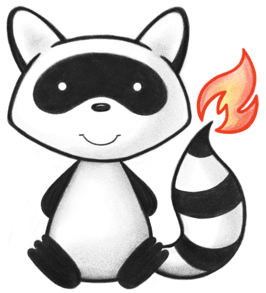
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.data; 021 022import ca.uhn.fhir.jpa.entity.TermValueSetConcept; 023import ca.uhn.fhir.jpa.model.entity.IdAndPartitionId; 024import org.springframework.data.domain.Pageable; 025import org.springframework.data.jpa.repository.JpaRepository; 026import org.springframework.data.jpa.repository.Modifying; 027import org.springframework.data.jpa.repository.Query; 028import org.springframework.data.repository.query.Param; 029 030import java.util.List; 031import java.util.Optional; 032 033public interface ITermValueSetConceptDao 034 extends JpaRepository<TermValueSetConcept, IdAndPartitionId>, IHapiFhirJpaRepository { 035 036 @Query("SELECT COUNT(*) FROM TermValueSetConcept vsc WHERE vsc.myValueSetPid = :pid") 037 Integer countByTermValueSetId(@Param("pid") Long theValueSetId); 038 039 @Query("DELETE FROM TermValueSetConcept vsc WHERE vsc.myValueSetPid = :pid") 040 @Modifying 041 void deleteByTermValueSetId(@Param("pid") Long theValueSetId); 042 043 @Query("SELECT vsc FROM TermValueSetConcept vsc WHERE vsc.myValueSetPid = :pid AND vsc.mySystem = :system_url") 044 List<TermValueSetConcept> findByTermValueSetIdSystemOnly( 045 Pageable thePage, @Param("pid") Long theValueSetId, @Param("system_url") String theSystem); 046 047 @Query( 048 "SELECT vsc FROM TermValueSetConcept vsc WHERE vsc.myValueSetPid = :pid AND vsc.mySystem = :system_url AND vsc.myCode = :codeval") 049 Optional<TermValueSetConcept> findByTermValueSetIdSystemAndCode( 050 @Param("pid") Long theValueSetId, @Param("system_url") String theSystem, @Param("codeval") String theCode); 051 052 @Query( 053 "SELECT vsc FROM TermValueSetConcept vsc WHERE vsc.myValueSetPid = :pid AND vsc.mySystem = :system_url AND vsc.mySystemVer = :system_version AND vsc.myCode = :codeval") 054 Optional<TermValueSetConcept> findByTermValueSetIdSystemAndCodeWithVersion( 055 @Param("pid") Long theValueSetId, 056 @Param("system_url") String theSystem, 057 @Param("system_version") String theSystemVersion, 058 @Param("codeval") String theCode); 059 060 @Query( 061 "SELECT vsc FROM TermValueSetConcept vsc WHERE vsc.myValueSet.myResourcePid = :resource_pid AND vsc.myCode = :codeval") 062 List<TermValueSetConcept> findByValueSetResourcePidAndCode( 063 @Param("resource_pid") Long theValueSetId, @Param("codeval") String theCode); 064 065 @Query( 066 "SELECT vsc FROM TermValueSetConcept vsc WHERE vsc.myValueSet.myResourcePid = :resource_pid AND vsc.mySystem = :system_url AND vsc.myCode = :codeval") 067 Optional<TermValueSetConcept> findByValueSetResourcePidSystemAndCode( 068 @Param("resource_pid") Long theValueSetId, 069 @Param("system_url") String theSystem, 070 @Param("codeval") String theCode); 071 072 @Query( 073 "SELECT vsc FROM TermValueSetConcept vsc WHERE vsc.myValueSet.myResourcePid = :resource_pid AND vsc.mySystem = :system_url AND vsc.mySystemVer = :system_version AND vsc.myCode = :codeval") 074 Optional<TermValueSetConcept> findByValueSetResourcePidSystemAndCodeWithVersion( 075 @Param("resource_pid") Long theValueSetId, 076 @Param("system_url") String theSystem, 077 @Param("system_version") String theSystemVersion, 078 @Param("codeval") String theCode); 079 080 @Query("SELECT vsc.myId FROM TermValueSetConcept vsc WHERE vsc.myValueSetPid = :pid ORDER BY vsc.myId") 081 List<Long> findIdsByTermValueSetId(@Param("pid") Long theValueSetId); 082 083 @Query("UPDATE TermValueSetConcept vsc SET vsc.myOrder = :order WHERE vsc.myId = :pid") 084 @Modifying 085 void updateOrderById(@Param("pid") Long theId, @Param("order") int theOrder); 086}