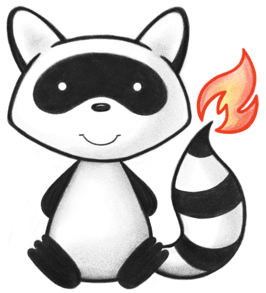
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import ca.uhn.fhir.jpa.model.entity.BasePartitionable; 023import ca.uhn.fhir.jpa.model.entity.IdAndPartitionId; 024import ca.uhn.fhir.util.ValidateUtil; 025import jakarta.annotation.Nonnull; 026import jakarta.persistence.Column; 027import jakarta.persistence.Entity; 028import jakarta.persistence.ForeignKey; 029import jakarta.persistence.GeneratedValue; 030import jakarta.persistence.GenerationType; 031import jakarta.persistence.Id; 032import jakarta.persistence.IdClass; 033import jakarta.persistence.Index; 034import jakarta.persistence.JoinColumn; 035import jakarta.persistence.JoinColumns; 036import jakarta.persistence.ManyToOne; 037import jakarta.persistence.OneToMany; 038import jakarta.persistence.SequenceGenerator; 039import jakarta.persistence.Table; 040import org.apache.commons.lang3.Validate; 041import org.apache.commons.lang3.builder.ToStringBuilder; 042import org.apache.commons.lang3.builder.ToStringStyle; 043 044import java.io.Serializable; 045import java.util.ArrayList; 046import java.util.List; 047 048import static org.apache.commons.lang3.StringUtils.length; 049 050@Entity 051@Table( 052 name = "TRM_CONCEPT_MAP_GROUP", 053 indexes = {@Index(name = "FK_TCMGROUP_CONCEPTMAP", columnList = "CONCEPT_MAP_PID")}) 054@IdClass(IdAndPartitionId.class) 055public class TermConceptMapGroup extends BasePartitionable implements Serializable { 056 private static final long serialVersionUID = 1L; 057 058 @Id() 059 @SequenceGenerator(name = "SEQ_CONCEPT_MAP_GROUP_PID", sequenceName = "SEQ_CONCEPT_MAP_GROUP_PID") 060 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_CONCEPT_MAP_GROUP_PID") 061 @Column(name = "PID") 062 private Long myId; 063 064 @ManyToOne() 065 @JoinColumns( 066 value = { 067 @JoinColumn( 068 name = "CONCEPT_MAP_PID", 069 insertable = false, 070 updatable = false, 071 nullable = false, 072 referencedColumnName = "PID"), 073 @JoinColumn( 074 name = "PARTITION_ID", 075 referencedColumnName = "PARTITION_ID", 076 insertable = false, 077 updatable = false, 078 nullable = false) 079 }, 080 foreignKey = @ForeignKey(name = "FK_TCMGROUP_CONCEPTMAP")) 081 private TermConceptMap myConceptMap; 082 083 @Column(name = "CONCEPT_MAP_PID", nullable = false) 084 private Long myConceptMapPid; 085 086 @Column(name = "SOURCE_URL", nullable = false, length = TermCodeSystem.MAX_URL_LENGTH) 087 private String mySource; 088 089 @Column(name = "SOURCE_VERSION", nullable = true, length = TermCodeSystemVersion.MAX_VERSION_LENGTH) 090 private String mySourceVersion; 091 092 @Column(name = "TARGET_URL", nullable = false, length = TermCodeSystem.MAX_URL_LENGTH) 093 private String myTarget; 094 095 @Column(name = "TARGET_VERSION", nullable = true, length = TermCodeSystemVersion.MAX_VERSION_LENGTH) 096 private String myTargetVersion; 097 098 @OneToMany(mappedBy = "myConceptMapGroup") 099 private List<TermConceptMapGroupElement> myConceptMapGroupElements; 100 101 @Column(name = "CONCEPT_MAP_URL", nullable = true, length = TermConceptMap.MAX_URL_LENGTH) 102 private String myConceptMapUrl; 103 104 @Column(name = "SOURCE_VS", nullable = true, length = TermValueSet.MAX_URL_LENGTH) 105 private String mySourceValueSet; 106 107 @Column(name = "TARGET_VS", nullable = true, length = TermValueSet.MAX_URL_LENGTH) 108 private String myTargetValueSet; 109 110 public TermConceptMap getConceptMap() { 111 return myConceptMap; 112 } 113 114 public TermConceptMapGroup setConceptMap(TermConceptMap theTermConceptMap) { 115 myConceptMap = theTermConceptMap; 116 myConceptMapPid = theTermConceptMap.getId(); 117 Validate.notNull(myConceptMapPid, "Concept map pid must not be null"); 118 setPartitionId(theTermConceptMap.getPartitionId()); 119 return this; 120 } 121 122 public List<TermConceptMapGroupElement> getConceptMapGroupElements() { 123 if (myConceptMapGroupElements == null) { 124 myConceptMapGroupElements = new ArrayList<>(); 125 } 126 127 return myConceptMapGroupElements; 128 } 129 130 public String getConceptMapUrl() { 131 if (myConceptMapUrl == null) { 132 myConceptMapUrl = getConceptMap().getUrl(); 133 } 134 return myConceptMapUrl; 135 } 136 137 public Long getId() { 138 return myId; 139 } 140 141 public String getSource() { 142 return mySource; 143 } 144 145 public TermConceptMapGroup setSource(@Nonnull String theSource) { 146 ValidateUtil.isNotBlankOrThrowIllegalArgument(theSource, "theSource must not be null or empty"); 147 ValidateUtil.isNotTooLongOrThrowIllegalArgument( 148 theSource, 149 TermCodeSystem.MAX_URL_LENGTH, 150 "Source exceeds maximum length (" + TermCodeSystem.MAX_URL_LENGTH + "): " + length(theSource)); 151 this.mySource = theSource; 152 return this; 153 } 154 155 public String getSourceValueSet() { 156 if (mySourceValueSet == null) { 157 mySourceValueSet = getConceptMap().getSource(); 158 } 159 return mySourceValueSet; 160 } 161 162 public String getSourceVersion() { 163 return mySourceVersion; 164 } 165 166 public TermConceptMapGroup setSourceVersion(String theSourceVersion) { 167 ValidateUtil.isNotTooLongOrThrowIllegalArgument( 168 theSourceVersion, 169 TermCodeSystemVersion.MAX_VERSION_LENGTH, 170 "Source version ID exceeds maximum length (" + TermCodeSystemVersion.MAX_VERSION_LENGTH + "): " 171 + length(theSourceVersion)); 172 mySourceVersion = theSourceVersion; 173 return this; 174 } 175 176 public String getTarget() { 177 return myTarget; 178 } 179 180 public TermConceptMapGroup setTarget(@Nonnull String theTarget) { 181 ValidateUtil.isNotBlankOrThrowIllegalArgument(theTarget, "theTarget must not be null or empty"); 182 ValidateUtil.isNotTooLongOrThrowIllegalArgument( 183 theTarget, 184 TermCodeSystem.MAX_URL_LENGTH, 185 "Target exceeds maximum length (" + TermCodeSystem.MAX_URL_LENGTH + "): " + length(theTarget)); 186 this.myTarget = theTarget; 187 return this; 188 } 189 190 public String getTargetValueSet() { 191 if (myTargetValueSet == null) { 192 myTargetValueSet = getConceptMap().getTarget(); 193 } 194 return myTargetValueSet; 195 } 196 197 public String getTargetVersion() { 198 return myTargetVersion; 199 } 200 201 public TermConceptMapGroup setTargetVersion(String theTargetVersion) { 202 ValidateUtil.isNotTooLongOrThrowIllegalArgument( 203 theTargetVersion, 204 TermCodeSystemVersion.MAX_VERSION_LENGTH, 205 "Target version ID exceeds maximum length (" + TermCodeSystemVersion.MAX_VERSION_LENGTH + "): " 206 + length(theTargetVersion)); 207 myTargetVersion = theTargetVersion; 208 return this; 209 } 210 211 @Override 212 public String toString() { 213 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 214 .append("myId", myId) 215 .append(myConceptMap != null ? ("myConceptMap - id=" + myConceptMap.getId()) : ("myConceptMap=(null)")) 216 .append("mySource", mySource) 217 .append("mySourceVersion", mySourceVersion) 218 .append("myTarget", myTarget) 219 .append("myTargetVersion", myTargetVersion) 220 .append( 221 myConceptMapGroupElements != null 222 ? ("myConceptMapGroupElements - size=" + myConceptMapGroupElements.size()) 223 : ("myConceptMapGroupElements=(null)")) 224 .append("myConceptMapUrl", this.getConceptMapUrl()) 225 .append("mySourceValueSet", this.getSourceValueSet()) 226 .append("myTargetValueSet", this.getTargetValueSet()) 227 .toString(); 228 } 229}