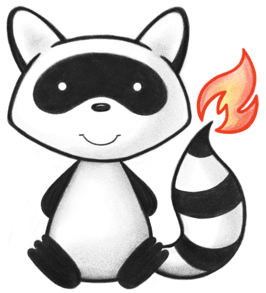
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao; 021 022import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoSubscription; 023import ca.uhn.fhir.jpa.dao.data.ISubscriptionTableDao; 024import ca.uhn.fhir.jpa.entity.SubscriptionTable; 025import ca.uhn.fhir.jpa.model.cross.IBasePersistedResource; 026import ca.uhn.fhir.jpa.model.entity.ResourceTable; 027import ca.uhn.fhir.model.dstu2.resource.Subscription; 028import ca.uhn.fhir.rest.api.server.RequestDetails; 029import ca.uhn.fhir.rest.api.server.storage.TransactionDetails; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031import org.hl7.fhir.instance.model.api.IIdType; 032import org.springframework.beans.factory.annotation.Autowired; 033import org.springframework.transaction.PlatformTransactionManager; 034 035import java.util.Date; 036 037public class FhirResourceDaoSubscriptionDstu2 extends BaseHapiFhirResourceDao<Subscription> 038 implements IFhirResourceDaoSubscription<Subscription> { 039 040 @Autowired 041 private ISubscriptionTableDao mySubscriptionTableDao; 042 043 @Autowired 044 private PlatformTransactionManager myTxManager; 045 046 @Override 047 public Long getSubscriptionTablePidForSubscriptionResource( 048 IIdType theId, RequestDetails theRequest, TransactionDetails theTransactionDetails) { 049 ResourceTable entity = readEntityLatestVersion(theId, theRequest, theTransactionDetails); 050 SubscriptionTable table = 051 mySubscriptionTableDao.findOneByResourcePid(entity.getId().getId()); 052 if (table == null) { 053 return null; 054 } 055 return table.getId(); 056 } 057 058 @Override 059 public ResourceTable updateEntity( 060 RequestDetails theRequest, 061 IBaseResource theResource, 062 IBasePersistedResource theEntity, 063 Date theDeletedTimestampOrNull, 064 boolean thePerformIndexing, 065 boolean theUpdateVersion, 066 TransactionDetails theTransactionDetails, 067 boolean theForceUpdate, 068 boolean theCreateNewHistoryEntry) { 069 ResourceTable retVal = super.updateEntity( 070 theRequest, 071 theResource, 072 theEntity, 073 theDeletedTimestampOrNull, 074 thePerformIndexing, 075 theUpdateVersion, 076 theTransactionDetails, 077 theForceUpdate, 078 theCreateNewHistoryEntry); 079 080 if (theDeletedTimestampOrNull != null) { 081 mySubscriptionTableDao.deleteAllForSubscription( 082 ((ResourceTable) theEntity).getResourceId().getId()); 083 } 084 return retVal; 085 } 086}