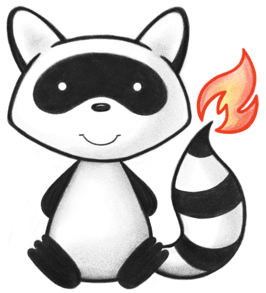
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.config.util; 021 022import net.ttddyy.dsproxy.support.ProxyDataSource; 023import org.apache.commons.dbcp2.BasicDataSource; 024import org.slf4j.Logger; 025import org.slf4j.LoggerFactory; 026 027import java.sql.SQLException; 028import java.util.Optional; 029import javax.sql.DataSource; 030 031/** 032 * Utility to hide complexity involved in obtaining connection pool information 033 */ 034public class ConnectionPoolInfoProvider implements IConnectionPoolInfoProvider { 035 private static final Logger ourLog = LoggerFactory.getLogger(ConnectionPoolInfoProvider.class); 036 037 private IConnectionPoolInfoProvider myProvider; 038 039 public ConnectionPoolInfoProvider(DataSource theDataSource) { 040 if (theDataSource.getClass().isAssignableFrom(BasicDataSource.class)) { 041 myProvider = new BasicDataSourceConnectionPoolInfoProvider((BasicDataSource) theDataSource); 042 return; 043 } 044 045 if (theDataSource.getClass().isAssignableFrom(ProxyDataSource.class)) { 046 boolean basiDataSourceWrapped; 047 try { 048 basiDataSourceWrapped = theDataSource.isWrapperFor(BasicDataSource.class); 049 if (basiDataSourceWrapped) { 050 BasicDataSource basicDataSource = theDataSource.unwrap(BasicDataSource.class); 051 myProvider = new BasicDataSourceConnectionPoolInfoProvider(basicDataSource); 052 } 053 } catch (SQLException ignored) { 054 } 055 } 056 } 057 058 @Override 059 public Optional<Integer> getTotalConnectionSize() { 060 return myProvider == null ? Optional.empty() : myProvider.getTotalConnectionSize(); 061 } 062 063 @Override 064 public Optional<Integer> getActiveConnections() { 065 return myProvider == null ? Optional.empty() : myProvider.getActiveConnections(); 066 } 067 068 @Override 069 public Optional<Long> getMaxWaitMillis() { 070 return myProvider == null ? Optional.empty() : myProvider.getMaxWaitMillis(); 071 } 072}