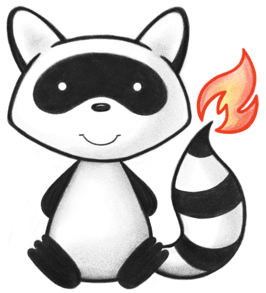
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.FetchType; 025import jakarta.persistence.GeneratedValue; 026import jakarta.persistence.GenerationType; 027import jakarta.persistence.Id; 028import jakarta.persistence.Index; 029import jakarta.persistence.OneToMany; 030import jakarta.persistence.SequenceGenerator; 031import jakarta.persistence.Table; 032import jakarta.persistence.Temporal; 033import jakarta.persistence.TemporalType; 034import jakarta.persistence.UniqueConstraint; 035import jakarta.persistence.Version; 036import org.apache.commons.lang3.builder.ToStringBuilder; 037import org.apache.commons.lang3.builder.ToStringStyle; 038import org.hl7.fhir.r5.model.InstantType; 039 040import java.io.Serializable; 041import java.util.ArrayList; 042import java.util.Collection; 043import java.util.Date; 044 045import static ca.uhn.fhir.rest.api.Constants.UUID_LENGTH; 046import static org.apache.commons.lang3.StringUtils.isNotBlank; 047import static org.apache.commons.lang3.StringUtils.left; 048 049/* 050 * These classes are no longer needed. 051 * Metadata on the job is contained in the job itself 052 * (no separate storage required). 053 * 054 * See the BulkExportAppCtx for job details 055 */ 056@Entity 057@Table( 058 name = BulkExportJobEntity.HFJ_BLK_EXPORT_JOB, 059 uniqueConstraints = {@UniqueConstraint(name = "IDX_BLKEX_JOB_ID", columnNames = "JOB_ID")}, 060 indexes = {@Index(name = "IDX_BLKEX_EXPTIME", columnList = "EXP_TIME")}) 061@Deprecated 062public class BulkExportJobEntity implements Serializable { 063 064 public static final int REQUEST_LENGTH = 1024; 065 public static final int STATUS_MESSAGE_LEN = 500; 066 public static final String JOB_ID = "JOB_ID"; 067 public static final String HFJ_BLK_EXPORT_JOB = "HFJ_BLK_EXPORT_JOB"; 068 069 @Id 070 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_BLKEXJOB_PID") 071 @SequenceGenerator(name = "SEQ_BLKEXJOB_PID", sequenceName = "SEQ_BLKEXJOB_PID") 072 @Column(name = "PID") 073 private Long myId; 074 075 @Column(name = JOB_ID, length = UUID_LENGTH, nullable = false) 076 private String myJobId; 077 078 @Column(name = "JOB_STATUS", length = 10, nullable = false) 079 private String myStatus; 080 081 @Temporal(TemporalType.TIMESTAMP) 082 @Column(name = "CREATED_TIME", nullable = false) 083 private Date myCreated; 084 085 @Temporal(TemporalType.TIMESTAMP) 086 @Column(name = "STATUS_TIME", nullable = false) 087 private Date myStatusTime; 088 089 @Temporal(TemporalType.TIMESTAMP) 090 @Column(name = "EXP_TIME", nullable = true) 091 private Date myExpiry; 092 093 @Column(name = "REQUEST", nullable = false, length = REQUEST_LENGTH) 094 private String myRequest; 095 096 @OneToMany(fetch = FetchType.LAZY, mappedBy = "myJob", orphanRemoval = false) 097 private Collection<BulkExportCollectionEntity> myCollections; 098 099 @Version 100 @Column(name = "OPTLOCK", nullable = false) 101 private int myVersion; 102 103 @Temporal(TemporalType.TIMESTAMP) 104 @Column(name = "EXP_SINCE", nullable = true) 105 private Date mySince; 106 107 @Column(name = "STATUS_MESSAGE", nullable = true, length = STATUS_MESSAGE_LEN) 108 private String myStatusMessage; 109 110 public Date getCreated() { 111 return myCreated; 112 } 113 114 public void setCreated(Date theCreated) { 115 myCreated = theCreated; 116 } 117 118 public String getStatusMessage() { 119 return myStatusMessage; 120 } 121 122 public void setStatusMessage(String theStatusMessage) { 123 myStatusMessage = left(theStatusMessage, STATUS_MESSAGE_LEN); 124 } 125 126 public String getRequest() { 127 return myRequest; 128 } 129 130 public void setRequest(String theRequest) { 131 myRequest = theRequest; 132 } 133 134 public void setExpiry(Date theExpiry) { 135 myExpiry = theExpiry; 136 } 137 138 public Collection<BulkExportCollectionEntity> getCollections() { 139 if (myCollections == null) { 140 myCollections = new ArrayList<>(); 141 } 142 return myCollections; 143 } 144 145 public String getJobId() { 146 return myJobId; 147 } 148 149 public void setJobId(String theJobId) { 150 myJobId = theJobId; 151 } 152 153 @Override 154 public String toString() { 155 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 156 b.append("pid", myId); 157 if (isNotBlank(myJobId)) { 158 b.append("jobId", myJobId); 159 } 160 if (myStatus != null) { 161 b.append("status", myStatus + " " + new InstantType(myStatusTime).getValueAsString()); 162 } 163 b.append("created", new InstantType(myCreated).getValueAsString()); 164 b.append("expiry", new InstantType(myExpiry).getValueAsString()); 165 b.append("request", myRequest); 166 b.append("since", mySince); 167 if (isNotBlank(myStatusMessage)) { 168 b.append("statusMessage", myStatusMessage); 169 } 170 return b.toString(); 171 } 172 173 public String getStatus() { 174 return myStatus; 175 } 176 177 public void setStatus(String theStatus) { 178 if (myStatus != theStatus) { 179 myStatusTime = new Date(); 180 myStatus = theStatus; 181 } 182 } 183 184 public Date getStatusTime() { 185 return myStatusTime; 186 } 187 188 public int getVersion() { 189 return myVersion; 190 } 191 192 public void setVersion(int theVersion) { 193 myVersion = theVersion; 194 } 195 196 public Long getId() { 197 return myId; 198 } 199 200 public Date getSince() { 201 if (mySince != null) { 202 return new Date(mySince.getTime()); 203 } 204 return null; 205 } 206 207 public void setSince(Date theSince) { 208 mySince = theSince; 209 } 210}