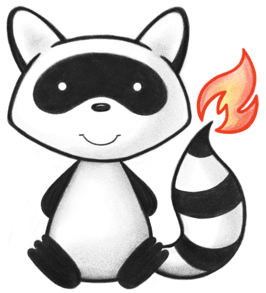
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.data; 021 022import ca.uhn.fhir.jpa.entity.TermConceptMap; 023import ca.uhn.fhir.jpa.model.entity.IdAndPartitionId; 024import org.springframework.data.domain.Pageable; 025import org.springframework.data.jpa.repository.JpaRepository; 026import org.springframework.data.jpa.repository.Modifying; 027import org.springframework.data.jpa.repository.Query; 028import org.springframework.data.repository.query.Param; 029 030import java.util.List; 031import java.util.Optional; 032 033public interface ITermConceptMapDao extends JpaRepository<TermConceptMap, IdAndPartitionId>, IHapiFhirJpaRepository { 034 @Query("DELETE FROM TermConceptMap cm WHERE cm.myId = :pid") 035 @Modifying 036 void deleteTermConceptMapById(@Param("pid") Long theId); 037 038 @Query("SELECT cm FROM TermConceptMap cm WHERE cm.myResourcePid = :resource_pid") 039 Optional<TermConceptMap> findTermConceptMapByResourcePid(@Param("resource_pid") Long theResourcePid); 040 041 // Keep backwards compatibility, recommend to use findTermConceptMapByUrlAndNullVersion instead 042 @Deprecated 043 @Query("SELECT cm FROM TermConceptMap cm WHERE cm.myUrl = :url and cm.myVersion is null") 044 Optional<TermConceptMap> findTermConceptMapByUrl(@Param("url") String theUrl); 045 046 @Query("SELECT cm FROM TermConceptMap cm WHERE cm.myUrl = :url and cm.myVersion is null") 047 Optional<TermConceptMap> findTermConceptMapByUrlAndNullVersion(@Param("url") String theUrl); 048 049 // Note that last updated version is considered current version. 050 @Query( 051 value = 052 "SELECT cm FROM TermConceptMap cm INNER JOIN ResourceTable r ON r = cm.myResource WHERE cm.myUrl = :url ORDER BY r.myUpdated DESC") 053 List<TermConceptMap> getTermConceptMapEntitiesByUrlOrderByMostRecentUpdate( 054 Pageable thePage, @Param("url") String theUrl); 055 056 @Query("SELECT cm FROM TermConceptMap cm WHERE cm.myUrl = :url AND cm.myVersion = :version") 057 Optional<TermConceptMap> findTermConceptMapByUrlAndVersion( 058 @Param("url") String theUrl, @Param("version") String theVersion); 059}