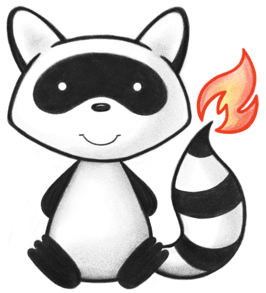
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.config.dstu3; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.support.IValidationSupport; 024import ca.uhn.fhir.jpa.api.IDaoRegistry; 025import ca.uhn.fhir.jpa.api.dao.IFhirSystemDao; 026import ca.uhn.fhir.jpa.config.GeneratedDaoAndResourceProviderConfigDstu3; 027import ca.uhn.fhir.jpa.config.JpaConfig; 028import ca.uhn.fhir.jpa.dao.ITransactionProcessorVersionAdapter; 029import ca.uhn.fhir.jpa.dao.dstu3.TransactionProcessorVersionAdapterDstu3; 030import ca.uhn.fhir.jpa.graphql.GraphQLProvider; 031import ca.uhn.fhir.jpa.graphql.GraphQLProviderWithIntrospection; 032import ca.uhn.fhir.jpa.provider.JpaSystemProvider; 033import ca.uhn.fhir.jpa.term.TermLoaderSvcImpl; 034import ca.uhn.fhir.jpa.term.TermVersionAdapterSvcDstu3; 035import ca.uhn.fhir.jpa.term.api.ITermCodeSystemStorageSvc; 036import ca.uhn.fhir.jpa.term.api.ITermDeferredStorageSvc; 037import ca.uhn.fhir.jpa.term.api.ITermLoaderSvc; 038import ca.uhn.fhir.jpa.term.api.ITermVersionAdapterSvc; 039import ca.uhn.fhir.rest.server.util.ISearchParamRegistry; 040import org.hl7.fhir.dstu3.model.Bundle; 041import org.hl7.fhir.dstu3.model.Meta; 042import org.hl7.fhir.utilities.graphql.IGraphQLStorageServices; 043import org.springframework.context.annotation.Bean; 044import org.springframework.context.annotation.Configuration; 045import org.springframework.context.annotation.Import; 046import org.springframework.context.annotation.Lazy; 047import org.springframework.transaction.annotation.EnableTransactionManagement; 048 049@Configuration 050@EnableTransactionManagement 051@Import({FhirContextDstu3Config.class, GeneratedDaoAndResourceProviderConfigDstu3.class, JpaConfig.class}) 052public class JpaDstu3Config { 053 @Bean 054 public ITermVersionAdapterSvc terminologyVersionAdapterSvc() { 055 return new TermVersionAdapterSvcDstu3(); 056 } 057 058 @Bean(name = JpaConfig.GRAPHQL_PROVIDER_NAME) 059 @Lazy 060 public GraphQLProvider graphQLProvider( 061 FhirContext theFhirContext, 062 IGraphQLStorageServices theGraphqlStorageServices, 063 IValidationSupport theValidationSupport, 064 ISearchParamRegistry theSearchParamRegistry, 065 IDaoRegistry theDaoRegistry) { 066 return new GraphQLProviderWithIntrospection( 067 theFhirContext, 068 theValidationSupport, 069 theGraphqlStorageServices, 070 theSearchParamRegistry, 071 theDaoRegistry); 072 } 073 074 @Bean 075 public ITransactionProcessorVersionAdapter transactionProcessorVersionFacade() { 076 return new TransactionProcessorVersionAdapterDstu3(); 077 } 078 079 @Bean(name = "mySystemDaoDstu3") 080 public IFhirSystemDao<Bundle, Meta> systemDaoDstu3() { 081 return new ca.uhn.fhir.jpa.dao.dstu3.FhirSystemDaoDstu3(); 082 } 083 084 @Bean(name = "mySystemProviderDstu3") 085 public JpaSystemProvider<Bundle, Meta> systemProviderDstu3(FhirContext theFhirContext) { 086 JpaSystemProvider<Bundle, Meta> retVal = new JpaSystemProvider<>(); 087 retVal.setContext(theFhirContext); 088 retVal.setDao(systemDaoDstu3()); 089 return retVal; 090 } 091 092 @Bean 093 public ITermLoaderSvc termLoaderService( 094 ITermDeferredStorageSvc theDeferredStorageSvc, ITermCodeSystemStorageSvc theCodeSystemStorageSvc) { 095 return new TermLoaderSvcImpl(theDeferredStorageSvc, theCodeSystemStorageSvc); 096 } 097}