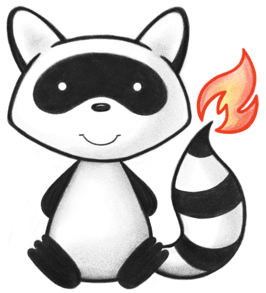
001package ca.uhn.fhir.jpa.config; 002 003import java.util.ArrayList; 004import java.util.List; 005import java.util.concurrent.Executor; 006import java.util.concurrent.Executors; 007 008import org.springframework.transaction.PlatformTransactionManager; 009import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 010 011import org.springframework.beans.factory.annotation.Autowired; 012import org.springframework.context.annotation.Bean; 013import org.springframework.context.annotation.Configuration; 014import org.springframework.context.annotation.Lazy; 015import org.springframework.data.jpa.repository.config.EnableJpaRepositories; 016import org.springframework.scheduling.annotation.EnableScheduling; 017import org.springframework.scheduling.annotation.SchedulingConfigurer; 018import org.springframework.scheduling.config.ScheduledTaskRegistrar; 019 020import ca.uhn.fhir.rest.api.IResourceSupportedSvc; 021import ca.uhn.fhir.context.FhirContext; 022import ca.uhn.fhir.model.api.IResource; 023import ca.uhn.fhir.rest.server.IResourceProvider; 024import ca.uhn.fhir.jpa.api.dao.*; 025import ca.uhn.fhir.jpa.dao.*; 026import ca.uhn.fhir.rest.server.provider.ResourceProviderFactory; 027 028@Configuration 029public class GeneratedDaoAndResourceProviderConfigR5 { 030@Autowired 031FhirContext myFhirContext; 032 033 @Bean(name="myResourceProvidersR5") 034 public ResourceProviderFactory resourceProvidersR5(IResourceSupportedSvc theResourceSupportedSvc) { 035 ResourceProviderFactory retVal = new ResourceProviderFactory(); 036 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Account") ? rpAccountR5() : null); 037 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ActivityDefinition") ? rpActivityDefinitionR5() : null); 038 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ActorDefinition") ? rpActorDefinitionR5() : null); 039 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AdministrableProductDefinition") ? rpAdministrableProductDefinitionR5() : null); 040 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AdverseEvent") ? rpAdverseEventR5() : null); 041 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AllergyIntolerance") ? rpAllergyIntoleranceR5() : null); 042 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Appointment") ? rpAppointmentR5() : null); 043 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AppointmentResponse") ? rpAppointmentResponseR5() : null); 044 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ArtifactAssessment") ? rpArtifactAssessmentR5() : null); 045 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("AuditEvent") ? rpAuditEventR5() : null); 046 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Basic") ? rpBasicR5() : null); 047 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Binary") ? rpBinaryR5() : null); 048 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BiologicallyDerivedProduct") ? rpBiologicallyDerivedProductR5() : null); 049 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BiologicallyDerivedProductDispense") ? rpBiologicallyDerivedProductDispenseR5() : null); 050 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("BodyStructure") ? rpBodyStructureR5() : null); 051 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Bundle") ? rpBundleR5() : null); 052 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CapabilityStatement") ? rpCapabilityStatementR5() : null); 053 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CarePlan") ? rpCarePlanR5() : null); 054 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CareTeam") ? rpCareTeamR5() : null); 055 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ChargeItem") ? rpChargeItemR5() : null); 056 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ChargeItemDefinition") ? rpChargeItemDefinitionR5() : null); 057 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Citation") ? rpCitationR5() : null); 058 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Claim") ? rpClaimR5() : null); 059 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClaimResponse") ? rpClaimResponseR5() : null); 060 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClinicalImpression") ? rpClinicalImpressionR5() : null); 061 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ClinicalUseDefinition") ? rpClinicalUseDefinitionR5() : null); 062 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CodeSystem") ? rpCodeSystemR5() : null); 063 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Communication") ? rpCommunicationR5() : null); 064 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CommunicationRequest") ? rpCommunicationRequestR5() : null); 065 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CompartmentDefinition") ? rpCompartmentDefinitionR5() : null); 066 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Composition") ? rpCompositionR5() : null); 067 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ConceptMap") ? rpConceptMapR5() : null); 068 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Condition") ? rpConditionR5() : null); 069 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ConditionDefinition") ? rpConditionDefinitionR5() : null); 070 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Consent") ? rpConsentR5() : null); 071 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Contract") ? rpContractR5() : null); 072 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Coverage") ? rpCoverageR5() : null); 073 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CoverageEligibilityRequest") ? rpCoverageEligibilityRequestR5() : null); 074 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("CoverageEligibilityResponse") ? rpCoverageEligibilityResponseR5() : null); 075 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DetectedIssue") ? rpDetectedIssueR5() : null); 076 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Device") ? rpDeviceR5() : null); 077 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceAssociation") ? rpDeviceAssociationR5() : null); 078 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceDefinition") ? rpDeviceDefinitionR5() : null); 079 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceDispense") ? rpDeviceDispenseR5() : null); 080 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceMetric") ? rpDeviceMetricR5() : null); 081 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceRequest") ? rpDeviceRequestR5() : null); 082 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DeviceUsage") ? rpDeviceUsageR5() : null); 083 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DiagnosticReport") ? rpDiagnosticReportR5() : null); 084 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("DocumentReference") ? rpDocumentReferenceR5() : null); 085 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Encounter") ? rpEncounterR5() : null); 086 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EncounterHistory") ? rpEncounterHistoryR5() : null); 087 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Endpoint") ? rpEndpointR5() : null); 088 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentRequest") ? rpEnrollmentRequestR5() : null); 089 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EnrollmentResponse") ? rpEnrollmentResponseR5() : null); 090 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EpisodeOfCare") ? rpEpisodeOfCareR5() : null); 091 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EventDefinition") ? rpEventDefinitionR5() : null); 092 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Evidence") ? rpEvidenceR5() : null); 093 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EvidenceReport") ? rpEvidenceReportR5() : null); 094 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("EvidenceVariable") ? rpEvidenceVariableR5() : null); 095 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExampleScenario") ? rpExampleScenarioR5() : null); 096 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ExplanationOfBenefit") ? rpExplanationOfBenefitR5() : null); 097 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("FamilyMemberHistory") ? rpFamilyMemberHistoryR5() : null); 098 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Flag") ? rpFlagR5() : null); 099 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("FormularyItem") ? rpFormularyItemR5() : null); 100 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GenomicStudy") ? rpGenomicStudyR5() : null); 101 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Goal") ? rpGoalR5() : null); 102 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GraphDefinition") ? rpGraphDefinitionR5() : null); 103 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Group") ? rpGroupR5() : null); 104 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("GuidanceResponse") ? rpGuidanceResponseR5() : null); 105 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("HealthcareService") ? rpHealthcareServiceR5() : null); 106 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImagingSelection") ? rpImagingSelectionR5() : null); 107 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImagingStudy") ? rpImagingStudyR5() : null); 108 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Immunization") ? rpImmunizationR5() : null); 109 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImmunizationEvaluation") ? rpImmunizationEvaluationR5() : null); 110 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImmunizationRecommendation") ? rpImmunizationRecommendationR5() : null); 111 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ImplementationGuide") ? rpImplementationGuideR5() : null); 112 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Ingredient") ? rpIngredientR5() : null); 113 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("InsurancePlan") ? rpInsurancePlanR5() : null); 114 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("InventoryItem") ? rpInventoryItemR5() : null); 115 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("InventoryReport") ? rpInventoryReportR5() : null); 116 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Invoice") ? rpInvoiceR5() : null); 117 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Library") ? rpLibraryR5() : null); 118 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Linkage") ? rpLinkageR5() : null); 119 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("List") ? rpListResourceR5() : null); 120 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Location") ? rpLocationR5() : null); 121 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ManufacturedItemDefinition") ? rpManufacturedItemDefinitionR5() : null); 122 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Measure") ? rpMeasureR5() : null); 123 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MeasureReport") ? rpMeasureReportR5() : null); 124 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Medication") ? rpMedicationR5() : null); 125 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationAdministration") ? rpMedicationAdministrationR5() : null); 126 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationDispense") ? rpMedicationDispenseR5() : null); 127 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationKnowledge") ? rpMedicationKnowledgeR5() : null); 128 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationRequest") ? rpMedicationRequestR5() : null); 129 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicationStatement") ? rpMedicationStatementR5() : null); 130 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MedicinalProductDefinition") ? rpMedicinalProductDefinitionR5() : null); 131 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageDefinition") ? rpMessageDefinitionR5() : null); 132 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MessageHeader") ? rpMessageHeaderR5() : null); 133 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("MolecularSequence") ? rpMolecularSequenceR5() : null); 134 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NamingSystem") ? rpNamingSystemR5() : null); 135 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NutritionIntake") ? rpNutritionIntakeR5() : null); 136 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NutritionOrder") ? rpNutritionOrderR5() : null); 137 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("NutritionProduct") ? rpNutritionProductR5() : null); 138 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Observation") ? rpObservationR5() : null); 139 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ObservationDefinition") ? rpObservationDefinitionR5() : null); 140 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationDefinition") ? rpOperationDefinitionR5() : null); 141 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OperationOutcome") ? rpOperationOutcomeR5() : null); 142 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Organization") ? rpOrganizationR5() : null); 143 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("OrganizationAffiliation") ? rpOrganizationAffiliationR5() : null); 144 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PackagedProductDefinition") ? rpPackagedProductDefinitionR5() : null); 145 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Parameters") ? rpParametersR5() : null); 146 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Patient") ? rpPatientR5() : null); 147 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentNotice") ? rpPaymentNoticeR5() : null); 148 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PaymentReconciliation") ? rpPaymentReconciliationR5() : null); 149 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Permission") ? rpPermissionR5() : null); 150 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Person") ? rpPersonR5() : null); 151 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PlanDefinition") ? rpPlanDefinitionR5() : null); 152 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Practitioner") ? rpPractitionerR5() : null); 153 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("PractitionerRole") ? rpPractitionerRoleR5() : null); 154 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Procedure") ? rpProcedureR5() : null); 155 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Provenance") ? rpProvenanceR5() : null); 156 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Questionnaire") ? rpQuestionnaireR5() : null); 157 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("QuestionnaireResponse") ? rpQuestionnaireResponseR5() : null); 158 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RegulatedAuthorization") ? rpRegulatedAuthorizationR5() : null); 159 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RelatedPerson") ? rpRelatedPersonR5() : null); 160 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RequestOrchestration") ? rpRequestOrchestrationR5() : null); 161 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Requirements") ? rpRequirementsR5() : null); 162 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchStudy") ? rpResearchStudyR5() : null); 163 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ResearchSubject") ? rpResearchSubjectR5() : null); 164 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("RiskAssessment") ? rpRiskAssessmentR5() : null); 165 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Schedule") ? rpScheduleR5() : null); 166 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SearchParameter") ? rpSearchParameterR5() : null); 167 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ServiceRequest") ? rpServiceRequestR5() : null); 168 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Slot") ? rpSlotR5() : null); 169 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Specimen") ? rpSpecimenR5() : null); 170 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SpecimenDefinition") ? rpSpecimenDefinitionR5() : null); 171 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureDefinition") ? rpStructureDefinitionR5() : null); 172 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("StructureMap") ? rpStructureMapR5() : null); 173 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Subscription") ? rpSubscriptionR5() : null); 174 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubscriptionStatus") ? rpSubscriptionStatusR5() : null); 175 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubscriptionTopic") ? rpSubscriptionTopicR5() : null); 176 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Substance") ? rpSubstanceR5() : null); 177 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceDefinition") ? rpSubstanceDefinitionR5() : null); 178 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceNucleicAcid") ? rpSubstanceNucleicAcidR5() : null); 179 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstancePolymer") ? rpSubstancePolymerR5() : null); 180 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceProtein") ? rpSubstanceProteinR5() : null); 181 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceReferenceInformation") ? rpSubstanceReferenceInformationR5() : null); 182 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SubstanceSourceMaterial") ? rpSubstanceSourceMaterialR5() : null); 183 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyDelivery") ? rpSupplyDeliveryR5() : null); 184 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("SupplyRequest") ? rpSupplyRequestR5() : null); 185 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Task") ? rpTaskR5() : null); 186 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TerminologyCapabilities") ? rpTerminologyCapabilitiesR5() : null); 187 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestPlan") ? rpTestPlanR5() : null); 188 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestReport") ? rpTestReportR5() : null); 189 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("TestScript") ? rpTestScriptR5() : null); 190 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("Transport") ? rpTransportR5() : null); 191 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("ValueSet") ? rpValueSetR5() : null); 192 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("VerificationResult") ? rpVerificationResultR5() : null); 193 retVal.addSupplier(() -> theResourceSupportedSvc.isSupported("VisionPrescription") ? rpVisionPrescriptionR5() : null); 194 return retVal; 195 } 196 197 198 @Bean(name="myResourceDaosR5") 199 public List<IFhirResourceDao<?>> resourceDaosR5(IResourceSupportedSvc theResourceSupportedSvc) { 200 List<IFhirResourceDao<?>> retVal = new ArrayList<IFhirResourceDao<?>>(); 201 if (theResourceSupportedSvc.isSupported("Account")) { 202 retVal.add(daoAccountR5()); 203 } 204 if (theResourceSupportedSvc.isSupported("ActivityDefinition")) { 205 retVal.add(daoActivityDefinitionR5()); 206 } 207 if (theResourceSupportedSvc.isSupported("ActorDefinition")) { 208 retVal.add(daoActorDefinitionR5()); 209 } 210 if (theResourceSupportedSvc.isSupported("AdministrableProductDefinition")) { 211 retVal.add(daoAdministrableProductDefinitionR5()); 212 } 213 if (theResourceSupportedSvc.isSupported("AdverseEvent")) { 214 retVal.add(daoAdverseEventR5()); 215 } 216 if (theResourceSupportedSvc.isSupported("AllergyIntolerance")) { 217 retVal.add(daoAllergyIntoleranceR5()); 218 } 219 if (theResourceSupportedSvc.isSupported("Appointment")) { 220 retVal.add(daoAppointmentR5()); 221 } 222 if (theResourceSupportedSvc.isSupported("AppointmentResponse")) { 223 retVal.add(daoAppointmentResponseR5()); 224 } 225 if (theResourceSupportedSvc.isSupported("ArtifactAssessment")) { 226 retVal.add(daoArtifactAssessmentR5()); 227 } 228 if (theResourceSupportedSvc.isSupported("AuditEvent")) { 229 retVal.add(daoAuditEventR5()); 230 } 231 if (theResourceSupportedSvc.isSupported("Basic")) { 232 retVal.add(daoBasicR5()); 233 } 234 if (theResourceSupportedSvc.isSupported("Binary")) { 235 retVal.add(daoBinaryR5()); 236 } 237 if (theResourceSupportedSvc.isSupported("BiologicallyDerivedProduct")) { 238 retVal.add(daoBiologicallyDerivedProductR5()); 239 } 240 if (theResourceSupportedSvc.isSupported("BiologicallyDerivedProductDispense")) { 241 retVal.add(daoBiologicallyDerivedProductDispenseR5()); 242 } 243 if (theResourceSupportedSvc.isSupported("BodyStructure")) { 244 retVal.add(daoBodyStructureR5()); 245 } 246 if (theResourceSupportedSvc.isSupported("Bundle")) { 247 retVal.add(daoBundleR5()); 248 } 249 if (theResourceSupportedSvc.isSupported("CapabilityStatement")) { 250 retVal.add(daoCapabilityStatementR5()); 251 } 252 if (theResourceSupportedSvc.isSupported("CarePlan")) { 253 retVal.add(daoCarePlanR5()); 254 } 255 if (theResourceSupportedSvc.isSupported("CareTeam")) { 256 retVal.add(daoCareTeamR5()); 257 } 258 if (theResourceSupportedSvc.isSupported("ChargeItem")) { 259 retVal.add(daoChargeItemR5()); 260 } 261 if (theResourceSupportedSvc.isSupported("ChargeItemDefinition")) { 262 retVal.add(daoChargeItemDefinitionR5()); 263 } 264 if (theResourceSupportedSvc.isSupported("Citation")) { 265 retVal.add(daoCitationR5()); 266 } 267 if (theResourceSupportedSvc.isSupported("Claim")) { 268 retVal.add(daoClaimR5()); 269 } 270 if (theResourceSupportedSvc.isSupported("ClaimResponse")) { 271 retVal.add(daoClaimResponseR5()); 272 } 273 if (theResourceSupportedSvc.isSupported("ClinicalImpression")) { 274 retVal.add(daoClinicalImpressionR5()); 275 } 276 if (theResourceSupportedSvc.isSupported("ClinicalUseDefinition")) { 277 retVal.add(daoClinicalUseDefinitionR5()); 278 } 279 if (theResourceSupportedSvc.isSupported("CodeSystem")) { 280 retVal.add(daoCodeSystemR5()); 281 } 282 if (theResourceSupportedSvc.isSupported("Communication")) { 283 retVal.add(daoCommunicationR5()); 284 } 285 if (theResourceSupportedSvc.isSupported("CommunicationRequest")) { 286 retVal.add(daoCommunicationRequestR5()); 287 } 288 if (theResourceSupportedSvc.isSupported("CompartmentDefinition")) { 289 retVal.add(daoCompartmentDefinitionR5()); 290 } 291 if (theResourceSupportedSvc.isSupported("Composition")) { 292 retVal.add(daoCompositionR5()); 293 } 294 if (theResourceSupportedSvc.isSupported("ConceptMap")) { 295 retVal.add(daoConceptMapR5()); 296 } 297 if (theResourceSupportedSvc.isSupported("Condition")) { 298 retVal.add(daoConditionR5()); 299 } 300 if (theResourceSupportedSvc.isSupported("ConditionDefinition")) { 301 retVal.add(daoConditionDefinitionR5()); 302 } 303 if (theResourceSupportedSvc.isSupported("Consent")) { 304 retVal.add(daoConsentR5()); 305 } 306 if (theResourceSupportedSvc.isSupported("Contract")) { 307 retVal.add(daoContractR5()); 308 } 309 if (theResourceSupportedSvc.isSupported("Coverage")) { 310 retVal.add(daoCoverageR5()); 311 } 312 if (theResourceSupportedSvc.isSupported("CoverageEligibilityRequest")) { 313 retVal.add(daoCoverageEligibilityRequestR5()); 314 } 315 if (theResourceSupportedSvc.isSupported("CoverageEligibilityResponse")) { 316 retVal.add(daoCoverageEligibilityResponseR5()); 317 } 318 if (theResourceSupportedSvc.isSupported("DetectedIssue")) { 319 retVal.add(daoDetectedIssueR5()); 320 } 321 if (theResourceSupportedSvc.isSupported("Device")) { 322 retVal.add(daoDeviceR5()); 323 } 324 if (theResourceSupportedSvc.isSupported("DeviceAssociation")) { 325 retVal.add(daoDeviceAssociationR5()); 326 } 327 if (theResourceSupportedSvc.isSupported("DeviceDefinition")) { 328 retVal.add(daoDeviceDefinitionR5()); 329 } 330 if (theResourceSupportedSvc.isSupported("DeviceDispense")) { 331 retVal.add(daoDeviceDispenseR5()); 332 } 333 if (theResourceSupportedSvc.isSupported("DeviceMetric")) { 334 retVal.add(daoDeviceMetricR5()); 335 } 336 if (theResourceSupportedSvc.isSupported("DeviceRequest")) { 337 retVal.add(daoDeviceRequestR5()); 338 } 339 if (theResourceSupportedSvc.isSupported("DeviceUsage")) { 340 retVal.add(daoDeviceUsageR5()); 341 } 342 if (theResourceSupportedSvc.isSupported("DiagnosticReport")) { 343 retVal.add(daoDiagnosticReportR5()); 344 } 345 if (theResourceSupportedSvc.isSupported("DocumentReference")) { 346 retVal.add(daoDocumentReferenceR5()); 347 } 348 if (theResourceSupportedSvc.isSupported("Encounter")) { 349 retVal.add(daoEncounterR5()); 350 } 351 if (theResourceSupportedSvc.isSupported("EncounterHistory")) { 352 retVal.add(daoEncounterHistoryR5()); 353 } 354 if (theResourceSupportedSvc.isSupported("Endpoint")) { 355 retVal.add(daoEndpointR5()); 356 } 357 if (theResourceSupportedSvc.isSupported("EnrollmentRequest")) { 358 retVal.add(daoEnrollmentRequestR5()); 359 } 360 if (theResourceSupportedSvc.isSupported("EnrollmentResponse")) { 361 retVal.add(daoEnrollmentResponseR5()); 362 } 363 if (theResourceSupportedSvc.isSupported("EpisodeOfCare")) { 364 retVal.add(daoEpisodeOfCareR5()); 365 } 366 if (theResourceSupportedSvc.isSupported("EventDefinition")) { 367 retVal.add(daoEventDefinitionR5()); 368 } 369 if (theResourceSupportedSvc.isSupported("Evidence")) { 370 retVal.add(daoEvidenceR5()); 371 } 372 if (theResourceSupportedSvc.isSupported("EvidenceReport")) { 373 retVal.add(daoEvidenceReportR5()); 374 } 375 if (theResourceSupportedSvc.isSupported("EvidenceVariable")) { 376 retVal.add(daoEvidenceVariableR5()); 377 } 378 if (theResourceSupportedSvc.isSupported("ExampleScenario")) { 379 retVal.add(daoExampleScenarioR5()); 380 } 381 if (theResourceSupportedSvc.isSupported("ExplanationOfBenefit")) { 382 retVal.add(daoExplanationOfBenefitR5()); 383 } 384 if (theResourceSupportedSvc.isSupported("FamilyMemberHistory")) { 385 retVal.add(daoFamilyMemberHistoryR5()); 386 } 387 if (theResourceSupportedSvc.isSupported("Flag")) { 388 retVal.add(daoFlagR5()); 389 } 390 if (theResourceSupportedSvc.isSupported("FormularyItem")) { 391 retVal.add(daoFormularyItemR5()); 392 } 393 if (theResourceSupportedSvc.isSupported("GenomicStudy")) { 394 retVal.add(daoGenomicStudyR5()); 395 } 396 if (theResourceSupportedSvc.isSupported("Goal")) { 397 retVal.add(daoGoalR5()); 398 } 399 if (theResourceSupportedSvc.isSupported("GraphDefinition")) { 400 retVal.add(daoGraphDefinitionR5()); 401 } 402 if (theResourceSupportedSvc.isSupported("Group")) { 403 retVal.add(daoGroupR5()); 404 } 405 if (theResourceSupportedSvc.isSupported("GuidanceResponse")) { 406 retVal.add(daoGuidanceResponseR5()); 407 } 408 if (theResourceSupportedSvc.isSupported("HealthcareService")) { 409 retVal.add(daoHealthcareServiceR5()); 410 } 411 if (theResourceSupportedSvc.isSupported("ImagingSelection")) { 412 retVal.add(daoImagingSelectionR5()); 413 } 414 if (theResourceSupportedSvc.isSupported("ImagingStudy")) { 415 retVal.add(daoImagingStudyR5()); 416 } 417 if (theResourceSupportedSvc.isSupported("Immunization")) { 418 retVal.add(daoImmunizationR5()); 419 } 420 if (theResourceSupportedSvc.isSupported("ImmunizationEvaluation")) { 421 retVal.add(daoImmunizationEvaluationR5()); 422 } 423 if (theResourceSupportedSvc.isSupported("ImmunizationRecommendation")) { 424 retVal.add(daoImmunizationRecommendationR5()); 425 } 426 if (theResourceSupportedSvc.isSupported("ImplementationGuide")) { 427 retVal.add(daoImplementationGuideR5()); 428 } 429 if (theResourceSupportedSvc.isSupported("Ingredient")) { 430 retVal.add(daoIngredientR5()); 431 } 432 if (theResourceSupportedSvc.isSupported("InsurancePlan")) { 433 retVal.add(daoInsurancePlanR5()); 434 } 435 if (theResourceSupportedSvc.isSupported("InventoryItem")) { 436 retVal.add(daoInventoryItemR5()); 437 } 438 if (theResourceSupportedSvc.isSupported("InventoryReport")) { 439 retVal.add(daoInventoryReportR5()); 440 } 441 if (theResourceSupportedSvc.isSupported("Invoice")) { 442 retVal.add(daoInvoiceR5()); 443 } 444 if (theResourceSupportedSvc.isSupported("Library")) { 445 retVal.add(daoLibraryR5()); 446 } 447 if (theResourceSupportedSvc.isSupported("Linkage")) { 448 retVal.add(daoLinkageR5()); 449 } 450 if (theResourceSupportedSvc.isSupported("List")) { 451 retVal.add(daoListResourceR5()); 452 } 453 if (theResourceSupportedSvc.isSupported("Location")) { 454 retVal.add(daoLocationR5()); 455 } 456 if (theResourceSupportedSvc.isSupported("ManufacturedItemDefinition")) { 457 retVal.add(daoManufacturedItemDefinitionR5()); 458 } 459 if (theResourceSupportedSvc.isSupported("Measure")) { 460 retVal.add(daoMeasureR5()); 461 } 462 if (theResourceSupportedSvc.isSupported("MeasureReport")) { 463 retVal.add(daoMeasureReportR5()); 464 } 465 if (theResourceSupportedSvc.isSupported("Medication")) { 466 retVal.add(daoMedicationR5()); 467 } 468 if (theResourceSupportedSvc.isSupported("MedicationAdministration")) { 469 retVal.add(daoMedicationAdministrationR5()); 470 } 471 if (theResourceSupportedSvc.isSupported("MedicationDispense")) { 472 retVal.add(daoMedicationDispenseR5()); 473 } 474 if (theResourceSupportedSvc.isSupported("MedicationKnowledge")) { 475 retVal.add(daoMedicationKnowledgeR5()); 476 } 477 if (theResourceSupportedSvc.isSupported("MedicationRequest")) { 478 retVal.add(daoMedicationRequestR5()); 479 } 480 if (theResourceSupportedSvc.isSupported("MedicationStatement")) { 481 retVal.add(daoMedicationStatementR5()); 482 } 483 if (theResourceSupportedSvc.isSupported("MedicinalProductDefinition")) { 484 retVal.add(daoMedicinalProductDefinitionR5()); 485 } 486 if (theResourceSupportedSvc.isSupported("MessageDefinition")) { 487 retVal.add(daoMessageDefinitionR5()); 488 } 489 if (theResourceSupportedSvc.isSupported("MessageHeader")) { 490 retVal.add(daoMessageHeaderR5()); 491 } 492 if (theResourceSupportedSvc.isSupported("MolecularSequence")) { 493 retVal.add(daoMolecularSequenceR5()); 494 } 495 if (theResourceSupportedSvc.isSupported("NamingSystem")) { 496 retVal.add(daoNamingSystemR5()); 497 } 498 if (theResourceSupportedSvc.isSupported("NutritionIntake")) { 499 retVal.add(daoNutritionIntakeR5()); 500 } 501 if (theResourceSupportedSvc.isSupported("NutritionOrder")) { 502 retVal.add(daoNutritionOrderR5()); 503 } 504 if (theResourceSupportedSvc.isSupported("NutritionProduct")) { 505 retVal.add(daoNutritionProductR5()); 506 } 507 if (theResourceSupportedSvc.isSupported("Observation")) { 508 retVal.add(daoObservationR5()); 509 } 510 if (theResourceSupportedSvc.isSupported("ObservationDefinition")) { 511 retVal.add(daoObservationDefinitionR5()); 512 } 513 if (theResourceSupportedSvc.isSupported("OperationDefinition")) { 514 retVal.add(daoOperationDefinitionR5()); 515 } 516 if (theResourceSupportedSvc.isSupported("OperationOutcome")) { 517 retVal.add(daoOperationOutcomeR5()); 518 } 519 if (theResourceSupportedSvc.isSupported("Organization")) { 520 retVal.add(daoOrganizationR5()); 521 } 522 if (theResourceSupportedSvc.isSupported("OrganizationAffiliation")) { 523 retVal.add(daoOrganizationAffiliationR5()); 524 } 525 if (theResourceSupportedSvc.isSupported("PackagedProductDefinition")) { 526 retVal.add(daoPackagedProductDefinitionR5()); 527 } 528 if (theResourceSupportedSvc.isSupported("Parameters")) { 529 retVal.add(daoParametersR5()); 530 } 531 if (theResourceSupportedSvc.isSupported("Patient")) { 532 retVal.add(daoPatientR5()); 533 } 534 if (theResourceSupportedSvc.isSupported("PaymentNotice")) { 535 retVal.add(daoPaymentNoticeR5()); 536 } 537 if (theResourceSupportedSvc.isSupported("PaymentReconciliation")) { 538 retVal.add(daoPaymentReconciliationR5()); 539 } 540 if (theResourceSupportedSvc.isSupported("Permission")) { 541 retVal.add(daoPermissionR5()); 542 } 543 if (theResourceSupportedSvc.isSupported("Person")) { 544 retVal.add(daoPersonR5()); 545 } 546 if (theResourceSupportedSvc.isSupported("PlanDefinition")) { 547 retVal.add(daoPlanDefinitionR5()); 548 } 549 if (theResourceSupportedSvc.isSupported("Practitioner")) { 550 retVal.add(daoPractitionerR5()); 551 } 552 if (theResourceSupportedSvc.isSupported("PractitionerRole")) { 553 retVal.add(daoPractitionerRoleR5()); 554 } 555 if (theResourceSupportedSvc.isSupported("Procedure")) { 556 retVal.add(daoProcedureR5()); 557 } 558 if (theResourceSupportedSvc.isSupported("Provenance")) { 559 retVal.add(daoProvenanceR5()); 560 } 561 if (theResourceSupportedSvc.isSupported("Questionnaire")) { 562 retVal.add(daoQuestionnaireR5()); 563 } 564 if (theResourceSupportedSvc.isSupported("QuestionnaireResponse")) { 565 retVal.add(daoQuestionnaireResponseR5()); 566 } 567 if (theResourceSupportedSvc.isSupported("RegulatedAuthorization")) { 568 retVal.add(daoRegulatedAuthorizationR5()); 569 } 570 if (theResourceSupportedSvc.isSupported("RelatedPerson")) { 571 retVal.add(daoRelatedPersonR5()); 572 } 573 if (theResourceSupportedSvc.isSupported("RequestOrchestration")) { 574 retVal.add(daoRequestOrchestrationR5()); 575 } 576 if (theResourceSupportedSvc.isSupported("Requirements")) { 577 retVal.add(daoRequirementsR5()); 578 } 579 if (theResourceSupportedSvc.isSupported("ResearchStudy")) { 580 retVal.add(daoResearchStudyR5()); 581 } 582 if (theResourceSupportedSvc.isSupported("ResearchSubject")) { 583 retVal.add(daoResearchSubjectR5()); 584 } 585 if (theResourceSupportedSvc.isSupported("RiskAssessment")) { 586 retVal.add(daoRiskAssessmentR5()); 587 } 588 if (theResourceSupportedSvc.isSupported("Schedule")) { 589 retVal.add(daoScheduleR5()); 590 } 591 if (theResourceSupportedSvc.isSupported("SearchParameter")) { 592 retVal.add(daoSearchParameterR5()); 593 } 594 if (theResourceSupportedSvc.isSupported("ServiceRequest")) { 595 retVal.add(daoServiceRequestR5()); 596 } 597 if (theResourceSupportedSvc.isSupported("Slot")) { 598 retVal.add(daoSlotR5()); 599 } 600 if (theResourceSupportedSvc.isSupported("Specimen")) { 601 retVal.add(daoSpecimenR5()); 602 } 603 if (theResourceSupportedSvc.isSupported("SpecimenDefinition")) { 604 retVal.add(daoSpecimenDefinitionR5()); 605 } 606 if (theResourceSupportedSvc.isSupported("StructureDefinition")) { 607 retVal.add(daoStructureDefinitionR5()); 608 } 609 if (theResourceSupportedSvc.isSupported("StructureMap")) { 610 retVal.add(daoStructureMapR5()); 611 } 612 if (theResourceSupportedSvc.isSupported("Subscription")) { 613 retVal.add(daoSubscriptionR5()); 614 } 615 if (theResourceSupportedSvc.isSupported("SubscriptionStatus")) { 616 retVal.add(daoSubscriptionStatusR5()); 617 } 618 if (theResourceSupportedSvc.isSupported("SubscriptionTopic")) { 619 retVal.add(daoSubscriptionTopicR5()); 620 } 621 if (theResourceSupportedSvc.isSupported("Substance")) { 622 retVal.add(daoSubstanceR5()); 623 } 624 if (theResourceSupportedSvc.isSupported("SubstanceDefinition")) { 625 retVal.add(daoSubstanceDefinitionR5()); 626 } 627 if (theResourceSupportedSvc.isSupported("SubstanceNucleicAcid")) { 628 retVal.add(daoSubstanceNucleicAcidR5()); 629 } 630 if (theResourceSupportedSvc.isSupported("SubstancePolymer")) { 631 retVal.add(daoSubstancePolymerR5()); 632 } 633 if (theResourceSupportedSvc.isSupported("SubstanceProtein")) { 634 retVal.add(daoSubstanceProteinR5()); 635 } 636 if (theResourceSupportedSvc.isSupported("SubstanceReferenceInformation")) { 637 retVal.add(daoSubstanceReferenceInformationR5()); 638 } 639 if (theResourceSupportedSvc.isSupported("SubstanceSourceMaterial")) { 640 retVal.add(daoSubstanceSourceMaterialR5()); 641 } 642 if (theResourceSupportedSvc.isSupported("SupplyDelivery")) { 643 retVal.add(daoSupplyDeliveryR5()); 644 } 645 if (theResourceSupportedSvc.isSupported("SupplyRequest")) { 646 retVal.add(daoSupplyRequestR5()); 647 } 648 if (theResourceSupportedSvc.isSupported("Task")) { 649 retVal.add(daoTaskR5()); 650 } 651 if (theResourceSupportedSvc.isSupported("TerminologyCapabilities")) { 652 retVal.add(daoTerminologyCapabilitiesR5()); 653 } 654 if (theResourceSupportedSvc.isSupported("TestPlan")) { 655 retVal.add(daoTestPlanR5()); 656 } 657 if (theResourceSupportedSvc.isSupported("TestReport")) { 658 retVal.add(daoTestReportR5()); 659 } 660 if (theResourceSupportedSvc.isSupported("TestScript")) { 661 retVal.add(daoTestScriptR5()); 662 } 663 if (theResourceSupportedSvc.isSupported("Transport")) { 664 retVal.add(daoTransportR5()); 665 } 666 if (theResourceSupportedSvc.isSupported("ValueSet")) { 667 retVal.add(daoValueSetR5()); 668 } 669 if (theResourceSupportedSvc.isSupported("VerificationResult")) { 670 retVal.add(daoVerificationResultR5()); 671 } 672 if (theResourceSupportedSvc.isSupported("VisionPrescription")) { 673 retVal.add(daoVisionPrescriptionR5()); 674 } 675 return retVal; 676 } 677 678 @Bean(name="myAccountDaoR5") 679 public 680 IFhirResourceDao<org.hl7.fhir.r5.model.Account> 681 daoAccountR5() { 682 683 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Account> retVal; 684 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Account>(); 685 retVal.setResourceType(org.hl7.fhir.r5.model.Account.class); 686 retVal.setContext(myFhirContext); 687 return retVal; 688 } 689 690 @Bean(name="myAccountRpR5") 691 @Lazy 692 public ca.uhn.fhir.jpa.rp.r5.AccountResourceProvider rpAccountR5() { 693 ca.uhn.fhir.jpa.rp.r5.AccountResourceProvider retVal; 694 retVal = new ca.uhn.fhir.jpa.rp.r5.AccountResourceProvider(); 695 retVal.setContext(myFhirContext); 696 retVal.setDao(daoAccountR5()); 697 return retVal; 698 } 699 700 @Bean(name="myActivityDefinitionDaoR5") 701 public 702 IFhirResourceDao<org.hl7.fhir.r5.model.ActivityDefinition> 703 daoActivityDefinitionR5() { 704 705 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ActivityDefinition> retVal; 706 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ActivityDefinition>(); 707 retVal.setResourceType(org.hl7.fhir.r5.model.ActivityDefinition.class); 708 retVal.setContext(myFhirContext); 709 return retVal; 710 } 711 712 @Bean(name="myActivityDefinitionRpR5") 713 @Lazy 714 public ca.uhn.fhir.jpa.rp.r5.ActivityDefinitionResourceProvider rpActivityDefinitionR5() { 715 ca.uhn.fhir.jpa.rp.r5.ActivityDefinitionResourceProvider retVal; 716 retVal = new ca.uhn.fhir.jpa.rp.r5.ActivityDefinitionResourceProvider(); 717 retVal.setContext(myFhirContext); 718 retVal.setDao(daoActivityDefinitionR5()); 719 return retVal; 720 } 721 722 @Bean(name="myActorDefinitionDaoR5") 723 public 724 IFhirResourceDao<org.hl7.fhir.r5.model.ActorDefinition> 725 daoActorDefinitionR5() { 726 727 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ActorDefinition> retVal; 728 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ActorDefinition>(); 729 retVal.setResourceType(org.hl7.fhir.r5.model.ActorDefinition.class); 730 retVal.setContext(myFhirContext); 731 return retVal; 732 } 733 734 @Bean(name="myActorDefinitionRpR5") 735 @Lazy 736 public ca.uhn.fhir.jpa.rp.r5.ActorDefinitionResourceProvider rpActorDefinitionR5() { 737 ca.uhn.fhir.jpa.rp.r5.ActorDefinitionResourceProvider retVal; 738 retVal = new ca.uhn.fhir.jpa.rp.r5.ActorDefinitionResourceProvider(); 739 retVal.setContext(myFhirContext); 740 retVal.setDao(daoActorDefinitionR5()); 741 return retVal; 742 } 743 744 @Bean(name="myAdministrableProductDefinitionDaoR5") 745 public 746 IFhirResourceDao<org.hl7.fhir.r5.model.AdministrableProductDefinition> 747 daoAdministrableProductDefinitionR5() { 748 749 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.AdministrableProductDefinition> retVal; 750 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.AdministrableProductDefinition>(); 751 retVal.setResourceType(org.hl7.fhir.r5.model.AdministrableProductDefinition.class); 752 retVal.setContext(myFhirContext); 753 return retVal; 754 } 755 756 @Bean(name="myAdministrableProductDefinitionRpR5") 757 @Lazy 758 public ca.uhn.fhir.jpa.rp.r5.AdministrableProductDefinitionResourceProvider rpAdministrableProductDefinitionR5() { 759 ca.uhn.fhir.jpa.rp.r5.AdministrableProductDefinitionResourceProvider retVal; 760 retVal = new ca.uhn.fhir.jpa.rp.r5.AdministrableProductDefinitionResourceProvider(); 761 retVal.setContext(myFhirContext); 762 retVal.setDao(daoAdministrableProductDefinitionR5()); 763 return retVal; 764 } 765 766 @Bean(name="myAdverseEventDaoR5") 767 public 768 IFhirResourceDao<org.hl7.fhir.r5.model.AdverseEvent> 769 daoAdverseEventR5() { 770 771 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.AdverseEvent> retVal; 772 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.AdverseEvent>(); 773 retVal.setResourceType(org.hl7.fhir.r5.model.AdverseEvent.class); 774 retVal.setContext(myFhirContext); 775 return retVal; 776 } 777 778 @Bean(name="myAdverseEventRpR5") 779 @Lazy 780 public ca.uhn.fhir.jpa.rp.r5.AdverseEventResourceProvider rpAdverseEventR5() { 781 ca.uhn.fhir.jpa.rp.r5.AdverseEventResourceProvider retVal; 782 retVal = new ca.uhn.fhir.jpa.rp.r5.AdverseEventResourceProvider(); 783 retVal.setContext(myFhirContext); 784 retVal.setDao(daoAdverseEventR5()); 785 return retVal; 786 } 787 788 @Bean(name="myAllergyIntoleranceDaoR5") 789 public 790 IFhirResourceDao<org.hl7.fhir.r5.model.AllergyIntolerance> 791 daoAllergyIntoleranceR5() { 792 793 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.AllergyIntolerance> retVal; 794 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.AllergyIntolerance>(); 795 retVal.setResourceType(org.hl7.fhir.r5.model.AllergyIntolerance.class); 796 retVal.setContext(myFhirContext); 797 return retVal; 798 } 799 800 @Bean(name="myAllergyIntoleranceRpR5") 801 @Lazy 802 public ca.uhn.fhir.jpa.rp.r5.AllergyIntoleranceResourceProvider rpAllergyIntoleranceR5() { 803 ca.uhn.fhir.jpa.rp.r5.AllergyIntoleranceResourceProvider retVal; 804 retVal = new ca.uhn.fhir.jpa.rp.r5.AllergyIntoleranceResourceProvider(); 805 retVal.setContext(myFhirContext); 806 retVal.setDao(daoAllergyIntoleranceR5()); 807 return retVal; 808 } 809 810 @Bean(name="myAppointmentDaoR5") 811 public 812 IFhirResourceDao<org.hl7.fhir.r5.model.Appointment> 813 daoAppointmentR5() { 814 815 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Appointment> retVal; 816 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Appointment>(); 817 retVal.setResourceType(org.hl7.fhir.r5.model.Appointment.class); 818 retVal.setContext(myFhirContext); 819 return retVal; 820 } 821 822 @Bean(name="myAppointmentRpR5") 823 @Lazy 824 public ca.uhn.fhir.jpa.rp.r5.AppointmentResourceProvider rpAppointmentR5() { 825 ca.uhn.fhir.jpa.rp.r5.AppointmentResourceProvider retVal; 826 retVal = new ca.uhn.fhir.jpa.rp.r5.AppointmentResourceProvider(); 827 retVal.setContext(myFhirContext); 828 retVal.setDao(daoAppointmentR5()); 829 return retVal; 830 } 831 832 @Bean(name="myAppointmentResponseDaoR5") 833 public 834 IFhirResourceDao<org.hl7.fhir.r5.model.AppointmentResponse> 835 daoAppointmentResponseR5() { 836 837 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.AppointmentResponse> retVal; 838 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.AppointmentResponse>(); 839 retVal.setResourceType(org.hl7.fhir.r5.model.AppointmentResponse.class); 840 retVal.setContext(myFhirContext); 841 return retVal; 842 } 843 844 @Bean(name="myAppointmentResponseRpR5") 845 @Lazy 846 public ca.uhn.fhir.jpa.rp.r5.AppointmentResponseResourceProvider rpAppointmentResponseR5() { 847 ca.uhn.fhir.jpa.rp.r5.AppointmentResponseResourceProvider retVal; 848 retVal = new ca.uhn.fhir.jpa.rp.r5.AppointmentResponseResourceProvider(); 849 retVal.setContext(myFhirContext); 850 retVal.setDao(daoAppointmentResponseR5()); 851 return retVal; 852 } 853 854 @Bean(name="myArtifactAssessmentDaoR5") 855 public 856 IFhirResourceDao<org.hl7.fhir.r5.model.ArtifactAssessment> 857 daoArtifactAssessmentR5() { 858 859 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ArtifactAssessment> retVal; 860 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ArtifactAssessment>(); 861 retVal.setResourceType(org.hl7.fhir.r5.model.ArtifactAssessment.class); 862 retVal.setContext(myFhirContext); 863 return retVal; 864 } 865 866 @Bean(name="myArtifactAssessmentRpR5") 867 @Lazy 868 public ca.uhn.fhir.jpa.rp.r5.ArtifactAssessmentResourceProvider rpArtifactAssessmentR5() { 869 ca.uhn.fhir.jpa.rp.r5.ArtifactAssessmentResourceProvider retVal; 870 retVal = new ca.uhn.fhir.jpa.rp.r5.ArtifactAssessmentResourceProvider(); 871 retVal.setContext(myFhirContext); 872 retVal.setDao(daoArtifactAssessmentR5()); 873 return retVal; 874 } 875 876 @Bean(name="myAuditEventDaoR5") 877 public 878 IFhirResourceDao<org.hl7.fhir.r5.model.AuditEvent> 879 daoAuditEventR5() { 880 881 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.AuditEvent> retVal; 882 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.AuditEvent>(); 883 retVal.setResourceType(org.hl7.fhir.r5.model.AuditEvent.class); 884 retVal.setContext(myFhirContext); 885 return retVal; 886 } 887 888 @Bean(name="myAuditEventRpR5") 889 @Lazy 890 public ca.uhn.fhir.jpa.rp.r5.AuditEventResourceProvider rpAuditEventR5() { 891 ca.uhn.fhir.jpa.rp.r5.AuditEventResourceProvider retVal; 892 retVal = new ca.uhn.fhir.jpa.rp.r5.AuditEventResourceProvider(); 893 retVal.setContext(myFhirContext); 894 retVal.setDao(daoAuditEventR5()); 895 return retVal; 896 } 897 898 @Bean(name="myBasicDaoR5") 899 public 900 IFhirResourceDao<org.hl7.fhir.r5.model.Basic> 901 daoBasicR5() { 902 903 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Basic> retVal; 904 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Basic>(); 905 retVal.setResourceType(org.hl7.fhir.r5.model.Basic.class); 906 retVal.setContext(myFhirContext); 907 return retVal; 908 } 909 910 @Bean(name="myBasicRpR5") 911 @Lazy 912 public ca.uhn.fhir.jpa.rp.r5.BasicResourceProvider rpBasicR5() { 913 ca.uhn.fhir.jpa.rp.r5.BasicResourceProvider retVal; 914 retVal = new ca.uhn.fhir.jpa.rp.r5.BasicResourceProvider(); 915 retVal.setContext(myFhirContext); 916 retVal.setDao(daoBasicR5()); 917 return retVal; 918 } 919 920 @Bean(name="myBinaryDaoR5") 921 public 922 IFhirResourceDao<org.hl7.fhir.r5.model.Binary> 923 daoBinaryR5() { 924 925 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Binary> retVal; 926 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Binary>(); 927 retVal.setResourceType(org.hl7.fhir.r5.model.Binary.class); 928 retVal.setContext(myFhirContext); 929 return retVal; 930 } 931 932 @Bean(name="myBinaryRpR5") 933 @Lazy 934 public ca.uhn.fhir.jpa.rp.r5.BinaryResourceProvider rpBinaryR5() { 935 ca.uhn.fhir.jpa.rp.r5.BinaryResourceProvider retVal; 936 retVal = new ca.uhn.fhir.jpa.rp.r5.BinaryResourceProvider(); 937 retVal.setContext(myFhirContext); 938 retVal.setDao(daoBinaryR5()); 939 return retVal; 940 } 941 942 @Bean(name="myBiologicallyDerivedProductDaoR5") 943 public 944 IFhirResourceDao<org.hl7.fhir.r5.model.BiologicallyDerivedProduct> 945 daoBiologicallyDerivedProductR5() { 946 947 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.BiologicallyDerivedProduct> retVal; 948 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.BiologicallyDerivedProduct>(); 949 retVal.setResourceType(org.hl7.fhir.r5.model.BiologicallyDerivedProduct.class); 950 retVal.setContext(myFhirContext); 951 return retVal; 952 } 953 954 @Bean(name="myBiologicallyDerivedProductRpR5") 955 @Lazy 956 public ca.uhn.fhir.jpa.rp.r5.BiologicallyDerivedProductResourceProvider rpBiologicallyDerivedProductR5() { 957 ca.uhn.fhir.jpa.rp.r5.BiologicallyDerivedProductResourceProvider retVal; 958 retVal = new ca.uhn.fhir.jpa.rp.r5.BiologicallyDerivedProductResourceProvider(); 959 retVal.setContext(myFhirContext); 960 retVal.setDao(daoBiologicallyDerivedProductR5()); 961 return retVal; 962 } 963 964 @Bean(name="myBiologicallyDerivedProductDispenseDaoR5") 965 public 966 IFhirResourceDao<org.hl7.fhir.r5.model.BiologicallyDerivedProductDispense> 967 daoBiologicallyDerivedProductDispenseR5() { 968 969 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.BiologicallyDerivedProductDispense> retVal; 970 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.BiologicallyDerivedProductDispense>(); 971 retVal.setResourceType(org.hl7.fhir.r5.model.BiologicallyDerivedProductDispense.class); 972 retVal.setContext(myFhirContext); 973 return retVal; 974 } 975 976 @Bean(name="myBiologicallyDerivedProductDispenseRpR5") 977 @Lazy 978 public ca.uhn.fhir.jpa.rp.r5.BiologicallyDerivedProductDispenseResourceProvider rpBiologicallyDerivedProductDispenseR5() { 979 ca.uhn.fhir.jpa.rp.r5.BiologicallyDerivedProductDispenseResourceProvider retVal; 980 retVal = new ca.uhn.fhir.jpa.rp.r5.BiologicallyDerivedProductDispenseResourceProvider(); 981 retVal.setContext(myFhirContext); 982 retVal.setDao(daoBiologicallyDerivedProductDispenseR5()); 983 return retVal; 984 } 985 986 @Bean(name="myBodyStructureDaoR5") 987 public 988 IFhirResourceDao<org.hl7.fhir.r5.model.BodyStructure> 989 daoBodyStructureR5() { 990 991 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.BodyStructure> retVal; 992 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.BodyStructure>(); 993 retVal.setResourceType(org.hl7.fhir.r5.model.BodyStructure.class); 994 retVal.setContext(myFhirContext); 995 return retVal; 996 } 997 998 @Bean(name="myBodyStructureRpR5") 999 @Lazy 1000 public ca.uhn.fhir.jpa.rp.r5.BodyStructureResourceProvider rpBodyStructureR5() { 1001 ca.uhn.fhir.jpa.rp.r5.BodyStructureResourceProvider retVal; 1002 retVal = new ca.uhn.fhir.jpa.rp.r5.BodyStructureResourceProvider(); 1003 retVal.setContext(myFhirContext); 1004 retVal.setDao(daoBodyStructureR5()); 1005 return retVal; 1006 } 1007 1008 @Bean(name="myBundleDaoR5") 1009 public 1010 IFhirResourceDao<org.hl7.fhir.r5.model.Bundle> 1011 daoBundleR5() { 1012 1013 ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<org.hl7.fhir.r5.model.Bundle> retVal; 1014 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoBundle<>(); 1015 retVal.setResourceType(org.hl7.fhir.r5.model.Bundle.class); 1016 retVal.setContext(myFhirContext); 1017 return retVal; 1018 } 1019 1020 @Bean(name="myBundleRpR5") 1021 @Lazy 1022 public ca.uhn.fhir.jpa.rp.r5.BundleResourceProvider rpBundleR5() { 1023 ca.uhn.fhir.jpa.rp.r5.BundleResourceProvider retVal; 1024 retVal = new ca.uhn.fhir.jpa.rp.r5.BundleResourceProvider(); 1025 retVal.setContext(myFhirContext); 1026 retVal.setDao(daoBundleR5()); 1027 return retVal; 1028 } 1029 1030 @Bean(name="myCapabilityStatementDaoR5") 1031 public 1032 IFhirResourceDao<org.hl7.fhir.r5.model.CapabilityStatement> 1033 daoCapabilityStatementR5() { 1034 1035 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.CapabilityStatement> retVal; 1036 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.CapabilityStatement>(); 1037 retVal.setResourceType(org.hl7.fhir.r5.model.CapabilityStatement.class); 1038 retVal.setContext(myFhirContext); 1039 return retVal; 1040 } 1041 1042 @Bean(name="myCapabilityStatementRpR5") 1043 @Lazy 1044 public ca.uhn.fhir.jpa.rp.r5.CapabilityStatementResourceProvider rpCapabilityStatementR5() { 1045 ca.uhn.fhir.jpa.rp.r5.CapabilityStatementResourceProvider retVal; 1046 retVal = new ca.uhn.fhir.jpa.rp.r5.CapabilityStatementResourceProvider(); 1047 retVal.setContext(myFhirContext); 1048 retVal.setDao(daoCapabilityStatementR5()); 1049 return retVal; 1050 } 1051 1052 @Bean(name="myCarePlanDaoR5") 1053 public 1054 IFhirResourceDao<org.hl7.fhir.r5.model.CarePlan> 1055 daoCarePlanR5() { 1056 1057 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.CarePlan> retVal; 1058 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.CarePlan>(); 1059 retVal.setResourceType(org.hl7.fhir.r5.model.CarePlan.class); 1060 retVal.setContext(myFhirContext); 1061 return retVal; 1062 } 1063 1064 @Bean(name="myCarePlanRpR5") 1065 @Lazy 1066 public ca.uhn.fhir.jpa.rp.r5.CarePlanResourceProvider rpCarePlanR5() { 1067 ca.uhn.fhir.jpa.rp.r5.CarePlanResourceProvider retVal; 1068 retVal = new ca.uhn.fhir.jpa.rp.r5.CarePlanResourceProvider(); 1069 retVal.setContext(myFhirContext); 1070 retVal.setDao(daoCarePlanR5()); 1071 return retVal; 1072 } 1073 1074 @Bean(name="myCareTeamDaoR5") 1075 public 1076 IFhirResourceDao<org.hl7.fhir.r5.model.CareTeam> 1077 daoCareTeamR5() { 1078 1079 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.CareTeam> retVal; 1080 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.CareTeam>(); 1081 retVal.setResourceType(org.hl7.fhir.r5.model.CareTeam.class); 1082 retVal.setContext(myFhirContext); 1083 return retVal; 1084 } 1085 1086 @Bean(name="myCareTeamRpR5") 1087 @Lazy 1088 public ca.uhn.fhir.jpa.rp.r5.CareTeamResourceProvider rpCareTeamR5() { 1089 ca.uhn.fhir.jpa.rp.r5.CareTeamResourceProvider retVal; 1090 retVal = new ca.uhn.fhir.jpa.rp.r5.CareTeamResourceProvider(); 1091 retVal.setContext(myFhirContext); 1092 retVal.setDao(daoCareTeamR5()); 1093 return retVal; 1094 } 1095 1096 @Bean(name="myChargeItemDaoR5") 1097 public 1098 IFhirResourceDao<org.hl7.fhir.r5.model.ChargeItem> 1099 daoChargeItemR5() { 1100 1101 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ChargeItem> retVal; 1102 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ChargeItem>(); 1103 retVal.setResourceType(org.hl7.fhir.r5.model.ChargeItem.class); 1104 retVal.setContext(myFhirContext); 1105 return retVal; 1106 } 1107 1108 @Bean(name="myChargeItemRpR5") 1109 @Lazy 1110 public ca.uhn.fhir.jpa.rp.r5.ChargeItemResourceProvider rpChargeItemR5() { 1111 ca.uhn.fhir.jpa.rp.r5.ChargeItemResourceProvider retVal; 1112 retVal = new ca.uhn.fhir.jpa.rp.r5.ChargeItemResourceProvider(); 1113 retVal.setContext(myFhirContext); 1114 retVal.setDao(daoChargeItemR5()); 1115 return retVal; 1116 } 1117 1118 @Bean(name="myChargeItemDefinitionDaoR5") 1119 public 1120 IFhirResourceDao<org.hl7.fhir.r5.model.ChargeItemDefinition> 1121 daoChargeItemDefinitionR5() { 1122 1123 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ChargeItemDefinition> retVal; 1124 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ChargeItemDefinition>(); 1125 retVal.setResourceType(org.hl7.fhir.r5.model.ChargeItemDefinition.class); 1126 retVal.setContext(myFhirContext); 1127 return retVal; 1128 } 1129 1130 @Bean(name="myChargeItemDefinitionRpR5") 1131 @Lazy 1132 public ca.uhn.fhir.jpa.rp.r5.ChargeItemDefinitionResourceProvider rpChargeItemDefinitionR5() { 1133 ca.uhn.fhir.jpa.rp.r5.ChargeItemDefinitionResourceProvider retVal; 1134 retVal = new ca.uhn.fhir.jpa.rp.r5.ChargeItemDefinitionResourceProvider(); 1135 retVal.setContext(myFhirContext); 1136 retVal.setDao(daoChargeItemDefinitionR5()); 1137 return retVal; 1138 } 1139 1140 @Bean(name="myCitationDaoR5") 1141 public 1142 IFhirResourceDao<org.hl7.fhir.r5.model.Citation> 1143 daoCitationR5() { 1144 1145 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Citation> retVal; 1146 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Citation>(); 1147 retVal.setResourceType(org.hl7.fhir.r5.model.Citation.class); 1148 retVal.setContext(myFhirContext); 1149 return retVal; 1150 } 1151 1152 @Bean(name="myCitationRpR5") 1153 @Lazy 1154 public ca.uhn.fhir.jpa.rp.r5.CitationResourceProvider rpCitationR5() { 1155 ca.uhn.fhir.jpa.rp.r5.CitationResourceProvider retVal; 1156 retVal = new ca.uhn.fhir.jpa.rp.r5.CitationResourceProvider(); 1157 retVal.setContext(myFhirContext); 1158 retVal.setDao(daoCitationR5()); 1159 return retVal; 1160 } 1161 1162 @Bean(name="myClaimDaoR5") 1163 public 1164 IFhirResourceDao<org.hl7.fhir.r5.model.Claim> 1165 daoClaimR5() { 1166 1167 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Claim> retVal; 1168 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Claim>(); 1169 retVal.setResourceType(org.hl7.fhir.r5.model.Claim.class); 1170 retVal.setContext(myFhirContext); 1171 return retVal; 1172 } 1173 1174 @Bean(name="myClaimRpR5") 1175 @Lazy 1176 public ca.uhn.fhir.jpa.rp.r5.ClaimResourceProvider rpClaimR5() { 1177 ca.uhn.fhir.jpa.rp.r5.ClaimResourceProvider retVal; 1178 retVal = new ca.uhn.fhir.jpa.rp.r5.ClaimResourceProvider(); 1179 retVal.setContext(myFhirContext); 1180 retVal.setDao(daoClaimR5()); 1181 return retVal; 1182 } 1183 1184 @Bean(name="myClaimResponseDaoR5") 1185 public 1186 IFhirResourceDao<org.hl7.fhir.r5.model.ClaimResponse> 1187 daoClaimResponseR5() { 1188 1189 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ClaimResponse> retVal; 1190 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ClaimResponse>(); 1191 retVal.setResourceType(org.hl7.fhir.r5.model.ClaimResponse.class); 1192 retVal.setContext(myFhirContext); 1193 return retVal; 1194 } 1195 1196 @Bean(name="myClaimResponseRpR5") 1197 @Lazy 1198 public ca.uhn.fhir.jpa.rp.r5.ClaimResponseResourceProvider rpClaimResponseR5() { 1199 ca.uhn.fhir.jpa.rp.r5.ClaimResponseResourceProvider retVal; 1200 retVal = new ca.uhn.fhir.jpa.rp.r5.ClaimResponseResourceProvider(); 1201 retVal.setContext(myFhirContext); 1202 retVal.setDao(daoClaimResponseR5()); 1203 return retVal; 1204 } 1205 1206 @Bean(name="myClinicalImpressionDaoR5") 1207 public 1208 IFhirResourceDao<org.hl7.fhir.r5.model.ClinicalImpression> 1209 daoClinicalImpressionR5() { 1210 1211 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ClinicalImpression> retVal; 1212 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ClinicalImpression>(); 1213 retVal.setResourceType(org.hl7.fhir.r5.model.ClinicalImpression.class); 1214 retVal.setContext(myFhirContext); 1215 return retVal; 1216 } 1217 1218 @Bean(name="myClinicalImpressionRpR5") 1219 @Lazy 1220 public ca.uhn.fhir.jpa.rp.r5.ClinicalImpressionResourceProvider rpClinicalImpressionR5() { 1221 ca.uhn.fhir.jpa.rp.r5.ClinicalImpressionResourceProvider retVal; 1222 retVal = new ca.uhn.fhir.jpa.rp.r5.ClinicalImpressionResourceProvider(); 1223 retVal.setContext(myFhirContext); 1224 retVal.setDao(daoClinicalImpressionR5()); 1225 return retVal; 1226 } 1227 1228 @Bean(name="myClinicalUseDefinitionDaoR5") 1229 public 1230 IFhirResourceDao<org.hl7.fhir.r5.model.ClinicalUseDefinition> 1231 daoClinicalUseDefinitionR5() { 1232 1233 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ClinicalUseDefinition> retVal; 1234 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ClinicalUseDefinition>(); 1235 retVal.setResourceType(org.hl7.fhir.r5.model.ClinicalUseDefinition.class); 1236 retVal.setContext(myFhirContext); 1237 return retVal; 1238 } 1239 1240 @Bean(name="myClinicalUseDefinitionRpR5") 1241 @Lazy 1242 public ca.uhn.fhir.jpa.rp.r5.ClinicalUseDefinitionResourceProvider rpClinicalUseDefinitionR5() { 1243 ca.uhn.fhir.jpa.rp.r5.ClinicalUseDefinitionResourceProvider retVal; 1244 retVal = new ca.uhn.fhir.jpa.rp.r5.ClinicalUseDefinitionResourceProvider(); 1245 retVal.setContext(myFhirContext); 1246 retVal.setDao(daoClinicalUseDefinitionR5()); 1247 return retVal; 1248 } 1249 1250 @Bean(name="myCodeSystemDaoR5") 1251 public 1252 IFhirResourceDaoCodeSystem<org.hl7.fhir.r5.model.CodeSystem> 1253 daoCodeSystemR5() { 1254 1255 ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem<org.hl7.fhir.r5.model.CodeSystem> retVal; 1256 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoCodeSystem<>(); 1257 retVal.setResourceType(org.hl7.fhir.r5.model.CodeSystem.class); 1258 retVal.setContext(myFhirContext); 1259 return retVal; 1260 } 1261 1262 @Bean(name="myCodeSystemRpR5") 1263 @Lazy 1264 public ca.uhn.fhir.jpa.rp.r5.CodeSystemResourceProvider rpCodeSystemR5() { 1265 ca.uhn.fhir.jpa.rp.r5.CodeSystemResourceProvider retVal; 1266 retVal = new ca.uhn.fhir.jpa.rp.r5.CodeSystemResourceProvider(); 1267 retVal.setContext(myFhirContext); 1268 retVal.setDao(daoCodeSystemR5()); 1269 return retVal; 1270 } 1271 1272 @Bean(name="myCommunicationDaoR5") 1273 public 1274 IFhirResourceDao<org.hl7.fhir.r5.model.Communication> 1275 daoCommunicationR5() { 1276 1277 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Communication> retVal; 1278 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Communication>(); 1279 retVal.setResourceType(org.hl7.fhir.r5.model.Communication.class); 1280 retVal.setContext(myFhirContext); 1281 return retVal; 1282 } 1283 1284 @Bean(name="myCommunicationRpR5") 1285 @Lazy 1286 public ca.uhn.fhir.jpa.rp.r5.CommunicationResourceProvider rpCommunicationR5() { 1287 ca.uhn.fhir.jpa.rp.r5.CommunicationResourceProvider retVal; 1288 retVal = new ca.uhn.fhir.jpa.rp.r5.CommunicationResourceProvider(); 1289 retVal.setContext(myFhirContext); 1290 retVal.setDao(daoCommunicationR5()); 1291 return retVal; 1292 } 1293 1294 @Bean(name="myCommunicationRequestDaoR5") 1295 public 1296 IFhirResourceDao<org.hl7.fhir.r5.model.CommunicationRequest> 1297 daoCommunicationRequestR5() { 1298 1299 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.CommunicationRequest> retVal; 1300 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.CommunicationRequest>(); 1301 retVal.setResourceType(org.hl7.fhir.r5.model.CommunicationRequest.class); 1302 retVal.setContext(myFhirContext); 1303 return retVal; 1304 } 1305 1306 @Bean(name="myCommunicationRequestRpR5") 1307 @Lazy 1308 public ca.uhn.fhir.jpa.rp.r5.CommunicationRequestResourceProvider rpCommunicationRequestR5() { 1309 ca.uhn.fhir.jpa.rp.r5.CommunicationRequestResourceProvider retVal; 1310 retVal = new ca.uhn.fhir.jpa.rp.r5.CommunicationRequestResourceProvider(); 1311 retVal.setContext(myFhirContext); 1312 retVal.setDao(daoCommunicationRequestR5()); 1313 return retVal; 1314 } 1315 1316 @Bean(name="myCompartmentDefinitionDaoR5") 1317 public 1318 IFhirResourceDao<org.hl7.fhir.r5.model.CompartmentDefinition> 1319 daoCompartmentDefinitionR5() { 1320 1321 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.CompartmentDefinition> retVal; 1322 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.CompartmentDefinition>(); 1323 retVal.setResourceType(org.hl7.fhir.r5.model.CompartmentDefinition.class); 1324 retVal.setContext(myFhirContext); 1325 return retVal; 1326 } 1327 1328 @Bean(name="myCompartmentDefinitionRpR5") 1329 @Lazy 1330 public ca.uhn.fhir.jpa.rp.r5.CompartmentDefinitionResourceProvider rpCompartmentDefinitionR5() { 1331 ca.uhn.fhir.jpa.rp.r5.CompartmentDefinitionResourceProvider retVal; 1332 retVal = new ca.uhn.fhir.jpa.rp.r5.CompartmentDefinitionResourceProvider(); 1333 retVal.setContext(myFhirContext); 1334 retVal.setDao(daoCompartmentDefinitionR5()); 1335 return retVal; 1336 } 1337 1338 @Bean(name="myCompositionDaoR5") 1339 public 1340 IFhirResourceDaoComposition<org.hl7.fhir.r5.model.Composition> 1341 daoCompositionR5() { 1342 1343 ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<org.hl7.fhir.r5.model.Composition> retVal; 1344 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoComposition<>(); 1345 retVal.setResourceType(org.hl7.fhir.r5.model.Composition.class); 1346 retVal.setContext(myFhirContext); 1347 return retVal; 1348 } 1349 1350 @Bean(name="myCompositionRpR5") 1351 @Lazy 1352 public ca.uhn.fhir.jpa.rp.r5.CompositionResourceProvider rpCompositionR5() { 1353 ca.uhn.fhir.jpa.rp.r5.CompositionResourceProvider retVal; 1354 retVal = new ca.uhn.fhir.jpa.rp.r5.CompositionResourceProvider(); 1355 retVal.setContext(myFhirContext); 1356 retVal.setDao(daoCompositionR5()); 1357 return retVal; 1358 } 1359 1360 @Bean(name="myConceptMapDaoR5") 1361 public 1362 IFhirResourceDaoConceptMap<org.hl7.fhir.r5.model.ConceptMap> 1363 daoConceptMapR5() { 1364 1365 ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<org.hl7.fhir.r5.model.ConceptMap> retVal; 1366 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoConceptMap<>(); 1367 retVal.setResourceType(org.hl7.fhir.r5.model.ConceptMap.class); 1368 retVal.setContext(myFhirContext); 1369 return retVal; 1370 } 1371 1372 @Bean(name="myConceptMapRpR5") 1373 @Lazy 1374 public ca.uhn.fhir.jpa.rp.r5.ConceptMapResourceProvider rpConceptMapR5() { 1375 ca.uhn.fhir.jpa.rp.r5.ConceptMapResourceProvider retVal; 1376 retVal = new ca.uhn.fhir.jpa.rp.r5.ConceptMapResourceProvider(); 1377 retVal.setContext(myFhirContext); 1378 retVal.setDao(daoConceptMapR5()); 1379 return retVal; 1380 } 1381 1382 @Bean(name="myConditionDaoR5") 1383 public 1384 IFhirResourceDao<org.hl7.fhir.r5.model.Condition> 1385 daoConditionR5() { 1386 1387 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Condition> retVal; 1388 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Condition>(); 1389 retVal.setResourceType(org.hl7.fhir.r5.model.Condition.class); 1390 retVal.setContext(myFhirContext); 1391 return retVal; 1392 } 1393 1394 @Bean(name="myConditionRpR5") 1395 @Lazy 1396 public ca.uhn.fhir.jpa.rp.r5.ConditionResourceProvider rpConditionR5() { 1397 ca.uhn.fhir.jpa.rp.r5.ConditionResourceProvider retVal; 1398 retVal = new ca.uhn.fhir.jpa.rp.r5.ConditionResourceProvider(); 1399 retVal.setContext(myFhirContext); 1400 retVal.setDao(daoConditionR5()); 1401 return retVal; 1402 } 1403 1404 @Bean(name="myConditionDefinitionDaoR5") 1405 public 1406 IFhirResourceDao<org.hl7.fhir.r5.model.ConditionDefinition> 1407 daoConditionDefinitionR5() { 1408 1409 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ConditionDefinition> retVal; 1410 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ConditionDefinition>(); 1411 retVal.setResourceType(org.hl7.fhir.r5.model.ConditionDefinition.class); 1412 retVal.setContext(myFhirContext); 1413 return retVal; 1414 } 1415 1416 @Bean(name="myConditionDefinitionRpR5") 1417 @Lazy 1418 public ca.uhn.fhir.jpa.rp.r5.ConditionDefinitionResourceProvider rpConditionDefinitionR5() { 1419 ca.uhn.fhir.jpa.rp.r5.ConditionDefinitionResourceProvider retVal; 1420 retVal = new ca.uhn.fhir.jpa.rp.r5.ConditionDefinitionResourceProvider(); 1421 retVal.setContext(myFhirContext); 1422 retVal.setDao(daoConditionDefinitionR5()); 1423 return retVal; 1424 } 1425 1426 @Bean(name="myConsentDaoR5") 1427 public 1428 IFhirResourceDao<org.hl7.fhir.r5.model.Consent> 1429 daoConsentR5() { 1430 1431 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Consent> retVal; 1432 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Consent>(); 1433 retVal.setResourceType(org.hl7.fhir.r5.model.Consent.class); 1434 retVal.setContext(myFhirContext); 1435 return retVal; 1436 } 1437 1438 @Bean(name="myConsentRpR5") 1439 @Lazy 1440 public ca.uhn.fhir.jpa.rp.r5.ConsentResourceProvider rpConsentR5() { 1441 ca.uhn.fhir.jpa.rp.r5.ConsentResourceProvider retVal; 1442 retVal = new ca.uhn.fhir.jpa.rp.r5.ConsentResourceProvider(); 1443 retVal.setContext(myFhirContext); 1444 retVal.setDao(daoConsentR5()); 1445 return retVal; 1446 } 1447 1448 @Bean(name="myContractDaoR5") 1449 public 1450 IFhirResourceDao<org.hl7.fhir.r5.model.Contract> 1451 daoContractR5() { 1452 1453 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Contract> retVal; 1454 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Contract>(); 1455 retVal.setResourceType(org.hl7.fhir.r5.model.Contract.class); 1456 retVal.setContext(myFhirContext); 1457 return retVal; 1458 } 1459 1460 @Bean(name="myContractRpR5") 1461 @Lazy 1462 public ca.uhn.fhir.jpa.rp.r5.ContractResourceProvider rpContractR5() { 1463 ca.uhn.fhir.jpa.rp.r5.ContractResourceProvider retVal; 1464 retVal = new ca.uhn.fhir.jpa.rp.r5.ContractResourceProvider(); 1465 retVal.setContext(myFhirContext); 1466 retVal.setDao(daoContractR5()); 1467 return retVal; 1468 } 1469 1470 @Bean(name="myCoverageDaoR5") 1471 public 1472 IFhirResourceDao<org.hl7.fhir.r5.model.Coverage> 1473 daoCoverageR5() { 1474 1475 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Coverage> retVal; 1476 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Coverage>(); 1477 retVal.setResourceType(org.hl7.fhir.r5.model.Coverage.class); 1478 retVal.setContext(myFhirContext); 1479 return retVal; 1480 } 1481 1482 @Bean(name="myCoverageRpR5") 1483 @Lazy 1484 public ca.uhn.fhir.jpa.rp.r5.CoverageResourceProvider rpCoverageR5() { 1485 ca.uhn.fhir.jpa.rp.r5.CoverageResourceProvider retVal; 1486 retVal = new ca.uhn.fhir.jpa.rp.r5.CoverageResourceProvider(); 1487 retVal.setContext(myFhirContext); 1488 retVal.setDao(daoCoverageR5()); 1489 return retVal; 1490 } 1491 1492 @Bean(name="myCoverageEligibilityRequestDaoR5") 1493 public 1494 IFhirResourceDao<org.hl7.fhir.r5.model.CoverageEligibilityRequest> 1495 daoCoverageEligibilityRequestR5() { 1496 1497 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.CoverageEligibilityRequest> retVal; 1498 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.CoverageEligibilityRequest>(); 1499 retVal.setResourceType(org.hl7.fhir.r5.model.CoverageEligibilityRequest.class); 1500 retVal.setContext(myFhirContext); 1501 return retVal; 1502 } 1503 1504 @Bean(name="myCoverageEligibilityRequestRpR5") 1505 @Lazy 1506 public ca.uhn.fhir.jpa.rp.r5.CoverageEligibilityRequestResourceProvider rpCoverageEligibilityRequestR5() { 1507 ca.uhn.fhir.jpa.rp.r5.CoverageEligibilityRequestResourceProvider retVal; 1508 retVal = new ca.uhn.fhir.jpa.rp.r5.CoverageEligibilityRequestResourceProvider(); 1509 retVal.setContext(myFhirContext); 1510 retVal.setDao(daoCoverageEligibilityRequestR5()); 1511 return retVal; 1512 } 1513 1514 @Bean(name="myCoverageEligibilityResponseDaoR5") 1515 public 1516 IFhirResourceDao<org.hl7.fhir.r5.model.CoverageEligibilityResponse> 1517 daoCoverageEligibilityResponseR5() { 1518 1519 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.CoverageEligibilityResponse> retVal; 1520 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.CoverageEligibilityResponse>(); 1521 retVal.setResourceType(org.hl7.fhir.r5.model.CoverageEligibilityResponse.class); 1522 retVal.setContext(myFhirContext); 1523 return retVal; 1524 } 1525 1526 @Bean(name="myCoverageEligibilityResponseRpR5") 1527 @Lazy 1528 public ca.uhn.fhir.jpa.rp.r5.CoverageEligibilityResponseResourceProvider rpCoverageEligibilityResponseR5() { 1529 ca.uhn.fhir.jpa.rp.r5.CoverageEligibilityResponseResourceProvider retVal; 1530 retVal = new ca.uhn.fhir.jpa.rp.r5.CoverageEligibilityResponseResourceProvider(); 1531 retVal.setContext(myFhirContext); 1532 retVal.setDao(daoCoverageEligibilityResponseR5()); 1533 return retVal; 1534 } 1535 1536 @Bean(name="myDetectedIssueDaoR5") 1537 public 1538 IFhirResourceDao<org.hl7.fhir.r5.model.DetectedIssue> 1539 daoDetectedIssueR5() { 1540 1541 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DetectedIssue> retVal; 1542 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DetectedIssue>(); 1543 retVal.setResourceType(org.hl7.fhir.r5.model.DetectedIssue.class); 1544 retVal.setContext(myFhirContext); 1545 return retVal; 1546 } 1547 1548 @Bean(name="myDetectedIssueRpR5") 1549 @Lazy 1550 public ca.uhn.fhir.jpa.rp.r5.DetectedIssueResourceProvider rpDetectedIssueR5() { 1551 ca.uhn.fhir.jpa.rp.r5.DetectedIssueResourceProvider retVal; 1552 retVal = new ca.uhn.fhir.jpa.rp.r5.DetectedIssueResourceProvider(); 1553 retVal.setContext(myFhirContext); 1554 retVal.setDao(daoDetectedIssueR5()); 1555 return retVal; 1556 } 1557 1558 @Bean(name="myDeviceDaoR5") 1559 public 1560 IFhirResourceDao<org.hl7.fhir.r5.model.Device> 1561 daoDeviceR5() { 1562 1563 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Device> retVal; 1564 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Device>(); 1565 retVal.setResourceType(org.hl7.fhir.r5.model.Device.class); 1566 retVal.setContext(myFhirContext); 1567 return retVal; 1568 } 1569 1570 @Bean(name="myDeviceRpR5") 1571 @Lazy 1572 public ca.uhn.fhir.jpa.rp.r5.DeviceResourceProvider rpDeviceR5() { 1573 ca.uhn.fhir.jpa.rp.r5.DeviceResourceProvider retVal; 1574 retVal = new ca.uhn.fhir.jpa.rp.r5.DeviceResourceProvider(); 1575 retVal.setContext(myFhirContext); 1576 retVal.setDao(daoDeviceR5()); 1577 return retVal; 1578 } 1579 1580 @Bean(name="myDeviceAssociationDaoR5") 1581 public 1582 IFhirResourceDao<org.hl7.fhir.r5.model.DeviceAssociation> 1583 daoDeviceAssociationR5() { 1584 1585 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DeviceAssociation> retVal; 1586 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DeviceAssociation>(); 1587 retVal.setResourceType(org.hl7.fhir.r5.model.DeviceAssociation.class); 1588 retVal.setContext(myFhirContext); 1589 return retVal; 1590 } 1591 1592 @Bean(name="myDeviceAssociationRpR5") 1593 @Lazy 1594 public ca.uhn.fhir.jpa.rp.r5.DeviceAssociationResourceProvider rpDeviceAssociationR5() { 1595 ca.uhn.fhir.jpa.rp.r5.DeviceAssociationResourceProvider retVal; 1596 retVal = new ca.uhn.fhir.jpa.rp.r5.DeviceAssociationResourceProvider(); 1597 retVal.setContext(myFhirContext); 1598 retVal.setDao(daoDeviceAssociationR5()); 1599 return retVal; 1600 } 1601 1602 @Bean(name="myDeviceDefinitionDaoR5") 1603 public 1604 IFhirResourceDao<org.hl7.fhir.r5.model.DeviceDefinition> 1605 daoDeviceDefinitionR5() { 1606 1607 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DeviceDefinition> retVal; 1608 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DeviceDefinition>(); 1609 retVal.setResourceType(org.hl7.fhir.r5.model.DeviceDefinition.class); 1610 retVal.setContext(myFhirContext); 1611 return retVal; 1612 } 1613 1614 @Bean(name="myDeviceDefinitionRpR5") 1615 @Lazy 1616 public ca.uhn.fhir.jpa.rp.r5.DeviceDefinitionResourceProvider rpDeviceDefinitionR5() { 1617 ca.uhn.fhir.jpa.rp.r5.DeviceDefinitionResourceProvider retVal; 1618 retVal = new ca.uhn.fhir.jpa.rp.r5.DeviceDefinitionResourceProvider(); 1619 retVal.setContext(myFhirContext); 1620 retVal.setDao(daoDeviceDefinitionR5()); 1621 return retVal; 1622 } 1623 1624 @Bean(name="myDeviceDispenseDaoR5") 1625 public 1626 IFhirResourceDao<org.hl7.fhir.r5.model.DeviceDispense> 1627 daoDeviceDispenseR5() { 1628 1629 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DeviceDispense> retVal; 1630 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DeviceDispense>(); 1631 retVal.setResourceType(org.hl7.fhir.r5.model.DeviceDispense.class); 1632 retVal.setContext(myFhirContext); 1633 return retVal; 1634 } 1635 1636 @Bean(name="myDeviceDispenseRpR5") 1637 @Lazy 1638 public ca.uhn.fhir.jpa.rp.r5.DeviceDispenseResourceProvider rpDeviceDispenseR5() { 1639 ca.uhn.fhir.jpa.rp.r5.DeviceDispenseResourceProvider retVal; 1640 retVal = new ca.uhn.fhir.jpa.rp.r5.DeviceDispenseResourceProvider(); 1641 retVal.setContext(myFhirContext); 1642 retVal.setDao(daoDeviceDispenseR5()); 1643 return retVal; 1644 } 1645 1646 @Bean(name="myDeviceMetricDaoR5") 1647 public 1648 IFhirResourceDao<org.hl7.fhir.r5.model.DeviceMetric> 1649 daoDeviceMetricR5() { 1650 1651 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DeviceMetric> retVal; 1652 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DeviceMetric>(); 1653 retVal.setResourceType(org.hl7.fhir.r5.model.DeviceMetric.class); 1654 retVal.setContext(myFhirContext); 1655 return retVal; 1656 } 1657 1658 @Bean(name="myDeviceMetricRpR5") 1659 @Lazy 1660 public ca.uhn.fhir.jpa.rp.r5.DeviceMetricResourceProvider rpDeviceMetricR5() { 1661 ca.uhn.fhir.jpa.rp.r5.DeviceMetricResourceProvider retVal; 1662 retVal = new ca.uhn.fhir.jpa.rp.r5.DeviceMetricResourceProvider(); 1663 retVal.setContext(myFhirContext); 1664 retVal.setDao(daoDeviceMetricR5()); 1665 return retVal; 1666 } 1667 1668 @Bean(name="myDeviceRequestDaoR5") 1669 public 1670 IFhirResourceDao<org.hl7.fhir.r5.model.DeviceRequest> 1671 daoDeviceRequestR5() { 1672 1673 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DeviceRequest> retVal; 1674 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DeviceRequest>(); 1675 retVal.setResourceType(org.hl7.fhir.r5.model.DeviceRequest.class); 1676 retVal.setContext(myFhirContext); 1677 return retVal; 1678 } 1679 1680 @Bean(name="myDeviceRequestRpR5") 1681 @Lazy 1682 public ca.uhn.fhir.jpa.rp.r5.DeviceRequestResourceProvider rpDeviceRequestR5() { 1683 ca.uhn.fhir.jpa.rp.r5.DeviceRequestResourceProvider retVal; 1684 retVal = new ca.uhn.fhir.jpa.rp.r5.DeviceRequestResourceProvider(); 1685 retVal.setContext(myFhirContext); 1686 retVal.setDao(daoDeviceRequestR5()); 1687 return retVal; 1688 } 1689 1690 @Bean(name="myDeviceUsageDaoR5") 1691 public 1692 IFhirResourceDao<org.hl7.fhir.r5.model.DeviceUsage> 1693 daoDeviceUsageR5() { 1694 1695 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DeviceUsage> retVal; 1696 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DeviceUsage>(); 1697 retVal.setResourceType(org.hl7.fhir.r5.model.DeviceUsage.class); 1698 retVal.setContext(myFhirContext); 1699 return retVal; 1700 } 1701 1702 @Bean(name="myDeviceUsageRpR5") 1703 @Lazy 1704 public ca.uhn.fhir.jpa.rp.r5.DeviceUsageResourceProvider rpDeviceUsageR5() { 1705 ca.uhn.fhir.jpa.rp.r5.DeviceUsageResourceProvider retVal; 1706 retVal = new ca.uhn.fhir.jpa.rp.r5.DeviceUsageResourceProvider(); 1707 retVal.setContext(myFhirContext); 1708 retVal.setDao(daoDeviceUsageR5()); 1709 return retVal; 1710 } 1711 1712 @Bean(name="myDiagnosticReportDaoR5") 1713 public 1714 IFhirResourceDao<org.hl7.fhir.r5.model.DiagnosticReport> 1715 daoDiagnosticReportR5() { 1716 1717 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DiagnosticReport> retVal; 1718 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DiagnosticReport>(); 1719 retVal.setResourceType(org.hl7.fhir.r5.model.DiagnosticReport.class); 1720 retVal.setContext(myFhirContext); 1721 return retVal; 1722 } 1723 1724 @Bean(name="myDiagnosticReportRpR5") 1725 @Lazy 1726 public ca.uhn.fhir.jpa.rp.r5.DiagnosticReportResourceProvider rpDiagnosticReportR5() { 1727 ca.uhn.fhir.jpa.rp.r5.DiagnosticReportResourceProvider retVal; 1728 retVal = new ca.uhn.fhir.jpa.rp.r5.DiagnosticReportResourceProvider(); 1729 retVal.setContext(myFhirContext); 1730 retVal.setDao(daoDiagnosticReportR5()); 1731 return retVal; 1732 } 1733 1734 @Bean(name="myDocumentReferenceDaoR5") 1735 public 1736 IFhirResourceDao<org.hl7.fhir.r5.model.DocumentReference> 1737 daoDocumentReferenceR5() { 1738 1739 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.DocumentReference> retVal; 1740 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.DocumentReference>(); 1741 retVal.setResourceType(org.hl7.fhir.r5.model.DocumentReference.class); 1742 retVal.setContext(myFhirContext); 1743 return retVal; 1744 } 1745 1746 @Bean(name="myDocumentReferenceRpR5") 1747 @Lazy 1748 public ca.uhn.fhir.jpa.rp.r5.DocumentReferenceResourceProvider rpDocumentReferenceR5() { 1749 ca.uhn.fhir.jpa.rp.r5.DocumentReferenceResourceProvider retVal; 1750 retVal = new ca.uhn.fhir.jpa.rp.r5.DocumentReferenceResourceProvider(); 1751 retVal.setContext(myFhirContext); 1752 retVal.setDao(daoDocumentReferenceR5()); 1753 return retVal; 1754 } 1755 1756 @Bean(name="myEncounterDaoR5") 1757 public 1758 IFhirResourceDaoEncounter<org.hl7.fhir.r5.model.Encounter> 1759 daoEncounterR5() { 1760 1761 ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<org.hl7.fhir.r5.model.Encounter> retVal; 1762 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoEncounter<>(); 1763 retVal.setResourceType(org.hl7.fhir.r5.model.Encounter.class); 1764 retVal.setContext(myFhirContext); 1765 return retVal; 1766 } 1767 1768 @Bean(name="myEncounterRpR5") 1769 @Lazy 1770 public ca.uhn.fhir.jpa.rp.r5.EncounterResourceProvider rpEncounterR5() { 1771 ca.uhn.fhir.jpa.rp.r5.EncounterResourceProvider retVal; 1772 retVal = new ca.uhn.fhir.jpa.rp.r5.EncounterResourceProvider(); 1773 retVal.setContext(myFhirContext); 1774 retVal.setDao(daoEncounterR5()); 1775 return retVal; 1776 } 1777 1778 @Bean(name="myEncounterHistoryDaoR5") 1779 public 1780 IFhirResourceDao<org.hl7.fhir.r5.model.EncounterHistory> 1781 daoEncounterHistoryR5() { 1782 1783 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.EncounterHistory> retVal; 1784 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.EncounterHistory>(); 1785 retVal.setResourceType(org.hl7.fhir.r5.model.EncounterHistory.class); 1786 retVal.setContext(myFhirContext); 1787 return retVal; 1788 } 1789 1790 @Bean(name="myEncounterHistoryRpR5") 1791 @Lazy 1792 public ca.uhn.fhir.jpa.rp.r5.EncounterHistoryResourceProvider rpEncounterHistoryR5() { 1793 ca.uhn.fhir.jpa.rp.r5.EncounterHistoryResourceProvider retVal; 1794 retVal = new ca.uhn.fhir.jpa.rp.r5.EncounterHistoryResourceProvider(); 1795 retVal.setContext(myFhirContext); 1796 retVal.setDao(daoEncounterHistoryR5()); 1797 return retVal; 1798 } 1799 1800 @Bean(name="myEndpointDaoR5") 1801 public 1802 IFhirResourceDao<org.hl7.fhir.r5.model.Endpoint> 1803 daoEndpointR5() { 1804 1805 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Endpoint> retVal; 1806 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Endpoint>(); 1807 retVal.setResourceType(org.hl7.fhir.r5.model.Endpoint.class); 1808 retVal.setContext(myFhirContext); 1809 return retVal; 1810 } 1811 1812 @Bean(name="myEndpointRpR5") 1813 @Lazy 1814 public ca.uhn.fhir.jpa.rp.r5.EndpointResourceProvider rpEndpointR5() { 1815 ca.uhn.fhir.jpa.rp.r5.EndpointResourceProvider retVal; 1816 retVal = new ca.uhn.fhir.jpa.rp.r5.EndpointResourceProvider(); 1817 retVal.setContext(myFhirContext); 1818 retVal.setDao(daoEndpointR5()); 1819 return retVal; 1820 } 1821 1822 @Bean(name="myEnrollmentRequestDaoR5") 1823 public 1824 IFhirResourceDao<org.hl7.fhir.r5.model.EnrollmentRequest> 1825 daoEnrollmentRequestR5() { 1826 1827 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.EnrollmentRequest> retVal; 1828 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.EnrollmentRequest>(); 1829 retVal.setResourceType(org.hl7.fhir.r5.model.EnrollmentRequest.class); 1830 retVal.setContext(myFhirContext); 1831 return retVal; 1832 } 1833 1834 @Bean(name="myEnrollmentRequestRpR5") 1835 @Lazy 1836 public ca.uhn.fhir.jpa.rp.r5.EnrollmentRequestResourceProvider rpEnrollmentRequestR5() { 1837 ca.uhn.fhir.jpa.rp.r5.EnrollmentRequestResourceProvider retVal; 1838 retVal = new ca.uhn.fhir.jpa.rp.r5.EnrollmentRequestResourceProvider(); 1839 retVal.setContext(myFhirContext); 1840 retVal.setDao(daoEnrollmentRequestR5()); 1841 return retVal; 1842 } 1843 1844 @Bean(name="myEnrollmentResponseDaoR5") 1845 public 1846 IFhirResourceDao<org.hl7.fhir.r5.model.EnrollmentResponse> 1847 daoEnrollmentResponseR5() { 1848 1849 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.EnrollmentResponse> retVal; 1850 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.EnrollmentResponse>(); 1851 retVal.setResourceType(org.hl7.fhir.r5.model.EnrollmentResponse.class); 1852 retVal.setContext(myFhirContext); 1853 return retVal; 1854 } 1855 1856 @Bean(name="myEnrollmentResponseRpR5") 1857 @Lazy 1858 public ca.uhn.fhir.jpa.rp.r5.EnrollmentResponseResourceProvider rpEnrollmentResponseR5() { 1859 ca.uhn.fhir.jpa.rp.r5.EnrollmentResponseResourceProvider retVal; 1860 retVal = new ca.uhn.fhir.jpa.rp.r5.EnrollmentResponseResourceProvider(); 1861 retVal.setContext(myFhirContext); 1862 retVal.setDao(daoEnrollmentResponseR5()); 1863 return retVal; 1864 } 1865 1866 @Bean(name="myEpisodeOfCareDaoR5") 1867 public 1868 IFhirResourceDao<org.hl7.fhir.r5.model.EpisodeOfCare> 1869 daoEpisodeOfCareR5() { 1870 1871 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.EpisodeOfCare> retVal; 1872 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.EpisodeOfCare>(); 1873 retVal.setResourceType(org.hl7.fhir.r5.model.EpisodeOfCare.class); 1874 retVal.setContext(myFhirContext); 1875 return retVal; 1876 } 1877 1878 @Bean(name="myEpisodeOfCareRpR5") 1879 @Lazy 1880 public ca.uhn.fhir.jpa.rp.r5.EpisodeOfCareResourceProvider rpEpisodeOfCareR5() { 1881 ca.uhn.fhir.jpa.rp.r5.EpisodeOfCareResourceProvider retVal; 1882 retVal = new ca.uhn.fhir.jpa.rp.r5.EpisodeOfCareResourceProvider(); 1883 retVal.setContext(myFhirContext); 1884 retVal.setDao(daoEpisodeOfCareR5()); 1885 return retVal; 1886 } 1887 1888 @Bean(name="myEventDefinitionDaoR5") 1889 public 1890 IFhirResourceDao<org.hl7.fhir.r5.model.EventDefinition> 1891 daoEventDefinitionR5() { 1892 1893 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.EventDefinition> retVal; 1894 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.EventDefinition>(); 1895 retVal.setResourceType(org.hl7.fhir.r5.model.EventDefinition.class); 1896 retVal.setContext(myFhirContext); 1897 return retVal; 1898 } 1899 1900 @Bean(name="myEventDefinitionRpR5") 1901 @Lazy 1902 public ca.uhn.fhir.jpa.rp.r5.EventDefinitionResourceProvider rpEventDefinitionR5() { 1903 ca.uhn.fhir.jpa.rp.r5.EventDefinitionResourceProvider retVal; 1904 retVal = new ca.uhn.fhir.jpa.rp.r5.EventDefinitionResourceProvider(); 1905 retVal.setContext(myFhirContext); 1906 retVal.setDao(daoEventDefinitionR5()); 1907 return retVal; 1908 } 1909 1910 @Bean(name="myEvidenceDaoR5") 1911 public 1912 IFhirResourceDao<org.hl7.fhir.r5.model.Evidence> 1913 daoEvidenceR5() { 1914 1915 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Evidence> retVal; 1916 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Evidence>(); 1917 retVal.setResourceType(org.hl7.fhir.r5.model.Evidence.class); 1918 retVal.setContext(myFhirContext); 1919 return retVal; 1920 } 1921 1922 @Bean(name="myEvidenceRpR5") 1923 @Lazy 1924 public ca.uhn.fhir.jpa.rp.r5.EvidenceResourceProvider rpEvidenceR5() { 1925 ca.uhn.fhir.jpa.rp.r5.EvidenceResourceProvider retVal; 1926 retVal = new ca.uhn.fhir.jpa.rp.r5.EvidenceResourceProvider(); 1927 retVal.setContext(myFhirContext); 1928 retVal.setDao(daoEvidenceR5()); 1929 return retVal; 1930 } 1931 1932 @Bean(name="myEvidenceReportDaoR5") 1933 public 1934 IFhirResourceDao<org.hl7.fhir.r5.model.EvidenceReport> 1935 daoEvidenceReportR5() { 1936 1937 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.EvidenceReport> retVal; 1938 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.EvidenceReport>(); 1939 retVal.setResourceType(org.hl7.fhir.r5.model.EvidenceReport.class); 1940 retVal.setContext(myFhirContext); 1941 return retVal; 1942 } 1943 1944 @Bean(name="myEvidenceReportRpR5") 1945 @Lazy 1946 public ca.uhn.fhir.jpa.rp.r5.EvidenceReportResourceProvider rpEvidenceReportR5() { 1947 ca.uhn.fhir.jpa.rp.r5.EvidenceReportResourceProvider retVal; 1948 retVal = new ca.uhn.fhir.jpa.rp.r5.EvidenceReportResourceProvider(); 1949 retVal.setContext(myFhirContext); 1950 retVal.setDao(daoEvidenceReportR5()); 1951 return retVal; 1952 } 1953 1954 @Bean(name="myEvidenceVariableDaoR5") 1955 public 1956 IFhirResourceDao<org.hl7.fhir.r5.model.EvidenceVariable> 1957 daoEvidenceVariableR5() { 1958 1959 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.EvidenceVariable> retVal; 1960 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.EvidenceVariable>(); 1961 retVal.setResourceType(org.hl7.fhir.r5.model.EvidenceVariable.class); 1962 retVal.setContext(myFhirContext); 1963 return retVal; 1964 } 1965 1966 @Bean(name="myEvidenceVariableRpR5") 1967 @Lazy 1968 public ca.uhn.fhir.jpa.rp.r5.EvidenceVariableResourceProvider rpEvidenceVariableR5() { 1969 ca.uhn.fhir.jpa.rp.r5.EvidenceVariableResourceProvider retVal; 1970 retVal = new ca.uhn.fhir.jpa.rp.r5.EvidenceVariableResourceProvider(); 1971 retVal.setContext(myFhirContext); 1972 retVal.setDao(daoEvidenceVariableR5()); 1973 return retVal; 1974 } 1975 1976 @Bean(name="myExampleScenarioDaoR5") 1977 public 1978 IFhirResourceDao<org.hl7.fhir.r5.model.ExampleScenario> 1979 daoExampleScenarioR5() { 1980 1981 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ExampleScenario> retVal; 1982 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ExampleScenario>(); 1983 retVal.setResourceType(org.hl7.fhir.r5.model.ExampleScenario.class); 1984 retVal.setContext(myFhirContext); 1985 return retVal; 1986 } 1987 1988 @Bean(name="myExampleScenarioRpR5") 1989 @Lazy 1990 public ca.uhn.fhir.jpa.rp.r5.ExampleScenarioResourceProvider rpExampleScenarioR5() { 1991 ca.uhn.fhir.jpa.rp.r5.ExampleScenarioResourceProvider retVal; 1992 retVal = new ca.uhn.fhir.jpa.rp.r5.ExampleScenarioResourceProvider(); 1993 retVal.setContext(myFhirContext); 1994 retVal.setDao(daoExampleScenarioR5()); 1995 return retVal; 1996 } 1997 1998 @Bean(name="myExplanationOfBenefitDaoR5") 1999 public 2000 IFhirResourceDao<org.hl7.fhir.r5.model.ExplanationOfBenefit> 2001 daoExplanationOfBenefitR5() { 2002 2003 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ExplanationOfBenefit> retVal; 2004 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ExplanationOfBenefit>(); 2005 retVal.setResourceType(org.hl7.fhir.r5.model.ExplanationOfBenefit.class); 2006 retVal.setContext(myFhirContext); 2007 return retVal; 2008 } 2009 2010 @Bean(name="myExplanationOfBenefitRpR5") 2011 @Lazy 2012 public ca.uhn.fhir.jpa.rp.r5.ExplanationOfBenefitResourceProvider rpExplanationOfBenefitR5() { 2013 ca.uhn.fhir.jpa.rp.r5.ExplanationOfBenefitResourceProvider retVal; 2014 retVal = new ca.uhn.fhir.jpa.rp.r5.ExplanationOfBenefitResourceProvider(); 2015 retVal.setContext(myFhirContext); 2016 retVal.setDao(daoExplanationOfBenefitR5()); 2017 return retVal; 2018 } 2019 2020 @Bean(name="myFamilyMemberHistoryDaoR5") 2021 public 2022 IFhirResourceDao<org.hl7.fhir.r5.model.FamilyMemberHistory> 2023 daoFamilyMemberHistoryR5() { 2024 2025 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.FamilyMemberHistory> retVal; 2026 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.FamilyMemberHistory>(); 2027 retVal.setResourceType(org.hl7.fhir.r5.model.FamilyMemberHistory.class); 2028 retVal.setContext(myFhirContext); 2029 return retVal; 2030 } 2031 2032 @Bean(name="myFamilyMemberHistoryRpR5") 2033 @Lazy 2034 public ca.uhn.fhir.jpa.rp.r5.FamilyMemberHistoryResourceProvider rpFamilyMemberHistoryR5() { 2035 ca.uhn.fhir.jpa.rp.r5.FamilyMemberHistoryResourceProvider retVal; 2036 retVal = new ca.uhn.fhir.jpa.rp.r5.FamilyMemberHistoryResourceProvider(); 2037 retVal.setContext(myFhirContext); 2038 retVal.setDao(daoFamilyMemberHistoryR5()); 2039 return retVal; 2040 } 2041 2042 @Bean(name="myFlagDaoR5") 2043 public 2044 IFhirResourceDao<org.hl7.fhir.r5.model.Flag> 2045 daoFlagR5() { 2046 2047 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Flag> retVal; 2048 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Flag>(); 2049 retVal.setResourceType(org.hl7.fhir.r5.model.Flag.class); 2050 retVal.setContext(myFhirContext); 2051 return retVal; 2052 } 2053 2054 @Bean(name="myFlagRpR5") 2055 @Lazy 2056 public ca.uhn.fhir.jpa.rp.r5.FlagResourceProvider rpFlagR5() { 2057 ca.uhn.fhir.jpa.rp.r5.FlagResourceProvider retVal; 2058 retVal = new ca.uhn.fhir.jpa.rp.r5.FlagResourceProvider(); 2059 retVal.setContext(myFhirContext); 2060 retVal.setDao(daoFlagR5()); 2061 return retVal; 2062 } 2063 2064 @Bean(name="myFormularyItemDaoR5") 2065 public 2066 IFhirResourceDao<org.hl7.fhir.r5.model.FormularyItem> 2067 daoFormularyItemR5() { 2068 2069 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.FormularyItem> retVal; 2070 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.FormularyItem>(); 2071 retVal.setResourceType(org.hl7.fhir.r5.model.FormularyItem.class); 2072 retVal.setContext(myFhirContext); 2073 return retVal; 2074 } 2075 2076 @Bean(name="myFormularyItemRpR5") 2077 @Lazy 2078 public ca.uhn.fhir.jpa.rp.r5.FormularyItemResourceProvider rpFormularyItemR5() { 2079 ca.uhn.fhir.jpa.rp.r5.FormularyItemResourceProvider retVal; 2080 retVal = new ca.uhn.fhir.jpa.rp.r5.FormularyItemResourceProvider(); 2081 retVal.setContext(myFhirContext); 2082 retVal.setDao(daoFormularyItemR5()); 2083 return retVal; 2084 } 2085 2086 @Bean(name="myGenomicStudyDaoR5") 2087 public 2088 IFhirResourceDao<org.hl7.fhir.r5.model.GenomicStudy> 2089 daoGenomicStudyR5() { 2090 2091 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.GenomicStudy> retVal; 2092 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.GenomicStudy>(); 2093 retVal.setResourceType(org.hl7.fhir.r5.model.GenomicStudy.class); 2094 retVal.setContext(myFhirContext); 2095 return retVal; 2096 } 2097 2098 @Bean(name="myGenomicStudyRpR5") 2099 @Lazy 2100 public ca.uhn.fhir.jpa.rp.r5.GenomicStudyResourceProvider rpGenomicStudyR5() { 2101 ca.uhn.fhir.jpa.rp.r5.GenomicStudyResourceProvider retVal; 2102 retVal = new ca.uhn.fhir.jpa.rp.r5.GenomicStudyResourceProvider(); 2103 retVal.setContext(myFhirContext); 2104 retVal.setDao(daoGenomicStudyR5()); 2105 return retVal; 2106 } 2107 2108 @Bean(name="myGoalDaoR5") 2109 public 2110 IFhirResourceDao<org.hl7.fhir.r5.model.Goal> 2111 daoGoalR5() { 2112 2113 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Goal> retVal; 2114 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Goal>(); 2115 retVal.setResourceType(org.hl7.fhir.r5.model.Goal.class); 2116 retVal.setContext(myFhirContext); 2117 return retVal; 2118 } 2119 2120 @Bean(name="myGoalRpR5") 2121 @Lazy 2122 public ca.uhn.fhir.jpa.rp.r5.GoalResourceProvider rpGoalR5() { 2123 ca.uhn.fhir.jpa.rp.r5.GoalResourceProvider retVal; 2124 retVal = new ca.uhn.fhir.jpa.rp.r5.GoalResourceProvider(); 2125 retVal.setContext(myFhirContext); 2126 retVal.setDao(daoGoalR5()); 2127 return retVal; 2128 } 2129 2130 @Bean(name="myGraphDefinitionDaoR5") 2131 public 2132 IFhirResourceDao<org.hl7.fhir.r5.model.GraphDefinition> 2133 daoGraphDefinitionR5() { 2134 2135 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.GraphDefinition> retVal; 2136 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.GraphDefinition>(); 2137 retVal.setResourceType(org.hl7.fhir.r5.model.GraphDefinition.class); 2138 retVal.setContext(myFhirContext); 2139 return retVal; 2140 } 2141 2142 @Bean(name="myGraphDefinitionRpR5") 2143 @Lazy 2144 public ca.uhn.fhir.jpa.rp.r5.GraphDefinitionResourceProvider rpGraphDefinitionR5() { 2145 ca.uhn.fhir.jpa.rp.r5.GraphDefinitionResourceProvider retVal; 2146 retVal = new ca.uhn.fhir.jpa.rp.r5.GraphDefinitionResourceProvider(); 2147 retVal.setContext(myFhirContext); 2148 retVal.setDao(daoGraphDefinitionR5()); 2149 return retVal; 2150 } 2151 2152 @Bean(name="myGroupDaoR5") 2153 public 2154 IFhirResourceDao<org.hl7.fhir.r5.model.Group> 2155 daoGroupR5() { 2156 2157 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Group> retVal; 2158 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Group>(); 2159 retVal.setResourceType(org.hl7.fhir.r5.model.Group.class); 2160 retVal.setContext(myFhirContext); 2161 return retVal; 2162 } 2163 2164 @Bean(name="myGroupRpR5") 2165 @Lazy 2166 public ca.uhn.fhir.jpa.rp.r5.GroupResourceProvider rpGroupR5() { 2167 ca.uhn.fhir.jpa.rp.r5.GroupResourceProvider retVal; 2168 retVal = new ca.uhn.fhir.jpa.rp.r5.GroupResourceProvider(); 2169 retVal.setContext(myFhirContext); 2170 retVal.setDao(daoGroupR5()); 2171 return retVal; 2172 } 2173 2174 @Bean(name="myGuidanceResponseDaoR5") 2175 public 2176 IFhirResourceDao<org.hl7.fhir.r5.model.GuidanceResponse> 2177 daoGuidanceResponseR5() { 2178 2179 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.GuidanceResponse> retVal; 2180 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.GuidanceResponse>(); 2181 retVal.setResourceType(org.hl7.fhir.r5.model.GuidanceResponse.class); 2182 retVal.setContext(myFhirContext); 2183 return retVal; 2184 } 2185 2186 @Bean(name="myGuidanceResponseRpR5") 2187 @Lazy 2188 public ca.uhn.fhir.jpa.rp.r5.GuidanceResponseResourceProvider rpGuidanceResponseR5() { 2189 ca.uhn.fhir.jpa.rp.r5.GuidanceResponseResourceProvider retVal; 2190 retVal = new ca.uhn.fhir.jpa.rp.r5.GuidanceResponseResourceProvider(); 2191 retVal.setContext(myFhirContext); 2192 retVal.setDao(daoGuidanceResponseR5()); 2193 return retVal; 2194 } 2195 2196 @Bean(name="myHealthcareServiceDaoR5") 2197 public 2198 IFhirResourceDao<org.hl7.fhir.r5.model.HealthcareService> 2199 daoHealthcareServiceR5() { 2200 2201 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.HealthcareService> retVal; 2202 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.HealthcareService>(); 2203 retVal.setResourceType(org.hl7.fhir.r5.model.HealthcareService.class); 2204 retVal.setContext(myFhirContext); 2205 return retVal; 2206 } 2207 2208 @Bean(name="myHealthcareServiceRpR5") 2209 @Lazy 2210 public ca.uhn.fhir.jpa.rp.r5.HealthcareServiceResourceProvider rpHealthcareServiceR5() { 2211 ca.uhn.fhir.jpa.rp.r5.HealthcareServiceResourceProvider retVal; 2212 retVal = new ca.uhn.fhir.jpa.rp.r5.HealthcareServiceResourceProvider(); 2213 retVal.setContext(myFhirContext); 2214 retVal.setDao(daoHealthcareServiceR5()); 2215 return retVal; 2216 } 2217 2218 @Bean(name="myImagingSelectionDaoR5") 2219 public 2220 IFhirResourceDao<org.hl7.fhir.r5.model.ImagingSelection> 2221 daoImagingSelectionR5() { 2222 2223 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ImagingSelection> retVal; 2224 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ImagingSelection>(); 2225 retVal.setResourceType(org.hl7.fhir.r5.model.ImagingSelection.class); 2226 retVal.setContext(myFhirContext); 2227 return retVal; 2228 } 2229 2230 @Bean(name="myImagingSelectionRpR5") 2231 @Lazy 2232 public ca.uhn.fhir.jpa.rp.r5.ImagingSelectionResourceProvider rpImagingSelectionR5() { 2233 ca.uhn.fhir.jpa.rp.r5.ImagingSelectionResourceProvider retVal; 2234 retVal = new ca.uhn.fhir.jpa.rp.r5.ImagingSelectionResourceProvider(); 2235 retVal.setContext(myFhirContext); 2236 retVal.setDao(daoImagingSelectionR5()); 2237 return retVal; 2238 } 2239 2240 @Bean(name="myImagingStudyDaoR5") 2241 public 2242 IFhirResourceDao<org.hl7.fhir.r5.model.ImagingStudy> 2243 daoImagingStudyR5() { 2244 2245 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ImagingStudy> retVal; 2246 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ImagingStudy>(); 2247 retVal.setResourceType(org.hl7.fhir.r5.model.ImagingStudy.class); 2248 retVal.setContext(myFhirContext); 2249 return retVal; 2250 } 2251 2252 @Bean(name="myImagingStudyRpR5") 2253 @Lazy 2254 public ca.uhn.fhir.jpa.rp.r5.ImagingStudyResourceProvider rpImagingStudyR5() { 2255 ca.uhn.fhir.jpa.rp.r5.ImagingStudyResourceProvider retVal; 2256 retVal = new ca.uhn.fhir.jpa.rp.r5.ImagingStudyResourceProvider(); 2257 retVal.setContext(myFhirContext); 2258 retVal.setDao(daoImagingStudyR5()); 2259 return retVal; 2260 } 2261 2262 @Bean(name="myImmunizationDaoR5") 2263 public 2264 IFhirResourceDao<org.hl7.fhir.r5.model.Immunization> 2265 daoImmunizationR5() { 2266 2267 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Immunization> retVal; 2268 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Immunization>(); 2269 retVal.setResourceType(org.hl7.fhir.r5.model.Immunization.class); 2270 retVal.setContext(myFhirContext); 2271 return retVal; 2272 } 2273 2274 @Bean(name="myImmunizationRpR5") 2275 @Lazy 2276 public ca.uhn.fhir.jpa.rp.r5.ImmunizationResourceProvider rpImmunizationR5() { 2277 ca.uhn.fhir.jpa.rp.r5.ImmunizationResourceProvider retVal; 2278 retVal = new ca.uhn.fhir.jpa.rp.r5.ImmunizationResourceProvider(); 2279 retVal.setContext(myFhirContext); 2280 retVal.setDao(daoImmunizationR5()); 2281 return retVal; 2282 } 2283 2284 @Bean(name="myImmunizationEvaluationDaoR5") 2285 public 2286 IFhirResourceDao<org.hl7.fhir.r5.model.ImmunizationEvaluation> 2287 daoImmunizationEvaluationR5() { 2288 2289 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ImmunizationEvaluation> retVal; 2290 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ImmunizationEvaluation>(); 2291 retVal.setResourceType(org.hl7.fhir.r5.model.ImmunizationEvaluation.class); 2292 retVal.setContext(myFhirContext); 2293 return retVal; 2294 } 2295 2296 @Bean(name="myImmunizationEvaluationRpR5") 2297 @Lazy 2298 public ca.uhn.fhir.jpa.rp.r5.ImmunizationEvaluationResourceProvider rpImmunizationEvaluationR5() { 2299 ca.uhn.fhir.jpa.rp.r5.ImmunizationEvaluationResourceProvider retVal; 2300 retVal = new ca.uhn.fhir.jpa.rp.r5.ImmunizationEvaluationResourceProvider(); 2301 retVal.setContext(myFhirContext); 2302 retVal.setDao(daoImmunizationEvaluationR5()); 2303 return retVal; 2304 } 2305 2306 @Bean(name="myImmunizationRecommendationDaoR5") 2307 public 2308 IFhirResourceDao<org.hl7.fhir.r5.model.ImmunizationRecommendation> 2309 daoImmunizationRecommendationR5() { 2310 2311 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ImmunizationRecommendation> retVal; 2312 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ImmunizationRecommendation>(); 2313 retVal.setResourceType(org.hl7.fhir.r5.model.ImmunizationRecommendation.class); 2314 retVal.setContext(myFhirContext); 2315 return retVal; 2316 } 2317 2318 @Bean(name="myImmunizationRecommendationRpR5") 2319 @Lazy 2320 public ca.uhn.fhir.jpa.rp.r5.ImmunizationRecommendationResourceProvider rpImmunizationRecommendationR5() { 2321 ca.uhn.fhir.jpa.rp.r5.ImmunizationRecommendationResourceProvider retVal; 2322 retVal = new ca.uhn.fhir.jpa.rp.r5.ImmunizationRecommendationResourceProvider(); 2323 retVal.setContext(myFhirContext); 2324 retVal.setDao(daoImmunizationRecommendationR5()); 2325 return retVal; 2326 } 2327 2328 @Bean(name="myImplementationGuideDaoR5") 2329 public 2330 IFhirResourceDao<org.hl7.fhir.r5.model.ImplementationGuide> 2331 daoImplementationGuideR5() { 2332 2333 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ImplementationGuide> retVal; 2334 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ImplementationGuide>(); 2335 retVal.setResourceType(org.hl7.fhir.r5.model.ImplementationGuide.class); 2336 retVal.setContext(myFhirContext); 2337 return retVal; 2338 } 2339 2340 @Bean(name="myImplementationGuideRpR5") 2341 @Lazy 2342 public ca.uhn.fhir.jpa.rp.r5.ImplementationGuideResourceProvider rpImplementationGuideR5() { 2343 ca.uhn.fhir.jpa.rp.r5.ImplementationGuideResourceProvider retVal; 2344 retVal = new ca.uhn.fhir.jpa.rp.r5.ImplementationGuideResourceProvider(); 2345 retVal.setContext(myFhirContext); 2346 retVal.setDao(daoImplementationGuideR5()); 2347 return retVal; 2348 } 2349 2350 @Bean(name="myIngredientDaoR5") 2351 public 2352 IFhirResourceDao<org.hl7.fhir.r5.model.Ingredient> 2353 daoIngredientR5() { 2354 2355 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Ingredient> retVal; 2356 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Ingredient>(); 2357 retVal.setResourceType(org.hl7.fhir.r5.model.Ingredient.class); 2358 retVal.setContext(myFhirContext); 2359 return retVal; 2360 } 2361 2362 @Bean(name="myIngredientRpR5") 2363 @Lazy 2364 public ca.uhn.fhir.jpa.rp.r5.IngredientResourceProvider rpIngredientR5() { 2365 ca.uhn.fhir.jpa.rp.r5.IngredientResourceProvider retVal; 2366 retVal = new ca.uhn.fhir.jpa.rp.r5.IngredientResourceProvider(); 2367 retVal.setContext(myFhirContext); 2368 retVal.setDao(daoIngredientR5()); 2369 return retVal; 2370 } 2371 2372 @Bean(name="myInsurancePlanDaoR5") 2373 public 2374 IFhirResourceDao<org.hl7.fhir.r5.model.InsurancePlan> 2375 daoInsurancePlanR5() { 2376 2377 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.InsurancePlan> retVal; 2378 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.InsurancePlan>(); 2379 retVal.setResourceType(org.hl7.fhir.r5.model.InsurancePlan.class); 2380 retVal.setContext(myFhirContext); 2381 return retVal; 2382 } 2383 2384 @Bean(name="myInsurancePlanRpR5") 2385 @Lazy 2386 public ca.uhn.fhir.jpa.rp.r5.InsurancePlanResourceProvider rpInsurancePlanR5() { 2387 ca.uhn.fhir.jpa.rp.r5.InsurancePlanResourceProvider retVal; 2388 retVal = new ca.uhn.fhir.jpa.rp.r5.InsurancePlanResourceProvider(); 2389 retVal.setContext(myFhirContext); 2390 retVal.setDao(daoInsurancePlanR5()); 2391 return retVal; 2392 } 2393 2394 @Bean(name="myInventoryItemDaoR5") 2395 public 2396 IFhirResourceDao<org.hl7.fhir.r5.model.InventoryItem> 2397 daoInventoryItemR5() { 2398 2399 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.InventoryItem> retVal; 2400 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.InventoryItem>(); 2401 retVal.setResourceType(org.hl7.fhir.r5.model.InventoryItem.class); 2402 retVal.setContext(myFhirContext); 2403 return retVal; 2404 } 2405 2406 @Bean(name="myInventoryItemRpR5") 2407 @Lazy 2408 public ca.uhn.fhir.jpa.rp.r5.InventoryItemResourceProvider rpInventoryItemR5() { 2409 ca.uhn.fhir.jpa.rp.r5.InventoryItemResourceProvider retVal; 2410 retVal = new ca.uhn.fhir.jpa.rp.r5.InventoryItemResourceProvider(); 2411 retVal.setContext(myFhirContext); 2412 retVal.setDao(daoInventoryItemR5()); 2413 return retVal; 2414 } 2415 2416 @Bean(name="myInventoryReportDaoR5") 2417 public 2418 IFhirResourceDao<org.hl7.fhir.r5.model.InventoryReport> 2419 daoInventoryReportR5() { 2420 2421 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.InventoryReport> retVal; 2422 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.InventoryReport>(); 2423 retVal.setResourceType(org.hl7.fhir.r5.model.InventoryReport.class); 2424 retVal.setContext(myFhirContext); 2425 return retVal; 2426 } 2427 2428 @Bean(name="myInventoryReportRpR5") 2429 @Lazy 2430 public ca.uhn.fhir.jpa.rp.r5.InventoryReportResourceProvider rpInventoryReportR5() { 2431 ca.uhn.fhir.jpa.rp.r5.InventoryReportResourceProvider retVal; 2432 retVal = new ca.uhn.fhir.jpa.rp.r5.InventoryReportResourceProvider(); 2433 retVal.setContext(myFhirContext); 2434 retVal.setDao(daoInventoryReportR5()); 2435 return retVal; 2436 } 2437 2438 @Bean(name="myInvoiceDaoR5") 2439 public 2440 IFhirResourceDao<org.hl7.fhir.r5.model.Invoice> 2441 daoInvoiceR5() { 2442 2443 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Invoice> retVal; 2444 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Invoice>(); 2445 retVal.setResourceType(org.hl7.fhir.r5.model.Invoice.class); 2446 retVal.setContext(myFhirContext); 2447 return retVal; 2448 } 2449 2450 @Bean(name="myInvoiceRpR5") 2451 @Lazy 2452 public ca.uhn.fhir.jpa.rp.r5.InvoiceResourceProvider rpInvoiceR5() { 2453 ca.uhn.fhir.jpa.rp.r5.InvoiceResourceProvider retVal; 2454 retVal = new ca.uhn.fhir.jpa.rp.r5.InvoiceResourceProvider(); 2455 retVal.setContext(myFhirContext); 2456 retVal.setDao(daoInvoiceR5()); 2457 return retVal; 2458 } 2459 2460 @Bean(name="myLibraryDaoR5") 2461 public 2462 IFhirResourceDao<org.hl7.fhir.r5.model.Library> 2463 daoLibraryR5() { 2464 2465 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Library> retVal; 2466 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Library>(); 2467 retVal.setResourceType(org.hl7.fhir.r5.model.Library.class); 2468 retVal.setContext(myFhirContext); 2469 return retVal; 2470 } 2471 2472 @Bean(name="myLibraryRpR5") 2473 @Lazy 2474 public ca.uhn.fhir.jpa.rp.r5.LibraryResourceProvider rpLibraryR5() { 2475 ca.uhn.fhir.jpa.rp.r5.LibraryResourceProvider retVal; 2476 retVal = new ca.uhn.fhir.jpa.rp.r5.LibraryResourceProvider(); 2477 retVal.setContext(myFhirContext); 2478 retVal.setDao(daoLibraryR5()); 2479 return retVal; 2480 } 2481 2482 @Bean(name="myLinkageDaoR5") 2483 public 2484 IFhirResourceDao<org.hl7.fhir.r5.model.Linkage> 2485 daoLinkageR5() { 2486 2487 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Linkage> retVal; 2488 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Linkage>(); 2489 retVal.setResourceType(org.hl7.fhir.r5.model.Linkage.class); 2490 retVal.setContext(myFhirContext); 2491 return retVal; 2492 } 2493 2494 @Bean(name="myLinkageRpR5") 2495 @Lazy 2496 public ca.uhn.fhir.jpa.rp.r5.LinkageResourceProvider rpLinkageR5() { 2497 ca.uhn.fhir.jpa.rp.r5.LinkageResourceProvider retVal; 2498 retVal = new ca.uhn.fhir.jpa.rp.r5.LinkageResourceProvider(); 2499 retVal.setContext(myFhirContext); 2500 retVal.setDao(daoLinkageR5()); 2501 return retVal; 2502 } 2503 2504 @Bean(name="myListDaoR5") 2505 public 2506 IFhirResourceDao<org.hl7.fhir.r5.model.ListResource> 2507 daoListResourceR5() { 2508 2509 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ListResource> retVal; 2510 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ListResource>(); 2511 retVal.setResourceType(org.hl7.fhir.r5.model.ListResource.class); 2512 retVal.setContext(myFhirContext); 2513 return retVal; 2514 } 2515 2516 @Bean(name="myListResourceRpR5") 2517 @Lazy 2518 public ca.uhn.fhir.jpa.rp.r5.ListResourceResourceProvider rpListResourceR5() { 2519 ca.uhn.fhir.jpa.rp.r5.ListResourceResourceProvider retVal; 2520 retVal = new ca.uhn.fhir.jpa.rp.r5.ListResourceResourceProvider(); 2521 retVal.setContext(myFhirContext); 2522 retVal.setDao(daoListResourceR5()); 2523 return retVal; 2524 } 2525 2526 @Bean(name="myLocationDaoR5") 2527 public 2528 IFhirResourceDao<org.hl7.fhir.r5.model.Location> 2529 daoLocationR5() { 2530 2531 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Location> retVal; 2532 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Location>(); 2533 retVal.setResourceType(org.hl7.fhir.r5.model.Location.class); 2534 retVal.setContext(myFhirContext); 2535 return retVal; 2536 } 2537 2538 @Bean(name="myLocationRpR5") 2539 @Lazy 2540 public ca.uhn.fhir.jpa.rp.r5.LocationResourceProvider rpLocationR5() { 2541 ca.uhn.fhir.jpa.rp.r5.LocationResourceProvider retVal; 2542 retVal = new ca.uhn.fhir.jpa.rp.r5.LocationResourceProvider(); 2543 retVal.setContext(myFhirContext); 2544 retVal.setDao(daoLocationR5()); 2545 return retVal; 2546 } 2547 2548 @Bean(name="myManufacturedItemDefinitionDaoR5") 2549 public 2550 IFhirResourceDao<org.hl7.fhir.r5.model.ManufacturedItemDefinition> 2551 daoManufacturedItemDefinitionR5() { 2552 2553 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ManufacturedItemDefinition> retVal; 2554 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ManufacturedItemDefinition>(); 2555 retVal.setResourceType(org.hl7.fhir.r5.model.ManufacturedItemDefinition.class); 2556 retVal.setContext(myFhirContext); 2557 return retVal; 2558 } 2559 2560 @Bean(name="myManufacturedItemDefinitionRpR5") 2561 @Lazy 2562 public ca.uhn.fhir.jpa.rp.r5.ManufacturedItemDefinitionResourceProvider rpManufacturedItemDefinitionR5() { 2563 ca.uhn.fhir.jpa.rp.r5.ManufacturedItemDefinitionResourceProvider retVal; 2564 retVal = new ca.uhn.fhir.jpa.rp.r5.ManufacturedItemDefinitionResourceProvider(); 2565 retVal.setContext(myFhirContext); 2566 retVal.setDao(daoManufacturedItemDefinitionR5()); 2567 return retVal; 2568 } 2569 2570 @Bean(name="myMeasureDaoR5") 2571 public 2572 IFhirResourceDao<org.hl7.fhir.r5.model.Measure> 2573 daoMeasureR5() { 2574 2575 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Measure> retVal; 2576 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Measure>(); 2577 retVal.setResourceType(org.hl7.fhir.r5.model.Measure.class); 2578 retVal.setContext(myFhirContext); 2579 return retVal; 2580 } 2581 2582 @Bean(name="myMeasureRpR5") 2583 @Lazy 2584 public ca.uhn.fhir.jpa.rp.r5.MeasureResourceProvider rpMeasureR5() { 2585 ca.uhn.fhir.jpa.rp.r5.MeasureResourceProvider retVal; 2586 retVal = new ca.uhn.fhir.jpa.rp.r5.MeasureResourceProvider(); 2587 retVal.setContext(myFhirContext); 2588 retVal.setDao(daoMeasureR5()); 2589 return retVal; 2590 } 2591 2592 @Bean(name="myMeasureReportDaoR5") 2593 public 2594 IFhirResourceDao<org.hl7.fhir.r5.model.MeasureReport> 2595 daoMeasureReportR5() { 2596 2597 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MeasureReport> retVal; 2598 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MeasureReport>(); 2599 retVal.setResourceType(org.hl7.fhir.r5.model.MeasureReport.class); 2600 retVal.setContext(myFhirContext); 2601 return retVal; 2602 } 2603 2604 @Bean(name="myMeasureReportRpR5") 2605 @Lazy 2606 public ca.uhn.fhir.jpa.rp.r5.MeasureReportResourceProvider rpMeasureReportR5() { 2607 ca.uhn.fhir.jpa.rp.r5.MeasureReportResourceProvider retVal; 2608 retVal = new ca.uhn.fhir.jpa.rp.r5.MeasureReportResourceProvider(); 2609 retVal.setContext(myFhirContext); 2610 retVal.setDao(daoMeasureReportR5()); 2611 return retVal; 2612 } 2613 2614 @Bean(name="myMedicationDaoR5") 2615 public 2616 IFhirResourceDao<org.hl7.fhir.r5.model.Medication> 2617 daoMedicationR5() { 2618 2619 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Medication> retVal; 2620 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Medication>(); 2621 retVal.setResourceType(org.hl7.fhir.r5.model.Medication.class); 2622 retVal.setContext(myFhirContext); 2623 return retVal; 2624 } 2625 2626 @Bean(name="myMedicationRpR5") 2627 @Lazy 2628 public ca.uhn.fhir.jpa.rp.r5.MedicationResourceProvider rpMedicationR5() { 2629 ca.uhn.fhir.jpa.rp.r5.MedicationResourceProvider retVal; 2630 retVal = new ca.uhn.fhir.jpa.rp.r5.MedicationResourceProvider(); 2631 retVal.setContext(myFhirContext); 2632 retVal.setDao(daoMedicationR5()); 2633 return retVal; 2634 } 2635 2636 @Bean(name="myMedicationAdministrationDaoR5") 2637 public 2638 IFhirResourceDao<org.hl7.fhir.r5.model.MedicationAdministration> 2639 daoMedicationAdministrationR5() { 2640 2641 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MedicationAdministration> retVal; 2642 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MedicationAdministration>(); 2643 retVal.setResourceType(org.hl7.fhir.r5.model.MedicationAdministration.class); 2644 retVal.setContext(myFhirContext); 2645 return retVal; 2646 } 2647 2648 @Bean(name="myMedicationAdministrationRpR5") 2649 @Lazy 2650 public ca.uhn.fhir.jpa.rp.r5.MedicationAdministrationResourceProvider rpMedicationAdministrationR5() { 2651 ca.uhn.fhir.jpa.rp.r5.MedicationAdministrationResourceProvider retVal; 2652 retVal = new ca.uhn.fhir.jpa.rp.r5.MedicationAdministrationResourceProvider(); 2653 retVal.setContext(myFhirContext); 2654 retVal.setDao(daoMedicationAdministrationR5()); 2655 return retVal; 2656 } 2657 2658 @Bean(name="myMedicationDispenseDaoR5") 2659 public 2660 IFhirResourceDao<org.hl7.fhir.r5.model.MedicationDispense> 2661 daoMedicationDispenseR5() { 2662 2663 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MedicationDispense> retVal; 2664 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MedicationDispense>(); 2665 retVal.setResourceType(org.hl7.fhir.r5.model.MedicationDispense.class); 2666 retVal.setContext(myFhirContext); 2667 return retVal; 2668 } 2669 2670 @Bean(name="myMedicationDispenseRpR5") 2671 @Lazy 2672 public ca.uhn.fhir.jpa.rp.r5.MedicationDispenseResourceProvider rpMedicationDispenseR5() { 2673 ca.uhn.fhir.jpa.rp.r5.MedicationDispenseResourceProvider retVal; 2674 retVal = new ca.uhn.fhir.jpa.rp.r5.MedicationDispenseResourceProvider(); 2675 retVal.setContext(myFhirContext); 2676 retVal.setDao(daoMedicationDispenseR5()); 2677 return retVal; 2678 } 2679 2680 @Bean(name="myMedicationKnowledgeDaoR5") 2681 public 2682 IFhirResourceDao<org.hl7.fhir.r5.model.MedicationKnowledge> 2683 daoMedicationKnowledgeR5() { 2684 2685 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MedicationKnowledge> retVal; 2686 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MedicationKnowledge>(); 2687 retVal.setResourceType(org.hl7.fhir.r5.model.MedicationKnowledge.class); 2688 retVal.setContext(myFhirContext); 2689 return retVal; 2690 } 2691 2692 @Bean(name="myMedicationKnowledgeRpR5") 2693 @Lazy 2694 public ca.uhn.fhir.jpa.rp.r5.MedicationKnowledgeResourceProvider rpMedicationKnowledgeR5() { 2695 ca.uhn.fhir.jpa.rp.r5.MedicationKnowledgeResourceProvider retVal; 2696 retVal = new ca.uhn.fhir.jpa.rp.r5.MedicationKnowledgeResourceProvider(); 2697 retVal.setContext(myFhirContext); 2698 retVal.setDao(daoMedicationKnowledgeR5()); 2699 return retVal; 2700 } 2701 2702 @Bean(name="myMedicationRequestDaoR5") 2703 public 2704 IFhirResourceDao<org.hl7.fhir.r5.model.MedicationRequest> 2705 daoMedicationRequestR5() { 2706 2707 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MedicationRequest> retVal; 2708 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MedicationRequest>(); 2709 retVal.setResourceType(org.hl7.fhir.r5.model.MedicationRequest.class); 2710 retVal.setContext(myFhirContext); 2711 return retVal; 2712 } 2713 2714 @Bean(name="myMedicationRequestRpR5") 2715 @Lazy 2716 public ca.uhn.fhir.jpa.rp.r5.MedicationRequestResourceProvider rpMedicationRequestR5() { 2717 ca.uhn.fhir.jpa.rp.r5.MedicationRequestResourceProvider retVal; 2718 retVal = new ca.uhn.fhir.jpa.rp.r5.MedicationRequestResourceProvider(); 2719 retVal.setContext(myFhirContext); 2720 retVal.setDao(daoMedicationRequestR5()); 2721 return retVal; 2722 } 2723 2724 @Bean(name="myMedicationStatementDaoR5") 2725 public 2726 IFhirResourceDao<org.hl7.fhir.r5.model.MedicationStatement> 2727 daoMedicationStatementR5() { 2728 2729 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MedicationStatement> retVal; 2730 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MedicationStatement>(); 2731 retVal.setResourceType(org.hl7.fhir.r5.model.MedicationStatement.class); 2732 retVal.setContext(myFhirContext); 2733 return retVal; 2734 } 2735 2736 @Bean(name="myMedicationStatementRpR5") 2737 @Lazy 2738 public ca.uhn.fhir.jpa.rp.r5.MedicationStatementResourceProvider rpMedicationStatementR5() { 2739 ca.uhn.fhir.jpa.rp.r5.MedicationStatementResourceProvider retVal; 2740 retVal = new ca.uhn.fhir.jpa.rp.r5.MedicationStatementResourceProvider(); 2741 retVal.setContext(myFhirContext); 2742 retVal.setDao(daoMedicationStatementR5()); 2743 return retVal; 2744 } 2745 2746 @Bean(name="myMedicinalProductDefinitionDaoR5") 2747 public 2748 IFhirResourceDao<org.hl7.fhir.r5.model.MedicinalProductDefinition> 2749 daoMedicinalProductDefinitionR5() { 2750 2751 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MedicinalProductDefinition> retVal; 2752 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MedicinalProductDefinition>(); 2753 retVal.setResourceType(org.hl7.fhir.r5.model.MedicinalProductDefinition.class); 2754 retVal.setContext(myFhirContext); 2755 return retVal; 2756 } 2757 2758 @Bean(name="myMedicinalProductDefinitionRpR5") 2759 @Lazy 2760 public ca.uhn.fhir.jpa.rp.r5.MedicinalProductDefinitionResourceProvider rpMedicinalProductDefinitionR5() { 2761 ca.uhn.fhir.jpa.rp.r5.MedicinalProductDefinitionResourceProvider retVal; 2762 retVal = new ca.uhn.fhir.jpa.rp.r5.MedicinalProductDefinitionResourceProvider(); 2763 retVal.setContext(myFhirContext); 2764 retVal.setDao(daoMedicinalProductDefinitionR5()); 2765 return retVal; 2766 } 2767 2768 @Bean(name="myMessageDefinitionDaoR5") 2769 public 2770 IFhirResourceDao<org.hl7.fhir.r5.model.MessageDefinition> 2771 daoMessageDefinitionR5() { 2772 2773 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MessageDefinition> retVal; 2774 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MessageDefinition>(); 2775 retVal.setResourceType(org.hl7.fhir.r5.model.MessageDefinition.class); 2776 retVal.setContext(myFhirContext); 2777 return retVal; 2778 } 2779 2780 @Bean(name="myMessageDefinitionRpR5") 2781 @Lazy 2782 public ca.uhn.fhir.jpa.rp.r5.MessageDefinitionResourceProvider rpMessageDefinitionR5() { 2783 ca.uhn.fhir.jpa.rp.r5.MessageDefinitionResourceProvider retVal; 2784 retVal = new ca.uhn.fhir.jpa.rp.r5.MessageDefinitionResourceProvider(); 2785 retVal.setContext(myFhirContext); 2786 retVal.setDao(daoMessageDefinitionR5()); 2787 return retVal; 2788 } 2789 2790 @Bean(name="myMessageHeaderDaoR5") 2791 public 2792 IFhirResourceDao<org.hl7.fhir.r5.model.MessageHeader> 2793 daoMessageHeaderR5() { 2794 2795 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MessageHeader> retVal; 2796 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MessageHeader>(); 2797 retVal.setResourceType(org.hl7.fhir.r5.model.MessageHeader.class); 2798 retVal.setContext(myFhirContext); 2799 return retVal; 2800 } 2801 2802 @Bean(name="myMessageHeaderRpR5") 2803 @Lazy 2804 public ca.uhn.fhir.jpa.rp.r5.MessageHeaderResourceProvider rpMessageHeaderR5() { 2805 ca.uhn.fhir.jpa.rp.r5.MessageHeaderResourceProvider retVal; 2806 retVal = new ca.uhn.fhir.jpa.rp.r5.MessageHeaderResourceProvider(); 2807 retVal.setContext(myFhirContext); 2808 retVal.setDao(daoMessageHeaderR5()); 2809 return retVal; 2810 } 2811 2812 @Bean(name="myMolecularSequenceDaoR5") 2813 public 2814 IFhirResourceDao<org.hl7.fhir.r5.model.MolecularSequence> 2815 daoMolecularSequenceR5() { 2816 2817 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.MolecularSequence> retVal; 2818 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.MolecularSequence>(); 2819 retVal.setResourceType(org.hl7.fhir.r5.model.MolecularSequence.class); 2820 retVal.setContext(myFhirContext); 2821 return retVal; 2822 } 2823 2824 @Bean(name="myMolecularSequenceRpR5") 2825 @Lazy 2826 public ca.uhn.fhir.jpa.rp.r5.MolecularSequenceResourceProvider rpMolecularSequenceR5() { 2827 ca.uhn.fhir.jpa.rp.r5.MolecularSequenceResourceProvider retVal; 2828 retVal = new ca.uhn.fhir.jpa.rp.r5.MolecularSequenceResourceProvider(); 2829 retVal.setContext(myFhirContext); 2830 retVal.setDao(daoMolecularSequenceR5()); 2831 return retVal; 2832 } 2833 2834 @Bean(name="myNamingSystemDaoR5") 2835 public 2836 IFhirResourceDao<org.hl7.fhir.r5.model.NamingSystem> 2837 daoNamingSystemR5() { 2838 2839 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.NamingSystem> retVal; 2840 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.NamingSystem>(); 2841 retVal.setResourceType(org.hl7.fhir.r5.model.NamingSystem.class); 2842 retVal.setContext(myFhirContext); 2843 return retVal; 2844 } 2845 2846 @Bean(name="myNamingSystemRpR5") 2847 @Lazy 2848 public ca.uhn.fhir.jpa.rp.r5.NamingSystemResourceProvider rpNamingSystemR5() { 2849 ca.uhn.fhir.jpa.rp.r5.NamingSystemResourceProvider retVal; 2850 retVal = new ca.uhn.fhir.jpa.rp.r5.NamingSystemResourceProvider(); 2851 retVal.setContext(myFhirContext); 2852 retVal.setDao(daoNamingSystemR5()); 2853 return retVal; 2854 } 2855 2856 @Bean(name="myNutritionIntakeDaoR5") 2857 public 2858 IFhirResourceDao<org.hl7.fhir.r5.model.NutritionIntake> 2859 daoNutritionIntakeR5() { 2860 2861 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.NutritionIntake> retVal; 2862 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.NutritionIntake>(); 2863 retVal.setResourceType(org.hl7.fhir.r5.model.NutritionIntake.class); 2864 retVal.setContext(myFhirContext); 2865 return retVal; 2866 } 2867 2868 @Bean(name="myNutritionIntakeRpR5") 2869 @Lazy 2870 public ca.uhn.fhir.jpa.rp.r5.NutritionIntakeResourceProvider rpNutritionIntakeR5() { 2871 ca.uhn.fhir.jpa.rp.r5.NutritionIntakeResourceProvider retVal; 2872 retVal = new ca.uhn.fhir.jpa.rp.r5.NutritionIntakeResourceProvider(); 2873 retVal.setContext(myFhirContext); 2874 retVal.setDao(daoNutritionIntakeR5()); 2875 return retVal; 2876 } 2877 2878 @Bean(name="myNutritionOrderDaoR5") 2879 public 2880 IFhirResourceDao<org.hl7.fhir.r5.model.NutritionOrder> 2881 daoNutritionOrderR5() { 2882 2883 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.NutritionOrder> retVal; 2884 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.NutritionOrder>(); 2885 retVal.setResourceType(org.hl7.fhir.r5.model.NutritionOrder.class); 2886 retVal.setContext(myFhirContext); 2887 return retVal; 2888 } 2889 2890 @Bean(name="myNutritionOrderRpR5") 2891 @Lazy 2892 public ca.uhn.fhir.jpa.rp.r5.NutritionOrderResourceProvider rpNutritionOrderR5() { 2893 ca.uhn.fhir.jpa.rp.r5.NutritionOrderResourceProvider retVal; 2894 retVal = new ca.uhn.fhir.jpa.rp.r5.NutritionOrderResourceProvider(); 2895 retVal.setContext(myFhirContext); 2896 retVal.setDao(daoNutritionOrderR5()); 2897 return retVal; 2898 } 2899 2900 @Bean(name="myNutritionProductDaoR5") 2901 public 2902 IFhirResourceDao<org.hl7.fhir.r5.model.NutritionProduct> 2903 daoNutritionProductR5() { 2904 2905 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.NutritionProduct> retVal; 2906 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.NutritionProduct>(); 2907 retVal.setResourceType(org.hl7.fhir.r5.model.NutritionProduct.class); 2908 retVal.setContext(myFhirContext); 2909 return retVal; 2910 } 2911 2912 @Bean(name="myNutritionProductRpR5") 2913 @Lazy 2914 public ca.uhn.fhir.jpa.rp.r5.NutritionProductResourceProvider rpNutritionProductR5() { 2915 ca.uhn.fhir.jpa.rp.r5.NutritionProductResourceProvider retVal; 2916 retVal = new ca.uhn.fhir.jpa.rp.r5.NutritionProductResourceProvider(); 2917 retVal.setContext(myFhirContext); 2918 retVal.setDao(daoNutritionProductR5()); 2919 return retVal; 2920 } 2921 2922 @Bean(name="myObservationDaoR5") 2923 public 2924 IFhirResourceDaoObservation<org.hl7.fhir.r5.model.Observation> 2925 daoObservationR5() { 2926 2927 ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<org.hl7.fhir.r5.model.Observation> retVal; 2928 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoObservation<>(); 2929 retVal.setResourceType(org.hl7.fhir.r5.model.Observation.class); 2930 retVal.setContext(myFhirContext); 2931 return retVal; 2932 } 2933 2934 @Bean(name="myObservationRpR5") 2935 @Lazy 2936 public ca.uhn.fhir.jpa.rp.r5.ObservationResourceProvider rpObservationR5() { 2937 ca.uhn.fhir.jpa.rp.r5.ObservationResourceProvider retVal; 2938 retVal = new ca.uhn.fhir.jpa.rp.r5.ObservationResourceProvider(); 2939 retVal.setContext(myFhirContext); 2940 retVal.setDao(daoObservationR5()); 2941 return retVal; 2942 } 2943 2944 @Bean(name="myObservationDefinitionDaoR5") 2945 public 2946 IFhirResourceDao<org.hl7.fhir.r5.model.ObservationDefinition> 2947 daoObservationDefinitionR5() { 2948 2949 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ObservationDefinition> retVal; 2950 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ObservationDefinition>(); 2951 retVal.setResourceType(org.hl7.fhir.r5.model.ObservationDefinition.class); 2952 retVal.setContext(myFhirContext); 2953 return retVal; 2954 } 2955 2956 @Bean(name="myObservationDefinitionRpR5") 2957 @Lazy 2958 public ca.uhn.fhir.jpa.rp.r5.ObservationDefinitionResourceProvider rpObservationDefinitionR5() { 2959 ca.uhn.fhir.jpa.rp.r5.ObservationDefinitionResourceProvider retVal; 2960 retVal = new ca.uhn.fhir.jpa.rp.r5.ObservationDefinitionResourceProvider(); 2961 retVal.setContext(myFhirContext); 2962 retVal.setDao(daoObservationDefinitionR5()); 2963 return retVal; 2964 } 2965 2966 @Bean(name="myOperationDefinitionDaoR5") 2967 public 2968 IFhirResourceDao<org.hl7.fhir.r5.model.OperationDefinition> 2969 daoOperationDefinitionR5() { 2970 2971 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.OperationDefinition> retVal; 2972 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.OperationDefinition>(); 2973 retVal.setResourceType(org.hl7.fhir.r5.model.OperationDefinition.class); 2974 retVal.setContext(myFhirContext); 2975 return retVal; 2976 } 2977 2978 @Bean(name="myOperationDefinitionRpR5") 2979 @Lazy 2980 public ca.uhn.fhir.jpa.rp.r5.OperationDefinitionResourceProvider rpOperationDefinitionR5() { 2981 ca.uhn.fhir.jpa.rp.r5.OperationDefinitionResourceProvider retVal; 2982 retVal = new ca.uhn.fhir.jpa.rp.r5.OperationDefinitionResourceProvider(); 2983 retVal.setContext(myFhirContext); 2984 retVal.setDao(daoOperationDefinitionR5()); 2985 return retVal; 2986 } 2987 2988 @Bean(name="myOperationOutcomeDaoR5") 2989 public 2990 IFhirResourceDao<org.hl7.fhir.r5.model.OperationOutcome> 2991 daoOperationOutcomeR5() { 2992 2993 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.OperationOutcome> retVal; 2994 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.OperationOutcome>(); 2995 retVal.setResourceType(org.hl7.fhir.r5.model.OperationOutcome.class); 2996 retVal.setContext(myFhirContext); 2997 return retVal; 2998 } 2999 3000 @Bean(name="myOperationOutcomeRpR5") 3001 @Lazy 3002 public ca.uhn.fhir.jpa.rp.r5.OperationOutcomeResourceProvider rpOperationOutcomeR5() { 3003 ca.uhn.fhir.jpa.rp.r5.OperationOutcomeResourceProvider retVal; 3004 retVal = new ca.uhn.fhir.jpa.rp.r5.OperationOutcomeResourceProvider(); 3005 retVal.setContext(myFhirContext); 3006 retVal.setDao(daoOperationOutcomeR5()); 3007 return retVal; 3008 } 3009 3010 @Bean(name="myOrganizationDaoR5") 3011 public 3012 IFhirResourceDao<org.hl7.fhir.r5.model.Organization> 3013 daoOrganizationR5() { 3014 3015 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Organization> retVal; 3016 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Organization>(); 3017 retVal.setResourceType(org.hl7.fhir.r5.model.Organization.class); 3018 retVal.setContext(myFhirContext); 3019 return retVal; 3020 } 3021 3022 @Bean(name="myOrganizationRpR5") 3023 @Lazy 3024 public ca.uhn.fhir.jpa.rp.r5.OrganizationResourceProvider rpOrganizationR5() { 3025 ca.uhn.fhir.jpa.rp.r5.OrganizationResourceProvider retVal; 3026 retVal = new ca.uhn.fhir.jpa.rp.r5.OrganizationResourceProvider(); 3027 retVal.setContext(myFhirContext); 3028 retVal.setDao(daoOrganizationR5()); 3029 return retVal; 3030 } 3031 3032 @Bean(name="myOrganizationAffiliationDaoR5") 3033 public 3034 IFhirResourceDao<org.hl7.fhir.r5.model.OrganizationAffiliation> 3035 daoOrganizationAffiliationR5() { 3036 3037 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.OrganizationAffiliation> retVal; 3038 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.OrganizationAffiliation>(); 3039 retVal.setResourceType(org.hl7.fhir.r5.model.OrganizationAffiliation.class); 3040 retVal.setContext(myFhirContext); 3041 return retVal; 3042 } 3043 3044 @Bean(name="myOrganizationAffiliationRpR5") 3045 @Lazy 3046 public ca.uhn.fhir.jpa.rp.r5.OrganizationAffiliationResourceProvider rpOrganizationAffiliationR5() { 3047 ca.uhn.fhir.jpa.rp.r5.OrganizationAffiliationResourceProvider retVal; 3048 retVal = new ca.uhn.fhir.jpa.rp.r5.OrganizationAffiliationResourceProvider(); 3049 retVal.setContext(myFhirContext); 3050 retVal.setDao(daoOrganizationAffiliationR5()); 3051 return retVal; 3052 } 3053 3054 @Bean(name="myPackagedProductDefinitionDaoR5") 3055 public 3056 IFhirResourceDao<org.hl7.fhir.r5.model.PackagedProductDefinition> 3057 daoPackagedProductDefinitionR5() { 3058 3059 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.PackagedProductDefinition> retVal; 3060 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.PackagedProductDefinition>(); 3061 retVal.setResourceType(org.hl7.fhir.r5.model.PackagedProductDefinition.class); 3062 retVal.setContext(myFhirContext); 3063 return retVal; 3064 } 3065 3066 @Bean(name="myPackagedProductDefinitionRpR5") 3067 @Lazy 3068 public ca.uhn.fhir.jpa.rp.r5.PackagedProductDefinitionResourceProvider rpPackagedProductDefinitionR5() { 3069 ca.uhn.fhir.jpa.rp.r5.PackagedProductDefinitionResourceProvider retVal; 3070 retVal = new ca.uhn.fhir.jpa.rp.r5.PackagedProductDefinitionResourceProvider(); 3071 retVal.setContext(myFhirContext); 3072 retVal.setDao(daoPackagedProductDefinitionR5()); 3073 return retVal; 3074 } 3075 3076 @Bean(name="myParametersDaoR5") 3077 public 3078 IFhirResourceDao<org.hl7.fhir.r5.model.Parameters> 3079 daoParametersR5() { 3080 3081 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Parameters> retVal; 3082 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Parameters>(); 3083 retVal.setResourceType(org.hl7.fhir.r5.model.Parameters.class); 3084 retVal.setContext(myFhirContext); 3085 return retVal; 3086 } 3087 3088 @Bean(name="myParametersRpR5") 3089 @Lazy 3090 public ca.uhn.fhir.jpa.rp.r5.ParametersResourceProvider rpParametersR5() { 3091 ca.uhn.fhir.jpa.rp.r5.ParametersResourceProvider retVal; 3092 retVal = new ca.uhn.fhir.jpa.rp.r5.ParametersResourceProvider(); 3093 retVal.setContext(myFhirContext); 3094 retVal.setDao(daoParametersR5()); 3095 return retVal; 3096 } 3097 3098 @Bean(name="myPatientDaoR5") 3099 public 3100 IFhirResourceDaoPatient<org.hl7.fhir.r5.model.Patient> 3101 daoPatientR5() { 3102 3103 ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<org.hl7.fhir.r5.model.Patient> retVal; 3104 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoPatient<>(); 3105 retVal.setResourceType(org.hl7.fhir.r5.model.Patient.class); 3106 retVal.setContext(myFhirContext); 3107 return retVal; 3108 } 3109 3110 @Bean(name="myPatientRpR5") 3111 @Lazy 3112 public ca.uhn.fhir.jpa.rp.r5.PatientResourceProvider rpPatientR5() { 3113 ca.uhn.fhir.jpa.rp.r5.PatientResourceProvider retVal; 3114 retVal = new ca.uhn.fhir.jpa.rp.r5.PatientResourceProvider(); 3115 retVal.setContext(myFhirContext); 3116 retVal.setDao(daoPatientR5()); 3117 return retVal; 3118 } 3119 3120 @Bean(name="myPaymentNoticeDaoR5") 3121 public 3122 IFhirResourceDao<org.hl7.fhir.r5.model.PaymentNotice> 3123 daoPaymentNoticeR5() { 3124 3125 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.PaymentNotice> retVal; 3126 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.PaymentNotice>(); 3127 retVal.setResourceType(org.hl7.fhir.r5.model.PaymentNotice.class); 3128 retVal.setContext(myFhirContext); 3129 return retVal; 3130 } 3131 3132 @Bean(name="myPaymentNoticeRpR5") 3133 @Lazy 3134 public ca.uhn.fhir.jpa.rp.r5.PaymentNoticeResourceProvider rpPaymentNoticeR5() { 3135 ca.uhn.fhir.jpa.rp.r5.PaymentNoticeResourceProvider retVal; 3136 retVal = new ca.uhn.fhir.jpa.rp.r5.PaymentNoticeResourceProvider(); 3137 retVal.setContext(myFhirContext); 3138 retVal.setDao(daoPaymentNoticeR5()); 3139 return retVal; 3140 } 3141 3142 @Bean(name="myPaymentReconciliationDaoR5") 3143 public 3144 IFhirResourceDao<org.hl7.fhir.r5.model.PaymentReconciliation> 3145 daoPaymentReconciliationR5() { 3146 3147 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.PaymentReconciliation> retVal; 3148 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.PaymentReconciliation>(); 3149 retVal.setResourceType(org.hl7.fhir.r5.model.PaymentReconciliation.class); 3150 retVal.setContext(myFhirContext); 3151 return retVal; 3152 } 3153 3154 @Bean(name="myPaymentReconciliationRpR5") 3155 @Lazy 3156 public ca.uhn.fhir.jpa.rp.r5.PaymentReconciliationResourceProvider rpPaymentReconciliationR5() { 3157 ca.uhn.fhir.jpa.rp.r5.PaymentReconciliationResourceProvider retVal; 3158 retVal = new ca.uhn.fhir.jpa.rp.r5.PaymentReconciliationResourceProvider(); 3159 retVal.setContext(myFhirContext); 3160 retVal.setDao(daoPaymentReconciliationR5()); 3161 return retVal; 3162 } 3163 3164 @Bean(name="myPermissionDaoR5") 3165 public 3166 IFhirResourceDao<org.hl7.fhir.r5.model.Permission> 3167 daoPermissionR5() { 3168 3169 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Permission> retVal; 3170 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Permission>(); 3171 retVal.setResourceType(org.hl7.fhir.r5.model.Permission.class); 3172 retVal.setContext(myFhirContext); 3173 return retVal; 3174 } 3175 3176 @Bean(name="myPermissionRpR5") 3177 @Lazy 3178 public ca.uhn.fhir.jpa.rp.r5.PermissionResourceProvider rpPermissionR5() { 3179 ca.uhn.fhir.jpa.rp.r5.PermissionResourceProvider retVal; 3180 retVal = new ca.uhn.fhir.jpa.rp.r5.PermissionResourceProvider(); 3181 retVal.setContext(myFhirContext); 3182 retVal.setDao(daoPermissionR5()); 3183 return retVal; 3184 } 3185 3186 @Bean(name="myPersonDaoR5") 3187 public 3188 IFhirResourceDao<org.hl7.fhir.r5.model.Person> 3189 daoPersonR5() { 3190 3191 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Person> retVal; 3192 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Person>(); 3193 retVal.setResourceType(org.hl7.fhir.r5.model.Person.class); 3194 retVal.setContext(myFhirContext); 3195 return retVal; 3196 } 3197 3198 @Bean(name="myPersonRpR5") 3199 @Lazy 3200 public ca.uhn.fhir.jpa.rp.r5.PersonResourceProvider rpPersonR5() { 3201 ca.uhn.fhir.jpa.rp.r5.PersonResourceProvider retVal; 3202 retVal = new ca.uhn.fhir.jpa.rp.r5.PersonResourceProvider(); 3203 retVal.setContext(myFhirContext); 3204 retVal.setDao(daoPersonR5()); 3205 return retVal; 3206 } 3207 3208 @Bean(name="myPlanDefinitionDaoR5") 3209 public 3210 IFhirResourceDao<org.hl7.fhir.r5.model.PlanDefinition> 3211 daoPlanDefinitionR5() { 3212 3213 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.PlanDefinition> retVal; 3214 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.PlanDefinition>(); 3215 retVal.setResourceType(org.hl7.fhir.r5.model.PlanDefinition.class); 3216 retVal.setContext(myFhirContext); 3217 return retVal; 3218 } 3219 3220 @Bean(name="myPlanDefinitionRpR5") 3221 @Lazy 3222 public ca.uhn.fhir.jpa.rp.r5.PlanDefinitionResourceProvider rpPlanDefinitionR5() { 3223 ca.uhn.fhir.jpa.rp.r5.PlanDefinitionResourceProvider retVal; 3224 retVal = new ca.uhn.fhir.jpa.rp.r5.PlanDefinitionResourceProvider(); 3225 retVal.setContext(myFhirContext); 3226 retVal.setDao(daoPlanDefinitionR5()); 3227 return retVal; 3228 } 3229 3230 @Bean(name="myPractitionerDaoR5") 3231 public 3232 IFhirResourceDao<org.hl7.fhir.r5.model.Practitioner> 3233 daoPractitionerR5() { 3234 3235 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Practitioner> retVal; 3236 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Practitioner>(); 3237 retVal.setResourceType(org.hl7.fhir.r5.model.Practitioner.class); 3238 retVal.setContext(myFhirContext); 3239 return retVal; 3240 } 3241 3242 @Bean(name="myPractitionerRpR5") 3243 @Lazy 3244 public ca.uhn.fhir.jpa.rp.r5.PractitionerResourceProvider rpPractitionerR5() { 3245 ca.uhn.fhir.jpa.rp.r5.PractitionerResourceProvider retVal; 3246 retVal = new ca.uhn.fhir.jpa.rp.r5.PractitionerResourceProvider(); 3247 retVal.setContext(myFhirContext); 3248 retVal.setDao(daoPractitionerR5()); 3249 return retVal; 3250 } 3251 3252 @Bean(name="myPractitionerRoleDaoR5") 3253 public 3254 IFhirResourceDao<org.hl7.fhir.r5.model.PractitionerRole> 3255 daoPractitionerRoleR5() { 3256 3257 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.PractitionerRole> retVal; 3258 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.PractitionerRole>(); 3259 retVal.setResourceType(org.hl7.fhir.r5.model.PractitionerRole.class); 3260 retVal.setContext(myFhirContext); 3261 return retVal; 3262 } 3263 3264 @Bean(name="myPractitionerRoleRpR5") 3265 @Lazy 3266 public ca.uhn.fhir.jpa.rp.r5.PractitionerRoleResourceProvider rpPractitionerRoleR5() { 3267 ca.uhn.fhir.jpa.rp.r5.PractitionerRoleResourceProvider retVal; 3268 retVal = new ca.uhn.fhir.jpa.rp.r5.PractitionerRoleResourceProvider(); 3269 retVal.setContext(myFhirContext); 3270 retVal.setDao(daoPractitionerRoleR5()); 3271 return retVal; 3272 } 3273 3274 @Bean(name="myProcedureDaoR5") 3275 public 3276 IFhirResourceDao<org.hl7.fhir.r5.model.Procedure> 3277 daoProcedureR5() { 3278 3279 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Procedure> retVal; 3280 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Procedure>(); 3281 retVal.setResourceType(org.hl7.fhir.r5.model.Procedure.class); 3282 retVal.setContext(myFhirContext); 3283 return retVal; 3284 } 3285 3286 @Bean(name="myProcedureRpR5") 3287 @Lazy 3288 public ca.uhn.fhir.jpa.rp.r5.ProcedureResourceProvider rpProcedureR5() { 3289 ca.uhn.fhir.jpa.rp.r5.ProcedureResourceProvider retVal; 3290 retVal = new ca.uhn.fhir.jpa.rp.r5.ProcedureResourceProvider(); 3291 retVal.setContext(myFhirContext); 3292 retVal.setDao(daoProcedureR5()); 3293 return retVal; 3294 } 3295 3296 @Bean(name="myProvenanceDaoR5") 3297 public 3298 IFhirResourceDao<org.hl7.fhir.r5.model.Provenance> 3299 daoProvenanceR5() { 3300 3301 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Provenance> retVal; 3302 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Provenance>(); 3303 retVal.setResourceType(org.hl7.fhir.r5.model.Provenance.class); 3304 retVal.setContext(myFhirContext); 3305 return retVal; 3306 } 3307 3308 @Bean(name="myProvenanceRpR5") 3309 @Lazy 3310 public ca.uhn.fhir.jpa.rp.r5.ProvenanceResourceProvider rpProvenanceR5() { 3311 ca.uhn.fhir.jpa.rp.r5.ProvenanceResourceProvider retVal; 3312 retVal = new ca.uhn.fhir.jpa.rp.r5.ProvenanceResourceProvider(); 3313 retVal.setContext(myFhirContext); 3314 retVal.setDao(daoProvenanceR5()); 3315 return retVal; 3316 } 3317 3318 @Bean(name="myQuestionnaireDaoR5") 3319 public 3320 IFhirResourceDao<org.hl7.fhir.r5.model.Questionnaire> 3321 daoQuestionnaireR5() { 3322 3323 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Questionnaire> retVal; 3324 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Questionnaire>(); 3325 retVal.setResourceType(org.hl7.fhir.r5.model.Questionnaire.class); 3326 retVal.setContext(myFhirContext); 3327 return retVal; 3328 } 3329 3330 @Bean(name="myQuestionnaireRpR5") 3331 @Lazy 3332 public ca.uhn.fhir.jpa.rp.r5.QuestionnaireResourceProvider rpQuestionnaireR5() { 3333 ca.uhn.fhir.jpa.rp.r5.QuestionnaireResourceProvider retVal; 3334 retVal = new ca.uhn.fhir.jpa.rp.r5.QuestionnaireResourceProvider(); 3335 retVal.setContext(myFhirContext); 3336 retVal.setDao(daoQuestionnaireR5()); 3337 return retVal; 3338 } 3339 3340 @Bean(name="myQuestionnaireResponseDaoR5") 3341 public 3342 IFhirResourceDao<org.hl7.fhir.r5.model.QuestionnaireResponse> 3343 daoQuestionnaireResponseR5() { 3344 3345 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.QuestionnaireResponse> retVal; 3346 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.QuestionnaireResponse>(); 3347 retVal.setResourceType(org.hl7.fhir.r5.model.QuestionnaireResponse.class); 3348 retVal.setContext(myFhirContext); 3349 return retVal; 3350 } 3351 3352 @Bean(name="myQuestionnaireResponseRpR5") 3353 @Lazy 3354 public ca.uhn.fhir.jpa.rp.r5.QuestionnaireResponseResourceProvider rpQuestionnaireResponseR5() { 3355 ca.uhn.fhir.jpa.rp.r5.QuestionnaireResponseResourceProvider retVal; 3356 retVal = new ca.uhn.fhir.jpa.rp.r5.QuestionnaireResponseResourceProvider(); 3357 retVal.setContext(myFhirContext); 3358 retVal.setDao(daoQuestionnaireResponseR5()); 3359 return retVal; 3360 } 3361 3362 @Bean(name="myRegulatedAuthorizationDaoR5") 3363 public 3364 IFhirResourceDao<org.hl7.fhir.r5.model.RegulatedAuthorization> 3365 daoRegulatedAuthorizationR5() { 3366 3367 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.RegulatedAuthorization> retVal; 3368 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.RegulatedAuthorization>(); 3369 retVal.setResourceType(org.hl7.fhir.r5.model.RegulatedAuthorization.class); 3370 retVal.setContext(myFhirContext); 3371 return retVal; 3372 } 3373 3374 @Bean(name="myRegulatedAuthorizationRpR5") 3375 @Lazy 3376 public ca.uhn.fhir.jpa.rp.r5.RegulatedAuthorizationResourceProvider rpRegulatedAuthorizationR5() { 3377 ca.uhn.fhir.jpa.rp.r5.RegulatedAuthorizationResourceProvider retVal; 3378 retVal = new ca.uhn.fhir.jpa.rp.r5.RegulatedAuthorizationResourceProvider(); 3379 retVal.setContext(myFhirContext); 3380 retVal.setDao(daoRegulatedAuthorizationR5()); 3381 return retVal; 3382 } 3383 3384 @Bean(name="myRelatedPersonDaoR5") 3385 public 3386 IFhirResourceDao<org.hl7.fhir.r5.model.RelatedPerson> 3387 daoRelatedPersonR5() { 3388 3389 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.RelatedPerson> retVal; 3390 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.RelatedPerson>(); 3391 retVal.setResourceType(org.hl7.fhir.r5.model.RelatedPerson.class); 3392 retVal.setContext(myFhirContext); 3393 return retVal; 3394 } 3395 3396 @Bean(name="myRelatedPersonRpR5") 3397 @Lazy 3398 public ca.uhn.fhir.jpa.rp.r5.RelatedPersonResourceProvider rpRelatedPersonR5() { 3399 ca.uhn.fhir.jpa.rp.r5.RelatedPersonResourceProvider retVal; 3400 retVal = new ca.uhn.fhir.jpa.rp.r5.RelatedPersonResourceProvider(); 3401 retVal.setContext(myFhirContext); 3402 retVal.setDao(daoRelatedPersonR5()); 3403 return retVal; 3404 } 3405 3406 @Bean(name="myRequestOrchestrationDaoR5") 3407 public 3408 IFhirResourceDao<org.hl7.fhir.r5.model.RequestOrchestration> 3409 daoRequestOrchestrationR5() { 3410 3411 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.RequestOrchestration> retVal; 3412 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.RequestOrchestration>(); 3413 retVal.setResourceType(org.hl7.fhir.r5.model.RequestOrchestration.class); 3414 retVal.setContext(myFhirContext); 3415 return retVal; 3416 } 3417 3418 @Bean(name="myRequestOrchestrationRpR5") 3419 @Lazy 3420 public ca.uhn.fhir.jpa.rp.r5.RequestOrchestrationResourceProvider rpRequestOrchestrationR5() { 3421 ca.uhn.fhir.jpa.rp.r5.RequestOrchestrationResourceProvider retVal; 3422 retVal = new ca.uhn.fhir.jpa.rp.r5.RequestOrchestrationResourceProvider(); 3423 retVal.setContext(myFhirContext); 3424 retVal.setDao(daoRequestOrchestrationR5()); 3425 return retVal; 3426 } 3427 3428 @Bean(name="myRequirementsDaoR5") 3429 public 3430 IFhirResourceDao<org.hl7.fhir.r5.model.Requirements> 3431 daoRequirementsR5() { 3432 3433 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Requirements> retVal; 3434 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Requirements>(); 3435 retVal.setResourceType(org.hl7.fhir.r5.model.Requirements.class); 3436 retVal.setContext(myFhirContext); 3437 return retVal; 3438 } 3439 3440 @Bean(name="myRequirementsRpR5") 3441 @Lazy 3442 public ca.uhn.fhir.jpa.rp.r5.RequirementsResourceProvider rpRequirementsR5() { 3443 ca.uhn.fhir.jpa.rp.r5.RequirementsResourceProvider retVal; 3444 retVal = new ca.uhn.fhir.jpa.rp.r5.RequirementsResourceProvider(); 3445 retVal.setContext(myFhirContext); 3446 retVal.setDao(daoRequirementsR5()); 3447 return retVal; 3448 } 3449 3450 @Bean(name="myResearchStudyDaoR5") 3451 public 3452 IFhirResourceDao<org.hl7.fhir.r5.model.ResearchStudy> 3453 daoResearchStudyR5() { 3454 3455 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ResearchStudy> retVal; 3456 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ResearchStudy>(); 3457 retVal.setResourceType(org.hl7.fhir.r5.model.ResearchStudy.class); 3458 retVal.setContext(myFhirContext); 3459 return retVal; 3460 } 3461 3462 @Bean(name="myResearchStudyRpR5") 3463 @Lazy 3464 public ca.uhn.fhir.jpa.rp.r5.ResearchStudyResourceProvider rpResearchStudyR5() { 3465 ca.uhn.fhir.jpa.rp.r5.ResearchStudyResourceProvider retVal; 3466 retVal = new ca.uhn.fhir.jpa.rp.r5.ResearchStudyResourceProvider(); 3467 retVal.setContext(myFhirContext); 3468 retVal.setDao(daoResearchStudyR5()); 3469 return retVal; 3470 } 3471 3472 @Bean(name="myResearchSubjectDaoR5") 3473 public 3474 IFhirResourceDao<org.hl7.fhir.r5.model.ResearchSubject> 3475 daoResearchSubjectR5() { 3476 3477 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ResearchSubject> retVal; 3478 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ResearchSubject>(); 3479 retVal.setResourceType(org.hl7.fhir.r5.model.ResearchSubject.class); 3480 retVal.setContext(myFhirContext); 3481 return retVal; 3482 } 3483 3484 @Bean(name="myResearchSubjectRpR5") 3485 @Lazy 3486 public ca.uhn.fhir.jpa.rp.r5.ResearchSubjectResourceProvider rpResearchSubjectR5() { 3487 ca.uhn.fhir.jpa.rp.r5.ResearchSubjectResourceProvider retVal; 3488 retVal = new ca.uhn.fhir.jpa.rp.r5.ResearchSubjectResourceProvider(); 3489 retVal.setContext(myFhirContext); 3490 retVal.setDao(daoResearchSubjectR5()); 3491 return retVal; 3492 } 3493 3494 @Bean(name="myRiskAssessmentDaoR5") 3495 public 3496 IFhirResourceDao<org.hl7.fhir.r5.model.RiskAssessment> 3497 daoRiskAssessmentR5() { 3498 3499 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.RiskAssessment> retVal; 3500 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.RiskAssessment>(); 3501 retVal.setResourceType(org.hl7.fhir.r5.model.RiskAssessment.class); 3502 retVal.setContext(myFhirContext); 3503 return retVal; 3504 } 3505 3506 @Bean(name="myRiskAssessmentRpR5") 3507 @Lazy 3508 public ca.uhn.fhir.jpa.rp.r5.RiskAssessmentResourceProvider rpRiskAssessmentR5() { 3509 ca.uhn.fhir.jpa.rp.r5.RiskAssessmentResourceProvider retVal; 3510 retVal = new ca.uhn.fhir.jpa.rp.r5.RiskAssessmentResourceProvider(); 3511 retVal.setContext(myFhirContext); 3512 retVal.setDao(daoRiskAssessmentR5()); 3513 return retVal; 3514 } 3515 3516 @Bean(name="myScheduleDaoR5") 3517 public 3518 IFhirResourceDao<org.hl7.fhir.r5.model.Schedule> 3519 daoScheduleR5() { 3520 3521 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Schedule> retVal; 3522 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Schedule>(); 3523 retVal.setResourceType(org.hl7.fhir.r5.model.Schedule.class); 3524 retVal.setContext(myFhirContext); 3525 return retVal; 3526 } 3527 3528 @Bean(name="myScheduleRpR5") 3529 @Lazy 3530 public ca.uhn.fhir.jpa.rp.r5.ScheduleResourceProvider rpScheduleR5() { 3531 ca.uhn.fhir.jpa.rp.r5.ScheduleResourceProvider retVal; 3532 retVal = new ca.uhn.fhir.jpa.rp.r5.ScheduleResourceProvider(); 3533 retVal.setContext(myFhirContext); 3534 retVal.setDao(daoScheduleR5()); 3535 return retVal; 3536 } 3537 3538 @Bean(name="mySearchParameterDaoR5") 3539 public 3540 IFhirResourceDaoSearchParameter<org.hl7.fhir.r5.model.SearchParameter> 3541 daoSearchParameterR5() { 3542 3543 ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<org.hl7.fhir.r5.model.SearchParameter> retVal; 3544 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoSearchParameter<>(); 3545 retVal.setResourceType(org.hl7.fhir.r5.model.SearchParameter.class); 3546 retVal.setContext(myFhirContext); 3547 return retVal; 3548 } 3549 3550 @Bean(name="mySearchParameterRpR5") 3551 @Lazy 3552 public ca.uhn.fhir.jpa.rp.r5.SearchParameterResourceProvider rpSearchParameterR5() { 3553 ca.uhn.fhir.jpa.rp.r5.SearchParameterResourceProvider retVal; 3554 retVal = new ca.uhn.fhir.jpa.rp.r5.SearchParameterResourceProvider(); 3555 retVal.setContext(myFhirContext); 3556 retVal.setDao(daoSearchParameterR5()); 3557 return retVal; 3558 } 3559 3560 @Bean(name="myServiceRequestDaoR5") 3561 public 3562 IFhirResourceDao<org.hl7.fhir.r5.model.ServiceRequest> 3563 daoServiceRequestR5() { 3564 3565 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.ServiceRequest> retVal; 3566 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.ServiceRequest>(); 3567 retVal.setResourceType(org.hl7.fhir.r5.model.ServiceRequest.class); 3568 retVal.setContext(myFhirContext); 3569 return retVal; 3570 } 3571 3572 @Bean(name="myServiceRequestRpR5") 3573 @Lazy 3574 public ca.uhn.fhir.jpa.rp.r5.ServiceRequestResourceProvider rpServiceRequestR5() { 3575 ca.uhn.fhir.jpa.rp.r5.ServiceRequestResourceProvider retVal; 3576 retVal = new ca.uhn.fhir.jpa.rp.r5.ServiceRequestResourceProvider(); 3577 retVal.setContext(myFhirContext); 3578 retVal.setDao(daoServiceRequestR5()); 3579 return retVal; 3580 } 3581 3582 @Bean(name="mySlotDaoR5") 3583 public 3584 IFhirResourceDao<org.hl7.fhir.r5.model.Slot> 3585 daoSlotR5() { 3586 3587 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Slot> retVal; 3588 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Slot>(); 3589 retVal.setResourceType(org.hl7.fhir.r5.model.Slot.class); 3590 retVal.setContext(myFhirContext); 3591 return retVal; 3592 } 3593 3594 @Bean(name="mySlotRpR5") 3595 @Lazy 3596 public ca.uhn.fhir.jpa.rp.r5.SlotResourceProvider rpSlotR5() { 3597 ca.uhn.fhir.jpa.rp.r5.SlotResourceProvider retVal; 3598 retVal = new ca.uhn.fhir.jpa.rp.r5.SlotResourceProvider(); 3599 retVal.setContext(myFhirContext); 3600 retVal.setDao(daoSlotR5()); 3601 return retVal; 3602 } 3603 3604 @Bean(name="mySpecimenDaoR5") 3605 public 3606 IFhirResourceDao<org.hl7.fhir.r5.model.Specimen> 3607 daoSpecimenR5() { 3608 3609 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Specimen> retVal; 3610 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Specimen>(); 3611 retVal.setResourceType(org.hl7.fhir.r5.model.Specimen.class); 3612 retVal.setContext(myFhirContext); 3613 return retVal; 3614 } 3615 3616 @Bean(name="mySpecimenRpR5") 3617 @Lazy 3618 public ca.uhn.fhir.jpa.rp.r5.SpecimenResourceProvider rpSpecimenR5() { 3619 ca.uhn.fhir.jpa.rp.r5.SpecimenResourceProvider retVal; 3620 retVal = new ca.uhn.fhir.jpa.rp.r5.SpecimenResourceProvider(); 3621 retVal.setContext(myFhirContext); 3622 retVal.setDao(daoSpecimenR5()); 3623 return retVal; 3624 } 3625 3626 @Bean(name="mySpecimenDefinitionDaoR5") 3627 public 3628 IFhirResourceDao<org.hl7.fhir.r5.model.SpecimenDefinition> 3629 daoSpecimenDefinitionR5() { 3630 3631 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SpecimenDefinition> retVal; 3632 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SpecimenDefinition>(); 3633 retVal.setResourceType(org.hl7.fhir.r5.model.SpecimenDefinition.class); 3634 retVal.setContext(myFhirContext); 3635 return retVal; 3636 } 3637 3638 @Bean(name="mySpecimenDefinitionRpR5") 3639 @Lazy 3640 public ca.uhn.fhir.jpa.rp.r5.SpecimenDefinitionResourceProvider rpSpecimenDefinitionR5() { 3641 ca.uhn.fhir.jpa.rp.r5.SpecimenDefinitionResourceProvider retVal; 3642 retVal = new ca.uhn.fhir.jpa.rp.r5.SpecimenDefinitionResourceProvider(); 3643 retVal.setContext(myFhirContext); 3644 retVal.setDao(daoSpecimenDefinitionR5()); 3645 return retVal; 3646 } 3647 3648 @Bean(name="myStructureDefinitionDaoR5") 3649 public 3650 IFhirResourceDaoStructureDefinition<org.hl7.fhir.r5.model.StructureDefinition> 3651 daoStructureDefinitionR5() { 3652 3653 ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<org.hl7.fhir.r5.model.StructureDefinition> retVal; 3654 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoStructureDefinition<>(); 3655 retVal.setResourceType(org.hl7.fhir.r5.model.StructureDefinition.class); 3656 retVal.setContext(myFhirContext); 3657 return retVal; 3658 } 3659 3660 @Bean(name="myStructureDefinitionRpR5") 3661 @Lazy 3662 public ca.uhn.fhir.jpa.rp.r5.StructureDefinitionResourceProvider rpStructureDefinitionR5() { 3663 ca.uhn.fhir.jpa.rp.r5.StructureDefinitionResourceProvider retVal; 3664 retVal = new ca.uhn.fhir.jpa.rp.r5.StructureDefinitionResourceProvider(); 3665 retVal.setContext(myFhirContext); 3666 retVal.setDao(daoStructureDefinitionR5()); 3667 return retVal; 3668 } 3669 3670 @Bean(name="myStructureMapDaoR5") 3671 public 3672 IFhirResourceDao<org.hl7.fhir.r5.model.StructureMap> 3673 daoStructureMapR5() { 3674 3675 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.StructureMap> retVal; 3676 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.StructureMap>(); 3677 retVal.setResourceType(org.hl7.fhir.r5.model.StructureMap.class); 3678 retVal.setContext(myFhirContext); 3679 return retVal; 3680 } 3681 3682 @Bean(name="myStructureMapRpR5") 3683 @Lazy 3684 public ca.uhn.fhir.jpa.rp.r5.StructureMapResourceProvider rpStructureMapR5() { 3685 ca.uhn.fhir.jpa.rp.r5.StructureMapResourceProvider retVal; 3686 retVal = new ca.uhn.fhir.jpa.rp.r5.StructureMapResourceProvider(); 3687 retVal.setContext(myFhirContext); 3688 retVal.setDao(daoStructureMapR5()); 3689 return retVal; 3690 } 3691 3692 @Bean(name="mySubscriptionDaoR5") 3693 public 3694 IFhirResourceDaoSubscription<org.hl7.fhir.r5.model.Subscription> 3695 daoSubscriptionR5() { 3696 3697 ca.uhn.fhir.jpa.dao.r5.FhirResourceDaoSubscriptionR5 retVal; 3698 retVal = new ca.uhn.fhir.jpa.dao.r5.FhirResourceDaoSubscriptionR5(); 3699 retVal.setResourceType(org.hl7.fhir.r5.model.Subscription.class); 3700 retVal.setContext(myFhirContext); 3701 return retVal; 3702 } 3703 3704 @Bean(name="mySubscriptionRpR5") 3705 @Lazy 3706 public ca.uhn.fhir.jpa.rp.r5.SubscriptionResourceProvider rpSubscriptionR5() { 3707 ca.uhn.fhir.jpa.rp.r5.SubscriptionResourceProvider retVal; 3708 retVal = new ca.uhn.fhir.jpa.rp.r5.SubscriptionResourceProvider(); 3709 retVal.setContext(myFhirContext); 3710 retVal.setDao(daoSubscriptionR5()); 3711 return retVal; 3712 } 3713 3714 @Bean(name="mySubscriptionStatusDaoR5") 3715 public 3716 IFhirResourceDao<org.hl7.fhir.r5.model.SubscriptionStatus> 3717 daoSubscriptionStatusR5() { 3718 3719 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SubscriptionStatus> retVal; 3720 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SubscriptionStatus>(); 3721 retVal.setResourceType(org.hl7.fhir.r5.model.SubscriptionStatus.class); 3722 retVal.setContext(myFhirContext); 3723 return retVal; 3724 } 3725 3726 @Bean(name="mySubscriptionStatusRpR5") 3727 @Lazy 3728 public ca.uhn.fhir.jpa.rp.r5.SubscriptionStatusResourceProvider rpSubscriptionStatusR5() { 3729 ca.uhn.fhir.jpa.rp.r5.SubscriptionStatusResourceProvider retVal; 3730 retVal = new ca.uhn.fhir.jpa.rp.r5.SubscriptionStatusResourceProvider(); 3731 retVal.setContext(myFhirContext); 3732 retVal.setDao(daoSubscriptionStatusR5()); 3733 return retVal; 3734 } 3735 3736 @Bean(name="mySubscriptionTopicDaoR5") 3737 public 3738 IFhirResourceDao<org.hl7.fhir.r5.model.SubscriptionTopic> 3739 daoSubscriptionTopicR5() { 3740 3741 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SubscriptionTopic> retVal; 3742 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SubscriptionTopic>(); 3743 retVal.setResourceType(org.hl7.fhir.r5.model.SubscriptionTopic.class); 3744 retVal.setContext(myFhirContext); 3745 return retVal; 3746 } 3747 3748 @Bean(name="mySubscriptionTopicRpR5") 3749 @Lazy 3750 public ca.uhn.fhir.jpa.rp.r5.SubscriptionTopicResourceProvider rpSubscriptionTopicR5() { 3751 ca.uhn.fhir.jpa.rp.r5.SubscriptionTopicResourceProvider retVal; 3752 retVal = new ca.uhn.fhir.jpa.rp.r5.SubscriptionTopicResourceProvider(); 3753 retVal.setContext(myFhirContext); 3754 retVal.setDao(daoSubscriptionTopicR5()); 3755 return retVal; 3756 } 3757 3758 @Bean(name="mySubstanceDaoR5") 3759 public 3760 IFhirResourceDao<org.hl7.fhir.r5.model.Substance> 3761 daoSubstanceR5() { 3762 3763 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Substance> retVal; 3764 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Substance>(); 3765 retVal.setResourceType(org.hl7.fhir.r5.model.Substance.class); 3766 retVal.setContext(myFhirContext); 3767 return retVal; 3768 } 3769 3770 @Bean(name="mySubstanceRpR5") 3771 @Lazy 3772 public ca.uhn.fhir.jpa.rp.r5.SubstanceResourceProvider rpSubstanceR5() { 3773 ca.uhn.fhir.jpa.rp.r5.SubstanceResourceProvider retVal; 3774 retVal = new ca.uhn.fhir.jpa.rp.r5.SubstanceResourceProvider(); 3775 retVal.setContext(myFhirContext); 3776 retVal.setDao(daoSubstanceR5()); 3777 return retVal; 3778 } 3779 3780 @Bean(name="mySubstanceDefinitionDaoR5") 3781 public 3782 IFhirResourceDao<org.hl7.fhir.r5.model.SubstanceDefinition> 3783 daoSubstanceDefinitionR5() { 3784 3785 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SubstanceDefinition> retVal; 3786 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SubstanceDefinition>(); 3787 retVal.setResourceType(org.hl7.fhir.r5.model.SubstanceDefinition.class); 3788 retVal.setContext(myFhirContext); 3789 return retVal; 3790 } 3791 3792 @Bean(name="mySubstanceDefinitionRpR5") 3793 @Lazy 3794 public ca.uhn.fhir.jpa.rp.r5.SubstanceDefinitionResourceProvider rpSubstanceDefinitionR5() { 3795 ca.uhn.fhir.jpa.rp.r5.SubstanceDefinitionResourceProvider retVal; 3796 retVal = new ca.uhn.fhir.jpa.rp.r5.SubstanceDefinitionResourceProvider(); 3797 retVal.setContext(myFhirContext); 3798 retVal.setDao(daoSubstanceDefinitionR5()); 3799 return retVal; 3800 } 3801 3802 @Bean(name="mySubstanceNucleicAcidDaoR5") 3803 public 3804 IFhirResourceDao<org.hl7.fhir.r5.model.SubstanceNucleicAcid> 3805 daoSubstanceNucleicAcidR5() { 3806 3807 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SubstanceNucleicAcid> retVal; 3808 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SubstanceNucleicAcid>(); 3809 retVal.setResourceType(org.hl7.fhir.r5.model.SubstanceNucleicAcid.class); 3810 retVal.setContext(myFhirContext); 3811 return retVal; 3812 } 3813 3814 @Bean(name="mySubstanceNucleicAcidRpR5") 3815 @Lazy 3816 public ca.uhn.fhir.jpa.rp.r5.SubstanceNucleicAcidResourceProvider rpSubstanceNucleicAcidR5() { 3817 ca.uhn.fhir.jpa.rp.r5.SubstanceNucleicAcidResourceProvider retVal; 3818 retVal = new ca.uhn.fhir.jpa.rp.r5.SubstanceNucleicAcidResourceProvider(); 3819 retVal.setContext(myFhirContext); 3820 retVal.setDao(daoSubstanceNucleicAcidR5()); 3821 return retVal; 3822 } 3823 3824 @Bean(name="mySubstancePolymerDaoR5") 3825 public 3826 IFhirResourceDao<org.hl7.fhir.r5.model.SubstancePolymer> 3827 daoSubstancePolymerR5() { 3828 3829 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SubstancePolymer> retVal; 3830 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SubstancePolymer>(); 3831 retVal.setResourceType(org.hl7.fhir.r5.model.SubstancePolymer.class); 3832 retVal.setContext(myFhirContext); 3833 return retVal; 3834 } 3835 3836 @Bean(name="mySubstancePolymerRpR5") 3837 @Lazy 3838 public ca.uhn.fhir.jpa.rp.r5.SubstancePolymerResourceProvider rpSubstancePolymerR5() { 3839 ca.uhn.fhir.jpa.rp.r5.SubstancePolymerResourceProvider retVal; 3840 retVal = new ca.uhn.fhir.jpa.rp.r5.SubstancePolymerResourceProvider(); 3841 retVal.setContext(myFhirContext); 3842 retVal.setDao(daoSubstancePolymerR5()); 3843 return retVal; 3844 } 3845 3846 @Bean(name="mySubstanceProteinDaoR5") 3847 public 3848 IFhirResourceDao<org.hl7.fhir.r5.model.SubstanceProtein> 3849 daoSubstanceProteinR5() { 3850 3851 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SubstanceProtein> retVal; 3852 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SubstanceProtein>(); 3853 retVal.setResourceType(org.hl7.fhir.r5.model.SubstanceProtein.class); 3854 retVal.setContext(myFhirContext); 3855 return retVal; 3856 } 3857 3858 @Bean(name="mySubstanceProteinRpR5") 3859 @Lazy 3860 public ca.uhn.fhir.jpa.rp.r5.SubstanceProteinResourceProvider rpSubstanceProteinR5() { 3861 ca.uhn.fhir.jpa.rp.r5.SubstanceProteinResourceProvider retVal; 3862 retVal = new ca.uhn.fhir.jpa.rp.r5.SubstanceProteinResourceProvider(); 3863 retVal.setContext(myFhirContext); 3864 retVal.setDao(daoSubstanceProteinR5()); 3865 return retVal; 3866 } 3867 3868 @Bean(name="mySubstanceReferenceInformationDaoR5") 3869 public 3870 IFhirResourceDao<org.hl7.fhir.r5.model.SubstanceReferenceInformation> 3871 daoSubstanceReferenceInformationR5() { 3872 3873 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SubstanceReferenceInformation> retVal; 3874 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SubstanceReferenceInformation>(); 3875 retVal.setResourceType(org.hl7.fhir.r5.model.SubstanceReferenceInformation.class); 3876 retVal.setContext(myFhirContext); 3877 return retVal; 3878 } 3879 3880 @Bean(name="mySubstanceReferenceInformationRpR5") 3881 @Lazy 3882 public ca.uhn.fhir.jpa.rp.r5.SubstanceReferenceInformationResourceProvider rpSubstanceReferenceInformationR5() { 3883 ca.uhn.fhir.jpa.rp.r5.SubstanceReferenceInformationResourceProvider retVal; 3884 retVal = new ca.uhn.fhir.jpa.rp.r5.SubstanceReferenceInformationResourceProvider(); 3885 retVal.setContext(myFhirContext); 3886 retVal.setDao(daoSubstanceReferenceInformationR5()); 3887 return retVal; 3888 } 3889 3890 @Bean(name="mySubstanceSourceMaterialDaoR5") 3891 public 3892 IFhirResourceDao<org.hl7.fhir.r5.model.SubstanceSourceMaterial> 3893 daoSubstanceSourceMaterialR5() { 3894 3895 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SubstanceSourceMaterial> retVal; 3896 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SubstanceSourceMaterial>(); 3897 retVal.setResourceType(org.hl7.fhir.r5.model.SubstanceSourceMaterial.class); 3898 retVal.setContext(myFhirContext); 3899 return retVal; 3900 } 3901 3902 @Bean(name="mySubstanceSourceMaterialRpR5") 3903 @Lazy 3904 public ca.uhn.fhir.jpa.rp.r5.SubstanceSourceMaterialResourceProvider rpSubstanceSourceMaterialR5() { 3905 ca.uhn.fhir.jpa.rp.r5.SubstanceSourceMaterialResourceProvider retVal; 3906 retVal = new ca.uhn.fhir.jpa.rp.r5.SubstanceSourceMaterialResourceProvider(); 3907 retVal.setContext(myFhirContext); 3908 retVal.setDao(daoSubstanceSourceMaterialR5()); 3909 return retVal; 3910 } 3911 3912 @Bean(name="mySupplyDeliveryDaoR5") 3913 public 3914 IFhirResourceDao<org.hl7.fhir.r5.model.SupplyDelivery> 3915 daoSupplyDeliveryR5() { 3916 3917 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SupplyDelivery> retVal; 3918 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SupplyDelivery>(); 3919 retVal.setResourceType(org.hl7.fhir.r5.model.SupplyDelivery.class); 3920 retVal.setContext(myFhirContext); 3921 return retVal; 3922 } 3923 3924 @Bean(name="mySupplyDeliveryRpR5") 3925 @Lazy 3926 public ca.uhn.fhir.jpa.rp.r5.SupplyDeliveryResourceProvider rpSupplyDeliveryR5() { 3927 ca.uhn.fhir.jpa.rp.r5.SupplyDeliveryResourceProvider retVal; 3928 retVal = new ca.uhn.fhir.jpa.rp.r5.SupplyDeliveryResourceProvider(); 3929 retVal.setContext(myFhirContext); 3930 retVal.setDao(daoSupplyDeliveryR5()); 3931 return retVal; 3932 } 3933 3934 @Bean(name="mySupplyRequestDaoR5") 3935 public 3936 IFhirResourceDao<org.hl7.fhir.r5.model.SupplyRequest> 3937 daoSupplyRequestR5() { 3938 3939 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.SupplyRequest> retVal; 3940 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.SupplyRequest>(); 3941 retVal.setResourceType(org.hl7.fhir.r5.model.SupplyRequest.class); 3942 retVal.setContext(myFhirContext); 3943 return retVal; 3944 } 3945 3946 @Bean(name="mySupplyRequestRpR5") 3947 @Lazy 3948 public ca.uhn.fhir.jpa.rp.r5.SupplyRequestResourceProvider rpSupplyRequestR5() { 3949 ca.uhn.fhir.jpa.rp.r5.SupplyRequestResourceProvider retVal; 3950 retVal = new ca.uhn.fhir.jpa.rp.r5.SupplyRequestResourceProvider(); 3951 retVal.setContext(myFhirContext); 3952 retVal.setDao(daoSupplyRequestR5()); 3953 return retVal; 3954 } 3955 3956 @Bean(name="myTaskDaoR5") 3957 public 3958 IFhirResourceDao<org.hl7.fhir.r5.model.Task> 3959 daoTaskR5() { 3960 3961 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Task> retVal; 3962 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Task>(); 3963 retVal.setResourceType(org.hl7.fhir.r5.model.Task.class); 3964 retVal.setContext(myFhirContext); 3965 return retVal; 3966 } 3967 3968 @Bean(name="myTaskRpR5") 3969 @Lazy 3970 public ca.uhn.fhir.jpa.rp.r5.TaskResourceProvider rpTaskR5() { 3971 ca.uhn.fhir.jpa.rp.r5.TaskResourceProvider retVal; 3972 retVal = new ca.uhn.fhir.jpa.rp.r5.TaskResourceProvider(); 3973 retVal.setContext(myFhirContext); 3974 retVal.setDao(daoTaskR5()); 3975 return retVal; 3976 } 3977 3978 @Bean(name="myTerminologyCapabilitiesDaoR5") 3979 public 3980 IFhirResourceDao<org.hl7.fhir.r5.model.TerminologyCapabilities> 3981 daoTerminologyCapabilitiesR5() { 3982 3983 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.TerminologyCapabilities> retVal; 3984 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.TerminologyCapabilities>(); 3985 retVal.setResourceType(org.hl7.fhir.r5.model.TerminologyCapabilities.class); 3986 retVal.setContext(myFhirContext); 3987 return retVal; 3988 } 3989 3990 @Bean(name="myTerminologyCapabilitiesRpR5") 3991 @Lazy 3992 public ca.uhn.fhir.jpa.rp.r5.TerminologyCapabilitiesResourceProvider rpTerminologyCapabilitiesR5() { 3993 ca.uhn.fhir.jpa.rp.r5.TerminologyCapabilitiesResourceProvider retVal; 3994 retVal = new ca.uhn.fhir.jpa.rp.r5.TerminologyCapabilitiesResourceProvider(); 3995 retVal.setContext(myFhirContext); 3996 retVal.setDao(daoTerminologyCapabilitiesR5()); 3997 return retVal; 3998 } 3999 4000 @Bean(name="myTestPlanDaoR5") 4001 public 4002 IFhirResourceDao<org.hl7.fhir.r5.model.TestPlan> 4003 daoTestPlanR5() { 4004 4005 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.TestPlan> retVal; 4006 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.TestPlan>(); 4007 retVal.setResourceType(org.hl7.fhir.r5.model.TestPlan.class); 4008 retVal.setContext(myFhirContext); 4009 return retVal; 4010 } 4011 4012 @Bean(name="myTestPlanRpR5") 4013 @Lazy 4014 public ca.uhn.fhir.jpa.rp.r5.TestPlanResourceProvider rpTestPlanR5() { 4015 ca.uhn.fhir.jpa.rp.r5.TestPlanResourceProvider retVal; 4016 retVal = new ca.uhn.fhir.jpa.rp.r5.TestPlanResourceProvider(); 4017 retVal.setContext(myFhirContext); 4018 retVal.setDao(daoTestPlanR5()); 4019 return retVal; 4020 } 4021 4022 @Bean(name="myTestReportDaoR5") 4023 public 4024 IFhirResourceDao<org.hl7.fhir.r5.model.TestReport> 4025 daoTestReportR5() { 4026 4027 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.TestReport> retVal; 4028 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.TestReport>(); 4029 retVal.setResourceType(org.hl7.fhir.r5.model.TestReport.class); 4030 retVal.setContext(myFhirContext); 4031 return retVal; 4032 } 4033 4034 @Bean(name="myTestReportRpR5") 4035 @Lazy 4036 public ca.uhn.fhir.jpa.rp.r5.TestReportResourceProvider rpTestReportR5() { 4037 ca.uhn.fhir.jpa.rp.r5.TestReportResourceProvider retVal; 4038 retVal = new ca.uhn.fhir.jpa.rp.r5.TestReportResourceProvider(); 4039 retVal.setContext(myFhirContext); 4040 retVal.setDao(daoTestReportR5()); 4041 return retVal; 4042 } 4043 4044 @Bean(name="myTestScriptDaoR5") 4045 public 4046 IFhirResourceDao<org.hl7.fhir.r5.model.TestScript> 4047 daoTestScriptR5() { 4048 4049 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.TestScript> retVal; 4050 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.TestScript>(); 4051 retVal.setResourceType(org.hl7.fhir.r5.model.TestScript.class); 4052 retVal.setContext(myFhirContext); 4053 return retVal; 4054 } 4055 4056 @Bean(name="myTestScriptRpR5") 4057 @Lazy 4058 public ca.uhn.fhir.jpa.rp.r5.TestScriptResourceProvider rpTestScriptR5() { 4059 ca.uhn.fhir.jpa.rp.r5.TestScriptResourceProvider retVal; 4060 retVal = new ca.uhn.fhir.jpa.rp.r5.TestScriptResourceProvider(); 4061 retVal.setContext(myFhirContext); 4062 retVal.setDao(daoTestScriptR5()); 4063 return retVal; 4064 } 4065 4066 @Bean(name="myTransportDaoR5") 4067 public 4068 IFhirResourceDao<org.hl7.fhir.r5.model.Transport> 4069 daoTransportR5() { 4070 4071 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.Transport> retVal; 4072 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.Transport>(); 4073 retVal.setResourceType(org.hl7.fhir.r5.model.Transport.class); 4074 retVal.setContext(myFhirContext); 4075 return retVal; 4076 } 4077 4078 @Bean(name="myTransportRpR5") 4079 @Lazy 4080 public ca.uhn.fhir.jpa.rp.r5.TransportResourceProvider rpTransportR5() { 4081 ca.uhn.fhir.jpa.rp.r5.TransportResourceProvider retVal; 4082 retVal = new ca.uhn.fhir.jpa.rp.r5.TransportResourceProvider(); 4083 retVal.setContext(myFhirContext); 4084 retVal.setDao(daoTransportR5()); 4085 return retVal; 4086 } 4087 4088 @Bean(name="myValueSetDaoR5") 4089 public 4090 IFhirResourceDaoValueSet<org.hl7.fhir.r5.model.ValueSet> 4091 daoValueSetR5() { 4092 4093 ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<org.hl7.fhir.r5.model.ValueSet> retVal; 4094 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDaoValueSet<>(); 4095 retVal.setResourceType(org.hl7.fhir.r5.model.ValueSet.class); 4096 retVal.setContext(myFhirContext); 4097 return retVal; 4098 } 4099 4100 @Bean(name="myValueSetRpR5") 4101 @Lazy 4102 public ca.uhn.fhir.jpa.rp.r5.ValueSetResourceProvider rpValueSetR5() { 4103 ca.uhn.fhir.jpa.rp.r5.ValueSetResourceProvider retVal; 4104 retVal = new ca.uhn.fhir.jpa.rp.r5.ValueSetResourceProvider(); 4105 retVal.setContext(myFhirContext); 4106 retVal.setDao(daoValueSetR5()); 4107 return retVal; 4108 } 4109 4110 @Bean(name="myVerificationResultDaoR5") 4111 public 4112 IFhirResourceDao<org.hl7.fhir.r5.model.VerificationResult> 4113 daoVerificationResultR5() { 4114 4115 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.VerificationResult> retVal; 4116 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.VerificationResult>(); 4117 retVal.setResourceType(org.hl7.fhir.r5.model.VerificationResult.class); 4118 retVal.setContext(myFhirContext); 4119 return retVal; 4120 } 4121 4122 @Bean(name="myVerificationResultRpR5") 4123 @Lazy 4124 public ca.uhn.fhir.jpa.rp.r5.VerificationResultResourceProvider rpVerificationResultR5() { 4125 ca.uhn.fhir.jpa.rp.r5.VerificationResultResourceProvider retVal; 4126 retVal = new ca.uhn.fhir.jpa.rp.r5.VerificationResultResourceProvider(); 4127 retVal.setContext(myFhirContext); 4128 retVal.setDao(daoVerificationResultR5()); 4129 return retVal; 4130 } 4131 4132 @Bean(name="myVisionPrescriptionDaoR5") 4133 public 4134 IFhirResourceDao<org.hl7.fhir.r5.model.VisionPrescription> 4135 daoVisionPrescriptionR5() { 4136 4137 ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao<org.hl7.fhir.r5.model.VisionPrescription> retVal; 4138 retVal = new ca.uhn.fhir.jpa.dao.JpaResourceDao<org.hl7.fhir.r5.model.VisionPrescription>(); 4139 retVal.setResourceType(org.hl7.fhir.r5.model.VisionPrescription.class); 4140 retVal.setContext(myFhirContext); 4141 return retVal; 4142 } 4143 4144 @Bean(name="myVisionPrescriptionRpR5") 4145 @Lazy 4146 public ca.uhn.fhir.jpa.rp.r5.VisionPrescriptionResourceProvider rpVisionPrescriptionR5() { 4147 ca.uhn.fhir.jpa.rp.r5.VisionPrescriptionResourceProvider retVal; 4148 retVal = new ca.uhn.fhir.jpa.rp.r5.VisionPrescriptionResourceProvider(); 4149 retVal.setContext(myFhirContext); 4150 retVal.setDao(daoVisionPrescriptionR5()); 4151 return retVal; 4152 } 4153 4154 4155 4156 4157 4158 4159}