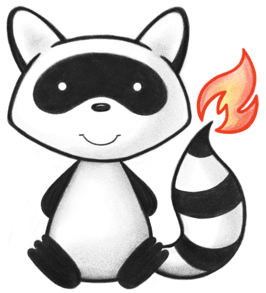
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.config; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.jpa.api.dao.IFhirSystemDao; 024import ca.uhn.fhir.jpa.dao.ITransactionProcessorVersionAdapter; 025import ca.uhn.fhir.jpa.dao.TransactionProcessorVersionAdapterDstu2; 026import ca.uhn.fhir.jpa.provider.JpaSystemProvider; 027import ca.uhn.fhir.jpa.term.TermVersionAdapterSvcDstu2; 028import ca.uhn.fhir.jpa.term.api.ITermVersionAdapterSvc; 029import ca.uhn.fhir.model.dstu2.composite.MetaDt; 030import ca.uhn.fhir.model.dstu2.resource.Bundle; 031import org.springframework.context.annotation.Bean; 032import org.springframework.context.annotation.Configuration; 033import org.springframework.context.annotation.Import; 034import org.springframework.transaction.annotation.EnableTransactionManagement; 035 036@Configuration 037@EnableTransactionManagement 038@Import({FhirContextDstu2Config.class, GeneratedDaoAndResourceProviderConfigDstu2.class, JpaConfig.class}) 039public class JpaDstu2Config { 040 @Bean 041 public ITransactionProcessorVersionAdapter transactionProcessorVersionFacade() { 042 return new TransactionProcessorVersionAdapterDstu2(); 043 } 044 045 @Bean 046 public ITermVersionAdapterSvc translationAdaptorVersion() { 047 return new TermVersionAdapterSvcDstu2(); 048 } 049 050 @Bean(name = "mySystemDaoDstu2") 051 public IFhirSystemDao<Bundle, MetaDt> systemDaoDstu2() { 052 ca.uhn.fhir.jpa.dao.FhirSystemDaoDstu2 retVal = new ca.uhn.fhir.jpa.dao.FhirSystemDaoDstu2(); 053 return retVal; 054 } 055 056 @Bean(name = "mySystemProviderDstu2") 057 public JpaSystemProvider<Bundle, MetaDt> systemProviderDstu2(FhirContext theFhirContext) { 058 JpaSystemProvider<Bundle, MetaDt> retVal = new ca.uhn.fhir.jpa.provider.JpaSystemProvider<>(); 059 retVal.setDao(systemDaoDstu2()); 060 retVal.setContext(theFhirContext); 061 return retVal; 062 } 063}