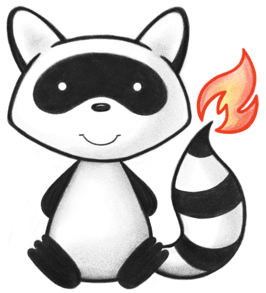
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.config; 021 022import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 023import ca.uhn.fhir.util.ReflectionUtil; 024import com.google.common.annotations.VisibleForTesting; 025import org.apache.commons.lang3.StringUtils; 026import org.apache.commons.lang3.Validate; 027import org.hibernate.dialect.Dialect; 028import org.hibernate.search.engine.cfg.BackendSettings; 029import org.springframework.beans.factory.annotation.Autowired; 030import org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean; 031 032import javax.sql.DataSource; 033 034public class HibernatePropertiesProvider { 035 036 @Autowired 037 private LocalContainerEntityManagerFactoryBean myEntityManagerFactory; 038 039 private Dialect myDialect; 040 private String myHibernateSearchBackend; 041 042 @Autowired 043 private JpaStorageSettings myStorageSettings; 044 045 @VisibleForTesting 046 public void setDialectForUnitTest(Dialect theDialect) { 047 myDialect = theDialect; 048 } 049 050 public Dialect getDialect() { 051 Dialect dialect = myDialect; 052 if (dialect == null) { 053 String dialectClass = 054 (String) myEntityManagerFactory.getJpaPropertyMap().get("hibernate.dialect"); 055 dialect = ReflectionUtil.newInstanceOrReturnNull(dialectClass, Dialect.class); 056 Validate.notNull(dialect, "Unable to create instance of class: %s", dialectClass); 057 myDialect = dialect; 058 } 059 060 return dialect; 061 } 062 063 public String getHibernateSearchBackend() { 064 String hibernateSearchBackend = myHibernateSearchBackend; 065 if (StringUtils.isBlank(hibernateSearchBackend)) { 066 hibernateSearchBackend = (String) 067 myEntityManagerFactory.getJpaPropertyMap().get(BackendSettings.backendKey(BackendSettings.TYPE)); 068 Validate.notNull( 069 hibernateSearchBackend, BackendSettings.backendKey(BackendSettings.TYPE) + " property is unset!"); 070 myHibernateSearchBackend = hibernateSearchBackend; 071 } 072 return myHibernateSearchBackend; 073 } 074 075 public DataSource getDataSource() { 076 return myEntityManagerFactory.getDataSource(); 077 } 078 079 public boolean isOracleDialect() { 080 return getDialect() instanceof org.hibernate.dialect.OracleDialect; 081 } 082}