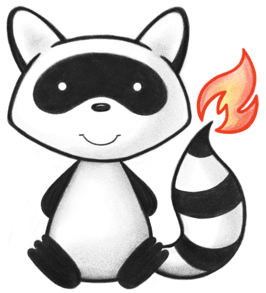
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import ca.uhn.fhir.batch2.model.WorkChunkMetadata; 023import ca.uhn.fhir.batch2.model.WorkChunkStatusEnum; 024import jakarta.persistence.Column; 025import jakarta.persistence.Entity; 026import jakarta.persistence.EnumType; 027import jakarta.persistence.Enumerated; 028import jakarta.persistence.Id; 029import org.hibernate.annotations.Immutable; 030import org.hibernate.annotations.JdbcTypeCode; 031import org.hibernate.annotations.Subselect; 032import org.hibernate.type.SqlTypes; 033 034import java.io.Serializable; 035 036import static ca.uhn.fhir.batch2.model.JobDefinition.ID_MAX_LENGTH; 037 038/** 039 * A view for a Work Chunk that contains only the most necessary information 040 * to satisfy the no-data path. 041 */ 042@Entity 043@Immutable 044@Subselect("SELECT e.id as id, " 045 + " e.seq as seq," 046 + " e.stat as state, " 047 + " e.instance_id as instance_id, " 048 + " e.definition_id as job_definition_id, " 049 + " e.definition_ver as job_definition_version, " 050 + " e.tgt_step_id as target_step_id " 051 + "FROM BT2_WORK_CHUNK e") 052public class Batch2WorkChunkMetadataView implements Serializable { 053 054 @Id 055 @Column(name = "ID", length = ID_MAX_LENGTH) 056 private String myId; 057 058 @Column(name = "SEQ", nullable = false) 059 private int mySequence; 060 061 @Column(name = "STATE", length = ID_MAX_LENGTH, nullable = false) 062 @Enumerated(EnumType.STRING) 063 @JdbcTypeCode(SqlTypes.VARCHAR) 064 private WorkChunkStatusEnum myStatus; 065 066 @Column(name = "INSTANCE_ID", length = ID_MAX_LENGTH, nullable = false) 067 private String myInstanceId; 068 069 @Column(name = "JOB_DEFINITION_ID", length = ID_MAX_LENGTH, nullable = false) 070 private String myJobDefinitionId; 071 072 @Column(name = "JOB_DEFINITION_VERSION", nullable = false) 073 private int myJobDefinitionVersion; 074 075 @Column(name = "TARGET_STEP_ID", length = ID_MAX_LENGTH, nullable = false) 076 private String myTargetStepId; 077 078 public String getId() { 079 return myId; 080 } 081 082 public void setId(String theId) { 083 myId = theId; 084 } 085 086 public int getSequence() { 087 return mySequence; 088 } 089 090 public void setSequence(int theSequence) { 091 mySequence = theSequence; 092 } 093 094 public WorkChunkStatusEnum getStatus() { 095 return myStatus; 096 } 097 098 public void setStatus(WorkChunkStatusEnum theStatus) { 099 myStatus = theStatus; 100 } 101 102 public String getInstanceId() { 103 return myInstanceId; 104 } 105 106 public void setInstanceId(String theInstanceId) { 107 myInstanceId = theInstanceId; 108 } 109 110 public String getJobDefinitionId() { 111 return myJobDefinitionId; 112 } 113 114 public void setJobDefinitionId(String theJobDefinitionId) { 115 myJobDefinitionId = theJobDefinitionId; 116 } 117 118 public int getJobDefinitionVersion() { 119 return myJobDefinitionVersion; 120 } 121 122 public void setJobDefinitionVersion(int theJobDefinitionVersion) { 123 myJobDefinitionVersion = theJobDefinitionVersion; 124 } 125 126 public String getTargetStepId() { 127 return myTargetStepId; 128 } 129 130 public void setTargetStepId(String theTargetStepId) { 131 myTargetStepId = theTargetStepId; 132 } 133 134 public WorkChunkMetadata toChunkMetadata() { 135 WorkChunkMetadata metadata = new WorkChunkMetadata(); 136 metadata.setId(getId()); 137 metadata.setInstanceId(getInstanceId()); 138 metadata.setSequence(getSequence()); 139 metadata.setStatus(getStatus()); 140 metadata.setJobDefinitionId(getJobDefinitionId()); 141 metadata.setJobDefinitionVersion(getJobDefinitionVersion()); 142 metadata.setTargetStepId(getTargetStepId()); 143 return metadata; 144 } 145}