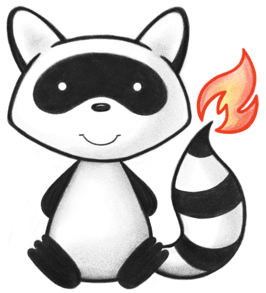
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.entity; 021 022import java.util.Collections; 023import java.util.HashMap; 024import java.util.Map; 025 026/** 027 * This enum is used to indicate the pre-expansion status of a given ValueSet in the terminology tables. In this context, 028 * an expanded ValueSet has its included concepts stored in the terminology tables as well. 029 */ 030public enum TermValueSetPreExpansionStatusEnum { 031 /* 032 * Sorting agnostic. 033 */ 034 035 NOT_EXPANDED("notExpanded", "The ValueSet is waiting to be picked up and pre-expanded by a scheduled task."), 036 EXPANSION_IN_PROGRESS( 037 "expansionInProgress", 038 "The ValueSet has been picked up by a scheduled task and pre-expansion is in progress."), 039 EXPANDED("expanded", "The ValueSet has been picked up by a scheduled task and pre-expansion is complete."), 040 FAILED_TO_EXPAND( 041 "failedToExpand", "The ValueSet has been picked up by a scheduled task and pre-expansion has failed."); 042 043 private static Map<String, TermValueSetPreExpansionStatusEnum> ourValues; 044 private String myCode; 045 private String myDescription; 046 047 TermValueSetPreExpansionStatusEnum(String theCode, String theDescription) { 048 myCode = theCode; 049 myDescription = theDescription; 050 } 051 052 public String getCode() { 053 return myCode; 054 } 055 056 public String getDescription() { 057 return myDescription; 058 } 059 060 public static TermValueSetPreExpansionStatusEnum fromCode(String theCode) { 061 if (ourValues == null) { 062 HashMap<String, TermValueSetPreExpansionStatusEnum> values = 063 new HashMap<String, TermValueSetPreExpansionStatusEnum>(); 064 for (TermValueSetPreExpansionStatusEnum next : values()) { 065 values.put(next.getCode(), next); 066 } 067 ourValues = Collections.unmodifiableMap(values); 068 } 069 return ourValues.get(theCode); 070 } 071 072 /** 073 * Convert from Enum ordinal to Enum type. 074 * 075 * Usage: 076 * 077 * <code>TermValueSetExpansionStatusEnum termValueSetExpansionStatusEnum = TermValueSetExpansionStatusEnum.values[ordinal];</code> 078 */ 079 public static final TermValueSetPreExpansionStatusEnum values[] = values(); 080}