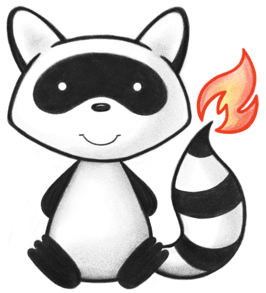
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoEncounter; 024import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 025import ca.uhn.fhir.jpa.searchparam.SearchParameterMap.EverythingModeEnum; 026import ca.uhn.fhir.rest.api.SortSpec; 027import ca.uhn.fhir.rest.api.server.IBundleProvider; 028import ca.uhn.fhir.rest.param.DateRangeParam; 029import ca.uhn.fhir.rest.param.StringParam; 030import jakarta.servlet.http.HttpServletRequest; 031import org.hl7.fhir.instance.model.api.IBaseResource; 032import org.hl7.fhir.instance.model.api.IIdType; 033import org.hl7.fhir.instance.model.api.IPrimitiveType; 034 035import java.util.Collections; 036 037public class JpaResourceDaoEncounter<T extends IBaseResource> extends BaseHapiFhirResourceDao<T> 038 implements IFhirResourceDaoEncounter<T> { 039 040 @Override 041 public IBundleProvider encounterInstanceEverything( 042 HttpServletRequest theServletRequest, 043 IIdType theId, 044 IPrimitiveType<Integer> theCount, 045 IPrimitiveType<Integer> theOffset, 046 DateRangeParam theLastUpdated, 047 SortSpec theSort) { 048 SearchParameterMap paramMap = new SearchParameterMap(); 049 if (theCount != null) { 050 paramMap.setCount(theCount.getValue()); 051 } 052 if (theOffset != null) { 053 throw new IllegalArgumentException( 054 Msg.code(1128) + "Everything operation does not support offset searching"); 055 } 056 057 // paramMap.setRevIncludes(Collections.singleton(IResource.INCLUDE_ALL.asRecursive())); 058 paramMap.setIncludes(Collections.singleton(IBaseResource.INCLUDE_ALL.asRecursive())); 059 paramMap.setEverythingMode( 060 theId != null ? EverythingModeEnum.ENCOUNTER_INSTANCE : EverythingModeEnum.ENCOUNTER_TYPE); 061 paramMap.setSort(theSort); 062 paramMap.setLastUpdated(theLastUpdated); 063 if (theId != null) { 064 paramMap.add("_id", new StringParam(theId.getIdPart())); 065 } 066 IBundleProvider retVal = search(paramMap); 067 return retVal; 068 } 069 070 @Override 071 public IBundleProvider encounterTypeEverything( 072 HttpServletRequest theServletRequest, 073 IPrimitiveType<Integer> theCount, 074 IPrimitiveType<Integer> theOffset, 075 DateRangeParam theLastUpdated, 076 SortSpec theSort) { 077 return encounterInstanceEverything(theServletRequest, null, theCount, theOffset, theLastUpdated, theSort); 078 } 079}