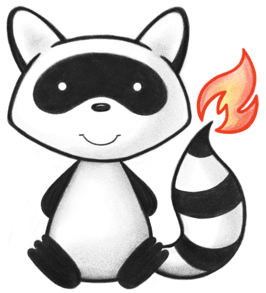
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.model.api.ExtensionDt; 025import ca.uhn.fhir.model.api.IResource; 026import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 027import ca.uhn.fhir.model.dstu2.resource.Bundle; 028import ca.uhn.fhir.model.dstu2.resource.OperationOutcome; 029import ca.uhn.fhir.model.dstu2.valueset.BundleTypeEnum; 030import ca.uhn.fhir.model.dstu2.valueset.HTTPVerbEnum; 031import ca.uhn.fhir.model.dstu2.valueset.IssueSeverityEnum; 032import ca.uhn.fhir.model.dstu2.valueset.IssueTypeEnum; 033import ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException; 034import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.IBaseExtension; 037import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 038import org.hl7.fhir.instance.model.api.IBaseResource; 039 040import java.util.Date; 041import java.util.List; 042import java.util.Optional; 043 044public class TransactionProcessorVersionAdapterDstu2 045 implements ITransactionProcessorVersionAdapter<Bundle, Bundle.Entry> { 046 @Override 047 public void setResponseStatus(Bundle.Entry theBundleEntry, String theStatus) { 048 theBundleEntry.getResponse().setStatus(theStatus); 049 } 050 051 @Override 052 public void setResponseLastModified(Bundle.Entry theBundleEntry, Date theLastModified) { 053 theBundleEntry.getResponse().setLastModified(theLastModified, TemporalPrecisionEnum.MILLI); 054 } 055 056 @Override 057 public void setResource(Bundle.Entry theBundleEntry, IBaseResource theResource) { 058 theBundleEntry.setResource((IResource) theResource); 059 } 060 061 @Override 062 public IBaseResource getResource(Bundle.Entry theBundleEntry) { 063 return theBundleEntry.getResource(); 064 } 065 066 @Override 067 public String getBundleType(Bundle theRequest) { 068 if (theRequest.getType() == null) { 069 return null; 070 } 071 return theRequest.getTypeElement().getValue(); 072 } 073 074 @Override 075 public void populateEntryWithOperationOutcome(BaseServerResponseException theCaughtEx, Bundle.Entry theEntry) { 076 OperationOutcome oo = new OperationOutcome(); 077 oo.addIssue() 078 .setSeverity(IssueSeverityEnum.ERROR) 079 .setDiagnostics(theCaughtEx.getMessage()) 080 .setCode(IssueTypeEnum.EXCEPTION); 081 theEntry.setResource(oo); 082 } 083 084 @Override 085 public Bundle createBundle(String theBundleType) { 086 Bundle resp = new Bundle(); 087 try { 088 resp.setType(BundleTypeEnum.forCode(theBundleType)); 089 } catch (FHIRException theE) { 090 throw new InternalErrorException(Msg.code(936) + "Unknown bundle type: " + theBundleType); 091 } 092 return resp; 093 } 094 095 @Override 096 public List<Bundle.Entry> getEntries(Bundle theRequest) { 097 return theRequest.getEntry(); 098 } 099 100 @Override 101 public void addEntry(Bundle theBundle, Bundle.Entry theEntry) { 102 theBundle.addEntry(theEntry); 103 } 104 105 @Override 106 public Bundle.Entry addEntry(Bundle theBundle) { 107 return theBundle.addEntry(); 108 } 109 110 @Override 111 public String getEntryRequestVerb(FhirContext theContext, Bundle.Entry theEntry) { 112 String retVal = null; 113 HTTPVerbEnum value = theEntry.getRequest().getMethodElement().getValueAsEnum(); 114 if (value != null) { 115 retVal = value.getCode(); 116 } 117 return retVal; 118 } 119 120 @Override 121 public String getFullUrl(Bundle.Entry theEntry) { 122 return theEntry.getFullUrl(); 123 } 124 125 @Override 126 public void setFullUrl(Bundle.Entry theEntry, String theFullUrl) { 127 theEntry.setFullUrl(theFullUrl); 128 } 129 130 @Override 131 public String getEntryIfNoneExist(Bundle.Entry theEntry) { 132 return theEntry.getRequest().getIfNoneExist(); 133 } 134 135 @Override 136 public String getEntryRequestUrl(Bundle.Entry theEntry) { 137 return theEntry.getRequest().getUrl(); 138 } 139 140 @Override 141 public void setResponseLocation(Bundle.Entry theEntry, String theResponseLocation) { 142 theEntry.getResponse().setLocation(theResponseLocation); 143 } 144 145 @Override 146 public void setResponseETag(Bundle.Entry theEntry, String theEtag) { 147 theEntry.getResponse().setEtag(theEtag); 148 } 149 150 @Override 151 public String getEntryRequestIfMatch(Bundle.Entry theEntry) { 152 return theEntry.getRequest().getIfMatch(); 153 } 154 155 @Override 156 public String getEntryRequestIfNoneExist(Bundle.Entry theEntry) { 157 return theEntry.getRequest().getIfNoneExist(); 158 } 159 160 @Override 161 public String getEntryRequestIfNoneMatch(Bundle.Entry theEntry) { 162 return theEntry.getRequest().getIfNoneMatch(); 163 } 164 165 @Override 166 public void setResponseOutcome(Bundle.Entry theEntry, IBaseOperationOutcome theOperationOutcome) { 167 theEntry.setResource((IResource) theOperationOutcome); 168 } 169 170 @Override 171 public void setRequestVerb(Bundle.Entry theEntry, String theVerb) { 172 theEntry.getRequest().setMethod(HTTPVerbEnum.forCode(theVerb)); 173 } 174 175 @Override 176 public void setRequestUrl(Bundle.Entry theEntry, String theUrl) { 177 theEntry.getRequest().setUrl(theUrl); 178 } 179 180 @Override 181 public Optional<IBaseExtension<?, ?>> getEntryRequestExtensionByUrl(Bundle.Entry theEntry, String theUrl) { 182 List<ExtensionDt> extensions = theEntry.getRequest().getUndeclaredExtensionsByUrl(theUrl); 183 return extensions.isEmpty() ? Optional.empty() : Optional.ofNullable(extensions.get(0)); 184 } 185}