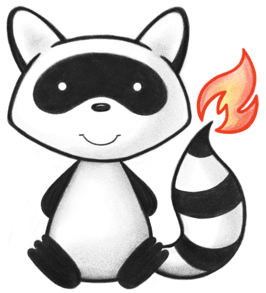
001/* 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.packages; 021 022import com.fasterxml.jackson.annotation.JsonAutoDetect; 023import com.fasterxml.jackson.annotation.JsonInclude; 024import com.fasterxml.jackson.annotation.JsonProperty; 025import io.swagger.v3.oas.annotations.media.Schema; 026 027import java.util.ArrayList; 028import java.util.List; 029 030@Schema(description = "Represents an NPM package search response") 031@JsonInclude(JsonInclude.Include.NON_NULL) 032@JsonAutoDetect( 033 creatorVisibility = JsonAutoDetect.Visibility.NONE, 034 fieldVisibility = JsonAutoDetect.Visibility.NONE, 035 getterVisibility = JsonAutoDetect.Visibility.NONE, 036 isGetterVisibility = JsonAutoDetect.Visibility.NONE, 037 setterVisibility = JsonAutoDetect.Visibility.NONE) 038public class NpmPackageSearchResultJson { 039 040 @JsonProperty("objects") 041 private List<ObjectElement> myObjects; 042 043 @JsonProperty("total") 044 private int myTotal; 045 046 public List<ObjectElement> getObjects() { 047 if (myObjects == null) { 048 myObjects = new ArrayList<>(); 049 } 050 return myObjects; 051 } 052 053 public ObjectElement addObject() { 054 ObjectElement object = new ObjectElement(); 055 getObjects().add(object); 056 return object; 057 } 058 059 public int getTotal() { 060 return myTotal; 061 } 062 063 public void setTotal(int theTotal) { 064 myTotal = theTotal; 065 } 066 067 public boolean hasPackageWithId(String thePackageId) { 068 return getObjects().stream().anyMatch(t -> t.getPackage().getName().equals(thePackageId)); 069 } 070 071 public Package getPackageWithId(String thePackageId) { 072 return getObjects().stream() 073 .map(t -> t.getPackage()) 074 .filter(t -> t.getName().equals(thePackageId)) 075 .findFirst() 076 .orElseThrow(() -> new IllegalArgumentException()); 077 } 078 079 @JsonInclude(JsonInclude.Include.NON_NULL) 080 @JsonAutoDetect( 081 creatorVisibility = JsonAutoDetect.Visibility.NONE, 082 fieldVisibility = JsonAutoDetect.Visibility.NONE, 083 getterVisibility = JsonAutoDetect.Visibility.NONE, 084 isGetterVisibility = JsonAutoDetect.Visibility.NONE, 085 setterVisibility = JsonAutoDetect.Visibility.NONE) 086 public static class ObjectElement { 087 088 @JsonProperty("package") 089 private Package myPackage; 090 091 public Package getPackage() { 092 if (myPackage == null) { 093 myPackage = new Package(); 094 } 095 return myPackage; 096 } 097 } 098 099 @JsonInclude(JsonInclude.Include.NON_NULL) 100 @JsonAutoDetect( 101 creatorVisibility = JsonAutoDetect.Visibility.NONE, 102 fieldVisibility = JsonAutoDetect.Visibility.NONE, 103 getterVisibility = JsonAutoDetect.Visibility.NONE, 104 isGetterVisibility = JsonAutoDetect.Visibility.NONE, 105 setterVisibility = JsonAutoDetect.Visibility.NONE) 106 public static class Package { 107 108 @JsonProperty("name") 109 private String myName; 110 111 @JsonProperty("version") 112 private String myVersion; 113 114 @JsonProperty("description") 115 private String myDescription; 116 117 @JsonProperty("fhirVersion") 118 private List<String> myFhirVersion; 119 120 @Schema(description = "The size of this package in bytes", example = "1000") 121 @JsonProperty("_bytes") 122 private long myBytes; 123 124 public long getBytes() { 125 return myBytes; 126 } 127 128 public Package setBytes(long theBytes) { 129 myBytes = theBytes; 130 return this; 131 } 132 133 public String getName() { 134 return myName; 135 } 136 137 public Package setName(String theName) { 138 myName = theName; 139 return this; 140 } 141 142 public String getDescription() { 143 return myDescription; 144 } 145 146 public Package setDescription(String theDescription) { 147 myDescription = theDescription; 148 return this; 149 } 150 151 public List<String> getFhirVersion() { 152 if (myFhirVersion == null) { 153 myFhirVersion = new ArrayList<>(); 154 } 155 return myFhirVersion; 156 } 157 158 public String getVersion() { 159 return myVersion; 160 } 161 162 public Package setVersion(String theVersion) { 163 myVersion = theVersion; 164 return this; 165 } 166 167 public Package addFhirVersion(String theFhirVersionId) { 168 if (!getFhirVersion().contains(theFhirVersionId)) { 169 getFhirVersion().add(theFhirVersionId); 170 getFhirVersion().sort(PackageVersionComparator.INSTANCE); 171 } 172 return this; 173 } 174 } 175}