviceUseStatement>(); 1519 retVal.setResourceType(org.hl7.fhir.r4b.model.DeviceUseStatement.class); 1520 retVal.setContext(myFhirContext); 1521 return retVal; 1522 } 1523 1524 @Bean(name="myDeviceUseStatementRpR4B") 1525 @Lazy 1526 public ca.uhn.fhir.jpa.rp.r4b.DeviceUseStatementResourceProvider rpDeviceUseStatementR4B() { 1527 ca.uhn.fhir.jpa.rp.r4b.DeviceUseStatementResourceProvider retVal; 1528 retVal = new ca.uhn.fhir.jpa.rp.r4b.DeviceUseStatementResourceProvider(); 1529 retVal.setContext(myFhirContext); 1530 retVal.setDao(daoDeviceUseStatementR4B()); 1531 return retVal; 1532 } 1533 1534 @Bean(name="myDiagnosticReportDaoR4B") 1535 public 1536 IFhirResourceDao<org.hl7.fhir.r4b.model.DiagnosticReport> 1537 daoDiagnosticReportR4B() { 1538 1539 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.DiagnosticReport> retVal; 1540 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.DiagnosticReport>(); 1541 retVal.setResourceType(org.hl7.fhir.r4b.model.DiagnosticReport.class); 1542 retVal.setContext(myFhirContext); 1543 return retVal; 1544 } 1545 1546 @Bean(name="myDiagnosticReportRpR4B") 1547 @Lazy 1548 public ca.uhn.fhir.jpa.rp.r4b.DiagnosticReportResourceProvider rpDiagnosticReportR4B() { 1549 ca.uhn.fhir.jpa.rp.r4b.DiagnosticReportResourceProvider retVal; 1550 retVal = new ca.uhn.fhir.jpa.rp.r4b.DiagnosticReportResourceProvider(); 1551 retVal.setContext(myFhirContext); 1552 retVal.setDao(daoDiagnosticReportR4B()); 1553 return retVal; 1554 } 1555 1556 @Bean(name="myDocumentManifestDaoR4B") 1557 public 1558 IFhirResourceDao<org.hl7.fhir.r4b.model.DocumentManifest> 1559 daoDocumentManifestR4B() { 1560 1561 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.DocumentManifest> retVal; 1562 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.DocumentManifest>(); 1563 retVal.setResourceType(org.hl7.fhir.r4b.model.DocumentManifest.class); 1564 retVal.setContext(myFhirContext); 1565 return retVal; 1566 } 1567 1568 @Bean(name="myDocumentManifestRpR4B") 1569 @Lazy 1570 public ca.uhn.fhir.jpa.rp.r4b.DocumentManifestResourceProvider rpDocumentManifestR4B() { 1571 ca.uhn.fhir.jpa.rp.r4b.DocumentManifestResourceProvider retVal; 1572 retVal = new ca.uhn.fhir.jpa.rp.r4b.DocumentManifestResourceProvider(); 1573 retVal.setContext(myFhirContext); 1574 retVal.setDao(daoDocumentManifestR4B()); 1575 return retVal; 1576 } 1577 1578 @Bean(name="myDocumentReferenceDaoR4B") 1579 public 1580 IFhirResourceDao<org.hl7.fhir.r4b.model.DocumentReference> 1581 daoDocumentReferenceR4B() { 1582 1583 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.DocumentReference> retVal; 1584 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.DocumentReference>(); 1585 retVal.setResourceType(org.hl7.fhir.r4b.model.DocumentReference.class); 1586 retVal.setContext(myFhirContext); 1587 return retVal; 1588 } 1589 1590 @Bean(name="myDocumentReferenceRpR4B") 1591 @Lazy 1592 public ca.uhn.fhir.jpa.rp.r4b.DocumentReferenceResourceProvider rpDocumentReferenceR4B() { 1593 ca.uhn.fhir.jpa.rp.r4b.DocumentReferenceResourceProvider retVal; 1594 retVal = new ca.uhn.fhir.jpa.rp.r4b.DocumentReferenceResourceProvider(); 1595 retVal.setContext(myFhirContext); 1596 retVal.setDao(daoDocumentReferenceR4B()); 1597 return retVal; 1598 } 1599 1600 @Bean(name="myEncounterDaoR4B") 1601 public 1602 IFhirResourceDaoEncounter<org.hl7.fhir.r4b.model.Encounter> 1603 daoEncounterR4B() { 1604 1605 ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<org.hl7.fhir.r4b.model.Encounter> retVal; 1606 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<>(); 1607 retVal.setResourceType(org.hl7.fhir.r4b.model.Encounter.class); 1608 retVal.setContext(myFhirContext); 1609 return retVal; 1610 } 1611 1612 @Bean(name="myEncounterRpR4B") 1613 @Lazy 1614 public ca.uhn.fhir.jpa.rp.r4b.EncounterResourceProvider rpEncounterR4B() { 1615 ca.uhn.fhir.jpa.rp.r4b.EncounterResourceProvider retVal; 1616 retVal = new ca.uhn.fhir.jpa.rp.r4b.EncounterResourceProvider(); 1617 retVal.setContext(myFhirContext); 1618 retVal.setDao(daoEncounterR4B()); 1619 return retVal; 1620 } 1621 1622 @Bean(name="myEndpointDaoR4B") 1623 public 1624 IFhirResourceDao<org.hl7.fhir.r4b.model.Endpoint> 1625 daoEndpointR4B() { 1626 1627 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Endpoint> retVal; 1628 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Endpoint>(); 1629 retVal.setResourceType(org.hl7.fhir.r4b.model.Endpoint.class); 1630 retVal.setContext(myFhirContext); 1631 return retVal; 1632 } 1633 1634 @Bean(name="myEndpointRpR4B") 1635 @Lazy 1636 public ca.uhn.fhir.jpa.rp.r4b.EndpointResourceProvider rpEndpointR4B() { 1637 ca.uhn.fhir.jpa.rp.r4b.EndpointResourceProvider retVal; 1638 retVal = new ca.uhn.fhir.jpa.rp.r4b.EndpointResourceProvider(); 1639 retVal.setContext(myFhirContext); 1640 retVal.setDao(daoEndpointR4B()); 1641 return retVal; 1642 } 1643 1644 @Bean(name="myEnrollmentRequestDaoR4B") 1645 public 1646 IFhirResourceDao<org.hl7.fhir.r4b.model.EnrollmentRequest> 1647 daoEnrollmentRequestR4B() { 1648 1649 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.EnrollmentRequest> retVal; 1650 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.EnrollmentRequest>(); 1651 retVal.setResourceType(org.hl7.fhir.r4b.model.EnrollmentRequest.class); 1652 retVal.setContext(myFhirContext); 1653 return retVal; 1654 } 1655 1656 @Bean(name="myEnrollmentRequestRpR4B") 1657 @Lazy 1658 public ca.uhn.fhir.jpa.rp.r4b.EnrollmentRequestResourceProvider rpEnrollmentRequestR4B() { 1659 ca.uhn.fhir.jpa.rp.r4b.EnrollmentRequestResourceProvider retVal; 1660 retVal = new ca.uhn.fhir.jpa.rp.r4b.EnrollmentRequestResourceProvider(); 1661 retVal.setContext(myFhirContext); 1662 retVal.setDao(daoEnrollmentRequestR4B()); 1663 return retVal; 1664 } 1665 1666 @Bean(name="myEnrollmentResponseDaoR4B") 1667 public 1668 IFhirResourceDao<org.hl7.fhir.r4b.model.EnrollmentResponse> 1669 daoEnrollmentResponseR4B() { 1670 1671 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.EnrollmentResponse> retVal; 1672 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.EnrollmentResponse>(); 1673 retVal.setResourceType(org.hl7.fhir.r4b.model.EnrollmentResponse.class); 1674 retVal.setContext(myFhirContext); 1675 return retVal; 1676 } 1677 1678 @Bean(name="myEnrollmentResponseRpR4B") 1679 @Lazy 1680 public ca.uhn.fhir.jpa.rp.r4b.EnrollmentResponseResourceProvider rpEnrollmentResponseR4B() { 1681 ca.uhn.fhir.jpa.rp.r4b.EnrollmentResponseResourceProvider retVal; 1682 retVal = new ca.uhn.fhir.jpa.rp.r4b.EnrollmentResponseResourceProvider(); 1683 retVal.setContext(myFhirContext); 1684 retVal.setDao(daoEnrollmentResponseR4B()); 1685 return retVal; 1686 } 1687 1688 @Bean(name="myEpisodeOfCareDaoR4B") 1689 public 1690 IFhirResourceDao<org.hl7.fhir.r4b.model.EpisodeOfCare> 1691 daoEpisodeOfCareR4B() { 1692 1693 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.EpisodeOfCare> retVal; 1694 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.EpisodeOfCare>(); 1695 retVal.setResourceType(org.hl7.fhir.r4b.model.EpisodeOfCare.class); 1696 retVal.setContext(myFhirContext); 1697 return retVal; 1698 } 1699 1700 @Bean(name="myEpisodeOfCareRpR4B") 1701 @Lazy 1702 public ca.uhn.fhir.jpa.rp.r4b.EpisodeOfCareResourceProvider rpEpisodeOfCareR4B() { 1703 ca.uhn.fhir.jpa.rp.r4b.EpisodeOfCareResourceProvider retVal; 1704 retVal = new ca.uhn.fhir.jpa.rp.r4b.EpisodeOfCareResourceProvider(); 1705 retVal.setContext(myFhirContext); 1706 retVal.setDao(daoEpisodeOfCareR4B()); 1707 return retVal; 1708 } 1709 1710 @Bean(name="myEventDefinitionDaoR4B") 1711 public 1712 IFhirResourceDao<org.hl7.fhir.r4b.model.EventDefinition> 1713 daoEventDefinitionR4B() { 1714 1715 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.EventDefinition> retVal; 1716 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.EventDefinition>(); 1717 retVal.setResourceType(org.hl7.fhir.r4b.model.EventDefinition.class); 1718 retVal.setContext(myFhirContext); 1719 return retVal; 1720 } 1721 1722 @Bean(name="myEventDefinitionRpR4B") 1723 @Lazy 1724 public ca.uhn.fhir.jpa.rp.r4b.EventDefinitionResourceProvider rpEventDefinitionR4B() { 1725 ca.uhn.fhir.jpa.rp.r4b.EventDefinitionResourceProvider retVal; 1726 retVal = new ca.uhn.fhir.jpa.rp.r4b.EventDefinitionResourceProvider(); 1727 retVal.setContext(myFhirContext); 1728 retVal.setDao(daoEventDefinitionR4B()); 1729 return retVal; 1730 } 1731 1732 @Bean(name="myEvidenceDaoR4B") 1733 public 1734 IFhirResourceDao<org.hl7.fhir.r4b.model.Evidence> 1735 daoEvidenceR4B() { 1736 1737 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Evidence> retVal; 1738 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Evidence>(); 1739 retVal.setResourceType(org.hl7.fhir.r4b.model.Evidence.class); 1740 retVal.setContext(myFhirContext); 1741 return retVal; 1742 } 1743 1744 @Bean(name="myEvidenceRpR4B") 1745 @Lazy 1746 public ca.uhn.fhir.jpa.rp.r4b.EvidenceResourceProvider rpEvidenceR4B() { 1747 ca.uhn.fhir.jpa.rp.r4b.EvidenceResourceProvider retVal; 1748 retVal = new ca.uhn.fhir.jpa.rp.r4b.EvidenceResourceProvider(); 1749 retVal.setContext(myFhirContext); 1750 retVal.setDao(daoEvidenceR4B()); 1751 return retVal; 1752 } 1753 1754 @Bean(name="myEvidenceReportDaoR4B") 1755 public 1756 IFhirResourceDao<org.hl7.fhir.r4b.model.EvidenceReport> 1757 daoEvidenceReportR4B() { 1758 1759 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.EvidenceReport> retVal; 1760 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.EvidenceReport>(); 1761 retVal.setResourceType(org.hl7.fhir.r4b.model.EvidenceReport.class); 1762 retVal.setContext(myFhirContext); 1763 return retVal; 1764 } 1765 1766 @Bean(name="myEvidenceReportRpR4B") 1767 @Lazy 1768 public ca.uhn.fhir.jpa.rp.r4b.EvidenceReportResourceProvider rpEvidenceReportR4B() { 1769 ca.uhn.fhir.jpa.rp.r4b.EvidenceReportResourceProvider retVal; 1770 retVal = new ca.uhn.fhir.jpa.rp.r4b.EvidenceReportResourceProvider(); 1771 retVal.setContext(myFhirContext); 1772 retVal.setDao(daoEvidenceReportR4B()); 1773 return retVal; 1774 } 1775 1776 @Bean(name="myEvidenceVariableDaoR4B") 1777 public 1778 IFhirResourceDao<org.hl7.fhir.r4b.model.EvidenceVariable> 1779 daoEvidenceVariableR4B() { 1780 1781 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.EvidenceVariable> retVal; 1782 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.EvidenceVariable>(); 1783 retVal.setResourceType(org.hl7.fhir.r4b.model.EvidenceVariable.class); 1784 retVal.setContext(myFhirContext); 1785 return retVal; 1786 } 1787 1788 @Bean(name="myEvidenceVariableRpR4B") 1789 @Lazy 1790 public ca.uhn.fhir.jpa.rp.r4b.EvidenceVariableResourceProvider rpEvidenceVariableR4B() { 1791 ca.uhn.fhir.jpa.rp.r4b.EvidenceVariableResourceProvider retVal; 1792 retVal = new ca.uhn.fhir.jpa.rp.r4b.EvidenceVariableResourceProvider(); 1793 retVal.setContext(myFhirContext); 1794 retVal.setDao(daoEvidenceVariableR4B()); 1795 return retVal; 1796 } 1797 1798 @Bean(name="myExampleScenarioDaoR4B") 1799 public 1800 IFhirResourceDao<org.hl7.fhir.r4b.model.ExampleScenario> 1801 daoExampleScenarioR4B() { 1802 1803 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ExampleScenario> retVal; 1804 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ExampleScenario>(); 1805 retVal.setResourceType(org.hl7.fhir.r4b.model.ExampleScenario.class); 1806 retVal.setContext(myFhirContext); 1807 return retVal; 1808 } 1809 1810 @Bean(name="myExampleScenarioRpR4B") 1811 @Lazy 1812 public ca.uhn.fhir.jpa.rp.r4b.ExampleScenarioResourceProvider rpExampleScenarioR4B() { 1813 ca.uhn.fhir.jpa.rp.r4b.ExampleScenarioResourceProvider retVal; 1814 retVal = new ca.uhn.fhir.jpa.rp.r4b.ExampleScenarioResourceProvider(); 1815 retVal.setContext(myFhirContext); 1816 retVal.setDao(daoExampleScenarioR4B()); 1817 return retVal; 1818 } 1819 1820 @Bean(name="myExplanationOfBenefitDaoR4B") 1821 public 1822 IFhirResourceDao<org.hl7.fhir.r4b.model.ExplanationOfBenefit> 1823 daoExplanationOfBenefitR4B() { 1824 1825 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ExplanationOfBenefit> retVal; 1826 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ExplanationOfBenefit>(); 1827 retVal.setResourceType(org.hl7.fhir.r4b.model.ExplanationOfBenefit.class); 1828 retVal.setContext(myFhirContext); 1829 return retVal; 1830 } 1831 1832 @Bean(name="myExplanationOfBenefitRpR4B") 1833 @Lazy 1834 public ca.uhn.fhir.jpa.rp.r4b.ExplanationOfBenefitResourceProvider rpExplanationOfBenefitR4B() { 1835 ca.uhn.fhir.jpa.rp.r4b.ExplanationOfBenefitResourceProvider retVal; 1836 retVal = new ca.uhn.fhir.jpa.rp.r4b.ExplanationOfBenefitResourceProvider(); 1837 retVal.setContext(myFhirContext); 1838 retVal.setDao(daoExplanationOfBenefitR4B()); 1839 return retVal; 1840 } 1841 1842 @Bean(name="myFamilyMemberHistoryDaoR4B") 1843 public 1844 IFhirResourceDao<org.hl7.fhir.r4b.model.FamilyMemberHistory> 1845 daoFamilyMemberHistoryR4B() { 1846 1847 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.FamilyMemberHistory> retVal; 1848 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.FamilyMemberHistory>(); 1849 retVal.setResourceType(org.hl7.fhir.r4b.model.FamilyMemberHistory.class); 1850 retVal.setContext(myFhirContext); 1851 return retVal; 1852 } 1853 1854 @Bean(name="myFamilyMemberHistoryRpR4B") 1855 @Lazy 1856 public ca.uhn.fhir.jpa.rp.r4b.FamilyMemberHistoryResourceProvider rpFamilyMemberHistoryR4B() { 1857 ca.uhn.fhir.jpa.rp.r4b.FamilyMemberHistoryResourceProvider retVal; 1858 retVal = new ca.uhn.fhir.jpa.rp.r4b.FamilyMemberHistoryResourceProvider(); 1859 retVal.setContext(myFhirContext); 1860 retVal.setDao(daoFamilyMemberHistoryR4B()); 1861 return retVal; 1862 } 1863 1864 @Bean(name="myFlagDaoR4B") 1865 public 1866 IFhirResourceDao<org.hl7.fhir.r4b.model.Flag> 1867 daoFlagR4B() { 1868 1869 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Flag> retVal; 1870 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Flag>(); 1871 retVal.setResourceType(org.hl7.fhir.r4b.model.Flag.class); 1872 retVal.setContext(myFhirContext); 1873 return retVal; 1874 } 1875 1876 @Bean(name="myFlagRpR4B") 1877 @Lazy 1878 public ca.uhn.fhir.jpa.rp.r4b.FlagResourceProvider rpFlagR4B() { 1879 ca.uhn.fhir.jpa.rp.r4b.FlagResourceProvider retVal; 1880 retVal = new ca.uhn.fhir.jpa.rp.r4b.FlagResourceProvider(); 1881 retVal.setContext(myFhirContext); 1882 retVal.setDao(daoFlagR4B()); 1883 return retVal; 1884 } 1885 1886 @Bean(name="myGoalDaoR4B") 1887 public 1888 IFhirResourceDao<org.hl7.fhir.r4b.model.Goal> 1889 daoGoalR4B() { 1890 1891 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Goal> retVal; 1892 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Goal>(); 1893 retVal.setResourceType(org.hl7.fhir.r4b.model.Goal.class); 1894 retVal.setContext(myFhirContext); 1895 return retVal; 1896 } 1897 1898 @Bean(name="myGoalRpR4B") 1899 @Lazy 1900 public ca.uhn.fhir.jpa.rp.r4b.GoalResourceProvider rpGoalR4B() { 1901 ca.uhn.fhir.jpa.rp.r4b.GoalResourceProvider retVal; 1902 retVal = new ca.uhn.fhir.jpa.rp.r4b.GoalResourceProvider(); 1903 retVal.setContext(myFhirContext); 1904 retVal.setDao(daoGoalR4B()); 1905 return retVal; 1906 } 1907 1908 @Bean(name="myGraphDefinitionDaoR4B") 1909 public 1910 IFhirResourceDao<org.hl7.fhir.r4b.model.GraphDefinition> 1911 daoGraphDefinitionR4B() { 1912 1913 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.GraphDefinition> retVal; 1914 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.GraphDefinition>(); 1915 retVal.setResourceType(org.hl7.fhir.r4b.model.GraphDefinition.class); 1916 retVal.setContext(myFhirContext); 1917 return retVal; 1918 } 1919 1920 @Bean(name="myGraphDefinitionRpR4B") 1921 @Lazy 1922 public ca.uhn.fhir.jpa.rp.r4b.GraphDefinitionResourceProvider rpGraphDefinitionR4B() { 1923 ca.uhn.fhir.jpa.rp.r4b.GraphDefinitionResourceProvider retVal; 1924 retVal = new ca.uhn.fhir.jpa.rp.r4b.GraphDefinitionResourceProvider(); 1925 retVal.setContext(myFhirContext); 1926 retVal.setDao(daoGraphDefinitionR4B()); 1927 return retVal; 1928 } 1929 1930 @Bean(name="myGroupDaoR4B") 1931 public 1932 IFhirResourceDao<org.hl7.fhir.r4b.model.Group> 1933 daoGroupR4B() { 1934 1935 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Group> retVal; 1936 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Group>(); 1937 retVal.setResourceType(org.hl7.fhir.r4b.model.Group.class); 1938 retVal.setContext(myFhirContext); 1939 return retVal; 1940 } 1941 1942 @Bean(name="myGroupRpR4B") 1943 @Lazy 1944 public ca.uhn.fhir.jpa.rp.r4b.GroupResourceProvider rpGroupR4B() { 1945 ca.uhn.fhir.jpa.rp.r4b.GroupResourceProvider retVal; 1946 retVal = new ca.uhn.fhir.jpa.rp.r4b.GroupResourceProvider(); 1947 retVal.setContext(myFhirContext); 1948 retVal.setDao(daoGroupR4B()); 1949 return retVal; 1950 } 1951 1952 @Bean(name="myGuidanceResponseDaoR4B") 1953 public 1954 IFhirResourceDao<org.hl7.fhir.r4b.model.GuidanceResponse> 1955 daoGuidanceResponseR4B() { 1956 1957 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.GuidanceResponse> retVal; 1958 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.GuidanceResponse>(); 1959 retVal.setResourceType(org.hl7.fhir.r4b.model.GuidanceResponse.class); 1960 retVal.setContext(myFhirContext); 1961 return retVal; 1962 } 1963 1964 @Bean(name="myGuidanceResponseRpR4B") 1965 @Lazy 1966 public ca.uhn.fhir.jpa.rp.r4b.GuidanceResponseResourceProvider rpGuidanceResponseR4B() { 1967 ca.uhn.fhir.jpa.rp.r4b.GuidanceResponseResourceProvider retVal; 1968 retVal = new ca.uhn.fhir.jpa.rp.r4b.GuidanceResponseResourceProvider(); 1969 retVal.setContext(myFhirContext); 1970 retVal.setDao(daoGuidanceResponseR4B()); 1971 return retVal; 1972 } 1973 1974 @Bean(name="myHealthcareServiceDaoR4B") 1975 public 1976 IFhirResourceDao<org.hl7.fhir.r4b.model.HealthcareService> 1977 daoHealthcareServiceR4B() { 1978 1979 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.HealthcareService> retVal; 1980 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.HealthcareService>(); 1981 retVal.setResourceType(org.hl7.fhir.r4b.model.HealthcareService.class); 1982 retVal.setContext(myFhirContext); 1983 return retVal; 1984 } 1985 1986 @Bean(name="myHealthcareServiceRpR4B") 1987 @Lazy 1988 public ca.uhn.fhir.jpa.rp.r4b.HealthcareServiceResourceProvider rpHealthcareServiceR4B() { 1989 ca.uhn.fhir.jpa.rp.r4b.HealthcareServiceResourceProvider retVal; 1990 retVal = new ca.uhn.fhir.jpa.rp.r4b.HealthcareServiceResourceProvider(); 1991 retVal.setContext(myFhirContext); 1992 retVal.setDao(daoHealthcareServiceR4B()); 1993 return retVal; 1994 } 1995 1996 @Bean(name="myImagingStudyDaoR4B") 1997 public 1998 IFhirResourceDao<org.hl7.fhir.r4b.model.ImagingStudy> 1999 daoImagingStudyR4B() { 2000 2001 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ImagingStudy> retVal; 2002 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ImagingStudy>(); 2003 retVal.setResourceType(org.hl7.fhir.r4b.model.ImagingStudy.class); 2004 retVal.setContext(myFhirContext); 2005 return retVal; 2006 } 2007 2008 @Bean(name="myImagingStudyRpR4B") 2009 @Lazy 2010 public ca.uhn.fhir.jpa.rp.r4b.ImagingStudyResourceProvider rpImagingStudyR4B() { 2011 ca.uhn.fhir.jpa.rp.r4b.ImagingStudyResourceProvider retVal; 2012 retVal = new ca.uhn.fhir.jpa.rp.r4b.ImagingStudyResourceProvider(); 2013 retVal.setContext(myFhirContext); 2014 retVal.setDao(daoImagingStudyR4B()); 2015 return retVal; 2016 } 2017 2018 @Bean(name="myImmunizationDaoR4B") 2019 public 2020 IFhirResourceDao<org.hl7.fhir.r4b.model.Immunization> 2021 daoImmunizationR4B() { 2022 2023 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Immunization> retVal; 2024 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Immunization>(); 2025 retVal.setResourceType(org.hl7.fhir.r4b.model.Immunization.class); 2026 retVal.setContext(myFhirContext); 2027 return retVal; 2028 } 2029 2030 @Bean(name="myImmunizationRpR4B") 2031 @Lazy 2032 public ca.uhn.fhir.jpa.rp.r4b.ImmunizationResourceProvider rpImmunizationR4B() { 2033 ca.uhn.fhir.jpa.rp.r4b.ImmunizationResourceProvider retVal; 2034 retVal = new ca.uhn.fhir.jpa.rp.r4b.ImmunizationResourceProvider(); 2035 retVal.setContext(myFhirContext); 2036 retVal.setDao(daoImmunizationR4B()); 2037 return retVal; 2038 } 2039 2040 @Bean(name="myImmunizationEvaluationDaoR4B") 2041 public 2042 IFhirResourceDao<org.hl7.fhir.r4b.model.ImmunizationEvaluation> 2043 daoImmunizationEvaluationR4B() { 2044 2045 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ImmunizationEvaluation> retVal; 2046 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ImmunizationEvaluation>(); 2047 retVal.setResourceType(org.hl7.fhir.r4b.model.ImmunizationEvaluation.class); 2048 retVal.setContext(myFhirContext); 2049 return retVal; 2050 } 2051 2052 @Bean(name="myImmunizationEvaluationRpR4B") 2053 @Lazy 2054 public ca.uhn.fhir.jpa.rp.r4b.ImmunizationEvaluationResourceProvider rpImmunizationEvaluationR4B() { 2055 ca.uhn.fhir.jpa.rp.r4b.ImmunizationEvaluationResourceProvider retVal; 2056 retVal = new ca.uhn.fhir.jpa.rp.r4b.ImmunizationEvaluationResourceProvider(); 2057 retVal.setContext(myFhirContext); 2058 retVal.setDao(daoImmunizationEvaluationR4B()); 2059 return retVal; 2060 } 2061 2062 @Bean(name="myImmunizationRecommendationDaoR4B") 2063 public 2064 IFhirResourceDao<org.hl7.fhir.r4b.model.ImmunizationRecommendation> 2065 daoImmunizationRecommendationR4B() { 2066 2067 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ImmunizationRecommendation> retVal; 2068 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ImmunizationRecommendation>(); 2069 retVal.setResourceType(org.hl7.fhir.r4b.model.ImmunizationRecommendation.class); 2070 retVal.setContext(myFhirContext); 2071 return retVal; 2072 } 2073 2074 @Bean(name="myImmunizationRecommendationRpR4B") 2075 @Lazy 2076 public ca.uhn.fhir.jpa.rp.r4b.ImmunizationRecommendationResourceProvider rpImmunizationRecommendationR4B() { 2077 ca.uhn.fhir.jpa.rp.r4b.ImmunizationRecommendationResourceProvider retVal; 2078 retVal = new ca.uhn.fhir.jpa.rp.r4b.ImmunizationRecommendationResourceProvider(); 2079 retVal.setContext(myFhirContext); 2080 retVal.setDao(daoImmunizationRecommendationR4B()); 2081 return retVal; 2082 } 2083 2084 @Bean(name="myImplementationGuideDaoR4B") 2085 public 2086 IFhirResourceDao<org.hl7.fhir.r4b.model.ImplementationGuide> 2087 daoImplementationGuideR4B() { 2088 2089 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ImplementationGuide> retVal; 2090 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ImplementationGuide>(); 2091 retVal.setResourceType(org.hl7.fhir.r4b.model.ImplementationGuide.class); 2092 retVal.setContext(myFhirContext); 2093 return retVal; 2094 } 2095 2096 @Bean(name="myImplementationGuideRpR4B") 2097 @Lazy 2098 public ca.uhn.fhir.jpa.rp.r4b.ImplementationGuideResourceProvider rpImplementationGuideR4B() { 2099 ca.uhn.fhir.jpa.rp.r4b.ImplementationGuideResourceProvider retVal; 2100 retVal = new ca.uhn.fhir.jpa.rp.r4b.ImplementationGuideResourceProvider(); 2101 retVal.setContext(myFhirContext); 2102 retVal.setDao(daoImplementationGuideR4B()); 2103 return retVal; 2104 } 2105 2106 @Bean(name="myIngredientDaoR4B") 2107 public 2108 IFhirResourceDao<org.hl7.fhir.r4b.model.Ingredient> 2109 daoIngredientR4B() { 2110 2111 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Ingredient> retVal; 2112 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Ingredient>(); 2113 retVal.setResourceType(org.hl7.fhir.r4b.model.Ingredient.class); 2114 retVal.setContext(myFhirContext); 2115 return retVal; 2116 } 2117 2118 @Bean(name="myIngredientRpR4B") 2119 @Lazy 2120 public ca.uhn.fhir.jpa.rp.r4b.IngredientResourceProvider rpIngredientR4B() { 2121 ca.uhn.fhir.jpa.rp.r4b.IngredientResourceProvider retVal; 2122 retVal = new ca.uhn.fhir.jpa.rp.r4b.IngredientResourceProvider(); 2123 retVal.setContext(myFhirContext); 2124 retVal.setDao(daoIngredientR4B()); 2125 return retVal; 2126 } 2127 2128 @Bean(name="myInsurancePlanDaoR4B") 2129 public 2130 IFhirResourceDao<org.hl7.fhir.r4b.model.InsurancePlan> 2131 daoInsurancePlanR4B() { 2132 2133 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.InsurancePlan> retVal; 2134 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.InsurancePlan>(); 2135 retVal.setResourceType(org.hl7.fhir.r4b.model.InsurancePlan.class); 2136 retVal.setContext(myFhirContext); 2137 return retVal; 2138 } 2139 2140 @Bean(name="myInsurancePlanRpR4B") 2141 @Lazy 2142 public ca.uhn.fhir.jpa.rp.r4b.InsurancePlanResourceProvider rpInsurancePlanR4B() { 2143 ca.uhn.fhir.jpa.rp.r4b.InsurancePlanResourceProvider retVal; 2144 retVal = new ca.uhn.fhir.jpa.rp.r4b.InsurancePlanResourceProvider(); 2145 retVal.setContext(myFhirContext); 2146 retVal.setDao(daoInsurancePlanR4B()); 2147 return retVal; 2148 } 2149 2150 @Bean(name="myInvoiceDaoR4B") 2151 public 2152 IFhirResourceDao<org.hl7.fhir.r4b.model.Invoice> 2153 daoInvoiceR4B() { 2154 2155 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Invoice> retVal; 2156 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Invoice>(); 2157 retVal.setResourceType(org.hl7.fhir.r4b.model.Invoice.class); 2158 retVal.setContext(myFhirContext); 2159 return retVal; 2160 } 2161 2162 @Bean(name="myInvoiceRpR4B") 2163 @Lazy 2164 public ca.uhn.fhir.jpa.rp.r4b.InvoiceResourceProvider rpInvoiceR4B() { 2165 ca.uhn.fhir.jpa.rp.r4b.InvoiceResourceProvider retVal; 2166 retVal = new ca.uhn.fhir.jpa.rp.r4b.InvoiceResourceProvider(); 2167 retVal.setContext(myFhirContext); 2168 retVal.setDao(daoInvoiceR4B()); 2169 return retVal; 2170 } 2171 2172 @Bean(name="myLibraryDaoR4B") 2173 public 2174 IFhirResourceDao<org.hl7.fhir.r4b.model.Library> 2175 daoLibraryR4B() { 2176 2177 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Library> retVal; 2178 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Library>(); 2179 retVal.setResourceType(org.hl7.fhir.r4b.model.Library.class); 2180 retVal.setContext(myFhirContext); 2181 return retVal; 2182 } 2183 2184 @Bean(name="myLibraryRpR4B") 2185 @Lazy 2186 public ca.uhn.fhir.jpa.rp.r4b.LibraryResourceProvider rpLibraryR4B() { 2187 ca.uhn.fhir.jpa.rp.r4b.LibraryResourceProvider retVal; 2188 retVal = new ca.uhn.fhir.jpa.rp.r4b.LibraryResourceProvider(); 2189 retVal.setContext(myFhirContext); 2190 retVal.setDao(daoLibraryR4B()); 2191 return retVal; 2192 } 2193 2194 @Bean(name="myLinkageDaoR4B") 2195 public 2196 IFhirResourceDao<org.hl7.fhir.r4b.model.Linkage> 2197 daoLinkageR4B() { 2198 2199 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Linkage> retVal; 2200 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Linkage>(); 2201 retVal.setResourceType(org.hl7.fhir.r4b.model.Linkage.class); 2202 retVal.setContext(myFhirContext); 2203 return retVal; 2204 } 2205 2206 @Bean(name="myLinkageRpR4B") 2207 @Lazy 2208 public ca.uhn.fhir.jpa.rp.r4b.LinkageResourceProvider rpLinkageR4B() { 2209 ca.uhn.fhir.jpa.rp.r4b.LinkageResourceProvider retVal; 2210 retVal = new ca.uhn.fhir.jpa.rp.r4b.LinkageResourceProvider(); 2211 retVal.setContext(myFhirContext); 2212 retVal.setDao(daoLinkageR4B()); 2213 return retVal; 2214 } 2215 2216 @Bean(name="myListDaoR4B") 2217 public 2218 IFhirResourceDao<org.hl7.fhir.r4b.model.ListResource> 2219 daoListResourceR4B() { 2220 2221 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ListResource> retVal; 2222 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ListResource>(); 2223 retVal.setResourceType(org.hl7.fhir.r4b.model.ListResource.class); 2224 retVal.setContext(myFhirContext); 2225 return retVal; 2226 } 2227 2228 @Bean(name="myListResourceRpR4B") 2229 @Lazy 2230 public ca.uhn.fhir.jpa.rp.r4b.ListResourceResourceProvider rpListResourceR4B() { 2231 ca.uhn.fhir.jpa.rp.r4b.ListResourceResourceProvider retVal; 2232 retVal = new ca.uhn.fhir.jpa.rp.r4b.ListResourceResourceProvider(); 2233 retVal.setContext(myFhirContext); 2234 retVal.setDao(daoListResourceR4B()); 2235 return retVal; 2236 } 2237 2238 @Bean(name="myLocationDaoR4B") 2239 public 2240 IFhirResourceDao<org.hl7.fhir.r4b.model.Location> 2241 daoLocationR4B() { 2242 2243 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Location> retVal; 2244 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Location>(); 2245 retVal.setResourceType(org.hl7.fhir.r4b.model.Location.class); 2246 retVal.setContext(myFhirContext); 2247 return retVal; 2248 } 2249 2250 @Bean(name="myLocationRpR4B") 2251 @Lazy 2252 public ca.uhn.fhir.jpa.rp.r4b.LocationResourceProvider rpLocationR4B() { 2253 ca.uhn.fhir.jpa.rp.r4b.LocationResourceProvider retVal; 2254 retVal = new ca.uhn.fhir.jpa.rp.r4b.LocationResourceProvider(); 2255 retVal.setContext(myFhirContext); 2256 retVal.setDao(daoLocationR4B()); 2257 return retVal; 2258 } 2259 2260 @Bean(name="myManufacturedItemDefinitionDaoR4B") 2261 public 2262 IFhirResourceDao<org.hl7.fhir.r4b.model.ManufacturedItemDefinition> 2263 daoManufacturedItemDefinitionR4B() { 2264 2265 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ManufacturedItemDefinition> retVal; 2266 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ManufacturedItemDefinition>(); 2267 retVal.setResourceType(org.hl7.fhir.r4b.model.ManufacturedItemDefinition.class); 2268 retVal.setContext(myFhirContext); 2269 return retVal; 2270 } 2271 2272 @Bean(name="myManufacturedItemDefinitionRpR4B") 2273 @Lazy 2274 public ca.uhn.fhir.jpa.rp.r4b.ManufacturedItemDefinitionResourceProvider rpManufacturedItemDefinitionR4B() { 2275 ca.uhn.fhir.jpa.rp.r4b.ManufacturedItemDefinitionResourceProvider retVal; 2276 retVal = new ca.uhn.fhir.jpa.rp.r4b.ManufacturedItemDefinitionResourceProvider(); 2277 retVal.setContext(myFhirContext); 2278 retVal.setDao(daoManufacturedItemDefinitionR4B()); 2279 return retVal; 2280 } 2281 2282 @Bean(name="myMeasureDaoR4B") 2283 public 2284 IFhirResourceDao<org.hl7.fhir.r4b.model.Measure> 2285 daoMeasureR4B() { 2286 2287 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Measure> retVal; 2288 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Measure>(); 2289 retVal.setResourceType(org.hl7.fhir.r4b.model.Measure.class); 2290 retVal.setContext(myFhirContext); 2291 return retVal; 2292 } 2293 2294 @Bean(name="myMeasureRpR4B") 2295 @Lazy 2296 public ca.uhn.fhir.jpa.rp.r4b.MeasureResourceProvider rpMeasureR4B() { 2297 ca.uhn.fhir.jpa.rp.r4b.MeasureResourceProvider retVal; 2298 retVal = new ca.uhn.fhir.jpa.rp.r4b.MeasureResourceProvider(); 2299 retVal.setContext(myFhirContext); 2300 retVal.setDao(daoMeasureR4B()); 2301 return retVal; 2302 } 2303 2304 @Bean(name="myMeasureReportDaoR4B") 2305 public 2306 IFhirResourceDao<org.hl7.fhir.r4b.model.MeasureReport> 2307 daoMeasureReportR4B() { 2308 2309 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MeasureReport> retVal; 2310 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MeasureReport>(); 2311 retVal.setResourceType(org.hl7.fhir.r4b.model.MeasureReport.class); 2312 retVal.setContext(myFhirContext); 2313 return retVal; 2314 } 2315 2316 @Bean(name="myMeasureReportRpR4B") 2317 @Lazy 2318 public ca.uhn.fhir.jpa.rp.r4b.MeasureReportResourceProvider rpMeasureReportR4B() { 2319 ca.uhn.fhir.jpa.rp.r4b.MeasureReportResourceProvider retVal; 2320 retVal = new ca.uhn.fhir.jpa.rp.r4b.MeasureReportResourceProvider(); 2321 retVal.setContext(myFhirContext); 2322 retVal.setDao(daoMeasureReportR4B()); 2323 return retVal; 2324 } 2325 2326 @Bean(name="myMediaDaoR4B") 2327 public 2328 IFhirResourceDao<org.hl7.fhir.r4b.model.Media> 2329 daoMediaR4B() { 2330 2331 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Media> retVal; 2332 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Media>(); 2333 retVal.setResourceType(org.hl7.fhir.r4b.model.Media.class); 2334 retVal.setContext(myFhirContext); 2335 return retVal; 2336 } 2337 2338 @Bean(name="myMediaRpR4B") 2339 @Lazy 2340 public ca.uhn.fhir.jpa.rp.r4b.MediaResourceProvider rpMediaR4B() { 2341 ca.uhn.fhir.jpa.rp.r4b.MediaResourceProvider retVal; 2342 retVal = new ca.uhn.fhir.jpa.rp.r4b.MediaResourceProvider(); 2343 retVal.setContext(myFhirContext); 2344 retVal.setDao(daoMediaR4B()); 2345 return retVal; 2346 } 2347 2348 @Bean(name="myMedicationDaoR4B") 2349 public 2350 IFhirResourceDao<org.hl7.fhir.r4b.model.Medication> 2351 daoMedicationR4B() { 2352 2353 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Medication> retVal; 2354 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Medication>(); 2355 retVal.setResourceType(org.hl7.fhir.r4b.model.Medication.class); 2356 retVal.setContext(myFhirContext); 2357 return retVal; 2358 } 2359 2360 @Bean(name="myMedicationRpR4B") 2361 @Lazy 2362 public ca.uhn.fhir.jpa.rp.r4b.MedicationResourceProvider rpMedicationR4B() { 2363 ca.uhn.fhir.jpa.rp.r4b.MedicationResourceProvider retVal; 2364 retVal = new ca.uhn.fhir.jpa.rp.r4b.MedicationResourceProvider(); 2365 retVal.setContext(myFhirContext); 2366 retVal.setDao(daoMedicationR4B()); 2367 return retVal; 2368 } 2369 2370 @Bean(name="myMedicationAdministrationDaoR4B") 2371 public 2372 IFhirResourceDao<org.hl7.fhir.r4b.model.MedicationAdministration> 2373 daoMedicationAdministrationR4B() { 2374 2375 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MedicationAdministration> retVal; 2376 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MedicationAdministration>(); 2377 retVal.setResourceType(org.hl7.fhir.r4b.model.MedicationAdministration.class); 2378 retVal.setContext(myFhirContext); 2379 return retVal; 2380 } 2381 2382 @Bean(name="myMedicationAdministrationRpR4B") 2383 @Lazy 2384 public ca.uhn.fhir.jpa.rp.r4b.MedicationAdministrationResourceProvider rpMedicationAdministrationR4B() { 2385 ca.uhn.fhir.jpa.rp.r4b.MedicationAdministrationResourceProvider retVal; 2386 retVal = new ca.uhn.fhir.jpa.rp.r4b.MedicationAdministrationResourceProvider(); 2387 retVal.setContext(myFhirContext); 2388 retVal.setDao(daoMedicationAdministrationR4B()); 2389 return retVal; 2390 } 2391 2392 @Bean(name="myMedicationDispenseDaoR4B") 2393 public 2394 IFhirResourceDao<org.hl7.fhir.r4b.model.MedicationDispense> 2395 daoMedicationDispenseR4B() { 2396 2397 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MedicationDispense> retVal; 2398 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MedicationDispense>(); 2399 retVal.setResourceType(org.hl7.fhir.r4b.model.MedicationDispense.class); 2400 retVal.setContext(myFhirContext); 2401 return retVal; 2402 } 2403 2404 @Bean(name="myMedicationDispenseRpR4B") 2405 @Lazy 2406 public ca.uhn.fhir.jpa.rp.r4b.MedicationDispenseResourceProvider rpMedicationDispenseR4B() { 2407 ca.uhn.fhir.jpa.rp.r4b.MedicationDispenseResourceProvider retVal; 2408 retVal = new ca.uhn.fhir.jpa.rp.r4b.MedicationDispenseResourceProvider(); 2409 retVal.setContext(myFhirContext); 2410 retVal.setDao(daoMedicationDispenseR4B()); 2411 return retVal; 2412 } 2413 2414 @Bean(name="myMedicationKnowledgeDaoR4B") 2415 public 2416 IFhirResourceDao<org.hl7.fhir.r4b.model.MedicationKnowledge> 2417 daoMedicationKnowledgeR4B() { 2418 2419 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MedicationKnowledge> retVal; 2420 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MedicationKnowledge>(); 2421 retVal.setResourceType(org.hl7.fhir.r4b.model.MedicationKnowledge.class); 2422 retVal.setContext(myFhirContext); 2423 return retVal; 2424 } 2425 2426 @Bean(name="myMedicationKnowledgeRpR4B") 2427 @Lazy 2428 public ca.uhn.fhir.jpa.rp.r4b.MedicationKnowledgeResourceProvider rpMedicationKnowledgeR4B() { 2429 ca.uhn.fhir.jpa.rp.r4b.MedicationKnowledgeResourceProvider retVal; 2430 retVal = new ca.uhn.fhir.jpa.rp.r4b.MedicationKnowledgeResourceProvider(); 2431 retVal.setContext(myFhirContext); 2432 retVal.setDao(daoMedicationKnowledgeR4B()); 2433 return retVal; 2434 } 2435 2436 @Bean(name="myMedicationRequestDaoR4B") 2437 public 2438 IFhirResourceDao<org.hl7.fhir.r4b.model.MedicationRequest> 2439 daoMedicationRequestR4B() { 2440 2441 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MedicationRequest> retVal; 2442 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MedicationRequest>(); 2443 retVal.setResourceType(org.hl7.fhir.r4b.model.MedicationRequest.class); 2444 retVal.setContext(myFhirContext); 2445 return retVal; 2446 } 2447 2448 @Bean(name="myMedicationRequestRpR4B") 2449 @Lazy 2450 public ca.uhn.fhir.jpa.rp.r4b.MedicationRequestResourceProvider rpMedicationRequestR4B() { 2451 ca.uhn.fhir.jpa.rp.r4b.MedicationRequestResourceProvider retVal; 2452 retVal = new ca.uhn.fhir.jpa.rp.r4b.MedicationRequestResourceProvider(); 2453 retVal.setContext(myFhirContext); 2454 retVal.setDao(daoMedicationRequestR4B()); 2455 return retVal; 2456 } 2457 2458 @Bean(name="myMedicationStatementDaoR4B") 2459 public 2460 IFhirResourceDao<org.hl7.fhir.r4b.model.MedicationStatement> 2461 daoMedicationStatementR4B() { 2462 2463 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MedicationStatement> retVal; 2464 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MedicationStatement>(); 2465 retVal.setResourceType(org.hl7.fhir.r4b.model.MedicationStatement.class); 2466 retVal.setContext(myFhirContext); 2467 return retVal; 2468 } 2469 2470 @Bean(name="myMedicationStatementRpR4B") 2471 @Lazy 2472 public ca.uhn.fhir.jpa.rp.r4b.MedicationStatementResourceProvider rpMedicationStatementR4B() { 2473 ca.uhn.fhir.jpa.rp.r4b.MedicationStatementResourceProvider retVal; 2474 retVal = new ca.uhn.fhir.jpa.rp.r4b.MedicationStatementResourceProvider(); 2475 retVal.setContext(myFhirContext); 2476 retVal.setDao(daoMedicationStatementR4B()); 2477 return retVal; 2478 } 2479 2480 @Bean(name="myMedicinalProductDefinitionDaoR4B") 2481 public 2482 IFhirResourceDao<org.hl7.fhir.r4b.model.MedicinalProductDefinition> 2483 daoMedicinalProductDefinitionR4B() { 2484 2485 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MedicinalProductDefinition> retVal; 2486 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MedicinalProductDefinition>(); 2487 retVal.setResourceType(org.hl7.fhir.r4b.model.MedicinalProductDefinition.class); 2488 retVal.setContext(myFhirContext); 2489 return retVal; 2490 } 2491 2492 @Bean(name="myMedicinalProductDefinitionRpR4B") 2493 @Lazy 2494 public ca.uhn.fhir.jpa.rp.r4b.MedicinalProductDefinitionResourceProvider rpMedicinalProductDefinitionR4B() { 2495 ca.uhn.fhir.jpa.rp.r4b.MedicinalProductDefinitionResourceProvider retVal; 2496 retVal = new ca.uhn.fhir.jpa.rp.r4b.MedicinalProductDefinitionResourceProvider(); 2497 retVal.setContext(myFhirContext); 2498 retVal.setDao(daoMedicinalProductDefinitionR4B()); 2499 return retVal; 2500 } 2501 2502 @Bean(name="myMessageDefinitionDaoR4B") 2503 public 2504 IFhirResourceDao<org.hl7.fhir.r4b.model.MessageDefinition> 2505 daoMessageDefinitionR4B() { 2506 2507 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MessageDefinition> retVal; 2508 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MessageDefinition>(); 2509 retVal.setResourceType(org.hl7.fhir.r4b.model.MessageDefinition.class); 2510 retVal.setContext(myFhirContext); 2511 return retVal; 2512 } 2513 2514 @Bean(name="myMessageDefinitionRpR4B") 2515 @Lazy 2516 public ca.uhn.fhir.jpa.rp.r4b.MessageDefinitionResourceProvider rpMessageDefinitionR4B() { 2517 ca.uhn.fhir.jpa.rp.r4b.MessageDefinitionResourceProvider retVal; 2518 retVal = new ca.uhn.fhir.jpa.rp.r4b.MessageDefinitionResourceProvider(); 2519 retVal.setContext(myFhirContext); 2520 retVal.setDao(daoMessageDefinitionR4B()); 2521 return retVal; 2522 } 2523 2524 @Bean(name="myMessageHeaderDaoR4B") 2525 public 2526 IFhirResourceDao<org.hl7.fhir.r4b.model.MessageHeader> 2527 daoMessageHeaderR4B() { 2528 2529 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MessageHeader> retVal; 2530 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MessageHeader>(); 2531 retVal.setResourceType(org.hl7.fhir.r4b.model.MessageHeader.class); 2532 retVal.setContext(myFhirContext); 2533 return retVal; 2534 } 2535 2536 @Bean(name="myMessageHeaderRpR4B") 2537 @Lazy 2538 public ca.uhn.fhir.jpa.rp.r4b.MessageHeaderResourceProvider rpMessageHeaderR4B() { 2539 ca.uhn.fhir.jpa.rp.r4b.MessageHeaderResourceProvider retVal; 2540 retVal = new ca.uhn.fhir.jpa.rp.r4b.MessageHeaderResourceProvider(); 2541 retVal.setContext(myFhirContext); 2542 retVal.setDao(daoMessageHeaderR4B()); 2543 return retVal; 2544 } 2545 2546 @Bean(name="myMolecularSequenceDaoR4B") 2547 public 2548 IFhirResourceDao<org.hl7.fhir.r4b.model.MolecularSequence> 2549 daoMolecularSequenceR4B() { 2550 2551 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.MolecularSequence> retVal; 2552 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.MolecularSequence>(); 2553 retVal.setResourceType(org.hl7.fhir.r4b.model.MolecularSequence.class); 2554 retVal.setContext(myFhirContext); 2555 return retVal; 2556 } 2557 2558 @Bean(name="myMolecularSequenceRpR4B") 2559 @Lazy 2560 public ca.uhn.fhir.jpa.rp.r4b.MolecularSequenceResourceProvider rpMolecularSequenceR4B() { 2561 ca.uhn.fhir.jpa.rp.r4b.MolecularSequenceResourceProvider retVal; 2562 retVal = new ca.uhn.fhir.jpa.rp.r4b.MolecularSequenceResourceProvider(); 2563 retVal.setContext(myFhirContext); 2564 retVal.setDao(daoMolecularSequenceR4B()); 2565 return retVal; 2566 } 2567 2568 @Bean(name="myNamingSystemDaoR4B") 2569 public 2570 IFhirResourceDao<org.hl7.fhir.r4b.model.NamingSystem> 2571 daoNamingSystemR4B() { 2572 2573 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.NamingSystem> retVal; 2574 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.NamingSystem>(); 2575 retVal.setResourceType(org.hl7.fhir.r4b.model.NamingSystem.class); 2576 retVal.setContext(myFhirContext); 2577 return retVal; 2578 } 2579 2580 @Bean(name="myNamingSystemRpR4B") 2581 @Lazy 2582 public ca.uhn.fhir.jpa.rp.r4b.NamingSystemResourceProvider rpNamingSystemR4B() { 2583 ca.uhn.fhir.jpa.rp.r4b.NamingSystemResourceProvider retVal; 2584 retVal = new ca.uhn.fhir.jpa.rp.r4b.NamingSystemResourceProvider(); 2585 retVal.setContext(myFhirContext); 2586 retVal.setDao(daoNamingSystemR4B()); 2587 return retVal; 2588 } 2589 2590 @Bean(name="myNutritionOrderDaoR4B") 2591 public 2592 IFhirResourceDao<org.hl7.fhir.r4b.model.NutritionOrder> 2593 daoNutritionOrderR4B() { 2594 2595 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.NutritionOrder> retVal; 2596 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.NutritionOrder>(); 2597 retVal.setResourceType(org.hl7.fhir.r4b.model.NutritionOrder.class); 2598 retVal.setContext(myFhirContext); 2599 return retVal; 2600 } 2601 2602 @Bean(name="myNutritionOrderRpR4B") 2603 @Lazy 2604 public ca.uhn.fhir.jpa.rp.r4b.NutritionOrderResourceProvider rpNutritionOrderR4B() { 2605 ca.uhn.fhir.jpa.rp.r4b.NutritionOrderResourceProvider retVal; 2606 retVal = new ca.uhn.fhir.jpa.rp.r4b.NutritionOrderResourceProvider(); 2607 retVal.setContext(myFhirContext); 2608 retVal.setDao(daoNutritionOrderR4B()); 2609 return retVal; 2610 } 2611 2612 @Bean(name="myNutritionProductDaoR4B") 2613 public 2614 IFhirResourceDao<org.hl7.fhir.r4b.model.NutritionProduct> 2615 daoNutritionProductR4B() { 2616 2617 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.NutritionProduct> retVal; 2618 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.NutritionProduct>(); 2619 retVal.setResourceType(org.hl7.fhir.r4b.model.NutritionProduct.class); 2620 retVal.setContext(myFhirContext); 2621 return retVal; 2622 } 2623 2624 @Bean(name="myNutritionProductRpR4B") 2625 @Lazy 2626 public ca.uhn.fhir.jpa.rp.r4b.NutritionProductResourceProvider rpNutritionProductR4B() { 2627 ca.uhn.fhir.jpa.rp.r4b.NutritionProductResourceProvider retVal; 2628 retVal = new ca.uhn.fhir.jpa.rp.r4b.NutritionProductResourceProvider(); 2629 retVal.setContext(myFhirContext); 2630 retVal.setDao(daoNutritionProductR4B()); 2631 return retVal; 2632 } 2633 2634 @Bean(name="myObservationDaoR4B") 2635 public 2636 IFhirResourceDaoObservation<org.hl7.fhir.r4b.model.Observation> 2637 daoObservationR4B() { 2638 2639 ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<org.hl7.fhir.r4b.model.Observation> retVal; 2640 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<>(); 2641 retVal.setResourceType(org.hl7.fhir.r4b.model.Observation.class); 2642 retVal.setContext(myFhirContext); 2643 return retVal; 2644 } 2645 2646 @Bean(name="myObservationRpR4B") 2647 @Lazy 2648 public ca.uhn.fhir.jpa.rp.r4b.ObservationResourceProvider rpObservationR4B() { 2649 ca.uhn.fhir.jpa.rp.r4b.ObservationResourceProvider retVal; 2650 retVal = new ca.uhn.fhir.jpa.rp.r4b.ObservationResourceProvider(); 2651 retVal.setContext(myFhirContext); 2652 retVal.setDao(daoObservationR4B()); 2653 return retVal; 2654 } 2655 2656 @Bean(name="myObservationDefinitionDaoR4B") 2657 public 2658 IFhirResourceDao<org.hl7.fhir.r4b.model.ObservationDefinition> 2659 daoObservationDefinitionR4B() { 2660 2661 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ObservationDefinition> retVal; 2662 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ObservationDefinition>(); 2663 retVal.setResourceType(org.hl7.fhir.r4b.model.ObservationDefinition.class); 2664 retVal.setContext(myFhirContext); 2665 return retVal; 2666 } 2667 2668 @Bean(name="myObservationDefinitionRpR4B") 2669 @Lazy 2670 public ca.uhn.fhir.jpa.rp.r4b.ObservationDefinitionResourceProvider rpObservationDefinitionR4B() { 2671 ca.uhn.fhir.jpa.rp.r4b.ObservationDefinitionResourceProvider retVal; 2672 retVal = new ca.uhn.fhir.jpa.rp.r4b.ObservationDefinitionResourceProvider(); 2673 retVal.setContext(myFhirContext); 2674 retVal.setDao(daoObservationDefinitionR4B()); 2675 return retVal; 2676 } 2677 2678 @Bean(name="myOperationDefinitionDaoR4B") 2679 public 2680 IFhirResourceDao<org.hl7.fhir.r4b.model.OperationDefinition> 2681 daoOperationDefinitionR4B() { 2682 2683 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.OperationDefinition> retVal; 2684 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.OperationDefinition>(); 2685 retVal.setResourceType(org.hl7.fhir.r4b.model.OperationDefinition.class); 2686 retVal.setContext(myFhirContext); 2687 return retVal; 2688 } 2689 2690 @Bean(name="myOperationDefinitionRpR4B") 2691 @Lazy 2692 public ca.uhn.fhir.jpa.rp.r4b.OperationDefinitionResourceProvider rpOperationDefinitionR4B() { 2693 ca.uhn.fhir.jpa.rp.r4b.OperationDefinitionResourceProvider retVal; 2694 retVal = new ca.uhn.fhir.jpa.rp.r4b.OperationDefinitionResourceProvider(); 2695 retVal.setContext(myFhirContext); 2696 retVal.setDao(daoOperationDefinitionR4B()); 2697 return retVal; 2698 } 2699 2700 @Bean(name="myOperationOutcomeDaoR4B") 2701 public 2702 IFhirResourceDao<org.hl7.fhir.r4b.model.OperationOutcome> 2703 daoOperationOutcomeR4B() { 2704 2705 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.OperationOutcome> retVal; 2706 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.OperationOutcome>(); 2707 retVal.setResourceType(org.hl7.fhir.r4b.model.OperationOutcome.class); 2708 retVal.setContext(myFhirContext); 2709 return retVal; 2710 } 2711 2712 @Bean(name="myOperationOutcomeRpR4B") 2713 @Lazy 2714 public ca.uhn.fhir.jpa.rp.r4b.OperationOutcomeResourceProvider rpOperationOutcomeR4B() { 2715 ca.uhn.fhir.jpa.rp.r4b.OperationOutcomeResourceProvider retVal; 2716 retVal = new ca.uhn.fhir.jpa.rp.r4b.OperationOutcomeResourceProvider(); 2717 retVal.setContext(myFhirContext); 2718 retVal.setDao(daoOperationOutcomeR4B()); 2719 return retVal; 2720 } 2721 2722 @Bean(name="myOrganizationDaoR4B") 2723 public 2724 IFhirResourceDao<org.hl7.fhir.r4b.model.Organization> 2725 daoOrganizationR4B() { 2726 2727 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Organization> retVal; 2728 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Organization>(); 2729 retVal.setResourceType(org.hl7.fhir.r4b.model.Organization.class); 2730 retVal.setContext(myFhirContext); 2731 return retVal; 2732 } 2733 2734 @Bean(name="myOrganizationRpR4B") 2735 @Lazy 2736 public ca.uhn.fhir.jpa.rp.r4b.OrganizationResourceProvider rpOrganizationR4B() { 2737 ca.uhn.fhir.jpa.rp.r4b.OrganizationResourceProvider retVal; 2738 retVal = new ca.uhn.fhir.jpa.rp.r4b.OrganizationResourceProvider(); 2739 retVal.setContext(myFhirContext); 2740 retVal.setDao(daoOrganizationR4B()); 2741 return retVal; 2742 } 2743 2744 @Bean(name="myOrganizationAffiliationDaoR4B") 2745 public 2746 IFhirResourceDao<org.hl7.fhir.r4b.model.OrganizationAffiliation> 2747 daoOrganizationAffiliationR4B() { 2748 2749 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.OrganizationAffiliation> retVal; 2750 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.OrganizationAffiliation>(); 2751 retVal.setResourceType(org.hl7.fhir.r4b.model.OrganizationAffiliation.class); 2752 retVal.setContext(myFhirContext); 2753 return retVal; 2754 } 2755 2756 @Bean(name="myOrganizationAffiliationRpR4B") 2757 @Lazy 2758 public ca.uhn.fhir.jpa.rp.r4b.OrganizationAffiliationResourceProvider rpOrganizationAffiliationR4B() { 2759 ca.uhn.fhir.jpa.rp.r4b.OrganizationAffiliationResourceProvider retVal; 2760 retVal = new ca.uhn.fhir.jpa.rp.r4b.OrganizationAffiliationResourceProvider(); 2761 retVal.setContext(myFhirContext); 2762 retVal.setDao(daoOrganizationAffiliationR4B()); 2763 return retVal; 2764 } 2765 2766 @Bean(name="myPackagedProductDefinitionDaoR4B") 2767 public 2768 IFhirResourceDao<org.hl7.fhir.r4b.model.PackagedProductDefinition> 2769 daoPackagedProductDefinitionR4B() { 2770 2771 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.PackagedProductDefinition> retVal; 2772 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.PackagedProductDefinition>(); 2773 retVal.setResourceType(org.hl7.fhir.r4b.model.PackagedProductDefinition.class); 2774 retVal.setContext(myFhirContext); 2775 return retVal; 2776 } 2777 2778 @Bean(name="myPackagedProductDefinitionRpR4B") 2779 @Lazy 2780 public ca.uhn.fhir.jpa.rp.r4b.PackagedProductDefinitionResourceProvider rpPackagedProductDefinitionR4B() { 2781 ca.uhn.fhir.jpa.rp.r4b.PackagedProductDefinitionResourceProvider retVal; 2782 retVal = new ca.uhn.fhir.jpa.rp.r4b.PackagedProductDefinitionResourceProvider(); 2783 retVal.setContext(myFhirContext); 2784 retVal.setDao(daoPackagedProductDefinitionR4B()); 2785 return retVal; 2786 } 2787 2788 @Bean(name="myParametersDaoR4B") 2789 public 2790 IFhirResourceDao<org.hl7.fhir.r4b.model.Parameters> 2791 daoParametersR4B() { 2792 2793 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Parameters> retVal; 2794 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Parameters>(); 2795 retVal.setResourceType(org.hl7.fhir.r4b.model.Parameters.class); 2796 retVal.setContext(myFhirContext); 2797 return retVal; 2798 } 2799 2800 @Bean(name="myParametersRpR4B") 2801 @Lazy 2802 public ca.uhn.fhir.jpa.rp.r4b.ParametersResourceProvider rpParametersR4B() { 2803 ca.uhn.fhir.jpa.rp.r4b.ParametersResourceProvider retVal; 2804 retVal = new ca.uhn.fhir.jpa.rp.r4b.ParametersResourceProvider(); 2805 retVal.setContext(myFhirContext); 2806 retVal.setDao(daoParametersR4B()); 2807 return retVal; 2808 } 2809 2810 @Bean(name="myPatientDaoR4B") 2811 public 2812 IFhirResourceDaoPatient<org.hl7.fhir.r4b.model.Patient> 2813 daoPatientR4B() { 2814 2815 ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<org.hl7.fhir.r4b.model.Patient> retVal; 2816 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<>(); 2817 retVal.setResourceType(org.hl7.fhir.r4b.model.Patient.class); 2818 retVal.setContext(myFhirContext); 2819 return retVal; 2820 } 2821 2822 @Bean(name="myPatientRpR4B") 2823 @Lazy 2824 public ca.uhn.fhir.jpa.rp.r4b.PatientResourceProvider rpPatientR4B() { 2825 ca.uhn.fhir.jpa.rp.r4b.PatientResourceProvider retVal; 2826 retVal = new ca.uhn.fhir.jpa.rp.r4b.PatientResourceProvider(); 2827 retVal.setContext(myFhirContext); 2828 retVal.setDao(daoPatientR4B()); 2829 return retVal; 2830 } 2831 2832 @Bean(name="myPaymentNoticeDaoR4B") 2833 public 2834 IFhirResourceDao<org.hl7.fhir.r4b.model.PaymentNotice> 2835 daoPaymentNoticeR4B() { 2836 2837 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.PaymentNotice> retVal; 2838 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.PaymentNotice>(); 2839 retVal.setResourceType(org.hl7.fhir.r4b.model.PaymentNotice.class); 2840 retVal.setContext(myFhirContext); 2841 return retVal; 2842 } 2843 2844 @Bean(name="myPaymentNoticeRpR4B") 2845 @Lazy 2846 public ca.uhn.fhir.jpa.rp.r4b.PaymentNoticeResourceProvider rpPaymentNoticeR4B() { 2847 ca.uhn.fhir.jpa.rp.r4b.PaymentNoticeResourceProvider retVal; 2848 retVal = new ca.uhn.fhir.jpa.rp.r4b.PaymentNoticeResourceProvider(); 2849 retVal.setContext(myFhirContext); 2850 retVal.setDao(daoPaymentNoticeR4B()); 2851 return retVal; 2852 } 2853 2854 @Bean(name="myPaymentReconciliationDaoR4B") 2855 public 2856 IFhirResourceDao<org.hl7.fhir.r4b.model.PaymentReconciliation> 2857 daoPaymentReconciliationR4B() { 2858 2859 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.PaymentReconciliation> retVal; 2860 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.PaymentReconciliation>(); 2861 retVal.setResourceType(org.hl7.fhir.r4b.model.PaymentReconciliation.class); 2862 retVal.setContext(myFhirContext); 2863 return retVal; 2864 } 2865 2866 @Bean(name="myPaymentReconciliationRpR4B") 2867 @Lazy 2868 public ca.uhn.fhir.jpa.rp.r4b.PaymentReconciliationResourceProvider rpPaymentReconciliationR4B() { 2869 ca.uhn.fhir.jpa.rp.r4b.PaymentReconciliationResourceProvider retVal; 2870 retVal = new ca.uhn.fhir.jpa.rp.r4b.PaymentReconciliationResourceProvider(); 2871 retVal.setContext(myFhirContext); 2872 retVal.setDao(daoPaymentReconciliationR4B()); 2873 return retVal; 2874 } 2875 2876 @Bean(name="myPersonDaoR4B") 2877 public 2878 IFhirResourceDao<org.hl7.fhir.r4b.model.Person> 2879 daoPersonR4B() { 2880 2881 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Person> retVal; 2882 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Person>(); 2883 retVal.setResourceType(org.hl7.fhir.r4b.model.Person.class); 2884 retVal.setContext(myFhirContext); 2885 return retVal; 2886 } 2887 2888 @Bean(name="myPersonRpR4B") 2889 @Lazy 2890 public ca.uhn.fhir.jpa.rp.r4b.PersonResourceProvider rpPersonR4B() { 2891 ca.uhn.fhir.jpa.rp.r4b.PersonResourceProvider retVal; 2892 retVal = new ca.uhn.fhir.jpa.rp.r4b.PersonResourceProvider(); 2893 retVal.setContext(myFhirContext); 2894 retVal.setDao(daoPersonR4B()); 2895 return retVal; 2896 } 2897 2898 @Bean(name="myPlanDefinitionDaoR4B") 2899 public 2900 IFhirResourceDao<org.hl7.fhir.r4b.model.PlanDefinition> 2901 daoPlanDefinitionR4B() { 2902 2903 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.PlanDefinition> retVal; 2904 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.PlanDefinition>(); 2905 retVal.setResourceType(org.hl7.fhir.r4b.model.PlanDefinition.class); 2906 retVal.setContext(myFhirContext); 2907 return retVal; 2908 } 2909 2910 @Bean(name="myPlanDefinitionRpR4B") 2911 @Lazy 2912 public ca.uhn.fhir.jpa.rp.r4b.PlanDefinitionResourceProvider rpPlanDefinitionR4B() { 2913 ca.uhn.fhir.jpa.rp.r4b.PlanDefinitionResourceProvider retVal; 2914 retVal = new ca.uhn.fhir.jpa.rp.r4b.PlanDefinitionResourceProvider(); 2915 retVal.setContext(myFhirContext); 2916 retVal.setDao(daoPlanDefinitionR4B()); 2917 return retVal; 2918 } 2919 2920 @Bean(name="myPractitionerDaoR4B") 2921 public 2922 IFhirResourceDao<org.hl7.fhir.r4b.model.Practitioner> 2923 daoPractitionerR4B() { 2924 2925 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Practitioner> retVal; 2926 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Practitioner>(); 2927 retVal.setResourceType(org.hl7.fhir.r4b.model.Practitioner.class); 2928 retVal.setContext(myFhirContext); 2929 return retVal; 2930 } 2931 2932 @Bean(name="myPractitionerRpR4B") 2933 @Lazy 2934 public ca.uhn.fhir.jpa.rp.r4b.PractitionerResourceProvider rpPractitionerR4B() { 2935 ca.uhn.fhir.jpa.rp.r4b.PractitionerResourceProvider retVal; 2936 retVal = new ca.uhn.fhir.jpa.rp.r4b.PractitionerResourceProvider(); 2937 retVal.setContext(myFhirContext); 2938 retVal.setDao(daoPractitionerR4B()); 2939 return retVal; 2940 } 2941 2942 @Bean(name="myPractitionerRoleDaoR4B") 2943 public 2944 IFhirResourceDao<org.hl7.fhir.r4b.model.PractitionerRole> 2945 daoPractitionerRoleR4B() { 2946 2947 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.PractitionerRole> retVal; 2948 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.PractitionerRole>(); 2949 retVal.setResourceType(org.hl7.fhir.r4b.model.PractitionerRole.class); 2950 retVal.setContext(myFhirContext); 2951 return retVal; 2952 } 2953 2954 @Bean(name="myPractitionerRoleRpR4B") 2955 @Lazy 2956 public ca.uhn.fhir.jpa.rp.r4b.PractitionerRoleResourceProvider rpPractitionerRoleR4B() { 2957 ca.uhn.fhir.jpa.rp.r4b.PractitionerRoleResourceProvider retVal; 2958 retVal = new ca.uhn.fhir.jpa.rp.r4b.PractitionerRoleResourceProvider(); 2959 retVal.setContext(myFhirContext); 2960 retVal.setDao(daoPractitionerRoleR4B()); 2961 return retVal; 2962 } 2963 2964 @Bean(name="myProcedureDaoR4B") 2965 public 2966 IFhirResourceDao<org.hl7.fhir.r4b.model.Procedure> 2967 daoProcedureR4B() { 2968 2969 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Procedure> retVal; 2970 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Procedure>(); 2971 retVal.setResourceType(org.hl7.fhir.r4b.model.Procedure.class); 2972 retVal.setContext(myFhirContext); 2973 return retVal; 2974 } 2975 2976 @Bean(name="myProcedureRpR4B") 2977 @Lazy 2978 public ca.uhn.fhir.jpa.rp.r4b.ProcedureResourceProvider rpProcedureR4B() { 2979 ca.uhn.fhir.jpa.rp.r4b.ProcedureResourceProvider retVal; 2980 retVal = new ca.uhn.fhir.jpa.rp.r4b.ProcedureResourceProvider(); 2981 retVal.setContext(myFhirContext); 2982 retVal.setDao(daoProcedureR4B()); 2983 return retVal; 2984 } 2985 2986 @Bean(name="myProvenanceDaoR4B") 2987 public 2988 IFhirResourceDao<org.hl7.fhir.r4b.model.Provenance> 2989 daoProvenanceR4B() { 2990 2991 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Provenance> retVal; 2992 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Provenance>(); 2993 retVal.setResourceType(org.hl7.fhir.r4b.model.Provenance.class); 2994 retVal.setContext(myFhirContext); 2995 return retVal; 2996 } 2997 2998 @Bean(name="myProvenanceRpR4B") 2999 @Lazy 3000 public ca.uhn.fhir.jpa.rp.r4b.ProvenanceResourceProvider rpProvenanceR4B() { 3001 ca.uhn.fhir.jpa.rp.r4b.ProvenanceResourceProvider retVal; 3002 retVal = new ca.uhn.fhir.jpa.rp.r4b.ProvenanceResourceProvider(); 3003 retVal.setContext(myFhirContext); 3004 retVal.setDao(daoProvenanceR4B()); 3005 return retVal; 3006 } 3007 3008 @Bean(name="myQuestionnaireDaoR4B") 3009 public 3010 IFhirResourceDao<org.hl7.fhir.r4b.model.Questionnaire> 3011 daoQuestionnaireR4B() { 3012 3013 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Questionnaire> retVal; 3014 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Questionnaire>(); 3015 retVal.setResourceType(org.hl7.fhir.r4b.model.Questionnaire.class); 3016 retVal.setContext(myFhirContext); 3017 return retVal; 3018 } 3019 3020 @Bean(name="myQuestionnaireRpR4B") 3021 @Lazy 3022 public ca.uhn.fhir.jpa.rp.r4b.QuestionnaireResourceProvider rpQuestionnaireR4B() { 3023 ca.uhn.fhir.jpa.rp.r4b.QuestionnaireResourceProvider retVal; 3024 retVal = new ca.uhn.fhir.jpa.rp.r4b.QuestionnaireResourceProvider(); 3025 retVal.setContext(myFhirContext); 3026 retVal.setDao(daoQuestionnaireR4B()); 3027 return retVal; 3028 } 3029 3030 @Bean(name="myQuestionnaireResponseDaoR4B") 3031 public 3032 IFhirResourceDao<org.hl7.fhir.r4b.model.QuestionnaireResponse> 3033 daoQuestionnaireResponseR4B() { 3034 3035 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.QuestionnaireResponse> retVal; 3036 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.QuestionnaireResponse>(); 3037 retVal.setResourceType(org.hl7.fhir.r4b.model.QuestionnaireResponse.class); 3038 retVal.setContext(myFhirContext); 3039 return retVal; 3040 } 3041 3042 @Bean(name="myQuestionnaireResponseRpR4B") 3043 @Lazy 3044 public ca.uhn.fhir.jpa.rp.r4b.QuestionnaireResponseResourceProvider rpQuestionnaireResponseR4B() { 3045 ca.uhn.fhir.jpa.rp.r4b.QuestionnaireResponseResourceProvider retVal; 3046 retVal = new ca.uhn.fhir.jpa.rp.r4b.QuestionnaireResponseResourceProvider(); 3047 retVal.setContext(myFhirContext); 3048 retVal.setDao(daoQuestionnaireResponseR4B()); 3049 return retVal; 3050 } 3051 3052 @Bean(name="myRegulatedAuthorizationDaoR4B") 3053 public 3054 IFhirResourceDao<org.hl7.fhir.r4b.model.RegulatedAuthorization> 3055 daoRegulatedAuthorizationR4B() { 3056 3057 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.RegulatedAuthorization> retVal; 3058 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.RegulatedAuthorization>(); 3059 retVal.setResourceType(org.hl7.fhir.r4b.model.RegulatedAuthorization.class); 3060 retVal.setContext(myFhirContext); 3061 return retVal; 3062 } 3063 3064 @Bean(name="myRegulatedAuthorizationRpR4B") 3065 @Lazy 3066 public ca.uhn.fhir.jpa.rp.r4b.RegulatedAuthorizationResourceProvider rpRegulatedAuthorizationR4B() { 3067 ca.uhn.fhir.jpa.rp.r4b.RegulatedAuthorizationResourceProvider retVal; 3068 retVal = new ca.uhn.fhir.jpa.rp.r4b.RegulatedAuthorizationResourceProvider(); 3069 retVal.setContext(myFhirContext); 3070 retVal.setDao(daoRegulatedAuthorizationR4B()); 3071 return retVal; 3072 } 3073 3074 @Bean(name="myRelatedPersonDaoR4B") 3075 public 3076 IFhirResourceDao<org.hl7.fhir.r4b.model.RelatedPerson> 3077 daoRelatedPersonR4B() { 3078 3079 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.RelatedPerson> retVal; 3080 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.RelatedPerson>(); 3081 retVal.setResourceType(org.hl7.fhir.r4b.model.RelatedPerson.class); 3082 retVal.setContext(myFhirContext); 3083 return retVal; 3084 } 3085 3086 @Bean(name="myRelatedPersonRpR4B") 3087 @Lazy 3088 public ca.uhn.fhir.jpa.rp.r4b.RelatedPersonResourceProvider rpRelatedPersonR4B() { 3089 ca.uhn.fhir.jpa.rp.r4b.RelatedPersonResourceProvider retVal; 3090 retVal = new ca.uhn.fhir.jpa.rp.r4b.RelatedPersonResourceProvider(); 3091 retVal.setContext(myFhirContext); 3092 retVal.setDao(daoRelatedPersonR4B()); 3093 return retVal; 3094 } 3095 3096 @Bean(name="myRequestGroupDaoR4B") 3097 public 3098 IFhirResourceDao<org.hl7.fhir.r4b.model.RequestGroup> 3099 daoRequestGroupR4B() { 3100 3101 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.RequestGroup> retVal; 3102 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.RequestGroup>(); 3103 retVal.setResourceType(org.hl7.fhir.r4b.model.RequestGroup.class); 3104 retVal.setContext(myFhirContext); 3105 return retVal; 3106 } 3107 3108 @Bean(name="myRequestGroupRpR4B") 3109 @Lazy 3110 public ca.uhn.fhir.jpa.rp.r4b.RequestGroupResourceProvider rpRequestGroupR4B() { 3111 ca.uhn.fhir.jpa.rp.r4b.RequestGroupResourceProvider retVal; 3112 retVal = new ca.uhn.fhir.jpa.rp.r4b.RequestGroupResourceProvider(); 3113 retVal.setContext(myFhirContext); 3114 retVal.setDao(daoRequestGroupR4B()); 3115 return retVal; 3116 } 3117 3118 @Bean(name="myResearchDefinitionDaoR4B") 3119 public 3120 IFhirResourceDao<org.hl7.fhir.r4b.model.ResearchDefinition> 3121 daoResearchDefinitionR4B() { 3122 3123 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ResearchDefinition> retVal; 3124 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ResearchDefinition>(); 3125 retVal.setResourceType(org.hl7.fhir.r4b.model.ResearchDefinition.class); 3126 retVal.setContext(myFhirContext); 3127 return retVal; 3128 } 3129 3130 @Bean(name="myResearchDefinitionRpR4B") 3131 @Lazy 3132 public ca.uhn.fhir.jpa.rp.r4b.ResearchDefinitionResourceProvider rpResearchDefinitionR4B() { 3133 ca.uhn.fhir.jpa.rp.r4b.ResearchDefinitionResourceProvider retVal; 3134 retVal = new ca.uhn.fhir.jpa.rp.r4b.ResearchDefinitionResourceProvider(); 3135 retVal.setContext(myFhirContext); 3136 retVal.setDao(daoResearchDefinitionR4B()); 3137 return retVal; 3138 } 3139 3140 @Bean(name="myResearchElementDefinitionDaoR4B") 3141 public 3142 IFhirResourceDao<org.hl7.fhir.r4b.model.ResearchElementDefinition> 3143 daoResearchElementDefinitionR4B() { 3144 3145 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ResearchElementDefinition> retVal; 3146 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ResearchElementDefinition>(); 3147 retVal.setResourceType(org.hl7.fhir.r4b.model.ResearchElementDefinition.class); 3148 retVal.setContext(myFhirContext); 3149 return retVal; 3150 } 3151 3152 @Bean(name="myResearchElementDefinitionRpR4B") 3153 @Lazy 3154 public ca.uhn.fhir.jpa.rp.r4b.ResearchElementDefinitionResourceProvider rpResearchElementDefinitionR4B() { 3155 ca.uhn.fhir.jpa.rp.r4b.ResearchElementDefinitionResourceProvider retVal; 3156 retVal = new ca.uhn.fhir.jpa.rp.r4b.ResearchElementDefinitionResourceProvider(); 3157 retVal.setContext(myFhirContext); 3158 retVal.setDao(daoResearchElementDefinitionR4B()); 3159 return retVal; 3160 } 3161 3162 @Bean(name="myResearchStudyDaoR4B") 3163 public 3164 IFhirResourceDao<org.hl7.fhir.r4b.model.ResearchStudy> 3165 daoResearchStudyR4B() { 3166 3167 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ResearchStudy> retVal; 3168 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ResearchStudy>(); 3169 retVal.setResourceType(org.hl7.fhir.r4b.model.ResearchStudy.class); 3170 retVal.setContext(myFhirContext); 3171 return retVal; 3172 } 3173 3174 @Bean(name="myResearchStudyRpR4B") 3175 @Lazy 3176 public ca.uhn.fhir.jpa.rp.r4b.ResearchStudyResourceProvider rpResearchStudyR4B() { 3177 ca.uhn.fhir.jpa.rp.r4b.ResearchStudyResourceProvider retVal; 3178 retVal = new ca.uhn.fhir.jpa.rp.r4b.ResearchStudyResourceProvider(); 3179 retVal.setContext(myFhirContext); 3180 retVal.setDao(daoResearchStudyR4B()); 3181 return retVal; 3182 } 3183 3184 @Bean(name="myResearchSubjectDaoR4B") 3185 public 3186 IFhirResourceDao<org.hl7.fhir.r4b.model.ResearchSubject> 3187 daoResearchSubjectR4B() { 3188 3189 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ResearchSubject> retVal; 3190 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ResearchSubject>(); 3191 retVal.setResourceType(org.hl7.fhir.r4b.model.ResearchSubject.class); 3192 retVal.setContext(myFhirContext); 3193 return retVal; 3194 } 3195 3196 @Bean(name="myResearchSubjectRpR4B") 3197 @Lazy 3198 public ca.uhn.fhir.jpa.rp.r4b.ResearchSubjectResourceProvider rpResearchSubjectR4B() { 3199 ca.uhn.fhir.jpa.rp.r4b.ResearchSubjectResourceProvider retVal; 3200 retVal = new ca.uhn.fhir.jpa.rp.r4b.ResearchSubjectResourceProvider(); 3201 retVal.setContext(myFhirContext); 3202 retVal.setDao(daoResearchSubjectR4B()); 3203 return retVal; 3204 } 3205 3206 @Bean(name="myRiskAssessmentDaoR4B") 3207 public 3208 IFhirResourceDao<org.hl7.fhir.r4b.model.RiskAssessment> 3209 daoRiskAssessmentR4B() { 3210 3211 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.RiskAssessment> retVal; 3212 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.RiskAssessment>(); 3213 retVal.setResourceType(org.hl7.fhir.r4b.model.RiskAssessment.class); 3214 retVal.setContext(myFhirContext); 3215 return retVal; 3216 } 3217 3218 @Bean(name="myRiskAssessmentRpR4B") 3219 @Lazy 3220 public ca.uhn.fhir.jpa.rp.r4b.RiskAssessmentResourceProvider rpRiskAssessmentR4B() { 3221 ca.uhn.fhir.jpa.rp.r4b.RiskAssessmentResourceProvider retVal; 3222 retVal = new ca.uhn.fhir.jpa.rp.r4b.RiskAssessmentResourceProvider(); 3223 retVal.setContext(myFhirContext); 3224 retVal.setDao(daoRiskAssessmentR4B()); 3225 return retVal; 3226 } 3227 3228 @Bean(name="myScheduleDaoR4B") 3229 public 3230 IFhirResourceDao<org.hl7.fhir.r4b.model.Schedule> 3231 daoScheduleR4B() { 3232 3233 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Schedule> retVal; 3234 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Schedule>(); 3235 retVal.setResourceType(org.hl7.fhir.r4b.model.Schedule.class); 3236 retVal.setContext(myFhirContext); 3237 return retVal; 3238 } 3239 3240 @Bean(name="myScheduleRpR4B") 3241 @Lazy 3242 public ca.uhn.fhir.jpa.rp.r4b.ScheduleResourceProvider rpScheduleR4B() { 3243 ca.uhn.fhir.jpa.rp.r4b.ScheduleResourceProvider retVal; 3244 retVal = new ca.uhn.fhir.jpa.rp.r4b.ScheduleResourceProvider(); 3245 retVal.setContext(myFhirContext); 3246 retVal.setDao(daoScheduleR4B()); 3247 return retVal; 3248 } 3249 3250 @Bean(name="mySearchParameterDaoR4B") 3251 public 3252 IFhirResourceDaoSearchParameter<org.hl7.fhir.r4b.model.SearchParameter> 3253 daoSearchParameterR4B() { 3254 3255 ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<org.hl7.fhir.r4b.model.SearchParameter> retVal; 3256 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<>(); 3257 retVal.setResourceType(org.hl7.fhir.r4b.model.SearchParameter.class); 3258 retVal.setContext(myFhirContext); 3259 return retVal; 3260 } 3261 3262 @Bean(name="mySearchParameterRpR4B") 3263 @Lazy 3264 public ca.uhn.fhir.jpa.rp.r4b.SearchParameterResourceProvider rpSearchParameterR4B() { 3265 ca.uhn.fhir.jpa.rp.r4b.SearchParameterResourceProvider retVal; 3266 retVal = new ca.uhn.fhir.jpa.rp.r4b.SearchParameterResourceProvider(); 3267 retVal.setContext(myFhirContext); 3268 retVal.setDao(daoSearchParameterR4B()); 3269 return retVal; 3270 } 3271 3272 @Bean(name="myServiceRequestDaoR4B") 3273 public 3274 IFhirResourceDao<org.hl7.fhir.r4b.model.ServiceRequest> 3275 daoServiceRequestR4B() { 3276 3277 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.ServiceRequest> retVal; 3278 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.ServiceRequest>(); 3279 retVal.setResourceType(org.hl7.fhir.r4b.model.ServiceRequest.class); 3280 retVal.setContext(myFhirContext); 3281 return retVal; 3282 } 3283 3284 @Bean(name="myServiceRequestRpR4B") 3285 @Lazy 3286 public ca.uhn.fhir.jpa.rp.r4b.ServiceRequestResourceProvider rpServiceRequestR4B() { 3287 ca.uhn.fhir.jpa.rp.r4b.ServiceRequestResourceProvider retVal; 3288 retVal = new ca.uhn.fhir.jpa.rp.r4b.ServiceRequestResourceProvider(); 3289 retVal.setContext(myFhirContext); 3290 retVal.setDao(daoServiceRequestR4B()); 3291 return retVal; 3292 } 3293 3294 @Bean(name="mySlotDaoR4B") 3295 public 3296 IFhirResourceDao<org.hl7.fhir.r4b.model.Slot> 3297 daoSlotR4B() { 3298 3299 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Slot> retVal; 3300 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Slot>(); 3301 retVal.setResourceType(org.hl7.fhir.r4b.model.Slot.class); 3302 retVal.setContext(myFhirContext); 3303 return retVal; 3304 } 3305 3306 @Bean(name="mySlotRpR4B") 3307 @Lazy 3308 public ca.uhn.fhir.jpa.rp.r4b.SlotResourceProvider rpSlotR4B() { 3309 ca.uhn.fhir.jpa.rp.r4b.SlotResourceProvider retVal; 3310 retVal = new ca.uhn.fhir.jpa.rp.r4b.SlotResourceProvider(); 3311 retVal.setContext(myFhirContext); 3312 retVal.setDao(daoSlotR4B()); 3313 return retVal; 3314 } 3315 3316 @Bean(name="mySpecimenDaoR4B") 3317 public 3318 IFhirResourceDao<org.hl7.fhir.r4b.model.Specimen> 3319 daoSpecimenR4B() { 3320 3321 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Specimen> retVal; 3322 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Specimen>(); 3323 retVal.setResourceType(org.hl7.fhir.r4b.model.Specimen.class); 3324 retVal.setContext(myFhirContext); 3325 return retVal; 3326 } 3327 3328 @Bean(name="mySpecimenRpR4B") 3329 @Lazy 3330 public ca.uhn.fhir.jpa.rp.r4b.SpecimenResourceProvider rpSpecimenR4B() { 3331 ca.uhn.fhir.jpa.rp.r4b.SpecimenResourceProvider retVal; 3332 retVal = new ca.uhn.fhir.jpa.rp.r4b.SpecimenResourceProvider(); 3333 retVal.setContext(myFhirContext); 3334 retVal.setDao(daoSpecimenR4B()); 3335 return retVal; 3336 } 3337 3338 @Bean(name="mySpecimenDefinitionDaoR4B") 3339 public 3340 IFhirResourceDao<org.hl7.fhir.r4b.model.SpecimenDefinition> 3341 daoSpecimenDefinitionR4B() { 3342 3343 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.SpecimenDefinition> retVal; 3344 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.SpecimenDefinition>(); 3345 retVal.setResourceType(org.hl7.fhir.r4b.model.SpecimenDefinition.class); 3346 retVal.setContext(myFhirContext); 3347 return retVal; 3348 } 3349 3350 @Bean(name="mySpecimenDefinitionRpR4B") 3351 @Lazy 3352 public ca.uhn.fhir.jpa.rp.r4b.SpecimenDefinitionResourceProvider rpSpecimenDefinitionR4B() { 3353 ca.uhn.fhir.jpa.rp.r4b.SpecimenDefinitionResourceProvider retVal; 3354 retVal = new ca.uhn.fhir.jpa.rp.r4b.SpecimenDefinitionResourceProvider(); 3355 retVal.setContext(myFhirContext); 3356 retVal.setDao(daoSpecimenDefinitionR4B()); 3357 return retVal; 3358 } 3359 3360 @Bean(name="myStructureDefinitionDaoR4B") 3361 public 3362 IFhirResourceDaoStructureDefinition<org.hl7.fhir.r4b.model.StructureDefinition> 3363 daoStructureDefinitionR4B() { 3364 3365 ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<org.hl7.fhir.r4b.model.StructureDefinition> retVal; 3366 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<>(); 3367 retVal.setResourceType(org.hl7.fhir.r4b.model.StructureDefinition.class); 3368 retVal.setContext(myFhirContext); 3369 return retVal; 3370 } 3371 3372 @Bean(name="myStructureDefinitionRpR4B") 3373 @Lazy 3374 public ca.uhn.fhir.jpa.rp.r4b.StructureDefinitionResourceProvider rpStructureDefinitionR4B() { 3375 ca.uhn.fhir.jpa.rp.r4b.StructureDefinitionResourceProvider retVal; 3376 retVal = new ca.uhn.fhir.jpa.rp.r4b.StructureDefinitionResourceProvider(); 3377 retVal.setContext(myFhirContext); 3378 retVal.setDao(daoStructureDefinitionR4B()); 3379 return retVal; 3380 } 3381 3382 @Bean(name="myStructureMapDaoR4B") 3383 public 3384 IFhirResourceDao<org.hl7.fhir.r4b.model.StructureMap> 3385 daoStructureMapR4B() { 3386 3387 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.StructureMap> retVal; 3388 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.StructureMap>(); 3389 retVal.setResourceType(org.hl7.fhir.r4b.model.StructureMap.class); 3390 retVal.setContext(myFhirContext); 3391 return retVal; 3392 } 3393 3394 @Bean(name="myStructureMapRpR4B") 3395 @Lazy 3396 public ca.uhn.fhir.jpa.rp.r4b.StructureMapResourceProvider rpStructureMapR4B() { 3397 ca.uhn.fhir.jpa.rp.r4b.StructureMapResourceProvider retVal; 3398 retVal = new ca.uhn.fhir.jpa.rp.r4b.StructureMapResourceProvider(); 3399 retVal.setContext(myFhirContext); 3400 retVal.setDao(daoStructureMapR4B()); 3401 return retVal; 3402 } 3403 3404 @Bean(name="mySubscriptionDaoR4B") 3405 public 3406 IFhirResourceDaoSubscription<org.hl7.fhir.r4b.model.Subscription> 3407 daoSubscriptionR4B() { 3408 3409 ca.uhn.fhir.jpa.dao.r4b.FhirResourceDaoSubscriptionR4B retVal; 3410 retVal = new ca.uhn.fhir.jpa.dao.r4b.FhirResourceDaoSubscriptionR4B(); 3411 retVal.setResourceType(org.hl7.fhir.r4b.model.Subscription.class); 3412 retVal.setContext(myFhirContext); 3413 return retVal; 3414 } 3415 3416 @Bean(name="mySubscriptionRpR4B") 3417 @Lazy 3418 public ca.uhn.fhir.jpa.rp.r4b.SubscriptionResourceProvider rpSubscriptionR4B() { 3419 ca.uhn.fhir.jpa.rp.r4b.SubscriptionResourceProvider retVal; 3420 retVal = new ca.uhn.fhir.jpa.rp.r4b.SubscriptionResourceProvider(); 3421 retVal.setContext(myFhirContext); 3422 retVal.setDao(daoSubscriptionR4B()); 3423 return retVal; 3424 } 3425 3426 @Bean(name="mySubscriptionStatusDaoR4B") 3427 public 3428 IFhirResourceDao<org.hl7.fhir.r4b.model.SubscriptionStatus> 3429 daoSubscriptionStatusR4B() { 3430 3431 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.SubscriptionStatus> retVal; 3432 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.SubscriptionStatus>(); 3433 retVal.setResourceType(org.hl7.fhir.r4b.model.SubscriptionStatus.class); 3434 retVal.setContext(myFhirContext); 3435 return retVal; 3436 } 3437 3438 @Bean(name="mySubscriptionStatusRpR4B") 3439 @Lazy 3440 public ca.uhn.fhir.jpa.rp.r4b.SubscriptionStatusResourceProvider rpSubscriptionStatusR4B() { 3441 ca.uhn.fhir.jpa.rp.r4b.SubscriptionStatusResourceProvider retVal; 3442 retVal = new ca.uhn.fhir.jpa.rp.r4b.SubscriptionStatusResourceProvider(); 3443 retVal.setContext(myFhirContext); 3444 retVal.setDao(daoSubscriptionStatusR4B()); 3445 return retVal; 3446 } 3447 3448 @Bean(name="mySubscriptionTopicDaoR4B") 3449 public 3450 IFhirResourceDao<org.hl7.fhir.r4b.model.SubscriptionTopic> 3451 daoSubscriptionTopicR4B() { 3452 3453 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.SubscriptionTopic> retVal; 3454 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.SubscriptionTopic>(); 3455 retVal.setResourceType(org.hl7.fhir.r4b.model.SubscriptionTopic.class); 3456 retVal.setContext(myFhirContext); 3457 return retVal; 3458 } 3459 3460 @Bean(name="mySubscriptionTopicRpR4B") 3461 @Lazy 3462 public ca.uhn.fhir.jpa.rp.r4b.SubscriptionTopicResourceProvider rpSubscriptionTopicR4B() { 3463 ca.uhn.fhir.jpa.rp.r4b.SubscriptionTopicResourceProvider retVal; 3464 retVal = new ca.uhn.fhir.jpa.rp.r4b.SubscriptionTopicResourceProvider(); 3465 retVal.setContext(myFhirContext); 3466 retVal.setDao(daoSubscriptionTopicR4B()); 3467 return retVal; 3468 } 3469 3470 @Bean(name="mySubstanceDaoR4B") 3471 public 3472 IFhirResourceDao<org.hl7.fhir.r4b.model.Substance> 3473 daoSubstanceR4B() { 3474 3475 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Substance> retVal; 3476 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Substance>(); 3477 retVal.setResourceType(org.hl7.fhir.r4b.model.Substance.class); 3478 retVal.setContext(myFhirContext); 3479 return retVal; 3480 } 3481 3482 @Bean(name="mySubstanceRpR4B") 3483 @Lazy 3484 public ca.uhn.fhir.jpa.rp.r4b.SubstanceResourceProvider rpSubstanceR4B() { 3485 ca.uhn.fhir.jpa.rp.r4b.SubstanceResourceProvider retVal; 3486 retVal = new ca.uhn.fhir.jpa.rp.r4b.SubstanceResourceProvider(); 3487 retVal.setContext(myFhirContext); 3488 retVal.setDao(daoSubstanceR4B()); 3489 return retVal; 3490 } 3491 3492 @Bean(name="mySubstanceDefinitionDaoR4B") 3493 public 3494 IFhirResourceDao<org.hl7.fhir.r4b.model.SubstanceDefinition> 3495 daoSubstanceDefinitionR4B() { 3496 3497 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.SubstanceDefinition> retVal; 3498 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.SubstanceDefinition>(); 3499 retVal.setResourceType(org.hl7.fhir.r4b.model.SubstanceDefinition.class); 3500 retVal.setContext(myFhirContext); 3501 return retVal; 3502 } 3503 3504 @Bean(name="mySubstanceDefinitionRpR4B") 3505 @Lazy 3506 public ca.uhn.fhir.jpa.rp.r4b.SubstanceDefinitionResourceProvider rpSubstanceDefinitionR4B() { 3507 ca.uhn.fhir.jpa.rp.r4b.SubstanceDefinitionResourceProvider retVal; 3508 retVal = new ca.uhn.fhir.jpa.rp.r4b.SubstanceDefinitionResourceProvider(); 3509 retVal.setContext(myFhirContext); 3510 retVal.setDao(daoSubstanceDefinitionR4B()); 3511 return retVal; 3512 } 3513 3514 @Bean(name="mySupplyDeliveryDaoR4B") 3515 public 3516 IFhirResourceDao<org.hl7.fhir.r4b.model.SupplyDelivery> 3517 daoSupplyDeliveryR4B() { 3518 3519 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.SupplyDelivery> retVal; 3520 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.SupplyDelivery>(); 3521 retVal.setResourceType(org.hl7.fhir.r4b.model.SupplyDelivery.class); 3522 retVal.setContext(myFhirContext); 3523 return retVal; 3524 } 3525 3526 @Bean(name="mySupplyDeliveryRpR4B") 3527 @Lazy 3528 public ca.uhn.fhir.jpa.rp.r4b.SupplyDeliveryResourceProvider rpSupplyDeliveryR4B() { 3529 ca.uhn.fhir.jpa.rp.r4b.SupplyDeliveryResourceProvider retVal; 3530 retVal = new ca.uhn.fhir.jpa.rp.r4b.SupplyDeliveryResourceProvider(); 3531 retVal.setContext(myFhirContext); 3532 retVal.setDao(daoSupplyDeliveryR4B()); 3533 return retVal; 3534 } 3535 3536 @Bean(name="mySupplyRequestDaoR4B") 3537 public 3538 IFhirResourceDao<org.hl7.fhir.r4b.model.SupplyRequest> 3539 daoSupplyRequestR4B() { 3540 3541 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.SupplyRequest> retVal; 3542 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.SupplyRequest>(); 3543 retVal.setResourceType(org.hl7.fhir.r4b.model.SupplyRequest.class); 3544 retVal.setContext(myFhirContext); 3545 return retVal; 3546 } 3547 3548 @Bean(name="mySupplyRequestRpR4B") 3549 @Lazy 3550 public ca.uhn.fhir.jpa.rp.r4b.SupplyRequestResourceProvider rpSupplyRequestR4B() { 3551 ca.uhn.fhir.jpa.rp.r4b.SupplyRequestResourceProvider retVal; 3552 retVal = new ca.uhn.fhir.jpa.rp.r4b.SupplyRequestResourceProvider(); 3553 retVal.setContext(myFhirContext); 3554 retVal.setDao(daoSupplyRequestR4B()); 3555 return retVal; 3556 } 3557 3558 @Bean(name="myTaskDaoR4B") 3559 public 3560 IFhirResourceDao<org.hl7.fhir.r4b.model.Task> 3561 daoTaskR4B() { 3562 3563 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.Task> retVal; 3564 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.Task>(); 3565 retVal.setResourceType(org.hl7.fhir.r4b.model.Task.class); 3566 retVal.setContext(myFhirContext); 3567 return retVal; 3568 } 3569 3570 @Bean(name="myTaskRpR4B") 3571 @Lazy 3572 public ca.uhn.fhir.jpa.rp.r4b.TaskResourceProvider rpTaskR4B() { 3573 ca.uhn.fhir.jpa.rp.r4b.TaskResourceProvider retVal; 3574 retVal = new ca.uhn.fhir.jpa.rp.r4b.TaskResourceProvider(); 3575 retVal.setContext(myFhirContext); 3576 retVal.setDao(daoTaskR4B()); 3577 return retVal; 3578 } 3579 3580 @Bean(name="myTerminologyCapabilitiesDaoR4B") 3581 public 3582 IFhirResourceDao<org.hl7.fhir.r4b.model.TerminologyCapabilities> 3583 daoTerminologyCapabilitiesR4B() { 3584 3585 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.TerminologyCapabilities> retVal; 3586 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.TerminologyCapabilities>(); 3587 retVal.setResourceType(org.hl7.fhir.r4b.model.TerminologyCapabilities.class); 3588 retVal.setContext(myFhirContext); 3589 return retVal; 3590 } 3591 3592 @Bean(name="myTerminologyCapabilitiesRpR4B") 3593 @Lazy 3594 public ca.uhn.fhir.jpa.rp.r4b.TerminologyCapabilitiesResourceProvider rpTerminologyCapabilitiesR4B() { 3595 ca.uhn.fhir.jpa.rp.r4b.TerminologyCapabilitiesResourceProvider retVal; 3596 retVal = new ca.uhn.fhir.jpa.rp.r4b.TerminologyCapabilitiesResourceProvider(); 3597 retVal.setContext(myFhirContext); 3598 retVal.setDao(daoTerminologyCapabilitiesR4B()); 3599 return retVal; 3600 } 3601 3602 @Bean(name="myTestReportDaoR4B") 3603 public 3604 IFhirResourceDao<org.hl7.fhir.r4b.model.TestReport> 3605 daoTestReportR4B() { 3606 3607 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.TestReport> retVal; 3608 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.TestReport>(); 3609 retVal.setResourceType(org.hl7.fhir.r4b.model.TestReport.class); 3610 retVal.setContext(myFhirContext); 3611 return retVal; 3612 } 3613 3614 @Bean(name="myTestReportRpR4B") 3615 @Lazy 3616 public ca.uhn.fhir.jpa.rp.r4b.TestReportResourceProvider rpTestReportR4B() { 3617 ca.uhn.fhir.jpa.rp.r4b.TestReportResourceProvider retVal; 3618 retVal = new ca.uhn.fhir.jpa.rp.r4b.TestReportResourceProvider(); 3619 retVal.setContext(myFhirContext); 3620 retVal.setDao(daoTestReportR4B()); 3621 return retVal; 3622 } 3623 3624 @Bean(name="myTestScriptDaoR4B") 3625 public 3626 IFhirResourceDao<org.hl7.fhir.r4b.model.TestScript> 3627 daoTestScriptR4B() { 3628 3629 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.TestScript> retVal; 3630 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.TestScript>(); 3631 retVal.setResourceType(org.hl7.fhir.r4b.model.TestScript.class); 3632 retVal.setContext(myFhirContext); 3633 return retVal; 3634 } 3635 3636 @Bean(name="myTestScriptRpR4B") 3637 @Lazy 3638 public ca.uhn.fhir.jpa.rp.r4b.TestScriptResourceProvider rpTestScriptR4B() { 3639 ca.uhn.fhir.jpa.rp.r4b.TestScriptResourceProvider retVal; 3640 retVal = new ca.uhn.fhir.jpa.rp.r4b.TestScriptResourceProvider(); 3641 retVal.setContext(myFhirContext); 3642 retVal.setDao(daoTestScriptR4B()); 3643 return retVal; 3644 } 3645 3646 @Bean(name="myValueSetDaoR4B") 3647 public 3648 IFhirResourceDaoValueSet<org.hl7.fhir.r4b.model.ValueSet> 3649 daoValueSetR4B() { 3650 3651 ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<org.hl7.fhir.r4b.model.ValueSet> retVal; 3652 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<>(); 3653 retVal.setResourceType(org.hl7.fhir.r4b.model.ValueSet.class); 3654 retVal.setContext(myFhirContext); 3655 return retVal; 3656 } 3657 3658 @Bean(name="myValueSetRpR4B") 3659 @Lazy 3660 public ca.uhn.fhir.jpa.rp.r4b.ValueSetResourceProvider rpValueSetR4B() { 3661 ca.uhn.fhir.jpa.rp.r4b.ValueSetResourceProvider retVal; 3662 retVal = new ca.uhn.fhir.jpa.rp.r4b.ValueSetResourceProvider(); 3663 retVal.setContext(myFhirContext); 3664 retVal.setDao(daoValueSetR4B()); 3665 return retVal; 3666 } 3667 3668 @Bean(name="myVerificationResultDaoR4B") 3669 public 3670 IFhirResourceDao<org.hl7.fhir.r4b.model.VerificationResult> 3671 daoVerificationResultR4B() { 3672 3673 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.VerificationResult> retVal; 3674 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.VerificationResult>(); 3675 retVal.setResourceType(org.hl7.fhir.r4b.model.VerificationResult.class); 3676 retVal.setContext(myFhirContext); 3677 return retVal; 3678 } 3679 3680 @Bean(name="myVerificationResultRpR4B") 3681 @Lazy 3682 public ca.uhn.fhir.jpa.rp.r4b.VerificationResultResourceProvider rpVerificationResultR4B() { 3683 ca.uhn.fhir.jpa.rp.r4b.VerificationResultResourceProvider retVal; 3684 retVal = new ca.uhn.fhir.jpa.rp.r4b.VerificationResultResourceProvider(); 3685 retVal.setContext(myFhirContext); 3686 retVal.setDao(daoVerificationResultR4B()); 3687 return retVal; 3688 } 3689 3690 @Bean(name="myVisionPrescriptionDaoR4B") 3691 public 3692 IFhirResourceDao<org.hl7.fhir.r4b.model.VisionPrescription> 3693 daoVisionPrescriptionR4B() { 3694 3695 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r4b.model.VisionPrescription> retVal; 3696 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r4b.model.VisionPrescription>(); 3697 retVal.setResourceType(org.hl7.fhir.r4b.model.VisionPrescription.class); 3698 retVal.setContext(myFhirContext); 3699 return retVal; 3700 } 3701 3702 @Bean(name="myVisionPrescriptionRpR4B") 3703 @Lazy 3704 public ca.uhn.fhir.jpa.rp.r4b.VisionPrescriptionResourceProvider rpVisionPrescriptionR4B() { 3705 ca.uhn.fhir.jpa.rp.r4b.VisionPrescriptionResourceProvider retVal; 3706 retVal = new ca.uhn.fhir.jpa.rp.r4b.VisionPrescriptionResourceProvider(); 3707 retVal.setContext(myFhirContext); 3708 retVal.setDao(daoVisionPrescriptionR4B()); 3709 return retVal; 3710 } 3711 3712 3713 3714 3715 3716 3717}