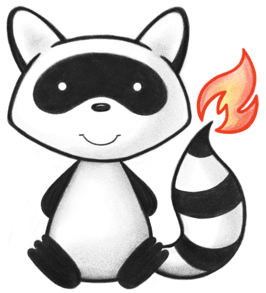
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.data; 021 022import ca.uhn.fhir.jpa.entity.Search; 023import com.google.errorprone.annotations.CanIgnoreReturnValue; 024import org.springframework.data.jpa.repository.JpaRepository; 025import org.springframework.data.jpa.repository.Modifying; 026import org.springframework.data.jpa.repository.Query; 027import org.springframework.data.repository.query.Param; 028 029import java.util.Collection; 030import java.util.Date; 031import java.util.Optional; 032import java.util.Set; 033import java.util.stream.Stream; 034 035public interface ISearchDao extends JpaRepository<Search, Long>, IHapiFhirJpaRepository { 036 037 @Query("SELECT s FROM Search s LEFT OUTER JOIN FETCH s.myIncludes WHERE s.myUuid = :uuid") 038 Optional<Search> findByUuidAndFetchIncludes(@Param("uuid") String theUuid); 039 040 @Query( 041 "SELECT s.myId FROM Search s WHERE (s.myCreated < :cutoff) AND (s.myExpiryOrNull IS NULL OR s.myExpiryOrNull < :now) AND (s.myDeleted IS NULL OR s.myDeleted = FALSE)") 042 Stream<Long> findWhereCreatedBefore(@Param("cutoff") Date theCutoff, @Param("now") Date theNow); 043 044 @Query("SELECT new ca.uhn.fhir.jpa.dao.data.SearchIdAndResultSize(" + "s.myId, " 045 + "(select max(sr.myOrder) as maxOrder from SearchResult sr where sr.mySearchPid = s.myId)) " 046 + "FROM Search s WHERE s.myDeleted = TRUE") 047 Stream<SearchIdAndResultSize> findDeleted(); 048 049 @Query( 050 "SELECT s FROM Search s WHERE s.myResourceType = :type AND s.mySearchQueryStringHash = :hash AND (s.myCreated > :cutoff) AND s.myDeleted = FALSE AND s.myStatus <> 'FAILED'") 051 Collection<Search> findWithCutoffOrExpiry( 052 @Param("type") String theResourceType, 053 @Param("hash") int theHashCode, 054 @Param("cutoff") Date theCreatedCutoff); 055 056 @Query("SELECT COUNT(s) FROM Search s WHERE s.myDeleted = TRUE") 057 int countDeleted(); 058 059 @Modifying 060 @Query("UPDATE Search s SET s.myDeleted = :deleted WHERE s.myId in (:pids)") 061 @CanIgnoreReturnValue 062 int updateDeleted(@Param("pids") Set<Long> thePid, @Param("deleted") boolean theDeleted); 063 064 @Modifying 065 @Query("DELETE FROM Search s WHERE s.myId = :pid") 066 void deleteByPid(@Param("pid") Long theId); 067 068 @Modifying 069 @Query("DELETE FROM Search s WHERE s.myId in (:pids)") 070 void deleteByPids(@Param("pids") Collection<Long> theSearchToDelete); 071}