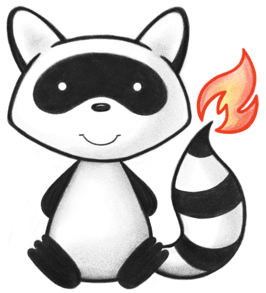
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.GeneratedValue; 025import jakarta.persistence.GenerationType; 026import jakarta.persistence.Id; 027import jakarta.persistence.SequenceGenerator; 028import jakarta.persistence.Table; 029import jakarta.persistence.Temporal; 030import jakarta.persistence.TemporalType; 031import jakarta.persistence.UniqueConstraint; 032 033import java.util.Date; 034 035@Entity 036@Table( 037 name = "HFJ_SUBSCRIPTION_STATS", 038 uniqueConstraints = { 039 @UniqueConstraint( 040 name = "IDX_SUBSC_RESID", 041 columnNames = {"RES_ID"}), 042 }) 043public class SubscriptionTable { 044 045 @Temporal(TemporalType.TIMESTAMP) 046 @Column(name = "CREATED_TIME", nullable = false, insertable = true, updatable = false) 047 private Date myCreated; 048 049 @Id 050 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_SUBSCRIPTION_ID") 051 @SequenceGenerator(name = "SEQ_SUBSCRIPTION_ID", sequenceName = "SEQ_SUBSCRIPTION_ID") 052 @Column(name = "PID", insertable = false, updatable = false) 053 private Long myId; 054 055 @Column(name = "RES_ID", nullable = true) 056 private Long myResId; 057 058 /** 059 * Constructor 060 */ 061 public SubscriptionTable() { 062 super(); 063 } 064 065 public Date getCreated() { 066 return myCreated; 067 } 068 069 public void setCreated(Date theCreated) { 070 myCreated = theCreated; 071 } 072 073 public Long getId() { 074 return myId; 075 } 076}