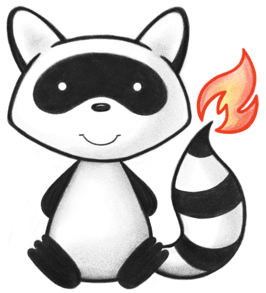
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.GeneratedValue; 025import jakarta.persistence.GenerationType; 026import jakarta.persistence.Id; 027import jakarta.persistence.SequenceGenerator; 028import jakarta.persistence.Table; 029import jakarta.persistence.UniqueConstraint; 030import org.apache.commons.lang3.Validate; 031import org.apache.commons.lang3.builder.ToStringBuilder; 032 033import java.io.Serializable; 034 035@Entity 036@Table( 037 name = "HFJ_SEARCH_RESULT", 038 uniqueConstraints = { 039 @UniqueConstraint( 040 name = "IDX_SEARCHRES_ORDER", 041 columnNames = {"SEARCH_PID", "SEARCH_ORDER"}) 042 }) 043public class SearchResult implements Serializable { 044 045 private static final long serialVersionUID = 1L; 046 047 @Deprecated(since = "6.10", forRemoval = true) // migrating to composite PK on searchPid,Order 048 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_SEARCH_RES") 049 @SequenceGenerator(name = "SEQ_SEARCH_RES", sequenceName = "SEQ_SEARCH_RES") 050 @Id 051 @Column(name = "PID") 052 private Long myId; 053 054 @Column(name = "SEARCH_PID", insertable = true, updatable = false, nullable = false) 055 private Long mySearchPid; 056 057 @Column(name = "SEARCH_ORDER", insertable = true, updatable = false, nullable = false) 058 private int myOrder; 059 060 @Column(name = "RESOURCE_PID", insertable = true, updatable = false, nullable = false) 061 private Long myResourcePid; 062 063 @Column(name = "RESOURCE_PARTITION_ID", insertable = true, updatable = false, nullable = true) 064 private Integer myResourcePartitionId; 065 066 /** 067 * Constructor 068 */ 069 public SearchResult() { 070 // nothing 071 } 072 073 /** 074 * Constructor 075 */ 076 public SearchResult(Search theSearch) { 077 Validate.notNull(theSearch.getId()); 078 mySearchPid = theSearch.getId(); 079 } 080 081 @Override 082 public String toString() { 083 return new ToStringBuilder(this) 084 .append("search", mySearchPid) 085 .append("order", myOrder) 086 .append("resourcePid", myResourcePid) 087 .append("resourcePartitionId", myResourcePartitionId) 088 .toString(); 089 } 090 091 @Override 092 public boolean equals(Object theObj) { 093 if (!(theObj instanceof SearchResult)) { 094 return false; 095 } 096 return myResourcePid.equals(((SearchResult) theObj).myResourcePid); 097 } 098 099 public int getOrder() { 100 return myOrder; 101 } 102 103 public void setOrder(int theOrder) { 104 myOrder = theOrder; 105 } 106 107 public Long getResourcePid() { 108 return myResourcePid; 109 } 110 111 public SearchResult setResourcePid(Long theResourcePid) { 112 myResourcePid = theResourcePid; 113 return this; 114 } 115 116 public Integer getResourcePartitionId() { 117 return myResourcePartitionId; 118 } 119 120 public void setResourcePartitionId(Integer theResourcePartitionId) { 121 myResourcePartitionId = theResourcePartitionId; 122 } 123 124 @Override 125 public int hashCode() { 126 return myResourcePid.hashCode(); 127 } 128}