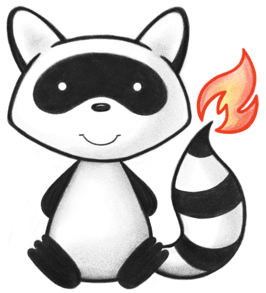
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import ca.uhn.fhir.rest.api.Constants; 023import com.google.common.annotations.VisibleForTesting; 024import jakarta.persistence.Column; 025import jakarta.persistence.Entity; 026import jakarta.persistence.GeneratedValue; 027import jakarta.persistence.GenerationType; 028import jakarta.persistence.Id; 029import jakarta.persistence.SequenceGenerator; 030import jakarta.persistence.Table; 031import jakarta.persistence.Temporal; 032import jakarta.persistence.TemporalType; 033import org.apache.commons.lang3.builder.ToStringBuilder; 034import org.apache.commons.lang3.builder.ToStringStyle; 035import org.hl7.fhir.r4.model.InstantType; 036 037import java.io.Serializable; 038import java.util.Date; 039 040/** 041 * @deprecated use new batch2 reindex job 042 */ 043@Deprecated 044@Entity 045@Table(name = "HFJ_RES_REINDEX_JOB") 046public class ResourceReindexJobEntity implements Serializable { 047 @Id 048 @SequenceGenerator(name = "SEQ_RES_REINDEX_JOB", sequenceName = "SEQ_RES_REINDEX_JOB") 049 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_RES_REINDEX_JOB") 050 @Column(name = "PID") 051 private Long myId; 052 053 @Column(name = "RES_TYPE", nullable = true, length = Constants.MAX_RESOURCE_NAME_LENGTH) 054 private String myResourceType; 055 /** 056 * Inclusive 057 */ 058 @Column(name = "UPDATE_THRESHOLD_HIGH", nullable = false) 059 @Temporal(TemporalType.TIMESTAMP) 060 private Date myThresholdHigh; 061 062 @Column(name = "JOB_DELETED", nullable = false) 063 private boolean myDeleted; 064 /** 065 * Inclusive 066 */ 067 @Column(name = "UPDATE_THRESHOLD_LOW", nullable = true) 068 @Temporal(TemporalType.TIMESTAMP) 069 private Date myThresholdLow; 070 071 @Column(name = "SUSPENDED_UNTIL", nullable = true) 072 @Temporal(TemporalType.TIMESTAMP) 073 private Date mySuspendedUntil; 074 075 @Column(name = "REINDEX_COUNT", nullable = true) 076 private Integer myReindexCount; 077 078 public Integer getReindexCount() { 079 return myReindexCount; 080 } 081 082 public void setReindexCount(Integer theReindexCount) { 083 myReindexCount = theReindexCount; 084 } 085 086 public Date getSuspendedUntil() { 087 return mySuspendedUntil; 088 } 089 090 public void setSuspendedUntil(Date theSuspendedUntil) { 091 mySuspendedUntil = theSuspendedUntil; 092 } 093 094 /** 095 * Inclusive 096 */ 097 public Date getThresholdLow() { 098 Date retVal = myThresholdLow; 099 if (retVal != null) { 100 retVal = new Date(retVal.getTime()); 101 } 102 return retVal; 103 } 104 105 /** 106 * Inclusive 107 */ 108 public void setThresholdLow(Date theThresholdLow) { 109 myThresholdLow = theThresholdLow; 110 } 111 112 public String getResourceType() { 113 return myResourceType; 114 } 115 116 public void setResourceType(String theResourceType) { 117 myResourceType = theResourceType; 118 } 119 120 /** 121 * Inclusive 122 */ 123 public Date getThresholdHigh() { 124 Date retVal = myThresholdHigh; 125 if (retVal != null) { 126 retVal = new Date(retVal.getTime()); 127 } 128 return retVal; 129 } 130 131 /** 132 * Inclusive 133 */ 134 public void setThresholdHigh(Date theThresholdHigh) { 135 myThresholdHigh = theThresholdHigh; 136 } 137 138 public Long getId() { 139 return myId; 140 } 141 142 @VisibleForTesting 143 public void setIdForUnitTest(long theId) { 144 myId = theId; 145 } 146 147 public void setDeleted(boolean theDeleted) { 148 myDeleted = theDeleted; 149 } 150 151 @Override 152 public String toString() { 153 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 154 .append("id", myId) 155 .append("resourceType", myResourceType) 156 .append("thresholdLow", new InstantType(myThresholdLow)) 157 .append("thresholdHigh", new InstantType(myThresholdHigh)); 158 if (myDeleted) { 159 b.append("deleted", myDeleted); 160 } 161 if (mySuspendedUntil != null) { 162 b.append("suspendedUntil", mySuspendedUntil); 163 } 164 return b.toString(); 165 } 166}