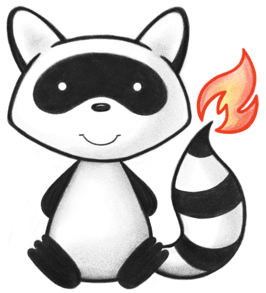
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.packages; 021 022import ca.uhn.fhir.rest.server.util.JsonDateDeserializer; 023import ca.uhn.fhir.rest.server.util.JsonDateSerializer; 024import com.fasterxml.jackson.annotation.JsonAutoDetect; 025import com.fasterxml.jackson.annotation.JsonInclude; 026import com.fasterxml.jackson.annotation.JsonProperty; 027import com.fasterxml.jackson.databind.annotation.JsonDeserialize; 028import com.fasterxml.jackson.databind.annotation.JsonSerialize; 029import io.swagger.v3.oas.annotations.media.Schema; 030import jakarta.annotation.Nonnull; 031 032import java.util.Date; 033import java.util.LinkedHashMap; 034import java.util.Map; 035 036@Schema(description = "Represents an NPM package metadata response") 037@JsonInclude(JsonInclude.Include.NON_NULL) 038@JsonAutoDetect( 039 creatorVisibility = JsonAutoDetect.Visibility.NONE, 040 fieldVisibility = JsonAutoDetect.Visibility.NONE, 041 getterVisibility = JsonAutoDetect.Visibility.NONE, 042 isGetterVisibility = JsonAutoDetect.Visibility.NONE, 043 setterVisibility = JsonAutoDetect.Visibility.NONE) 044public class NpmPackageMetadataJson { 045 046 @JsonProperty("dist-tags") 047 private DistTags myDistTags; 048 049 @JsonProperty("modified") 050 @JsonSerialize(using = JsonDateSerializer.class) 051 @JsonDeserialize(using = JsonDateDeserializer.class) 052 private Date myModified; 053 054 @JsonProperty("name") 055 private String myName; 056 057 @JsonProperty("versions") 058 private Map<String, Version> myVersionIdToVersion; 059 060 public void addVersion(Version theVersion) { 061 getVersions().put(theVersion.getVersion(), theVersion); 062 } 063 064 @Nonnull 065 public Map<String, Version> getVersions() { 066 if (myVersionIdToVersion == null) { 067 myVersionIdToVersion = new LinkedHashMap<>(); 068 } 069 return myVersionIdToVersion; 070 } 071 072 public DistTags getDistTags() { 073 return myDistTags; 074 } 075 076 public void setDistTags(DistTags theDistTags) { 077 myDistTags = theDistTags; 078 } 079 080 public void setModified(Date theModified) { 081 myModified = theModified; 082 } 083 084 public void setName(String theName) { 085 myName = theName; 086 } 087 088 public static class DistTags { 089 090 @JsonProperty("latest") 091 private String myLatest; 092 093 public String getLatest() { 094 return myLatest; 095 } 096 097 public DistTags setLatest(String theLatest) { 098 myLatest = theLatest; 099 return this; 100 } 101 } 102 103 @JsonInclude(JsonInclude.Include.NON_NULL) 104 @JsonAutoDetect( 105 creatorVisibility = JsonAutoDetect.Visibility.NONE, 106 fieldVisibility = JsonAutoDetect.Visibility.NONE, 107 getterVisibility = JsonAutoDetect.Visibility.NONE, 108 isGetterVisibility = JsonAutoDetect.Visibility.NONE, 109 setterVisibility = JsonAutoDetect.Visibility.NONE) 110 public static class Version { 111 112 @JsonProperty("name") 113 private String myName; 114 115 @JsonProperty("version") 116 private String myVersion; 117 118 @JsonProperty("author") 119 private String myAuthor; 120 121 @JsonProperty("description") 122 private String myDescription; 123 124 @JsonProperty("fhirVersion") 125 private String myFhirVersion; 126 127 @Schema(description = "The size of this package in bytes", example = "1000") 128 @JsonProperty("_bytes") 129 private long myBytes; 130 131 public String getAuthor() { 132 return myAuthor; 133 } 134 135 public void setAuthor(String theAuthor) { 136 myAuthor = theAuthor; 137 } 138 139 public String getName() { 140 return myName; 141 } 142 143 public void setName(String theName) { 144 myName = theName; 145 } 146 147 public String getDescription() { 148 return myDescription; 149 } 150 151 public void setDescription(String theDescription) { 152 myDescription = theDescription; 153 } 154 155 public String getFhirVersion() { 156 return myFhirVersion; 157 } 158 159 public void setFhirVersion(String theFhirVersion) { 160 myFhirVersion = theFhirVersion; 161 } 162 163 public String getVersion() { 164 return myVersion; 165 } 166 167 public void setVersion(String theVersion) { 168 myVersion = theVersion; 169 } 170 171 public long getBytes() { 172 return myBytes; 173 } 174 175 public void setBytes(long theBytes) { 176 myBytes = theBytes; 177 } 178 } 179}