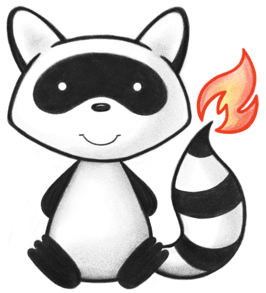
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.packages; 021 022import ca.uhn.fhir.context.FhirVersionEnum; 023import jakarta.annotation.Nullable; 024 025import java.util.Objects; 026import java.util.StringJoiner; 027 028/** 029 * Request parameters for {@link IHapiPackageCacheManager#findPackageAsset(FindPackageAssetRequest)} 030 */ 031public class FindPackageAssetRequest { 032 033 private final FhirVersionEnum myFhirVersion; 034 private final String myCanonicalUrl; 035 private final String myPackageId; 036 037 @Nullable 038 private final String myVersion; 039 040 public static FindPackageAssetRequest withVersion( 041 FhirVersionEnum theFhirVersion, String theCanonicalUrl, String thePackageId, String theVersion) { 042 return new FindPackageAssetRequest(theFhirVersion, theCanonicalUrl, thePackageId, theVersion); 043 } 044 045 public static FindPackageAssetRequest noVersion( 046 FhirVersionEnum theFhirVersion, String theCanonicalUrl, String thePackageId) { 047 return new FindPackageAssetRequest(theFhirVersion, theCanonicalUrl, thePackageId, null); 048 } 049 050 private FindPackageAssetRequest( 051 FhirVersionEnum myFhirVersion, String myCanonicalUrl, String myPackageId, @Nullable String myVersion) { 052 this.myFhirVersion = myFhirVersion; 053 this.myCanonicalUrl = myCanonicalUrl; 054 this.myPackageId = myPackageId; 055 this.myVersion = myVersion; 056 } 057 058 public FhirVersionEnum getFhirVersion() { 059 return myFhirVersion; 060 } 061 062 public String getCanonicalUrl() { 063 return myCanonicalUrl; 064 } 065 066 public String getPackageId() { 067 return myPackageId; 068 } 069 070 @Nullable 071 public String getVersion() { 072 return myVersion; 073 } 074 075 @Override 076 public boolean equals(Object object) { 077 if (object == null || getClass() != object.getClass()) { 078 return false; 079 } 080 FindPackageAssetRequest that = (FindPackageAssetRequest) object; 081 return myFhirVersion == that.myFhirVersion 082 && Objects.equals(myCanonicalUrl, that.myCanonicalUrl) 083 && Objects.equals(myPackageId, that.myPackageId) 084 && Objects.equals(myVersion, that.myVersion); 085 } 086 087 @Override 088 public int hashCode() { 089 return Objects.hash(myFhirVersion, myCanonicalUrl, myPackageId, myVersion); 090 } 091 092 @Override 093 public String toString() { 094 return new StringJoiner(", ", FindPackageAssetRequest.class.getSimpleName() + "[", "]") 095 .add("myFhirVersion=" + myFhirVersion) 096 .add("myCanonicalUrl='" + myCanonicalUrl + "'") 097 .add("myPackageId='" + myPackageId + "'") 098 .add("myVersion='" + myVersion + "'") 099 .toString(); 100 } 101}