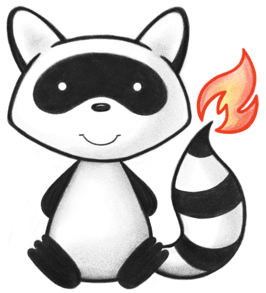
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.esr; 021 022import jakarta.annotation.Nonnull; 023import org.apache.commons.lang3.Validate; 024 025import java.util.HashMap; 026import java.util.Map; 027 028import static org.apache.commons.lang3.StringUtils.defaultString; 029 030public class ExternallyStoredResourceServiceRegistry { 031 032 private static final String VALID_ID_PATTERN = "[a-zA-Z0-9_.-]+"; 033 private final Map<String, IExternallyStoredResourceService> myIdToProvider = new HashMap<>(); 034 035 /** 036 * Registers a new provider. Do not call this method after the server has been started. 037 * 038 * @param theProvider The provider to register. 039 */ 040 public void registerProvider(@Nonnull IExternallyStoredResourceService theProvider) { 041 String id = defaultString(theProvider.getId()); 042 Validate.isTrue( 043 id.matches(VALID_ID_PATTERN), 044 "Invalid provider ID (must match pattern " + VALID_ID_PATTERN + "): %s", 045 id); 046 Validate.isTrue(!myIdToProvider.containsKey(id), "Already have a provider with ID: %s", id); 047 048 myIdToProvider.put(id, theProvider); 049 } 050 051 /** 052 * Do we have any providers registered? 053 */ 054 public boolean hasProviders() { 055 return !myIdToProvider.isEmpty(); 056 } 057 058 /** 059 * Clears all registered providers. This method is mostly intended for unit tests. 060 */ 061 public void clearProviders() { 062 myIdToProvider.clear(); 063 } 064 065 @Nonnull 066 public IExternallyStoredResourceService getProvider(@Nonnull String theProviderId) { 067 IExternallyStoredResourceService retVal = myIdToProvider.get(theProviderId); 068 Validate.notNull(retVal, "Invalid ESR provider ID: %s", theProviderId); 069 return retVal; 070 } 071}