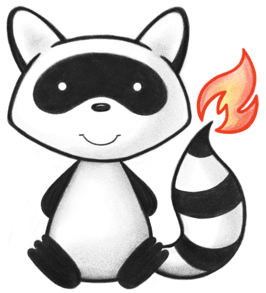
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.GeneratedValue; 025import jakarta.persistence.GenerationType; 026import jakarta.persistence.Id; 027import jakarta.persistence.SequenceGenerator; 028import jakarta.persistence.Table; 029import org.apache.commons.lang3.builder.ToStringBuilder; 030import org.hibernate.envers.RevisionEntity; 031import org.hibernate.envers.RevisionNumber; 032import org.hibernate.envers.RevisionTimestamp; 033 034import java.io.Serializable; 035import java.util.Date; 036 037/** 038 * This class exists strictly to override the default names used to generate Hibernate Envers revision table. 039 * <p> 040 * It is not actually invoked by any hapi-fhir code or code that uses hapi-fhir. 041 * <p> 042 * Specificallyy (at this writing), the class overrides names for: 043 * 044 * <ol> 045 * <li>The table name itself</li> 046 * <li>The ID generator sequence</li> 047 * </ol> 048 * 049 */ 050@Entity 051@RevisionEntity 052@Table(name = "HFJ_REVINFO") 053public class HapiFhirEnversRevision implements Serializable { 054 055 private static final long serialVersionUID = 1L; 056 057 @Id 058 @SequenceGenerator(name = "SEQ_HFJ_REVINFO", sequenceName = "SEQ_HFJ_REVINFO") 059 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_HFJ_REVINFO") 060 @RevisionNumber 061 @Column(name = "REV", nullable = false) 062 private long myRev; 063 064 @RevisionTimestamp 065 @Column(name = "REVTSTMP") 066 private Date myRevtstmp; 067 068 public long getRev() { 069 return myRev; 070 } 071 072 public void setRev(long theRev) { 073 myRev = theRev; 074 } 075 076 public Date getRevtstmp() { 077 return myRevtstmp; 078 } 079 080 public void setRevtstmp(Date theRevtstmp) { 081 myRevtstmp = theRevtstmp; 082 } 083 084 @Override 085 public String toString() { 086 return new ToStringBuilder(this) 087 .append("myRev", myRev) 088 .append("myRevtstmp", myRevtstmp) 089 .toString(); 090 } 091}