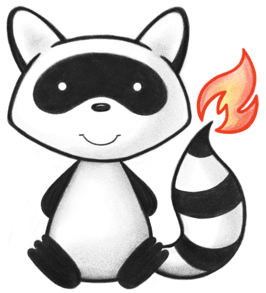
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.data; 021 022import ca.uhn.fhir.jpa.entity.TermCodeSystem; 023import ca.uhn.fhir.jpa.entity.TermCodeSystemVersion; 024import ca.uhn.fhir.jpa.model.dao.JpaPid; 025import ca.uhn.fhir.jpa.model.entity.IdAndPartitionId; 026import org.springframework.data.jpa.repository.JpaRepository; 027import org.springframework.data.jpa.repository.Modifying; 028import org.springframework.data.jpa.repository.Query; 029import org.springframework.data.repository.query.Param; 030 031import java.util.List; 032import java.util.Optional; 033 034public interface ITermCodeSystemVersionDao 035 extends JpaRepository<TermCodeSystemVersion, IdAndPartitionId>, IHapiFhirJpaRepository { 036 037 @Modifying 038 @Query("DELETE FROM TermCodeSystemVersion csv WHERE csv.myCodeSystem = :cs") 039 void deleteForCodeSystem(@Param("cs") TermCodeSystem theCodeSystem); 040 041 /** 042 * @return Return type is [PartitionId,Pid] 043 */ 044 @Query( 045 "SELECT t.myPartitionIdValue, t.myId FROM TermCodeSystemVersion t WHERE t.myCodeSystemPid = :codesystem_pid order by t.myId") 046 List<Object[]> findSortedPidsByCodeSystemPid(@Param("codesystem_pid") Long theCodeSystemPid); 047 048 @Query( 049 "SELECT cs FROM TermCodeSystemVersion cs WHERE cs.myCodeSystemPid = :codesystem_pid AND cs.myCodeSystemVersionId = :codesystem_version_id") 050 TermCodeSystemVersion findByCodeSystemPidAndVersion( 051 @Param("codesystem_pid") Long theCodeSystemPid, 052 @Param("codesystem_version_id") String theCodeSystemVersionId); 053 054 @Query("SELECT tcsv FROM TermCodeSystemVersion tcsv INNER JOIN FETCH TermCodeSystem tcs on tcs = tcsv.myCodeSystem " 055 + "WHERE tcs.myCodeSystemUri = :code_system_uri AND tcsv.myCodeSystemVersionId = :codesystem_version_id") 056 TermCodeSystemVersion findByCodeSystemUriAndVersion( 057 @Param("code_system_uri") String theCodeSystemUri, 058 @Param("codesystem_version_id") String theCodeSystemVersionId); 059 060 @Query( 061 "SELECT cs FROM TermCodeSystemVersion cs WHERE cs.myCodeSystemPid = :codesystem_pid AND cs.myCodeSystemVersionId IS NULL") 062 TermCodeSystemVersion findByCodeSystemPidVersionIsNull(@Param("codesystem_pid") Long theCodeSystemPid); 063 064 @Query("SELECT cs FROM TermCodeSystemVersion cs WHERE cs.myResource.myPid = :resource_id") 065 List<TermCodeSystemVersion> findByCodeSystemResourcePid(@Param("resource_id") JpaPid theCodeSystemResourcePid); 066 067 @Query("SELECT csv FROM TermCodeSystemVersion csv " + "JOIN TermCodeSystem cs ON (cs.myCurrentVersion = csv) " 068 + "WHERE cs.myResource.myPid.myId = :resource_id") 069 TermCodeSystemVersion findCurrentVersionForCodeSystemResourcePid( 070 @Param("resource_id") Long theCodeSystemResourcePid); 071 072 /** 073 * // TODO: JA2 Use partitioned query here 074 */ 075 @Deprecated 076 @Query("SELECT cs FROM TermCodeSystemVersion cs WHERE cs.myId = :pid") 077 Optional<TermCodeSystemVersion> findByPid(@Param("pid") long theVersionPid); 078}