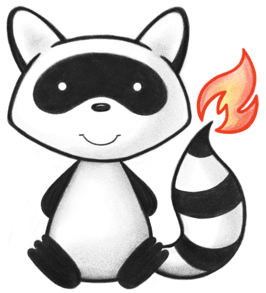
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.packages; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.FhirVersionEnum; 024import ca.uhn.fhir.context.support.IValidationSupport; 025import jakarta.annotation.Nullable; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027import org.springframework.beans.factory.annotation.Autowired; 028 029import java.util.List; 030 031public class NpmJpaValidationSupport implements IValidationSupport { 032 033 @Autowired 034 private FhirContext myFhirContext; 035 036 @Autowired 037 private IHapiPackageCacheManager myHapiPackageCacheManager; 038 039 @Override 040 public FhirContext getFhirContext() { 041 return myFhirContext; 042 } 043 044 @Override 045 public IBaseResource fetchValueSet(String theUri) { 046 return fetchResource("ValueSet", theUri); 047 } 048 049 @Override 050 public IBaseResource fetchCodeSystem(String theUri) { 051 return fetchResource("CodeSystem", theUri); 052 } 053 054 @Override 055 public IBaseResource fetchStructureDefinition(String theUri) { 056 return fetchResource("StructureDefinition", theUri); 057 } 058 059 @Nullable 060 public IBaseResource fetchResource(String theResourceType, String theUri) { 061 FhirVersionEnum fhirVersion = myFhirContext.getVersion().getVersion(); 062 IBaseResource asset = myHapiPackageCacheManager.loadPackageAssetByUrl(fhirVersion, theUri); 063 if (asset != null) { 064 Class<? extends IBaseResource> type = 065 myFhirContext.getResourceDefinition(theResourceType).getImplementingClass(); 066 if (type.isAssignableFrom(asset.getClass())) { 067 return asset; 068 } 069 } 070 return null; 071 } 072 073 @SuppressWarnings("unchecked") 074 @Nullable 075 @Override 076 public <T extends IBaseResource> List<T> fetchAllStructureDefinitions() { 077 FhirVersionEnum fhirVersion = myFhirContext.getVersion().getVersion(); 078 return (List<T>) myHapiPackageCacheManager.loadPackageAssetsByType(fhirVersion, "StructureDefinition"); 079 } 080}