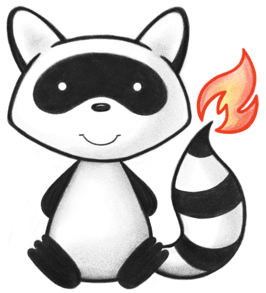
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.config.r5; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.support.IValidationSupport; 024import ca.uhn.fhir.jpa.api.IDaoRegistry; 025import ca.uhn.fhir.jpa.api.dao.IFhirSystemDao; 026import ca.uhn.fhir.jpa.config.GeneratedDaoAndResourceProviderConfigR5; 027import ca.uhn.fhir.jpa.config.JpaConfig; 028import ca.uhn.fhir.jpa.dao.ITransactionProcessorVersionAdapter; 029import ca.uhn.fhir.jpa.dao.r5.TransactionProcessorVersionAdapterR5; 030import ca.uhn.fhir.jpa.graphql.GraphQLProvider; 031import ca.uhn.fhir.jpa.graphql.GraphQLProviderWithIntrospection; 032import ca.uhn.fhir.jpa.provider.JpaSystemProvider; 033import ca.uhn.fhir.jpa.term.TermLoaderSvcImpl; 034import ca.uhn.fhir.jpa.term.TermVersionAdapterSvcR5; 035import ca.uhn.fhir.jpa.term.api.ITermCodeSystemStorageSvc; 036import ca.uhn.fhir.jpa.term.api.ITermDeferredStorageSvc; 037import ca.uhn.fhir.jpa.term.api.ITermLoaderSvc; 038import ca.uhn.fhir.jpa.term.api.ITermVersionAdapterSvc; 039import ca.uhn.fhir.rest.server.util.ISearchParamRegistry; 040import org.hl7.fhir.r5.model.Bundle; 041import org.hl7.fhir.r5.model.Meta; 042import org.hl7.fhir.utilities.graphql.IGraphQLStorageServices; 043import org.springframework.context.annotation.Bean; 044import org.springframework.context.annotation.Configuration; 045import org.springframework.context.annotation.Import; 046import org.springframework.context.annotation.Lazy; 047import org.springframework.transaction.annotation.EnableTransactionManagement; 048 049@Configuration 050@EnableTransactionManagement 051@Import({FhirContextR5Config.class, GeneratedDaoAndResourceProviderConfigR5.class, JpaConfig.class}) 052public class JpaR5Config { 053 054 @Bean 055 public ITermVersionAdapterSvc terminologyVersionAdapterSvc() { 056 return new TermVersionAdapterSvcR5(); 057 } 058 059 @Bean 060 public ITransactionProcessorVersionAdapter transactionProcessorVersionFacade() { 061 return new TransactionProcessorVersionAdapterR5(); 062 } 063 064 @Bean(name = JpaConfig.GRAPHQL_PROVIDER_NAME) 065 @Lazy 066 public GraphQLProvider graphQLProvider( 067 FhirContext theFhirContext, 068 IGraphQLStorageServices theGraphqlStorageServices, 069 IValidationSupport theValidationSupport, 070 ISearchParamRegistry theSearchParamRegistry, 071 IDaoRegistry theDaoRegistry) { 072 return new GraphQLProviderWithIntrospection( 073 theFhirContext, 074 theValidationSupport, 075 theGraphqlStorageServices, 076 theSearchParamRegistry, 077 theDaoRegistry); 078 } 079 080 @Bean(name = "mySystemDaoR5") 081 public IFhirSystemDao<Bundle, Meta> systemDaoR5() { 082 ca.uhn.fhir.jpa.dao.r5.FhirSystemDaoR5 retVal = new ca.uhn.fhir.jpa.dao.r5.FhirSystemDaoR5(); 083 return retVal; 084 } 085 086 @Bean(name = "mySystemProviderR5") 087 public JpaSystemProvider<Bundle, Meta> systemProviderR5(FhirContext theFhirContext) { 088 JpaSystemProvider<Bundle, Meta> retVal = new JpaSystemProvider<>(); 089 retVal.setContext(theFhirContext); 090 retVal.setDao(systemDaoR5()); 091 return retVal; 092 } 093 094 @Bean 095 public ITermLoaderSvc terminologyLoaderService( 096 ITermDeferredStorageSvc theDeferredStorageSvc, ITermCodeSystemStorageSvc theCodeSystemStorageSvc) { 097 return new TermLoaderSvcImpl(theDeferredStorageSvc, theCodeSystemStorageSvc); 098 } 099}