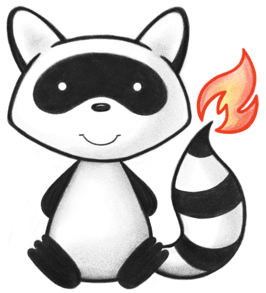
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.config.r4; 021 022import ca.uhn.fhir.batch2.api.IJobCoordinator; 023import ca.uhn.fhir.batch2.util.Batch2TaskHelper; 024import ca.uhn.fhir.context.FhirContext; 025import ca.uhn.fhir.context.support.IValidationSupport; 026import ca.uhn.fhir.jpa.api.IDaoRegistry; 027import ca.uhn.fhir.jpa.api.config.JpaStorageSettings; 028import ca.uhn.fhir.jpa.api.dao.DaoRegistry; 029import ca.uhn.fhir.jpa.api.dao.IFhirSystemDao; 030import ca.uhn.fhir.jpa.config.GeneratedDaoAndResourceProviderConfigR4; 031import ca.uhn.fhir.jpa.config.JpaConfig; 032import ca.uhn.fhir.jpa.dao.ITransactionProcessorVersionAdapter; 033import ca.uhn.fhir.jpa.dao.r4.TransactionProcessorVersionAdapterR4; 034import ca.uhn.fhir.jpa.dao.tx.HapiTransactionService; 035import ca.uhn.fhir.jpa.graphql.GraphQLProvider; 036import ca.uhn.fhir.jpa.graphql.GraphQLProviderWithIntrospection; 037import ca.uhn.fhir.jpa.partition.IRequestPartitionHelperSvc; 038import ca.uhn.fhir.jpa.provider.IReplaceReferencesSvc; 039import ca.uhn.fhir.jpa.provider.JpaSystemProvider; 040import ca.uhn.fhir.jpa.provider.merge.PatientMergeProvider; 041import ca.uhn.fhir.jpa.provider.merge.ResourceMergeService; 042import ca.uhn.fhir.jpa.term.TermLoaderSvcImpl; 043import ca.uhn.fhir.jpa.term.TermVersionAdapterSvcR4; 044import ca.uhn.fhir.jpa.term.api.ITermCodeSystemStorageSvc; 045import ca.uhn.fhir.jpa.term.api.ITermDeferredStorageSvc; 046import ca.uhn.fhir.jpa.term.api.ITermLoaderSvc; 047import ca.uhn.fhir.jpa.term.api.ITermVersionAdapterSvc; 048import ca.uhn.fhir.rest.server.util.ISearchParamRegistry; 049import org.hl7.fhir.r4.model.Bundle; 050import org.hl7.fhir.r4.model.Meta; 051import org.hl7.fhir.utilities.graphql.IGraphQLStorageServices; 052import org.springframework.context.annotation.Bean; 053import org.springframework.context.annotation.Configuration; 054import org.springframework.context.annotation.Import; 055import org.springframework.context.annotation.Lazy; 056import org.springframework.transaction.annotation.EnableTransactionManagement; 057 058@Configuration 059@EnableTransactionManagement 060@Import({FhirContextR4Config.class, GeneratedDaoAndResourceProviderConfigR4.class, JpaConfig.class}) 061public class JpaR4Config { 062 063 @Bean 064 public ITermVersionAdapterSvc terminologyVersionAdapterSvc() { 065 return new TermVersionAdapterSvcR4(); 066 } 067 068 @Bean 069 public ITransactionProcessorVersionAdapter transactionProcessorVersionFacade() { 070 return new TransactionProcessorVersionAdapterR4(); 071 } 072 073 @Bean(name = JpaConfig.GRAPHQL_PROVIDER_NAME) 074 @Lazy 075 public GraphQLProvider graphQLProvider( 076 FhirContext theFhirContext, 077 IGraphQLStorageServices theGraphqlStorageServices, 078 IValidationSupport theValidationSupport, 079 ISearchParamRegistry theSearchParamRegistry, 080 IDaoRegistry theDaoRegistry) { 081 return new GraphQLProviderWithIntrospection( 082 theFhirContext, 083 theValidationSupport, 084 theGraphqlStorageServices, 085 theSearchParamRegistry, 086 theDaoRegistry); 087 } 088 089 @Bean(name = "mySystemDaoR4") 090 public IFhirSystemDao<Bundle, Meta> systemDaoR4() { 091 ca.uhn.fhir.jpa.dao.r4.FhirSystemDaoR4 retVal = new ca.uhn.fhir.jpa.dao.r4.FhirSystemDaoR4(); 092 return retVal; 093 } 094 095 @Bean(name = "mySystemProviderR4") 096 public JpaSystemProvider<Bundle, Meta> systemProviderR4(FhirContext theFhirContext) { 097 JpaSystemProvider<Bundle, Meta> retVal = new JpaSystemProvider<>(); 098 retVal.setContext(theFhirContext); 099 retVal.setDao(systemDaoR4()); 100 return retVal; 101 } 102 103 @Bean 104 public ITermLoaderSvc termLoaderService( 105 ITermDeferredStorageSvc theDeferredStorageSvc, ITermCodeSystemStorageSvc theCodeSystemStorageSvc) { 106 return new TermLoaderSvcImpl(theDeferredStorageSvc, theCodeSystemStorageSvc); 107 } 108 109 @Bean 110 public ResourceMergeService resourceMergeService( 111 DaoRegistry theDaoRegistry, 112 IReplaceReferencesSvc theReplaceReferencesSvc, 113 HapiTransactionService theHapiTransactionService, 114 IRequestPartitionHelperSvc theRequestPartitionHelperSvc, 115 IJobCoordinator theJobCoordinator, 116 Batch2TaskHelper theBatch2TaskHelper, 117 JpaStorageSettings theStorageSettings) { 118 119 return new ResourceMergeService( 120 theStorageSettings, 121 theDaoRegistry, 122 theReplaceReferencesSvc, 123 theHapiTransactionService, 124 theRequestPartitionHelperSvc, 125 theJobCoordinator, 126 theBatch2TaskHelper); 127 } 128 129 @Bean 130 public PatientMergeProvider patientMergeProvider( 131 FhirContext theFhirContext, DaoRegistry theDaoRegistry, ResourceMergeService theResourceMergeService) { 132 return new PatientMergeProvider(theFhirContext, theDaoRegistry, theResourceMergeService); 133 } 134}