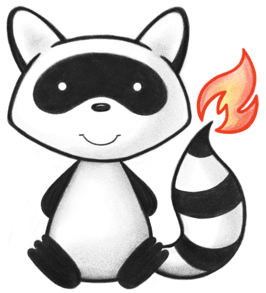
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.index; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import ca.uhn.fhir.jpa.model.dao.JpaPid; 024import ca.uhn.fhir.jpa.model.entity.ResourceTable; 025import ca.uhn.fhir.jpa.searchparam.extractor.BaseSearchParamWithInlineReferencesExtractor; 026import ca.uhn.fhir.jpa.searchparam.extractor.ISearchParamExtractor; 027import ca.uhn.fhir.jpa.searchparam.extractor.ISearchParamWithInlineReferencesExtractor; 028import ca.uhn.fhir.jpa.searchparam.extractor.ResourceIndexedSearchParams; 029import ca.uhn.fhir.jpa.searchparam.extractor.SearchParamExtractorService; 030import ca.uhn.fhir.rest.api.server.RequestDetails; 031import ca.uhn.fhir.rest.api.server.storage.TransactionDetails; 032import com.google.common.annotations.VisibleForTesting; 033import jakarta.persistence.EntityManager; 034import jakarta.persistence.PersistenceContext; 035import jakarta.persistence.PersistenceContextType; 036import org.hl7.fhir.instance.model.api.IBaseResource; 037import org.springframework.beans.factory.annotation.Autowired; 038import org.springframework.context.annotation.Lazy; 039import org.springframework.stereotype.Service; 040 041@Service 042@Lazy 043public class SearchParamWithInlineReferencesExtractor extends BaseSearchParamWithInlineReferencesExtractor<JpaPid> 044 implements ISearchParamWithInlineReferencesExtractor { 045 046 @PersistenceContext(type = PersistenceContextType.TRANSACTION) 047 protected EntityManager myEntityManager; 048 049 @Autowired 050 private SearchParamExtractorService mySearchParamExtractorService; 051 052 @VisibleForTesting 053 public void setSearchParamExtractorService(SearchParamExtractorService theSearchParamExtractorService) { 054 mySearchParamExtractorService = theSearchParamExtractorService; 055 } 056 057 public void populateFromResource( 058 RequestPartitionId theRequestPartitionId, 059 ResourceIndexedSearchParams theParams, 060 TransactionDetails theTransactionDetails, 061 ResourceTable theEntity, 062 IBaseResource theResource, 063 ResourceIndexedSearchParams theExistingParams, 064 RequestDetails theRequest, 065 boolean thePerformIndexing) { 066 if (thePerformIndexing) { 067 // Perform inline match URL substitution 068 extractInlineReferences(theRequest, theResource, theTransactionDetails); 069 } 070 071 mySearchParamExtractorService.extractFromResource( 072 theRequestPartitionId, 073 theRequest, 074 theParams, 075 theExistingParams, 076 theEntity, 077 theResource, 078 theTransactionDetails, 079 thePerformIndexing, 080 ISearchParamExtractor.ALL_PARAMS); 081 } 082}