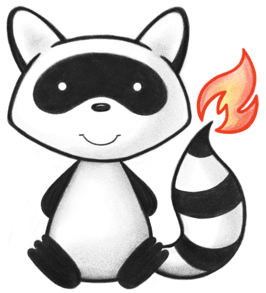
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.packages; 021 022import ca.uhn.fhir.context.FhirVersionEnum; 023import ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025import org.hl7.fhir.utilities.npm.IPackageCacheManager; 026import org.hl7.fhir.utilities.npm.NpmPackage; 027 028import java.io.IOException; 029import java.util.Date; 030import java.util.List; 031 032public interface IHapiPackageCacheManager extends IPackageCacheManager { 033 034 NpmPackage installPackage(PackageInstallationSpec theInstallationSpec) throws IOException; 035 036 IBaseResource loadPackageAssetByUrl(FhirVersionEnum theFhirVersion, String theCanonicalUrl); 037 038 List<NpmPackageAssetInfoJson> findPackageAssetInfoByUrl(FhirVersionEnum theFhirVersion, String theCanonicalUrl); 039 040 IBaseResource findPackageAsset(FindPackageAssetRequest theFindPackageAssetRequest); 041 042 NpmPackageMetadataJson loadPackageMetadata(String thePackageId) throws ResourceNotFoundException; 043 044 PackageContents loadPackageContents(String thePackageId, String theVersion); 045 046 NpmPackageSearchResultJson search(PackageSearchSpec thePackageSearchSpec); 047 048 PackageDeleteOutcomeJson uninstallPackage(String thePackageId, String theVersion); 049 050 List<IBaseResource> loadPackageAssetsByType(FhirVersionEnum theFhirVersion, String theResourceType); 051 052 class PackageContents { 053 054 private byte[] myBytes; 055 private String myPackageId; 056 private String myVersion; 057 private Date myLastModified; 058 059 /** 060 * Constructor 061 */ 062 public PackageContents() { 063 super(); 064 } 065 066 public byte[] getBytes() { 067 return myBytes; 068 } 069 070 public PackageContents setBytes(byte[] theBytes) { 071 myBytes = theBytes; 072 return this; 073 } 074 075 public String getPackageId() { 076 return myPackageId; 077 } 078 079 public PackageContents setPackageId(String thePackageId) { 080 myPackageId = thePackageId; 081 return this; 082 } 083 084 public String getVersion() { 085 return myVersion; 086 } 087 088 public PackageContents setVersion(String theVersion) { 089 myVersion = theVersion; 090 return this; 091 } 092 093 public Date getLastModified() { 094 return myLastModified; 095 } 096 097 public PackageContents setLastModified(Date theLastModified) { 098 myLastModified = theLastModified; 099 return this; 100 } 101 } 102}