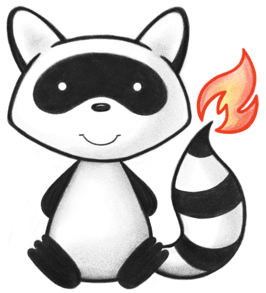
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.data; 021 022import ca.uhn.fhir.batch2.model.BatchInstanceStatusDTO; 023import ca.uhn.fhir.batch2.model.StatusEnum; 024import ca.uhn.fhir.jpa.entity.Batch2JobInstanceEntity; 025import org.springframework.data.domain.Pageable; 026import org.springframework.data.jpa.repository.JpaRepository; 027import org.springframework.data.jpa.repository.Modifying; 028import org.springframework.data.jpa.repository.Query; 029import org.springframework.data.repository.query.Param; 030 031import java.util.Date; 032import java.util.List; 033import java.util.Set; 034 035public interface IBatch2JobInstanceRepository 036 extends JpaRepository<Batch2JobInstanceEntity, String>, IHapiFhirJpaRepository { 037 038 @Modifying 039 @Query( 040 "UPDATE Batch2JobInstanceEntity e SET e.myStatus = :status WHERE e.myId = :id and e.myStatus IN ( :prior_states )") 041 int updateInstanceStatusIfIn( 042 @Param("id") String theInstanceId, 043 @Param("status") StatusEnum theNewState, 044 @Param("prior_states") Set<StatusEnum> thePriorStates); 045 046 @Modifying 047 @Query("UPDATE Batch2JobInstanceEntity e SET e.myUpdateTime = :updated WHERE e.myId = :id") 048 int updateInstanceUpdateTime(@Param("id") String theInstanceId, @Param("updated") Date theUpdated); 049 050 @Modifying 051 @Query("UPDATE Batch2JobInstanceEntity e SET e.myCancelled = :cancelled WHERE e.myId = :id") 052 int updateInstanceCancelled(@Param("id") String theInstanceId, @Param("cancelled") boolean theCancelled); 053 054 @Modifying 055 @Query("UPDATE Batch2JobInstanceEntity e SET e.myWorkChunksPurged = true WHERE e.myId = :id") 056 int updateWorkChunksPurgedTrue(@Param("id") String theInstanceId); 057 058 @Query( 059 "SELECT b from Batch2JobInstanceEntity b WHERE b.myDefinitionId = :defId AND (b.myParamsJson = :params OR b.myParamsJsonVc = :params) AND b.myStatus IN( :stats )") 060 List<Batch2JobInstanceEntity> findInstancesByJobIdParamsAndStatus( 061 @Param("defId") String theDefinitionId, 062 @Param("params") String theParams, 063 @Param("stats") Set<StatusEnum> theStatus, 064 Pageable thePageable); 065 066 @Query( 067 "SELECT b from Batch2JobInstanceEntity b WHERE b.myDefinitionId = :defId AND (b.myParamsJson = :params OR b.myParamsJsonVc = :params)") 068 List<Batch2JobInstanceEntity> findInstancesByJobIdAndParams( 069 @Param("defId") String theDefinitionId, @Param("params") String theParams, Pageable thePageable); 070 071 @Query("SELECT b from Batch2JobInstanceEntity b WHERE b.myStatus = :status") 072 List<Batch2JobInstanceEntity> findInstancesByJobStatus(@Param("status") StatusEnum theState, Pageable thePageable); 073 074 @Query("SELECT count(b) from Batch2JobInstanceEntity b WHERE b.myStatus = :status") 075 Integer findTotalJobsOfStatus(@Param("status") StatusEnum theState); 076 077 @Query( 078 "SELECT b from Batch2JobInstanceEntity b WHERE b.myDefinitionId = :defId AND b.myStatus IN( :stats ) AND b.myEndTime < :cutoff") 079 List<Batch2JobInstanceEntity> findInstancesByJobIdAndStatusAndExpiry( 080 @Param("defId") String theDefinitionId, 081 @Param("stats") Set<StatusEnum> theStatus, 082 @Param("cutoff") Date theCutoff, 083 Pageable thePageable); 084 085 @Query( 086 "SELECT e FROM Batch2JobInstanceEntity e WHERE e.myDefinitionId = :jobDefinitionId AND e.myStatus IN :statuses") 087 List<Batch2JobInstanceEntity> fetchInstancesByJobDefinitionIdAndStatus( 088 @Param("jobDefinitionId") String theJobDefinitionId, 089 @Param("statuses") Set<StatusEnum> theIncompleteStatuses, 090 Pageable thePageRequest); 091 092 @Query("SELECT e FROM Batch2JobInstanceEntity e WHERE e.myDefinitionId = :jobDefinitionId") 093 List<Batch2JobInstanceEntity> findInstancesByJobDefinitionId( 094 @Param("jobDefinitionId") String theJobDefinitionId, Pageable thePageRequest); 095 096 @Query( 097 "SELECT new ca.uhn.fhir.batch2.model.BatchInstanceStatusDTO(e.myId, e.myStatus, e.myStartTime, e.myEndTime) FROM Batch2JobInstanceEntity e WHERE e.myId = :id") 098 BatchInstanceStatusDTO fetchBatchInstanceStatus(@Param("id") String theInstanceId); 099}