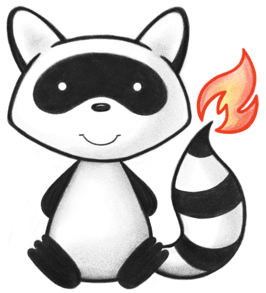
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.data; 021 022import ca.uhn.fhir.jpa.entity.TermCodeSystemVersion; 023import ca.uhn.fhir.jpa.entity.TermConcept; 024import org.springframework.data.domain.Page; 025import org.springframework.data.domain.Pageable; 026import org.springframework.data.jpa.repository.JpaRepository; 027import org.springframework.data.jpa.repository.Modifying; 028import org.springframework.data.jpa.repository.Query; 029import org.springframework.data.repository.query.Param; 030 031import java.util.List; 032import java.util.Optional; 033 034public interface ITermConceptDao extends JpaRepository<TermConcept, TermConcept.TermConceptPk>, IHapiFhirJpaRepository { 035 036 @Query("SELECT t FROM TermConcept t " + "LEFT JOIN FETCH t.myDesignations d " + "WHERE t.myId IN :pids") 037 List<TermConcept> fetchConceptsAndDesignationsByPid(@Param("pids") List<TermConcept.TermConceptPk> thePids); 038 039 @Query("SELECT t FROM TermConcept t " + "LEFT JOIN FETCH t.myDesignations d " 040 + "WHERE t.myCodeSystemVersionPid = :pid") 041 List<TermConcept> fetchConceptsAndDesignationsByVersionPid(@Param("pid") Long theCodeSystemVersionPid); 042 043 @Query("SELECT COUNT(t) FROM TermConcept t WHERE t.myCodeSystem.myId = :cs_pid") 044 Integer countByCodeSystemVersion(@Param("cs_pid") Long thePid); 045 046 @Query("SELECT c FROM TermConcept c WHERE c.myCodeSystemVersionPid = :csv_pid AND c.myCode = :code") 047 Optional<TermConcept> findByCodeSystemAndCode( 048 @Param("csv_pid") Long theCodeSystemVersionPid, @Param("code") String theCode); 049 050 @Query("FROM TermConcept WHERE myCodeSystemVersionPid = :csv_pid AND myCode in (:codeList)") 051 List<TermConcept> findByCodeSystemAndCodeList( 052 @Param("csv_pid") Long theCodeSystem, @Param("codeList") List<String> theCodeList); 053 054 @Modifying 055 @Query("DELETE FROM TermConcept WHERE myCodeSystem.myId = :cs_pid") 056 int deleteByCodeSystemVersion(@Param("cs_pid") Long thePid); 057 058 @Query("SELECT c FROM TermConcept c WHERE c.myCodeSystem = :code_system") 059 List<TermConcept> findByCodeSystemVersion(@Param("code_system") TermCodeSystemVersion theCodeSystem); 060 061 @Query("SELECT t FROM TermConcept t WHERE t.myIndexStatus = null") 062 Page<TermConcept> findResourcesRequiringReindexing(Pageable thePageRequest); 063}