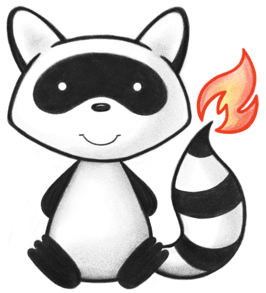
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.packages.loader; 021 022import org.hl7.fhir.utilities.npm.NpmPackage; 023 024import java.io.InputStream; 025 026public class NpmPackageData { 027 028 /** 029 * package id (npm id) 030 */ 031 private final String myPackageId; 032 033 /** 034 * package version id (npm version) 035 */ 036 private final String myPackageVersionId; 037 038 /** 039 * package description (url to find package, often) 040 */ 041 private final String mySourceDesc; 042 043 /** 044 * The raw bytes of the entire package 045 */ 046 private final byte[] myBytes; 047 048 /** 049 * The actual NpmPackage. 050 */ 051 private final NpmPackage myPackage; 052 053 /** 054 * The raw stream of the entire npm package contents 055 */ 056 private final InputStream myInputStream; 057 058 public NpmPackageData( 059 String thePackageId, 060 String thePackageVersionId, 061 String theSourceDesc, 062 byte[] theBytes, 063 NpmPackage thePackage, 064 InputStream theStream) { 065 myPackageId = thePackageId; 066 myPackageVersionId = thePackageVersionId; 067 mySourceDesc = theSourceDesc; 068 myBytes = theBytes; 069 myPackage = thePackage; 070 myInputStream = theStream; 071 } 072 073 public byte[] getBytes() { 074 return myBytes; 075 } 076 077 public NpmPackage getPackage() { 078 return myPackage; 079 } 080 081 public InputStream getInputStream() { 082 return myInputStream; 083 } 084 085 public String getPackageId() { 086 return myPackageId; 087 } 088 089 public String getPackageVersionId() { 090 return myPackageVersionId; 091 } 092 093 public String getSourceDesc() { 094 return mySourceDesc; 095 } 096}