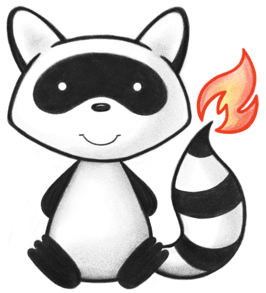
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import ca.uhn.fhir.jpa.model.entity.ResourceTable; 023import jakarta.persistence.Column; 024import jakarta.persistence.Entity; 025import jakarta.persistence.FetchType; 026import jakarta.persistence.ForeignKey; 027import jakarta.persistence.GeneratedValue; 028import jakarta.persistence.GenerationType; 029import jakarta.persistence.Id; 030import jakarta.persistence.JoinColumn; 031import jakarta.persistence.ManyToOne; 032import jakarta.persistence.OneToMany; 033import jakarta.persistence.SequenceGenerator; 034import jakarta.persistence.Table; 035import jakarta.persistence.Version; 036 037import java.io.Serializable; 038import java.util.ArrayList; 039import java.util.Collection; 040 041/* 042 * These classes are no longer needed. 043 * Metadata on the job is contained in the job itself 044 * (no separate storage required). 045 * 046 * See the BulkExportAppCtx for job details 047 */ 048@Entity 049@Table(name = "HFJ_BLK_EXPORT_COLLECTION") 050@Deprecated 051public class BulkExportCollectionEntity implements Serializable { 052 053 @Id 054 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_BLKEXCOL_PID") 055 @SequenceGenerator(name = "SEQ_BLKEXCOL_PID", sequenceName = "SEQ_BLKEXCOL_PID") 056 @Column(name = "PID") 057 private Long myId; 058 059 @ManyToOne() 060 @JoinColumn( 061 name = "JOB_PID", 062 referencedColumnName = "PID", 063 nullable = false, 064 foreignKey = @ForeignKey(name = "FK_BLKEXCOL_JOB")) 065 private BulkExportJobEntity myJob; 066 067 @Column(name = "RES_TYPE", length = ResourceTable.RESTYPE_LEN, nullable = false) 068 private String myResourceType; 069 070 @Column(name = "TYPE_FILTER", length = 1000, nullable = true) 071 private String myFilter; 072 073 @Version 074 @Column(name = "OPTLOCK", nullable = false) 075 private int myVersion; 076 077 @OneToMany(fetch = FetchType.LAZY, mappedBy = "myCollection") 078 private Collection<BulkExportCollectionFileEntity> myFiles; 079 080 public void setJob(BulkExportJobEntity theJob) { 081 myJob = theJob; 082 } 083 084 public String getResourceType() { 085 return myResourceType; 086 } 087 088 public void setResourceType(String theResourceType) { 089 myResourceType = theResourceType; 090 } 091 092 public String getFilter() { 093 return myFilter; 094 } 095 096 public void setFilter(String theFilter) { 097 myFilter = theFilter; 098 } 099 100 public int getVersion() { 101 return myVersion; 102 } 103 104 public void setVersion(int theVersion) { 105 myVersion = theVersion; 106 } 107 108 public Collection<BulkExportCollectionFileEntity> getFiles() { 109 if (myFiles == null) { 110 myFiles = new ArrayList<>(); 111 } 112 return myFiles; 113 } 114 115 public Long getId() { 116 return myId; 117 } 118}