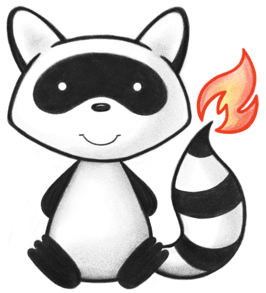
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.expunge; 021 022import org.apache.commons.lang3.Validate; 023import org.apache.commons.lang3.builder.EqualsBuilder; 024import org.apache.commons.lang3.builder.HashCodeBuilder; 025import org.apache.commons.lang3.builder.ToStringBuilder; 026import org.apache.commons.lang3.builder.ToStringStyle; 027 028public class ResourceForeignKey { 029 public final String myTable; 030 public final String myResourceIdColumn; 031 public final String myPartitionIdColumn; 032 033 public ResourceForeignKey(String theTable, String thePartitionIdColumn, String theResourceIdColumn) { 034 myTable = theTable; 035 myPartitionIdColumn = thePartitionIdColumn; 036 myResourceIdColumn = theResourceIdColumn; 037 } 038 039 @Override 040 public boolean equals(Object theO) { 041 if (this == theO) return true; 042 043 if (theO == null || getClass() != theO.getClass()) return false; 044 045 ResourceForeignKey that = (ResourceForeignKey) theO; 046 047 return new EqualsBuilder() 048 .append(myTable, that.myTable) 049 .append(myResourceIdColumn, that.myResourceIdColumn) 050 .append(myPartitionIdColumn, that.myPartitionIdColumn) 051 .isEquals(); 052 } 053 054 @Override 055 public int hashCode() { 056 return new HashCodeBuilder(17, 37) 057 .append(myTable) 058 .append(myPartitionIdColumn) 059 .append(myResourceIdColumn) 060 .toHashCode(); 061 } 062 063 @Override 064 public String toString() { 065 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 066 .append("table", myTable) 067 .append("resourceIdColumn", myResourceIdColumn) 068 .append("partitionIdColumn", myPartitionIdColumn) 069 .toString(); 070 } 071 072 public static final class ResourceForeignKeyBuilder { 073 private String myTable; 074 private String myResourceIdColumn; 075 private String myPartitionIdColumn; 076 077 public ResourceForeignKeyBuilder() {} 078 079 public void withTable(String myTable) { 080 this.myTable = myTable; 081 } 082 083 public void withResourceIdColumn(String myResourceIdColumn) { 084 this.myResourceIdColumn = myResourceIdColumn; 085 } 086 087 public void withPartitionIdColumn(String myPartitionIdColumn) { 088 this.myPartitionIdColumn = myPartitionIdColumn; 089 } 090 091 public ResourceForeignKey build() { 092 Validate.notBlank(myTable, "Table is required"); 093 Validate.notBlank(myResourceIdColumn, "ResourceIdColumn is required"); 094 Validate.notBlank( 095 myPartitionIdColumn, 096 "PartitionIdColumn is required for table " + myTable + " and resource ID: " + myResourceIdColumn); 097 return new ResourceForeignKey(this.myTable, this.myPartitionIdColumn, this.myResourceIdColumn); 098 } 099 } 100}