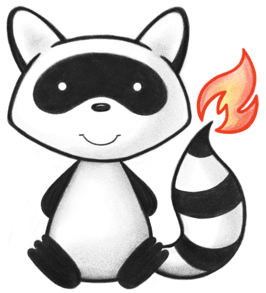
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.config; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.support.DefaultProfileValidationSupport; 024import ca.uhn.fhir.context.support.IValidationSupport; 025import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 026import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 027import ca.uhn.fhir.jpa.dao.JpaPersistedResourceValidationSupport; 028import ca.uhn.fhir.jpa.validation.FhirContextValidationSupportSvc; 029import ca.uhn.fhir.jpa.validation.ValidatorPolicyAdvisor; 030import ca.uhn.fhir.jpa.validation.ValidatorResourceFetcher; 031import ca.uhn.fhir.validation.IInstanceValidatorModule; 032import org.hl7.fhir.common.hapi.validation.support.InMemoryTerminologyServerValidationSupport; 033import org.hl7.fhir.common.hapi.validation.validator.FhirInstanceValidator; 034import org.hl7.fhir.r5.utils.validation.constants.BestPracticeWarningLevel; 035import org.springframework.beans.factory.annotation.Autowired; 036import org.springframework.context.annotation.Bean; 037import org.springframework.context.annotation.Configuration; 038import org.springframework.context.annotation.Lazy; 039 040@Configuration 041public class ValidationSupportConfig { 042 043 @Autowired 044 private FhirContext myFhirContext; 045 046 @Bean 047 public FhirContextValidationSupportSvc fhirValidationSupportSvc() { 048 return new FhirContextValidationSupportSvc(myFhirContext); 049 } 050 051 @Bean(name = JpaConfig.DEFAULT_PROFILE_VALIDATION_SUPPORT) 052 public DefaultProfileValidationSupport defaultProfileValidationSupport() { 053 return new DefaultProfileValidationSupport(myFhirContext); 054 } 055 056 @Bean 057 public InMemoryTerminologyServerValidationSupport inMemoryTerminologyServerValidationSupport( 058 FhirContext theFhirContext, JpaStorageSettings theStorageSettings) { 059 InMemoryTerminologyServerValidationSupport retVal = 060 new InMemoryTerminologyServerValidationSupport(theFhirContext); 061 retVal.setIssueSeverityForCodeDisplayMismatch(theStorageSettings.getIssueSeverityForCodeDisplayMismatch()); 062 return retVal; 063 } 064 065 @Bean(name = JpaConfig.JPA_VALIDATION_SUPPORT) 066 public IValidationSupport jpaValidationSupport(FhirContext theFhirContext) { 067 return new JpaPersistedResourceValidationSupport(theFhirContext); 068 } 069 070 @Bean(name = "myInstanceValidator") 071 public IInstanceValidatorModule instanceValidator( 072 FhirContext theFhirContext, IValidationSupport theValidationSupportChain, DaoRegistry theDaoRegistry) { 073 FhirInstanceValidator val = new FhirInstanceValidator(theValidationSupportChain); 074 val.setValidatorResourceFetcher( 075 jpaValidatorResourceFetcher(theFhirContext, theValidationSupportChain, theDaoRegistry)); 076 val.setValidatorPolicyAdvisor(jpaValidatorPolicyAdvisor()); 077 val.setBestPracticeWarningLevel(BestPracticeWarningLevel.Warning); 078 return val; 079 } 080 081 @Bean 082 @Lazy 083 public ValidatorResourceFetcher jpaValidatorResourceFetcher( 084 FhirContext theFhirContext, IValidationSupport theValidationSupport, DaoRegistry theDaoRegistry) { 085 return new ValidatorResourceFetcher(theFhirContext, theValidationSupport, theDaoRegistry); 086 } 087 088 @Bean 089 @Lazy 090 public ValidatorPolicyAdvisor jpaValidatorPolicyAdvisor() { 091 return new ValidatorPolicyAdvisor(); 092 } 093}