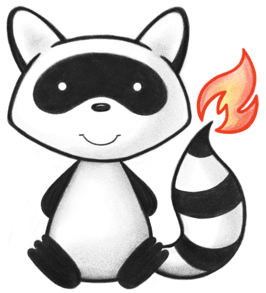
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao; 021 022import ca.uhn.fhir.context.support.IValidationSupport; 023import ca.uhn.fhir.context.support.TranslateConceptResults; 024import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoConceptMap; 025import ca.uhn.fhir.jpa.api.model.TranslationRequest; 026import ca.uhn.fhir.jpa.model.cross.IBasePersistedResource; 027import ca.uhn.fhir.jpa.model.entity.ResourceTable; 028import ca.uhn.fhir.jpa.term.api.ITermConceptMappingSvc; 029import ca.uhn.fhir.rest.api.server.RequestDetails; 030import ca.uhn.fhir.rest.api.server.storage.TransactionDetails; 031import ca.uhn.hapi.converters.canonical.VersionCanonicalizer; 032import org.hl7.fhir.instance.model.api.IBaseResource; 033import org.hl7.fhir.r4.model.ConceptMap; 034import org.springframework.beans.factory.annotation.Autowired; 035 036import java.util.Date; 037 038public class JpaResourceDaoConceptMap<T extends IBaseResource> extends JpaResourceDao<T> 039 implements IFhirResourceDaoConceptMap<T> { 040 @Autowired 041 private ITermConceptMappingSvc myTermConceptMappingSvc; 042 043 @Autowired 044 private IValidationSupport myValidationSupport; 045 046 @Autowired 047 private VersionCanonicalizer myVersionCanonicalizer; 048 049 @Override 050 public TranslateConceptResults translate( 051 TranslationRequest theTranslationRequest, RequestDetails theRequestDetails) { 052 IValidationSupport.TranslateCodeRequest translateCodeRequest = theTranslationRequest.asTranslateCodeRequest(); 053 return myValidationSupport.translateConcept(translateCodeRequest); 054 } 055 056 @Override 057 public ResourceTable updateEntity( 058 RequestDetails theRequestDetails, 059 IBaseResource theResource, 060 IBasePersistedResource theEntity, 061 Date theDeletedTimestampOrNull, 062 boolean thePerformIndexing, 063 boolean theUpdateVersion, 064 TransactionDetails theTransactionDetails, 065 boolean theForceUpdate, 066 boolean theCreateNewHistoryEntry) { 067 ResourceTable retVal = super.updateEntity( 068 theRequestDetails, 069 theResource, 070 theEntity, 071 theDeletedTimestampOrNull, 072 thePerformIndexing, 073 theUpdateVersion, 074 theTransactionDetails, 075 theForceUpdate, 076 theCreateNewHistoryEntry); 077 078 boolean entityWasSaved = !retVal.isUnchangedInCurrentOperation(); 079 boolean shouldProcessUpdate = entityWasSaved && thePerformIndexing; 080 if (shouldProcessUpdate) { 081 if (retVal.getDeleted() == null) { 082 ConceptMap conceptMap = myVersionCanonicalizer.conceptMapToCanonical(theResource); 083 myTermConceptMappingSvc.storeTermConceptMapAndChildren(retVal, conceptMap); 084 } else { 085 myTermConceptMappingSvc.deleteConceptMapAndChildren(retVal); 086 } 087 } 088 089 return retVal; 090 } 091}