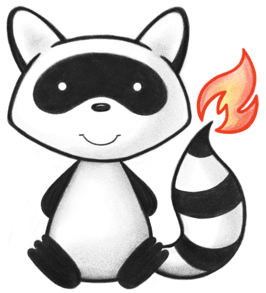
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.dstu3; 021 022import ca.uhn.fhir.jpa.api.dao.IFhirResourceDaoSubscription; 023import ca.uhn.fhir.jpa.dao.BaseHapiFhirResourceDao; 024import ca.uhn.fhir.jpa.dao.data.ISubscriptionTableDao; 025import ca.uhn.fhir.jpa.entity.SubscriptionTable; 026import ca.uhn.fhir.jpa.model.cross.IBasePersistedResource; 027import ca.uhn.fhir.jpa.model.entity.ResourceTable; 028import ca.uhn.fhir.rest.api.server.RequestDetails; 029import ca.uhn.fhir.rest.api.server.storage.TransactionDetails; 030import org.hl7.fhir.dstu3.model.Subscription; 031import org.hl7.fhir.instance.model.api.IBaseResource; 032import org.hl7.fhir.instance.model.api.IIdType; 033import org.springframework.beans.factory.annotation.Autowired; 034 035import java.util.Date; 036 037public class FhirResourceDaoSubscriptionDstu3 extends BaseHapiFhirResourceDao<Subscription> 038 implements IFhirResourceDaoSubscription<Subscription> { 039 040 @Autowired 041 private ISubscriptionTableDao mySubscriptionTableDao; 042 043 @Override 044 public Long getSubscriptionTablePidForSubscriptionResource( 045 IIdType theId, RequestDetails theRequest, TransactionDetails theTransactionDetails) { 046 ResourceTable entity = readEntityLatestVersion(theId, theRequest, theTransactionDetails); 047 SubscriptionTable table = 048 mySubscriptionTableDao.findOneByResourcePid(entity.getId().getId()); 049 if (table == null) { 050 return null; 051 } 052 return table.getId(); 053 } 054 055 @Override 056 public ResourceTable updateEntity( 057 RequestDetails theRequest, 058 IBaseResource theResource, 059 IBasePersistedResource theEntity, 060 Date theDeletedTimestampOrNull, 061 boolean thePerformIndexing, 062 boolean theUpdateVersion, 063 TransactionDetails theTransactionDetails, 064 boolean theForceUpdate, 065 boolean theCreateNewHistoryEntry) { 066 ResourceTable retVal = super.updateEntity( 067 theRequest, 068 theResource, 069 theEntity, 070 theDeletedTimestampOrNull, 071 thePerformIndexing, 072 theUpdateVersion, 073 theTransactionDetails, 074 theForceUpdate, 075 theCreateNewHistoryEntry); 076 077 if (theDeletedTimestampOrNull != null) { 078 mySubscriptionTableDao.deleteAllForSubscription( 079 ((ResourceTable) theEntity).getResourceId().getId()); 080 } 081 return retVal; 082 } 083}