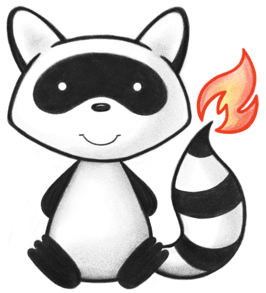
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import ca.uhn.fhir.interceptor.model.RequestPartitionId; 023import jakarta.persistence.Column; 024import jakarta.persistence.Entity; 025import jakarta.persistence.Id; 026import jakarta.persistence.Table; 027import jakarta.persistence.UniqueConstraint; 028 029import java.util.ArrayList; 030import java.util.List; 031 032@Entity 033@Table( 034 name = "HFJ_PARTITION", 035 uniqueConstraints = { 036 @UniqueConstraint( 037 name = "IDX_PART_NAME", 038 columnNames = {"PART_NAME"}) 039 }) 040public class PartitionEntity { 041 042 public static final int MAX_NAME_LENGTH = 200; 043 public static final int MAX_DESC_LENGTH = 200; 044 045 /** 046 * Note that unlike most PID columns in HAPI FHIR JPA, this one is an Integer, and isn't 047 * auto assigned. 048 */ 049 @Id 050 @Column(name = "PART_ID", nullable = false) 051 private Integer myId; 052 053 @Column(name = "PART_NAME", length = MAX_NAME_LENGTH, nullable = false) 054 private String myName; 055 056 @Column(name = "PART_DESC", length = MAX_DESC_LENGTH, nullable = true) 057 private String myDescription; 058 059 public Integer getId() { 060 return myId; 061 } 062 063 public PartitionEntity setId(Integer theId) { 064 myId = theId; 065 return this; 066 } 067 068 public String getName() { 069 return myName; 070 } 071 072 public PartitionEntity setName(String theName) { 073 myName = theName; 074 return this; 075 } 076 077 public String getDescription() { 078 return myDescription; 079 } 080 081 public void setDescription(String theDescription) { 082 myDescription = theDescription; 083 } 084 085 public RequestPartitionId toRequestPartitionId() { 086 return RequestPartitionId.fromPartitionIdAndName(getId(), getName()); 087 } 088 089 /** 090 * Build a RequestPartitionId from the ids and names in the entities. 091 * @param thePartitions the entities to use for ids and names 092 * @return a single RequestPartitionId covering all the entities 093 */ 094 public static RequestPartitionId buildRequestPartitionId(List<PartitionEntity> thePartitions) { 095 List<Integer> ids = new ArrayList<>(thePartitions.size()); 096 List<String> names = new ArrayList<>(thePartitions.size()); 097 for (PartitionEntity nextPartition : thePartitions) { 098 ids.add(nextPartition.getId()); 099 names.add(nextPartition.getName()); 100 } 101 102 return RequestPartitionId.forPartitionIdsAndNames(names, ids, null); 103 } 104}