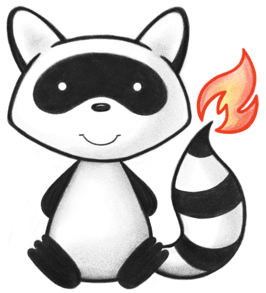
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import ca.uhn.fhir.util.ValidateUtil; 023import jakarta.annotation.Nonnull; 024import jakarta.persistence.Column; 025import jakarta.persistence.Entity; 026import jakarta.persistence.EnumType; 027import jakarta.persistence.Enumerated; 028import jakarta.persistence.ForeignKey; 029import jakarta.persistence.GeneratedValue; 030import jakarta.persistence.GenerationType; 031import jakarta.persistence.Id; 032import jakarta.persistence.Index; 033import jakarta.persistence.JoinColumn; 034import jakarta.persistence.ManyToOne; 035import jakarta.persistence.SequenceGenerator; 036import jakarta.persistence.Table; 037import org.apache.commons.lang3.builder.EqualsBuilder; 038import org.apache.commons.lang3.builder.HashCodeBuilder; 039import org.apache.commons.lang3.builder.ToStringBuilder; 040import org.apache.commons.lang3.builder.ToStringStyle; 041import org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence; 042 043import java.io.Serializable; 044 045import static org.apache.commons.lang3.StringUtils.length; 046 047@Entity 048@Table( 049 name = "TRM_CONCEPT_MAP_GRP_ELM_TGT", 050 indexes = { 051 @Index(name = "IDX_CNCPT_MP_GRP_ELM_TGT_CD", columnList = "TARGET_CODE"), 052 @Index(name = "FK_TCMGETARGET_ELEMENT", columnList = "CONCEPT_MAP_GRP_ELM_PID") 053 }) 054public class TermConceptMapGroupElementTarget implements Serializable { 055 private static final long serialVersionUID = 1L; 056 057 static final int MAX_EQUIVALENCE_LENGTH = 50; 058 059 @Id() 060 @SequenceGenerator(name = "SEQ_CNCPT_MAP_GRP_ELM_TGT_PID", sequenceName = "SEQ_CNCPT_MAP_GRP_ELM_TGT_PID") 061 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_CNCPT_MAP_GRP_ELM_TGT_PID") 062 @Column(name = "PID") 063 private Long myId; 064 065 @ManyToOne() 066 @JoinColumn( 067 name = "CONCEPT_MAP_GRP_ELM_PID", 068 nullable = false, 069 referencedColumnName = "PID", 070 foreignKey = @ForeignKey(name = "FK_TCMGETARGET_ELEMENT")) 071 private TermConceptMapGroupElement myConceptMapGroupElement; 072 073 @Column(name = "TARGET_CODE", nullable = true, length = TermConcept.MAX_CODE_LENGTH) 074 private String myCode; 075 076 @Column(name = "TARGET_DISPLAY", nullable = true, length = TermConcept.MAX_DISP_LENGTH) 077 private String myDisplay; 078 079 @Enumerated(EnumType.STRING) 080 @Column(name = "TARGET_EQUIVALENCE", nullable = true, length = MAX_EQUIVALENCE_LENGTH) 081 private ConceptMapEquivalence myEquivalence; 082 083 @Column(name = "CONCEPT_MAP_URL", nullable = true, length = TermConceptMap.MAX_URL_LENGTH) 084 private String myConceptMapUrl; 085 086 @Column(name = "SYSTEM_URL", nullable = true, length = TermCodeSystem.MAX_URL_LENGTH) 087 private String mySystem; 088 089 @Column(name = "SYSTEM_VERSION", nullable = true, length = TermCodeSystemVersion.MAX_VERSION_LENGTH) 090 private String mySystemVersion; 091 092 @Column(name = "VALUESET_URL", nullable = true, length = TermValueSet.MAX_URL_LENGTH) 093 private String myValueSet; 094 095 public String getCode() { 096 return myCode; 097 } 098 099 public TermConceptMapGroupElementTarget setCode(@Nonnull String theCode) { 100 ValidateUtil.isNotBlankOrThrowIllegalArgument(theCode, "theCode must not be null or empty"); 101 ValidateUtil.isNotTooLongOrThrowIllegalArgument( 102 theCode, 103 TermConcept.MAX_CODE_LENGTH, 104 "Code exceeds maximum length (" + TermConcept.MAX_CODE_LENGTH + "): " + length(theCode)); 105 myCode = theCode; 106 return this; 107 } 108 109 public TermConceptMapGroupElement getConceptMapGroupElement() { 110 return myConceptMapGroupElement; 111 } 112 113 public void setConceptMapGroupElement(TermConceptMapGroupElement theTermConceptMapGroupElement) { 114 myConceptMapGroupElement = theTermConceptMapGroupElement; 115 } 116 117 public String getConceptMapUrl() { 118 if (myConceptMapUrl == null) { 119 myConceptMapUrl = getConceptMapGroupElement() 120 .getConceptMapGroup() 121 .getConceptMap() 122 .getUrl(); 123 } 124 return myConceptMapUrl; 125 } 126 127 public String getDisplay() { 128 return myDisplay; 129 } 130 131 public TermConceptMapGroupElementTarget setDisplay(String theDisplay) { 132 myDisplay = theDisplay; 133 return this; 134 } 135 136 public ConceptMapEquivalence getEquivalence() { 137 return myEquivalence; 138 } 139 140 public TermConceptMapGroupElementTarget setEquivalence(ConceptMapEquivalence theEquivalence) { 141 myEquivalence = theEquivalence; 142 return this; 143 } 144 145 public Long getId() { 146 return myId; 147 } 148 149 public String getSystem() { 150 if (mySystem == null) { 151 mySystem = getConceptMapGroupElement().getConceptMapGroup().getTarget(); 152 } 153 return mySystem; 154 } 155 156 public String getSystemVersion() { 157 if (mySystemVersion == null) { 158 mySystemVersion = getConceptMapGroupElement().getConceptMapGroup().getTargetVersion(); 159 } 160 return mySystemVersion; 161 } 162 163 public String getValueSet() { 164 if (myValueSet == null) { 165 myValueSet = getConceptMapGroupElement().getConceptMapGroup().getTargetValueSet(); 166 } 167 return myValueSet; 168 } 169 170 @Override 171 public boolean equals(Object o) { 172 if (this == o) return true; 173 174 if (!(o instanceof TermConceptMapGroupElementTarget)) return false; 175 176 TermConceptMapGroupElementTarget that = (TermConceptMapGroupElementTarget) o; 177 178 return new EqualsBuilder() 179 .append(getCode(), that.getCode()) 180 .append(getEquivalence(), that.getEquivalence()) 181 .append(getSystem(), that.getSystem()) 182 .append(getSystemVersion(), that.getSystemVersion()) 183 .isEquals(); 184 } 185 186 @Override 187 public int hashCode() { 188 return new HashCodeBuilder(17, 37) 189 .append(getCode()) 190 .append(getEquivalence()) 191 .append(getSystem()) 192 .append(getSystemVersion()) 193 .toHashCode(); 194 } 195 196 @Override 197 public String toString() { 198 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 199 .append("myId", myId) 200 .append( 201 myConceptMapGroupElement != null 202 ? ("myConceptMapGroupElement - id=" + myConceptMapGroupElement.getId()) 203 : ("myConceptMapGroupElement=(null)")) 204 .append("myCode", myCode) 205 .append("myDisplay", myDisplay) 206 .append("myEquivalence", myEquivalence.toCode()) 207 .append("myConceptMapUrl", this.getConceptMapUrl()) 208 .append("mySystem", this.getSystem()) 209 .append("mySystemVersion", this.getSystemVersion()) 210 .append("myValueSet", this.getValueSet()) 211 .toString(); 212 } 213}