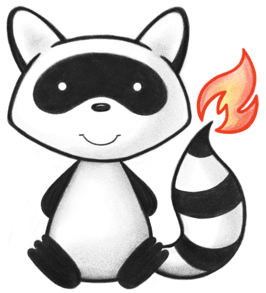
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.interceptor; 021 022import ca.uhn.fhir.jpa.dao.ISearchBuilder; 023import ca.uhn.fhir.jpa.model.dao.JpaPid; 024import ca.uhn.fhir.rest.api.server.IPreResourceAccessDetails; 025import ca.uhn.fhir.util.ICallable; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027 028import java.util.ArrayList; 029import java.util.Collections; 030import java.util.List; 031import javax.annotation.concurrent.NotThreadSafe; 032 033/** 034 * THIS CLASS IS NOT THREAD SAFE 035 */ 036@NotThreadSafe 037public class JpaPreResourceAccessDetails implements IPreResourceAccessDetails { 038 039 private final List<JpaPid> myResourcePids; 040 private final boolean[] myBlocked; 041 private final ICallable<ISearchBuilder> mySearchBuilderSupplier; 042 private List<IBaseResource> myResources; 043 044 public JpaPreResourceAccessDetails( 045 List<JpaPid> theResourcePids, ICallable<ISearchBuilder> theSearchBuilderSupplier) { 046 myResourcePids = theResourcePids; 047 myBlocked = new boolean[myResourcePids.size()]; 048 mySearchBuilderSupplier = theSearchBuilderSupplier; 049 } 050 051 @Override 052 public int size() { 053 return myResourcePids.size(); 054 } 055 056 @Override 057 public IBaseResource getResource(int theIndex) { 058 if (myResources == null) { 059 myResources = new ArrayList<>(myResourcePids.size()); 060 mySearchBuilderSupplier 061 .call() 062 .loadResourcesByPid(myResourcePids, Collections.emptySet(), myResources, false, null); 063 } 064 return myResources.get(theIndex); 065 } 066 067 @Override 068 public void setDontReturnResourceAtIndex(int theIndex) { 069 myBlocked[theIndex] = true; 070 } 071 072 public boolean isDontReturnResourceAtIndex(int theIndex) { 073 return myBlocked[theIndex]; 074 } 075}