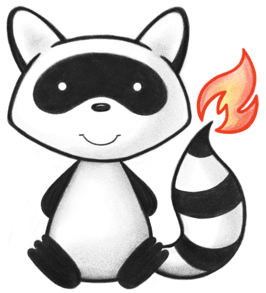
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.data; 021 022import ca.uhn.fhir.jpa.entity.TermValueSet; 023import ca.uhn.fhir.jpa.entity.TermValueSetPreExpansionStatusEnum; 024import org.springframework.data.domain.Pageable; 025import org.springframework.data.domain.Slice; 026import org.springframework.data.jpa.repository.JpaRepository; 027import org.springframework.data.jpa.repository.Query; 028import org.springframework.data.repository.query.Param; 029 030import java.util.List; 031import java.util.Optional; 032 033public interface ITermValueSetDao extends JpaRepository<TermValueSet, Long>, IHapiFhirJpaRepository { 034 035 @Query("SELECT vs FROM TermValueSet vs WHERE vs.myResourcePid = :resource_pid") 036 Optional<TermValueSet> findByResourcePid(@Param("resource_pid") Long theResourcePid); 037 038 // Keeping for backwards compatibility but recommend using findTermValueSetByUrlAndNullVersion instead. 039 @Deprecated 040 @Query("SELECT vs FROM TermValueSet vs WHERE vs.myUrl = :url") 041 Optional<TermValueSet> findByUrl(@Param("url") String theUrl); 042 043 @Query("SELECT vs FROM TermValueSet vs WHERE vs.myExpansionStatus = :expansion_status") 044 Slice<TermValueSet> findByExpansionStatus( 045 Pageable pageable, @Param("expansion_status") TermValueSetPreExpansionStatusEnum theExpansionStatus); 046 047 @Query( 048 value = 049 "SELECT vs FROM TermValueSet vs INNER JOIN ResourceTable r ON r.myId = vs.myResourcePid WHERE vs.myUrl = :url ORDER BY r.myUpdated DESC") 050 List<TermValueSet> findTermValueSetByUrl(Pageable thePage, @Param("url") String theUrl); 051 052 /** 053 * The current TermValueSet is not necessarily the last uploaded anymore, but the current VS resource 054 * is pointed by a specific ForcedId, so we locate current ValueSet as the one pointing to current VS resource 055 */ 056 @Query(value = "SELECT vs FROM TermValueSet vs where vs.myResource.myFhirId = :forcedId ") 057 Optional<TermValueSet> findTermValueSetByForcedId(@Param("forcedId") String theForcedId); 058 059 @Query("SELECT vs FROM TermValueSet vs WHERE vs.myUrl = :url AND vs.myVersion IS NULL") 060 Optional<TermValueSet> findTermValueSetByUrlAndNullVersion(@Param("url") String theUrl); 061 062 @Query("SELECT vs FROM TermValueSet vs WHERE vs.myUrl = :url AND vs.myVersion = :version") 063 Optional<TermValueSet> findTermValueSetByUrlAndVersion( 064 @Param("url") String theUrl, @Param("version") String theVersion); 065}