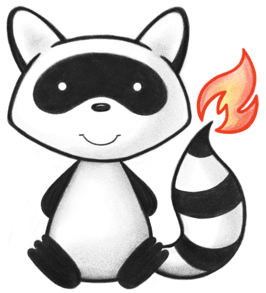
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobFileJson; 023import jakarta.persistence.Column; 024import jakarta.persistence.Entity; 025import jakarta.persistence.ForeignKey; 026import jakarta.persistence.GeneratedValue; 027import jakarta.persistence.GenerationType; 028import jakarta.persistence.Id; 029import jakarta.persistence.Index; 030import jakarta.persistence.JoinColumn; 031import jakarta.persistence.Lob; 032import jakarta.persistence.ManyToOne; 033import jakarta.persistence.SequenceGenerator; 034import jakarta.persistence.Table; 035import org.hibernate.Length; 036 037import java.io.Serializable; 038import java.nio.charset.StandardCharsets; 039 040import static org.apache.commons.lang3.StringUtils.left; 041 042@Entity 043@Table( 044 name = "HFJ_BLK_IMPORT_JOBFILE", 045 indexes = {@Index(name = "IDX_BLKIM_JOBFILE_JOBID", columnList = "JOB_PID")}) 046public class BulkImportJobFileEntity implements Serializable { 047 048 public static final int MAX_DESCRIPTION_LENGTH = 500; 049 050 @Id 051 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_BLKIMJOBFILE_PID") 052 @SequenceGenerator(name = "SEQ_BLKIMJOBFILE_PID", sequenceName = "SEQ_BLKIMJOBFILE_PID") 053 @Column(name = "PID") 054 private Long myId; 055 056 @ManyToOne 057 @JoinColumn( 058 name = "JOB_PID", 059 referencedColumnName = "PID", 060 nullable = false, 061 foreignKey = @ForeignKey(name = "FK_BLKIMJOBFILE_JOB")) 062 private BulkImportJobEntity myJob; 063 064 @Column(name = "FILE_SEQ", nullable = false) 065 private int myFileSequence; 066 067 @Column(name = "FILE_DESCRIPTION", nullable = true, length = MAX_DESCRIPTION_LENGTH) 068 private String myFileDescription; 069 070 @Lob // TODO: VC column added in 7.2.0 - Remove non-VC column later 071 @Column(name = "JOB_CONTENTS", nullable = true) 072 private byte[] myContents; 073 074 @Column(name = "JOB_CONTENTS_VC", nullable = true, length = Length.LONG32) 075 private String myContentsVc; 076 077 @Column(name = "TENANT_NAME", nullable = true, length = PartitionEntity.MAX_NAME_LENGTH) 078 private String myTenantName; 079 080 public String getFileDescription() { 081 return myFileDescription; 082 } 083 084 public void setFileDescription(String theFileDescription) { 085 myFileDescription = left(theFileDescription, MAX_DESCRIPTION_LENGTH); 086 } 087 088 public BulkImportJobEntity getJob() { 089 return myJob; 090 } 091 092 public void setJob(BulkImportJobEntity theJob) { 093 myJob = theJob; 094 } 095 096 public int getFileSequence() { 097 return myFileSequence; 098 } 099 100 public void setFileSequence(int theFileSequence) { 101 myFileSequence = theFileSequence; 102 } 103 104 public String getContents() { 105 if (myContentsVc != null) { 106 return myContentsVc; 107 } else { 108 return new String(myContents, StandardCharsets.UTF_8); 109 } 110 } 111 112 public void setContents(String theContents) { 113 myContentsVc = theContents; 114 myContents = null; 115 } 116 117 public BulkImportJobFileJson toJson() { 118 return new BulkImportJobFileJson().setContents(getContents()).setTenantName(getTenantName()); 119 } 120 121 public String getTenantName() { 122 return myTenantName; 123 } 124 125 public void setTenantName(String theTenantName) { 126 myTenantName = theTenantName; 127 } 128}