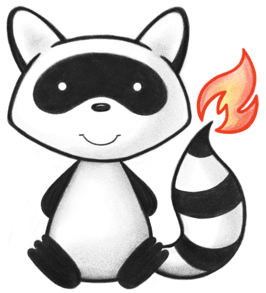
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobJson; 023import ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobStatusEnum; 024import ca.uhn.fhir.jpa.bulk.imprt.model.JobFileRowProcessingModeEnum; 025import jakarta.persistence.Column; 026import jakarta.persistence.Entity; 027import jakarta.persistence.EnumType; 028import jakarta.persistence.Enumerated; 029import jakarta.persistence.GeneratedValue; 030import jakarta.persistence.GenerationType; 031import jakarta.persistence.Id; 032import jakarta.persistence.SequenceGenerator; 033import jakarta.persistence.Table; 034import jakarta.persistence.Temporal; 035import jakarta.persistence.TemporalType; 036import jakarta.persistence.UniqueConstraint; 037import jakarta.persistence.Version; 038import org.hibernate.annotations.JdbcTypeCode; 039import org.hibernate.type.SqlTypes; 040 041import java.io.Serializable; 042import java.util.Date; 043 044import static ca.uhn.fhir.rest.api.Constants.UUID_LENGTH; 045import static org.apache.commons.lang3.StringUtils.left; 046 047@Entity 048@Table( 049 name = BulkImportJobEntity.HFJ_BLK_IMPORT_JOB, 050 uniqueConstraints = {@UniqueConstraint(name = "IDX_BLKIM_JOB_ID", columnNames = "JOB_ID")}) 051public class BulkImportJobEntity implements Serializable { 052 053 public static final String HFJ_BLK_IMPORT_JOB = "HFJ_BLK_IMPORT_JOB"; 054 public static final String JOB_ID = "JOB_ID"; 055 056 @Id 057 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_BLKIMJOB_PID") 058 @SequenceGenerator(name = "SEQ_BLKIMJOB_PID", sequenceName = "SEQ_BLKIMJOB_PID") 059 @Column(name = "PID") 060 private Long myId; 061 062 @Column(name = JOB_ID, length = UUID_LENGTH, nullable = false, updatable = false) 063 private String myJobId; 064 065 @Column(name = "JOB_DESC", nullable = true, length = BulkExportJobEntity.STATUS_MESSAGE_LEN) 066 private String myJobDescription; 067 068 @Enumerated(EnumType.STRING) 069 @JdbcTypeCode(SqlTypes.VARCHAR) 070 @Column(name = "JOB_STATUS", length = 10, nullable = false) 071 private BulkImportJobStatusEnum myStatus; 072 073 @Version 074 @Column(name = "OPTLOCK", nullable = false) 075 private int myVersion; 076 077 @Column(name = "FILE_COUNT", nullable = false) 078 private int myFileCount; 079 080 @Temporal(TemporalType.TIMESTAMP) 081 @Column(name = "STATUS_TIME", nullable = false) 082 private Date myStatusTime; 083 084 @Column(name = "STATUS_MESSAGE", nullable = true, length = BulkExportJobEntity.STATUS_MESSAGE_LEN) 085 private String myStatusMessage; 086 087 @Column(name = "ROW_PROCESSING_MODE", length = 20, nullable = false, updatable = false) 088 @JdbcTypeCode(SqlTypes.VARCHAR) 089 @Enumerated(EnumType.STRING) 090 private JobFileRowProcessingModeEnum myRowProcessingMode; 091 092 @Column(name = "BATCH_SIZE", nullable = false, updatable = false) 093 private int myBatchSize; 094 095 public String getJobDescription() { 096 return myJobDescription; 097 } 098 099 public void setJobDescription(String theJobDescription) { 100 myJobDescription = left(theJobDescription, BulkExportJobEntity.STATUS_MESSAGE_LEN); 101 } 102 103 public JobFileRowProcessingModeEnum getRowProcessingMode() { 104 return myRowProcessingMode; 105 } 106 107 public void setRowProcessingMode(JobFileRowProcessingModeEnum theRowProcessingMode) { 108 myRowProcessingMode = theRowProcessingMode; 109 } 110 111 public Date getStatusTime() { 112 return myStatusTime; 113 } 114 115 public void setStatusTime(Date theStatusTime) { 116 myStatusTime = theStatusTime; 117 } 118 119 public int getFileCount() { 120 return myFileCount; 121 } 122 123 public void setFileCount(int theFileCount) { 124 myFileCount = theFileCount; 125 } 126 127 public String getJobId() { 128 return myJobId; 129 } 130 131 public void setJobId(String theJobId) { 132 myJobId = theJobId; 133 } 134 135 public BulkImportJobStatusEnum getStatus() { 136 return myStatus; 137 } 138 139 /** 140 * Sets the status, updates the status time, and clears the status message 141 */ 142 public void setStatus(BulkImportJobStatusEnum theStatus) { 143 if (myStatus != theStatus) { 144 myStatus = theStatus; 145 setStatusTime(new Date()); 146 setStatusMessage(null); 147 } 148 } 149 150 public String getStatusMessage() { 151 return myStatusMessage; 152 } 153 154 public void setStatusMessage(String theStatusMessage) { 155 myStatusMessage = left(theStatusMessage, BulkExportJobEntity.STATUS_MESSAGE_LEN); 156 } 157 158 public BulkImportJobJson toJson() { 159 return new BulkImportJobJson() 160 .setProcessingMode(getRowProcessingMode()) 161 .setFileCount(getFileCount()) 162 .setJobDescription(getJobDescription()); 163 } 164 165 public int getBatchSize() { 166 return myBatchSize; 167 } 168 169 public void setBatchSize(int theBatchSize) { 170 myBatchSize = theBatchSize; 171 } 172}