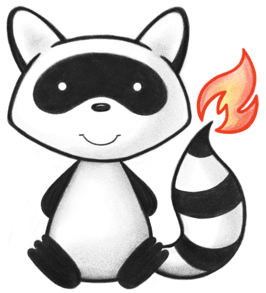
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao; 021 022import ca.uhn.fhir.jpa.model.entity.ResourceTable; 023import ca.uhn.fhir.jpa.model.search.ExtendedHSearchIndexData; 024import ca.uhn.fhir.jpa.search.autocomplete.ValueSetAutocompleteOptions; 025import ca.uhn.fhir.jpa.search.builder.ISearchQueryExecutor; 026import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 027import ca.uhn.fhir.jpa.searchparam.extractor.ResourceIndexedSearchParams; 028import ca.uhn.fhir.rest.api.server.RequestDetails; 029import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031 032import java.util.Collection; 033import java.util.List; 034 035@SuppressWarnings({"rawtypes"}) 036public interface IFulltextSearchSvc { 037 038 /** 039 * Search the Lucene/Elastic index for pids using params supported in theParams, 040 * consuming entries from theParams when used to query. 041 * 042 * @param theResourceName the resource name to restrict the query. 043 * @param theParams the full query - modified to return only params unused by the index. 044 * @param theRequestDetails The request details 045 * @return the pid list for the matchign resources. 046 */ 047 <T extends IResourcePersistentId> List<T> search( 048 String theResourceName, SearchParameterMap theParams, RequestDetails theRequestDetails); 049 050 /** 051 * Query the index for a plain list (non-scrollable) iterator of results. 052 * 053 * @param theResourceName e.g. Patient 054 * @param theParams The search query 055 * @param theMaxResultsToFetch maximum results to fetch 056 * @param theRequestDetails The request details 057 * @return Iterator of result PIDs 058 */ 059 ISearchQueryExecutor searchNotScrolled( 060 String theResourceName, 061 SearchParameterMap theParams, 062 Integer theMaxResultsToFetch, 063 RequestDetails theRequestDetails); 064 065 /** 066 * Autocomplete search for NIH $expand contextDirection=existing 067 * @param theOptions operation options 068 * @return a ValueSet with the search hits as the expansion. 069 */ 070 IBaseResource tokenAutocompleteValueSetSearch(ValueSetAutocompleteOptions theOptions); 071 072 <T extends IResourcePersistentId> List<T> everything( 073 String theResourceName, 074 SearchParameterMap theParams, 075 T theReferencingPid, 076 RequestDetails theRequestDetails); 077 078 boolean isDisabled(); 079 080 ExtendedHSearchIndexData extractLuceneIndexData( 081 IBaseResource theResource, ResourceIndexedSearchParams theNewParams); 082 083 /** 084 * Returns true if the parameter map can be handled for hibernate search. 085 * We have to filter out any queries that might use search params 086 * we only know how to handle in JPA. 087 * - 088 * See {@link ca.uhn.fhir.jpa.dao.search.ExtendedHSearchSearchBuilder#addAndConsumeAdvancedQueryClauses} 089 */ 090 boolean canUseHibernateSearch(String theResourceType, SearchParameterMap theParameterMap); 091 092 /** 093 * Re-publish the resource to the full-text index. 094 * - 095 * During update, hibernate search only republishes the entity if it has changed. 096 * During $reindex, we want to force the re-index. 097 * 098 * @param theEntity the fully populated ResourceTable entity 099 */ 100 void reindex(ResourceTable theEntity); 101 102 List<IResourcePersistentId> lastN(SearchParameterMap theParams, Integer theMaximumResults); 103 104 /** 105 * Returns inlined resource stored along with index mappings for matched identifiers 106 * 107 * @param thePids raw pids - we dont support versioned references 108 * @return Resources list or empty if nothing found 109 */ 110 List<IBaseResource> getResources(Collection<Long> thePids); 111 112 /** 113 * Returns accurate hit count 114 */ 115 long count(String theResourceName, SearchParameterMap theParams); 116 117 List<IBaseResource> searchForResources( 118 String theResourceType, SearchParameterMap theParams, RequestDetails theRequestDetails); 119 120 boolean supportsAllOf(SearchParameterMap theParams); 121 122 /** 123 * Given a resource type that is indexed by hibernate search, and a list of objects reprenting the IDs you wish to delete, 124 * this method will delete the resources from the Hibernate Search index. This is useful for situations where a deletion occurred 125 * outside a Hibernate ORM session, leaving dangling documents in the index. 126 * 127 * @param theClazz The class, which must be annotated with {@link org.hibernate.search.mapper.pojo.mapping.definition.annotation.Indexed} 128 * @param theGivenIds The list of IDs for the given document type. Note that while this is a List<Object>, the type must match the type of the `@Id` field on the given class. 129 */ 130 void deleteIndexedDocumentsByTypeAndId(Class theClazz, List<Object> theGivenIds); 131 132 /** 133 * Given a resource type and a {@link SearchParameterMap}, return true only if all sort terms are supported. 134 * 135 * @param theResourceName The resource type for the query. 136 * @param theParams The {@link SearchParameterMap} being searched with. 137 * @return true if all sort terms are supported, false otherwise. 138 */ 139 boolean supportsAllSortTerms(String theResourceName, SearchParameterMap theParams); 140}